## PyPI Statistics & Versions
| TK | TK 2.7 | Qt| WxPython | Web (Remi) |
| -- | -- | -- | -- | -- |
|  |  |  |  |  |
| [](http://pepy.tech/project/pysimplegui) | [](https://pepy.tech/project/pysimplegui27) | [](https://pepy.tech/project/pysimpleguiqt) | [](https://pepy.tech/project/pysimpleguiWx) | [](https://pepy.tech/project/pysimpleguiWeb) |
|  |  |  |  |  |
| [](https://pypi.python.org/pypi/PySimpleGUI/) | [](https://pypi.python.org/pypi/PySimpleGUI27/) | [](https://pypi.python.org/pypi/PySimpleGUIQt/) | [](https://pypi.python.org/pypi/PySimpleGUIWx/) | [](https://pypi.python.org/pypi/PySimpleGUIWeb/) |
--------------------------
## GitHub Statistics
| Issues | Commit Activity | Stars | Docs |
| -- | -- | -- | -- |
|  |  |  |  |
|  |  | | |
--------------------------
# PySimpleGUI User's Manual
## Python GUI For Humans - Transforms tkinter, Qt, Remi, WxPython into portable people-friendly Pythonic interfaces
## The Call Reference Section Moved to here
### This manual is crammed full of answers so start your search for answers here. Read/Search this prior to opening an Issue on GitHub. Press Control F and type.
---
# Jump-Start
## Install
```
pip install pysimplegui
or
pip3 install pysimplegui
```
### This Code
```python
import PySimpleGUI as sg
sg.theme('DarkAmber') # Add a touch of color
# All the stuff inside your window.
layout = [ [sg.Text('Some text on Row 1')],
[sg.Text('Enter something on Row 2'), sg.InputText()],
[sg.Button('Ok'), sg.Button('Cancel')] ]
# Create the Window
window = sg.Window('Window Title', layout)
# Event Loop to process "events" and get the "values" of the inputs
while True:
event, values = window.read()
if event == sg.WIN_CLOSED or event == 'Cancel': # if user closes window or clicks cancel
break
print('You entered ', values[0])
window.close()
```
### Makes This Window
and returns the value input as well as the button clicked.
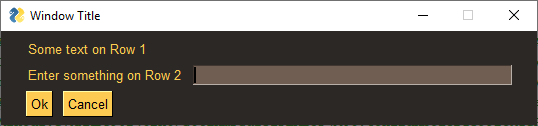
### Any Questions? It's that simple.
---
# 2021 Updates....
This documentation was originally written in 2018. So much has changed since, but the early stuff still runs just fine so there's not been a huge rush to update.
Some sections have been updated and added so it's not like this is a stale document.
## What's newer?
New APIs to save you time - not just for beginners
* Exec APIs (execution of subprocesses)
* User Settings APIs (management of settings in form of a dictionary)
* Threading API call - `write_event_value`
* True multiple windows support - `read_all_windows`
* System-wide global settings - theme, interpreter to use, Mac settings, ...
* Advanced error handling including launching your editor to line of code with error
* More advanced layouts - still trivial,maybe more trivial, to make windows
* Go wild - have a complicated (i.e. not simple) application... no problem here supporting your App. "It's on you". PSG solved the GUI problem, but you still have to make an application
* Docstrings - Tight integration with docstrings provides type checking and in-IDE documentation
* `sg.main` utilities - upgrade to the GitHub version in 1 click. Lots of good stuff built-into PySimpleGUI
* Demo Browser - Navigate the 315+ Demo Programs easily. Search, execute, edit all from one application
* Cookbook - There have been a number of updates in 2021
* This document has some sections new such as the User Settings APIs
### Dynamic Windows
- Making parts of windows expand/contract with the window.
- Swap out or collapse sections
- More parms in Elements to help with justification
- New `Push` & `VPush` Elements trivialize justification
### Threading
One simple call, `write_event_value`, solves threading issues.
Not ready for the threading module but need to run a thread? Use `window.perform_long_operation` to use threads. One call and done.
### What's Old?
The old parts of this documentation are the images. The good news is that things look better than you see here. Themes have made Windows colorful in 1 line of code.
------------------
#### Looking for a GUI package? Are you...
* looking to take your Python code from the world of command lines and into the convenience of a GUI?
* sitting on a Raspberry **Pi** with a touchscreen that's going to waste because you don't have the time to learn a GUI SDK?
* into Machine Learning and are sick of the command line?
* an IT guy/gal that has written some cool tools but due to corporate policies are unable to share unless it is an EXE file?
* wanting to share your program with your friends or families (that aren't so freakish that they have Python running)
* wanting to run a program in your system tray?
* a teacher wanting to teach your students how to program using a GUI?
* a student that wants to put a GUI onto your project that will blow away your teacher?
* looking for a GUI package that is "supported" and is being constantly developed to improve it?
* longing for documentation and scores of examples?
**Look no further, you've found your GUI package**.
#### The basics
* Create windows that look and operate _identically_ to those created directly with tkinter, Qt, WxPython, and Remi.
* Requires 1/2 to 1/10th the amount of code as underlying frameworks.
* One afternoon is all that is required to learn the PySimpleGUI package _and_ write your first custom GUI.
* Students can begin using within their first week of Python education.
* No callback functions. You do not need to write the word `class` _anywhere_ in your code.
* Access to nearly every underlying GUI Framework's Widgets.
* Supports both Python 2.7 & 3 when using tkinter.
* Supports both PySide2 and PyQt5 (limited support).
* Effortlessly move across tkinter, Qt, WxPython, and the Web (Remi) by changing only the import statement.
* The *only* way to write both desktop and web-based GUIs at the same time in Python.
* Developed from nothing as a pure Python implementation with Python friendly interfaces.
* Run your program in the System Tray using WxPython. Or, change the import and run it on Qt with no other changes.
* Works with Qt Designer.
* Built in Debugger.
* Actively maintained and enhanced - 4 ports are underway, all being used by users.
* Corporate as well as home users.
* Appealing to both newcomers to Python and experienced Pythonistas.
* The focus is entirely on the developer (you) and making their life easier, simplified, and in control.
* 170+ Demo Programs teach you how to integrate with many popular packages like OpenCV, Matplotlib, PyGame, etc.
* 200 pages of documentation, a Cookbook, and built-in help using docstrings. In short it's heavily documented.
## GUI Development does not have to be difficult nor painful. It can be (and is) FUN
#### What users are saying about PySimpleGUI
***(None of these comments were solicited & were not paid endorsements - other than a huge thank-you they received!)***
"I've been working to learn PyQT for the past week in my off time as an intro to GUI design and how to apply it to my existing scripts... Took me ~30 minutes to figure out PySimpleGUI and get my scripts working with a GUI."
"Python has been an absolute nightmare for me and I've avoided it like the plague. Until I saw PySimpleGUI."
"I've been pretty amazed at how much more intuitive it is than raw tk/qt. The dude developing it is super active on the project too, so if you come across situations that you just can't get the code to do what you want you can make bug/enhancement issues that are almost assured to get a meaningful response."
"This library is the easiest way of GUI programming in python! I'm totally in love with it"
"Wow that readme is extensive and great." (hear the love for docs often)
"Coming from R, Python is absolutely slick for GUIs. PySimpleGUI is a dream."
"I have been writing Python programs for about 4 or 5 months now. Up until this week, I never had luck with any UI libraries like Tkinter, Qt and Kivy. I went from not even being able to load a window in Tkinter reliably to making a loading screen, and full program in one night with PySimpleGUI."
"I love PySimpleGUI! I've been teaching it in my Python classes instead of Tkinter."
"I wish PySimpleGUI was available for every friggin programming language"
### START HERE - User Manual with Table of Contents
[ReadTheDocs](http://www.PySimpleGUI.org) <------ THE best place to read the docs due to TOC, all docs in 1 place, and better formatting. START here in your education. Easy to remember PySimpleGUI.org.
[The Call Reference](http://calls.PySimpleGUI.org) documentation is located on the same ReadTheDocs page as the main documentation, but it's on another tab that you'll find across the top of the page.
The quick way to remember the documentation addresses is to use these addresses:
http://docs.PySimpleGUI.org
http://calls.PySimpleGUI.org
#### Quick Links To Help and The Latest News and Releases
[Homepage - Lastest Readme and Code - GitHub](http://www.PySimpleGUI.com) Easy to remember: PySimpleGUI.com
[Announcements of Latest Developments, Release news, Misc](https://github.com/PySimpleGUI/PySimpleGUI/issues/142)
[COOKBOOK!](http://Cookbook.PySimpleGUI.org)
[Trinket an online Cookbook](http://Trinket.PySimpleGUI.org)
[Brief Tutorial](http://Tutorial.PySimpleGUI.org)
[Latest Demos and Master Branch on GitHub](https://github.com/PySimpleGUI/PySimpleGUI/tree/master/DemoPrograms)
[Repl.it Home for PySimpleGUI](https://repl.it/@PySimpleGUI)
[Lots of screenshots](https://www.bountysource.com/issues/60766522-screen-shots)
[How to submit an Issue](https://github.com/PySimpleGUI/PySimpleGUI/issues/1646)
[The YouTube videos](http://YouTube.PySimpleGUI.org) - If you like instructional videos, there are over 15 videos made by PySimpleGUI project over the first 18 months.
In 2020 a new series was begun. As of May 2020 there are 12 videos completed so far with many more to go....
- [PySimpleGUI 2020 - The most up to date information about PySimpleGUI](https://www.youtube.com/playlist?list=PLl8dD0doyrvFfzzniWS7FXrZefWWExJ2e)
- [5 part series of basics](https://www.youtube.com/playlist?list=PLl8dD0doyrvHMoJGTdMtgLuHymaqJVjzt)
- [10 part series of more detail](https://www.youtube.com/playlist?list=PLl8dD0doyrvGyXjORNvirTIZxKopJr8s0)
- [The Naked Truth (An update on the technology)](https://youtu.be/BFTxBmihsUY)
- There are numerous short videos also on that channel that demonstrate PySimpleGUI being used
YouTube Videos made by others. These have much higher production values than the above videos.
- A ***fantastic*** tutorial [PySimpleGUI Concepts - Video 1](https://youtu.be/cLcfLm_GgiM)
- Build a calculator [Python Calculator with GUI | PySimpleGUI | Texas Instruments DataMath II](https://youtu.be/x5LSTDdffFk)
- Notepad [Notepad in Python - PySimpleGUI](https://youtu.be/JQY641uynKo)
- File Search Engine [File Search Engine | Project for Python Portfolio with GUI | PySimpleGUI](https://youtu.be/IWDC9vcBIFQ)
# About The PySimpleGUI Documentation System
This User's Manual (also the project's readme) is one ***vital*** part of the PySimpleGUI programming environment. The best place to read it is at http://www.PySimpleGUI.org
If you are a professional or skilled in how to develop software, then you understand the role of documentation in the world of technology development. Use it, please.
***It WILL be required, at times, for you to read or search this document in order to be successful.***
Using Stack Overflow and other sites to post your questions has resulted in advice given by a lot of users that have never looked at the package and are sometimes just flat bad advice. When possible, post an Issue on this GitHub. Definitely go through the Issue checklist. Take a look through the docs again.
There are 5 resources that work together to provide you with the fastest path to success. They are:
1. This User's Manual
2. The Cookbook
3. The 170+ Demo Programs
4. Docstrings enable you to access help directly from Python or your IDE
5. Searching the GitHub Issues as a last resort (search both open and closed issues)
Pace yourself. The initial progress is exciting and FAST PACED. However, GUIs take time and thought to build. Take a deep breath and use the provided materials and you'll do fine. Don't skip the design phase of your GUI after you run some demos and get the hang of things. If you've tried other GUI frameworks before, successful or not, then you know you're already way ahead of the game using PySimpleGUI versus the underlying GUI frameworks. It may feel like the 3 days you've been working on your code has been forever, but by comparison of 3 days learning Qt, PySimpleGUI will look trivial to learn.
It is not by accident that this section, about documentation, is at the TOP of this document.
This documentation is not HUGE in length for a package this size. In fact it's still one document and it's the readme for the GitHub. It's not written in complex English. It is understandable by complete beginners. And pressing `Control+F` is all you need to do to search this document. USUALLY you'll find less than 6 matches.
## Documentation and Demos Get Out of Date
Sometimes the documentation doesn't match exactly the version of the code you're running. Sometimes demo programs haven't been updated to match a change made to the SDK. Things don't happen simultaneously generally speaking. So, it may very well be that you find an error or inconsistency or something no longer works with the latest version of an external library.
If you've found one of these problems, and you've searched to make sure it's not a simple mistake on your part, then by ALL means log an Issue on the GitHub. Don't be afraid to report problems if you've taken the simple steps of checking out the docs first.
# Platforms
## Hardware and OS Support
PySimpleGUI runs on Windows, Linux and Mac, just like tkinter, Qt, WxPython and Remi do. If you can get the underlying GUI Framework installed / running on your machine then PySimpleGUI will also run there.
### Hardware
* PC's, Desktop, Laptops
* Macs of all types
* Raspberry Pi
* Android devices like phones and tablets
* Virtual machine online (no hardware) - repl.it
### OS
* Windows 7, 8, 10
* Linux on PC - Tested on several distributions
* Linux on Raspberry Pi
* Linux on Android - Can use either Termux or PyDroid3
* Mac OS
#### Python versions
As of 9/25/2018 **both Python 3 and Python 2.7 are supported** when using **tkinter version** of PySimpleGUI! The Python 3 version is named `PySimpleGUI`. The Python 2.7 version is `PySimpleGUI27`. They are installed separately and the imports are different. See instructions in Installation section for more info. **None** of the other ports can use Python 2.
### Python 2.7 Code was be deleted from this GitHub on Dec 31, 2019
At the suggestion of the Python community at large, the experts, and security folks, the support for 2.7 was pulled from this Repo.
#### Warning - tkinter + Python 3.7.3 and later, including 3.8 has problems
The version of tkinter that is being supplied with the 3.7.3 and later versions of Python is known to have a problem with table colors. Basically, they don't work. As a result, if you want to use the plain PySimpleGUI running on tkinter, you should be using 3.7.2 or less. 3.6 is the version PySimpleGUI has chosen as the recommended version for most users.
## Output Devices
In addition to running as a desktop GUI, you can also run your GUI in a web browser by running PySimpleGUIWeb.
This is ideal for "headless" setups like a Raspberry Pi that is at the core of a robot or other design that does not have a normal display screen. For these devices, run a PySimpleGUIWeb program that never exits.
Then connect to your application by going to the Pi's IP address (and port #) using a browser and you'll be in communication with your application. You can use it to make configuration changes or even control a robot or other piece of hardware using buttons in your GUI.
## A Complete PySimpleGUI Program (Getting The Gist)
Before diving into details, here's a description of what PySimpleGUI is/does and why that is so powerful.
You keep hearing "custom window" in this document because that's what you're making and using... your own custom windows.
**ELEMENTS** is a word you'll see everywhere... in the code, documentation, ... Elements == PySimpleGUI's Widgets. As to not confuse a tkinter Button Widget with a PySimpleGUI Button Element, it was decided that PySimpleGUI's Widgets will be called Elements to avoid confusion.
Wouldn't it be nice if a GUI with 3 "rows" of Elements were defined in 3 lines of code? That's exactly how it's done. Each row of Elements is a list. Put all those lists together and you've got a window.
What about handling button clicks and stuff. That's 4 lines of the code below beginning with the while loop.
Now look at the `layout` variable and then look at the window graphic below. Defining a window is taking a design you can see visually and then visually creating it in code. One row of Elements = 1 line of code (can span more if your window is crowded). The window is exactly what we see in the code. A line of text, a line of text and an input area, and finally ok and cancel buttons.
This makes the coding process extremely quick and the amount of code very small
```python
import PySimpleGUI as sg
sg.theme('DarkAmber') # Add a little color to your windows
# All the stuff inside your window. This is the PSG magic code compactor...
layout = [ [sg.Text('Some text on Row 1')],
[sg.Text('Enter something on Row 2'), sg.InputText()],
[sg.OK(), sg.Cancel()]]
# Create the Window
window = sg.Window('Window Title', layout)
# Event Loop to process "events"
while True:
event, values = window.read()
if event in (sg.WIN_CLOSED, 'Cancel'):
break
window.close()
```
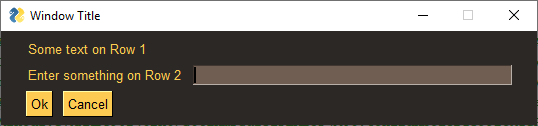
You got to admit that the code above is a lot more "fun" looking that tkinter code you've studied before. Adding stuff to your GUI is ***trivial***. You can clearly see the "mapping" of those 3 lines of code to specific Elements laid out in a Window. It's not a trick. It's how easy it is to code in PySimpleGUI. With this simple concept comes the ability to create any window layout you wish. There are parameters to move elements around inside the window should you need more control.
It's a thrill to complete your GUI project way ahead of what you estimated. Some people take that extra time to polish their GUI to make it even nicer, adding more bells and whistles because it's so easy and it's a lot of fun to see success after success as you write your program.
Some are more advanced users and push the boundaries out and extend PySimpleGUI using their own extensions.
Others, like IT people and hackers, are busily cranking out GUI program after GUI program, and creating tools that others can use. Finally, there's an easy way to throw a GUI onto your program and give it to someone. It's a pretty big leap in capability for some people. It's GREAT to hear these successes. It's motivating for everyone in the end. Your success can easily motivate the next person to give it a try and also potentially be successful.
Usually, there's a one to one mapping of a PySimpleGUI Element to a GUI Widget. A "Text Element" in PySimpleGUI == "Label Widget" in tkinter. What remains constant for you across all PySimpleGUI platforms is that no matter what the underlying GUI framework calls the thing that places text in your window, you'll always use the PySimpleGUI Text Element to access it.
### The final bit of magic is in how Elements are created and changed.
So far you've seen simple layouts with no customization of the Elements. Customizing and configuring Elements is another place PySimpleGUI utilizes the Python language to make your life easier.
What about Elements that have settings other than the standard system settings? What if I want my Text to be blue, with a Courier font on a green background. It's written quite simply:
```python
Text('This is some text', font='Courier 12', text_color='blue', background_color='green')
```
The Python named parameters are ***extensively*** in PySimpleGUI. They are key in making the code compact, readable, and trivial to write.
As you'll learn in later sections that discuss the parameters to the Elements, there are a LOT of options available to you should you choose to use them. The `Text Element` has 15 parameters that you can change. This is one reason why PyCharm is suggested as your IDE... it does a fantastic job of displaying documentation as you type in your code.
### That's *The* *Basics*
What do you think? Easier so far than your previous run-ins with GUIs in Python? Some programs, many in fact, are as simple as this example has been.
But PySimpleGUI certainly does **not** end here. This is the beginning. The scaffolding you'll build upon.
## The Underlying GUI Frameworks & Status of Each
At the moment there are 4 actively developed and maintained "ports" of PySimpleGUI. These include:
1. tkinter - Fully complete
2. Qt using Pyside2 - Alpha stage. Not all features for all Elements are done
3. WxPython - Development stage, pre-releaser. Not all Elements are done. Some known problems with multiple windows
4. Remi (Web browser support) - Development stage, pre-release.
While PySimpleGUI, the tkinter port, is the only 100% completed version of PySimpleGUI, the other 3 ports have a LOT of functionality in them and are in active use by a large portion of the installations. You can see the number of Pip installs at the very top of this document to get a comparison as to the size of the install base for each port. The "badges" are right after the logo.
## The Chain Link Fence
Maybe you've heard the "Walled Garden" term before. It's a boxing in effect.
While PySimpleGUI has a well-established parameter so you know where the edges are, there is no wall between you and the rest of the GUI framework. There's a chain link fence that's easy to reach through and get full access to the underlying frameworks.
The net result - it's easy to expand features that are not yet available in PySimpleGUI and easy to remove them too. Maybe the Listbox Element doesn't have a mode exposed that you want to enable. No problem, you can access the underlying Listbox Widget and make what is likely 1 or 2 calls and be done.
# The PySimpleGUI "Family"
## What's The Big Deal? What is it?
PySimpleGUI wraps tkinter, Qt, WxPython, and Remi so that you get all the same widgets, but you interact with them in a more friendly way that's common across the ports.
What does a wrapper do (Yo! PSG in the house!)? It does the layout, boilerplate code, creates and manages the GUI Widgets for you and presents you with a **simple, efficient interface.** Most importantly, it maps the Widgets in tkinter/Qt/Wx/Remi into PySimpleGUI Elements. Finally, it replaces the GUIs' event loop with one of our own.
You've seen examples of the code already. The big deal of all this is that anyone can create a GUI simply and quickly that matches GUIs written in the native GUI framework. You can create complex layouts with complex element interactions. And, that code you wrote to run on tkinter will also run on Qt by changing your import statement.
If you want a deeper explanation about the [architecture of PySimpleGUI](https://pysimplegui.readthedocs.io/en/latest/architecture/), you'll find it on ReadTheDocs in the same document as the Readme & Cookbook. There is a tab at the top with labels for each document.
## The "Ports"
There are distinct ports happening as mentioned above. Each has its own location on GitHub under the main project. Heac has its own Readme which is an *augmentation* of this document... they are meant to be used together.
PySimpleGUI is released on PyPI as 5 distinct packages.
1. PySimpleGUI - tkinter version
2. PySimpleGUI27 - tkinter version that runs on 2.7
3. PySimpleGUIWx - WxPython version
4. PySimpleGUIQt - PySided2 version
5. PySimpleGUIWeb - The web (Remi) version
You will need to install them separately
There is also an accompanying debugger known as `imwatchingyou`. If you are running the tkinter version of PySimpleGUI, you will not need to install the debugger as there is a version embedded directly into PySimpleGUI.
## Qt Version
Qt was the second port after tkinter. It is the 2nd most complete with the original PySimpleGUI (tkinter) being the most complete and is likely to continue to be the front-runner. All of the Elements are available on PySimpleGUIQt.
As mentioned previously each port has an area. For Qt, you can learn more on the [PySimpleGUIQt GitHub site](https://github.com/MikeTheWatchGuy/PySimpleGUI/tree/master/PySimpleGUIQt). **There is a separate Readme file for the Qt version** that you'll find there. This is true for all of the PySimpleGUI ports.
Give it a shot if you're looking for something a bit more "modern". PySimpleGUIQt is currently in **Alpha**. *All of the widgets are operational but some may not yet be full-featured.* If one is missing and your project needs it, log an Issue. It's how new features are born.
Here is a summary of the Qt Elements with no real effort spent on design clearly. It's an example of the "test harness" that is a part of each port. If you run the PySimpleGUI.py file itself then you'll see one of these tests.
As you can see, you've got a full array of GUI Elements to work with. All the standard ones are there in a single window. So don't be fooled into thinking PySimpleGUIQt is barely working or doesn't have many widgets to choose from. You even get TWO "Bonus Elements" - `Dial` and `Stretch`
## WxPython Version
[PySimpleGUIWx GitHub site](https://github.com/PySimpleGUI/PySimpleGUI/tree/master/PySimpleGUIWx). **There is a separate Readme file for the WxPython version**.
Started in late December 2018 PySimpleGUIWx started with the SystemTray Icon feature. This enabled the package to have one fully functioning feature that can be used along with tkinter to provide a complete program. The System Tray feature is complete and working very well. It was used not long ago in a corporate setting and has been performing with few problems reported.
The Windowing code was coming together with Reads operational. The elements were getting completed on a regular basis. But I ran into multiwindow problems. And it was at about this time that Remi was suggested as a port.
Remi (the "web port") overnight leapt the WxPython effort and Web became a #1 priority and continues to be. The thought is that the desktop was well represented with PySimpleGUI, PySimpleGUIQt, and PySimpleGUIWx. Between those ports is a solid windowing system and 2 system tray implementations and a nearly feature complete Qt effort. So, the team was switched over to PySimpleGUIWeb.
## Web Version (Remi)
[PySimpleGUIWeb GitHub site](https://github.com/PySimpleGUI/PySimpleGUI/tree/master/PySimpleGUIWeb). **There is a separate Readme file for the Web version**.
New for 2019, PySimpleGUIWeb. This is an exciting development! PySimpleGUI in your Web Browser!
The underlying framework supplying the web capability is the Python package Remi. https://github.com/dddomodossola/remi Remi provides the widgets as well as a web server for you to connect to. It's an exiting new platform to be running on and has temporarily bumped the WxPython port from the highest priority. PySimpleGUIWeb is the current high priority project.
**Use this solution for your Pi projects** that don't have anything connected in terms of input devices or display. Run your Pi in "headless" mode and then access it via the Web interface. This allows you to easily access and make changes to your Pi without having to hook up anything to it.
****It's not meant to "serve up web pages"****
PySimpleGUIWeb is first and foremost a **GUI**, a program's front-end. It is designed to have a single user connect and interact with the **GUI**.
If more than 1 person connects at a time, then both users will see the exact same stuff and will be interacting with the program as if a single user was using it.
## Android Version
PySimpleGUI runs on Android devices with the help of either the PyDroid3 app or the Termux app. Both are capable of running tkinter programs which means both are capable of running PySimpleGUI.
To use with PyDroid3 you will need to add this import to the top of all of your PySimpleGUI program files:
```python
import tkinter
```
This evidently triggers PyDroid3 that the application is going to need to use the GUI.
You will also want to create your windows with the `location` parameter set to `(0,0)`.
Here's a quick demo that uses OpenCV2 to display your webcam in a window that runs on PyDroid3:
```python
import tkinter
import cv2, PySimpleGUI as sg
USE_CAMERA = 0 # change to 1 for front facing camera
window, cap = sg.Window('Demo Application - OpenCV Integration', [[sg.Image(filename='', key='image')], ], location=(0, 0), grab_anywhere=True), cv2.VideoCapture(USE_CAMERA)
while window(timeout=20)[0] != sg.WIN_CLOSED:
window['image'](data=cv2.imencode('.png', cap.read()[1])[1].tobytes())
```
You will need to pip install opencv-python as well as PySimpleGUI to run this program.
Also, you must be using the Premium, yes paid, version of PyDroid3 in order to run OpenCV. The cost is CHEAP when compared to the rest of things in life. A movie ticket will cost you more. Which is more fun, seeing **your Python program** running on your phone and using your phone's camera, or some random movie currently playing? From experience, the Python choice is a winner. If you're cheap, well, then you won't get to use OpenCV. No, there is no secret commercial pact between the PySimpleGUI project and the PyDroid3 app team.
## Source code compatibility
In theory, your source code is completely portable from one platform to another by simply changing the import statement. That's the GOAL and surprisingly many times this 1-line change works. Seeing your code run on tkinter, then change the import to `import PySimpleGUIWeb as sg` and instead of a tkinter window, up pops your default browser with your window running on it is an incredible feeling.
But, ***caution is advised.*** As you've read already, some ports are further along than others. That means when you move from one port to another, some features may not work. There also may be some alignment tweaks if you have an application that precisely aligns Elements.
What does this mean, assuming it works? It means it takes a trivial amount of effort to move across GUI Frameworks. Don't like the way your GUI looks on tkinter? No problem, change over to try PySimpleGUIQt. Made a nice desktop app but want to bring it to the web too? Again, no problem, use PySimpleGUIWeb.
## repl.it Version
***Want to really get your mind blown?*** Check out this [PySimpleGUI program](https://repl.it/@PySimpleGUI/PySimpleGUIWeb-Demos) running in your web browser.
Thanks to the magic of repl.it and Remi it's possible to run PySimpleGUI code in a browser window without having Python running on your computer. This should be viewed as a teaching and demonstration aid. It is not meant to be a way of serving up web pages. It wouldn't work any way as each user forks and gets their own, completely different, workspace.
There are 2 ports of PySimpleGUI that run on repl.it - PySimpleGUI and PySimpleGUIWeb.
### PySimpleGUI (tkinter based)
The primary PySimpleGUI port works very well on repl.it due to the fact they've done an outstanding job getting tkinter to run on these virtual machines. Creating a program from scratch, you will want to choose the "Python with tkinter" project type.
The virtual screen size for the rendered windows isn't very large, so be mindful of your window's size or else you may end up with buttons you can't get to.
You may have to "install" the PySimpleGUI package for your project. If it doesn't automatically install it for you, then click on the cube along the left edge of the browser window and then type in PySimpleGUI or PySimpleGUIWeb depending on which you're using.
### PySimpleGUIWeb (Remi based)
For PySimpleGUIWeb programs you run using repl.it will automatically download and install the latest PySimpleGUIWeb from PyPI onto a virtual Python environment. All that is required is to type `import PySimpleGUIWeb` you'll have a Python environment up and running with the latest PyPI release of PySimpleGUIWeb.
### Creating a repl.it project from scratch / troubleshooting
To create your own repl.it PySimpleGUI project from scratch, first choose the type of Python virtual machine you want. For PySimpleGUI programs, choose the "Python with tkinter" project type. For PySimpleGUIWeb, choose the normal Python project.
There have been times where repl.it didn't do the auto import thing. If that doesn't work for some reason, you can install packages by clicking on the package button on the left side of the interface, typing in the package name (PySimpleGUI or PySimpleGUIWeb) and install it.
### Why this is so cool (listen up Teachers, tutorial writers)
***Educators*** in particular should be interested. Students can not only post their homework easily for their teacher to access, but teachers can also run the students programs online. No downloading needed. Run it and check the results.
For people wanting to share their code, especially when helping someone with a problem, it's a great place to do it. Those wishing to see your work do not have to be running Python nor have PySimpleGUI installed.
The way I use it is to first write my PySimpleGUI code on Windows, then copy and paste it into Repl.it.
Finally, you can embed these Repl.it windows into web pages, forum posts, etc. The "Share" button is capable of giving you the block of code for an "iframe" that will render into a working repl.it program in your page. It's amazing to see, but it can be slow to load.
### Repl.it is NOT a web server for you to "deploy" applications!
Repl.it is not meant to serve up applications and web pages. Trying to use it that way will not result in satisfactory results. It's simply too slow and too technical of an interface for trying to "deploy" using it. PySimpleGUIWeb isn't a great choice in serving web pages. It's purpose is more to build a GUI that runs in a browser.
## Macs
It's surprising that Python GUI code is completely cross platform from Windows to Mac to Linux. No source code changes. This is true for both PySimpleGUI and PySimpleGUIQt.
Historically, PySimpleGUI using tkinter have struggled on Macs. This was because of a problem setting button colors on the Mac. However, two events has turned this problem around entirely.
1. Use of ttk Buttons for Macs
2. Ability for Mac users to install Python from python.org rather than the Homebrew version with button problems
It's been a long road for Mac users with many deciding to use PySimpleGUIQt so that multi-colored windows could be made. It's completely understandable to want to make attractive windows that utilize colors.
PySimpleGUI now supports Macs, Linux, and Windows equally well. They all are able to use the "Themes" that automatically add color to your windows.
Be aware that Macs default to using ttk buttons. You can override this setting at the Window and Button levels. If you installed Python from python.org, then it's likely you can use the non-ttk buttons should you wish.
# Support
## Don't Suffer Silently
The GitHub Issues are checked *often*. Very often. **Please** post your questions and problems there and there only. Please don't post on Reddit, StackOverflow, on forums, until you've tried posting on the GitHub.
Why? *It will get you the best support possible.* Second, you'll be helping the project as what you're experiencing might very well be a bug, or even a *known* bug. Why spend hours thrashing, fighting against a known bug?
It's not a super-buggy package, but users do experience problems just the same. Maybe something's not explained well enough in the docs. Maybe you're making a common mistake. Maybe that feature isn't complete yet.
You won't look stupid posting an Issue on GitHub. It's just the opposite.
### How to log issues
**PySimpleGUI is an active project.** Bugs are fixed, features are added, often. Should you run into trouble, **open an issue** on the [GitHub site](http://www.PySimpleGUI.com) and you'll receive help. Posting questions on StackOverflow, Forums, Mailing lists, Reddit, etc, is not the fastest path to support and taking it may very well lead you astray as folks not familiar with the package struggle to help you. You may also run into the common response of "I don't know PySimpleGUI (and perhaps dislike it as a result), but I know you can do that with Qt".
Why only 1 location? It's simple.... it's where the bugs, enhancements, etc are tracked. It's THE spot on the Internet for this project. There's not driven by a freakish being in control, telling people how to do things, reasoning. It's so that YOU get the best and quickest support possible.
So, [open an Issue](https://github.com/PySimpleGUI/PySimpleGUI/issues/new/choose), choose "custom form" and fill it out completely. There are very good reasons behind all of the questions. Cutting corners only cuts your chances of getting help and getting quality help as it's difficult enough to debug remotely. Don't handicap people that want to help by not providing enough information.
**Be sure and run your program outside of your IDE** ***first***. Start your program from the shell using `python` or `python3` command. On numerous occasions much time was spent chasing problems caused by the IDE. By running from a command line, you take that whole question out of the problem, an important step.
***Don't sit and stew, trying the same thing over and over***, until you hate life... stop, and post an Issue on the GitHub. Someone **WILL** answer you. Support is included in the purchase price for this package (the quality level matches the price as well I'm afraid). Just don't be too upset when your free support turns out to be a little bit crappy, but it's free and typically good advice.
### Target Audience
PySimpleGUI is trying to serve the 80% of GUI *problems*. The other 20% go straight to tkinter, Qt, WxPython, Remi, or whatever fills that need. That 80% is **a huge problem space**.
***The "Simple" of PySimpleGUI describes how easy it is to use, not the nature of the problem space it solves.*** Note that people are not part of that description. It's not trying to solve GUI problems for 80% of the people trying it. PySimpleGUI tries to solve 80% of GUI ***problems***, regardless of the programmer's experience level.
Is file I/O in Python limited to only certain people? Is starting a thread, building a multi-threaded Python program incredibly difficult such that it takes a year to learn? No. It's quite easy. Like most things Python, you import the object from package and you use it. It is 2 lines of Python code to create and start a thread.
Why can't it be 2 lines of code to show a GUI window? What's SO special about the Python GUI libraries that they require you to follow a specific Object Oriented model of development? Other parts and packages of Python don't tend to do that.
The reason is because they didn't originate in Python. They are strangers in a strange land and they had to be "adapted". They started as C++ programs / SDKs, and remain that way too. There's a veneer of Python slapped onto the top of them, but that sure didn't make them fit the language as well as they could have.
PySimpleGUI is designed with both the beginner and the experienced developer in mind. Why? Because both tend to like compact code. Most like people, we just want to get sh\*t done, right? And, why not do it in a way that's like how most of Python works?
The beginners can begin working with GUIs ***in their first week of Python education***. The professionals can jump right into the deep end of the pool to use the entire array of Elements and their capabilities to build stuff like a database application.
Here's a good example of how PySimpleGUI serves these 2 groups.... the `InputText` Element has 16 potential parameters, yet you'll find 0 or 1 parameters set by beginners. Look at the examples throughout this document and you'll see the code fragments utilize a tiny fraction of the potential parameters / settings. Simple... **keep it simple for the default case**. This is part of the PySimpleGUI mission.
Some developers are heavily wedded to the existing GUI Framework Architectures (Qt, WxPython, tkinter). They like the existing GUI architectures (they're all roughly the same, except this one). If you're in that crowd, join the "20% Club" just down the street. There's plenty of room there with plenty of possible solutions.
But how about a quick stop-in for some open mindedness exercises. Maybe you will come up with an interesting suggestion even if you don't use it. Or maybe PySimpleGUI does something that inspires you to write something similar directly in Qt. And please, at least be civil about it. There is room for multiple architectures. Remember, you will not be *harmed* by writing some PySimpleGUI code just like you won't by writing some tkinter or Qt code. Your chances of feeling harmed is more likely from one of those 2.
#### Beginners & Easier Programs
There are a couple of reasons beginners stop in for a look. The first is to simply throw a simple GUI onto the front of an existing command line application. Or maybe you need to popup a box to get a filename. These can often be simple 1-line `popup` calls. Of course, you don't have to be a beginner to add a GUI onto one of your existing command line programs. Don't feel like because you're an advanced programmer, you need to have an advanced solution.
If you have a more intricate, complete, perhaps multi-window design in mind, then PySimpleGUI still could be your best choice.
This package is not only great to use as your first GUI package, but it also teaches how to design and utilize a GUI. It does it better than the existing GUIs by removing the syntax, and lengthy code that can take an otherwise very simple appearing program into something that's completely unrecognizable. With PySimpleGUI your 'layout' is all you need to examine to see the different GUI Elements that are being used.
Why does PySimpleGUI make it any easier to learn about GUIs? Because it removes the classes, callback functions, object oriented design to better get out of your way and let you focus entirely on your GUI and not how to represent it in code.
The result is 1/2 to 1/10th the amount of code that implements the exact same layout and widgets as you would get from coding yourself directly in Qt5. It's been tested many times... again and again, PySimpleGUI produces significantly less code than Qt and the frameworks it runs on.
Forget syntax completely and just look on the overall activities of a PySimpleGUI programmer. You have to design your window.... determine your inputs and your outputs, place buttons in strategic places, create menus, .... You'll be busy just doing all those things to design and define your GUI completely independent upon the underlying framework.
After you get all those design things done and are ready to build your GUI, it's then that you face the task of learning a GUI SDK. Why not start with the easy one that gives you many successes? You're JUST getting ***started***, so cut yourself a break and use PySimpleGUI so that you can quickly get the job done and move on to the next GUI challenge.
#### Advanced Programmers, Sharp Old-Timers, Code Slingers and Code Jockeys
It's not perfect, but PySimpleGUI is an amazing bit of technology. It's the programmer, the computer scientist, that has experience working with GUIs in the past that will recognize the power of this simple architecture.
What I hear from seasoned professionals is that PySimpleGUI saves them a **ton** of time. They've written GUI code before. They know how to lay out a window. These folks just want to get their window working and quick.
With the help of IDE's like PyCharm, Visual Studio and Wing (the officially supported IDE list) you get instant documentation on the calls you are making. On PyCharm you instantly see both the call signature but also the explanations about each parameter.
If the screenshots, demo programs and documentation don't convince you to at least **give it a try, once**, then you're way too busy, or ..... I dunno, I stopped guessing "why?" some time ago.
Some of the most reluctant of people to try PySimpleGUI have turned out to be some of the biggest supporters.
#### A Moment of Thanks To The PySimpleGUI Users
I want to thank the early users of PySimpleGUI that started in 2018. Your suggestions helped shape the package and have kept it moving forward at a fast pace.
For all the users, while I can't tell you the count of the number of times someone has said "thank you for PySimpleGUI" as part of logging and Issue, or a private message or email, but I can tell you that it's been significant.
***EVERY one of those "thank you" phrases, no matter how small you may think it is, helps tremendously.***
Sometimes it's what gets me past a problem or gets me to write yet more documentation to try and help people understand quicker and better. Let's just say the effect is always positive and often significant.
PySimpleGUI users have been super-nice. I doubt all Open Source Projects are this way, but I could be wrong and every GitHub repository has awesome users. If so, that's even more awesome!
**THANK YOU PySimpleGUI USERS!**
-------------------
# Learning Resources
***This document.... you must be willing to read this document if you expect to learn and use PySimpleGUI.***
If you're unwilling to even try to figure out how to do something or find a solution to a problem and have determined it's "easier to post a question first than to look at the docs", then this is not the GUI package for you. *If you're unwilling to help yourself, then don't expect someone else to try first.* You need to hold up your end of the bargain by at least doing some searches of this document.
While PySimpleGUI enables you to write code easily, it doesn't mean that it magically fills your head with knowledge on how to use it. The built-in docstrings help, but they can only go so far.
***Searching this document is as easy as pressing Control + F.***
This document is on the GitHub homepage, as the readme. http://www.PySimpleGUI.com will get you there. If you prefer a version with a Table of Contents on the left edge then you want to go to http://www.PySimpleGUI.org .
## The PySimpleGUI, Developer-Centric Model
You may think that you're being fed a line about all these claims that PySimpleGUI is built specifically to make your life easier and a lot more fun than the alternatives.... especially after reading the bit above about reading this manual.
### Psychological Warfare
Brainwashed. Know that there is an active campaign to get you to be successful using PySimpleGUI. The "Hook" to draw you in and keep you working on your program until you're satisfied is to work on the dopamine in your brain. Yes, your a PySimpleGUI rat, pressing on that bar that drops a food pellet reward in the form of a working program.
The way this works is to give you success after success, with very short intervals between. For this to work, what you're doing must work. The code you run must work. Make small changes to your program and run it over and over and over instead of trying to do one big massive set of changes. Turn one knob at a time and you'll be fine.
Find the keyboard shortcut for your IDE to run the currently shown program so that running the code requires 1 keystroke. On PyCharm, the key to run what you see is Control + Shift + F10. That's a lot to hold down at once. I programmed a hotkey on my keyboard so that it emits that combination of keys when I press it. Result is a single button to run.
### Tools
These tools were created to help you achieve a steady stream of these little successes.
* This readme and its example pieces of code
* The Cookbook & eCoobook - Copy, paste, run, success
* Demo Programs - Copy these small programs to give yourself an instant head-start
* Documentation shown in your IDE (docstrings) means you do not need to open any document to get the full assortment of options available to you for each Element & function call
The initial "get up and running" portion of PySimpleGUI should take you less than 5 minutes (the 5 minute challenge). ***The goal is 5 minutes from your decision "I'll give it a try" to having your first window up on the screen***. "Oh wow, it was that easy?!"
The primary learning paths for PySimpleGUI are:
* The Documentation
* Resist the urge to "Google It"
* The old-school way, "Read the Documenation", for this project, will be the most efficient path.
* PySimpleGUI Documentation - [http://www.PySimpleGUI.org](http://www.PySimpleGUI.org)
* Here you will find the main doc, the Cookbook, the Call Reference, the Readme... all in 1 location.
* This readme document on GitHub and in the main documentation
* PySimpleGUI GitHub: [http://www.PySimpleGUI.com](http://www.PySimpleGUI.com)
* The Cookbook - Recipes to get you going and quick
* [http://Cookbook.PySimpleGUI.org](http://Cookbook.PySimpleGUI.org)
* The online Interactive eCookbook is also available - [http://eCookbook.PySimpleGUI.org](http://eCookbook.PySimpleGUI.org)
* The Demo Programs - Start hacking on one of these running solutions
* [http://Demos.PySimpleGUI.org](http://Demos.PySimpleGUI.org)
* The easiest way to obtain and use them is by pip installing `psgdemos`
* The Udemy Course! "The Official PySimpleGUI Course"
* The is ONE and only 1 video course on the Internet that helps the PySimpleGUI financially.
