Merge branch 'master' into Dev-latest
This commit is contained in:
commit
b431d8acb8
|
@ -0,0 +1,24 @@
|
||||||
|
import PySimpleGUI as sg
|
||||||
|
|
||||||
|
# Demo of how columns work
|
||||||
|
# Form has on row 1 a vertical slider followed by a COLUMN with 7 rows
|
||||||
|
# Prior to the Column element, this layout was not possible
|
||||||
|
# Columns layouts look identical to form layouts, they are a list of lists of elements.
|
||||||
|
|
||||||
|
# sg.ChangeLookAndFeel('BlueMono')
|
||||||
|
|
||||||
|
# Column layout
|
||||||
|
col = [[sg.Text('col Row 1', text_color='white', background_color='blue')],
|
||||||
|
[sg.Text('col Row 2', text_color='white', background_color='blue'), sg.Input('col input 1')],
|
||||||
|
[sg.Text('col Row 3', text_color='white', background_color='blue'), sg.Input('col input 2')]]
|
||||||
|
|
||||||
|
layout = [[sg.Listbox(values=('Listbox Item 1', 'Listbox Item 2', 'Listbox Item 3'), select_mode=sg.LISTBOX_SELECT_MODE_MULTIPLE, size=(20,3)), sg.Column(col, background_color='blue')],
|
||||||
|
[sg.Input('Last input')],
|
||||||
|
[sg.OK()]]
|
||||||
|
|
||||||
|
# Display the form and get values
|
||||||
|
# If you're willing to not use the "context manager" design pattern, then it's possible
|
||||||
|
# to collapse the form display and read down to a single line of code.
|
||||||
|
button, values = sg.FlexForm('Compact 1-line form with column').LayoutAndRead(layout)
|
||||||
|
|
||||||
|
sg.MsgBox(button, values, line_width=200)
|
|
@ -867,6 +867,7 @@ class FlexForm:
|
||||||
'''
|
'''
|
||||||
Display a user defined for and return the filled in data
|
Display a user defined for and return the filled in data
|
||||||
'''
|
'''
|
||||||
|
|
||||||
def __init__(self, title, default_element_size=(DEFAULT_ELEMENT_SIZE[0], DEFAULT_ELEMENT_SIZE[1]), auto_size_text=None, auto_size_buttons=None, scale=(None, None), location=(None, None), button_color=None, font=None, progress_bar_color=(None, None), background_color=None, is_tabbed_form=False, border_depth=None, auto_close=False, auto_close_duration=DEFAULT_AUTOCLOSE_TIME, icon=DEFAULT_WINDOW_ICON, return_keyboard_events=False, use_default_focus=True, text_justification=None):
|
def __init__(self, title, default_element_size=(DEFAULT_ELEMENT_SIZE[0], DEFAULT_ELEMENT_SIZE[1]), auto_size_text=None, auto_size_buttons=None, scale=(None, None), location=(None, None), button_color=None, font=None, progress_bar_color=(None, None), background_color=None, is_tabbed_form=False, border_depth=None, auto_close=False, auto_close_duration=DEFAULT_AUTOCLOSE_TIME, icon=DEFAULT_WINDOW_ICON, return_keyboard_events=False, use_default_focus=True, text_justification=None):
|
||||||
self.AutoSizeText = auto_size_text if auto_size_text is not None else DEFAULT_AUTOSIZE_TEXT
|
self.AutoSizeText = auto_size_text if auto_size_text is not None else DEFAULT_AUTOSIZE_TEXT
|
||||||
self.AutoSizeButtons = auto_size_buttons if auto_size_buttons is not None else DEFAULT_AUTOSIZE_BUTTONS
|
self.AutoSizeButtons = auto_size_buttons if auto_size_buttons is not None else DEFAULT_AUTOSIZE_BUTTONS
|
||||||
|
@ -1034,6 +1035,7 @@ class FlexForm:
|
||||||
return BuildResults(self, False, self)
|
return BuildResults(self, False, self)
|
||||||
|
|
||||||
def KeyboardCallback(self, event ):
