More Readme
This commit is contained in:
parent
00ca5808ee
commit
fe2a5b1dba
278
readme.md
278
readme.md
|
@ -7,33 +7,40 @@ This really is a simple GUI, but also powerfully customizable.
|
|||
|
||||
I was frustrated by having to deal with the dos prompt when I had a powerful Windows machine right in front of me. Why is it SO difficult to do even the simplest of input/output to a window in Python??
|
||||
|
||||
Python itself doesn't have a simple solution... nor did the *many* GUI packages I tried. Most tried to do TOO MUCH, making it impossible for users to get started quickly. Others were just plain broken, requiring multiple files or other packages that were missing.
|
||||
With a simple GUI, it becomes practical to "associate" .py files with the python interpreter on Windows. Double click a py file and up pops a GUI window, a more pleasant experience than opening a dos Window and typing a command line.
|
||||
|
||||
Python itself doesn't have a simple GUI solution... nor did the *many* GUI packages I tried. Most tried to do TOO MUCH, making it impossible for users to get started quickly. Others were just plain broken, requiring multiple files or other packages that were missing.
|
||||
|
||||
The PySimpleGUI solution is focused on the ***developer***. How can the desired result be achieved in as little and as simple code as possible? This was the mantra used to create PySimpleGUI.
|
||||
|
||||
You can add a GUI to your command line with a single line of code. With 3 or 4 lines of code you can add a fully customized GUI.
|
||||
You can add a GUI to your command line with a single line of code. With 3 or 4 lines of code you can add a fully customized GUI. And for you Machine Learning folks out there, a **single line** progress meter call that you can drop into any loop.
|
||||
|
||||
The customization power comes from the form/dialog box builder that enables users to experience all of the normal GUI widgets without having to write a lot of code.
|
||||
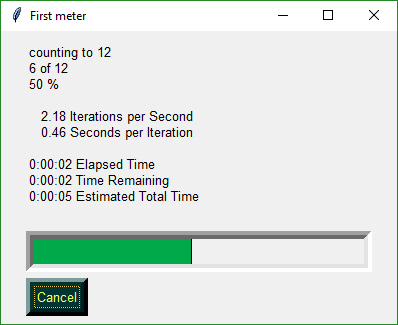
|
||||
|
||||
Features of PySimpleGUI include:
|
||||
Text
|
||||
Single Line Input
|
||||
Buttons including these types:
|
||||
File Browse
|
||||
Folder Browse
|
||||
Non-closing return
|
||||
Close form
|
||||
Checkboxes
|
||||
Radio Buttons
|
||||
Icons
|
||||
Multi-line Text Input
|
||||
Scroll-able Output
|
||||
Progress Bar
|
||||
Async/Non-Blocking Windows
|
||||
Tabbed forms
|
||||
Persistent Windows
|
||||
Redirect Python Output/Errors to scrolling Window
|
||||
'Higher level' APIs (e.g. MessageBox, YesNobox, ...)
|
||||
|
||||
The customization is via the form/dialog box builder that enables users to experience all of the normal GUI widgets without having to write a lot of code.
|
||||
|
||||
|
||||
> Features of PySimpleGUI include:
|
||||
> Text
|
||||
> Single Line Input
|
||||
> Buttons including these types: File Browse Folder Browse Non-closing return Close form
|
||||
> Checkboxes
|
||||
> Radio Buttons
|
||||
> Icons
|
||||
> Multi-line Text Input
|
||||
> Scroll-able Output
|
||||
> Progress Bar
|
||||
> Async/Non-Blocking Windows
|
||||
> Tabbed forms
|
||||
> Persistent Windows
|
||||
> Redirect Python Output/Errors to scrolling Window
|
||||
> 'Higher level' APIs (e.g. MessageBox, YesNobox, ...)
|
||||
|
||||
|
||||
An example of many widgets used on a single form. A little further down you'll find the FIFTEEN lines of code required to create this complex form.