* [http://udemy.pysimplegui.org/](http://udemy.pysimplegui.org/)
* There are 61 lessons that will teach you all aspects of PySimpleGUI
* It was a year in the making and covers features up through end of 2021
* It's the best course I've ever written and recorded (says Mike). Each lesson is compact, consise, focused, easy to understand
* The YouTube videos - If you like instructional videos, there are 15+ videos.
* **Caution** is advised... these videos are from 2020. **Much** has changed since they were made. They are still quite valid as what you're taught will work. But, you're missing 2 years of intense development that are not represented in these lessons. The Udemy course is a more complete and current course.
* 2020 to 2022 update - [https://youtu.be/lRuvKfilJnA](https://youtu.be/lRuvKfilJnA)-Lists a few of the many changes to PySimpleGUI since the 2020 lessons were recorded.
* [5 part series of basics](https://www.youtube.com/playlist?list=PLl8dD0doyrvHMoJGTdMtgLuHymaqJVjzt)
* [10 part series of more detail](https://www.youtube.com/playlist?list=PLl8dD0doyrvGyXjORNvirTIZxKopJr8s0)
Everything is geared towards giving you a "quick start" whether that be a Recipe or a Demo Program. The idea is to give you something running and let you hack away at it. As a developer this saves tremendous amounts of time.
You **start** with a working program, a GUI on the screen. Then have at it. If you break something (`"a happy little accident"` as Bob Ross put it), then you can always backtrack a little to a known working point.
A high percentage of users report both learning PySimpleGUI and completing their project in a single day.
This isn't a rare event and it's not bragging. GUI programming doesn't HAVE to be difficult by definition and PySimpleGUI has certainly made it much much more approachable and easier (not to mention simpler).
But there will be times that you need to read documentation, look at examples, when pushing into new, unknown territory. Don't guess... or more specifically, don't guess and then give up when it doesn't work.
## This Documentation, Call Reference and Cookbook
The quickest way to the docs is to visit:
http://www.PySimpleGUI.org
You will be auto-forwarded to the right destination. There are multiple tabs on ReadTheDocs.
The call reference is an important document as it explains every call and every parameter. Note that this same information is available to you via docstrings so that as you are writing your code, you can read the documentation without ever leaving your IDE (e.g. PyCharm)
## Demo Programs
The GitHub repo has the Demo Programs. There are ones built for plain PySimpleGUI that are usually portable to other versions of PySimpleGUI. And there are some that are associated with one of the other ports.
As of the start of 2022 **there are over 300 Demo Programs**.
These programs demonstrate to you how to use the Elements and how to integrate PySimpleGUI with some of the popular open source technologies such as OpenCV, PyGame, PyPlot, and Matplotlib to name a few.
Many Demo Programs that are in the main folder will run on multiple ports of PySimpleGUI. There are also port-specific Demo Programs. You'll find those in the folder with the port. So, Qt specific Demo Programs are in the PySimpleGUIQt folder.
## Jump Start! Get the Demo Programs & Demo Browser Quickly!
The over 300 Demo Programs will give you a jump-start and provide many design patterns for you to learn how to use PySimpleGUI and how to integrate PySimpleGUI with other packages. By far the best way to experience these demos is using the Demo Browser. This tool enables you to search, edit and run the Demo Programs.
### The `psgdemos` PyPI Package
In Jan 2022 a new package was added so that you can get both the Demo Programs and the Demo Browser by entering 1 install command.
To get them installed quickly along with the Demo Browser, use `pip` to install `psgdemos`:
`python -m pip install psgdemos`
or if you're in Linux, Mac, etc, that uses `python3` instead of `python` to launch Python:
`python3 -m pip install psgdemos`
Once installed, launch the demo browser by typing `psgdemos` from the command line"
`psgdemos`
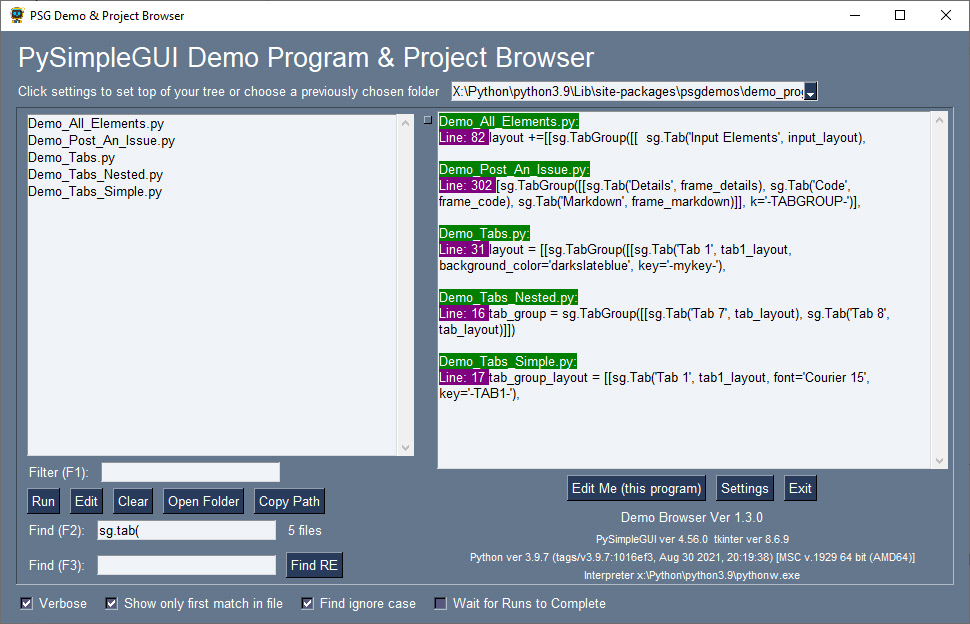
---
# The Quick Tour
Let's take a super-brief tour around PySimpleGUI before digging into the details. There are 2 levels of windowing support in PySimpleGUI - High Level and Customized.
The high-level calls are those that perform a lot of work for you. These are not custom made windows (those are the other way of interacting with PySimpleGUI).
Let's use one of these high level calls, the `popup` and use it to create our first window, the obligatory "Hello World". It's a single line of code. You can use these calls like print statements, adding as many parameters and types as you desire.
```python
import PySimpleGUI as sg
sg.popup('Hello From PySimpleGUI!', 'This is the shortest GUI program ever!')
```

Or how about a ***custom GUI*** in 1 line of code? No kidding this is a valid program and it uses Elements and produce the same Widgets like you normally would in a tkinter program. It's just been compacted together is all, strictly for demonstration purposes as there's no need to go that extreme in compactness, unless you have a reason to and then you can be thankful it's possible to do.
```python
import PySimpleGUI as sg
event, values = sg.Window('Get filename example', [[sg.Text('Filename')], [sg.Input(), sg.FileBrowse()], [sg.OK(), sg.Cancel()] ]).read(close=True)
```

## The Beauty of Simplicity
> One day I will find the right words, and they will be simple.
― Jack Kerouac
That's nice that you can crunch things into 1 line, like in the above example, but it's not readable. Let's add some whitespace so you can see the **beauty** of the PySimpleGUI code. And while we're at it, we'll change the color theme to something darker that's perhaps more attractive to some of you.
Take a moment and look at the code below. Can you "see" the window looking at the `layout` variable, knowing that each line of code represents a single row of Elements? There are 3 "rows" of Elements shown in the window and there are 3 lines of code that define it.
Creating and reading the user's inputs for the window occupy the last 2 lines of code, one to create the window, the last line shows the window to the user and gets the input values (what button they clicked, what was input in the Input Element)
```python
import PySimpleGUI as sg
sg.theme('Dark Grey 13')
layout = [[sg.Text('Filename')],
[sg.Input(), sg.FileBrowse()],
[sg.OK(), sg.Cancel()]]
window = sg.Window('Get filename example', layout)
event, values = window.read()
window.close()
```

Unlike other GUI SDKs, you can likely understand every line of code you just read, even though you have not yet read a single instructional line from this document about how you write Elements in a layout.
There are no pesky classes you are *required* to write, no callback functions to worry about. None of that is required to show a window with some text, an input area and 2 buttons using PySimpleGUI.
The same code, in tkinter, is 5 times longer and I'm guessing you won't be able to just read it and understand it. While you were reading through the code, did you notice there are no comments, yet you still were able to understand, using intuition alone.
You will find this theme of Simple everywhere in and around PySimpleGUI. It's a way of thinking as well as an architecture direction. Remember, you, Mr./Ms. Developer, are at the center of the package. So, from your vantage point, of course everything should look and feel simple.
Not only that, it's the Pythonic thing to do. Have a look at line 3 of the "Zen of Python".
> The Zen of Python, by Tim Peters
>
> Beautiful is better than ugly .
> Explicit is better than implicit .
> Simple is better than complex.
> Complex is better than complicated.
> Flat is better than nested.
> Sparse is better than dense.
> Readability counts.
> Special cases aren't special enough to break the rules.
> Although practicality beats purity.
> Errors should never pass silently.
> Unless explicitly silenced.
> In the face of ambiguity, refuse the temptation to guess.
> There should be one-- and preferably only one --obvious way to do it.
> Although that way may not be obvious at first unless you're Dutch.
> Now is better than never.
> Although never is often better than *right* now.
> If the implementation is hard to explain, it's a bad idea.
> If the implementation is easy to explain, it may be a good idea.
> Namespaces are one honking great idea -- let's do more of those!
I just hope reading all these pages of documentation is going to make you believe that we're breaking suggestion:
> If the implementation is hard to explain, it's a bad idea.
> If the implementation is easy to explain, it may be a good idea.
I don't think PySimpleGUI is ***difficult*** to explain, but I am striving to fully explain it so that you don't do this:
> In the face of ambiguity, refuse the temptation to guess.
Sometimes you can guess and be fine. Other times, things may work, but the side effects are potentially significant. Are be a much better way to solve a problem:
* Look in the documentation
* Log an Issue on GitHub!
------
# Some Examples
## Polishing Your Windows = Building "Beautiful Windows"
And STILL the Zen of Python fits:
> Beautiful is better than ugly.
but this fits too:
> Although practicality beats purity.
Find a balance that works for you.
"But tkinter sucks"
"It looks like the 1990s" (this one is often said by people that were not alive in the 1990s)
"What Python GUI SDK will make my window look beautiful?" (posted to Reddit at least every 2 weeks)
These windows below were ALL made using PySimpleGUI, the tkinter version and they look good enough to not be simply scoffed at and dismissed. Remember, developer, you have a rather significant hand in how your application looks and operates. You certainly cannot pin it all on the GUIs you're using.
So many posts on Reddit asking which GUI is going to result in a "beautiful window", as if there's a magic GUI library that pretties things up for you. There are some calls in PySimpleGUI that will help you. For example, you can make a single call to "Change the Theme" which loads predefined color pallets so your windows will instantly have colors that match.
***Beautiful windows are created***, not simply given to you. There are people that design and create artwork for user interfaces, you know that... right? Artists draw buttons, artwork that you include in the window to make it nicer. They understand color theory and how to design an attractive user interface.
### Custom Titlebars - A Trivial Start at Beautification
You can replace the titlebar that your operating system provides with one that is custom to your user interface by using the `Titlebar` element.
Normally, the Operating System provides the titlebar. This means they are unlikely to match your color scheme. Here is a Window with a dark color theme and the default titlebar provided by Windows.

It's an OK window. By adding a `Titlebar` element to your layout, then your window completely matches your color theme. Here is the same window with a PySimpleGUI `Titlebar` element

Regardless of the "Theme" you choose for your window, the Titlebar will match it.



## Some Examples Of More "Polished" Windows
A** note from the "2022 Mike"**... these windows were created 2 years ago. A lot more work has been completed to enable even better windows using packages like Pillow. In other words, these are not the current "best" examples.
The User Screenshots Gallery is currently housed in [Issue #10 on GitHub](https://github.com/PySimpleGUI/PySimpleGUI/issues/10). GitHub does very strange pagination. The MIDDLE portion of the text and images on the page is hidden and you have to repeatedly press a "Load More" link.
Some of these have been "polished", others like the Matplotlib example is more a functional example to show you it works.
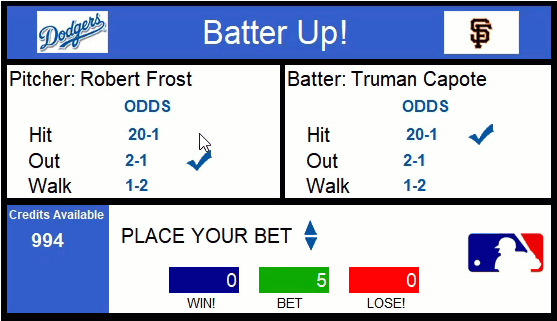
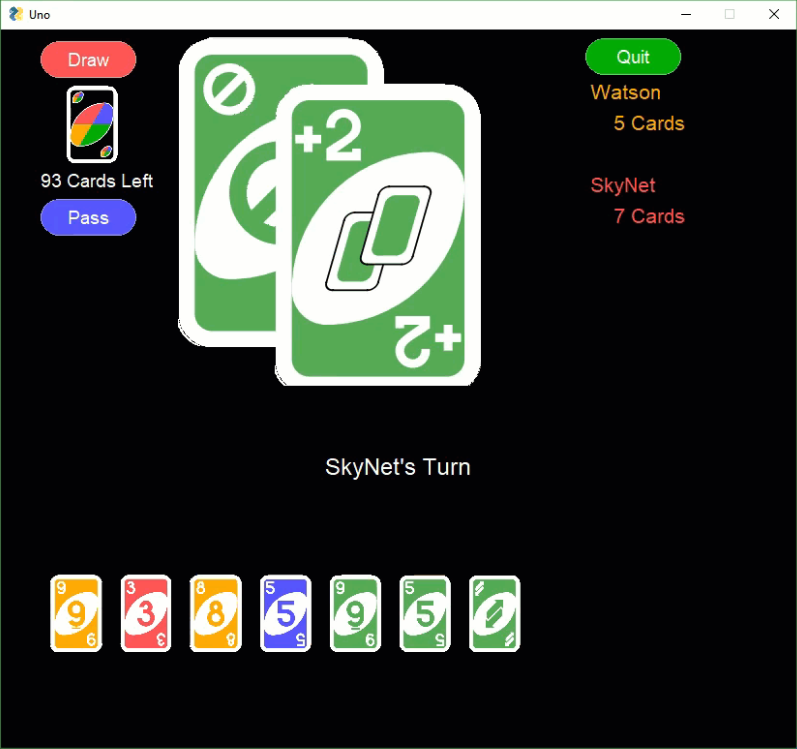
This chess program is capable of running multiple AI chess engines and was written by another user using PySimpleGUI.
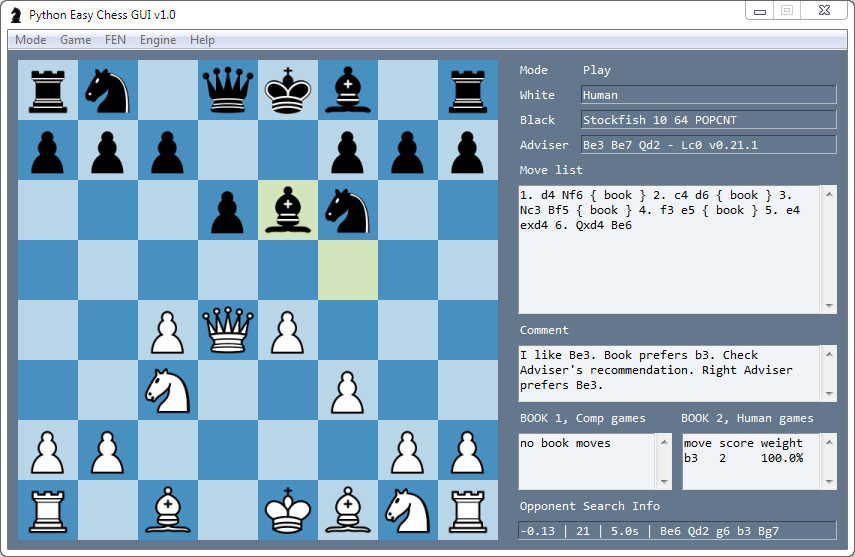
This downloader can download files as well as YouTube videos and metadata. If you're worried about multiple windows working, don't. Worried your project is "too much" or "too complex" for PySimpleGUI? Do an initial assessment if you want. Check out what others have done.
Your program have 2 or 3 windows and you're concerned? Below you'll see 11 windows open, each running independently with multiple tabs per window and progress meters that are all being updated concurrently.
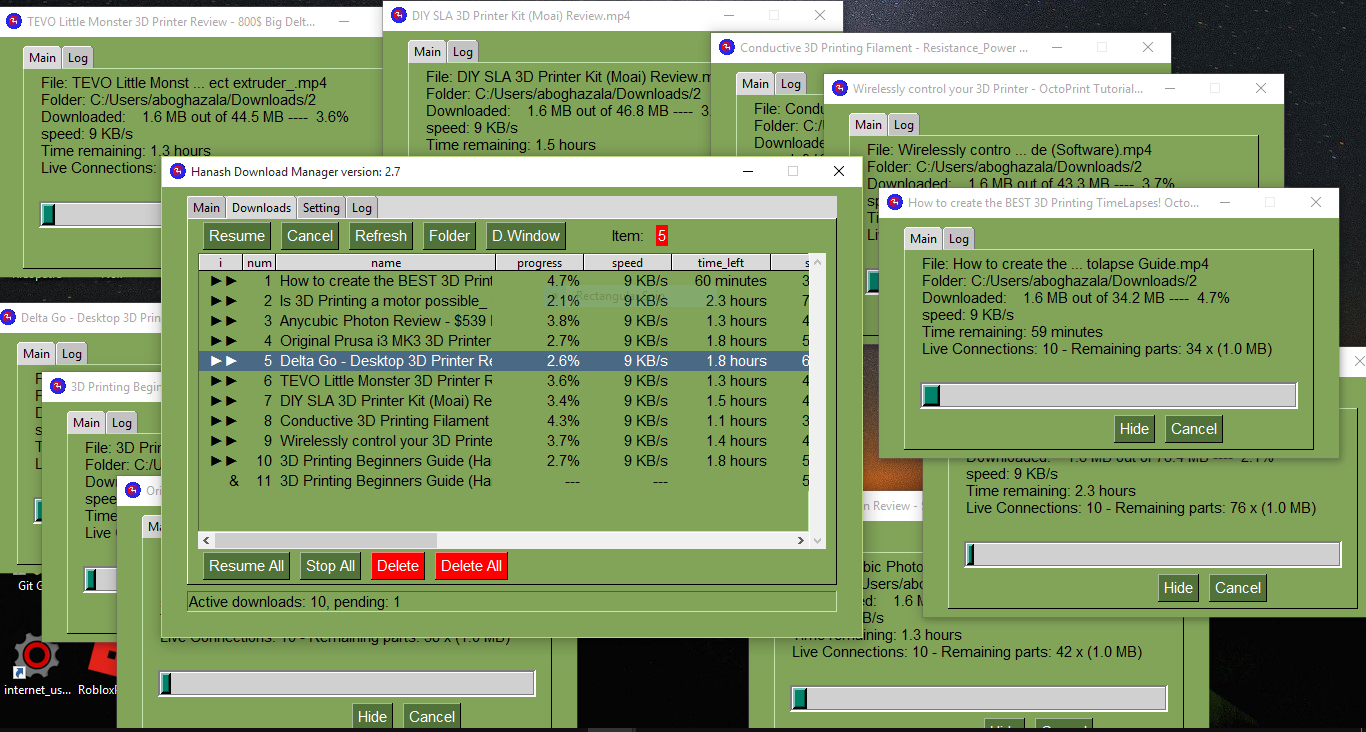
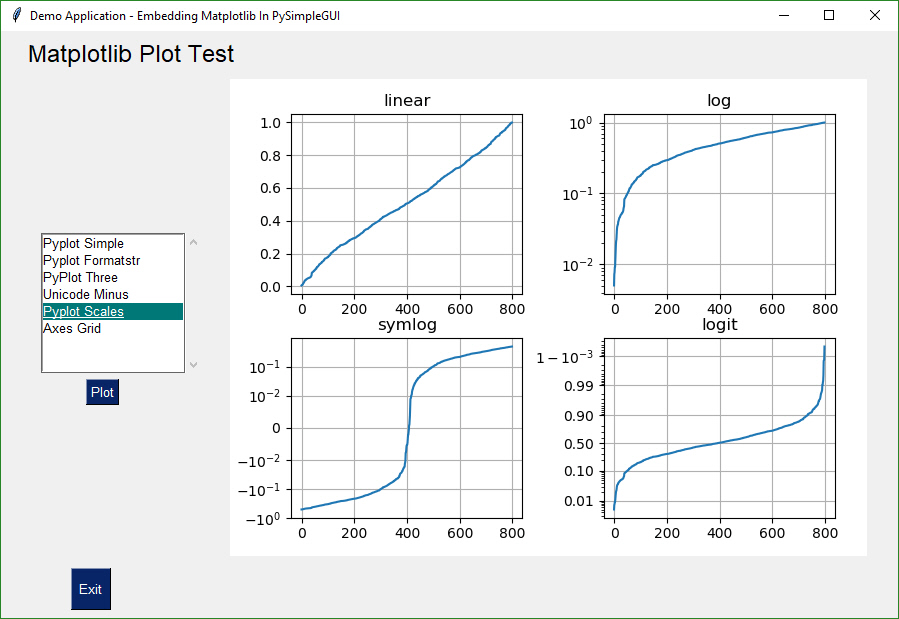
Just because you can't match a pair of socks doesn't mean your windows have to all look the same gray color. Choose from over 100 different "Themes". Add 1 line call to `theme` to instantly transform your window from gray to something more visually pleasing to interact with. If you misspell the theme name badly or specify a theme name is is missing from the table of allowed names, then a theme will be randomly assigned for you. Who knows, maybe the theme chosen you'll like and want to use instead of your original plan.
In PySimpleGUI release 4.6 the number of themes was dramatically increased from a couple dozen to over 100. To use the color schemes shown in the window below, add a call to `theme('Theme Name)` to your code, passing in the name of the desired color theme. To see this window and the list of available themes on your release of software, call the function `theme_previewer()`. This will create a window with the frames like those below. It will shows you exactly what's available in your version of PySimpleGUI.
In release 4.9 another 32 Color Themes were added... here are the current choices
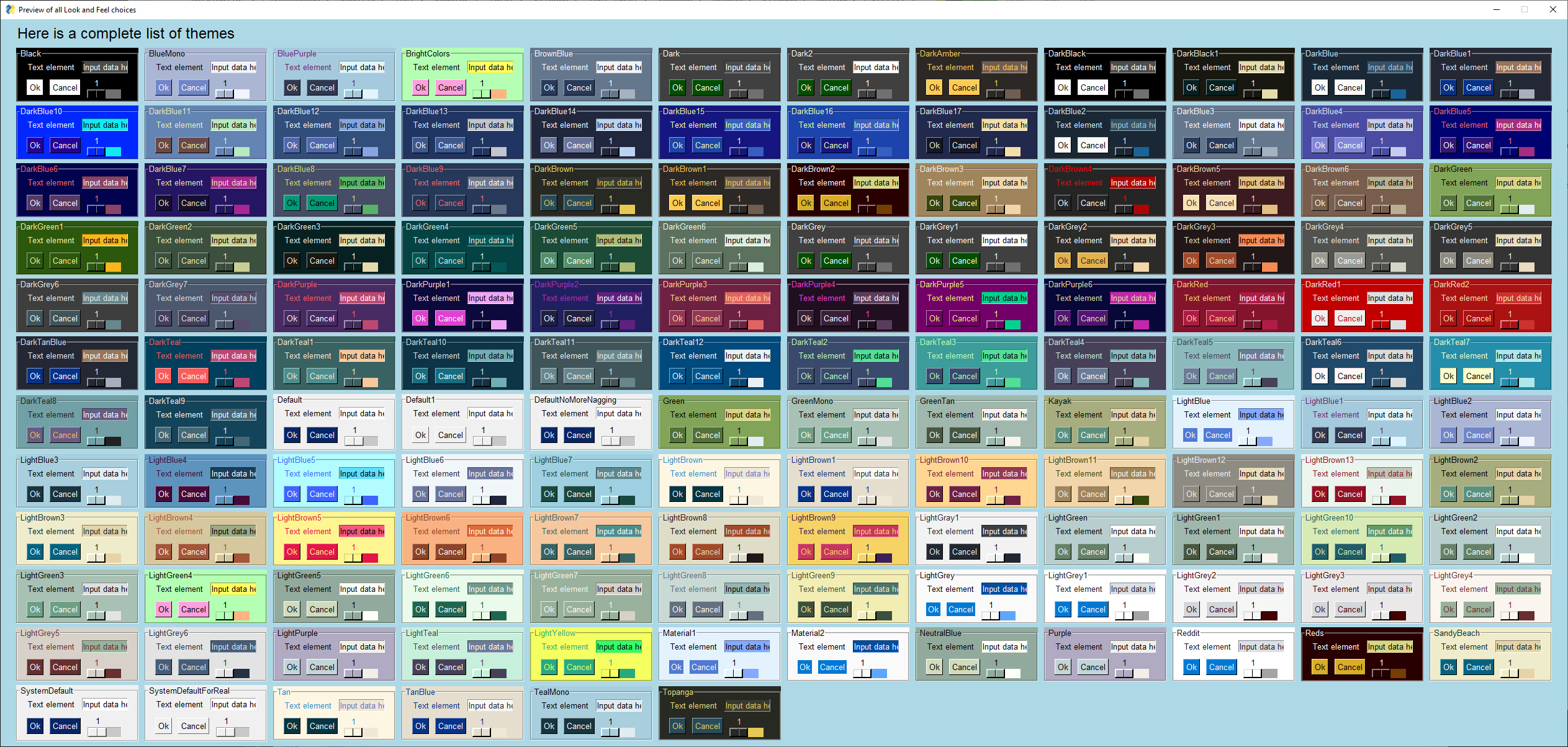
Make beautiful looking, alpha-blended (partially transparent) Rainmeter-style Desktop Widgets that run in the background.
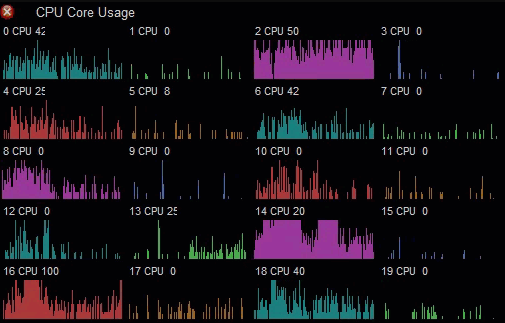
Want to build a Crossword Puzzle? No problem, the drawing primitives are there for you.
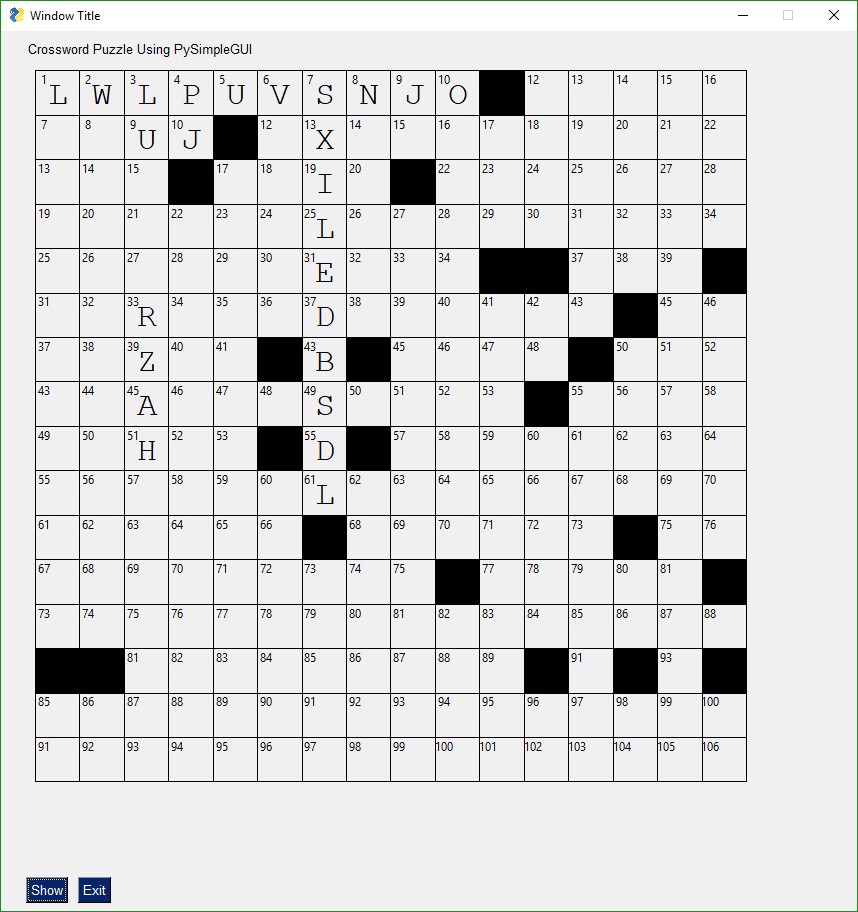
There are built-in drawing primitives
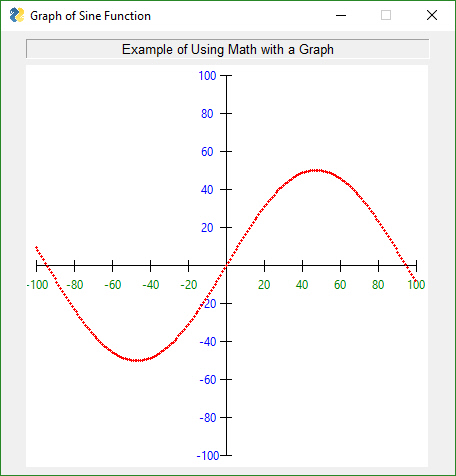
Frame from integration with a YOLO Machine Learning program that does object identification in realtime while allowing the user to adjust the algorithms settings using the sliders under the image. This level of interactivity with an AI algorithm is still unusual to find due to difficulty of merging the technologies of AI and GUI. It's no longer difficult. This program is under 200 lines of code.
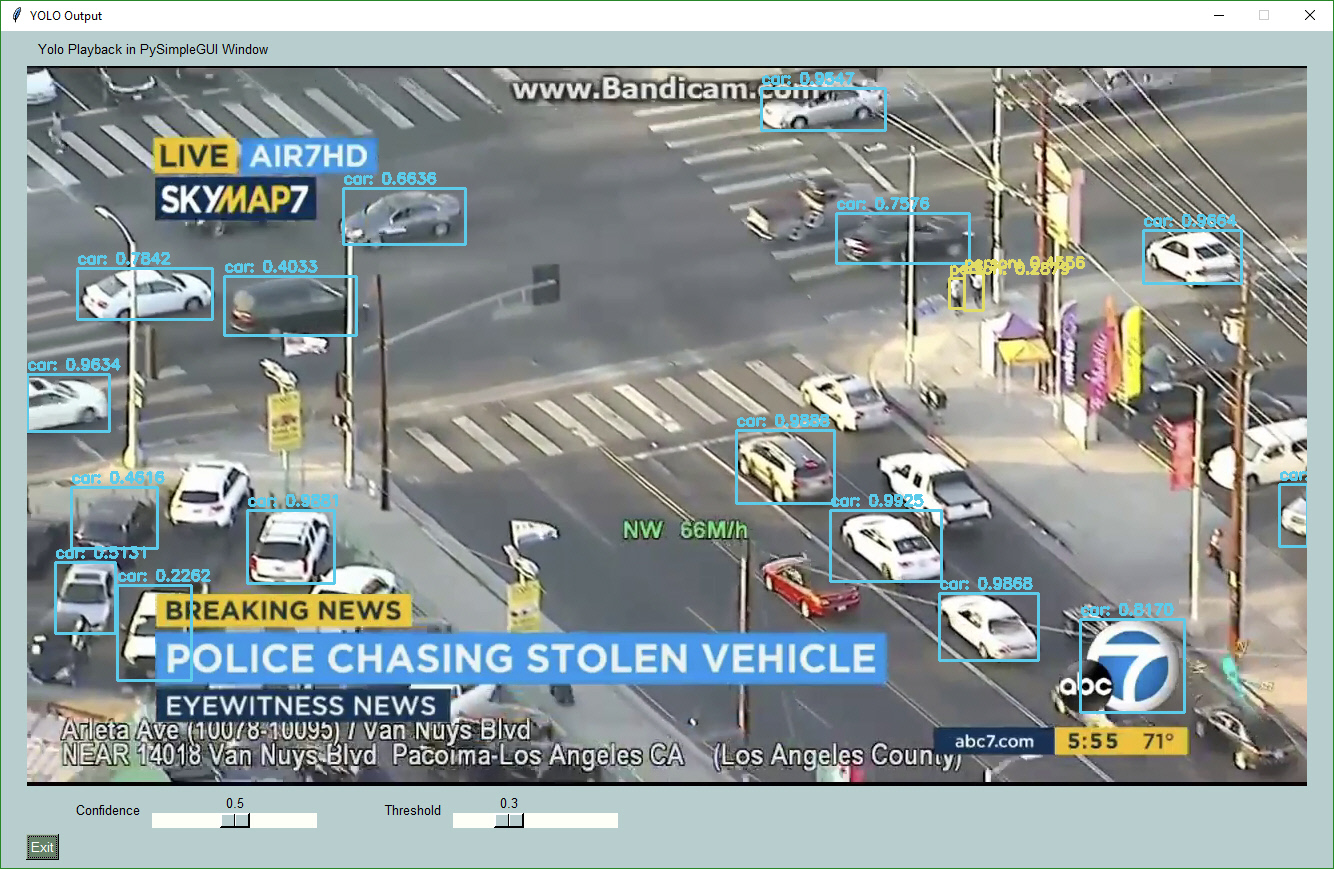
# Pi Windows
Perhaps you're looking for a way to interact with your **Raspberry Pi** in a more friendly way. Your PySimpleGUI code will run on a Pi with no problem. Tkinter is alive and well on the Pi platform. Here is a selection of some of the Elements shown on the Pi. You get the same Elements on the Pi as you do Windows and Linux.
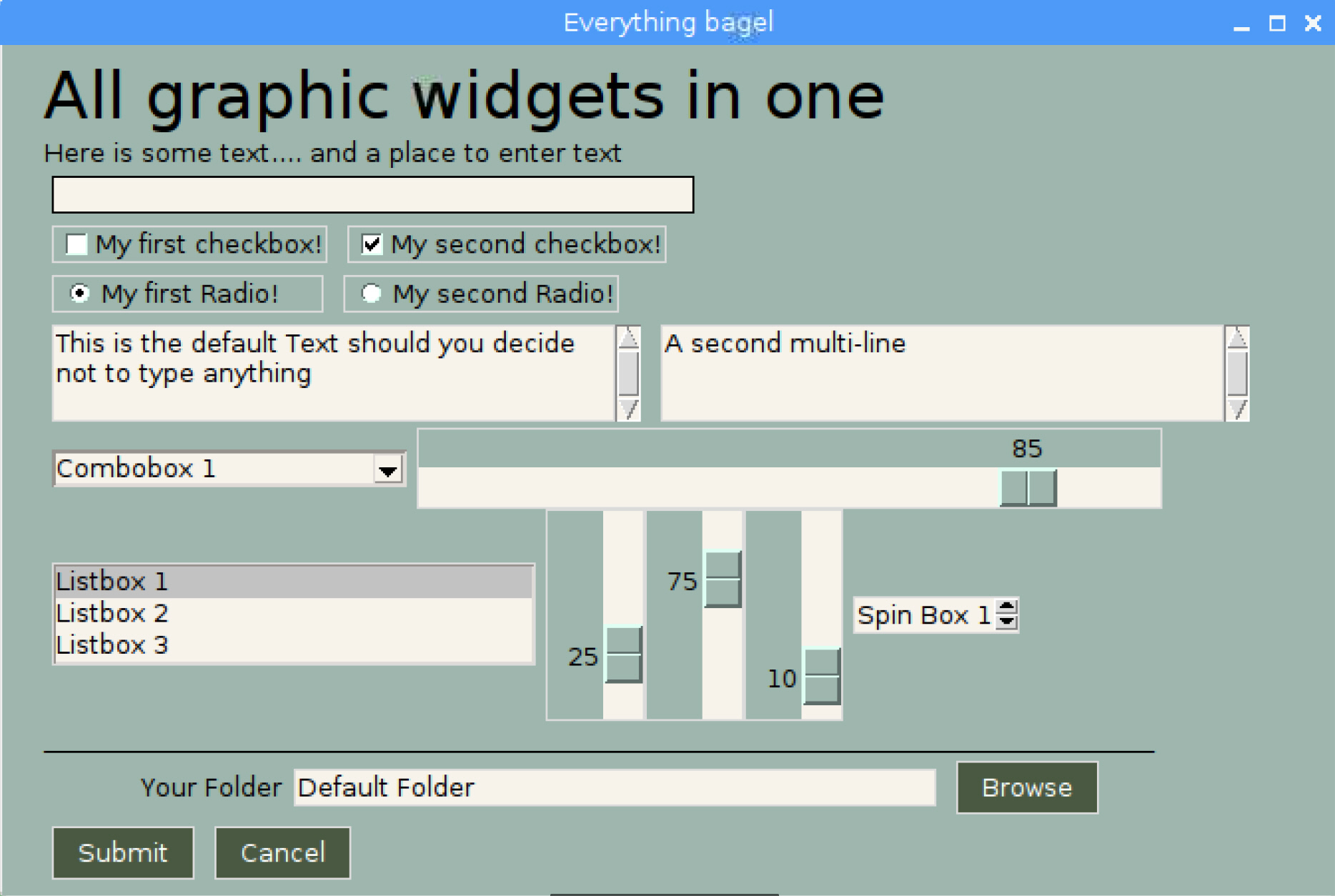
You can add custom artwork to make it look nice, like the Demo Program - Weather Forecast shown in this image:
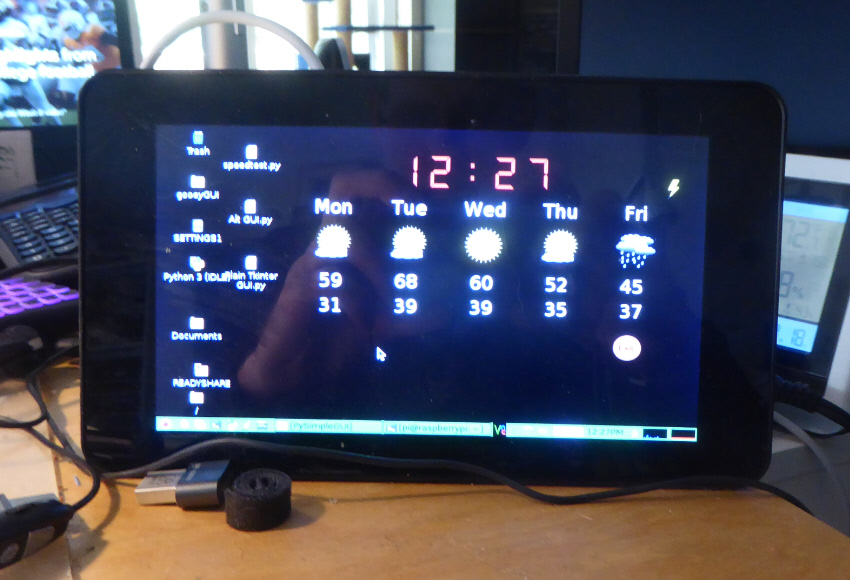
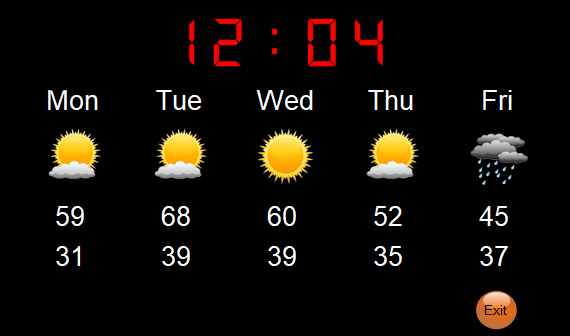
One thing to be aware of with Pi Windows, you cannot make them semi-transparent. This means that the `Window.Disappear` method will not work. Your window will not disappear. Setting the Alpha Channel will have no effect.
Don't forget that you can use custom artwork anywhere, including on the Pi. The weather application looks beautiful on the Pi. Notice there are no buttons or any of the normal looking Elements visible. It's possible to build nice looking applications, even on the lower-end platforms.
# Games
It's possible to create some cool games by simply using the built-in PySimpleGUI graphic primitives' like those used in this game of pong. PyGame can also be embedded into a PySimpleGUI window and code is provided to you demonstrating how. There is also a demonstration of using the pymunk physics package that can also be used for games.
Games haven't not been explored much, yet, using PySimpleGUI.
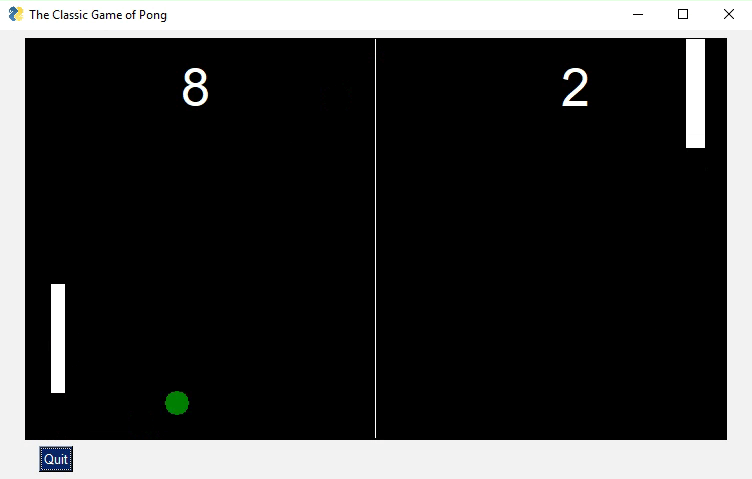
# Windows Programs That Look Like Windows Programs
***Do you have the desire to share your code with other people in your department, or with friends and family?*** Many of them may not have Python on their computer. And in the corporate environment, it may not be possible for you to install Python on their computer.
`PySimpleGUI + PyInstaller` to the rescue!!
Combining PySimpleGUI with PyInstaller creates something truly remarkable and special, a Python program that looks like a Windows WinForms application.
The application you see below with a working menu was created in 20 lines of Python code. It is a single .EXE file that launches straight into the screen you see. And more good news, the only icon you see on the taskbar is the window itself... there is no pesky shell window. Nice, huh?