|
def KeyboardCallback(self, event ):
|
||||||
|
|
||||||
self.LastButtonClicked = None
|
self.LastButtonClicked = None
|
||||||
self.FormRemainedOpen = True
|
self.FormRemainedOpen = True
|
||||||
if event.char != '':
|
if event.char != '':
|
||||||
|
@ -1053,6 +1055,7 @@ class FlexForm:
|
||||||
self.TKroot.quit()
|
self.TKroot.quit()
|
||||||
|
|
||||||
|
|
||||||
|
|
||||||
def _Close(self):
|
def _Close(self):
|
||||||
try:
|
try:
|
||||||
self.TKroot.update()
|
self.TKroot.update()
|
||||||
|
@ -1814,6 +1817,7 @@ def StartupTK(my_flex_form):
|
||||||
# root.bind('<Destroy>', MyFlexForm.DestroyedCallback())
|
# root.bind('<Destroy>', MyFlexForm.DestroyedCallback())
|
||||||
ConvertFlexToTK(my_flex_form)
|
ConvertFlexToTK(my_flex_form)
|
||||||
my_flex_form.SetIcon(my_flex_form.WindowIcon)
|
my_flex_form.SetIcon(my_flex_form.WindowIcon)
|
||||||
|
|
||||||
if my_flex_form.ReturnKeyboardEvents and not my_flex_form.NonBlocking:
|
if my_flex_form.ReturnKeyboardEvents and not my_flex_form.NonBlocking:
|
||||||
root.bind("<KeyRelease>", my_flex_form.KeyboardCallback)
|
root.bind("<KeyRelease>", my_flex_form.KeyboardCallback)
|
||||||
root.bind("<MouseWheel>", my_flex_form.MouseWheelCallback)
|
root.bind("<MouseWheel>", my_flex_form.MouseWheelCallback)
|
||||||
|
|
|
@ -535,4 +535,61 @@ Perhaps you don't want all the statistics that the EasyProgressMeter provides an
|
||||||
|
|
||||||
----
|
----
|
||||||
|
|
||||||
|
## The One-Line GUI
|
||||||
|
|
||||||
|
For those of you into super-compact code, a complete customized GUI can be specified, shown, and received the results using a single line of Python code. The way this is done is to combine the call to `FlexForm` and the call to `LayoutAndRead`. `FlexForm` returns a `FlexForm` object which has the `LayoutAndRead` method.
|
||||||
|
|
||||||
|
|
||||||
|
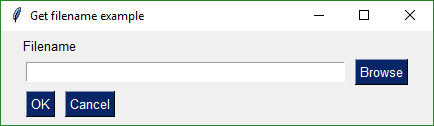
|
||||||
|
|
||||||
|
|
||||||
|
Instead of
|
||||||
|
|
||||||
|
import PySimpleGUI as sg
|
||||||
|
|
||||||
|
layout = [[sg.Text('Filename')],
|
||||||
|
[sg.Input(), sg.FileBrowse()],
|
||||||
|
[sg.OK(), sg.Cancel()] ]
|
||||||
|
|
||||||
|
button, (number,) = sg.FlexForm('Get filename example').LayoutAndRead(layout)
|
||||||
|
|
||||||
|
you can write this line of code for the exact same result (OK, two lines with the import):
|
||||||
|
|
||||||
|
import PySimpleGUI as sg
|
||||||
|
|
||||||
|
button, (filename,) = sg.FlexForm('Get filename example'). LayoutAndRead([[sg.Text('Filename')], [sg.Input(), sg.FileBrowse()], [sg.OK(), sg.Cancel()] ])
|
||||||
|
--------------------
|
||||||
|
## Multiple Columns
|
||||||
|
Starting in version 2.9 (not yet released but you can get from current GitHub) you can use the Column Element. A Column is required when you have a tall element to the left of smaller elements.
|
||||||
|
|
||||||
|
This example uses a Column. There is a Listbox on the left that is 3 rows high. To the right of it are 3 single rows of text and input. These 3 rows are in a Column Element.
|
||||||
|
|
||||||
|
To make it easier to see the Column in the window, the Column background has been shaded blue. The code is wordier than normal due to the blue shading. Each element in the column needs to have the color set to match blue background.