|
||||
|
||||
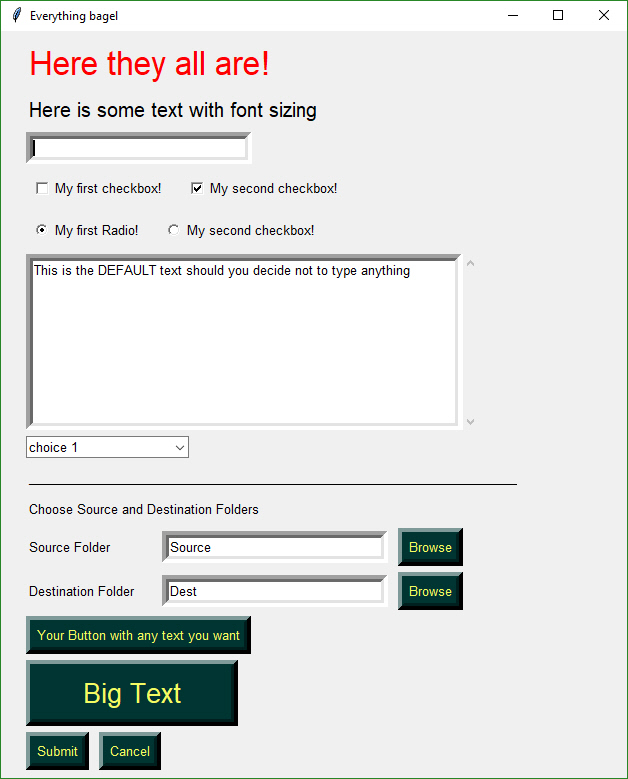
|
||||
|
||||
|
||||
## Getting Started with PySimpleGUI
|
||||
|
@ -132,11 +139,7 @@ The differences tend to be the number and types of buttons. Here are the calls
|
|||
import PySimpleGUI as SG
|
||||
|
||||
`SG.MsgBoxOK('This is an OK MsgBox')`
|
||||
<<<<<<< HEAD
|
||||
|
||||
=======
|
||||
|
||||
>>>>>>> 0b28fa3a917c83d2101bee375a0aadd816948156
|
||||
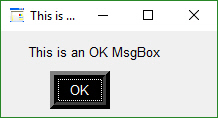
|
||||
|
||||
SG.MsgBoxOKCancel('This is an OK Cancel MsgBox')
|
||||
|
@ -160,11 +163,11 @@ The differences tend to be the number and types of buttons. Here are the calls
|
|||
|
||||
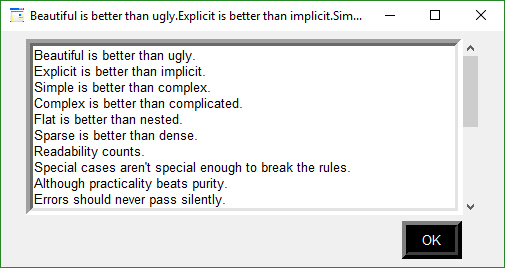
|
||||
|
||||
Take a moment to look at that last one. It's such a simple API call and yet the result is awesome. Rather than seeing text scrolling past on your display, you can capture that text and present it in a scrolled interface.
|
||||
Take a moment to look at that last one. It's such a simple API call and yet the result is awesome. Rather than seeing text scrolling past on your display, you can capture that text and present it in a scrolled interface. It's handy enough of an API call that it can also be called using the name `sprint` which is easier to remember than `ScrollectTextBox`.
|
||||
|
||||
#### High Level User Input
|
||||
|
||||
There are 3 very basic user input high-level function calls. It's expected that for most applications, a custom input form will be created.
|
||||
There are 3 very basic user input high-level function calls. It's expected that for most applications, a custom input form will be created. If you need only 1 value, then perhaps one of these high level functions will work.
|
||||
- GetTextBox
|
||||
- GetFileBox
|
||||
- GetFolderBox
|
||||
|
@ -181,14 +184,28 @@ There are 3 very basic user input high-level function calls. It's expected that
|
|||
|
||||
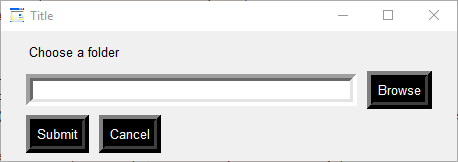
|
||||
|
||||
#### Progress Meter!
|
||||
We all have loops in our code. 'Isn't it joyful waiting, watching a counter scrolling past in a text window? How about one line of code to get a progress meter, that contains statistics about your code?