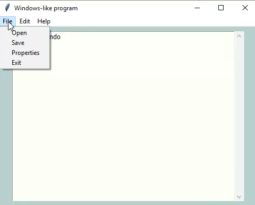
With a simple GUI, it becomes practical to "associate" .py files with the python interpreter on Windows. Double click a py file and up pops a GUI window, a more pleasant experience than opening a dos Window and typing a command line.
There is even a PySimpleGUI program that will take your PySimpleGUI program and turn it into an EXE. It's nice because you can use a GUI to select your file and all of the output is shown in the program's window, in realtime.
# Background - Why PySimpleGUI Came to Be
Feel free to skip all this if you don't care to know the backstory and reasons behind decisions.
There was a project looming and a GUI was needed. It wasn't a very complex GUI so thus began a search for a simplified GUI package that would enable me to work with tkinter easier. I found a few, and they were pretty popular too, but they lacked the full-compliment of Widgets and it was impossible to define my own window using those widgets.
A whacky idea came to mind... what if I wrote a simplified GUI and then used THAT to write my application. It would be a lot less code and it would be "easy" to write my application then. And that is exactly what was done.
First an early version of PySimpleGUI was written that had a subset of the Elements available today. It had just enough for my application. Then I wrote my application in PySimpleGUI.
Thus PySimpleGUI was born out of necessity and it's been the necessity of others that have helped evolve it into the package it is today. It would not be 1/2 as good without the help of the community.
Once PySimpleGUI was done, it was time to start working on "the ports". And, of course, also this documentation.
## The Non-OO and Non-Event-Driven Model
The two "advanced concepts" that beginning Python students have with GUIs are the use of classes and callbacks with their associated communication and coordination mechanisms (semaphores, queues, etc)
How do you make a GUI interface easy enough for first WEEK Python students?
This meant classes could be used to build and use it, but classes can not be part of the code the user writes. Of course, an OO design is quite possible to use with PySimpleGUI, but it's not a ***requirement***. The sample code and docs stay away from writing new classes in the user space for the most part.
What about those pesky callbacks? They're difficult for beginners to grasp and they're a bit of a pain in the ass to deal with. The way PySimpleGUI got around events was to utilize ***a "message passing" architecture*** instead.
Instead of a user function being called when there's some event, instead the information is "passed" to the user when they call the function `Window.read()`
***Everything*** is returned through this `Window.read` call. Of course the underlying GUI frameworks still perform callbacks, but they all happen inside of PySimpleGUI where they are turned into messages to pass to you.
All of the boilerplate code, the event handling, widget creation, frames containing widgets, etc, are **exactly the same** objects and calls that you would be writing if you wrote directly in tkinter, Qt, etc. With all of this code out of the way and done for you, that leaves you with the task of doing something useful with the information the user entered. THAT, after all, is the goal here.... getting user information and acting on it.
The full complement of Widgets are available to you via PySimpleGUI Elements. And those widgets are presented to you in a unique and fun way.
If you wish to learn more about the Architecture of PySimpleGUI, take a look at the [Architecture document located on ReadTheDocs](https://pysimplegui.readthedocs.io/en/latest/architecture/).
### The Result
A GUI that's appealing to a broad audience that is highly customizable, easy to program, and is solid with few bugs and rarely crashes (99% of the time it's some other error that causes a crash).
PySimpleGUI is becoming more and more popular. The number of installs and the number of successes grows daily. Pip installs have exceeded 350,000 in the first year of existence. Over 300 people a day visit the GitHub and the project has 1,800 stars (thank you awesome users!)
The number of ports is up to 4. The number of integrations with other technologies is constantly being expanded. It's a great time to try PySimpleGUI! You've got no more than 5 or 10 minutes to lose.
Caution is needed, however, when working with the unfinished ports. PySimpleGUI, the tkinter version, is the only fully complete port. Qt is next. All of its Elements are completed, but not all of the options of each element are done. PySimpleGUIWeb is next in order of completeness and then finally PySimpleGUIWx.
# Features
While simple to use, PySimpleGUI has significant depth to be explored by more advanced programmers. The feature set goes way beyond the requirements of a beginner programmer, and into the required features needed for complex multi-windowed GUIs.
**The SIMPLE part of PySimpleGUI is how much effort _you_ expend to write a GUI, not the complexity of the program you are able to create.** It's literally "simple" to do... and it is not limited to simple problems.
Features of PySimpleGUI include:
- Support for Python versions 2.7 and 3
- Text
- Single Line Input
- Buttons including these types:
- File Browse
- Files Browse
- Folder Browse
- SaveAs
- Normal button that returns event
- Close window
- Realtime
- Calendar chooser
- Color chooser
- Button Menu
- TTK Buttons or "normal" TK Buttons
- Checkboxes
- Radio Buttons
- Listbox
- Option Menu
- Menubar
- Button Menu
- Slider
- Spinner
- Dial
- Graph
- Frame with title
- Icons
- Multi-line Text Input
- Scroll-able Output
- Images
- Tables
- Trees
- Progress Bar Async/Non-Blocking Windows
- Tabbed windows
- Paned windows
- Persistent Windows
- Multiple Windows - Unlimited number of windows can be open at the same time
- Redirect Python Output/Errors to scrolling window
- 'Higher level' APIs (e.g. MessageBox, YesNobox, ...)
- Single-Line-Of-Code Progress Bar & Debug Print
- Complete control of colors, look and feel
- Selection of pre-defined palettes
- Button images
- Horizontal and Vertical Separators
- Return values as dictionary
- Set focus
- Bind return key to buttons
- Group widgets into a column and place into window anywhere
- Scrollable columns
- Keyboard low-level key capture
- Mouse scroll-wheel support
- Get Listbox values as they are selected
- Get slider, spinner, combo as they are changed
- Update elements in a live window
- Bulk window-fill operation
- Save / Load window to/from disk
- Borderless (no titlebar) windows (very classy looking)
- Always on top windows
- Menus with ALT-hotkey
- Right click pop-up menu
- Tooltips
- Clickable text
- Transparent windows
- Movable windows
- Animated GIFs
- No async programming required (no callbacks to worry about)
- Built-in debugger and REPL
- User expandable by accessing underlying GUI Framework widgets directly
- Exec APIs - wrapper for subprocessing and threading
- UserSettings APIs - wrapper for JSON and INI files
---
## Design Goals
With the developer being the focus, the center of it all, it was important to keep this mindset at all times, including now, today. Why is this such a big deal? Because this package was written so that the universe of Python applications can grow and can **include EVERYONE into the GUI tent.**
> Up in 5 minutes
Success #1 has to happen immediately. Installing and then running your first GUI program. FIVE minutes is the target. The Pip install is under 1 minute. Depending on your IDE and development environment, running your first piece of code could be a copy, paste, and run. This isn't a joke target; it's for real serious.
> Beginners and Advanced Together
Design an interface that both the complete beginner can understand and use that has enough depth that an advanced programmer can make some very nice looking GUIs amd not feel like they're playing with a "toy".
> Success After Success
Success after success.... this is the model that will win developer's hearts. This is what users love about PySimpleGUI. Make your development progress in a way you can run and test your code often. Add a little bit, run it, see it on your screen, smile, move on.
> Copy, Paste, Run.
The Cookbook and Demo Programs are there to fulfill this goal. First get the user seeing on their screen a working GUI that's similar in some way to what they want to create.
If you're wanting to play with OpenCV download the OpenCV Demo Programs and give them a try. Seeing your webcam running in the middle of a GUI window is quite a thrill if you're trying to integrate with the OpenCV package.
"Poof" instant running OpenCV based application == Happy Developer
> Make Simpler Than Expected Interfaces
The Single Line Progress Meter is a good example. It requires one and only 1 line of code. Printing to a debug window is as easy as replacing `print` with `sg.Print` which will route your console output to a scrolling debug window.
> Be Pythonic
Be Pythonic...
This one is difficult for me to define. The code implementing PySimpleGUI isn't PEP8 compliant, but it is consistent. The important thing was what the user saw and experienced while coding, NOT the choices for naming conventions in the implementation code. The user interface to PySimpleGUI now has a PEP8 compliant interface. The methods are snake_case now (in addition to retaining the older CamelCase names)
I ended up defining it as - attempt to use language constructs in a natural way and to exploit some of Python's interesting features. It's Python's lists and optional parameters make PySimpleGUI work smoothly.
Here are some Python-friendly aspects to PySimpleGUI:
- Windows are represented as Python lists of Elements
- Return values are an "event" such a button push and a list/dictionary of input values
- The SDK calls collapse down into a single line of Python code that presents a custom GUI and returns values should you want that extreme of a single-line solution
- Elements are all classes. Users interact with elements using class methods but are not required to write their own classes
- Allow keys and other identifiers be any format you want. Don't limit user to particular types needlessly.
- While some disagree with the single source file, I find the benefits greatly outweigh the negatives
#### Lofty Goals
> Teach GUI Programming to Beginners
By and large PySimpleGUI is a "pattern based" SDK. Complete beginners can copy these standard design patterns or demo programs and modify them without necessarily understanding all of the nuts and bolts of what's happening. For example, they can modify a layout by adding elements even though they may not yet grasp the list of lists concept of layouts.
Beginners certainly can add more `if event == 'my button':` statements to the event loop that they copied from the same design pattern. They will not have to write classes to use this package.
> Capture Budding Graphic Designers & Non-Programmers
The hope is that beginners that are interested in graphic design, and are taking a Python course, will have an easy way to express themselves, right from the start of their Python experience. Even if they're not the best programmers they will be able express themselves to show custom GUI layouts, colors and artwork with ease.
> Fill the GUI Gap (Democratize GUIs)
There is a noticeable gap in the Python GUI solution. Fill that gap and who knows what will happen. At the moment, to make a traditional GUI window using tkinter, Qt, WxPython and Remi, it takes much more than a week, or a month of Python education to use these GUI packages.
They are out of reach of the beginners. Often WAY out of reach. And yet, time and time again, beginners that say they JUST STARTED with Python will ask on a Forum or Reddit for a GUI package recommendation. 9 times out of 10 Qt is recommended. (smacking head with hand). What a waste of characters. You might as well have just told them, "give up".
> Is There a There?
Maybe there's no "there there". ***Or*** maybe a simple GUI API will enable Python to dominate yet another computing discipline like it has so many others. This is one attempt to find out. So far, it sure looks like there's PLENTY of demand in this area.
# Getting Started with PySimpleGUI
There is a "Troubleshooting" section towards the end of this document should you run into real trouble. It goes into more detail about what you can do to help yourself.
## Installing PySimpleGUI
Of course if you're installing for Qt, WxPython, Web, you'll use PySimpleGUIQt, PySimpleGUIWx, and PySimpleGUIWeb instead of straight PySimpleGUI in the instructions below. You should already have the underlying GUI Framework installed and perhaps tested. This includes tkinter, PySide2, WxPython, Remi
### Installing on Python 3
`pip install --upgrade PySimpleGUI`
On some systems you need to run pip3. (Linux and Mac)
`pip3 install --upgrade PySimpleGUI`
On a Raspberry Pi, this is should work:
`sudo pip3 install --upgrade pysimplegui`
Some users have found that upgrading required using an extra flag on the pip `--no-cache-dir`.
`pip install --upgrade --no-cache-dir PySimpleGUI`
On some versions of Linux you will need to first install pip. Need the Chicken before you can get the Egg (get it... Egg?)
`sudo apt install python3-pip`
`tkinter` is a requirement for PySimpleGUI (the only requirement). Some OS variants, such as Ubuntu, do not some with `tkinter` already installed. If you get an error similar to:
`ImportError: No module named tkinter`
then you need to install `tkinter`.
For python 2.7
`sudo apt-get install python-tk`
For python 3
`sudo apt-get install python3-tk`
More information about installing tkinter can be found here: https://www.techinfected.net/2015/09/how-to-install-and-use-tkinter-in-ubuntu-debian-linux-mint.html
### Installing typing module for Python 3.4 (Raspberry Pi)
In order for the docstrings to work correctly the `typing` module is used. In Python version 3.4 the typing module is not part of Python and must be installed separately. You'll see a warning printed on the console if this module isn't installed.
You can pip install `typing` just like PySimpleGUI. However it's not a requirement as PySimpleGUI will run fine without typing installed as it's only used by the docstrings.
### Installing for Python 2.7
**IMPORTANT** PySimpleGUI27 will disappear from the GitHub on Dec 31, 2019. PLEASE migrate to 3.6 at least. It's not painful for most people.
`pip install --upgrade PySimpleGUI27`
or
`pip2 install --upgrade PySimpleGUI27`
You may need to also install "future" for version 2.7
`pip install future`
or
`pip2 install future`
Like above, you may have to install either pip or tkinter. To do this on Python 2.7:
`sudo apt install python-pip`
`sudo apt install python-tkinter`
## Upgrading from GitHub Using PySimpleGUI
There is code in the PySimpleGUI package that upgrades your previously pip installed package to the latest version checked into GitHub.
It overwrites your PySimpleGUI.py file that you installed using `pip` with the currently posted version on GitHub. Using this method when you're ready to install the next version from PyPI or you want to maybe roll back to a PyPI release, you only need to run `pip`.
### The PySimpleGUI "Test Harness"
If you call `sg.main()` then you'll get the test harness and can use the upgrade feature.
After you've pip installed, you can use the commands `psgmain` to run the test harness or `psgupgrade` to invoke the GitHub upgrade code.
There have been problems on some machines when `psgmain` and `psgupgrade` are used to upgrade PySimpleGUI. The upgrade is in-place so there can be file locking problems. If you have trouble using these commands, then you can also upgrade using this command:
`python -m PySimpleGUI.PySimpleGUI upgrade`
The "Safest" approach is to call `sg.main()` from your code and then click the red upgrade button.
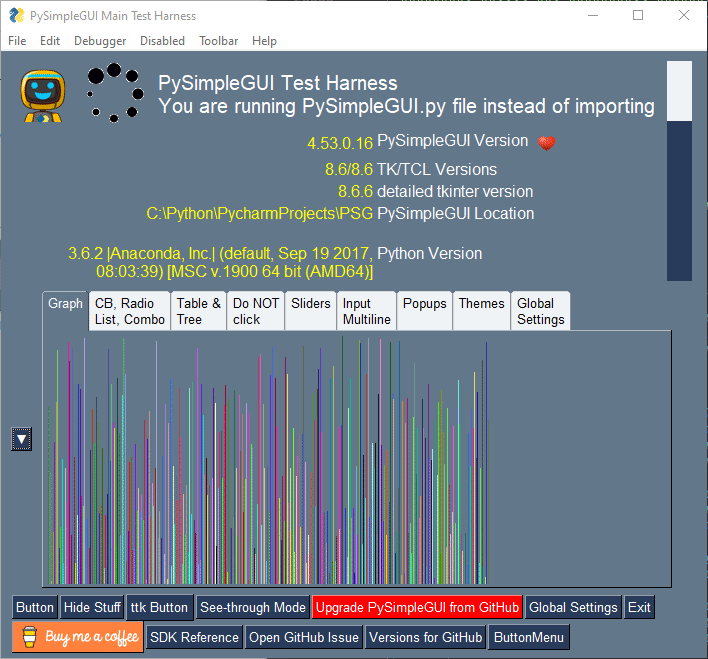
### Recovering From a Bad GitHub Release
If you run into a problem upgrading after upgrading from GitHub, you can likely use pip to uninstall, then re-install from PyPI to see if you can upgrade again from GitHub.
`pip uninstall PySimpleGUI`
`pip install PySimpleGUI`
### Testing your installation and Troubleshooting
Once you have installed, or copied the .py file to your app folder, you can test the installation using python.
#### The Quick Test
The PySimpleGUI Test Harness pictured in the previous section on GUI upgrades is the short program that's built into PySimpleGUI that serves multiple purposes. It exercises many/most of the available Elements, displays version and location data and works as a quick self-test.
`psgmain` is a command you can enter to the run PySimpleGUI test harness if you pip installed. You can also use:
From your command line type:
`python -m PySimpleGUI.PySimpleGUI`
If you're on Linux/Mac and need to run using the command `python3` then of course type that.
This will display the test harness window.
You can also test by using the REPL....
#### Instructions for Testing Python 2.7:
```python
>>> import PySimpleGUI27
>>> PySimpleGUI27.main()
```
#### Instructions for Testing Python 3:
```python3
>>> import PySimpleGUI
>>> PySimpleGUI.main()
```
You will see a "test harness" that exercises the SDK, tells you the version number, allows you to try a number of features as well as access the built-in GitHub upgrade utility.
### Finding Out Where Your PySimpleGUI Is Coming From
It's **critical** for you to be certain where your code is coming from and which version you're running.
Sometimes when debugging, questions arise as to exactly which PySimpleGUI you are running. The quick way to find this out is to again, run Python from the command line. This time you'll type:
```python3
>>> import PySimpleGUI as sg
>>> sg
```
When you type sg, Python will tell you the full patch to your PySimpleGUI file / package. This is critical information to know when debugging because it's really easy to forget you've got an old copy of PySimpleGUI laying around somewhere.
### Finding Out Where Your PySimpleGUI Is Coming From (from within your code)
If you continue to have troubles with getting the right version of PySimpleGUI loaded, THE ***definitive*** way to determine where your program is getting PySimpleGUI from is to add a print to your program. It's that *simple*! You can also get the version you are running by also printing
```python
import PySimpleGUI as sg
print(sg)
print(sg.version)
```
Just like when using the REPL >>> to determine the location, this `print` in your code will display the same path information.
### Manual installation
If you're not connected to the net on your target machine, or pip isn't working, or you want to run the latest code from GitHub, then all you have to do is place the single PySimpleGUI source file `PySimpleGUI.py` (for tkinter port) in your application's folder (the folder where the py file is that imports PySimpleGUI). Your application will load that local copy of PySimpleGUI as if it were a package.
Be ***sure*** that you delete this PySimpleGUI.py file if you install a newer pip version. Often the sequence of events is that a bug you've reported was fixed and checked into GitHub. You download the PySimpleGUI.py file (or the appropriately named one for your port) and put with your app. Then later your fix is posted with a new release on PyPI. You'll want to delete the GitHub one before you install from pip.
### Prerequisites
Python 2.7 or Python 3
tkinter
PySimpleGUI Runs on all Python3 platforms that have tkinter running on them. It has been tested on Windows, Mac, Linux, Raspberry Pi. Even runs on `pypy3`.
### EXE file creation
If you wish to create an EXE from your PySimpleGUI application, you will need to install `PyInstaller` or `cx_freeze`. There are instructions on how to create an EXE at the bottom of this document.
The PySimpleGUI EXE Maker can be found in a repo in the PySimpleGUI GitHub account. It's a simple front-end to pyinstaller.
## IDEs
A lot of people ask about IDEs, and many outright fear PyCharm. Compared to your journey of learning Python, learning to use PyCharm as your IDE is **nothing**. It's a DAY typically (from 1 to 8 hours). Or, if you're really really new, perhaps as much as a week *to get used to*. So, we're not talking about you needing to learn to flap your arms and fly.
If you found this package, then you're a bright person :-) Have some confidence in yourself for Christ sake.... I do. Not going to lead you off some cliff, promise!
Some IDEs provide virtual environments, but it's optional. PyCharm is one example. For these, you will either use their GUI interface to add packages or use their built-in terminal to do pip installs. **It's not recommended for beginners to be working with Virtual Environments.** They can be quite confusing. However, if you are a seasoned professional developer and know what you're doing, there is nothing about PySimpleGUI that will prevent you from working this way.
### Officially Supported IDEs
A number of IDEs have **known problems with PySimpleGUI**. IDLE, Spyder, and Thonny all have known, demonstrable, problems with intermittent or inconsistent results, **especially when a program exits** and you want to continue to work with it. *** Any IDE that is based on tkinter is going to have issues with the straight PySimpleGUI port.*** This is NOT a PySimpleGUI problem.
The official list of supported IDEs is:
1. PyCharm (or course this is THE IDE to use for use with PySimpleGUI)
2. Wing
3. Visual Studio
If you're on a Raspberry Pi or some other limited environment, then you'll may have to use IDLE or Thonny. Just be aware there could be problems using the debugger to debug due to both using tkinter.
### Using The Docstrings (Don't skip this section)
Beginning with the 4.0 release of PySimpleGUI, the tkinter port, a whole new world opened up for PySimpleGUI programmers, one where referencing the readme and ReadTheDocs documentation is no longer needed. PyCharm and Wing both support these docstrings REALLY well and I'm sure Visual Studio does too. Why is this important? Because it will teach you the PySimpleGUI SDK as you use the package.
Don't know the parameters and various options for the `InputText` Element? It's a piece of cake with PyCharm. You can set PyCharm to automatically display documentation about the class, function, method, etc, that your cursor is currently sitting on. You can also manually bring up the documentation by pressing CONTROL+Q. When you do, you'll be treated to a window similar to this:
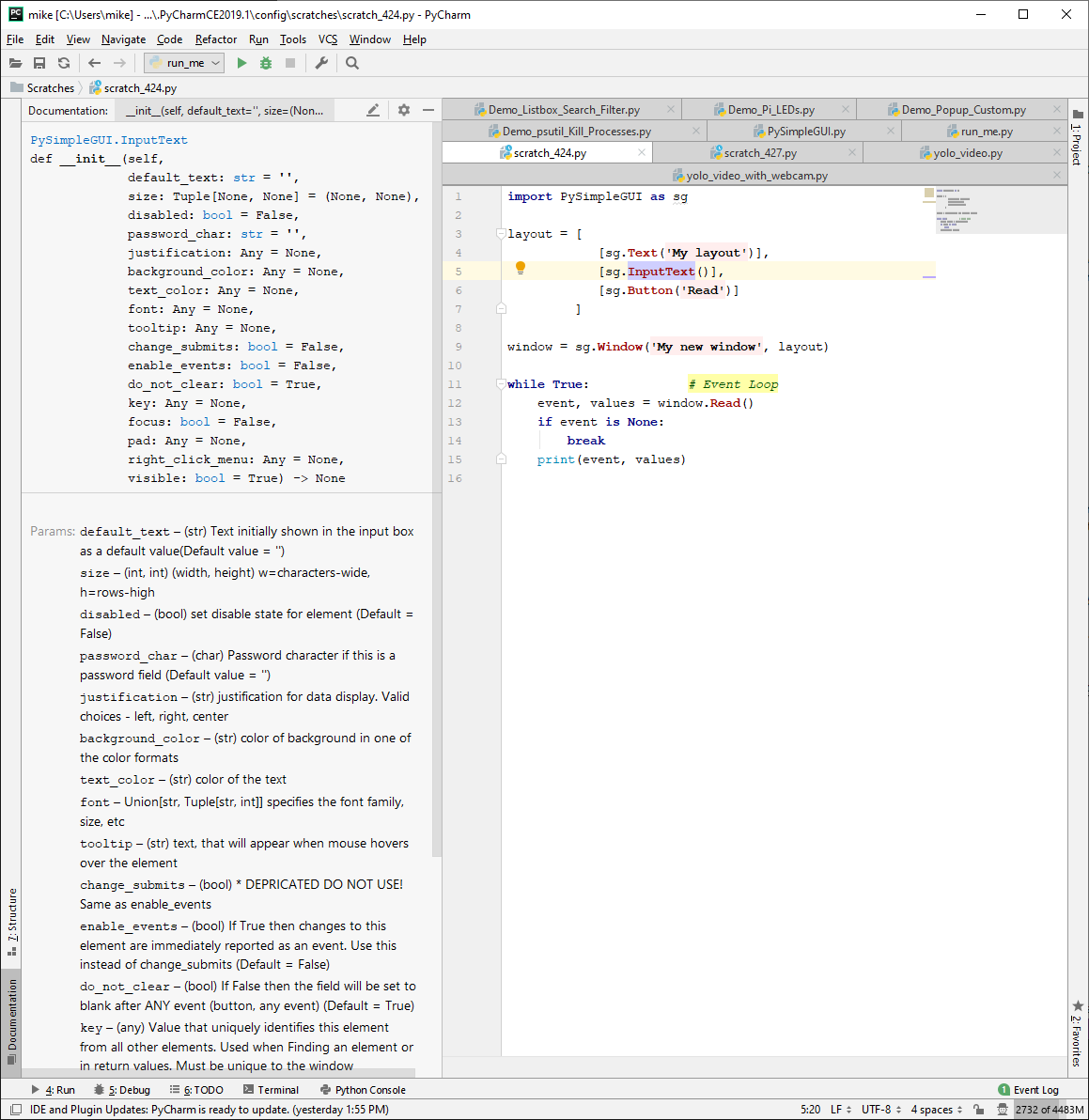
Note that my cursor is on `InputText`. On the left side of the screen, the `InputText` element's parameters are not just shown to you, but they are each individually described to you, and, the type is shown as well. *I mean, honestly, how much more could you ask for?*
OK, I suppose you could ask for a smaller window that just shows the parameters are you're typing them in. Well, OK, in PyCharm, when your cursor is between the `( )` press CONTROL+P. When you do, you'll be treated to a little window like this one:
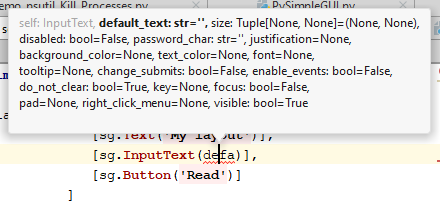
See.... written with the "Developer" in mind, at all times. It's about YOU, Mr/Ms Developer! So enjoy your package.
The other ports of PySimpleGUI (Qt, WxPython, Web) have not yet had their docstrings updated. They're NEXT in line to be better documented. Work on a tool has already begun to make that happen sooner than later.
#### Type Checking With Docstrings
In version 4.17.0 a new format started being used for docstrings. This new format more clearly specified the types for each parameter. It will take some time to get all of the parameter types correctly identified and documented.
Pay attention when you're working with PyCharm and you'll see where you may have a mismatch... or where there's a bad docstring, take your pick. It will shade your code in a way that makes mismatched types very clear to see.
## Using - Python 3
To use in your code, simply import....
`import PySimpleGUI as sg`
Then use either "high level" API calls or build your own windows.
`sg.popup('This is my first popup')`
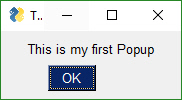
Yes, it's just that easy to have a window appear on the screen using Python. With PySimpleGUI, making a custom window appear isn't much more difficult. The goal is to get you running on your GUI within ***minutes***, not hours nor days.
### Python 3.7
If you must run 3.7, try 3.7.2. It does work with PySimpleGUI with no known issues.
***PySimpleGUI with Python 3.7.3 and 3.7.4+.*** tkinter is having issues with all the newer releases. Things like Table colors stopped working entirely.
March 2020 - Still not quite sure if all issues have been ironed out with tkinter in the 3.7 and 3.8 releases.
## Python 2.7
On December 31, 2019 the Python 2.7 version of PySimpleGUI will be **deleted** from the GitHub. Sorry but Legacy Python has no permanent home here. The security experts claim that supporting 2.7 is doing a disservice to the Python community. I understand why. There are some very narrow cases where 2.7 is required. If you have one, make a copy of PySimpleGUI27.py quickly before it disappears for good.
---
# PEP8 Bindings For Methods and Functions
Beginning with release 4.3 of PySimpleGUI, ***all methods and function calls*** have PEP8 equivalents. This capability is only available, for the moment, on the PySimpleGUI tkinter port. It is being added, as quickly as possible, to all of the ports.
As long as you know you're sticking with tkinter for the short term, it's safe to use the new bindings.
## The Non-PEP8 Methods and Functions
Why the need for these bindings? Simply put, the PySimpleGUI SDK has a PEP8 violation in the method and function names. PySimpleGUI uses CamelCase names for methods and functions. PEP8 suggests using snake_case_variables instead.
This has not caused any problems and few complaints, but it's important the the interfaces into PySimpleGUI be compliant. Perhaps one of the reasons for lack of complaints is that the Qt library also uses SnakeCase for its methods. This practice has the effect of labelling a package as being "not Pythonic" and also suggests that this package was originally used in another language and then ported to Python. This is exactly the situation with Qt. It was written for C++ and the interfaces continue to use C++ conventions.
***PySimpleGUI was written in Python, for Python.*** The reason for the name problem was one of ignorance. The PEP8 convention wasn't understood by the developers when PySimpleGUI was designed and implemented.
You can, and will be able to for some time, use both names. However, at some point in the future, the CamelCase names will disappear. A utility is planned to do the conversion for the developer when the old names are remove from PySimpleGUI.
The help system will work with both names as will your IDE's docstring viewing. However, the result found will show the CamelCase names. For example `help(sg.Window.read)` will show the CamelCase name of the method/function. This is what will be returned:
`Read(self, timeout=None, timeout_key='__TIMEOUT__', close=False)`
## The Renaming Convention
To convert a CamelCase method/function name to snake_case, you simply place an `_` where the Upper Case letter is located. If there are none, then only the first letter is changed.
`Window.FindElement` becomes `Window.find_element`
## Class Variables
For the time being, class variables will remain the way they are currently. It is unusual, in PySimpleGUI, for class variables to be modified or read by the user code so the impact of leaving them is rarely seen in your code.
# High Level API Calls - Popup's
"High level calls" are those that start with "popup". They are the most basic form of communications with the user. They are named after the type of window they create, a pop-up window. These windows are meant to be short lived while, either delivering information or collecting it, and then quickly disappearing.
Think of Popups as your first windows, sorta like your first bicycle. It worked well, but was limited. It probably wasn't long before you wanted more features and it seemed too limiting for your newly found sense of adventure.
When you've reached the point with Popups that you are thinking of filing a GitHub "Enhancement Issue" to get the Popup call extended to include a new feature that you think would be helpful.... not just to you but others is what you had in mind, right? For the good of others.
Well, don't file that enhancement request. Instead, it's at THIS time that you should immediately turn to the section entitled "Custom Window API Calls - Your First Window". Congratulations, you just graduated and are now an official "GUI Designer". Oh, never mind that you only started learning Python 2 weeks ago, you're a real GUI Designer now so buck up and start acting like one. Write a popup function of your own. And then, compact that function down to a **single line of code**. Yes, these popups can be written in 1 line of code. The secret is to use the `close` parameter on your call to `window.read()`
But, for now, let's stick with these 1-line window calls, the Popups. This is the list of popup calls available to you:
popup_animated
popup_annoying
popup_auto_close
popup_cancel
popup_error
popup_get_file
popup_get_folder
popup_get_text
popup_no_border
popup_no_buttons
popup_no_frame
popup_no_titlebar
popup_no_wait
popup_notify
popup_non_blocking
popup_ok
popup_ok_cancel
popup_quick
popup_quick_message
popup_scrolled
popup_timed
popup_yes_no
## Popup Output
Think of the `popup` call as the GUI equivalent of a `print` statement. It's your way of displaying results to a user in the windowed world. Each call to Popup will create a new Popup window.
`popup` calls are normally blocking. your program will stop executing until the user has closed the Popup window. A non-blocking window of Popup discussed in the async section.
Just like a print statement, you can pass any number of arguments you wish. They will all be turned into strings and displayed in the popup window.
There are a number of Popup output calls, each with a slightly different look or functionality (e.g. different button labels, window options).
The list of Popup output functions are:
- popup
- popup_ok
- popup_yes_no
- popup_cancel
- popup_ok_cancel
- popup_error
- popup_timed, popup_auto_close, popup_quick, popup_quick_message
- popup_no_waitWait, popup_non_blocking
- popup_notify
The trailing portion of the function name after Popup indicates what buttons are shown. `PopupYesNo` shows a pair of button with Yes and No on them. `PopupCancel` has a Cancel button, etc..
While these are "output" windows, they do collect input in the form of buttons. The Popup functions return the button that was clicked. If the Ok button was clicked, then Popup returns the string 'Ok'. If the user clicked the X button to close the window, then the button value returned is `None` or `WIN_CLOSED` is more explicit way of writing it.
The function `popup_timed` or `popup_auto_close` are popup windows that will automatically close after come period of time.
Here is a quick-reference showing how the Popup calls look.
```python
sg.popup('popup') # Shows OK button
sg.popup_ok('popup_ok') # Shows OK button
sg.popup_yes_no('popup_yes_no') # Shows Yes and No buttons
sg.popup_cancel('popup_cancel') # Shows Cancelled button
sg.popup_ok_cancel('popup_ok_cancel') # Shows OK and Cancel buttons
sg.popup_error('popup_error') # Shows red error button
sg.popup_timed('popup_timed') # Automatically closes
sg.popup_auto_close('popup_auto_close') # Same as PopupTimed
```
Preview of popups:
Popup - Display a popup Window with as many parms as you wish to include. This is the GUI equivalent of the
"print" statement. It's also great for "pausing" your program's flow until the user can read some error messages.
If this popup doesn't have the features you want, then you can easily make your own. Popups can be accomplished in 1 line of code:
choice, _ = sg.Window('Continue?', [[sg.T('Do you want to continue?')], [sg.Yes(s=10), sg.No(s=10)]], disable_close=True).read(close=True)
```
popup(args=*<1 or N object>,
title = None,
button_color = None,
background_color = None,
text_color = None,
button_type = 0,
auto_close = False,
auto_close_duration = None,
custom_text = (None, None),
non_blocking = False,
icon = None,
line_width = None,
font = None,
no_titlebar = False,
grab_anywhere = False,
keep_on_top = None,
location = (None, None),
relative_location = (None, None),
any_key_closes = False,
image = None,
modal = True,
button_justification = None,
drop_whitespace = True)
```
Parameter Descriptions:
|Type|Name|Meaning|
|--|--|--|
| Any | *args | Variable number of your arguments. Load up the call with stuff to see! |
| str | title | Optional title for the window. If none provided, the first arg will be used instead. |
| (str, str) or str | button_color | Color of the buttons shown (text color, button color) |
| str | background_color | Window's background color |
| str | text_color | text color |
| int | button_type | NOT USER SET! Determines which pre-defined buttons will be shown (Default value = POPUP_BUTTONS_OK). There are many Popup functions and they call Popup, changing this parameter to get the desired effect. |
| bool | auto_close | If True the window will automatically close |
| int | auto_close_duration | time in seconds to keep window open before closing it automatically |
| (str, str) or str | custom_text | A string or pair of strings that contain the text to display on the buttons |
| bool | non_blocking | If True then will immediately return from the function without waiting for the user's input. |
| str or bytes | icon | icon to display on the window. Same format as a Window call |
| int | line_width | Width of lines in characters. Defaults to MESSAGE_BOX_LINE_WIDTH |
| str or Tuple[font_name, size, modifiers] | font | specifies the font family, size, etc. Tuple or Single string format 'name size styles'. Styles: italic * roman bold normal underline overstrike |
| bool | no_titlebar | If True will not show the frame around the window and the titlebar across the top |
| bool | grab_anywhere | If True can grab anywhere to move the window. If no_titlebar is True, grab_anywhere should likely be enabled too |
| (int, int) | location | Location on screen to display the top left corner of window. Defaults to window centered on screen |
| (int, int) | relative_location | (x,y) location relative to the default location of the window, in pixels. Normally the window centers. This location is relative to the location the window would be created. Note they can be negative. |
| bool | keep_on_top | If True the window will remain above all current windows |
| bool | any_key_closes | If True then will turn on return_keyboard_events for the window which will cause window to close as soon as any key is pressed. Normally the return key only will close the window. Default is false. |
| str or bytes | image | Image to include at the top of the popup window |
| bool | modal | If True then makes the popup will behave like a Modal window... all other windows are non-operational until this one is closed. Default = True |
| bool | right_justify_buttons | If True then the buttons will be "pushed" to the right side of the Window |
| str | button_justification | Speficies if buttons should be left, right or centered. Default is left justified |
| bool | drop_whitespace | Controls is whitespace should be removed when wrapping text. Parameter is passed to textwrap.fill. Default is to drop whitespace (so popup remains backward compatible) |
| str or None | **RETURN** | Returns text of the button that was pressed. None will be returned if user closed window with X
The other output Popups are variations on parameters. Usually the button_type parameter is the primary one changed.
The choices for button_type are (you should not specify these yourself however):
```
POPUP_BUTTONS_YES_NO
POPUP_BUTTONS_CANCELLED
POPUP_BUTTONS_ERROR
POPUP_BUTTONS_OK_CANCEL
POPUP_BUTTONS_OK
POPUP_BUTTONS_NO_BUTTONS
```
**Note that you should not call Popup yourself with different button_types.** Rely on the Popup function named that sets that value for you. For example `popup_yes_no` will set the button type to POPUP_BUTTONS_YES_NO for you.
### Scrolled Output
There is a scrolled version of Popups should you have a lot of information to display.
Show a scrolled Popup window containing the user's text that was supplied. Use with as many items to print as you
want, just like a print statement.
```
popup_scrolled(args=*<1 or N object>,
title = None,
button_color = None,
background_color = None,
text_color = None,
yes_no = False,
no_buttons = False,
button_justification = "l",
auto_close = False,
auto_close_duration = None,
size = (None, None),
location = (None, None),
relative_location = (None, None),
non_blocking = False,
no_titlebar = False,
grab_anywhere = False,
keep_on_top = None,
font = None,
image = None,
icon = None,
modal = True,
no_sizegrip = False)
```
Parameter Descriptions:
|Type|Name|Meaning|
|--|--|--|
| Any | *args | Variable number of items to display |
| str | title | Title to display in the window. |
| (str, str) or str | button_color | button color (foreground, background) |
| bool | yes_no | If True, displays Yes and No buttons instead of Ok |
| bool | no_buttons | If True, no buttons will be shown. User will have to close using the "X" |
| str | button_justification | How buttons should be arranged. l, c, r for Left, Center or Right justified |
| bool | auto_close | if True window will close itself |
| int or float | auto_close_duration | Older versions only accept int. Time in seconds until window will close |
| (int, int) | size | (w,h) w=characters-wide, h=rows-high |
| (int, int) | location | Location on the screen to place the upper left corner of the window |
| (int, int) | relative_location | (x,y) location relative to the default location of the window, in pixels. Normally the window centers. This location is relative to the location the window would be created. Note they can be negative. |
| bool | non_blocking | if True the call will immediately return rather than waiting on user input |
| str | background_color | color of background |
| str | text_color | color of the text |
| bool | no_titlebar | If True no titlebar will be shown |
| bool | grab_anywhere | If True, than can grab anywhere to move the window (Default = False) |
| bool | keep_on_top | If True the window will remain above all current windows |
| (str or (str, int[, str]) or None) | font | specifies the font family, size, etc. Tuple or Single string format 'name size styles'. Styles: italic * roman bold normal underline overstrike |
| str or bytes | image | Image to include at the top of the popup window |
| bytes or str | icon | filename or base64 string to be used for the window's icon |
| bool | modal | If True then makes the popup will behave like a Modal window... all other windows are non-operational until this one is closed. Default = True |
| bool | no_sizegrip | If True no Sizegrip will be shown when there is no titlebar. It's only shown if there is no titlebar |
| str or None or TIMEOUT_KEY | **RETURN** | Returns text of the button that was pressed. None will be returned if user closed window with X
Typical usage:
```python
sg.popup_scrolled(my_text)
```
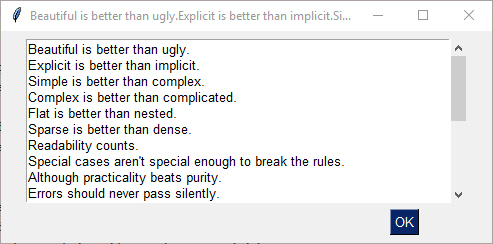
The `popup_scrolled` will auto-fit the window size to the size of the text. Specify `None` in the height field of a `size` parameter to get auto-sized height.
This call will create a scrolled box 80 characters wide and a height dependent upon the number of lines of text.
`sg.popup_scrolled(my_text, size=(80, None))`
Note that the default max number of lines before scrolling happens is set to 50. At 50 lines the scrolling will begin.
If `non_blocking` parameter is set, then the call will not blocking waiting for the user to close the window. Execution will immediately return to the user. Handy when you want to dump out debug info without disrupting the program flow.
### Non-Blocking Popups - popup_no_wait and the non_blocking parameter
Show Popup window and immediately return (does not block)
```
popup_no_wait(args=*<1 or N object>,
title = None,
button_type = 0,
button_color = None,
background_color = None,
text_color = None,
auto_close = False,
auto_close_duration = None,
non_blocking = True,
icon = None,
line_width = None,
font = None,
no_titlebar = False,
grab_anywhere = False,
keep_on_top = None,
location = (None, None),
relative_location = (None, None),
image = None,
modal = False)
```
Parameter Descriptions:
|Type|Name|Meaning|
|--|--|--|
| Any | *args | Variable number of items to display |
| str | title | Title to display in the window. |
| int | button_type | Determines which pre-defined buttons will be shown (Default value = POPUP_BUTTONS_OK). |
| (str, str) or str | button_color | button color (foreground, background) |
| str | background_color | color of background |
| str | text_color | color of the text |
| bool | auto_close | if True window will close itself |
| int or float | auto_close_duration | Older versions only accept int. Time in seconds until window will close |
| bool | non_blocking | if True the call will immediately return rather than waiting on user input |
| bytes or str | icon | filename or base64 string to be used for the window's icon |
| int | line_width | Width of lines in characters |
| (str or (str, int[, str]) or None) | font | specifies the font family, size, etc. Tuple or Single string format 'name size styles'. Styles: italic * roman bold normal underline overstrike |
| bool | no_titlebar | If True no titlebar will be shown |
| bool | grab_anywhere | If True: can grab anywhere to move the window (Default = False) |
| (int, int) | location | Location of upper left corner of the window |
| (int, int) | relative_location | (x,y) location relative to the default location of the window, in pixels. Normally the window centers. This location is relative to the location the window would be created. Note they can be negative. |
| str or bytes | image | Image to include at the top of the popup window |
| bool | modal | If True then makes the popup will behave like a Modal window... all other windows are non-operational until this one is closed. Default = False |
| str or None | **RETURN** | Reason for popup closing
The `popup` call `popup_no_wait` or `popup_non_blocking` will create a popup window and then immediately return control back to you. You can turn other popup calls into non-blocking popups if they have a `non_blocking` parameter. Setting `non_blocking` to True will cause the function to return immediately rather than waiting for the window to be closed.
This function is very handy for when you're **debugging** and want to display something as output but don't want to change the programs's overall timing by blocking. Think of it like a `print` statement. There are no return values on one of these Popups.
### Popup Parameter Combinations
So that you don't have to specify a potentially long list common parameters there are a number of popup functions that set combinations of parameters. For example `popup_quick_message` will show a non-blocking popup that autocloses and does not have a titlebar. You could achieve this same end result using the plain `popup` call.