|
||||||
|
|
||||||
|
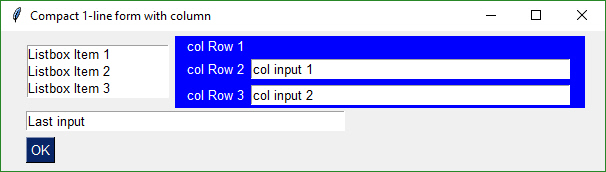
|
||||||
|
|
||||||
|
|
||||||
|
import PySimpleGUI as sg
|
||||||
|
|
||||||
|
# Demo of how columns work
|
||||||
|
# Form has on row 1 a vertical slider followed by a COLUMN with 7 rows
|
||||||
|
# Prior to the Column element, this layout was not possible
|
||||||
|
# Columns layouts look identical to form layouts, they are a list of lists of elements.
|
||||||
|
|
||||||
|
# sg.ChangeLookAndFeel('BlueMono')
|
||||||
|
|
||||||
|
# Column layout
|
||||||
|
col = [[sg.Text('col Row 1', text_color='white', background_color='blue')],
|
||||||
|
[sg.Text('col Row 2', text_color='white', background_color='blue'), sg.Input('col input 1')],
|
||||||
|
[sg.Text('col Row 3', text_color='white', background_color='blue'), sg.Input('col input 2')]]
|
||||||
|
|
||||||
|
layout = [[sg.Listbox(values=('Listbox Item 1', 'Listbox Item 2', 'Listbox Item 3'), select_mode=sg.LISTBOX_SELECT_MODE_MULTIPLE, size=(20,3)), sg.Column(col, background_color='blue')],
|
||||||
|
[sg.Input('Last input')],
|
||||||
|
[sg.OK()]]
|
||||||
|
|
||||||
|
# Display the form and get values
|
||||||
|
# If you're willing to not use the "context manager" design pattern, then it's possible
|
||||||
|
# to collapse the form display and read down to a single line of code.
|
||||||
|
button, values = sg.FlexForm('Compact 1-line form with column').LayoutAndRead(layout)
|
||||||
|
|
||||||
|
sg.MsgBox(button, values, line_width=200)
|
||||||
|
|
|
@ -59,13 +59,31 @@ Let's look at the first recipe from the book
|
||||||
print(button, values[0], values[1], values[2])
|
print(button, values[0], values[1], values[2])
|
||||||
|
|
||||||
|
|
||||||
It's a reasonable sized form.
|
It's a reasonably sized form.
|
||||||
|
|
||||||
|
|
||||||
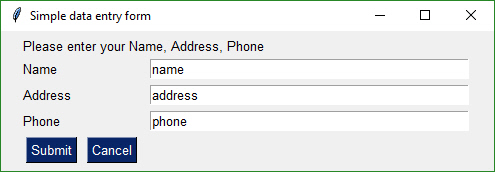
|
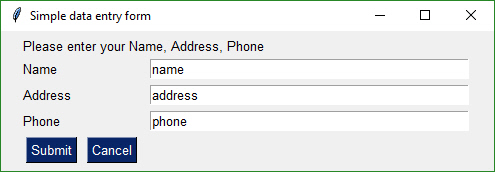
|
||||||
|
|
||||||
If you only need to collect a few values and they're all basically strings, then you would copy this recipe and modify it to suit your needs.
|
If you only need to collect a few values and they're all basically strings, then you would copy this recipe and modify it to suit your needs.
|
||||||
|
|
||||||
|
## The 5-line GUI
|
||||||
|
|
||||||
|
Not all GUIs take 5 minutes. Some take 5 lines of code. This is a GUI with a custom layout contained in 5 lines of code.
|
||||||
|
|
||||||
|
import PySimpleGUI as sg
|
||||||
|
|
||||||
|
form = sg.FlexForm('My first GUI')
|
||||||
|
|
||||||
|
layout = [ [sg.Text('Enter your name'), sg.InputText()],
|
||||||
|
[sg.OK()] ]
|
||||||
|
|
||||||
|
button, (name,) = form.LayoutAndRead(layout)
|
||||||
|
|
||||||
|
|
||||||
|
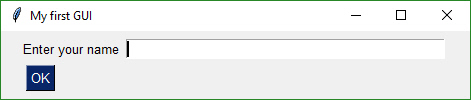
|
||||||
|
|
||||||
|
|
||||||
|
|
||||||
## Making Your Custom GUI
|
## Making Your Custom GUI
|
||||||
|
|
||||||
That 5-minute estimate wasn't the time it takes to copy and paste the code from the Cookbook. You should be able to modify the code within 5 minutes in order to get to your layout, assuming you've got a straightforward layout.
|
That 5-minute estimate wasn't the time it takes to copy and paste the code from the Cookbook. You should be able to modify the code within 5 minutes in order to get to your layout, assuming you've got a straightforward layout.
|
||||||
|
|
Loading…
Reference in New Issue