|
||||
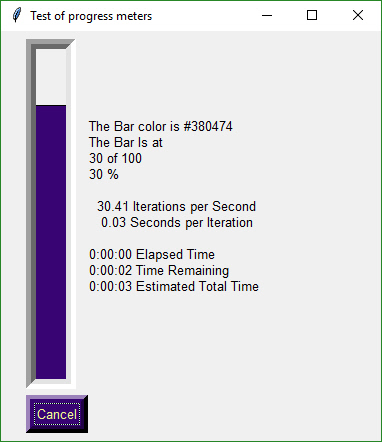
|
||||
|
||||
EasyProgressMeter(Title,
|
||||
CurrentValue,
|
||||
MaxValue,
|
||||
*args,
|
||||
Orientation=None,
|
||||
BarColor=DEFAULT_PROGRESS_BAR_COLOR,
|
||||
ButtonColor=None,
|
||||
Size=DEFAULT_PROGRESS_BAR_SIZE,
|
||||
Scale=(None, None),
|
||||
BorderWidth=DEFAULT_PROGRESS_BAR_BORDER_WIDTH):
|
||||
|
||||
# Custom Form API Calls
|
||||
|
||||
### Custom Form API Calls
|
||||
This is the FUN part of the programming of this GUI. In order to really get the most out of the API, you should be using an IDE that supports auto complete or will show you the definition of the function. This will make customizing go smoother.
|
||||
|
||||
It's both not enjoyable nor helpful to immediately jump into tweaking each and every little thing available to you. Let's start with a basic Browse for a file and do something with it.
|
||||
|
||||
# COPY THIS DESIGN PATTERN!
|
||||
## COPY THIS DESIGN PATTERN!
|
||||
|
||||
with SG.FlexForm('SHA-1 & 256 Hash', AutoSizeText=True) as form:
|
||||
form_rows = [[SG.Text('SHA-1 and SHA-256 Hashes for the file')],
|
||||
|
@ -201,7 +218,9 @@ This context manager contains all of the code needed to specify, show and retrie
|
|||
|
||||
It's important to use the "with" context manager. PySimpleGUI uses `tkinter`. `tkinter` is very picky about who releases objects and when. The `with` takes care of disposing of everything properly for you.
|
||||
|
||||
You will use this design pattern or code template for all of your "normal" (blocking) types of input forms.
|
||||
You will use this design pattern or code template for all of your "normal" (blocking) types of input forms. Copy it and modify it to suit your needs. This is the quickest way to get your code up and running with PySimpleGUI.
|
||||
|
||||
> Copy, Paste, Run.
|
||||
|
||||
PySimpleGUI's goal with the API is to be easy on the programmer. An attempt was made to make the program's code visually match the window on the screen. The way this is done is that a GUI is broken up into "Rows". Then each row is broke up into "Elements" or "Widgets". Each element is specified by names such as Text, Button, Checkbox, etc.
|
||||
|
||||
|
@ -220,7 +239,7 @@ Now we're on the second row of the form. On this row there are 2 elements. The
|
|||
|
||||
[SG.Submit(), SG.Cancel()]]
|
||||
|
||||
The last line of the `form_rows` variable assignment contains a Submit and a Cancel Button. These are buttons that will cause a form to return its valueso the caller.
|
||||
The last line of the `form_rows` variable assignment contains a Submit and a Cancel Button. These are buttons that will cause a form to return its value to the caller.
|
||||
|
||||
(button, (source_filename, )) = form.LayoutAndShow(form_rows)
|
||||
This is the code that **displays** the form, collects the information and returns the data collected. In this example we have a button return code and only 1 input field.
|
||||
|
@ -235,9 +254,14 @@ Don't forget all those ()'s of your values won't be coreectly assigned.
|
|||
|
||||
If you have a SINGLE value being returned, it is written this way:
|
||||
|
||||
(button, (value1,))
|
||||
(button, (value1,)) = form.LayoutAndShow(form_rows)
|
||||
Another way of parsing the return values is to store the list of values into a variable that is then referenced.
|
||||
|
||||
(button, (value)) = form.LayoutAndShow(form_rows)
|
||||
value1 = values[0]
|
||||
value2 = values[1]
|
||||
...
|
||||
|
||||
Forgetting the comma will mess you up but good
|
||||
|
||||
## All Widgets / Elements
|
||||
This code utilizes as many of the elements in one form as possible.