## Popup Input
There are Popup calls for single-item inputs. These follow the pattern of `popup_get` followed by the type of item to get. There are 3 of these input Popups to choose from, each with settings enabling customization.
- `popup_get_text` - get a single line of text
- `popup_get_file` - get a filename
- `popup_get_folder` - get a folder name
Use these Popups instead of making a custom window to get one data value, call the Popup input function to get the item from the user. If you find the parameters are unable to create the kind of window you are looking for, then it's time for you to create your own window.
### popup_get_text
Use this Popup to get a line of text from the user.
Display Popup with text entry field. Returns the text entered or None if closed / cancelled
```
popup_get_text(message,
title = None,
default_text = "",
password_char = "",
size = (None, None),
button_color = None,
background_color = None,
text_color = None,
icon = None,
font = None,
no_titlebar = False,
grab_anywhere = False,
keep_on_top = None,
location = (None, None),
relative_location = (None, None),
image = None,
history = False,
history_setting_filename = None,
modal = True)
```
Parameter Descriptions:
|Type|Name|Meaning|
|--|--|--|
| str | message | message displayed to user |
| str | title | Window title |
| str | default_text | default value to put into input area |
| str | password_char | character to be shown instead of actually typed characters. WARNING - if history=True then can't hide passwords |
| (int, int) | size | (width, height) of the InputText Element |
| (str, str) or str | button_color | Color of the button (text, background) |
| str | background_color | background color of the entire window |
| str | text_color | color of the message text |
| bytes or str | icon | filename or base64 string to be used for the window's icon |
| (str or (str, int[, str]) or None) | font | specifies the font family, size, etc. Tuple or Single string format 'name size styles'. Styles: italic * roman bold normal underline overstrike |
| bool | no_titlebar | If True no titlebar will be shown |
| bool | grab_anywhere | If True can click and drag anywhere in the window to move the window |
| bool | keep_on_top | If True the window will remain above all current windows |
| (int, int) | location | (x,y) Location on screen to display the upper left corner of window |
| (int, int) | relative_location | (x,y) location relative to the default location of the window, in pixels. Normally the window centers. This location is relative to the location the window would be created. Note they can be negative. |
| str or bytes | image | Image to include at the top of the popup window |
| bool | history | If True then enable a "history" feature that will display previous entries used. Uses settings filename provided or default if none provided |
| str | history_setting_filename | Filename to use for the User Settings. Will store list of previous entries in this settings file |
| bool | modal | If True then makes the popup will behave like a Modal window... all other windows are non-operational until this one is closed. Default = True |
| str or None | **RETURN** | Text entered or None if window was closed or cancel button clicked
```python
import PySimpleGUI as sg
text = sg.popup_get_text('Title', 'Please input something')
sg.popup('Results', 'The value returned from PopupGetText', text)
```
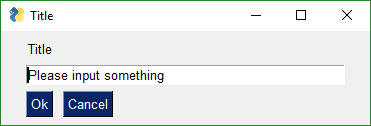
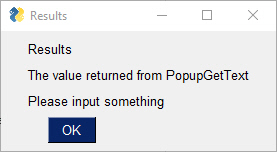
### popup_get_file
Gets one or more filenames from the user. There are options to configure the type of dialog box to show. Normally an "Open File" dialog box is shown.
Display popup window with text entry field and browse button so that a file can be chosen by user.
```
popup_get_file(message,
title = None,
default_path = "",
default_extension = "",
save_as = False,
multiple_files = False,
file_types = (('ALL Files', '*.* *'),),
no_window = False,
size = (None, None),
button_color = None,
background_color = None,
text_color = None,
icon = None,
font = None,
no_titlebar = False,
grab_anywhere = False,
keep_on_top = None,
location = (None, None),
relative_location = (None, None),
initial_folder = None,
image = None,
files_delimiter = ";",
modal = True,
history = False,
show_hidden = True,
history_setting_filename = None)
```
Parameter Descriptions:
|Type|Name|Meaning|
|--|--|--|
| str | message | message displayed to user |
| str | title | Window title |
| str | default_path | path to display to user as starting point (filled into the input field) |
| str | default_extension | If no extension entered by user, add this to filename (only used in saveas dialogs) |
| bool | save_as | if True, the "save as" dialog is shown which will verify before overwriting |
| bool | multiple_files | if True, then allows multiple files to be selected that are returned with ';' between each filename |
| Tuple[Tuple[str,str]] | file_types | List of extensions to show using wildcards. All files (the default) = (("ALL Files", "*.* *"),). |
| bool | no_window | if True, no PySimpleGUI window will be shown. Instead just the tkinter dialog is shown |
| (int, int) | size | (width, height) of the InputText Element or Combo element if using history feature |
| (str, str) or str | button_color | Color of the button (text, background) |
| str | background_color | background color of the entire window |
| str | text_color | color of the text |
| bytes or str | icon | filename or base64 string to be used for the window's icon |
| (str or (str, int[, str]) or None) | font | specifies the font family, size, etc. Tuple or Single string format 'name size styles'. Styles: italic * roman bold normal underline overstrike |
| bool | no_titlebar | If True no titlebar will be shown |
| bool | grab_anywhere | If True: can grab anywhere to move the window (Default = False) |
| bool | keep_on_top | If True the window will remain above all current windows |
| (int, int) | location | Location of upper left corner of the window |
| (int, int) | relative_location | (x,y) location relative to the default location of the window, in pixels. Normally the window centers. This location is relative to the location the window would be created. Note they can be negative. |
| str | initial_folder | location in filesystem to begin browsing |
| str or bytes | image | Image to include at the top of the popup window |
| str | files_delimiter | String to place between files when multiple files are selected. Normally a ; |
| bool | modal | If True then makes the popup will behave like a Modal window... all other windows are non-operational until this one is closed. Default = True |
| bool | history | If True then enable a "history" feature that will display previous entries used. Uses settings filename provided or default if none provided |
| bool | show_hidden | If True then enables the checkbox in the system dialog to select hidden files to be shown |
| str | history_setting_filename | Filename to use for the User Settings. Will store list of previous entries in this settings file |
| str or None | **RETURN** | string representing the file(s) chosen, None if cancelled or window closed with X
If configured as an Open File Popup then (save_as is not True) the dialog box will look like this.
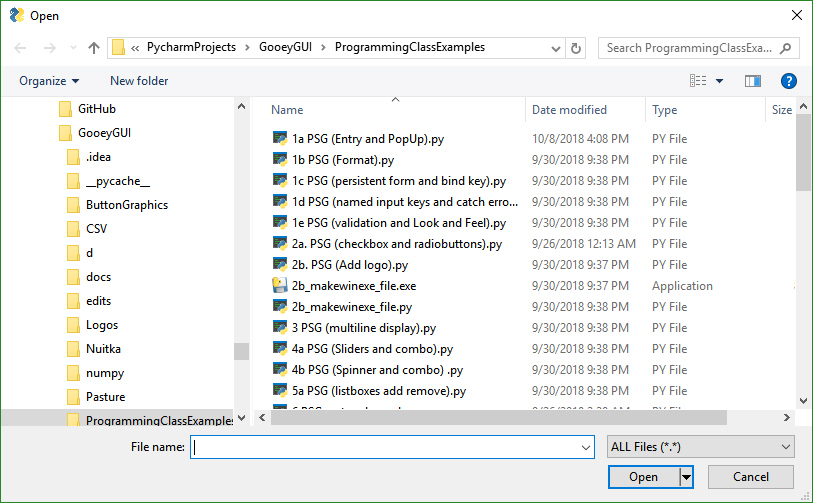
If you set the parameter save_As to True, then the dialog box looks like this:
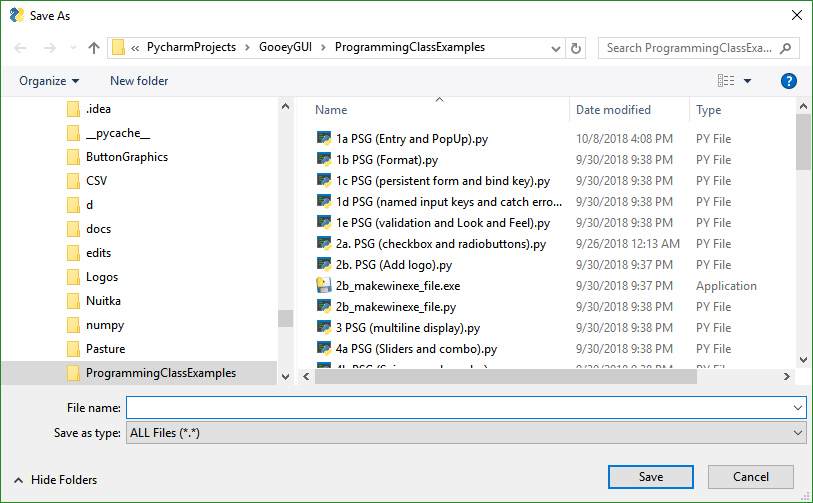
If you choose a filename that already exists, you'll get a warning popup box asking if it's OK. You can also specify a file that doesn't exist. With an "Open" dialog box you cannot choose a non-existing file.
A typical call produces this window.
```python
text = sg.popup_get_file('Please enter a file name')
sg.popup('Results', 'The value returned from popup_get_file', text)
```
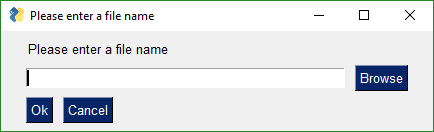
### popup_get_folder
The window created to get a folder name looks the same as the get a file name. The difference is in what the browse button does. `popup_get_file` shows an Open File dialog box while `popup_get_folder` shows an Open Folder dialog box.
Display popup with text entry field and browse button so that a folder can be chosen.
```
popup_get_folder(message,
title = None,
default_path = "",
no_window = False,
size = (None, None),
button_color = None,
background_color = None,
text_color = None,
icon = None,
font = None,
no_titlebar = False,
grab_anywhere = False,
keep_on_top = None,
location = (None, None),
relative_location = (None, None),
initial_folder = None,
image = None,
modal = True,
history = False,
history_setting_filename = None)
```
Parameter Descriptions:
|Type|Name|Meaning|
|--|--|--|
| str | message | message displayed to user |
| str | title | Window title |
| str | default_path | path to display to user as starting point (filled into the input field) |
| bool | no_window | if True, no PySimpleGUI window will be shown. Instead just the tkinter dialog is shown |
| (int, int) | size | (width, height) of the InputText Element |
| (str, str) or str | button_color | button color (foreground, background) |
| str | background_color | color of background |
| str | text_color | color of the text |
| bytes or str | icon | filename or base64 string to be used for the window's icon |
| (str or (str, int[, str]) or None) | font | specifies the font family, size, etc. Tuple or Single string format 'name size styles'. Styles: italic * roman bold normal underline overstrike |
| bool | no_titlebar | If True no titlebar will be shown |
| bool | grab_anywhere | If True: can grab anywhere to move the window (Default = False) |
| bool | keep_on_top | If True the window will remain above all current windows |
| (int, int) | location | Location of upper left corner of the window |
| (int, int) | relative_location | (x,y) location relative to the default location of the window, in pixels. Normally the window centers. This location is relative to the location the window would be created. Note they can be negative. |
| str | initial_folder | location in filesystem to begin browsing |
| str or bytes | image | Image to include at the top of the popup window |
| bool | modal | If True then makes the popup will behave like a Modal window... all other windows are non-operational until this one is closed. Default = True |
| bool | history | If True then enable a "history" feature that will display previous entries used. Uses settings filename provided or default if none provided |
| str | history_setting_filename | Filename to use for the User Settings. Will store list of previous entries in this settings file |
| str or None | **RETURN** | string representing the path chosen, None if cancelled or window closed with X
This is a typical call
```python
text = sg.popup_get_folder('Please enter a folder name')
sg.popup('Results', 'The value returned from popup_get_folder', text)
```
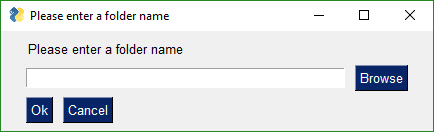
### popup_animated

The animated Popup enables you to easily display a "loading" style animation specified through a GIF file that is either stored in a file or a base64 variable.
Show animation one frame at a time. This function has its own internal clocking meaning you can call it at any frequency
and the rate the frames of video is shown remains constant. Maybe your frames update every 30 ms but your
event loop is running every 10 ms. You don't have to worry about delaying, just call it every time through the
loop.
```
popup_animated(image_source,
message = None,
background_color = None,
text_color = None,
font = None,
no_titlebar = True,
grab_anywhere = True,
keep_on_top = True,
location = (None, None),
relative_location = (None, None),
alpha_channel = None,
time_between_frames = 0,
transparent_color = None,
title = "",
icon = None)
```
Parameter Descriptions:
|Type|Name|Meaning|
|--|--|--|
| str or bytes or None | image_source | Either a filename or a base64 string. Use None to close the window. |
| str | message | An optional message to be shown with the animation |
| str | background_color | color of background |
| str | text_color | color of the text |
| str or tuple | font | specifies the font family, size, etc. Tuple or Single string format 'name size styles'. Styles: italic * roman bold normal underline overstrike |
| bool | no_titlebar | If True then the titlebar and window frame will not be shown |
| bool | grab_anywhere | If True then you can move the window just clicking anywhere on window, hold and drag |
| bool | keep_on_top | If True then Window will remain on top of all other windows currently shownn |
| (int, int) | location | (x,y) location on the screen to place the top left corner of your window. Default is to center on screen |
| (int, int) | relative_location | (x,y) location relative to the default location of the window, in pixels. Normally the window centers. This location is relative to the location the window would be created. Note they can be negative. |
| float | alpha_channel | Window transparency 0 = invisible 1 = completely visible. Values between are see through |
| int | time_between_frames | Amount of time in milliseconds between each frame |
| str | transparent_color | This color will be completely see-through in your window. Can even click through |
| str | title | Title that will be shown on the window |
| str or bytes | icon | Same as Window icon parameter. Can be either a filename or Base64 byte string. For Windows if filename, it MUST be ICO format. For Linux, must NOT be ICO |
| bool | **RETURN** | True if the window updated OK. False if the window was closed
***To close animated popups***, call PopupAnimated with `image_source=None`. This will close all of the currently open PopupAnimated windows.
# Progress Meters!
We all have loops in our code. 'Isn't it joyful waiting, watching a counter scrolling past in a text window? How about one line of code to get a progress meter, that contains statistics about your code?
```
one_line_progress_meter(title,
current_value,
max_value,
args=*<1 or N object>,
key = "OK for 1 meter",
orientation = "v",
bar_color = (None, None),
button_color = None,
size = (20, 20),
border_width = None,
grab_anywhere = False,
no_titlebar = False,
keep_on_top = None,
no_button = False)
```
Parameter Descriptions:
|Type|Name|Meaning|
|--|--|--|
| str | title | text to display in titlebar of window |
| int | current_value | current value |
| int | max_value | max value of progress meter |
| Any | *args | stuff to output as text in the window along with the meter |
| str or int or tuple or object | key | Used to differentiate between multiple meters. Used to cancel meter early. Now optional as there is a default value for single meters |
| str | orientation | 'horizontal' or 'vertical' ('h' or 'v' work) (Default value = 'vertical' / 'v') |
| (str, str) or str | bar_color | The 2 colors that make up a progress bar. Either a tuple of 2 strings or a string. Tuple - (bar, background). A string with 1 color changes the background of the bar only. A string with 2 colors separated by "on" like "red on blue" specifies a red bar on a blue background. |
| (str, str) or str | button_color | button color (foreground, background) |
| (int, int) | size | (w,h) w=characters-wide, h=rows-high (Default value = DEFAULT_PROGRESS_BAR_SIZE) |
| int | border_width | width of border around element |
| bool | grab_anywhere | If True: can grab anywhere to move the window (Default = False) |
| bool | no_titlebar | If True: no titlebar will be shown on the window |
| bool | keep_on_top | If True the window will remain above all current windows |
| bool | no_button | If True: window will be created without a cancel button |
| (bool) | **RETURN** | True if updated successfully. False if user closed the meter with the X or Cancel button
Here's the one-line Progress Meter in action!
```python
for i in range(1,10000):
sg.one_line_progress_meter('My Meter', i+1, 10000, 'key','Optional message')
```
That line of code resulted in this window popping up and updating.
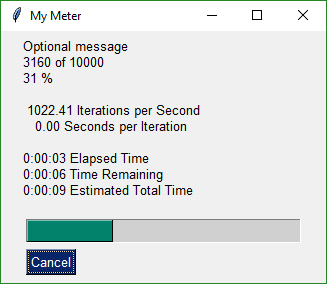
A meter AND fun statistics to watch while your machine grinds away, all for the price of 1 line of code.
With a little trickery you can provide a way to break out of your loop using the Progress Meter window. The cancel button results in a `False` return value from `one_line_progress_meter`. It normally returns `True`.
***Be sure and add one to your loop counter*** so that your counter goes from 1 to the max value. If you do not add one, your counter will never hit the max value. Instead it will go from 0 to max-1.
# Debug Output (easy_print = Print = eprint)
Another call in the 'Easy' families of APIs is `EasyPrint`. As is with other commonly used PySimpleGUI calls, there are other names for the same call. You can use `Print` or `eprint` in addition to `EasyPrint`. They all do the same thing, output to a debug window. If the debug window isn't open, then the first call will open it. No need to do anything but stick an 'sg.Print' call in your code. You can even replace your 'print' calls with calls to EasyPrint by simply sticking the statement
```python
print = sg.Print
```
at the top of your code.
`Print` is one of the better ones to use as it's easy to remember. It is simply `print` with a capital P. `sg.Print('this will go to the debug window')`
```python
import PySimpleGUI as sg
for i in range(100):
sg.Print(i)
```
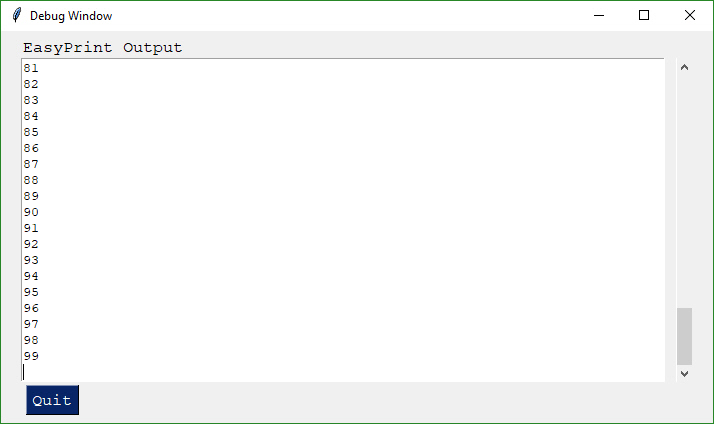
Or if you didn't want to change your code:
```python
import PySimpleGUI as sg
print=sg.Print
for i in range(100):
print(i)
```
Just like the standard print call, `easy_print` supports the `sep` and `end` keyword arguments. Other names that can be used to call `easy_print` include `Print`, `eprint`, If you want to close the window, call the function `easy_print_close`.
You can change the size of the debug window using the `set_options` call with the `debug_win_size` parameter.
There is an option to tell PySimpleGUI to reroute all of your stdout and stderr output to this window. To do so call easy_print with the parameter `do_not_reroute_stdout` set to `False`. After calling it once with this parameter set to True, all future calls to a normal `print` will go to the debug window.
If you close the debug window it will re-open the next time you Print to it. If you wish to close the window using your code, then you can call either `easy_print_close()` or `PrintClose()`
### Printing To Multiline Elements
Another technique for outputting information that you would normally print is to use the function `Multiline.print`. You'll find it discussed further into this document. The basic idea is that you can easily modify your normal `print` calls to route your printed information to your window.
---
# Custom window API Calls (Your First window)
This is the FUN part of the programming of this GUI. In order to really get the most out of the API, you should be using an IDE that supports auto complete or will show you the definition of the function. This will make customizing go smoother.
This first section on custom windows is for your typical, blocking, non-persistent window. By this I mean, when you "show" the window, the function will not return until the user has clicked a button or closed the window with an X.
Two other types of windows exist.
1. Persistent window - the `Window.read()` method returns and the window continues to be visible. This is good for applications like a chat window or a timer or anything that stays active on the screen for a while.
2. Asynchronous window - the trickiest of the lot. Great care must be exercised. Examples are an MP3 player or status dashboard. Async windows are updated (refreshed) on a periodic basis. You can spot them easily as they will have a `timeout` parameter on the call to read. `event, values = window.read(timeout=100)`
It's both not enjoyable nor helpful to immediately jump into tweaking each and every little thing available to you. Make some simple windows. Use the Cookbook and the Demo Programs as a way to learn and as a "starting point".
## The window Designer
The good news to newcomers to GUI programming is that PySimpleGUI has a window designer. Better yet, the window designer requires no training, no downloads, and everyone knows how to use it.
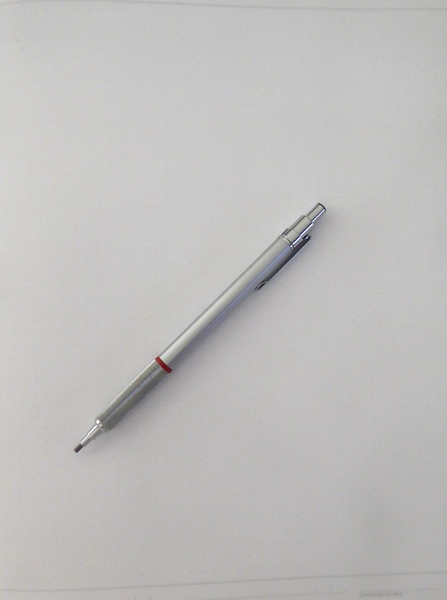
It's a manual process, but if you follow the instructions, it will take only a minute to do and the result will be a nice looking GUI. The steps you'll take are:
1. Sketch your GUI on paper
2. Divide your GUI up into rows
3. Label each Element with the Element name
4. Write your Python code using the labels as pseudo-code
Let's take a couple of examples.
**Enter a number**.... Popular beginner programs are often based on a game or logic puzzle that requires the user to enter something, like a number. The "high-low" answer game comes to mind where you try to guess the number based on high or low tips.
**Step 1- Sketch the GUI**
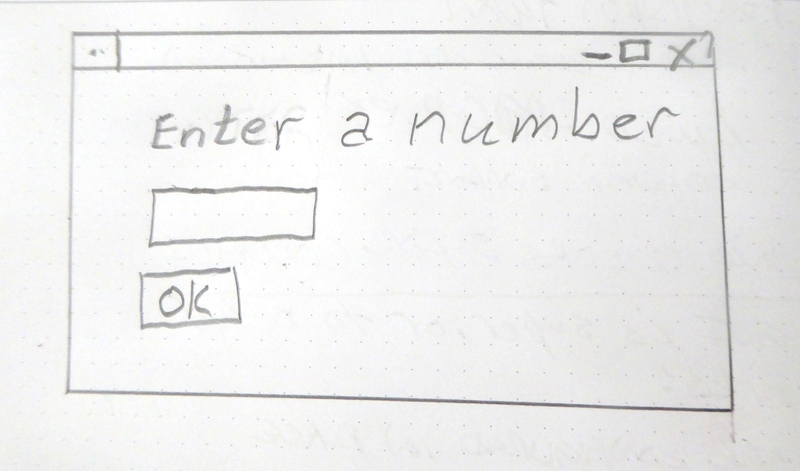
**Step 2 - Divide into rows**
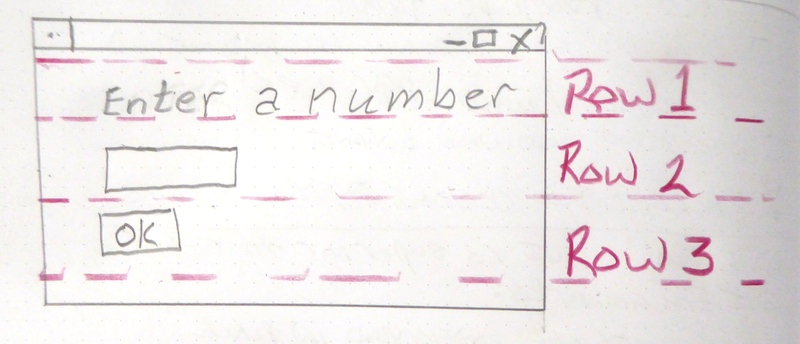
Step 3 - Label elements
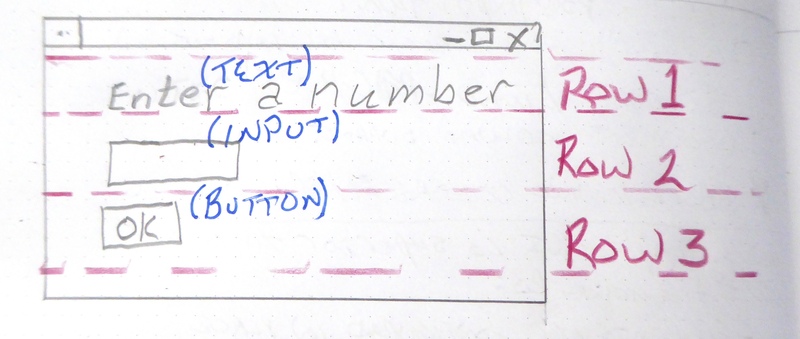
Step 4 - Write the code
The code we're writing is the layout of the GUI itself. This tutorial only focuses on getting the window code written, not the stuff to display it, get results.
We have only 1 element on the first row, some text. Rows are written as a "list of elements", so we'll need [ ] to make a list. Here's the code for row 1
```
[ sg.Text('Enter a number') ]
```
Row 2 has 1 elements, an input field.
```
[ sg.Input() ]
```
Row 3 has an OK button
```
[ sg.OK() ]
```
Now that we've got the 3 rows defined, they are put into a list that represents the entire window.
```
layout = [ [sg.Text('Enter a Number')],
[sg.Input()],
[sg.OK()] ]
```
Finally we can put it all together into a program that will display our window.
```python
import PySimpleGUI as sg
layout = [[sg.Text('Enter a Number')],
[sg.Input()],
[sg.OK()] ]
window = sg.Window('Enter a number example', layout)
event, values = window.read()
window.close()
sg.Popup(event, values[0])
```
Your call to `read` will normally return a dictionary, but will "look like a list" in how you access it. The first input field will be entry 0, the next one is 1, etc.. Later you'll learn about the `key` parameter which allows you to use your own values to identify elements instead of them being numbered for you.
### Example 2 - Get a filename
Let's say you've got a utility you've written that operates on some input file and you're ready to use a GUI to enter than filename rather than the command line. Follow the same steps as the previous example - draw your window on paper, break it up into rows, label the elements.
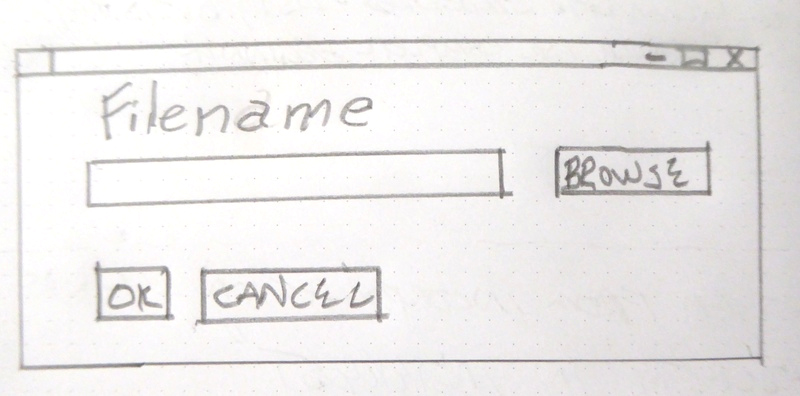

Writing the code for this one is just as straightforward. There is one tricky thing, that browse for a file button. Thankfully PySimpleGUI takes care of associating it with the input field next to it. As a result, the code looks almost exactly like the window on the paper.
```python
import PySimpleGUI as sg
sg.theme('Dark Blue 3') # please make your windows colorful
layout = [[sg.Text('Filename')],
[sg.Input(), sg.FileBrowse()],
[sg.OK(), sg.Cancel()] ]
window = sg.Window('Get filename example', layout)
event, values = window.read()
window.close()
sg.Popup(event, values[0])
```
Read on for detailed instructions on the calls that show the window and return your results.
# Copy these design patterns!
All of your PySimpleGUI programs will utilize one of these 2 design patterns depending on the type of window you're implementing.
Beginning in release 4.19.0 the constant WIN_CLOSED replaced using `None` as the event signaling that a window was closed.
## Pattern 1 A - "One-shot Window" - Read a window one time then close it
This will be the most common pattern you'll follow if you are not using an "event loop" (not reading the window multiple times). The window is read and closed.
The input fields in your window will be returned to you as a dictionary (syntactically it looks just like a list lookup)
```python
import PySimpleGUI as sg
sg.theme('Dark Blue 3') # please make your windows colorful
layout = [[sg.Text('SHA-1 and SHA-256 Hashes for the file')],
[sg.InputText(), sg.FileBrowse()],
[sg.Submit(), sg.Cancel()]]
window = sg.Window('SHA-1 & 256 Hash', layout)
event, values = window.read()
window.close()
source_filename = values[0] # the first input element is values[0]
```
## Pattern 1 B - "One-shot Window" - Read a window one time then close it (compact format)
Same as Pattern 1, but done in a highly compact way. This example uses the `close` parameter in `window.read` to automatically close the window as part of the read operation (new in version 4.16.0). This enables you to write a single line of code that will create, display, gather input and close a window. It's really powerful stuff!
```python
import PySimpleGUI as sg
sg.theme('Dark Blue 3') # please make your windows colorful
event, values = sg.Window('SHA-1 & 256 Hash', [[sg.Text('SHA-1 and SHA-256 Hashes for the file')],
[sg.InputText(), sg.FileBrowse()],
[sg.Submit(), sg.Cancel()]]).read(close=True)
source_filename = values[0] # the first input element is values[0]
```
## Pattern 2 A - Persistent window (multiple reads using an event loop)
Some of the more advanced programs operate with the window remaining visible on the screen. Input values are collected, but rather than closing the window, it is kept visible acting as a way to both output information to the user and gather input data.
This code will present a window and will print values until the user clicks the exit button or closes window using an X.
```python
import PySimpleGUI as sg
sg.theme('Dark Blue 3') # please make your windows colorful
layout = [[sg.Text('Persistent window')],
[sg.Input()],
[sg.Button('Read'), sg.Exit()]]
window = sg.Window('Window that stays open', layout)
while True:
event, values = window.read()
if event == sg.WIN_CLOSED or event == 'Exit':
break
print(event, values)
window.close()
```
## Pattern 2 B - Persistent window (multiple reads using an event loop + updates data in window)
This is a slightly more complex, but maybe more realistic version that reads input from the user and displays that input as text in the window. Your program is likely to be doing both of those activities (input and output) so this will give you a big jump-start.
Do not worry yet what all of these statements mean. Just copy it so you can begin to play with it, make some changes. Experiment to see how thing work.
This example introduces the concept of "keys". Keys are super important in PySimpleGUI as they enable you to identify and work with Elements using names you want to use. Keys can be (almost) ANYTHING, except `None` or a List (a tuple is fine). To access an input element's data that is read in the example below, you will use `values['-IN-']` instead of `values[0]` like before.
```python
import PySimpleGUI as sg
sg.theme('Dark Blue 3') # please make your windows colorful
layout = [[sg.Text('Your typed chars appear here:'), sg.Text(size=(12,1), key='-OUTPUT-')],
[sg.Input(key='-IN-')],
[sg.Button('Show'), sg.Button('Exit')]]
window = sg.Window('Window Title', layout)
while True: # Event Loop
event, values = window.read()
print(event, values)
if event == sg.WIN_CLOSED or event == 'Exit':
break
if event == 'Show':
# change the "output" element to be the value of "input" element
window['-OUTPUT-'].update(values['-IN-'])
window.close()
```
### Qt Designer
There actually is a PySimpleGUI Window Designer that uses Qt's window designer. It's outside the scope of this document however. You'll find the project here: https://github.com/nngogol/PySimpleGUIDesigner
I hope to start using it more soon.
## How GUI Programming in Python Should Look? At least for beginners ?
While one goal was making it simple to create a GUI another just as important goal was to do it in a Pythonic manner. Whether it achieved these goals is debatable, but it was an attempt just the same.
The key to custom windows in PySimpleGUI is to view windows as ROWS of GUI Elements. Each row is specified as a list of these Elements. Put the rows together and you've got a window. This means the GUI is defined as a series of Lists, a Pythonic way of looking at things.
### Let's dissect this little program
```python
import PySimpleGUI as sg
sg.theme('Dark Blue 3') # please make your windows colorful
layout = [[sg.Text('Rename files or folders')],
[sg.Text('Source for Folders', size=(15, 1)), sg.InputText(), sg.FolderBrowse()],
[sg.Text('Source for Files ', size=(15, 1)), sg.InputText(), sg.FileBrowse()],
[sg.Submit(), sg.Cancel()]]
window = sg.Window('Rename Files or Folders', layout)
event, values = window.read()
window.close()
folder_path, file_path = values[0], values[1] # get the data from the values dictionary
print(folder_path, file_path)
```
### Themes
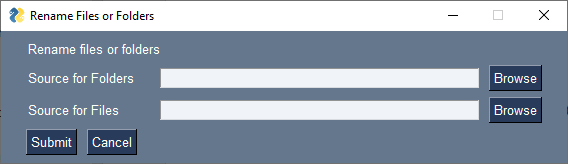
The first line of code after the import is a call to `theme`.
Until Dec 2019 the way a "theme" was specific in PySimpleGUI was to call `change_look_and_feel`. That call has been replaced by the more simple function `theme`.
### Window contents (The Layout)
Let's agree the window has 4 rows.
The first row only has **text** that reads `Rename files or folders`
The second row has 3 elements in it. First the **text** `Source for Folders`, then an **input** field, then a **browse** button.
Now let's look at how those 2 rows and the other two row from Python code:
```python
layout = [[sg.Text('Rename files or folders')],
[sg.Text('Source for Folders', size=(15, 1)), sg.InputText(), sg.FolderBrowse()],
[sg.Text('Source for Files ', size=(15, 1)), sg.InputText(), sg.FileBrowse()],
[sg.Submit(), sg.Cancel()]]
```
See how the source code mirrors the layout? You simply make lists for each row, then submit that table to PySimpleGUI to show and get values from.
And what about those return values? Most people simply want to show a window, get the input values and do something with them. So why break up the code into button callbacks, etc., when I simply want my window's input values to be given to me.
For return values the window is scanned from top to bottom, left to right. Each field that's an input field will occupy a spot in the return values.
In our example window, there are 2 fields, so the return values from this window will be a dictionary with 2 values in it. Remember, if you do not specify a `key` when creating an element, one will be created for you. They are ints starting with 0. In this example, we have 2 input elements. They will be addressable as values[0] and values[1]
### "Reading" the window's values (also displays the window)
```python
event, values = window.read()
folder_path, file_path = values[0], values[1]
```
In one statement we both show the window and read the user's inputs. In the next line of code the *dictionary* of return values is split into individual variables `folder_path` and `file_path`.
Isn't this what a Python programmer looking for a GUI wants? Something easy to work with to get the values and move on to the rest of the program, where the real action is taking place. Why write pages of GUI code when the same layout can be achieved with PySimpleGUI in 3 or 4 lines of code. 4 lines or 40? Most would choose 4.
## Return values
There are 2 return values from a call to `Window.read()`, an `event` that caused the `Read` to return and `values` a list or dictionary of values. If there are no elements with keys in the layout, then it will be a list. However, some elements, like some buttons, have a key automatically added to them. **It's best to use keys on all of your input type elements.**
### Two Return Values
All Window Read calls return 2 values. By convention a read statement is written:
```python
event, values = window.read()
```
You don't HAVE to write your reads in this way. You can name your variables however you want. But if you want to code them in a way that other programmers using PySimpleGUI are used to, then use this statement.
## Events
The first parameter `event` describes **why** the read completed. Events are one of these:
For all Windows:
* Button click
* Window closed using X
For Windows that have specifically enabled these. Please see the appropriate section in this document to learn about how to enable these and what the event return values are.
* Keyboard key press
* Mouse wheel up/down
* Menu item selected
* An Element Changed (slider, spinner, etc.)
* A list item was clicked
* Return key was pressed in input element
* Timeout waiting for event
* Text was clicked
* Combobox item chosen
* Table row selected
* etc.
***Most*** of the time the event will be a button click or the window was closed. The other Element-specific kinds of events happen when you set `enable_events=True` when you create the Element.
### Button Click Events
By default buttons will always return a click event, or in the case of realtime buttons, a button down event. You don't have to do anything to enable button clicks. To disable the events, disable the button using its Update method.
You can enable an additional "Button Modified" event by setting `enable_events=True` in the Button call. These events are triggered when something 'writes' to a button, ***usually*** it's because the button is listed as a "target" in another button.
The button value from a Read call will be one of 2 values:
1. The Button's text - Default
2. The Button's key - If a key is specified
If a button has a key set when it was created, then that key will be returned, regardless of what text is shown on the button. If no key is set, then the button text is returned. If no button was clicked, but the window returned anyway, the event value is the key that caused the event to be generated. For example, if `enable_events` is set on an `Input` Element and someone types a character into that `Input` box, then the event will be the key of the input box.
### Element Events
Some elements are capable of generating events when something happens to them. For example, when a slider is moved, or list item clicked on or table row clicked on. These events are not enabled by default. To enable events for an Element, set the parameter `enable_events=True`. This is the same as the older `click_submits` parameter. You will find the `click_submits` parameter still in the function definition. You can continue to use it. They are the same setting. An 'or' of the two values is used. In the future, click_submits will be removed so please migrate your code to using `enable_events`.
|Name|events|
| --- | --- |
| InputText | any change |
| Combo | item chosen |
| Listbox | selection changed |
| Radio | selection changed |
| Checkbox | selection changed |
| Spinner | new item selected |
| Multiline | any change |
| Text | clicked |
| Status Bar | clicked |
| Graph | clicked |
| Graph | dragged |
| Graph | drag ended (mouse up) |
| TabGroup | tab clicked |
| Slider | slider moved |
| Table | row selected |
| Tree | node selected |
| ButtonMenu | menu item chosen |
| Right click menu | menu item chosen |
### Other Events
#### Menubar menu item chosen for MenuBar menus and ButtonMenu menus
You will receive the key for the MenuBar and ButtonMenu. Use that key to read the value in the return values dictionary. The value shown will be the full text plus key for the menu item chosen. Remember that you can put keys onto menu items. You will get the text and the key together as you defined it in the menu
definition.
#### Right Click menu item chosen
Unlike menu bar and button menus, you will directly receive the menu item text and its key value. You will not do a dictionary lookup to get the value. It is the event code returned from WindowRead().
#### Windows - keyboard, mouse scroll wheel
Windows are capable of returning keyboard events. These are returned as either a single character or a string if it's a special key. Experiment is all I can say. The mouse scroll wheel events are also strings. Put a print in your code to see what's returned.
#### Timeouts
If you set a timeout parameter in your read, then the system TIMEOUT_KEY will be returned. If you specified your own timeout key in the Read call then that value will be what's returned instead.
## Window Closed Events
Detecting and correctly handling Windows being closed is an important part of your PySimpleGUI application. You will find in every event loop in every Demo Program an if statement that checks for the events that signal that a window has closed.
The most obvious way to close a window is to click the "X" in the upper right corner of the window (on Windows, Linux.... Mac doesn't use an "X" but still has a close button). On Windows systems, the keyboard keys ALT+F4 will force a Window to close. This is one way to close a window without using a mouse. Some programs can also send a "close" command to the window.
Regardless of how the close is performed on the window, PySimpleGUI returns an event for this closure.
### WIN_CLOSED Event
**The constant WIN_CLOSED (None) is returned when the user clicks the X to close a window.**
Typically, the check for a closed window happens right after the `window.read()` call returns. The reason for this is that operating on a closed window can result in errors. The check for closure is an "if" statement.
**ALWAYS** include a check for a closed window in your event loop.
### The Window Closed If Statement
There are 2 forms you'll find for this if statement in the documentation and the Demo Programs. One is "Pythonic" the other is more understandable by beginners. This is the format you'll see most often in the PySimpleGUI materials if the window has both a button that is used to signal the user wishes to exit. In this example, I'm using a "Quit" button:
```python
if event == sg.WIN_CLOSED or event == 'Exit':
break
```
The more "Pythonic" version of this same statement is:
```python
if event in (sg.WIN_CLOSED, 'Exit'):
break
```
In case you're yelling at your screen right now that the second form should always be used, remember that many of the PySimpleGUI users are new to Python. If the very first example of a PySimpleGUI program they see has that if statement in it, they will instantly be lost before they can even begin their journey. So, the decision was made to, you guessed it, go SIMPLE. The statement with the "or" is simpler to understand.
This notion of binary choices in programming that's crept in over the past couple decades... that there's a "BEST" or "only 1 right way"... loses the sophisticated thinking that a software engineer needs to be successful in a wide variety of situations. Every situation, every person, every problem... is unique. **There is no idealized best that always fits.**
### A Complete Example - Traditional Window Closed Check
Let's put this if statement into context so you can see where it goes and how it works with the event loop
```python
import PySimpleGUI as sg
layout = [[sg.Text('Very basic Window')],
[sg.Text('Click X in titlebar or the Exit button')],
[sg.Button('Go'), sg.Button('Exit')]]
window = sg.Window('Window Title', layout)
while True:
event, values = window.read()
print(event, values)
if event == sg.WIN_CLOSED or event == 'Exit':
break
window.close()
```
Notice that the line after the while loop is a call to `window.close()`. The reason for this is that exiting the loop can be in 2 ways.
* The "X" is clicked
* The Exit button is clicked
If the Exit button is clicked, the window will still be open. Get in the habit of closing your windows explicitly like this.
If the user clicked "X" and closed the window, then it will have been destroyed by the underlying framework. You should STILL call `window.close()` because come cleanup work may be needed and there is no harm in closing an already closed window.
tkinter can generate an error/warning sometimes if you don't close the window. For other ports, such as PySimpleGUIWeb, not closing the Window will potentially cause your program to continue to run in the background. This can cause your program to not be visible and yet consuming 100% of the CPU time. Not fun for your users.
### Window Close Confirmation
In 4.33.0 a new parameter, `enable_close_attempted_event`, was added to the `Window` object. This boolean parameter indicates if you would like to receive an event that a user **wants** to close the window rather than an event that the user **has** closed the window.
To enable this feature, the `Window` is created with something like this:
```python
window = sg.Window('Window Title', layout, enable_close_attempted_event=True)
```
When the close attempted feature is enabled, when the user clicks the "X" or types ALT+F4, you will not get a WIN_CLOSED event like previously, you will instead get an event `WINDOW_CLOSE_ATTEMPTED_EVENT` and the window will remain open.
Usually this feature is used to add a "close confirmation" popup. The flow goes something like this:
* Window is shown
* User clicks X
* A popup window is shown with message "Do you really want to close the window?"
* If confirmed a close is desired, the window is closed. It not, the event loop continues on, basically ignoring the event occurred.
### A Complete Example - Window Closed Confirmation (`enable_close_attempted_event=True`)
Returning to the example used above, there has been only 2 modifications.
1. Added the parameter `enable_close_attempted_event=True` to the call to `Window`
2. The if statement in the event loop has changed to add a confirmation
```python
import PySimpleGUI as sg
layout = [[sg.Text('Very basic Window')],
[sg.Text('Click X in titlebar or the Exit button')],
[sg.Button('Go'), sg.Button('Exit')]]
window = sg.Window('Window Title', layout, enable_close_attempted_event=True)
while True:
event, values = window.read()
print(event, values)
if (event == sg.WINDOW_CLOSE_ATTEMPTED_EVENT or event == 'Exit') and sg.popup_yes_no('Do you really want to exit?') == 'Yes':
break
window.close()
```
The event loop changed from a check like this:
```python
if event == sg.WIN_CLOSED or event == 'Exit':
break
```
To one like this:
```python
if (event == sg.WINDOW_CLOSE_ATTEMPTED_EVENT or event == 'Exit') and sg.popup_yes_no('Do you really want to exit?') == 'Yes':
break
```
Let's run this last program so you can see what all this looks like to users.
In both cases that a user previously exited the window, there is now an additional confirmation step.
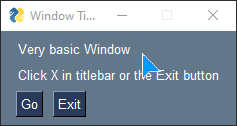
### Demo Programs.... A PySimpleGUI Program's Best Friend
Like all features of PySimpleGUI, one of the best resources available to you to learn about parameters, like this close attempted parameter, are the Demo Programs. When you run into a parameter or a feature you've not used before, one way to find some examples of its use is to use the Demo Browser to search through the demo programs. You'll find the Demo Browser described in the Cookbook.
As of this writing, the name of the Demo Program Browser is:
`Browser_START_HERE_Demo_Programs_Browser.py`
If you enter the parameter described in this section - `enable_close_attempted_event` you'll find a Demo Program that uses this parameter.
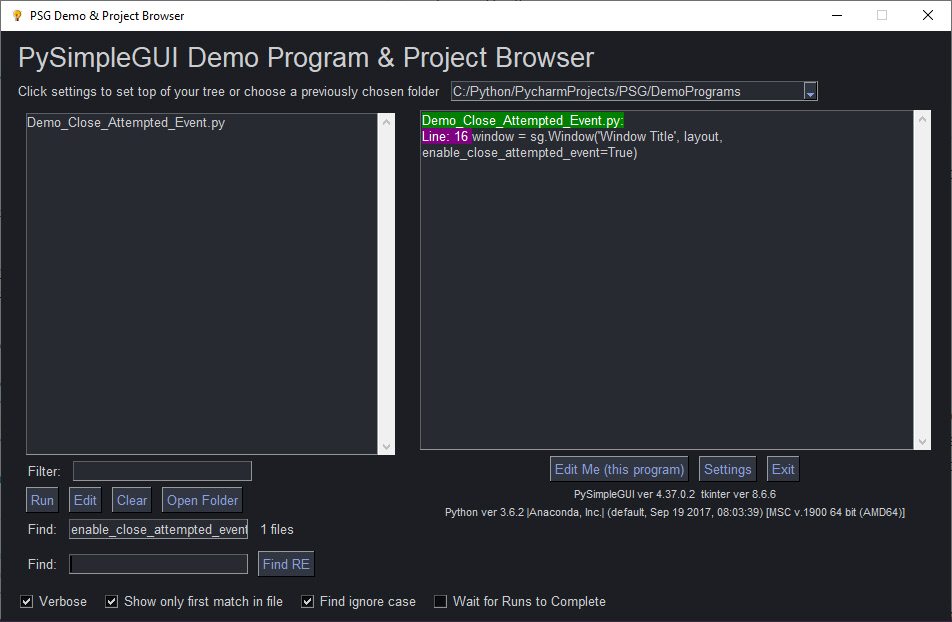
This demo shows you code similar to the code used in this section of the documentation. Use this Browser program! It will make finding examples ***much easier***!
See the section up above on the pip-installable version of the demos (search for - `psgdemos`) to get the super-quick method of getting the demo programs and the Demo Browser.
### The `values` Variable - Return values as a list
The second parameter from a Read call is either a list or a dictionary of the input fields on the Window.
By default return values are a list of values, one entry for each input field, but for all but the simplest of windows the return values will be a dictionary. This is because you are likely to use a 'key' in your layout. When you do, it forces the return values to be a dictionary.
Each of the Elements that are Input Elements will have a value in the list of return values. If you know for sure that the values will be returned as a list, then you could get clever and unpack directly into variables.
event, (filename, folder1, folder2, should_overwrite) = sg.Window('My title', window_rows).read()
Or, more commonly, you can unpack the return results separately. This is the preferred method because it works for **both** list and dictionary return values.
```python
event, values = sg.Window('My title', window_rows).read()
event, value_list = window.read()
value1 = value_list[0]
value2 = value_list[1]
...
```
However, this method isn't good when you have a lot of input fields. If you insert a new element into your window then you will have to shuffle your unpacks down, modifying each of the statements to reference `value_list[x]`.
The more common method is to request your values be returned as a dictionary by placing keys on the "important" elements (those that you wish to get values from and want to interact with)
### `values` Variable - Return values as a dictionary
For those of you that have not encountered a Python dictionary, don't freak out! Just copy and paste the sample code and modify it. Follow this design pattern and you'll be fine. And you might learn something along the way.
For windows longer than 3 or 4 fields you will want to use a dictionary to help you organize your return values. In almost all (if not all) of the demo programs you'll find the return values being passed as a dictionary. It is not a difficult concept to grasp, the syntax is easy to understand, and it makes for very readable code.
The most common window read statement you'll encounter looks something like this:
`window = sg.Window("My title", layout).read()`
To use a dictionary, you will need to:
* Mark each input element you wish to be in the dictionary with the keyword `key`.
If **any** element in the window has a `key`, then **all** of the return values are returned via a dictionary. If some elements do not have a key, then they are numbered starting at zero.
Let's take a look at your first dictionary-based window.
```python
import PySimpleGUI as sg
sg.theme('Dark Blue 3') # please make your windows colorful
layout = [
[sg.Text('Please enter your Name, Address, Phone')],
[sg.Text('Name', size=(15, 1)), sg.InputText('1', key='-NAME-')],
[sg.Text('Address', size=(15, 1)), sg.InputText('2', key='-ADDRESS-')],
[sg.Text('Phone', size=(15, 1)), sg.InputText('3', key='-PHONE-')],
[sg.Submit(), sg.Cancel()]
]
window = sg.Window('Simple data entry window', layout)
event, values = window.read()
window.close()
sg.Popup(event, values, values['-NAME-'], values['-ADDRESS-'], values['-PHONE-'])
```
To get the value of an input field, you use whatever value used as the `key` value as the index value. Thus to get the value of the name field, it is written as
values['-NAME-']
Think of the variable values in the same way as you would a list, however, instead of using 0,1,2, to reference each item in the list, use the values of the key. The Name field in the window above is referenced by `values['-NAME-']`.
You will find the key field used quite heavily in most PySimpleGUI windows unless the window is very simple.
One convention you'll see in many of the demo programs is keys being named in all caps with an underscores at the beginning and the end. You don't HAVE to do this... your key value may look like this:
`key = '-NAME-'`
The reason for this naming convention is that when you are scanning the code, these key values jump out at you. You instantly know it's a key. Try scanning the code above and see if those keys pop out.
`key = '-NAME-'`
## The Event Loop / Callback Functions
All GUIs have one thing in common, an "event loop". Usually the GUI framework runs the event loop for you, but sometimes you want greater control and will run your own event loop. You often hear the term event loop when discussing embedded systems or on a Raspberry Pi.
With PySimpleGUI if your window will remain open following button clicks, then your code will have an event loop. If your program shows a single "one-shot" window, collects the data and then has no other GUI interaction, then you don't need an event loop.
There's nothing mysterious about event loops... they are loops where you take care of.... wait for it..... *events*. Events are things like button clicks, key strokes, mouse scroll-wheel up/down.
This little program has a typical PySimpleGUI Event Loop.
The anatomy of a PySimpleGUI event loop is as follows, *generally speaking*.
* The actual "loop" part is a `while True` loop
* "Read" the event and any input values the window has
* Check to see if window was closed or user wishes to exit
* A series of `if event ....` statements
## All Elements
Here is a complete, short, program that contains *all of the PySimpleGUI Elements*.
```python
import PySimpleGUI as sg
"""
Demo - Element List
All 34 elements shown in 1 window as simply as possible.
Copyright 2022 PySimpleGUI
"""
use_custom_titlebar = False
def make_window(theme=None):
NAME_SIZE = 23
def name(name):
dots = NAME_SIZE-len(name)-2
return sg.Text(name + ' ' + '•'*dots, size=(NAME_SIZE,1), justification='r',pad=(0,0), font='Courier 10')
sg.theme(theme)
treedata = sg.TreeData()
treedata.Insert("", '_A_', 'Tree Item 1', [1234], )
treedata.Insert("", '_B_', 'B', [])
treedata.Insert("_A_", '_A1_', 'Sub Item 1', ['can', 'be', 'anything'], )
layout_l = [[name('Text'), sg.Text('Text')],
[name('Input'), sg.Input(s=15)],
[name('Multiline'), sg.Multiline(s=(15,2))],
[name('Output'), sg.Output(s=(15,2))],
[name('Combo'), sg.Combo(sg.theme_list(), default_value=sg.theme(), s=(15,22), enable_events=True, readonly=True, k='-COMBO-')],
[name('OptionMenu'), sg.OptionMenu(['OptionMenu',],s=(15,2))],
[name('Checkbox'), sg.Checkbox('Checkbox')],
[name('Radio'), sg.Radio('Radio', 1)],
[name('Spin'), sg.Spin(['Spin',], s=(15,2))],
[name('Button'), sg.Button('Button')],
[name('ButtonMenu'), sg.ButtonMenu('ButtonMenu', sg.MENU_RIGHT_CLICK_EDITME_EXIT)],
[name('Slider'), sg.Slider((0,10), orientation='h', s=(10,15))],
[name('Listbox'), sg.Listbox(['Listbox', 'Listbox 2'], no_scrollbar=True, s=(15,2))],
[name('Image'), sg.Image(sg.EMOJI_BASE64_HAPPY_THUMBS_UP)],
[name('Graph'), sg.Graph((125, 50), (0,0), (125,50), k='-GRAPH-')] ]
layout_r = [[name('Canvas'), sg.Canvas(background_color=sg.theme_button_color()[1], size=(125,50))],
[name('ProgressBar'), sg.ProgressBar(100, orientation='h', s=(10,20), k='-PBAR-')],
[name('Table'), sg.Table([[1,2,3], [4,5,6]], ['Col 1','Col 2','Col 3'], num_rows=2)],
[name('Tree'), sg.Tree(treedata, ['Heading',], num_rows=3)],
[name('Horizontal Separator'), sg.HSep()],
[name('Vertical Separator'), sg.VSep()],
[name('Frame'), sg.Frame('Frame', [[sg.T(s=15)]])],
[name('Column'), sg.Column([[sg.T(s=15)]])],
[name('Tab, TabGroup'), sg.TabGroup([[sg.Tab('Tab1',[[sg.T(s=(15,2))]]), sg.Tab('Tab2', [[]])]])],
[name('Pane'), sg.Pane([sg.Col([[sg.T('Pane 1')]]), sg.Col([[sg.T('Pane 2')]])])],
[name('Push'), sg.Push(), sg.T('Pushed over')],
[name('VPush'), sg.VPush()],
[name('Sizer'), sg.Sizer(1,1)],
[name('StatusBar'), sg.StatusBar('StatusBar')],
[name('Sizegrip'), sg.Sizegrip()] ]
layout = [[sg.MenubarCustom([['File', ['Exit']], ['Edit', ['Edit Me', ]]], k='-CUST MENUBAR-',p=0)] if use_custom_titlebar else [sg.Menu([['File', ['Exit']], ['Edit', ['Edit Me', ]]], k='-CUST MENUBAR-',p=0)],
[sg.Checkbox('Use Custom Titlebar & Menubar', use_custom_titlebar, enable_events=True, k='-USE CUSTOM TITLEBAR-')],
[sg.T('PySimpleGUI Elements - Use Combo to Change Themes', font='_ 18', justification='c', expand_x=True)],
[sg.Col(layout_l), sg.Col(layout_r)]]
window = sg.Window('The PySimpleGUI Element List', layout, finalize=True, right_click_menu=sg.MENU_RIGHT_CLICK_EDITME_VER_EXIT, keep_on_top=True, use_custom_titlebar=use_custom_titlebar)
window['-PBAR-'].update(30) # Show 30% complete on ProgressBar
window['-GRAPH-'].draw_image(data=sg.EMOJI_BASE64_HAPPY_JOY, location=(0,50)) # Draw something in the Graph Element
return window
# Start of the program...
window = make_window()
while True:
event, values = window.read()
sg.popup(event, values) # show the results of the read in a popup Window
if event == sg.WIN_CLOSED or event == 'Exit':
break
if values['-COMBO-'] != sg.theme():
sg.theme(values['-COMBO-'])
window.close()
window = make_window()
if event == '-USE CUSTOM TITLEBAR-':
use_custom_titlebar = values['-USE CUSTOM TITLEBAR-']
window.close()
window = make_window()
window.close()
```
When you run it, assuming you've not set a default theme in the system settings, you'll see a window that looks like this (when on Windows):
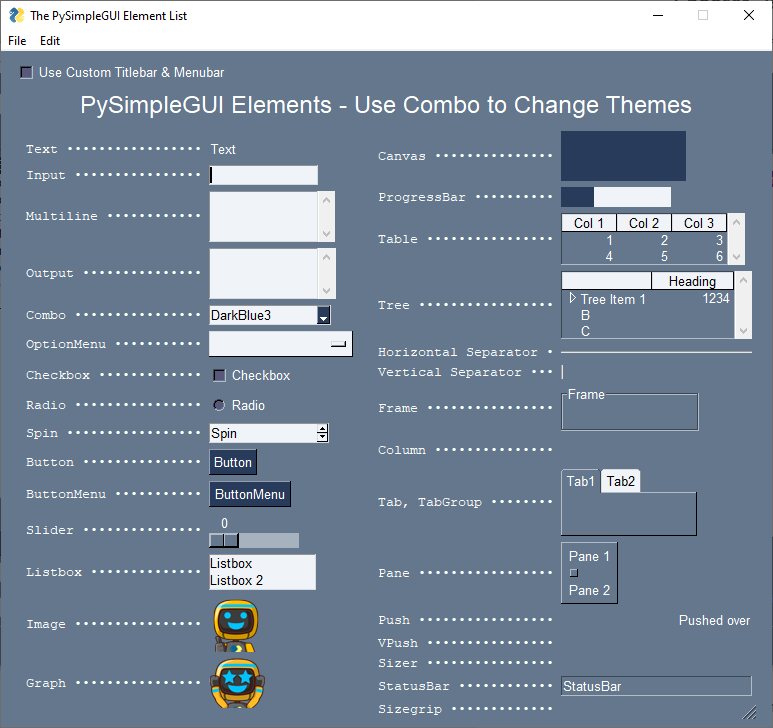
The checkbox at the top that reads "Use Custom Titlebar & Menubar" will enable you to see the what the window looks like using the PySimpleGUI Custom Titlebar and Custom Menubar.
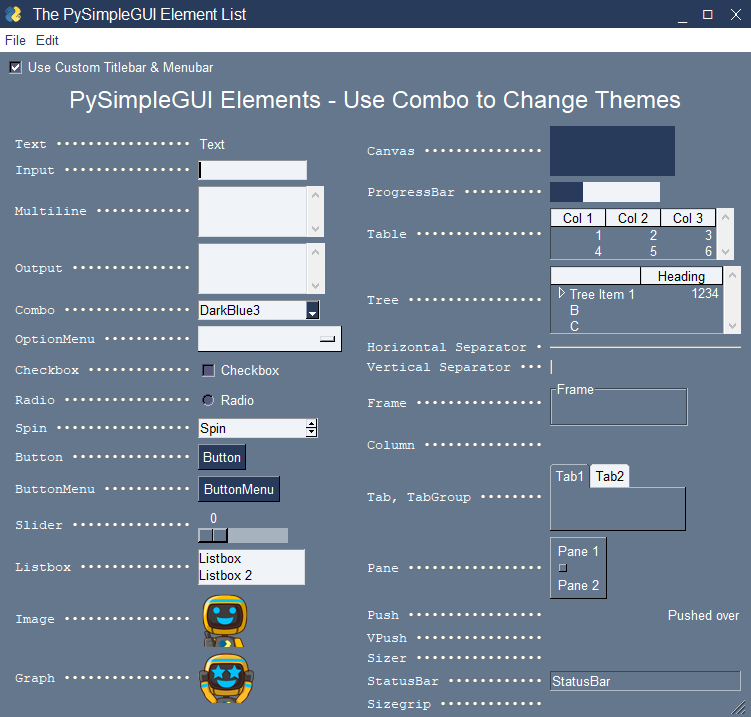
Using this one test program, you can easily browse the PySimpleGUI Themes by choosing a new color Theme using the Combo Element.
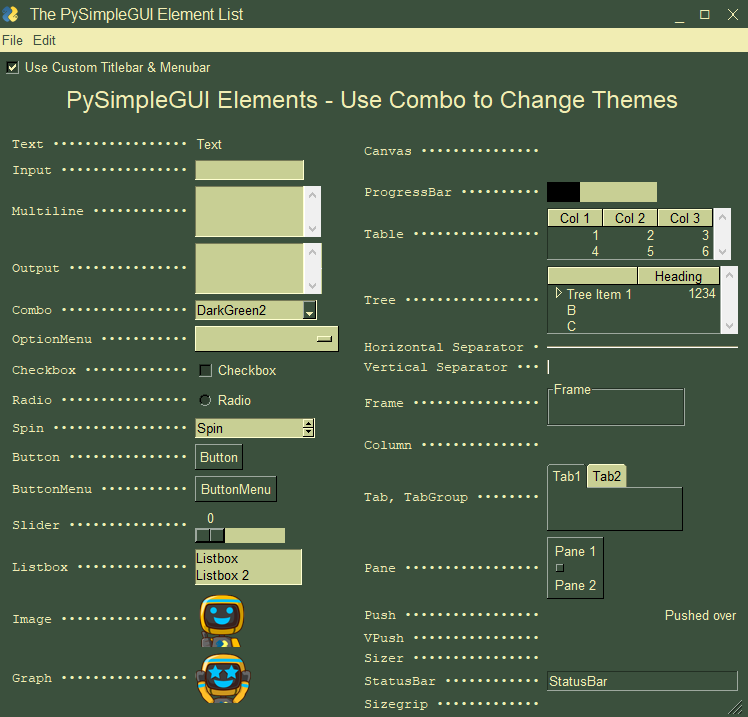
### But it's so UGLY!
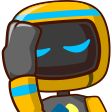
On Windows, using straight Python, this is the non-GUI alternative.
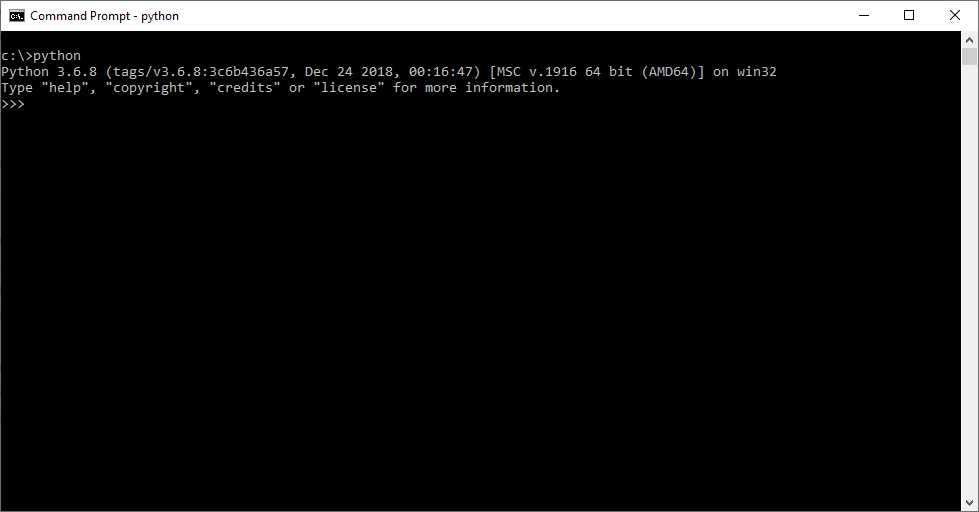
The 1970s and 1980s were a great era for computing, but I wouldn't call the user interfaces "attractive". Borland made some great tools in the 90's for doing TUIs. There are some excellent Python packages available for you if you're after a TUI! If that's the look you're after, give them a try. You may like the results. But, it's not what PySimpleGUI is about.
We're making GUIs for the masses, something you can give to anyone and they'll understand how to use it. If you asked a Windows user (i.e. a normal person, not a programmer) to open a command window in the past decade then you likely got a worried look back. Normal people aren't going to use your Python program that's command line based and be thrilled about it. Your programming pals will love your TUI, Mary, who works in accounting, will not.
The example window may look "ugly" or dated to you. ***It's fair to say that it looks dated***. While PySimpleGUI Themes instantly give you a color scheme that matches, it doesn't go much further in making tkinter more attractive/"modern".
***However***, you are far from being out of options to improve the look of your GUI. This bare-bones program is your **starting point**. This is where you begin the process of making an attractive user interface. You've not yet ***started*** to make your interface look better.
No effort has been made to make it look nice. It's purely functional. There are 34 Elements in the window. 34 Elements. Considering what it does, it's miraculous or in the least incredibly impressive. Why? Because in 65 lines of Python code that window was created, shown, and collected the results.
Let's be clear here... this window will take a massive amount of code using the conventional Python GUI packages. If you manage to write a tkinter, Qt or WxPython program that shows all of these Widgets in under 65 lines of Python code, then by all means send the code over.
Fair, side by side tests, recreating the exact same application, have shown numerous times that PySimpleGUI code is at least 1/2 to 1/10th the amount of code than tkinter requires.
There is no magic behind why it's so much shorter. PySimpleGUI is writing all the boilerplate code on your behalf. The same tkinter code is being executed that you would write by hand. The difference is that the code is contained in the PySimpleGUI source code instead of in your application code. That's all that's going on with "wrappers" like PySimpleGUI. Code is being generated and executed for you in order to simplify your code.
A large amount of time nor code is required to create custom Buttons and other elements, including entirely new and more complex elements such a Dials or Gauges. Transforming a button from plain to an image is a parameter to the Button element. It's... not... THAT... **HARD**. You're a programmer, write some code, or *add a parameter* if you want more attractive interfaces. The hooks are there waiting for you to be creative.
### Window Closed Events
**`Note, event values can be None`**. The value for `event` will be the text that is displayed on the button element when it was created or the key for the button. If the user closed the window using the "X" in the upper right corner of the window, then `event` will be `sg.WIN_CLOSED` which is equal to `None`. It is ***vitally*** ***important*** that your code contain the proper checks for `sg.WIN_CLOSED`.
For "persistent windows", **always give your users a way out of the window**. Otherwise you'll end up with windows that never properly close. It's literally 2 lines of code that you'll find in every Demo Program. While you're at it, make sure a `window.close()` call is after your event loop so that your window closes for sure.
### The `values` Dictionary
You can see in the results Popup window that the values returned are a dictionary. Each input field in the window generates one item in the return values list. Input fields often return a `string`. Check Boxes and Radio Buttons return `bool`. Sliders return float or perhaps int depending on how you configured it or which port you're using.
If your window has no keys and it has no buttons that are "browse" type of buttons, then it will return values to you as a list instead of a dictionary. If possible PySimpleGUI tries to return the values as a list to keep things simple.
Note in the list of return values in this example, many of the keys are numbers. That's because no keys were specified on any of the elements (although one was automatically made for you). If you don't specify a key for your element, then a number will be sequentially assigned. For elements that you don't plan on modifying or reading values from, like a Text Element, you can skip adding keys. For other elements, you'll likely want to add keys so that you can easily access the values and perform operations on them.
## Operations That Take a "Long Time"
If you're a Windows user you've seen windows show in their title bar "Not Responding" which is soon followed by a Windows popup stating that "Your program has stopped responding". Well, you too can make that message and popup appear if you so wish! All you need to do is execute an operation that takes "too long" (i.e. a few seconds) inside your event loop.
You have a couple of options for dealing this with. If your operation can be broken up into smaller parts, then you can call `Window.refresh()` occasionally to avoid this message. If you're running a loop for example, drop that call in with your other work. This will keep the GUI happy and Window's won't complain.
If, on the other hand, your operation is not under your control or you are unable to add `refresh` calls, then the next option available to you is to move your long operations into a thread.
### Threading Made Simple
You've been at Python for a couple weeks, bravely ventured into GUI world, and the function you called when a button click is detected takes 20 seconds to complete. Your window displays the "Not Responding" message. If you ask what to do on StackOverflow, you'll be told that "all you have to do is use threads".
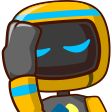
Two weeks into programming, and you've just been told you need to learn Threading?
This exact scenario is why the window method `perform_long_operation` was created. It's a way for you to "ease into" threading without learning about threading. Forget that thread was even mentioned. Let's just talk about "long operations" instead.
There are 2 ways you can use this call. If your function does not take any parameters, then you can enter the name of the function (**without the parentheses!**) as the first parameter to `perform_long_operation`.
```python
window.perform_long_operation(my_function, '-FUNCTION COMPLETED-')
```
If your function has parameters, then you'll need to use a lambda. Don't freak out at hearing "lambda" (yet). Just add it as in this example code...
```python
window.perform_long_operation(lambda: my_function_with_parms(10), '-FUNCTION COMPLETED-')
```
Write the first parameter was if you were calling your function, and then put `lambda: ` in front of that.
The second parameter to `perform_long_operation` is the event (a key) that you want to get back from `window.read()` when your function returns.
Finally, if your function has a return value, then that value will show up in the `values` dictionary with the key that you provided. In this example, `values['-FUNCTION COMPLETED-']` will contain the return value from your function.
```python
import PySimpleGUI as sg
import time
def my_function():
time.sleep(30)
def my_function_with_parms(duration):
time.sleep(duration)
return 'My Return Value'
layout = [ [sg.Text('Call a lengthy function')],
[sg.Button('Start'), sg.Button('Start 2'), sg.Button('Exit')] ]
window = sg.Window('Long Operation Example', layout)
while True:
event, values = window.read()
print(event, values)
if event == sg.WIN_CLOSED or event == 'Exit':
break
if event == 'Start':
window.perform_long_operation(my_function, '-FUNCTION COMPLETED-')
elif event == 'Start 2':
window.perform_long_operation(lambda: my_function_with_parms(10), '-FUNCTION COMPLETED-')
elif event == '-FUNCTION COMPLETED-':
sg.popup('Your function completed!')
window.close()
```
So, in summary, `window.perform_long_operation` starts and manages the thread on your behalf. You are returned an event when your "long operation" completes. If your function returns a value, then that value will show up in the `values` variable from your `window.read()` call. Threading made simple.
### `window.perform_long_operation`Has An Alias `window.start_thread`
If you're a threading pro, then maybe you would prefer the alias for `window.perform_long_operation`. In addition to this name for the method, you'll get the exact same results by calling `window.start_thread`. Since threading is becoming more popular due to the `write_event_value` capability, it makes sense to enable you to call a thread a thread if that's what you prefer... so enjoy the `start_thread` alias if that's how you roll.
### Threading the "New Way" - `Window.write_event_value`
This new function that is available currently only in the tkinter port as of July 2020 is exciting and represents the future way multi-threading is handled in PySimpleGUI.
Previously, a queue was used where your event loop would **poll** for incoming messages from a thread.
Now, threads can directly inject events into a Window so that it will show up in the `window.read()` calls. This allows a your event loop to "pend", waiting for normal window events as well as events being generated by threads. It is much more efficient than polling.
You can see this new capability in action in this demo: `Demo_Multithreaded_Write_Event_Value.py`
Just use the Demo Browser to search for `write_event_value` to get more examples.
Here is that program for your inspection and education. It's SO nice to no longer poll for threaded events.
```python
import threading
import time
import PySimpleGUI as sg
"""
Threaded Demo - Uses Window.write_event_value communications
Requires PySimpleGUI.py version 4.25.0 and later
This is a really important demo to understand if you're going to be using multithreading in PySimpleGUI.
Older mechanisms for multi-threading in PySimpleGUI relied on polling of a queue. The management of a communications
queue is now performed internally to PySimpleGUI.
The importance of using the new window.write_event_value call cannot be emphasized enough. It will hav a HUGE impact, in
a positive way, on your code to move to this mechanism as your code will simply "pend" waiting for an event rather than polling.
Copyright 2020 PySimpleGUI.org
"""
THREAD_EVENT = '-THREAD-'
cp = sg.cprint
def the_thread(window):
"""
The thread that communicates with the application through the window's events.
Once a second wakes and sends a new event and associated value to the window
"""
i = 0
while True:
time.sleep(1)
window.write_event_value('-THREAD-', (threading.current_thread().name, i)) # Data sent is a tuple of thread name and counter
cp('This is cheating from the thread', c='white on green')
i += 1
def main():
"""
The demo will display in the multiline info about the event and values dictionary as it is being
returned from window.read()
Every time "Start" is clicked a new thread is started
Try clicking "Dummy" to see that the window is active while the thread stuff is happening in the background
"""
layout = [ [sg.Text('Output Area - cprint\'s route to here', font='Any 15')],
[sg.Multiline(size=(65,20), key='-ML-', autoscroll=True, reroute_stdout=True, write_only=True, reroute_cprint=True)],
[sg.T('Input so you can see data in your dictionary')],
[sg.Input(key='-IN-', size=(30,1))],
[sg.B('Start A Thread'), sg.B('Dummy'), sg.Button('Exit')] ]
window = sg.Window('Window Title', layout, finalize=True)
while True: # Event Loop
event, values = window.read()
cp(event, values)
if event == sg.WIN_CLOSED or event == 'Exit':
break
if event.startswith('Start'):
threading.Thread(target=the_thread, args=(window,), daemon=True).start()
if event == THREAD_EVENT:
cp(f'Data from the thread ', colors='white on purple', end='')
cp(f'{values[THREAD_EVENT]}', colors='white on red')
window.close()
if __name__ == '__main__':
main()
```
You'll find plenty of examples to help you with these concepts if you look in the Demo Programs. So, pip install the Demo Programs and the Demo Browser so that you can easily search and find examples of what you're trying to accomplish.
---
# Building Custom Windows
You will find it ***much easier*** to write code using PySimpleGUI if you use an IDE such as ***PyCharm***. The features that show you documentation about the API call you are making will help you determine which settings you want to change, if any. In PyCharm, two commands are particularly helpful.
Control-Q (when cursor is on function name) brings up a box with the function definition
Control-P (when cursor inside function call "()") shows a list of parameters and their default values
## Synchronous / Asynchronous Windows
The most common use of PySimpleGUI is to display and collect information from the user. The most straightforward way to do this is using a "blocking" GUI call. Execution is "blocked" while waiting for the user to close the GUI window/dialog box.
You've already seen a number of examples above that use blocking windows. You'll know it blocks if the `Read` call has no timeout parameter.
A blocking Read (one that waits until an event happens) look like this:
```python
event, values = window.read()
```
A non-blocking / Async Read call looks like this:
```python
event, values = window.read(timeout=100)
```
You can learn more about these async / non-blocking windows toward the end of this document.
# Themes - Automatic Coloring of Your Windows
In Dec 2019 the function `change_look_and_feel` was replaced by `theme`. The concept remains the same, but a new group of function calls makes it a lot easier to manage colors and other settings.
By default the PySimpleGUI color theme is now `Dark Blue 3`. Gone are the "system default" gray colors. If you want your window to be devoid of all colors so that the system chooses the colors (gray) for you, then set the theme to 'gray gray gray'. This tells PySimpleGUI that you're a boring person.... no no no... I'm **just kidding!**.... it just means you want PySimpleGUI to not set any colors so that the default colors provided by the OS/tkinter will be used. It's a memorable theme name. There are several with "default" in the name and it got confusing which was the full-on-add-no-color name, so 'gray gray gray' was added to make it easy to recall.
There are 154 themes available. You can preview these themes by calling `theme_previewer()` which will create a LARGE window displaying all of the color themes available.
You can see the current available themes by calling `sg.theme_previewer()`. It creates a window that looked like this in June 2022:
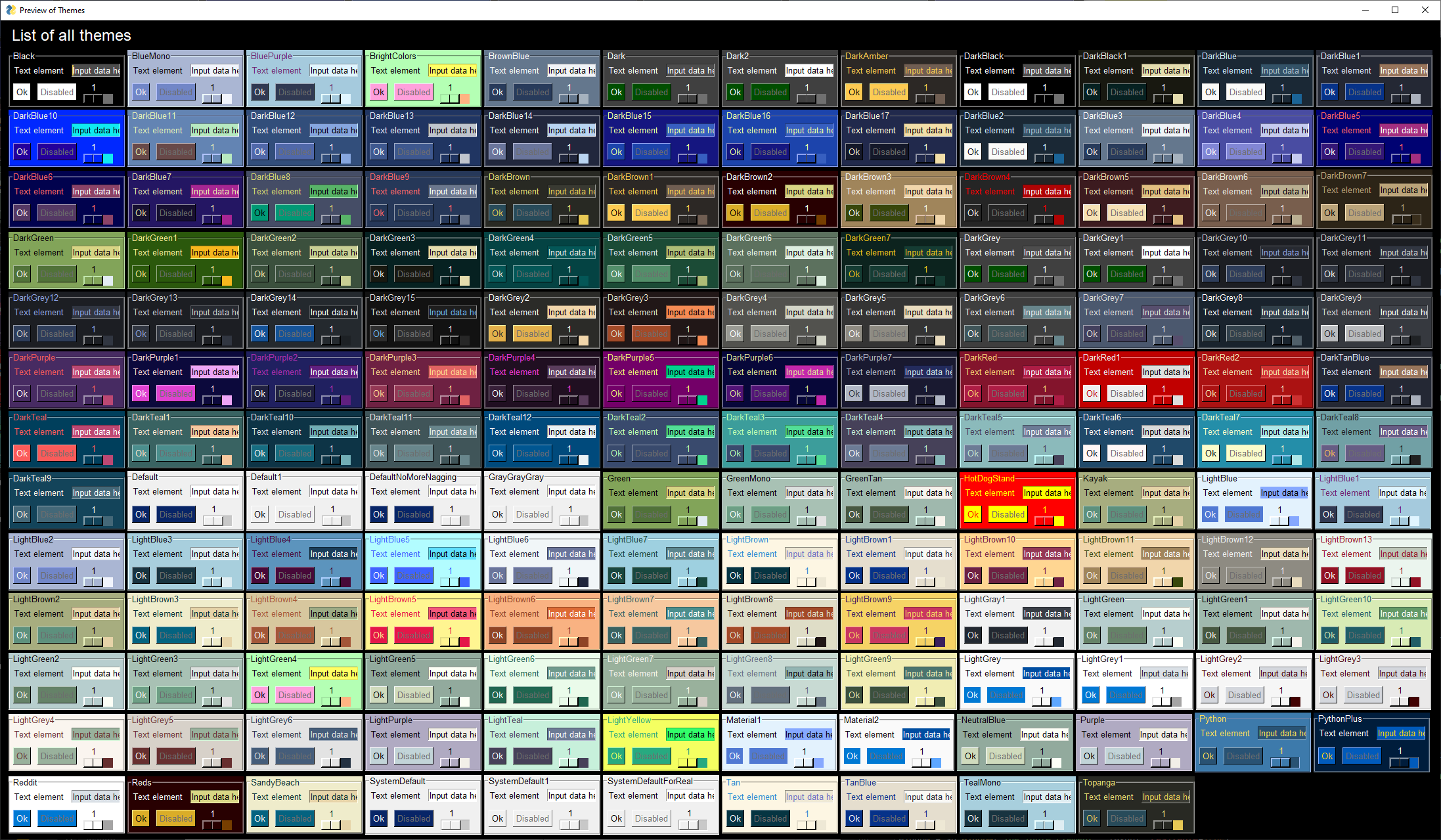
## Default is `Dark Blue 3`
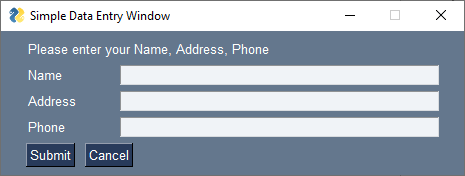
In Dec 2019 the default for all PySimpleGUI windows changed from the system gray with blue buttons to a more complete theme using a grayish blue with white text. Previously users were nagged into choosing color theme other than gray. Now it's done for you instead of nagging you.
If you're struggling with this color theme, then add a call to `theme` to change it.
## Theme Name Formula
Themes names that you specify can be "fuzzy". The text does not have to match exactly what you see printed. For example "Dark Blue 3" and "DarkBlue3" and "dark blue 3" all work.
One way to quickly determine the best setting for your window is to simply display your window using a lot of different themes. Add the line of code to set the theme - `theme('Dark Green 1')`, run your code, see if you like it, if not, change the theme string to `'Dark Green 2'` and try again. Repeat until you find something you like.
The "Formula" for the string is:
`Dark Color #`
or
`Light Color #`
Color can be Blue, Green, Black, Gray, Purple, Brown, Teal, Red. The # is optional or can be from 1 to XX. Some colors have a lot of choices. There are 13 "Light Brown" choices for example.
### "System" Default - No Colors (e.g. `"Gray Gray Gray"`)
If you're bent on having no colors at all in your window, then choose `Default 1` or `System Default 1`. Use the `"Gray Gray Gray"` theme if you really want to ensure system defaults only.
If you want the original PySimpleGUI color scheme of a blue button and everything else gray then you can get that with the theme `Default` or `System Default`.
## Theme Functions
The basic theme function call is `theme(theme_name)`. This sets the theme. Calling without a parameter, `theme()` will return the name of the current theme.
If you want to get or modify any of the theme settings, you can do it with these functions that you will find detailed information about in the function definitions section at the bottom of the document. Each will return the current value if no parameter is used.
You'll find a detailed list of the theme calls in the SDK Call Reference in this section:
https://pysimplegui.readthedocs.io/en/latest/call%20reference/#themes
```python
theme
theme_add_new
theme_background_color
theme_border_width
theme_button_color
theme_button_color_background
theme_button_color_text
theme_element_background_color
theme_element_text_color
theme_global
theme_input_background_color
theme_input_text_color
theme_list
theme_previewer
theme_previewer_swatches
theme_progress_bar_border_width
theme_progress_bar_color
theme_slider_border_width
theme_slider_color
theme_text_color
theme_text_element_background_color
theme_use_custom_titlebar
```
These will help you get a list of available choices.
```python
theme_list
theme_previewer
```
# Window Object - Beginning a window
The first step is to create the window object using the desired window customizations.
## Modal Windows (only applied to tkinter port currently
)
NOTE - as of PySimpleGUI 4.25.0 Modal Windows are supported! By default the `popup` windows that block will be marked Modal by default. This is a somewhat risky change because your existing applications will behave differently. However, in theory, you shouldn't have been interacting with other windows while the popup is active. All of those actions are at best queued. It's implementation dependent.
"Modal" in this case means that you must close this "modal" window before you will be able to interact with windows created before this window. Think about an "about" box. You normally have to close this little popup in most programs. So, that's what PySimpleGUI is doing now.
## Making your window modal
To make a Modal Window you have 2 options.
1. Set the `moodel=True` parameter in your Window calls.
2. Call the method `Window.make_modal()` to chance a window from non-modal to modal. There is no modal to non-modal. Don't see the need for one. If one comes up, sure!
### Disabling modal windows
Popups that block are the only windows that have modal on by default. There is a modal parameter than you can set to False to turn it off.
For the earlier than 4.25.0 and other ports of PySimpleGUI There is no direct support for "**modal windows**" in PySimpleGUI. All windows are accessible at all times unless you manually change the windows' settings.
**IMPORTANT** - Many of the `Window` methods require you to either call `Window.read` or `Window.Finalize` (or set `finalize=True` in your `Window` call) before you call the method. This is because these 2 calls are what actually creates the window using the underlying GUI Framework. Prior to one of those calls, the methods are likely to crash as they will not yet have their underlying widgets created.
### Window Location
PySimpleGUI computes the exact center of your window and centers the window on the screen. If you want to locate your window elsewhere, such as the system default of (0,0), if you have 2 ways of doing this. The first is when the window is created. Use the `location` parameter to set where the window. The second way of doing this is to use the `SetOptions` call which will set the default window location for all windows in the future.
#### Multiple Monitors and Linux
The auto-centering (default) location for your PySimpleGUI window may not be correct if you have multiple monitors on a Linux system. On Windows multiple monitors appear to work ok as the primary monitor the tkinter utilizes and reports on.
Linux users with multiple monitors that have a problem when running with the default location will need to specify the location the window should be placed when creating the window by setting the `location` parameter.
### Window Size
You can get your window's size by access the `Size` property. The window has to be Read once or Finalized in order for the value to be correct. Note that it's a property, not a call.
`my_windows_size = window.Size`
To finalize your window:
```python
window = Window('My Title', layout, finalize=True)
```
### Element Sizes
There are multiple ways to set the size of Elements. They are:
1. The global default size - change using `SetOptions` function
2. At the Window level - change using the parameter `default_element_size` in your call to `Window`
3. At the Element level - each element has a `size` parameter
Element sizes are measured in characters (there are exceptions). A Text Element with `size = (20,1)` has a size of 20 characters wide by 1 character tall.
The default Element size for PySimpleGUI is `(45,1)`.