|
||||
|
@ -269,11 +293,183 @@ This is a somewhat complex form with quite a bit of custom sizing to make things
|
|||
|
||||
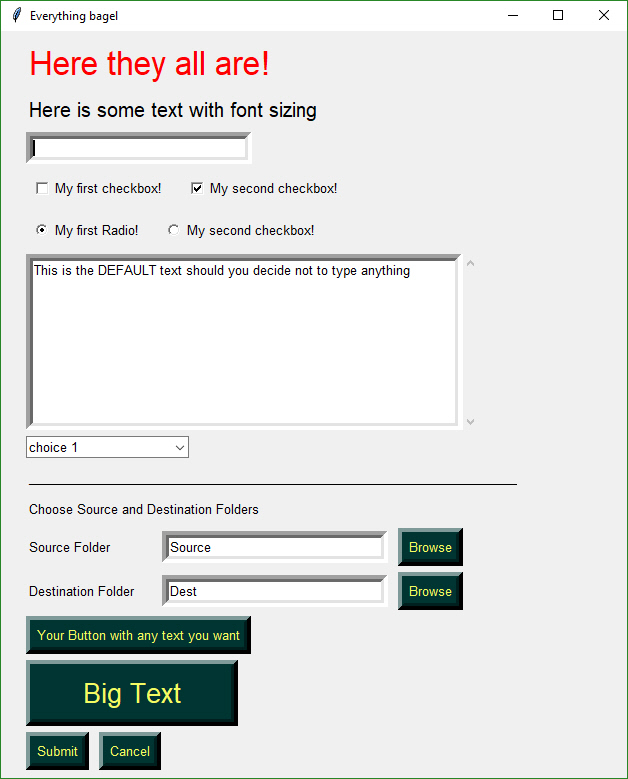
|
||||
|
||||
Clicking Submit caused the form call to return and the call to MsgBox is made to display the results.
|
||||
Clicking the Submit button caused the form call to return. The call to MsgBox resulted in this dialog box.
|
||||
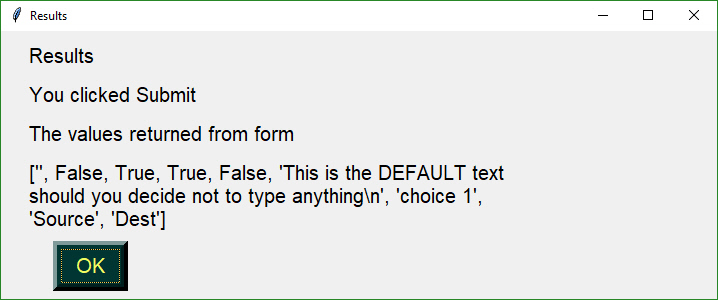
|
||||
|
||||
One important aspect of this example is the return codes:
|
||||
|
||||
## Built With
|
||||
(button, (values)) = form.LayoutAndShow(layout)
|
||||
The value for `button` will be the text that is displayed on the button element when it was created. If the user closed the form using something other than a button, then `button` will be `None`.
|
||||
|
||||
You can see in the MsgBox that the values returned are a list. Each input field in the form generates one item in the return values list. All input fields return a `string` except for Check Boxes and Radio Buttons. These return `bool`.
|
||||
|
||||
|
||||
|
||||
# Building Custom Forms
|
||||
You will find it much easier to write code using PySimpleGUI is you use features that show you documentation about the API call you are making. In PyCharm 2 commands are helpful.
|
||||
|
||||
> Control-Q (when cursor is on function name) brings up a box with the
|
||||
> function definition
|
||||
> Control-P (when cursor inside function call "()")
|
||||
> shows a list of parameters and their default values
|
||||
|
||||
## Synchronous Forms
|
||||
The most common use of PySimpleGUI is to display and collect information from the user. The most straightforward way to do this is using a "blocking" GUI call. Execution is "blocked" while waiting for the user to close the GUI form/dialog box.
|
||||
You've already seen a number of examples above that use blocking forms. Anytime you see a context manager used (see the `with` statement) it's most likely a blocking form. You can examine the show calls to be sure. If the form is a non-blocking form, it must indicate that in the call to `form.show`.