There are a couple of widgets where one of the size values is in pixels rather than characters. This is true for Progress Meters and Sliders. The second parameter is the 'height' in pixels.
### No Titlebar
Should you wish to create cool looking windows that are clean with no windows titlebar, use the no_titlebar option when creating the window.
Be sure an provide your user an "exit" button or they will not be able to close the window! When no titlebar is enabled, there will be no icon on your taskbar for the window. Without an exit button you will need to kill via taskmanager... not fun.
Windows with no titlebar rely on the grab anywhere option to be enabled or else you will be unable to move the window.
Windows without a titlebar can be used to easily create a floating launcher.
Linux users! Note that this setting has side effects for some of the other Elements. Multi-line input doesn't work at all, for example So, use with caution.

### Grab Anywhere
This is a feature unique to PySimpleGUI.
Note - there is a warning message printed out if the user closes a non-blocking window using a button with grab_anywhere enabled. There is no harm in these messages, but it may be distressing to the user. Should you wish to enable for a non-blocking window, simply get grab_anywhere = True when you create the window.
### Always on top
To keep a window on top of all other windows on the screen, set keep_on_top = True when the window is created. This feature makes for floating toolbars that are very helpful and always visible on your desktop.
### Focus
PySimpleGUI will set a default focus location for you. This generally means the first input field. You can set the focus to a particular element. If you are going to set the focus yourself, then you should turn off the automatic focus by setting `use_default_focus=False` in your Window call.
### TTK Buttons
Beginning in release 4.7.0 PySimpleGUI supports both "normal" tk Buttons and ttk Buttons. This change was needed so that Mac users can use colors on their buttons. There is a bug that causes tk Buttons to not show text when you attempt to change the button color. Note that this problem goes away if you install Python from the official Python.org site rather than using Homebrew. A number of users have switched and are quite happy since even tk Buttons work on the Mac after the switch.
By default Mac users will get ttk Buttons when a Button Element is used. All other platforms will get a normal tk Button. There are ways to override this behavior. One is by using the parameter `use_ttk_buttons` when you create your window. If set to True, all buttons will be ttk Buttons in the window. If set to False, all buttons will be normal tk Buttons. If not set then the platform or the Button Element determines which is used.
If a system-wide setting is desired, then the default can be set using `set_options`. This will affect all windows such as popups and the debug window.
### TTK Themes
tkinter has a number of "Themes" that can be used with ttk widgets. In PySimpleGUI these widgets include - Table, Tree, Combobox, Button, ProgressBar, Tabs & TabGroups. Some elements have a 'theme' parameter but these are no longer used and should be ignored. The initial release of PySimpleGUI attempted to mix themes in a single window but since have learned this is not possible so instead it is set at the Window or the system level.
If a system-wide setting is desired, then the default can be set using `set_options`. This will affect all windows such as popups and the debug window.
The ttk theme choices depend on the platform. Linux has a shorter number of selections than Windows.
The minimum list of TTK themes is:
- default
- alt
- clam
- classic
Most Windows systems have some additional themes that come standard with tkinter:
- default
- alt
- clam
- classic
- winnative
- vista
- xpnative
The list of available themes is populated automatically when you open the Global PySimpleGUI Settings window. Previously the list of themes was hard-coded by the PySimpleGUI code. Now the list is retrieved from tkinter.
There are constants defined to help you with code completion to determine what your choices are. Theme constants start with `THEME_`. For example, the "clam" theme is `THEME_CLAM`
If you try to manually set a TTK theme while making a Window or calling `set_options` and it is not a valid theme, you will be shown the list of valid themes in the error popup. The popup may resemble one like this:
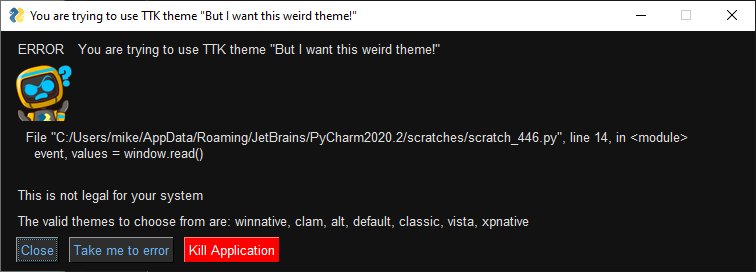
## Closing Windows
When you are completely done with a window, you should close it and then delete it so that the resources, in particular the tkinter resources, are properly cleaned up.
If you wish to do this in 1 line of code, here's your line:
```python
window.close(); del window
```
The delete helps with a problem multi-threaded application encounter where tkinter complains that it is being called from the wrong thread (not the program's main thread)
## Window Methods That Complete Formation of Window
After you have completed making your layout, stored in a variable called `layout` in these examples, you will create your window.
The creation part of a window involves 3 steps.
1. Create a `Window` object
2. Adding your Layout to the window
3. Optional - Finalize if want to make changes prior to `read` call
Over time the PySimpleGUI code has continued to compact, compress, so that as little code as possible will need to be written by the programmer.
### The Individual Calls
This is the "long form" as each method is called individually.
```python
window = sg.Window('My Title')
window.layout(layout)
window.finalize()
```
### Chaining The Calls (the old method)
The next level of compression that was done was to chain the calls together into a single line of code.
```python
window = sg.Window('My Title').Layout(layout).finalize()
```
### Using Parameters Instead of Calls (New Preferred Method)
Here's a novel concept, instead of using chaining, something that's foreign to beginners, use parameters to the `Window` call. And that is exactly what's happened as of 4.2 of the PySimpleGUI port.
```python
window = sg.Window('My Title', layout, finalize=True)
```
Rather than pushing the work onto the user of doing the layout and finalization calls, let the Window initialization code do it for you. Yea, it sounds totally obvious now, but it didn't a few months ago.
This capability has been added to all 4 PySimpleGUI ports but none are on PyPI just yet as there is some runtime required first to make sure nothing truly bad is going to happen.
Call to set the window layout. Must be called prior to `Read`. Most likely "chained" in line with the Window creation.
```python
window = sg.Window('My window title', layout)
```
#### `finalize()` or `Window` parameter `finalize=True`
Call to force a window to go through the final stages of initialization. This will cause the tkinter resources to be allocated so that they can then be modified. This also causes your window to appear. If you do not want your window to appear when Finalize is called, then set the Alpha to 0 in your window's creation parameters.
If you want to call an element's `Update` method or call a `Graph` element's drawing primitives, you ***must*** either call `Read` or `Finalize` prior to making those calls.
#### read(timeout=None, timeout_key=TIMEOUT_KEY, close=False)
Read the Window's input values and button clicks in a blocking-fashion
Returns event, values. Adding a timeout can be achieved by setting timeout=*number of milliseconds* before the Read times out after which a "timeout event" is returned. The value of timeout_key will be returned as the event. If you do not specify a timeout key, then the value `TIMEOUT_KEY` will be returned.
If you set the timeout = 0, then the Read will immediately return rather than waiting for input or for a timeout. It's a truly non-blocking "read" of the window.
# Layouts
While at this point in the documentation you've not been shown very much about each Element available, you should read this section carefully as you can use the techniques you learn in here to build better, shorter, and easier to understand PySimpleGUI code.
If it feels like this layout section is too much too soon, then come back to this section after you're learned about each Element. **Whatever order you find the least confusing is the best.**
While you've not learned about Elements yet, it makes sense for this section to be up front so that you'll have learned how to use the elements prior to learning how each element works. At this point in your PySimpleGUI education, it is better for you to grasp time efficient ways of working with Elements than what each Element does. By learning now how to assemble Elements now, you'll have a good model to put the elements you learn into.
There are *several* aspects of PySimpleGUI that make it more "Pythonic" than other Python GUI SDKs. One of the areas that is unique to PySimpleGUI is how a window's "layout" is defined, specified or built. A window's "layout" is simply a list of lists of elements. As you've already learned, these lists combine to form a complete window. This method of defining a window is super-powerful because lists are core to the Python language as a whole and thus are very easy to create and manipulate.
Think about that for a moment and compare/contrast with Qt, tkinter, etc.. With PySimpleGUI the location of your element in a matrix determines where that Element is shown in the window. It's so ***simple*** and that makes it incredibly powerful. Want to switch a row in your GUI that has text with the one below it that has an input element? No problem, swap the lines of code and you're done.
Layouts were designed to be visual. The idea is for you to be able to envision how a window will look by simply looking at the layout in the code. The CODE itself matches what is drawn on the screen. PySimpleGUI is a cross between straight Python code and a visual GUI designer.
In the process of creating your window, you can manipulate these lists of elements without having an impact on the elements or on your window. Until you perform a "layout" of the list, they are nothing more than lists containing objects (they just happen to be your window's elements).
Many times your window definition / layout will be a static, straightforward to create.
However, window layouts are not limited to being one of these statically defined list of Elements.
# Generated Layouts (For sure want to read if you have > 5 repeating elements/rows)
There are 5 specific techniques of generating layouts discussed in this section. They can be used alone or in combination with each other.
1. Layout + Layout concatenation `[[A]] + [[B]] = [[A], [B]]`
2. Element Addition on Same Row `[[A] + [B]] = [[A, B]]`
3. List Comprehension to generate a row `[A for x in range(10)] = [A,A,A,A,A...]`
4. List Comprehension to generate multiple rows `[[A] for x in range(10)] = [[A],[A],...]`
5. User Defined Elements / Compound Elements
## Example - List Comprehension To Concatenate Multiple Rows - "To Do" List Example
Let's create a little layout that will be used to make a to-do list using PySimpleGUI.
### Brute Force
```python
import PySimpleGUI as sg
layout = [
[sg.Text('1. '), sg.In(key=1)],
[sg.Text('2. '), sg.In(key=2)],
[sg.Text('3. '), sg.In(key=3)],
[sg.Text('4. '), sg.In(key=4)],
[sg.Text('5. '), sg.In(key=5)],
[sg.Button('Save'), sg.Button('Exit')]
]
window = sg.Window('To Do List Example', layout)
event, values = window.read()
```
The output from this script was this window:
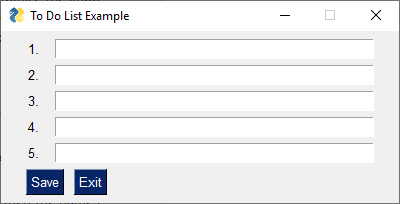
Take a moment and look at the code and the window that's generated. Are you able to look at the layout and envision the Window on the screen?
### Build By Concatenating Rows
The brute force method works great on a list that's 5 items long, but what if your todo list had 40 items on it. THEN what? Well, that's when we turn to a "generated" layout, a layout that is generated by your code. Replace the layout= stuff from the previous example with this definition of the layout.
```python
import PySimpleGUI as sg
layout = []
for i in range(1,6):
layout += [sg.Text(f'{i}. '), sg.In(key=i)],
layout += [[sg.Button('Save'), sg.Button('Exit')]]
window = sg.Window('To Do List Example', layout)
event, values = window.read()
```
It produces the exact same window of course. That's progress.... went from writing out every row of the GUI to generating every row. If we want 48 items as suggested, change the range(1,6) to range(1,48). Each time through the list another row is added onto the layout.
### Create Several Rows Using List Comprehension
BUT, we're not done yet!
This is **Python**, we're using lists to build something up, so we should be looking at ****list comprehensions****. Let's change the `for` loop into a list comprehension. Recall that our `for` loop was used to concatenate 6 rows into a layout.
```python
layout = [[sg.Text(f'{i}. '), sg.In(key=i)] for i in range(1,6)]
```
Here we've moved the `for` loop to inside of the list definition (a list comprehension)
### Concatenating Multiple Rows
We have our rows built using the list comprehension, now we just need the buttons. They can be easily "tacked onto the end" by simple addition.
```python
layout = [[sg.Text(f'{i}. '), sg.In(key=i)] for i in range(1,6)]
layout += [[sg.Button('Save'), sg.Button('Exit')]]
```
Anytime you have 2 layouts, you can concatenate them by simple addition. Make sure your layout is a "list of lists" layout. In the above example, we know the first line is a generated layout of the input rows. The last line adds onto the layout another layout... note the format being [ [ ] ].
This button definition is an entire layout, making it possible to add to our list comprehension
`[[sg.Button('Save'), sg.Button('Exit')]]`
It's quite readable code. The 2 layouts line up visually quite well.
But let's not stop there with compressing the code. How about removing that += and instead change the layout into a single line with just a `+` between the two sets of row.
Doing this concatenation on one line, we end up with this single statement that creates the **entire layout** for the GUI:
```python
layout = [[sg.Text(f'{i}. '), sg.In(key=i)] for i in range(1,6)] + [[sg.Button('Save'), sg.Button('Exit')]]
```
### Final "To Do List" Program
And here we have our final program... all **4** lines.
```python
import PySimpleGUI as sg
layout = [[sg.Text(f'{i}. '), sg.In(key=i)] for i in range(1,6)] + [[sg.Button('Save'), sg.Button('Exit')]]
window = sg.Window('To Do List Example', layout)
event, values = window.read()
```
If you really wanted to crunch things down, you can make it a 2 line program (an import and 1 line of code) by moving the layout into the call to `Window`
```python
import PySimpleGUI as sg
event, values = sg.Window('To Do List Example', layout=[[sg.Text(f'{i}. '), sg.In(key=i)] for i in range(1,6)] + [[sg.Button('Save'), sg.Button('Exit')]]).read()
```
## Example - List Comprehension to Build Rows - Table Simulation - Grid of Inputs
In this example we're building a "table" that is 4 wide by 10 high using `Input` elements
The end results we're seeking is something like this:
```
HEADER 1 HEADER 2 HEADER 3 HEADER 4
INPUT INPUT INPUT INPUT
INPUT INPUT INPUT INPUT
INPUT INPUT INPUT INPUT
INPUT INPUT INPUT INPUT
INPUT INPUT INPUT INPUT
INPUT INPUT INPUT INPUT
INPUT INPUT INPUT INPUT
INPUT INPUT INPUT INPUT
INPUT INPUT INPUT INPUT
INPUT INPUT INPUT INPUT
```
Once the code is completed, here is how the result will appear:
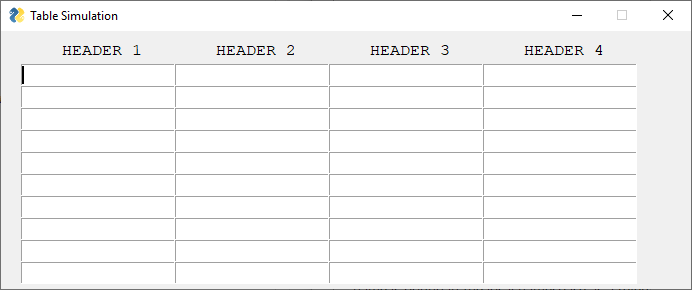
We're going to be building each row using a list comprehension and we'll build the table by concatenating rows using another list comprehension. That's a list comprehension that goes across and another list comprehension that goes down the layout, adding one row after another.
### Building the Header
First let's build the header. There are 2 concepts to notice here:
```python
import PySimpleGUI as sg
headings = ['HEADER 1', 'HEADER 2', 'HEADER 3','HEADER 4'] # the text of the headings
header = [[sg.Text(' ')] + [sg.Text(h, size=(14,1)) for h in headings]] # build header layout
```
There are 2 things in this code to note
1. The list comprehension that makes the heading elements
2. The spaces added onto the front
Let's start with the headers themselves.
This is the code that makes a row of Text Elements containing the text for the headers. The result is a list of Text Elements, a row.
```python
[sg.Text(h, size=(14,1)) for h in headings]
```
Then we add on a few spaces to shift the headers over so they are centered over their columns. We do this by simply adding a `Text` Element onto the front of that list of headings.
```python
header = [[sg.Text(' ')] + [sg.Text(h, size=(14,1)) for h in headings]]
```
This `header` variable is a layout with 1 row that has a bunch of `Text` elements with the headings.
### Building the Input Elements
The `Input` elements are arranged in a grid. To do this we will be using a double list comprehension. One will build the row the other will add the rows together to make the grid. Here's the line of code that does that:
```python
input_rows = [[sg.Input(size=(15,1), pad=(0,0)) for col in range(4)] for row in range(10)]
```
This portion of the statement makes a single row of 4 `Input` Elements
```python
[sg.Input(size=(15,1), pad=(0,0)) for col in range(4)]
```
Next we take that list of `Input` Elements and make as many of them as there are rows, 10 in this case. We're again using Python's awesome list comprehensions to add these rows together.
```python
input_rows = [[sg.Input(size=(15,1), pad=(0,0)) for col in range(4)] for row in range(10)]
```
The first part should look familiar since it was just discussed as being what builds a single row. To make the matrix, we simply take that single row and create 10 of them, each being a list.
### Putting it all together
Here is our final program that uses simple addition to add the headers onto the top of the input matrix. To make it more attractive, the color theme is set to 'Dark Brown 1'.
```python
import PySimpleGUI as sg
sg.theme('Dark Brown 1')
headings = ['HEADER 1', 'HEADER 2', 'HEADER 3','HEADER 4']
header = [[sg.Text(' ')] + [sg.Text(h, size=(14,1)) for h in headings]]
input_rows = [[sg.Input(size=(15,1), pad=(0,0)) for col in range(4)] for row in range(10)]
layout = header + input_rows
window = sg.Window('Table Simulation', layout, font='Courier 12')
event, values = window.read()
```
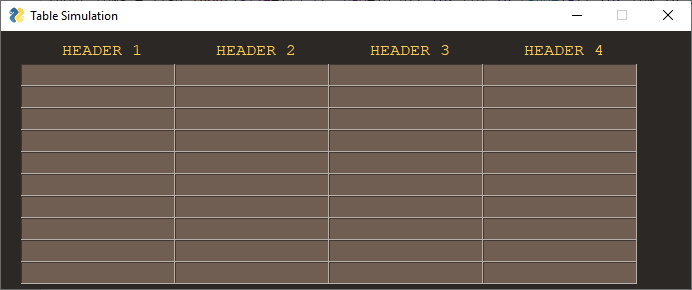
## User Defined Elements / Compound Elements
"User Defined Elements" and "Compound Elements" are one or more PySimpleGUI Elements that are wrapped in a function definition. In a layout, they have the appearance of being a custom elements of some type.
User Defined Elements are particularly useful when you set a lot of parameters on an element that you use over and over in your layout.
### Example - A Grid of Buttons for Calculator App
Let's say you're making a calculator application with buttons that have these settings:
* font = Helvetica 20
* size = 5,1
* button color = white on blue
The code for **one** of these buttons is:
```python
sg.Button('1', button_color=('white', 'blue'), size=(5, 1), font=("Helvetica", 20))
```
If you have 6 buttons across and 5 down, your layout will have 30 of these lines of text.
One row of these buttons could be written:
```python
[sg.Button('1', button_color=('white', 'blue'), size=(5, 1), font=("Helvetica", 20)),
sg.Button('2', button_color=('white', 'blue'), size=(5, 1), font=("Helvetica", 20)),
sg.Button('3', button_color=('white', 'blue'), size=(5, 1), font=("Helvetica", 20)),
sg.Button('log', button_color=('white', 'blue'), size=(5, 1), font=("Helvetica", 20)),
sg.Button('ln', button_color=('white', 'blue'), size=(5, 1), font=("Helvetica", 20)),
sg.Button('-', button_color=('white', 'blue'), size=(5, 1), font=("Helvetica", 20))],
```
By using User Defined Elements, you can significantly shorten your layouts. Let's call our element `CBtn`. It would be written like this:
```python
def CBtn(button_text):
return sg.Button(button_text, button_color=('white', 'blue'), size=(5, 1), font=("Helvetica", 20))
```
Using your new `CBtn` Element, you could rewrite the row of buttons above as:
```python
[CBtn('1'), CBtn('2'), CBtn('3'), CBtn('log'), CBtn('ln'), CBtn('-')],
```
See the tremendous amount of code you do not have to write! USE this construct any time you find yourself copying an element many times.
But let's not stop there.
Since we've been discussing list comprehensions, let's use them to create this row. The way to do that is to make a list of the symbols that go across the row make a loop that steps through that list. The result is a list that looks like this:
```python
[CBtn(t) for t in ('1','2','3', 'log', 'ln', '-')],
```
That code produces the same list as this one we created by hand:
```python
[CBtn('1'), CBtn('2'), CBtn('3'), CBtn('log'), CBtn('ln'), CBtn('-')],
```
### Compound Elements
Just like a `Button` can be returned from a User Defined Element, so can multiple Elements.
Going back to the To-Do List example we did earlier, we could have defined a User Defined Element that represented a To-Do Item and this time we're adding a checkbox. A single line from this list will be:
* The item # (a `Text` Element)
* A `Checkbox` Element to indicate completed
* An `Input` Element to type in what to do
The definition of our User Element is this `ToDoItem` function. It is a single User Element that is a combination of 3 PySimpleGUI Elements.
```python
def ToDoItem(num):
return [sg.Text(f'{num}. '), sg.CBox(''), sg.In()]
```
This makes creating a list of 5 to-do items downright trivial when combined with the list comprehension techniques we learned earlier. Here is the code required to create 5 entries in our to-do list.
```python
layout = [ToDoItem(x) for x in range(1,6)]
```
We can then literally add on the buttons
```python
layout = [ToDoItem(x) for x in range(1,6)] + [[sg.Button('Save'), sg.Button('Exit')]]
```
And here is our final program
```python
import PySimpleGUI as sg
def ToDoItem(num):
return [sg.Text(f'{num}. '), sg.CBox(''), sg.In()]
layout = [ToDoItem(x) for x in range(1,6)] + [[sg.Button('Save'), sg.Button('Exit')]]
window = sg.Window('To Do List Example', layout)
event, values = window.read()
```
And the window it creates looks like this:
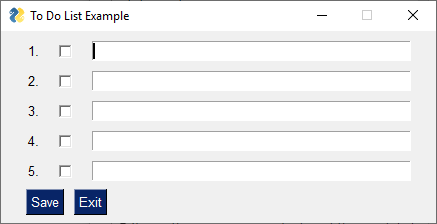
---
# Elements
You will find information on Elements and all other classes and functions are located in the Call Reference Tab of the documentation.
"Elements" are the building blocks used to create windows. Some GUI APIs use the term "Widget" to describe these graphic elements. So that it's clear when a PySimpleGUI *Element* is being referenced versus an underlying GUI Framework's *Widget*. PySimpleGUI Elements map to a GUI Framework Widget, usually in a 1-to-1 manner. For example, a Text Element is implemented in tkinter using a Label Widget.
## Table of Elements in Tkinter Port
Each port of PySimpleGUI has a core set of Elements as well as port-specific elements. Some port-specific elements include the Dial element in the Qt port, and the Pane element in the tkinter port.
| Element Name | Aliases | tkinter Widget | Description |
| :------------------ | :----------------------------- | :-------------------------------------- | :----------------------------------------------------------- |
| Text | T, Txt | tk.Label | One or more lines of Text |
| Input | I, In, InputText | tk.Entry | Single line text input |
| Combo | DD, Drop, DropDown, InputCombo | ttk.Combobox | A "Dropdown" list that can be edited too |
| OptionMenu | InputOptionMenu | tk.OptionMenu | Like a Combo |
| Multiline | ML, MLine | tk.Text or tk.scrolledtext.ScrolledText | Multiple lines of text for input or output |
| Output | | tk.Text (sorta) | Not suggested. Multiline is better choice. Re-routes stdout |
| Radio | R, Rad | tk.Radiobutton | Radio Buttons - choose 1 from several |
| Checkbox | CB, CBox, Check | tk.Checkbutton | Checkbox - binary choice Yes/No |
| Spin | Sp | tk.Spinbox | Choose 1 by using arrows. Can manually enter also |
| Button | B, Btn | tk.Button or ttk.Button | Button - plain or with image |
| Image | Im | tk.Label | A PNG or GIF image |
| Canvas | | tk.Canvas | A drawing area. Graph may be batter to use |
| Column | Col | Combination Canvas & Frame | Embeds layouts inside layouts |
| Frame | Fr | tk.LabelFrame | A frame with a title and a border |
| Tab | | tk.Frame | Container used with TabGroup |
| TabGroup | | ttk.Notebook | Holds Tabs in layouts |
| Pane | | tk.PanedWindow | Sliding columns (it's kinda weird but useful) |
| Graph | G | tk.Canvas | A drawing area with primitives |
| Slider | Sl | tk.Scale | Slider to choose from range of choices |
| Listbox | LB, LBox | tk.Listbox | Listbox - a list of choices |
| Menu | MenuBar, Menubar | tk.Menu | A standard Menubar |
| MenubarCustom | | Combined Elements | Custom colors and font for menubar |
| ButtonMenu | BM, BMenu | tk.Menubutton | Button that shows a menu |
| Titlebar | | Combined Elements | Custom colors for a titlebar |
| ProgressBar | PBar, Prog, Progress | ttk.Progressbar | |
| Table | | ttk.Treeview | A table with clickible cells and headers |
| Tree | | ttk.Treeview | A tree with collapsible sections |
| VerticalSeparator | VSep, VSeparator | ttk.Separator | Vertical line |
| HorizontalSeparator | HSep, HSeparator | ttk.Separator | Horizontal line |
| StatusBar | SBar | tk.Label | Statusbar for bottom of window |
| Sizegrip | SGrip | ttk.Sizegrip | A grip for the bottom right corner of a window |
| Push | P, Stretch | PySimpleGUI Elements | Pushes elements horizontally |
| VPush | VP, VStretch | PySimpleGUI Elements | Pushes rows vertically |
| Sizer | | Column Element | Creates a Width x Height number of pixels for padding/sizing |
## Layout Helper Functions
Your Window's layout is composed of lists of Elements. In addition to elements, these Layout Help Functions may also be present in a layout definition
| Layout Helper | Description |
| :------------ | :---------------------------------------------------------------------------------------------------------------------------------------- |
| pin | "Pins" an element to a location in a layout. If element transitions from invisible to visible, pin ensures element is in correct location |
| vtop | Vertically align element or row of elements to the top of the row |
| vbottom | Vertically align element or row of elements to the bottom of the row |
| vcenter | Vertically align element or row of elements to the center of the row |
## Keys
***Keys are a super important concept to understand in PySimpleGUI.***
If you are going to do anything beyond the basic stuff with your GUI, then you need to understand keys.
You can think of a "key" as a "name" for an element. Or an "identifier". It's a way for you to identify and talk about an element with the PySimpleGUI library. It's the exact same kind of key as a dictionary key. They must be unique to a window.
Keys are specified when the Element is created using the `key` parameter.
Keys are used in these ways:
* Specified when creating the element
* Returned as events. If an element causes an event, its key will be used
* In the `values` dictionary that is returned from `window.read()`
* To make updates (changes), to an element that's in the window
After you put a key in an element's definition, the values returned from `window.read` will use that key to tell you the value. For example, if you have an input element in your layout:
`Input(key='mykey')`
And your read looks like this: `event, values = Read()`
Then to get the input value from the read it would be: `values['mykey']`
You also use the same key if you want to call Update on an element. Please see the section Updating Elements to understand that usage. To get find an element object given the element's key, you can call the window method `find_element` (also written `FindElement`, `element`), or you can use the more common lookup mechanism:
```python
window['key']
```
While you'll often see keys written as strings in examples in this document, know that keys can be ***ANYTHING***.
Let's say you have a window with a grid of input elements. You could use their row and column location as a key (a tuple)
`key=(row, col)`
Then when you read the `values` variable that's returned to you from calling `Window.read()`, the key in the `values` variable will be whatever you used to create the element. In this case you would read the values as:
`values[(row, col)]`
Most of the time they are simple text strings. In the Demo Programs, keys are written with this convention:
`_KEY_NAME_` (underscore at beginning and end with all caps letters) or the most recent convention is to use a dash at the beginning and end (e.g. `'-KEY_NAME-'`). You don't have to follow the convention, but it's not a bad one to follow as other users are used to seeing this format and it's easy to spot when element keys are being used.
If you have an element object, to find its key, access the member variable `.Key` for the element. This assumes you've got the element in a variable already.
```python
text_elem = sg.Text('', key='-TEXT-')
the_key = text_elem.Key
```
### Default Keys
If you fail to place a key on an Element, then one will be created for you automatically.
For `Buttons`, the text on the button is that button's key. `Text` elements will default to the text's string (for when events are enabled and the text is clicked)
If the element is one of the input elements (one that will cause an generate an entry in the return values dictionary) and you fail to specify one, then a number will be assigned to it beginning with the number 0. The effect will be as if the values are represented as a list even if a dictionary is used.
### Menu Keys
Menu items can have keys associated with them as well. See the section on Menus for more information about these special keys. They aren't the same as Element keys. Like all elements, Menu Element have one of these Element keys. The individual menu item keys are different.
### `WRITE_ONLY_KEY` Modifier
Sometimes you have input elements (e.g. `Multiline`) that you are using as an output. The contents of these elements may get very long. You don't need to "read" these elements and doing so will potentially needlessly return a lot of data.
To tell PySimpleGUI that you do not want an element to return a value when `Window.read` is called, add the string `WRITE_ONLY_KEY` to your key name.
If your `Multiline` element was defined like this originally:
```python
sg.Multiline(size=(40,8), key='-MLINE-')
```
Then to turn off return values for that element, the `Multiline` element would be written like this:
```python
sg.Multiline(size=(40,8), key='-MLINE-' + sg.WRITE_ONLY_KEY)
```
## Key Errors - Key error recovery algorithm
In the primary (tkinter) port of PySimpleGUI, starting in version 4.27.0 (not yet on PyPI... but available on GitHub as 4.26.0.14+)
There are now 3 controls over key error handling and a whole new era of key reporting.
```python
SUPPRESS_ERROR_POPUPS = False
SUPPRESS_RAISE_KEY_ERRORS = False
SUPPRESS_KEY_GUESSING = False
```
You can modify these values by calling `set_options`.
```python
sg.set_options(suppress_raise_key_errors=False, suppress_error_popups=False, suppress_key_guessing=False)
```
A basic definition of them are:
`suppress_error_popups` - Disables error popups that are generated within PySimpleGUI itself to not be shown
`suppress_raise_key_errors` - Disables raising a key error if a key or a close match are not found
`suppress_key_guessing` - Disables the key guessing algorithm should you have a key error
With the defaults left as defined (all `False`), here is how key errors work.
This is the program being used in this example:
```python
import PySimpleGUI as sg
def main():
sg.set_options(suppress_raise_key_errors=False, suppress_error_popups=False, suppress_key_guessing=False)
layout = [ [sg.Text('My Window')],
[sg.Input(k='-IN-'), sg.Text(size=(12,1), key='-OUT-')],
[sg.Button('Go'), sg.Button('Exit')] ]
window = sg.Window('Window Title', layout, finalize=True)
while True: # Event Loop
event, values = window.read()
print(event, values)
if event == sg.WIN_CLOSED or event == 'Exit':
break
window['-O U T'].update(values['-IN-'])
window.close()
def func():
main()
func()
```
A few things to note about it:
* There are multiple levels of functions being called, not just a flat program
* There are 2 keys explicitly defined, both are text at this point (we'll change them later)
* There are 2 lookups happening, one with `window` the other with `values`
This key error recovery algorithm only applies to element keys being used to lookup keys inside of windows. The `values` key lookup is a plain dictionary and so nothing fancy is done for that lookup.
### Example 1 - Simple text string misspelling
In our example, this line of code has an error:
```python
window['-O U T'].update(values['-IN-'])
```
There are multiple problems with the key `'-OUT-'`. It is missing a dash and it has a bunch of extra spaces.
When the program runs, you'll first see the layout with no apparent problems:
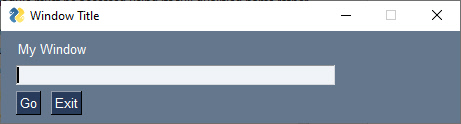
Clicking the OK button will cause the program to return from `window.read()` and thus hit our bad key. The result will be a popup window that resembles this:
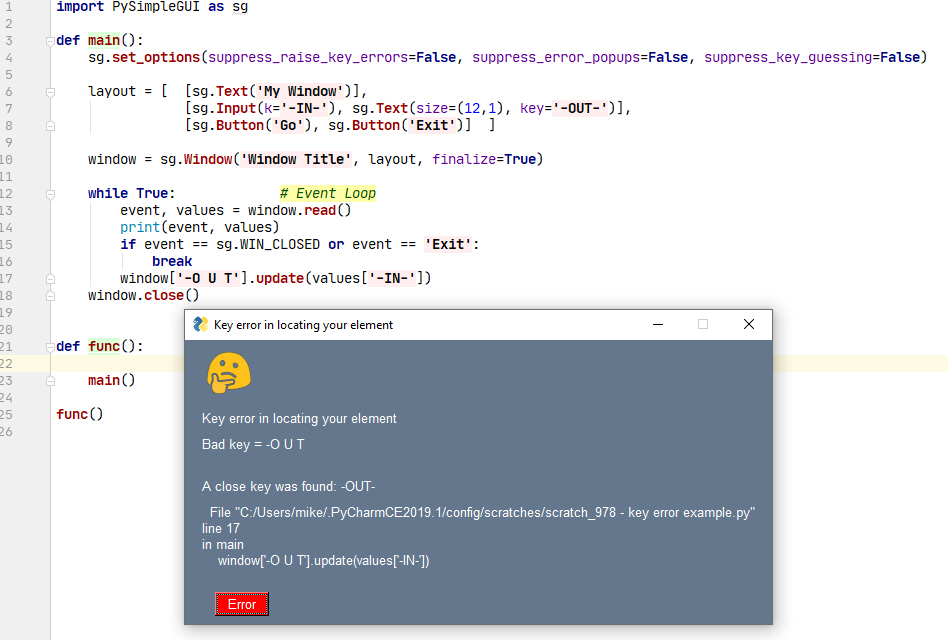
Note a few things about this error popup. Your shown your bad key and you're also shown what you likely meant. Additionally, you're shown the filename, the line number and the line of code itself that has the error.
Because this error was recoverable, the program continues to run after you close the error popup. The result is what you expect from this program... the output field is the same as your information input.
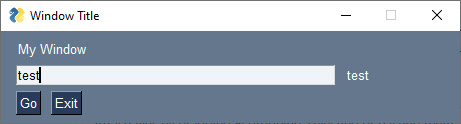
### Example 2 - Tuple error
Keys can be a variety of types, including tuples. In this particular program we have a tuple specified in the layout and have used an incorrect tuple in the lookup. Once again the recovery process worked and the program continued.
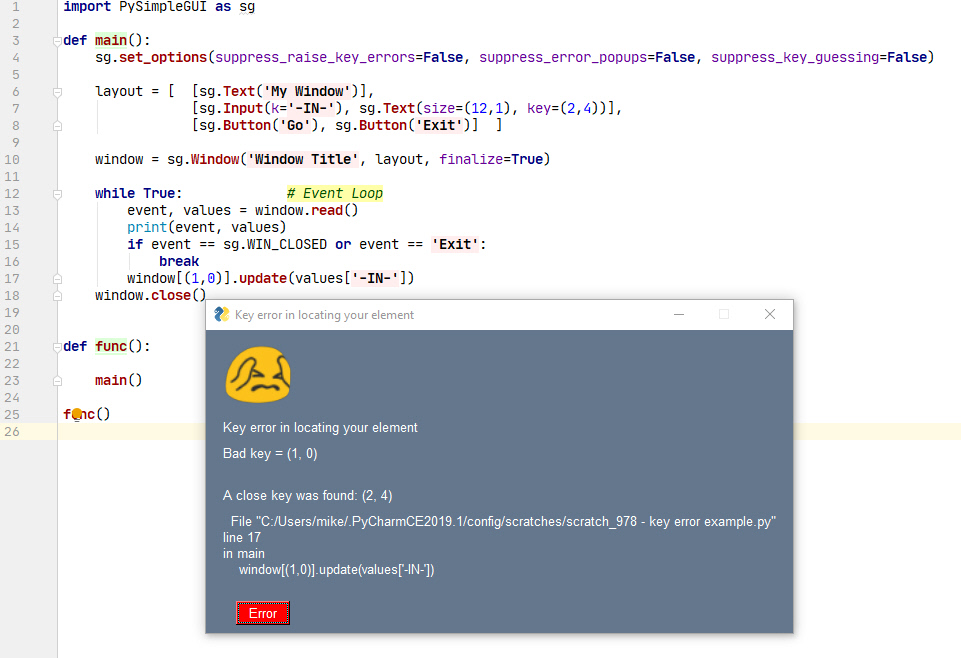
### Example 3 - No close match found
In this example, as you can see in the error popup, there was such a mismatch that no substitution could be performed.
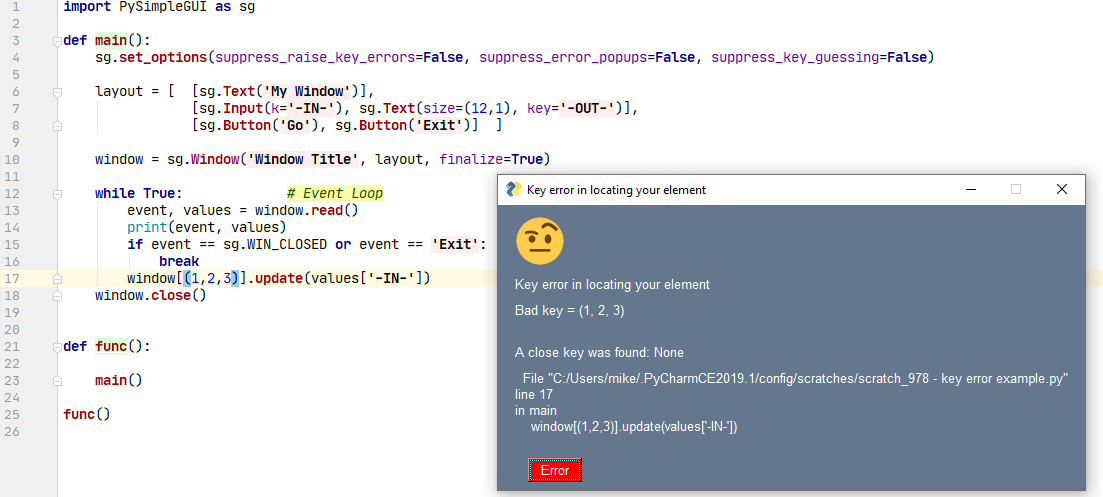
This is an unrecoverable error, so a key error exception is raised.
```python
Traceback (most recent call last):
File "C:/Users/mike/.PyCharmCE2019.1/config/scratches/scratch_978 - key error example.py", line 25, in
func()
File "C:/Users/mike/.PyCharmCE2019.1/config/scratches/scratch_978 - key error example.py", line 23, in func
main()
File "C:/Users/mike/.PyCharmCE2019.1/config/scratches/scratch_978 - key error example.py", line 17, in main
window[(1,2,3)].update(values['-IN-'])
File "C:\Python\PycharmProjects\PSG\PySimpleGUI.py", line 8591, in __getitem__
return self.FindElement(key)
File "C:\Python\PycharmProjects\PSG\PySimpleGUI.py", line 7709, in FindElement
raise KeyError(key)
KeyError: (1, 2, 3)
```
If you're running an IDE such as PyCharm, you can use the information from the assert to jump to the line of code in your IDE based on the crash data provided.
### Choose Your Desired Combination
There are enough controls on this error handling that you can control how you want your program to fail. If you don't want any popups, and no guessing and would instead like to simply get an exception when the key error happens, then call `set_options` with this combination:
```python
sg.set_options(suppress_raise_key_errors=False, suppress_error_popups=True, suppress_key_guessing=True)
```
This will cause Example #1 above to immediately get an exception when hitting the statement with the error. Even though the guessing is turned off, you're still provided with the closest match to help with your debugging....
```
** Error looking up your element using the key: -O U T The closest matching key: -OUT-
Traceback (most recent call last):
File "C:/Users/mike/.PyCharmCE2019.1/config/scratches/scratch_978 - key error example.py", line 25, in
func()
File "C:/Users/mike/.PyCharmCE2019.1/config/scratches/scratch_978 - key error example.py", line 23, in func
main()
File "C:/Users/mike/.PyCharmCE2019.1/config/scratches/scratch_978 - key error example.py", line 17, in main
window['-O U T'].update(values['-IN-'])
File "C:\Python\PycharmProjects\PSG\PySimpleGUI.py", line 8591, in __getitem__
return self.FindElement(key)
File "C:\Python\PycharmProjects\PSG\PySimpleGUI.py", line 7709, in FindElement
raise KeyError(key)
KeyError: '-O U T'
```
## Common Element Parameters
Some parameters that you will see on almost all Element creation calls include:
- key - Used with window[key], events, and in return value dictionary
- tooltip - Hover your mouse over the element and you'll get a popup with this text
- size - (width, height) - usually measured in characters-wide, rows-high. Sometimes they mean pixels
- font - specifies the font family, size, etc.
- colors - Color name or #RRGGBB string
- pad - Amount of padding to put around element
- enable_events - Turns on the element specific events
- visible - Make elements appear and disappear
#### Tooltip
Tooltips are text boxes that popup next to an element if you hold your mouse over the top of it. If you want to be extra kind to your window's user, then you can create tooltips for them by setting the parameter `tooltip` to some text string. You will need to supply your own line breaks / text wrapping. If you don't want to manually add them, then take a look at the standard library package `textwrap`.
Tooltips are one of those "polish" items that really dress-up a GUI and show's a level of sophistication. Go ahead, impress people, throw some tooltips into your GUI. You can change the color of the background of the tooltip on the tkinter version of PySimpleGUI by setting `TOOLTIP_BACKGROUND_COLOR` to the color string of your choice. The default value for the color is:
`TOOLTIP_BACKGROUND_COLOR = "#ffffe0"`
#### Size
Info on setting default element sizes is discussed in the Window section above.
Specifies the amount of room reserved for the Element. For elements that are character based, such a Text, it is (# characters, # rows). Sometimes it is a pixel measurement such as the Image element. And sometimes a mix like on the Slider element (characters long by pixels wide).
Some elements, Text and Button, have an auto-size setting that is `on` by default. It will size the element based on the contents. The result is that buttons and text fields will be the size of the string creating them. You can turn it off. For example, for Buttons, the effect will be that all buttons will be the same size in that window.
Beginning in release 4.47.0 sizes can also be an `int` in addition to a tuple. If an int is specified, then that value is taken as the width and the height is set to 1. If given `size=12` then it's the same as `size=(12,1)`
#### Element Sizes - Non-tkinter Ports (Qt, WxPython, Web)
In non-tkinter ports you can set the specific element sizes in 2 ways. One is to use the normal `size` parameter like you're used to using. This will be in characters and rows.
The other way is to use a new parameter, `size_px`. This parameter allows you to specify the size directly in pixels. A setting of `size_px=(300,200)` will create an Element that is 300 x 200 pixels.
Additionally, you can also indicate pixels using the `size` parameter, **if the size exceeds the threshold for conversion.** What does that mean? It means if your width is > 20 (`DEFAULT_PIXEL_TO_CHARS_CUTOFF`), then it is assumed you're talking pixels, not characters. However, some of the "normally large" Elements have a cutoff value of 100. These include, for example, the `Multline` and `Output` elements.
If you're curious about the math used to do the character to pixels conversion, it's quite crude, but functional. The conversion is completed with the help of this variable:
`DEFAULT_PIXELS_TO_CHARS_SCALING = (10,26)`
The conversion simply takes your `size[0]` and multiplies by 10 and your `size[1]` and multiplies it by 26.
##### Specifying Size as an INT
Beginning in version 4.47.0 you can specify a single int as the size. This will set the size to be a single row in height (1). Writing `size=10` is now the same as writing `size=(10,1)`. A tuple is created on your behalf when you specify a size and an int. This will save a considerable amount of typing, especially for the elements where you typically have only 1 row or can only have 1 row.
#### Colors
A string representing color. Anytime colors are involved, you can specify the tkinter color name such as 'lightblue' or an RGB hex value '#RRGGBB'. For buttons, the color parameter is a tuple (text color, background color)
Anytime colors are written as a tuple in PySimpleGUI, the way to figure out which color is the background is to replace the "," with the word "on". ('white', 'red') specifies a button that is "white on red". Works anywhere there's a color tuple.
#### Pad
The amount of room around the element in pixels. The default value is (5,3) which means leave 5 pixels on each side of the x-axis and 3 pixels on each side of the y-axis. You can change this on a global basis using a call to SetOptions, or on an element basis.
If you want more pixels on one side than the other, then you can split the number into 2 number. If you want 200 pixels on the left side, and 3 pixels on the right, the pad would be ((200,3), 3). In this example, only the x-axis is split.
##### Specifying pad as an INT
Starting in version 4.47.0, it's possible to set pad to be an int rather than a tuple. If an int is specified, then the pad is set to a tuple with each position being the same as the int. This reduces the code in your layout significantly if you use values such as (0,0) for your pad. This is not an uncommon value. Now you can write `pad=0` and you will get the same result as if you typed `pad=(0,0)`
#### Font
Specifies the font family, size, and style. Font families on Windows include:
* Arial
* Courier
* Comic,
* Fixedsys
* Times
* Verdana
* Helvetica (the default I think)
The fonts will vary from system to system, however, Tk 8.0 automatically maps Courier, Helvetica and Times to their corresponding native family names on all platforms. Also, font families cannot cause a font specification to fail on Tk 8.0 and greater.
If you wish to leave the font family set to the default, you can put anything not a font name as the family. The PySimpleGUI Demo programs and documentation use the family 'Any' to demonstrate this fact.. You could use "default" if that's more clear to you.
There are 2 formats that can be used to specify a font... a string, and a tuple
Tuple - (family, size, styles)
String - "Family Size Styles"
To specify an underlined, Helvetica font with a size of 15 the values:
('Helvetica', 15, 'underline italics')
'Helvetica 15 underline italics'
**Font Style - Valid font styles include:**
* italic
* roman
* bold
* normal
* underline
* overstrike
An example with many styles is:
```python
font='Courier 12 italic bold underline overstrike'
```
The same styles can be used with the tuple format for fonts.
#### Key
See the section above that has full information about keys.
#### Visible
Beginning in version 3.17 you can create Elements that are initially invisible that you can later make visible.
To create an invisible Element, place the element in the layout like you normally would and add the parameter
`visible=False`.
Later when you want to make that Element visible you simply call the Element's `Update` method and pass in the parameter `visible=True`
This feature works best on Qt, but does work on the tkinter version as well. The visible parameter can also be used with the Column and Frame "container" Elements.
Note - Tkinter elements behave differently than Qt elements in how they arrange themselves when going from invisible to visible.
tkinter elements tend to STACK themselves.
One workaround is to place the element in a Column with other elements on its row. This will hold the place of the row it is to be placed on. It will move the element to the end of the row however.
If you want to not only make the element invisible, on tkinter you can call `Element.
Qt elements tend to hold their place really well and the window resizes itself nicely. It is more precise and less clunky.
## Shortcut Functions / Multiple Function Names
Perhaps not the best idea, but one that's done none the less is the naming of methods and functions. Some of the more "Heavily Travelled Elements" (and methods/functions) have "shortcuts".
In other words, I am lazy and don't like to type. The result is multiple ways to do exactly the same thing. Typically, the Demo Programs and other examples use the full name, or at least a longer name. Thankfully PyCharm will show you the same documentation regardless which you use.
This enables you to code much quicker once you are used to using the SDK. The Text Element, for example, has 3 different names `Text`, `Txt` or`T`. InputText can also be written `Input` or `In` .
The shortcuts aren't limited to Elements. The `Window` method `Window.FindElement` can be written as `Window.Element` because it's such a commonly used function. BUT, even that has now been shortened to `window[key]`
It's an ongoing thing. If you don't stay up to date and one of the newer shortcuts is used, you'll need to simply rename that shortcut in the code. For examples Replace sg.T with sg.Text if your version doesn't have sg.T in it.
## Text Element | `T == Txt == Text`
Basic Element. It displays text.
```python
layout = [
[sg.Text('This is what a Text Element looks like')],
]
```
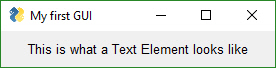
When creating a Text Element that you will later update, make sure you reserve enough characters for the new text. When a Text Element is created without a size parameter, it is created to exactly fit the characters provided.
With proportional spaced fonts (normally the default) the pixel size of one set of characters will differ from the pixel size of a different set of characters even though the set is of the same number of characters. In other words, not all letters use the same number of pixels. Look at the text you're reading right now and you will see this. An "i" takes up a less space then an "A".
---
## `Window.FindElement(key)` shortened to `Window[key]`
There's been a fantastic leap forward in making PySimpleGUI code more compact.
Instead of writing:
```python
window.FindElement(key).update(new_value)
```
You can now write it as:
```python
window[key].update(new_value)
```
This change has been released to PyPI for PySimpleGUI
MANY Thanks is owed to the nngogol that suggested and showed me how to do this. It's an incredible find as have been many of his suggestions.
## `Element.update()` -> `Element()` shortcut
This has to be one of the strangest syntactical constructs I've ever written.
It is best used in combination with `FindElement` (see prior section on how to shortcut `FindElement`).
Normally to change an element, you "find" it, then call its `update` method. The code usually looks like this, as you saw in the previous section:
```python
window[key].update(new_value)
```
The code can be further compressed by removing the `.update` characters, resulting in this very compact looking call:
```python
window[key](new_value)
```
Yes, that's a valid statement in Python.
What's happening is that the element itself is being called. You can also writing it like this:
```python
elem = sg.Text('Some text', key='-TEXT-')
elem('new text value')
```
Side note - you can also call your `window` variable directly. If you "call" it it will actually call `Window.read`.
```python
window = sg.Window(....)
event, values = window()
# is the same as
window = sg.Window(....)
event, values = window.read()
```
It is confusing looking however so when used, it might be a good idea to write a comment at the end of the statement to help out the poor beginner programmer coming along behind you.
Because it's such a foreign construct that someone with 1 week of Python classes will not recognize, the demos will continue to use the `.update` method.
It does not have to be used in conjuction with `FindElement`. The call works on any previously made Element. Sometimes elements are created, stored into a variable and then that variable is used in the layout. For example.
```python
graph_element = sg.Graph(...... lots of parms ......)
layout = [[graph_element]]
.
.
.
graph_element(background_color='blue') # this calls Graph.update for the previously defined element
```
Hopefully this isn't too confusing. Note that the methods these shortcuts replace will not be removed. You can continue to use the old constructs without changes.
---
### Fonts
Already discussed in the common parameters section. Either string or a tuple.
### Color in PySimpleGUI are in one of two formats - color name or RGB value.
Individual colors are specified using either the color names as defined in tkinter or an RGB string of this format:
"#RRGGBB" or "darkblue"
### `auto_size_text `
A `True` value for `auto_size_text`, when placed on Text Elements, indicates that the width of the Element should be shrunk do the width of the text. The default setting is True. You need to remember this when you create `Text` elements that you are using for output.
`Text(key='-TXTOUT-)` will create a `Text` Element that has 0 length. Notice that for Text elements with an empty string, no string value needs to be indicated. The default value for strings is `''` for Text Elements. Prior to release 4.45.0 you needed to reserve enough room for your longest string. If you tried to output a string that's 5 characters, it wasn't shown in the window because there wasn't enough room. The remedy was to manually set the size to what you expect to output
`Text(size=(15,1), key='-TXTOUT-)` creates a `Text` Element that can hold 15 characters.
With the newer versions, after 4.45.0, if you set no size at all, that is `size=(None, None)`, then both the `Text` element will grow and shrink to fit the text and so will the `Window`. Additionally, if you indicate that the height is `None` then the element will grow and shrink in their to match the string.
The way the `Text` element now works is truly auto-sized.
### Chortcut functions
The shorthand functions for `Text` are `Txt` and `T`
### Events `enable_events`
If you set the parameter `enable_events` then you will get an event if the user clicks on the Text.
## Multiline Element
This Element doubles as both an input and output Element.
```python
layout = [[sg.Multiline('This is what a Multi-line Text Element looks like', size=(45,5))]]
```
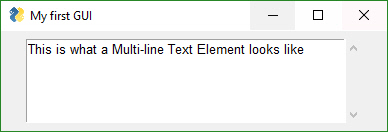
This element has been expanded upon quite a bit over time. Two of the more exciting additions have been
* Ability to output unique text and background colors on a per-character basis
* The `print` method that allows you to easily reroute your normally printed output to a multiline element instead
The `Multiline.print()` call is made using the same element-lookup technique you're used to using to call `update`. One of these lookups generally appear:
```python
window['-MULTILINE KEY-']
```
To change one of your normal prints to output to your multiline element, you simply add the above lookup expression to the front of your print statement.
```python
print('My variables are', a, b, c) # a normal print statement
window['-MULTILINE KEY-'].print('My variables are', a, b, c) # Routed to your multiline element
```
It gets even better though.... you can add color to your prints
```python
# Outputs red text on a yellow background
window['-MULTILINE KEY-'].print('My variables are', a, b, c, text_color='red', background_color='yellow')
```
### Redefine print
Another way to use this print capability is to redefine the `print` statement itself. This will allow you to leave your code entirely as is. By adding this line of code your entire program will output all printed information to a multiline element.
```python
print = lambda *args, **kwargs: window['-MULTILINE KEY-'].print(*args, **kwargs)
```
## Text Input Element | `InputText == Input == In`
```python
layout = [[sg.InputText('Default text')]]
```

---
#### Note about the `do_not_clear` parameter
This used to really trip people up, but don't think so anymore. The `do_not_clear` parameter is initialized when creating the InputText Element. If set to False, then the input field's contents will be erased after every `Window.read()` call. Use this setting for when your window is an "Input Form" type of window where you want all of the fields to be erased and start over again every time.
## Combo Element | `Combo == InputCombo == DropDown == Drop`
Also known as a drop-down list. Only required parameter is the list of choices. The return value is a string matching what's visible on the GUI.
```python
layout = [[sg.Combo(['choice 1', 'choice 2'])]]
```
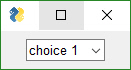
## Listbox Element
The standard listbox like you'll find in most GUIs. Note that the return values from this element will be a ***list of results, not a single result***. This is because the user can select more than 1 item from the list (if you set the right mode).
```python
layout = [[sg.Listbox(values=['Listbox 1', 'Listbox 2', 'Listbox 3'], size=(30, 6))]]
```
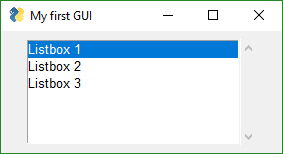
---
ListBoxes can cause a window to return from a Read call. If the flag `enable_events` is set, then when a user makes a selection, the Read immediately returns.
Another way ListBoxes can cause Reads to return is if the flag bind_return_key is set. If True, then if the user presses the return key while an entry is selected, then the Read returns. Also, if this flag is set, if the user double-clicks an entry it will return from the Read.
## Slider Element
Sliders have a couple of slider-specific settings as well as appearance settings. Examples include the `orientation` and `range` settings.
```python
layout = [[sg.Slider(range=(1,500),
default_value=222,
size=(20,15),
orientation='horizontal',
font=('Helvetica', 12))]]
```
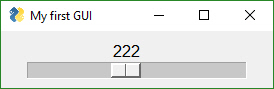
### Qt Sliders
There is an important difference between Qt and tkinter sliders. On Qt, the slider values must be integer, not float. If you want your slider to go from 0.1 to 1.0, then make your slider go from 1 to 10 and divide by 10. It's an easy math thing to do and not a big deal. Just deal with it.... you're writing software after all. Presumably you know how to do these things. ;-)
## Radio Button Element
Creates one radio button that is assigned to a group of radio buttons. Only 1 of the buttons in the group can be selected at any one time.
```python
layout = [
[sg.Radio('My first Radio!', "RADIO1", default=True),
sg.Radio('My second radio!', "RADIO1")]
]
```
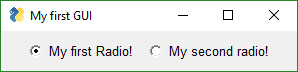
## Checkbox Element | `CBox == CB == Check`
Checkbox elements are like Radio Button elements. They return a bool indicating whether or not they are checked.
```python
layout = [[sg.Checkbox('My first Checkbox!', default=True), sg.Checkbox('My second Checkbox!')]]
```

## Spin Element
An up/down spinner control. The valid values are passed in as a list.
```python
layout = [[sg.Spin([i for i in range(1,11)], initial_value=1), sg.Text('Volume level')]]
```
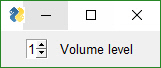
## Image Element
Images can be placed in your window provide they are in PNG, GIF, PPM/PGM format. JPGs cannot be shown because tkinter does not naively support JPGs. You can use the Python Imaging Library (PIL) package to convert your image to PNG prior to calling PySimpleGUI if your images are in JPG format.
```python
layout = [
[sg.Image(r'C:\PySimpleGUI\Logos\PySimpleGUI_Logo_320.png')],
]
```
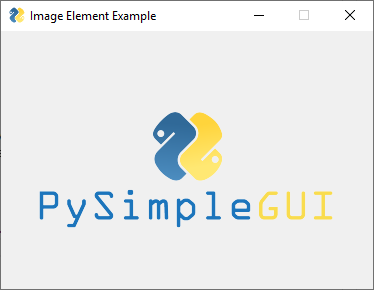
You can specify an animated GIF as an image and can animate the GIF by calling `UpdateAnimation`. Exciting stuff!
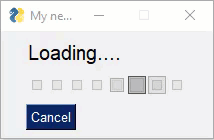
You can call the method without setting the `time_between_frames` value and it will show a frame and immediately move on to the next frame. This enables you to do the inter-frame timing.
## Button Element
Buttons are the most important element of all! They cause the majority of the action to happen. After all, it's a button press that will get you out of a window, whether it be Submit or Cancel, one way or another a button is involved in all windows. The only exception is to this is when the user closes the window using the "X" in the upper corner which means no button was involved.
The Types of buttons include:
* Folder Browse
* File Browse
* Files Browse
* File SaveAs
* File Save
* Close window (normal button)
* Read window
* Realtime
* Calendar Chooser
* Color Chooser
Close window - Normal buttons like Submit, Cancel, Yes, No, do NOT close the window... they used to. Now to close a window you need to use a CloseButton / CButton.
Folder Browse - When clicked a folder browse dialog box is opened. The results of the Folder Browse dialog box are written into one of the input fields of the window.
File Browse - Same as the Folder Browse except rather than choosing a folder, a single file is chosen.
Calendar Chooser - Opens a graphical calendar to select a date.
Color Chooser - Opens a color chooser dialog
Read window - This is a window button that will read a snapshot of all of the input fields, but does not close the window after it's clicked.
Realtime - This is another async window button. Normal button clicks occur after a button's click is released. Realtime buttons report a click the entire time the button is held down.
Most programs will use a combination of shortcut button calls (Submit, Cancel, etc.), normal Buttons which leave the windows open and CloseButtons that close the window when clicked.
Sometimes there are multiple names for the same function. This is simply to make the job of the programmer quicker and easier. Or they are old names that are no longer used but kept around so that existing programs don't break.
The 4 primary windows of PySimpleGUI buttons and their names are:
1. `Button`= `ReadButton` = `RButton` = `ReadFormButton` (Use `Button`, others are old methods)
2. `CloseButton` = `CButton`
3. `RealtimeButton`
4. `DummyButton`
You will find the long-form names in the older programs. ReadButton for example.
In Oct 2018, the definition of Button changed. Previously Button would CLOSE the window when clicked. It has been changed so the Button calls will leave the window open in exactly the same way as a ReadButton. They are the same calls now. To enables windows to be closed using buttons, a new button was added... `CloseButton` or `CButton`.
Your PySimpleGUI program is most likely going to contain only `Button` calls. The others are generally not found in user code.
The most basic Button element call to use is `Button`
```python
layout = [[sg.Button('Ok'), sg.Button('Cancel')]]
```
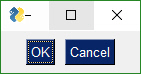
You will rarely see these 2 buttons in particular written this way. Recall that PySimpleGUI is focused on YOU (which generally directly means.... less typing). As a result, the code for the above window is normally written using shortcuts found in the next section.
You will typically see this instead of calls to `Button`:
```python
layout = [[sg.Ok(), sg.Cancel()]]
```
In reality `Button` is in fact being called on your behalf. Behind the scenes, `sg.Ok` and `sg.Cancel` call `Button` with the text set to `Ok` and `Cancel` and returning the results that then go into the layout. If you were to print the layout it will look identical to the first layout shown that has `Button` shown specifically in the layout.
### TTK Buttons & Macs
In 2019 support for ttk Buttons was added. This gets around the problem of not being able to change button colors on a Mac. There are a number of places you can control whether or not ttk buttons are used, be it on MAc or other platform.
TTK Buttons and TK Buttons operate slightly differently. Button highlighting is one different. How images and text are displayed at the same time is another. You've got options now that weren't there previously. It's nice to see that Mac users can finally use the color themes.
### Button Element Shortcuts
These Pre-made buttons are some of the most important elements of all because they are used so much. They all basically do the same thing, **set the button text to match the function name and set the parameters to commonly used values**. If you find yourself needing to create a custom button often because it's not on this list, please post a request on GitHub. . They include:
- OK
- Ok
- Submit
- Cancel
- Yes
- No
- Exit
- Quit
- Help
- Save
- SaveAs
- Open
### "Chooser" Buttons
These buttons are used to show dialog boxes that choose something like a filename, date, color, etc.. that are filled into an `InputText` Element (or some other "target".... see below regarding targets)
- CalendarButton
- ColorChooserButton
- FileBrowse
- FilesBrowse
- FileSaveAs
- FolderBrowse
**IMPORT NOTE ABOUT SHORTCUT BUTTONS**
Prior to release 3.11.0, these buttons closed the window. Starting with 3.11 they will not close the window. They act like RButtons (return the button text and do not close the window)
If you are having trouble with these buttons closing your window, please check your installed version of PySimpleGUI by typing `pip list` at a command prompt. Prior to 3.11 these buttons close your window.
Using older versions, if you want a Submit() button that does not close the window, then you would instead use RButton('Submit'). Using the new version, if you want a Submit button that closes the window like the sold Submit() call did, you would write that as `CloseButton('Submit')` or `CButton('Submit')`
### Button targets
The `FileBrowse`, `FolderBrowse`, `FileSaveAs` , `FilesSaveAs`, `CalendarButton`, `ColorChooserButton` buttons all fill-in values into another element located on the window. The target can be a Text Element or an InputText Element or the button itself. The location of the element is specified by the `target` variable in the function call.
The Target comes in two forms.
1. Key
2. (row, column)
Targets that are specified using a key will find its target element by using the target's key value. This is the "preferred" method.
If the Target is specified using (row, column) then it utilizes a grid system. The rows in your GUI are numbered starting with 0. The target can be specified as a hard coded grid item or it can be relative to the button.
The (row, col) targeting can only target elements that are in the same "container". Containers are the Window, Column and Frame Elements. A File Browse button located inside of a Column is unable to target elements outside of that Column.
The default value for `target` is `(ThisRow, -1)`. `ThisRow` is a special value that tells the GUI to use the same row as the button. The Y-value of -1 means the field one value to the left of the button. For a File or Folder Browse button, the field that it fills are generally to the left of the button is most cases. (ThisRow, -1) means the Element to the left of the button, on the same row.
If a value of `(None, None)` is chosen for the target, then the button itself will hold the information. Later the button can be queried for the value by using the button's key.
Let's examine this window as an example:
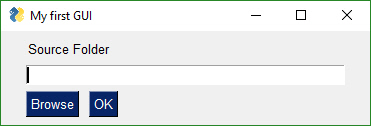
The `InputText` element is located at (1,0)... row 1, column 0. The `Browse` button is located at position (2,0). The Target for the button could be any of these values:
Target = (1,0)
Target = (-1,0)
The code for the entire window could be:
```python
layout = [[sg.T('Source Folder')],
[sg.In()],
[sg.FolderBrowse(target=(-1, 0)), sg.OK()]]
```
or if using keys, then the code would be:
```python
layout = [[sg.T('Source Folder')],
[sg.In(key='input')],
[sg.FolderBrowse(target='input'), sg.OK()]]
```
See how much easier the key method is?
#### Invisible Targets
One very handy trick is to make your target invisible. This will remove the ability to edit the chosen value like you normally would be able to with an Input Element. It's a way of making things look cleaner, less cluttered too perhaps.
### Save & Open Buttons
There are 4 different types of File/Folder open dialog box available. If you are looking for a file to open, the `FileBrowse` is what you want. If you want to save a file, `SaveAs` is the button. If you want to get a folder name, then `FolderBrowse` is the button to use. To open several files at once, use the `FilesBrowse` button. It will create a list of files that are separated by ';'
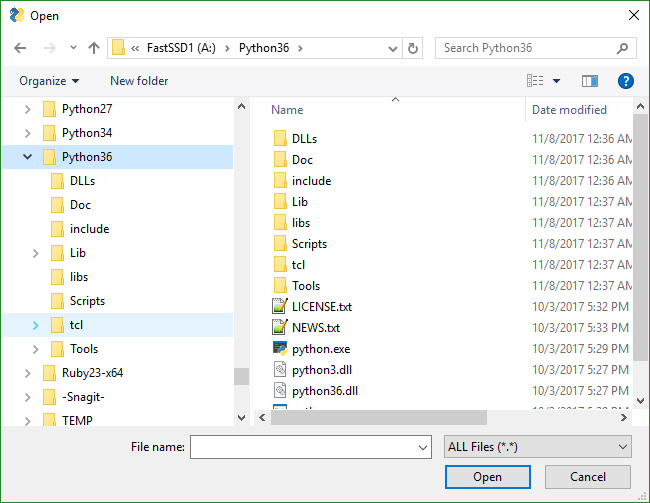
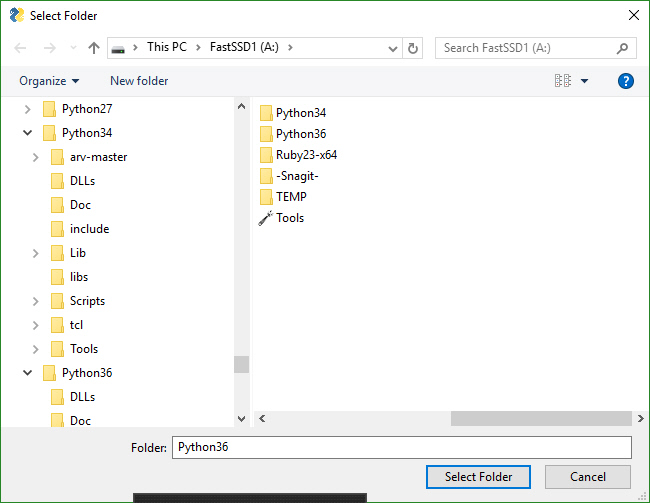
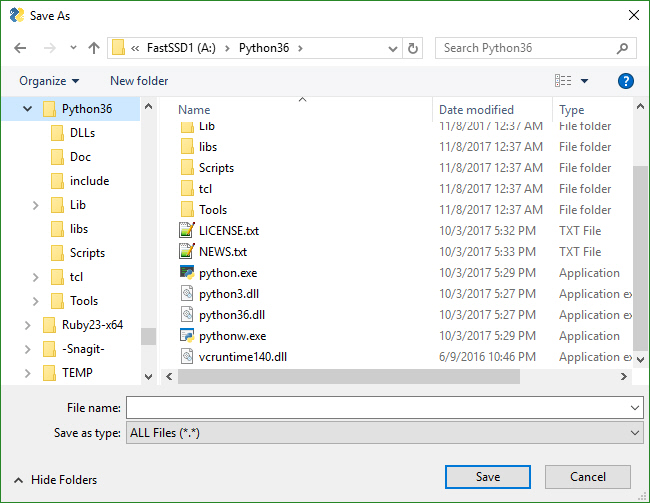
### Calendar Buttons
These buttons pop up a calendar chooser window. The chosen date is returned as a string.
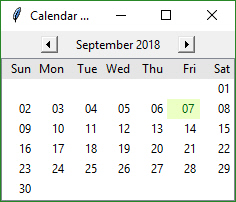
### Color Chooser Buttons
These buttons pop up a standard color chooser window. The result is returned as a tuple. One of the returned values is an RGB hex representation.
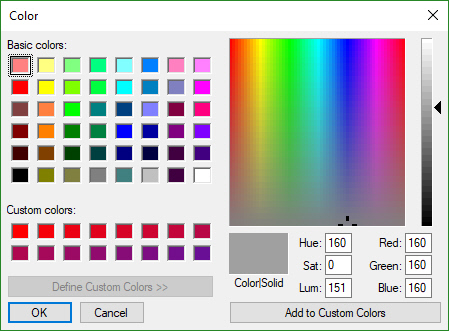
### Custom Buttons
Not all buttons are created equal. A button that closes a window is different that a button that returns from the window without closing it. If you want to define your own button, you will generally do this with the Button Element `Button`, which closes the window when clicked.
```python
layout = [[sg.Button('My Button')]]
```
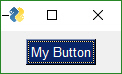
All buttons can have their text changed by changing the `button_text` parameter in the button call. It is this text that is returned when a window is read. This text will be what tells you which button was clicked. However, you can also use keys on your buttons so that they will be unique. If only the text were used, you would never be able to have 2 buttons in the same window with the same text.
```python
layout = [[sg.Button('My Button', key='_BUTTON_KEY_')]]
```
With this layout, the event that is returned from a `Window.read()` call when the button is clicked will be "`_BUTTON_KEY_`"
### Button Images
Now this is an exciting feature not found in many simplified packages.... images on buttons! You can make a pretty spiffy user interface with the help of a few button images.
This is one of the quickest and easiest ways to transform tkinter from a "1990s looking GUI" into a "modern GUI". If you don't like the default buttons, then simply bring your own button images and use those instead.
Your button images need to be in PNG or GIF format. When you make a button with an image, set the button background to the same color as the background. You can get the theme's background color by calling `theme_background_color()`
`TRANSPARENT_BUTTON` - **Important** - This is a legacy value that is misleading. It is currently defined as this constant value:
```python
TRANSPARENT_BUTTON = ('#F0F0F0', '#F0F0F0')
```
As you can see it is simply a tuple of 2 gray colors. The effect is that the button text and the button background color to a specific shade of gray. Way back in time, before you could change the background colors and all windows were gray, this value worked. But now that your button can be on any background color, you'll want to set the buttons color to match the background so that your button blends with the background color.
```python
sg.Button('Restart Song', button_color=(sg.theme_background_color(), sg.theme_background_color()),
image_filename=image_restart, image_size=(50, 50), image_subsample=2, border_width=0)
```
There are several parameters in `Button` elements that are used for button images.
```
image_filename - Filename of image. Can be a relative path
image_data - A Base64 image
image_size - Size of image in pixels
image_subsample - Amount to divide the size by. 2 means your image will be 1/2 the size. 3 means 1/3
```
Here's an example window made with button images.
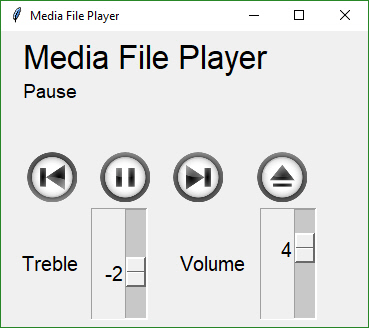
You'll find the source code in the file Demo Media Player. Here is what the button calls look like to create media player window
```python
sg.Button('Pause', button_color=(sg.theme_background_color(), sg.theme_background_color()),
image_filename=image_pause,
image_size=(50, 50),
image_subsample=2,
border_width=0)
```
Experimentation is sometimes required for these concepts to really sink in and they can vary depending on the underlying GUI framework.
Button Images do work so play with them. You can use PIL to change the size of your images before passing to PySimpleGUI.
### Realtime Buttons
Normally buttons are considered "clicked" when the mouse button is let UP after a downward click on the button. What about times when you need to read the raw up/down button values. A classic example for this is a robotic remote control. Building a remote control using a GUI is easy enough. One button for each of the directions is a start. Perhaps something like this:
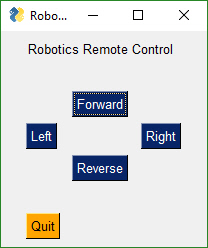
This window has 2 button types. There's the normal "Read Button" (Quit) and 4 "Realtime Buttons".
Here is the code to make, show and get results from this window:
```python
import PySimpleGUI as sg
gui_rows = [[sg.Text('Robotics Remote Control')],
[sg.T(' ' * 10), sg.RealtimeButton('Forward')],
[sg.RealtimeButton('Left'), sg.T(' ' * 15), sg.RealtimeButton('Right')],
[sg.T(' ' * 10), sg.RealtimeButton('Reverse')],
[sg.T('')],
[sg.Quit(button_color=('black', 'orange'))]
]
window = sg.Window('Robotics Remote Control', gui_rows)
#
# Some place later in your code...
# You need to perform a Read or Refresh call on your window every now and then or
# else it will apprear as if the program has locked up.
#
# your program's main loop
while (True):
# This is the code that reads and updates your window
event, values = window.read(timeout=50)
print(event)
if event in ('Quit', sg.WIN_CLOSED):
break
window.close() # Don't forget to close your window!
```
This loop will read button values and print them. When one of the Realtime buttons is clicked, the call to `window.read` will return a button name matching the name on the button that was depressed or the key if there was a key assigned to the button. It will continue to return values as long as the button remains depressed. Once released, the Read will return timeout events until a button is again clicked.
**File Types**
The `FileBrowse` & `SaveAs` buttons have an additional setting named `file_types`. This variable is used to filter the files shown in the file dialog box. The default value for this setting is
FileTypes=(("ALL Files", "*.*"),)
This code produces a window where the Browse button only shows files of type .TXT
layout = [[sg.In() ,sg.FileBrowse(file_types=(("Text Files", "*.txt"),))]]
NOTE - Mac users will not be able to use the file_types parameter. tkinter has a bug on Macs that will crash the program is a file_type is attempted so that feature had to be removed. Sorry about that!
***The ENTER key***
The ENTER key is an important part of data entry for windows. There's a long tradition of the enter key being used to quickly submit windows. PySimpleGUI implements this by tying the ENTER key to the first button that closes or reads a window.
The Enter Key can be "bound" to a particular button so that when the key is pressed, it causes the window to return as if the button was clicked. This is done using the `bind_return_key` parameter in the button calls.
If there are more than 1 button on a window, the FIRST button that is of type Close window or Read window is used. First is determined by scanning the window, top to bottom and left to right.
## ButtonMenu Element
The ButtonMenu element produces a unique kind of effect. It's a button, that when clicked, shows you a menu. It's like clicking one of the top-level menu items on a MenuBar. As a result, the menu definition take the format of a single menu entry from a normal menu definition. A normal menu definition is a list of lists. This definition is one of those lists.
```python
['Menu', ['&Pause Graph', 'Menu item::optional_key']]
```
The very first string normally specifies what is shown on the menu bar. In this case, the value is **not used**. You set the text for the button using a different parameter, the `button_text` parm.
One use of this element is to make a "fake menu bar" that has a colored background. Normal menu bars cannot have their background color changed. Not so with ButtonMenus.
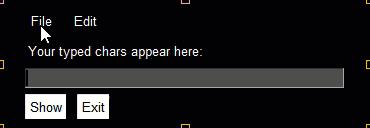
Return values for ButtonMenus are sent via the return values dictionary. If a selection is made, then an event is generated that will equal the ButtonMenu's key value. Use that key value to look up the value selected by the user. This is the same mechanism as the Menu Bar Element, but differs from the pop-up (right click) menu.
## VerticalSeparator Element
This element has limited usefulness and is being included more for completeness than anything else. It will draw a line between elements.
It works best when placed between columns or elements that span multiple rows. If on a "normal" row with elements that are only 1 row high, then it will only span that one row.
```python
VerticalSeparator(pad=None)
```
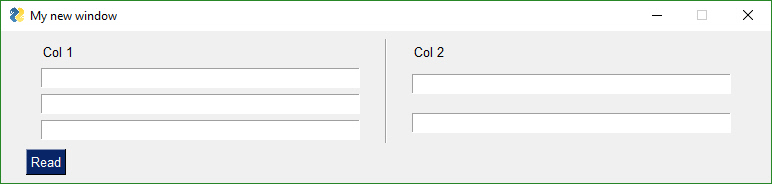
## HorizontalSeparator Element
In PySimpleGUI, the tkinter port, there is no `HorizontalSeparator` Element. One will be added as a "stub" so that code is portable. It will likely do nothing just like the `Stretch` Element.
An easy way to get a horizontal line in PySimpleGUI is to use a `Text` Element that contains a line of underscores
```python
sg.Text('_'*30) # make a horizontal line stretching 30 characters
```
## ProgressBar Element
The `ProgressBar` element is used to build custom Progress Bar windows. It is HIGHLY recommended that you use OneLineProgressMeter that provides a complete progress meter solution for you. Progress Meters are not easy to work with because the windows have to be non-blocking and they are tricky to debug.
The **easiest** way to get progress meters into your code is to use the `OneLineProgressMeter` API. This consists of a pair of functions, `OneLineProgressMeter` and `OneLineProgressMeterCancel`. You can easily cancel any progress meter by calling it with the current value = max value. This will mark the meter as expired and close the window.
You've already seen OneLineProgressMeter calls presented earlier in this readme.
```python
sg.OneLineProgressMeter('My Meter', i+1, 1000, 'key', 'Optional message')
```
The return value for `OneLineProgressMeter` is:
`True` if meter updated correctly
`False` if user clicked the Cancel button, closed the window, or vale reached the max value.
#### Progress Meter in Your window
Another way of using a Progress Meter with PySimpleGUI is to build a custom window with a `ProgressBar` Element in the window. You will need to run your window as a non-blocking window. When you are ready to update your progress bar, you call the `UpdateBar` method for the `ProgressBar` element itself.
```python
import PySimpleGUI as sg
# layout the window
layout = [[sg.Text('A custom progress meter')],
[sg.ProgressBar(1000, orientation='h', size=(20, 20), key='progressbar')],
[sg.Cancel()]]
# create the window`
window = sg.Window('Custom Progress Meter', layout)
progress_bar = window['progressbar']
# loop that would normally do something useful
for i in range(1000):
# check to see if the cancel button was clicked and exit loop if clicked
event, values = window.read(timeout=10)
if event == 'Cancel' or event == sg.WIN_CLOSED:
break
# update bar with loop value +1 so that bar eventually reaches the maximum
progress_bar.UpdateBar(i + 1)
# done with loop... need to destroy the window as it's still open
window.close()
```
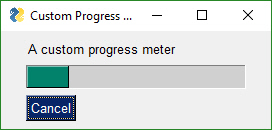
## Output Element
The Output Element is a re-direction of Stdout.
If you are looking for a way to quickly add the ability to show scrolling text within your window, then adding an `Output` Element is about as quick and easy as it gets.
**Anything "printed" will be displayed in this element.** This is the "trivial" way to show scrolling text in your window. It's as easy as dropping an Output Element into your window and then calling print as much as you want. The user will see a scrolling area of text inside their window.
***IMPORTANT*** You will NOT see what you `print` until you call either `window.read` or `window.Refresh`. If you want to immediately see what was printed, call `window.Refresh()` immediately after your print statement.
```python
Output(size=(80,20))
```
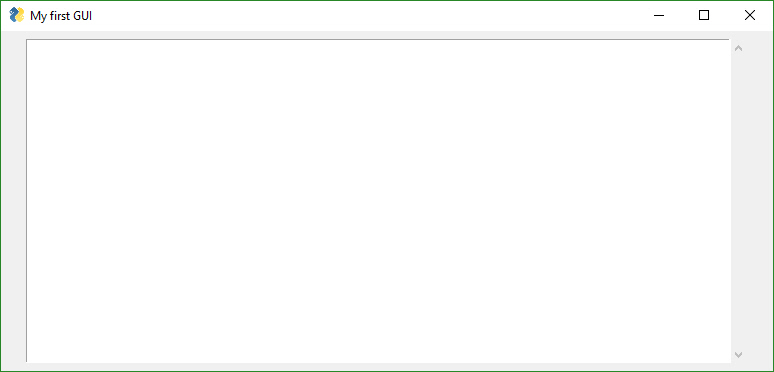
----
Here's a complete solution for a chat-window using an Output Element. To display data that's received, you would to simply "print" it and it will show up in the output area. You'll find this technique used in several Demo Programs including the HowDoI application.
```python
import PySimpleGUI as sg
def ChatBot():
layout = [[(sg.Text('This is where standard out is being routed', size=[40, 1]))],
[sg.Output(size=(80, 20))],
[sg.Multiline(size=(70, 5), enter_submits=True),
sg.Button('SEND', button_color=(sg.YELLOWS[0], sg.BLUES[0])),
sg.Button('EXIT', button_color=(sg.YELLOWS[0], sg.GREENS[0]))]]
window = sg.Window('Chat Window', layout, default_element_size=(30, 2))
# ---===--- Loop taking in user input and using it to query HowDoI web oracle --- #
while True:
event, value = window.read()
if event == 'SEND':
print(value)
else:
break
window.close()
ChatBot()
```
## Column Element & Frame, Tab "Container" Elements
Columns and Frames and Tabs are all "Container Elements" and behave similarly. This section focuses on Columns but can be applied elsewhere.
Starting in version 2.9 you'll be able to do more complex layouts by using the Column Element. Think of a Column as a window within a window. And, yes, you can have a Column within a Column if you want.
Columns are specified, like all "container elements", in exactly the same way as a window, as a list of lists.
Columns are needed when you want to specify more than 1 element in a single row.
For example, this layout has a single slider element that spans several rows followed by 7 `Text` and `Input` elements on the same row.
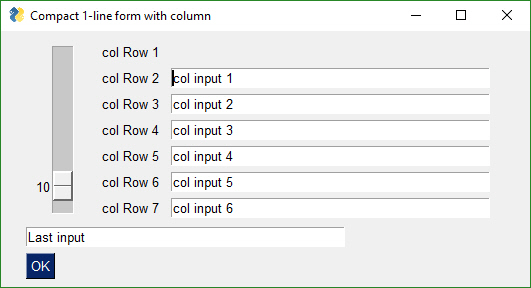
Without a Column Element you can't create a layout like this. But with it, you should be able to closely match any layout created using tkinter only.
```python
import PySimpleGUI as sg
# Demo of how columns work
# window has on row 1 a vertical slider followed by a COLUMN with 7 rows
# Prior to the Column element, this layout was not possible
# Columns layouts look identical to window layouts, they are a list of lists of elements.
window = sg.Window('Columns') # blank window
# Column layout
col = [[sg.Text('col Row 1')],
[sg.Text('col Row 2'), sg.Input('col input 1')],
[sg.Text('col Row 3'), sg.Input('col input 2')],
[sg.Text('col Row 4'), sg.Input('col input 3')],
[sg.Text('col Row 5'), sg.Input('col input 4')],
[sg.Text('col Row 6'), sg.Input('col input 5')],
[sg.Text('col Row 7'), sg.Input('col input 6')]]
layout = [[sg.Slider(range=(1,100), default_value=10, orientation='v', size=(8,20)), sg.Column(col)],
[sg.In('Last input')],
[sg.OK()]]
# Display the window and get values
window = sg.Window('Compact 1-line window with column', layout)
event, values = window.read()
window.close()
sg.Popup(event, values, line_width=200)
```
## Columns As a Way to Modify Elements
Sometimes Columns are used to contain a single elemnet, but to give that elemously it was difficult to do these kinds of layouts, if not impossible.
justify the `Column` element's row by setting the `Column`'s `justification` parameter.
You can also justify the entire contents within a `Column` by using the Column's `element_justification` parameter.
With these parameter's it is possible to create windows that have their contents centered. Previously this was very difficult to do.
This is currently only available in the primary PySimpleGUI port.
They can also be used to justify a group of elements in a particular way.
Placing `Column` elements inside `Columns` elements make it possible to create a multitude of
## Sizer Element
New in 4.3 is the `Sizer` Element. This element is used to help create a container of a particular size. It can be placed inside of these PySimpleGUI items:
* Column
* Frame
* Tab
* Window
The implementation of a `Sizer` is quite simple. It returns an empty `Column` element that has a pad value set to the values passed into the `Sizer`. Thus isn't not a class but rather a "Shortcut function" similar to the pre-defined Buttons.
This feature is only available in the tkinter port of PySimpleGUI at the moment. A cross port is needed.
----
## Frame Element (Labelled Frames, Frames with a title)
Frames work exactly the same way as Columns. You create layout that is then used to initialize the Frame. Like a Column element, it's a "Container Element" that holds one or more elements inside.
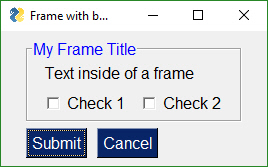
Notice how the Frame layout looks identical to a window layout. A window works exactly the same way as a Column and a Frame. They all are "container elements" - elements that contain other elements.
*These container Elements can be nested as deep as you want.* That's a pretty spiffy feature, right? Took a lot of work so be appreciative. Recursive code isn't trivial.
This code creates a window with a Frame and 2 buttons.