|
||||
|
||||
NON-BLOCKING form call:
|
||||
|
||||
form.Show(NonBlocking=True)
|
||||
|
||||
### Beginning a Form
|
||||
The first step is to create the form object using the desired form customization.
|
||||
|
||||
with FlexForm('Everything bagel', AutoSizeText=True, DefaultElementSize=(30,1)) as form:
|
||||
Let's go through the options available when creating a form.
|
||||
|
||||
def __init__(self, title,
|
||||
DefaultElementSize=(DEFAULT_ELEMENT_SIZE[0], DEFAULT_ELEMENT_SIZE[1]),
|
||||
AutoSizeText=DEFAULT_AUTOSIZE_TEXT,
|
||||
Scale=(None, None),
|
||||
Size=(None, None),
|
||||
Location=(None, None),
|
||||
ButtonColor=None,Font=None,
|
||||
ProgressBarColor=(None,None),
|
||||
IsTabbedForm=False,
|
||||
BorderDepth=None,
|
||||
AutoClose=False,
|
||||
AutoCloseDuration=DEFAULT_AUTOCLOSE_TIME,
|
||||
Icon=DEFAULT_WINDOW_ICON):
|
||||
|
||||
|
||||
#### Sizes
|
||||
Note several variables that deal with "size". Element sizes are measured in characters. A Text Element with a size of 20,1 has a size of 20 characters wide by 1 character tall.
|
||||
|
||||
The default Element size for PySimpleGUI is `(45,1)`.
|
||||
|
||||
Sizes can be set at the element level, or in this case, the size variables apply to all elements in the form. Setting `Size=(20,1)` in the form creation call will set all elements in the form to that size.
|
||||
|
||||
In addition to `size` there is a `scale` option. Scale will take the Element's size and scale it up or down depending on the scale value. `scale=(1,1)` doesn't change the Element's size. `scale=(2,1)` will set the Element's size to be twice as wide as the size setting.
|
||||
|
||||
|
||||
#### FlexForm - form-level variables overview
|
||||
A summary of the variables that can be changed when a FlexForm is created
|
||||
|
||||
> DefaultElementSize - set default size for all elements in the form
|
||||
> AutoSizeText - true/false autosizing turned on / off
|
||||
> Scale - set scale value for all elements
|
||||
> ButtonColor - default button color (foreground, background)
|
||||
> Font - font name and size for all text items
|
||||
> ProgressBarColor - progress bar colors
|
||||
> IsTabbedForm - true/false indicates form is a tabbed or normal form
|
||||
> BorderDepth - style setting for buttons, input fields
|
||||
> AutoClose - true/false indicates if form will automatically close
|
||||
> AutoCloseDuration - how long in seconds before closing form
|
||||
> Icon - filename for icon that's displayed on the window on taskbar
|
||||
|
||||
|
||||
## Elements
|
||||
"Elements" are the building blocks used to create forms. Some GUI APIs use the term Widget to describe these graphic elements.
|
||||
|
||||
> Text
|
||||
> Single Line Input
|
||||
> Buttons including these types: File Browse Folder Browse Non-closing return Close form
|
||||
> Checkboxes
|
||||
> Radio Buttons
|
||||
> Multi-line Text Input
|
||||
> Scroll-able Output
|
||||
> Progress Bar
|
||||
> Async/Non-Blocking Windows
|
||||
> Tabbed forms
|
||||
> Persistent Windows
|
||||
> Redirect Python Output/Errors to scrolling Window
|
||||
> 'Higher level' APIs (e.g. MessageBox, YesNobox, ...)
|
||||
|
||||
|
||||
### Output Elements
|
||||
Building a form is simply making lists of Elements. Each list is a row in the overall GUI dialog box. The definition looks something like this:
|
||||
|
||||
layout = [ [row 1 element, row 1 element],
|
||||
[row 2 element, row 2 element, row 2 element] ]
|
||||
The code is a crude representation of the GUI, laid out in text.
|
||||
#### Text Element
|
||||
|
||||
layout = [[SG.Text('This is what a Text Element looks like')]]
|
||||
|
||||
|
||||
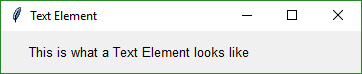
|
||||
|
||||
|
||||
The most basic element is the Text element. It simply displays text. Many of the 'options' that can be set for a Text element are shared by other elements. Size, Scale are a couple that you will see in every element.
|
||||
|
||||
Text(Text,
|
||||
Scale=(None, None),
|
||||
Size=(None, None),
|
||||
AutoSizeText=None,
|
||||
Font=None,
|
||||
TextColor=None)
|
||||
|
||||
Some commonly used elements have 'shorthand' versions of the functions to make the code more compact. The functions `T` and `Txt` are the same as calling `Text`.
|
||||
|
||||
**Fonts** in PySimpleGUI are always in this format:
|
||||
|
||||
(font_name, point_size)
|
||||
|
||||
The default font setting is
|
||||
|
||||
("Helvetica", 10)
|
||||
|
||||
**Colos** in PySimpleGUI are always in this format:
|
||||
|
||||
(foreground, background)
|
||||
|
||||
The values foreground and background can be the color names or the hex value formatted as a string:
|
||||
|
||||
"#RRGGBB"
|
||||
|
||||
#### Multiline Text Element
|
||||
|
||||
layout = [[SG.Multiline('This is what a Multi-line Text Element looks like', Size=(45,5))]]
|
||||
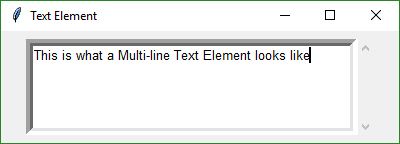
|
||||
This Element doubles as both an input and output Element. The `DefaultText` optional parameter is used to indicate what to output to the window.
|
||||
|
||||
Multiline(DefaultText='',
|
||||
EnterSubmits = False,
|
||||
Scale=(None, None),
|
||||
Size=(None, None),
|
||||
AutoSizeText=None)
|
||||
|
||||
> DefaultText - Text to display in the text box
|
||||
>EnterSubmits - Bool. If True, pressing Enter key submits form
|
||||
>Scale - Element's scale
|
||||
>Size - Element's size
|
||||
>AutoSizeText - Bool. Change width to match size of text
|
||||
|
||||
### Input Elements
|
||||
These make up the majority of the form definition. Optional variables at the Element level override the Form level values (e.g. `Size` is specified in the Element). All input Elements create an entry in the list of return values. A Text Input Element creates a string in the list of items returned.
|
||||
|
||||
#### Text Input Element
|
||||
|
||||
layout = [[SG.InputText('Default text')]]
|
||||
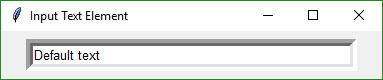
|
||||
|
||||
def InputText(DefaultText = '',
|
||||
Scale=(None, None),
|
||||
Size=(None, None),
|
||||
AutoSizeText=None)
|
||||
Shorthand functions that are equivalent to `InputText` are `Input` and `In`
|
||||
|
||||
#### Combo Element
|
||||
Also known as a drop-down list. Only required parameter is the list of choices. The return value is a string matching what's visible on the GUI.
|
||||
|
||||
layout = [[SG.InputCombo(['choice 1', 'choice 2'])]]
|
||||
|
||||
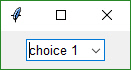
|
||||
|
||||
InputCombo(Values,
|
||||
Scale=(None, None),
|
||||
Size=(None, None),
|
||||
AutoSizeText=None)
|
||||
|
||||
|
||||
## Contributing
|
||||
|
@ -284,6 +480,12 @@ A MikeTheWatchGuy production... entirely responsible for this code
|
|||
|
||||
1.0.9 - July 10, 2018 - Initial Release
|
||||
|
||||
## Code Condition
|
||||
> Make it run
|
||||
> Make it right
|
||||
> Make it fast
|
||||
|
||||
It's a recipe for success if done right. PySimpleGUI has completed the "Make it run" phase. It's far from "right" in many ways. These are being worked on. The module is particularly poor on hiding implementation details, naming conventions, PEP 8. It was a learning exercise that turned into a somewhat complete GUI solution for lightweight problems.
|
||||
|
||||
## Authors
|
||||
|
||||
|
@ -296,3 +498,7 @@ This project is licensed under the MIT License - see the [LICENSE.md](LICENSE.md
|
|||
|
||||
* Jorj McKie was the motivator behind the entire project. His wxsimpleGUI concepts sparked PySimpleGUI into existence
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
|
Loading…
Reference in New Issue