```python
frame_layout = [
[sg.T('Text inside of a frame')],
[sg.CB('Check 1'), sg.CB('Check 2')],
]
layout = [
[sg.Frame('My Frame Title', frame_layout, font='Any 12', title_color='blue')],
[sg.Submit(), sg.Cancel()]
]
window = sg.Window('Frame with buttons', layout, font=("Helvetica", 12))
```
## Canvas Element
In my opinion, the tkinter Canvas Widget is the most powerful of the tkinter widget. While I try my best to completely isolate the user from anything that is tkinter related, the Canvas Element is the one exception. It enables integration with a number of other packages, often with spectacular results.
However, there's another way to get that power and that's through the Graph Element, an even MORE powerful Element as it uses a Canvas that you can directly access if needed. The Graph Element has a large number of drawing methods that the Canvas Element does not have. Plus, if you need to, you can access the Graph Element's "Canvas" through a member variable.
### Matplotlib, Pyplot Integration
**NOTE - The newest version of Matplotlib (3.1.0) no longer works with this technique. ** You must install 3.0.3 in order to use the Demo Matplotlib programs provided in the Demo Programs section.
One such integration is with Matplotlib and Pyplot. There is a Demo program written that you can use as a design pattern to get an understanding of how to use the Canvas Widget once you get it.
def Canvas(canvas - a tkinter canvasf if you created one. Normally not set
background_color - canvas color
size - size in pixels
pad - element padding for packing
key - key used to lookup element
tooltip - tooltip text)
The order of operations to obtain a tkinter Canvas Widget is:
```python
figure_x, figure_y, figure_w, figure_h = fig.bbox.bounds
# define the window layout
layout = [[sg.Text('Plot test')],
[sg.Canvas(size=(figure_w, figure_h), key='canvas')],
[sg.OK(pad=((figure_w / 2, 0), 3), size=(4, 2))]]
# create the window and show it without the plot
window = sg.Window('Demo Application - Embedding Matplotlib In PySimpleGUI', layout).Finalize()
# add the plot to the window
fig_photo = draw_figure(window['canvas'].TKCanvas, fig)
# show it all again and get buttons
event, values = window.read()
```
To get a tkinter Canvas Widget from PySimpleGUI, follow these steps:
* Add Canvas Element to your window
* Layout your window
* Call `window.Finalize()` - this is a critical step you must not forget
* Find the Canvas Element by looking up using key
* Your Canvas Widget Object will be the found_element.TKCanvas
* Draw on your canvas to your heart's content
* Call `window.read()` - Nothing will appear on your canvas until you call Read
See `Demo_Matplotlib.py` for a Recipe you can copy.
### Methods & Properties
TKCanvas - not a method but a property. Returns the tkinter Canvas Widget
## Graph Element
All you math fans will enjoy this Element... and all you non-math fans will enjoy it even more.
I've found nothing to be less fun than dealing with a graphic's coordinate system from a GUI Framework. It's always upside down from what I want. (0,0) is in the upper left hand corner.... sometimes... or was it in the lower left? In short, it's a **pain in the ass**.
How about the ability to get your own location of (0,0) and then using those coordinates instead of what tkinter provides? This results in a very powerful capability - working in your own units, and then displaying them in an area defined in pixels.
If you've ever been frustrated with where (0,0) is located on some surface you draw on, then fear not, your frustration ends right here. You get to draw using whatever coordinate system you want. Place (0,0) anywhere you want, including not anywhere on your Graph. You could define a Graph that's all negative numbers between -2.1 and -3.5 in the X axis and -3 to -8.2 in the Y axis
There are 3 values you'll need to supply the Graph Element. They are:
- Size of the canvas in pixels
- The lower left (x,y) coordinate of your coordinate system
- The upper right (x,y) coordinate of your coordinate system
After you supply those values you can scribble all of over your graph by creating Graph Figures. Graph Figures are created, and a Figure ID is obtained by calling:
- DrawCircle
- DrawLine
- DrawPoint
- DrawRectangle
- DrawOval
- DrawImage
You can move your figures around on the canvas by supplying the Figure ID the **x,y delta** to move. It does not move to an absolute position, but rather an offset from where the figure is now. (Use Relocate to move to a specific location)
graph.MoveFigure(my_circle, 10, 10)
You'll also use this ID to delete individual figures you've drawn:
```python
graph.DeleteFigure(my_circle)
```
### Mouse Events Inside Graph Elements
If you have enabled events for your Graph Element, then you can receive mouse click events. If you additionally enable `drag_submits` in your creation of the Graph Element, then you will also get events when you "DRAG" inside of a window. A "Drag" is defined as a left button down and then the mouse is moved.
When a drag event happens, the event will be the Graph Element's key. The `value` returned in the values dictionary is a tuple of the (x,y) location of the mouse currently.
This means you'll get a "stream" of events. If the mouse moves, you'll get at LEAST 1 and likely a lot more than 1 event.
### Mouse Up Event for Drags
When you've got `drag_submits` enabled, there's a sticky situation that arises.... what happens when you're done dragging and you've let go of the mouse button? How is the "Mouse Up" event relayed back to your code.
The "Mouse Up" will generate an event to you with the value: `Graph_key` + `'+UP'`. Thus, if your Graph Element has a key of `'_GRAPH_'`, then the event you will receive when the mouse button is released is: `'_GRAPH_+UP'`
Yea, it's a little weird, but it works. It's SIMPLE too. I recommend using the `.startswith` and `.endswith` built-ins when dealing with these kinds of string values.
Here is an example of the `events` and the `values dictionary` that was generated by clicking and dragging inside of a Graph Element with the key == 'graph':
```
graph {'graph': (159, 256)}
graph {'graph': (157, 256)}
graph {'graph': (157, 256)}
graph {'graph': (157, 254)}
graph {'graph': (157, 254)}
graph {'graph': (154, 254)}
graph {'graph': (154, 254)}
graph+UP {'graph': (154, 254)}
```
## Table Element
Table and Tree Elements are of the most complex in PySimpleGUI. They have a lot of options and a lot of unusual characteristics.
### `window.read()` return values from Table Element
The values returned from a `Window.read` call for the Table Element are a list of row numbers that are currently highlighted.
### The Qt `Table.Get()` call
New in **PySimpleGUIQt** is the addition of the `Table` method `Get`. This method returns the table that is currently being shown in the GUI. This method was required in order to obtain any edits the user may have made to the table.
For the tkinter port, it will return the same values that was passed in when the table was created because tkinter Tables cannot be modified by the user (please file an Issue if you know a way).
### Known `Table` visualization problem....
There has been an elusive problem where clicking on or near the table's header caused tkinter to go crazy and resize the columns continuously as you moved the mouse.
This problem has existed since the first release of the `Table` element. It was fixed in release 4.3.
### Known table colors in Python 3.7.3, 3.7.4, 3.8, ?
The tkinter that's been released in the past several releases of Python has a bug. Table colors of all types are not working, at all. The background of the rows never change. If that's important to you, you'll need to **downgrade** your Python version. 3.6 works really well with PySimpleGUI and tkinter.
### Empty Tables
If you wish to start your table as being an empty one, you will need to specify an empty table. This list comprehension will create an empty table with 15 rows and 6 columns.
```python
data = [['' for row in range(15)]for col in range(6)]
```
### Events from Tables
There are two ways to get events generated from Table Element.
`change_submits` event generated as soon as a row is clicked on
`bind_return_key` event generate when a row is double clicked or the return key is press while on a row.
## Tree Element
The Tree Element and Table Element are close cousins. Many of the parameters found in the Table Element apply to Tree Elements. In particular the heading information, column widths, etc..
Unlike Tables there is no standard format for trees. Thus the data structure passed to the Tree Element must be constructed. This is done using the TreeData class. The process is as follows:
- Get a TreeData Object
- "Insert" data into the tree
- Pass the filled in TreeData object to Tree Element
#### TreeData format
```python
def TreeData()
def Insert(self, parent, key, text, values, icon=None)
```
To "insert" data into the tree the TreeData method Insert is called.
```python
Insert(parent_key, key, display_text, values)
```
To indicate insertion at the head of the tree, use a parent key of "". So, every top-level node in the tree will have a parent node = ""
This code creates a TreeData object and populates with 3 values
```python
treedata = sg.TreeData()
treedata.Insert("", '_A_', 'A', [1,2,3])
treedata.Insert("", '_B_', 'B', [4,5,6])
treedata.Insert("_A_", '_A1_', 'A1', ['can','be','anything'])
```
Note that you ***can*** use the same values for display_text and keys. The only thing you have to watch for is that you cannot repeat keys.
When Reading a window the Table Element will return a list of rows that are selected by the user. The list will be empty is no rows are selected.
#### Icons on Tree Entries
If you wish to show an icon next to a tree item, then you specify the icon in the call to `Insert`. You pass in a filename or a Base64 bytes string using the optional `icon` parameter.
Here is the result of showing an icon with a tree entry.
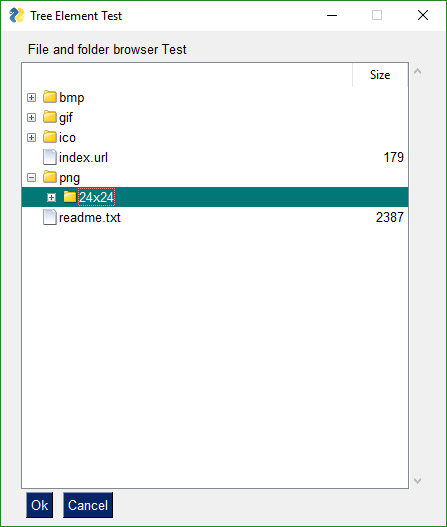
## Tab and Tab Group Elements
Tabs are another of PySimpleGUI "Container Elements". It is capable of "containing" a layout just as a window contains a layout. Other container elements include the `Column` and `Frame` elements.
Just like windows and the other container elements, the `Tab` Element has a layout consisting of any desired combination of Elements in any desired layouts. You can have Tabs inside of Tabs inside of Columns inside of Windows, etc..
`Tab` layouts look exactly like Window layouts, that is they are **a list of lists of Elements**.
*How you place a Tab element into a window is different than all other elements.* You cannot place a Tab directly into a Window's layout.
Also, tabs cannot be made invisible at this time. They have a visibility parameter but calling update will not change it.
Tabs are contained in TabGroups. They are **not** placed into other layouts. To get a Tab into your window, first place the `Tab` Element into a `TabGroup` Element and then place the `TabGroup` Element into the Window layout.
Let's look at this Window as an example:
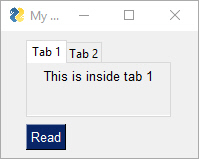
View of second tab:
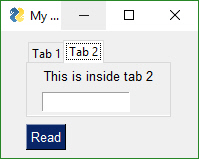
```python
tab1_layout = [[sg.T('This is inside tab 1')]]
tab2_layout = [[sg.T('This is inside tab 2')],
[sg.In(key='in')]]
```
The layout for the entire window looks like this:
```python
layout = [[sg.TabGroup([[sg.Tab('Tab 1', tab1_layout), sg.Tab('Tab 2', tab2_layout)]])],
[sg.Button('Read')]]
```
The Window layout has the TabGroup and within the tab Group are the two Tab elements.
One important thing to notice about all of these container Elements and Windows layouts... they all take a "list of lists" as the layout. They all have a layout that looks like this `[[ ]]`
You will want to keep this `[[ ]]` construct in your head a you're debugging your tabbed windows. It's easy to overlook one or two necessary ['s
As mentioned earlier, the old-style Tabs were limited to being at the Window-level only. In other words, the tabs were equal in size to the entire window. This is not the case with the "new-style" tabs. This is why you're not going to be upset when you discover your old code no longer works with the new PySimpleGUI release. It'll be worth the few moments it'll take to convert your code.
Check out what's possible with the NEW Tabs!
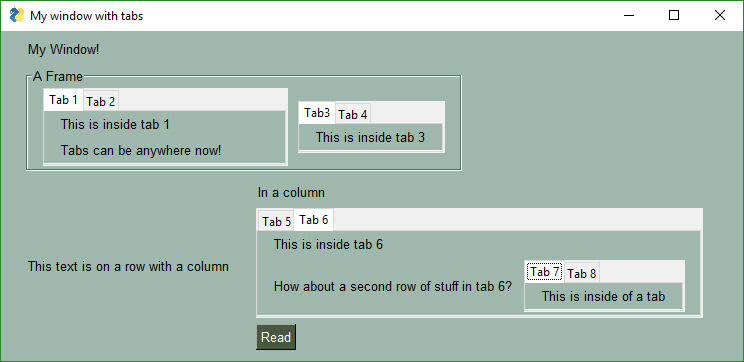
Check out Tabs 7 and 8. We've got a Window with a Column containing Tabs 5 and 6. On Tab 6 are... Tabs 7 and 8.
As of Release 3.8.0, not all of *options* shown in the API definitions of the Tab and TabGroup Elements are working. They are there as placeholders.
First we have the Tab layout definitions. They mirror what you see in the screen shots. Tab 1 has 1 Text Element in it. Tab 2 has a Text and an Input Element.
### Reading Tab Groups
Tab Groups now return a value when a Read returns. They return which tab is currently selected. There is also a `enable_events` parameter that can be set that causes a Read to return if a Tab in that group is selected / changed. The key or title belonging to the Tab that was switched to will be returned as the value
x## Pane Element
New in version 3.20 is the Pane Element, a super-cool tkinter feature. You won't find this one in PySimpleGUIQt, only PySimpleGUI. It's difficult to describe one of these things. Think of them as "Tabs without labels" that you can slide.
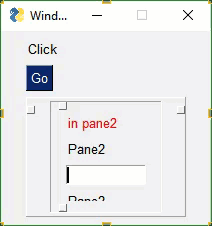
***Each "Pane" of a Pane Element must be a Column Element***. The parameter `pane_list` is a list of Column Elements.
Calls can get a little hairy looking if you try to declare everything in-line as you can see in this example.
```python
sg.Pane([col5, sg.Column([[sg.Pane([col1, col2, col4], handle_size=15, orientation='v', background_color=None, show_handle=True, visible=True, key='_PANE_', border_width=0, relief=sg.RELIEF_GROOVE),]]),col3 ], orientation='h', background_color=None, size=(160,160), relief=sg.RELIEF_RAISED, border_width=0)
```
Combing these with *visibility* make for an interesting interface with entire panes being hidden from view until neded by the user. It's one way of producing "dynamic" windows.
## Colors
Starting in version 2.5 you can change the background colors for the window and the Elements.
Your windows can go from this:
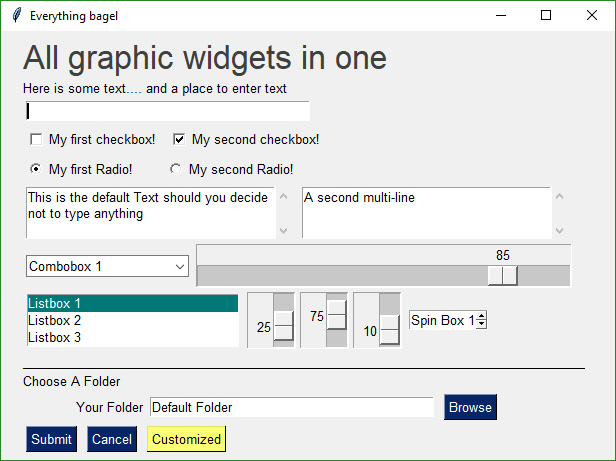
to this... with one function call...
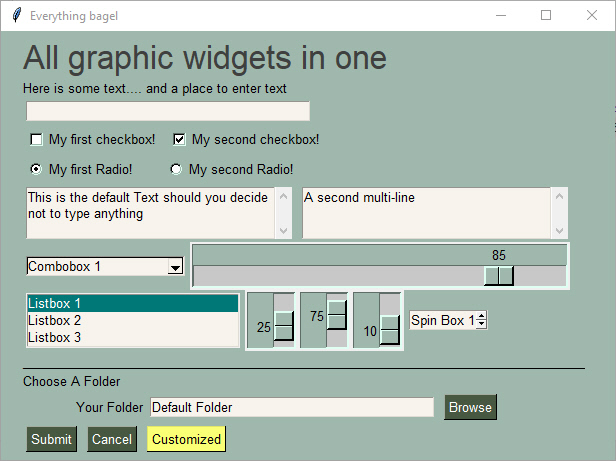
While you can do it on an element by element or window level basis, the easier way is to use either the `theme` calls or `set_options`. These calls will set colors for all window that are created.
Be aware that once you change these options they are changed for the rest of your program's execution. All of your windows will have that Theme, until you change it to something else.
This call sets a number of the different color options.
```python
SetOptions(background_color='#9FB8AD',
text_element_background_color='#9FB8AD',
element_background_color='#9FB8AD',
scrollbar_color=None,
input_elements_background_color='#F7F3EC',
progress_meter_color = ('green', 'blue')
button_color=('white','#475841'))
```
# SystemTray
In addition to running normal windows, it's now also possible to have an icon down in the system tray that you can read to get menu events. There is a new SystemTray object that is used much like a Window object. You first get one, then you perform Reads in order to get events.
## Tkinter version
While only PySimpleGUIQt and PySimpleGUIWx offer a true "system tray" feature, there is a simulated system tray feature that became available in 2020 for the tkinter version of PySimpleGUI. All of the same objects and method calls are the same and the effect is very similar to what you see with the Wx and Qt versions. The icon is placed in the bottom right corner of the window. Setting the location of it has not yet been exposed, but you can drag it to another location on your screen.
The idea of supporting Wx, Qt, and tkinter with the exact same source code is very appealing and is one of the reasons a tkinter version was developed. You can switch frameworks by simply changing your import statement to any of those 3 ports.
The balloons shown for the tkinter version is different than the message balloons shown by real system tray icons. Instead a nice semi-transparent window is shown. This window will fade in / out and is the same design as the one found in the [ptoaster package](https://github.com/PySimpleGUI/ptoaster).
## SystemTray Object
Here is the definition of the SystemTray object.
```python
SystemTray(menu=None, filename=None, data=None, data_base64=None, tooltip=None, metadata=None):
'''
SystemTray - create an icon in the system tray
:param menu: Menu definition
:param filename: filename for icon
:param data: in-ram image for icon
:param data_base64: basee-64 data for icon
:param tooltip: tooltip string
:param metadata: (Any) User metadata that can be set to ANYTHING
'''
```
You'll notice that there are 3 different ways to specify the icon image. The base-64 parameter allows you to define a variable in your .py code that is the encoded image so that you do not need any additional files. Very handy feature.
## System Tray Design Pattern
Here is a design pattern you can use to get a jump-start.
This program will create a system tray icon and perform a blocking Read. If the item "Open" is chosen from the system tray, then a popup is shown.
The same code can be executed on any of the Desktop versions of PySimpleGUI (tkinter, Qt, WxPython)
```python
import PySimpleGUIQt as sg
# import PySimpleGUIWx as sg
# import PySimpleGUI as sg
menu_def = ['BLANK', ['&Open', '---', '&Save', ['1', '2', ['a', 'b']], '&Properties', 'E&xit']]
tray = sg.SystemTray(menu=menu_def, filename=r'default_icon.ico')
while True: # The event loop
menu_item = tray.read()
print(menu_item)
if menu_item == 'Exit':
break
elif menu_item == 'Open':
sg.popup('Menu item chosen', menu_item)
```
The design pattern creates an icon that will display this menu:
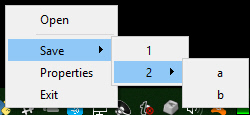
### Icons for System Trays
System Tray Icons are in PNG & GIF format when running on PySimpleGUI (tkinter version). PNG, GIF, and ICO formats will work for the Wx and Qt ports.
When specifying "icons", you can use 3 different formats.
* `filename`- filename
* `data_base64` - base64 byte string
* '`data` - in-ram bitmap or other "raw" image
You will find 3 parameters used to specify these 3 options on both the initialize statement and on the Update method.
For testing you may find using the built-in PySimpleGUI icon is a good place to start to make sure you've got everything coded correctly before bringing in outside image assets. It'll tell you quickly if you've got a problem with your icon file. To run using the default icon, use something like this to create the System Tray:
```python
tray = sg.SystemTray(menu=menu_def, data_base64=sg.DEFAULT_BASE64_ICON)
```
## Menu Definition
```python
menu_def = ['BLANK', ['&Open', '&Save', ['1', '2', ['a', 'b']], '!&Properties', 'E&xit']]
```
A menu is defined using a list. A "Menu entry" is a string that specifies:
* text shown
* keyboard shortcut
* key
See section on Menu Keys for more information on using keys with menus.
An entry without a key and keyboard shortcut is a simple string
`'Menu Item'`
If you want to make the "M" be a keyboard shortcut, place an `&` in front of the letter that is the shortcut.
`'&Menu Item'`
You can add "keys" to make menu items unique or as another way of identifying a menu item than the text shown. The key is added to the text portion by placing `::` after the text.
`'Menu Item::key'`
The first entry can be ignored.`'BLANK`' was chosen for this example. It's this way because normally you would specify these menus under some heading on a menu-bar. But here there is no heading so it's filled in with any value you want.
**Separators**
If you want a separator between 2 items, add the entry `'---'` and it will add a separator item at that place in your menu.
**Disabled menu entries**
If you want to disable a menu entry, place a `!` before the menu entry
## SystemTray Methods
### Read - Read the context menu or check for events
```python
def Read(timeout=None)
'''
Reads the context menu
:param timeout: Optional. Any value other than None indicates a non-blocking read
:return: String representing meny item chosen. None if nothing read.
'''
```
The `timeout` parameter specifies how long to wait for an event to take place. If nothing happens within the timeout period, then a "timeout event" is returned. These types of reads make it possible to run asynchronously. To run non-blocked, specify `timeout=0`on the Read call.
Read returns the menu text, complete with key, for the menu item chosen. If you specified `Open::key` as the menu entry, and the user clicked on `Open`, then you will receive the string `Open::key` upon completion of the Read.
#### Read special return values
In addition to Menu Items, the Read call can return several special values. They include:
EVENT_SYSTEM_TRAY_ICON_DOUBLE_CLICKED - Tray icon was double clicked
EVENT_SYSTEM_TRAY_ICON_ACTIVATED - Tray icon was single clicked
EVENT_SYSTEM_TRAY_MESSAGE_CLICKED - a message balloon was clicked
TIMEOUT_KEY is returned if no events are available if the timeout value is set in the Read call
### Hide
Hides the icon. Note that no message balloons are shown while an icon is hidden.
```python
def Hide()
```
### Close
Does the same thing as hide
```python
def Close()
```
### UnHide
Shows a previously hidden icon
```python
def UnHide()
```
### ShowMessage
Shows a balloon above the icon in the system tray area. You can specify your own icon to be shown in the balloon, or you can set `messageicon` to one of the preset values.
This message has a custom icon.
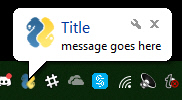
The preset `messageicon` values are:
SYSTEM_TRAY_MESSAGE_ICON_INFORMATION
SYSTEM_TRAY_MESSAGE_ICON_WARNING
SYSTEM_TRAY_MESSAGE_ICON_CRITICAL
SYSTEM_TRAY_MESSAGE_ICON_NOICON
```python
ShowMessage(title, message, filename=None, data=None, data_base64=None, messageicon=None, time=10000):
'''
Shows a balloon above icon in system tray
:param title: Title shown in balloon
:param message: Message to be displayed
:param filename: Optional icon filename
:param data: Optional in-ram icon
:param data_base64: Optional base64 icon
:param time: How long to display message in milliseconds :return:
'''
```
Note, on windows it may be necessary to make a registry change to enable message balloons to be seen. To fix this, you must create the DWORD you see in this screenshot.
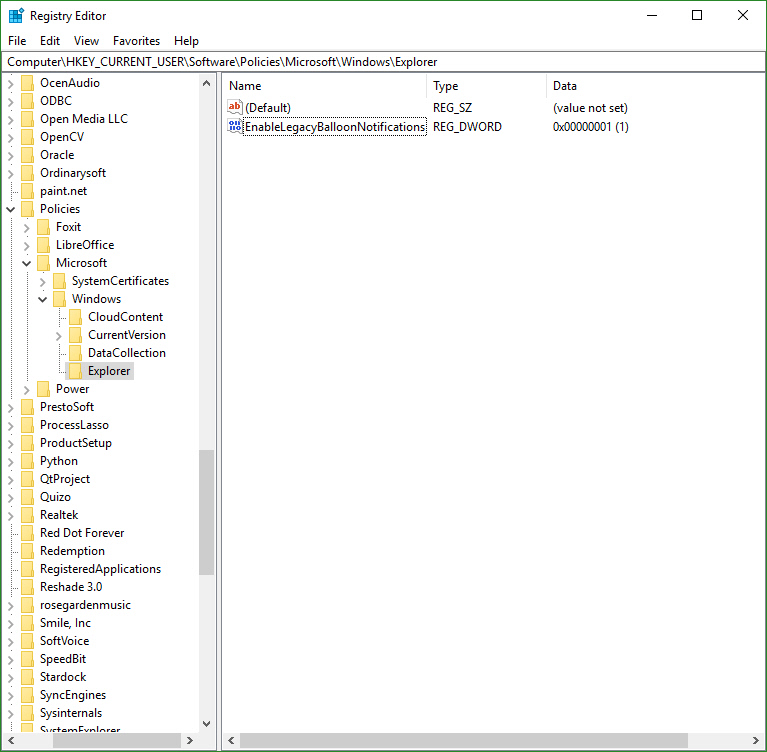
### Update
You can update any of these items within a SystemTray object
* Menu definition
* Icon
* Tooltip
Change them all or just 1.
### Notify Class Method
In addition to being able to show messages via the system tray, the tkinter port has the added capability of being able to display the system tray messages without having a system tray object defined. You can simply show a notification window. This perhaps removes the need for using the ptoaster package?
The method is a "class method" which means you can call it directly without first creating an instanciation of the object. To show a notification window, call `SystemTray.notify`.
This line of code
```python
sg.SystemTray.notify('Notification Title', 'This is the notification message')
```
Will show this window, fading it in and out:
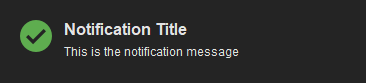
This is a blocking call so expect it to take a few seconds if you're fading the window in and out. There are options to control the fade, how long things are displayed, the alpha channel, etc.. See the call signature at the end of this document.
# Global Settings
There are multiple ways to customize PySimpleGUI. You can think of customizations as being done in a hierarchical manner
- Global
- Window
- Element
The function `set_options` is used to change settings that will apply globally. If it's a setting that applies to Windows, then that setting will apply not only to Windows that you create, but also to popup Windows.
Each lower level overrides the settings of the higher level. Once settings have been changed, they remain changed for the duration of the program (unless changed again).
After Global settings are settings made at Window level. These settings apply to a single `Window`. Fonts are a good example of a Windows-level setting. All elements within that `Window` will use the specified font.
The lowest level of setting is the element-level. It will modify one particular element's setting. Again using font as an example, if you set the `font` parameter on a `Text` element, then only that specific `Text` element will use the specified font.
# Persistent windows (Window stays open after button click)
Early versions of PySimpleGUI did not have a concept of "persisent window". Once a user clicked a button, the window would close. After some time, the functionality was expanded so that windows remained open by default.
## Input Fields that Auto-clear
Note that `InputText` and `MultiLine` Elements can be **cleared** when performing a `read`. If you want your input field to be cleared after a `window.read` then you can set the `do_not_clear` parameter to False when creating those elements. The clear is turned on and off on an element by element basis.
The reasoning behind this is that Persistent Windows are often "forms". When "submitting" a form you want to have all of the fields left blank so the next entry of data will start with a fresh window. Also, when implementing a "Chat Window" type of interface, after each read / send of the chat data, you want the input field cleared. Think of it as a Texting application. Would you want to have to clear your previous text if you want to send a second text?
## Basic Persistent Window Design Pattern
The design pattern for Persistent Windows was already shown to you earlier in the document... here it is for your convenience.
```python
import PySimpleGUI as sg
layout = [[sg.Text('Persistent window')],
[sg.Input()],
[sg.Button('Read'), sg.Exit()]]
window = sg.Window('Window that stays open', layout)
while True:
event, values = window.read()
print(event, values)
if event in (sg.WIN_CLOSED, 'Exit'):
break
window.close()
```
## read(timeout = t, timeout_key=TIMEOUT_KEY, close=False)
Read with a timeout is a very good thing for your GUIs to use in a non-blocking read situation. If your device can wait for a little while, then use this kind of read. The longer you're able to add to the timeout value, the less CPU time you'll be taking.
The idea to wait for some number of milliseconds before returning. It's a trivial way to make a window that runs on a periodic basis.
One way of thinking of reads with timeouts:
> During the timeout time, you are "yielding" the processor to do other tasks.
But it gets better than just being a good citizen....**your GUI will be more responsive than if you used a non-blocking read**
Let's say you had a device that you want to "poll" every 100ms. The "easy way out" and the only way out until recently was this:
```python
# YOU SHOULD NOT DO THIS....
while True: # Event Loop
event, values = window.ReadNonBlocking() # DO NOT USE THIS CALL ANYMORE
read_my_hardware() # process my device here
time.sleep(.1) # sleep 1/10 second DO NOT PUT SLEEPS IN YOUR EVENT LOOP!
```
This program will quickly test for user input, then deal with the hardware. Then it'll sleep for 100ms, while your gui is non-responsive, then it'll check in with your GUI again.
The better way using PySimpleGUI... using the Read Timeout mechanism, the sleep goes away.
```python
# This is the right way to poll for hardware
while True: # Event Loop
event, values = window.read(timeout = 100)
read_my_hardware() # process my device here
```
This event loop will run every 100 ms. You're making a `read` call, so anything that the use does will return back to you immediately, and you're waiting up to 100ms for the user to do something. If the user doesn't do anything, then the read will timeout and execution will return to the program.
## sg.TIMEOUT_KEY
If you're using a read with a timeout value, then an event value of None signifies that the window was closed, just like a normal `window.read`. That leaves the question of what it is set to when not other events are happening. This value will be the value of `TIMEOUT_KEY`. If you did not specify a timeout_key value in your call to read, then it will be set to a default value of:
`TIMEOUT_KEY = __timeout__`
If you wanted to test for "no event" in your loop, it would be written like this:
```python
while True:
event, value = window.read(timeout=10)
if event == sg.WIN_CLOSED:
break # the use has closed the window
if event == sg.TIMEOUT_KEY:
print("Nothing happened")
```
Use async windows sparingly. It's possible to have a window that appears to be async, but it is not. **Please** try to find other methods before going to async windows. The reason for this plea is that async windows poll tkinter over and over. If you do not have a timeout in your Read and you've got nothing else your program will block on, then you will eat up 100% of the CPU time. It's important to be a good citizen. Don't chew up CPU cycles needlessly. Sometimes your mouse wants to move ya know?
### `read(timeout=0)`
You may find some PySimpleGUI programs that set the timeout value to zero. This is a very dangerous thing to do. If you do not pend on something else in your event loop, then your program will consume 100% of your CPU. Remember that today's CPUs are multi-cored. You may see only 7% of your CPU is busy when you're running with timeout of 0. This is because task manager is reporting a system-wide CPU usage. The single core your program is running on is likely at 100%.
A true non-blocking (timeout=0) read is generally reserved as a "last resort". Too many times people use non-blocking reads when a blocking read will do just fine or a read with a timeout would work.
It's valid to use a timeout value of zero if you're in need of every bit of CPU horsepower in your application. Maybe your loop is doing something super-CPU intensive and you can't afford for the GUI to use any CPU time. This is the kind of situation where a timeout of zero is appropriate.
Be a good computing citizen. Run with a non-zero timeout so that other programs on your CPU will have time to run.
### Small Timeout Values (under 10ms)
***Do Not*** use a timeout of less than 10ms. Otherwise you will simply thrash, spending your time trying to do some GUI stuff, only to be interruped by a timeout timer before it can get anything done. The results are potentially disasterous.
There is a hybrid approach... a read with a timeout. You'll score much higher points on the impressive meter if you're able to use a lot less CPU time by using this type of read.
The most legit time to use a non-blocking window is when you're working directly with hardware. Maybe you're driving a serial bus. If you look at the Event Loop in the Demo_OpenCV_Webcam.py program, you'll see that the read is a non-blocking read. However, there is a place in the event loop where blocking occurs. The point in the loop where you will block is the call to read frames from the webcam. When a frame is available you want to quickly deliver it to the output device, so you don't want your GUI blocking. You want the read from the hardware to block.
Another example can be found in the demo for controlling a robot on a Raspberry Pi. In that application you want to read the direction buttons, forward, backward, etc., and immediately take action. If you are using RealtimeButtons, your only option at the moment is to use non-blocking windows. You have to set the timeout to zero if you want the buttons to be real-time responsive.
However, with these buttons, adding a sleep to your event loop will at least give other processes time to execute. It will, however, starve your GUI. The entire time you're sleeping, your GUI isn't executing.
### Periodically Calling`Read`
Let's say you do end up using non-blocking reads... then you've got some housekeeping to do. It's up to you to periodically "refresh" the visible GUI. The longer you wait between updates to your GUI the more sluggish your windows will feel. It is up to you to make these calls or your GUI will freeze.
There are 2 methods of interacting with non-blocking windows.
1. Read the window just as you would a normal window
2. "Refresh" the window's values without reading the window. It's a quick operation meant to show the user the latest values
With asynchronous windows the window is shown, user input is read, but your code keeps right on chugging. YOUR responsibility is to call `PySimpleGUI.read` on a periodic basis. Several times a second or more will produce a reasonably snappy GUI.
## Exiting (Closing) a Persistent Window
If your window has a special button that closes the window, then PySimpleGUI will automatically close the window for you. If all of your buttons are normal `Button` elements, then it'll be up to you to close the window when done.
To close a window, call the `close` method.
```python
window.close()
```
Beginning in version 4.16.0 you can use a `close` parameter in the `window.read` call to indicate that the window should be closed before returning from the read. This capability to an excellent way to make a single line Window to quickly get information.
This single line of code will display a window, get the user's input, close the window, and return the values as an event and a values dictionary.
```python
event, values = sg.Window('Login Window',
[[sg.T('Enter your Login ID'), sg.In(key='-ID-')],
[sg.B('OK'), sg.B('Cancel') ]]).read(close=True)
login_id = values['-ID-']
```
You can also make a custom popup quite easily:
```python
long_string = '123456789 '* 40
event, values = sg.Window('This is my customn popup',
[[sg.Text(long_string, size=(40,None))],
[sg.B('OK'), sg.B('Cancel') ]]).read(close=True)
```
Notice the height parameter of size is `None` in this case. For the tkinter port of PySimpleGUI this will cause the number of rows to "fit" the contents of the string to be displayed.
## Persistent Window Example - Running timer that updates
See the sample code on the GitHub named Demo Media Player for another example of Async windows. We're going to make a window and update one of the elements of that window every .01 seconds. Here's the entire code to do that.
```python
import PySimpleGUI as sg
import time
# ---------------- Create Form ----------------
sg.theme('Black')
sg.set_options(element_padding=(0, 0))
layout = [[sg.Text('')],
[sg.Text(size=(8, 2), font=('Helvetica', 20), justification='center', key='text')],
[sg.Button('Pause', key='button', button_color=('white', '#001480')),
sg.Button('Reset', button_color=('white', '#007339'), key='Reset'),
sg.Exit(button_color=('white', 'firebrick4'), key='Exit')]]
window = sg.Window('Running Timer', layout, no_titlebar=True, auto_size_buttons=False, keep_on_top=True, grab_anywhere=True)
# ---------------- main loop ----------------
current_time = 0
paused = False
start_time = int(round(time.time() * 100))
while (True):
# --------- Read and update window --------
event, values = window.read(timeout=10)
current_time = int(round(time.time() * 100)) - start_time
# --------- Display timer in window --------
window['text'].update('{:02d}:{:02d}.{:02d}'.format((current_time // 100) // 60,
(current_time // 100) % 60,
current_time % 100))
```
Previously this program was implemented using a sleep in the loop to control the clock tick. This version uses the new timeout parameter. The result is a window that reacts quicker then the one with the sleep and the accuracy is just as good.
## Instead of a Non-blocking Read --- Use `enable_events = True` or `return_keyboard_events = True`
Any time you are thinking "I want an X Element to cause a Y Element to do something", then you want to use the `enable_events` option.
***Instead of polling, try options that cause the window to return to you.*** By using non-blocking windows, you are *polling*. You can indeed create your application by polling. It will work. But you're going to be maxing out your processor and may even take longer to react to an event than if you used another technique.
**Examples**
One example is you have an input field that changes as you press buttons on an on-screen keypad.
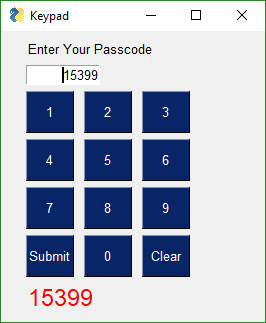
# Updating Elements (changing element's values in an active window)
If you want to change an Element's settings in your window after the window has been created, then you will call the Element's Update method.
**NOTE** a window **must be Read or Finalized** before any Update calls can be made. Also, not all settings available to you when you created the Element are available to you via its `update` method.
Here is an example of updating a Text Element
```python
import PySimpleGUI as sg
layout = [ [sg.Text('My layout', key='-TEXT-')],
[sg.Button('Read')]]
window = sg.Window('My new window', layout)
while True: # Event Loop
event, values = window.read()
if event == sg.WIN_CLOSED:
break
window['-TEXT-'].update('My new text value')
```
Notice the placement of the Update call. If you wanted to Update the Text Element *prior* to the Read call, outside of the event loop, then you must call Finalize on the window first.
In this example, the Update is done prior the Read. Because of this, the Finalize call is added to the Window creation.
```python
import PySimpleGUI as sg
layout = [ [sg.Text('My layout', key='-TEXT-')],
[sg.Button('Read')]]
window = sg.Window('My new window', layout, finalize=True)
window['-TEXT-'].update('My new text value')
while True: # Event Loop
event, values = window.read()
if event == sg.WIN_CLOSED:
break
```
Persistent windows remain open and thus continue to interact with the user after the Read has returned. Often the program wishes to communicate results (output information) or change an Element's values (such as populating a List Element).
You can use Update to do things like:
* Have one Element (appear to) make a change to another Element
* Disable a button, slider, input field, etc.
* Change a button's text
* Change an Element's text or background color
* Add text to a scrolling output window
* Change the choices in a list
* etc.
The way this is done is via an Update method that is available for nearly all of the Elements. Here is an example of a program that uses a persistent window that is updated.
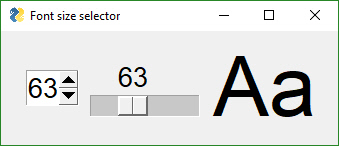
In some programs these updates happen in response to another Element. This program takes a Spinner and a Slider's input values and uses them to resize a Text Element. The Spinner and Slider are on the left, the Text element being changed is on the right.
```python
# Testing async window, see if can have a slider
# that adjusts the size of text displayed
import PySimpleGUI as sg
fontSize = 12
layout = [[sg.Spin([sz for sz in range(6, 172)], font=('Helvetica 20'), initial_value=fontSize, change_submits=True, key='spin'),
sg.Slider(range=(6,172), orientation='h', size=(10,20),
change_submits=True, key='slider', font=('Helvetica 20')),
sg.Text("Aa", size=(2, 1), font="Helvetica " + str(fontSize), key='text')]]
sz = fontSize
window = sg.Window("Font size selector", layout, grab_anywhere=False)
# Event Loop
while True:
event, values= window.read()
if event == sg.WIN_CLOSED:
break
sz_spin = int(values['spin'])
sz_slider = int(values['slider'])
sz = sz_spin if sz_spin != fontSize else sz_slider
if sz != fontSize:
fontSize = sz
font = "Helvetica " + str(fontSize)
window['text'].update(font=font)
window['slider'].update(sz)
window['spin'].update(sz)
print("Done.")
```
Inside the event loop we read the value of the Spinner and the Slider using those Elements' keys.
For example, `values['slider']` is the value of the Slider Element.
This program changes all 3 elements if either the Slider or the Spinner changes. This is done with these statements:
```python
window['text'].update(font=font)
window['slider'].update(sz)
window['spin'].update(sz)
```
Remember this design pattern because you will use it OFTEN if you use persistent windows.
It works as follows. The expression `window[key]` returns the Element object represented by the provided `key`. This element is then updated by calling it's `update` method. This is another example of Python's "chaining" feature. We could write this code using the long-form:
text_element = window['text']
text_element.update(font=font)
The takeaway from this exercise is that keys are key in PySimpleGUI's design. They are used to both read the values of the window and also to identify elements. As already mentioned, they are used in a wide variety of places.
### Locating Elements (FindElement == Element == Elem == [ ])
The Window method call that's used to find an element is:
`FindElement`
or the shortened version
`Element`
or even shorter (version 4.1+)
`Elem`
And now it's finally shortened down to:
window[key]
You'll find the pattern - `window.Element(key)` in older code. All of code after about 4.0 uses the shortened `window[key]` notation.
### ProgressBar / Progress Meters
Note that to change a progress meter's progress, you call `update_bar`, not `update`. A change to this is being considered for a future release.
# Cursors - Setting for Elements and Windows
It is possible to change the normal arrow cursor into something else by setting the cursor for an element or the entire window. The result will be the cursor changing when you move the mouse over the elements or Window.
One of the best examples is URLs. Users are accustomed to seeing a hand cursor when the mouse is moved over a link. By setting the cursor to a hand for a Text element that has text that is in the format of a URL, it signals to the user that it's a link that can be clicked.
The `set_cursor` method is used to set the cursor for an element. Perform an element look-up or use a variable containing an element, and call the `set_cursor` method, passing in a string that selects the cursor. The valid cursor names are documented in the tkinter docs as this call maps directly to a tkinter call.
These cursor strings were obtained from the Tk manual and are what you pass into the `set_cursor` methods.
## Windows Level Cursor
You can also set the cursor for the Window as a whole, including the margins and areas elements don't directly fill. Call `Window.set_cursor()` to set the cursor at the Window level.
## Valid Cursor Strings
```
X_cursor
arrow
based_arrow_down
based_arrow_up
boat
bogosity
bottom_left_corner
bottom_right_corner
bottom_side
bottom_tee
box_spiral
center_ptr
circle
clock
coffee_mug
cross
cross_reverse
crosshair
diamond_cross
dot
dotbox
double_arrow
draft_large
draft_small
draped_box
exchange
fleur
gobbler
gumby
hand1
hand2
heart
icon
iron_cross
left_ptr
left_side
left_tee
leftbutton
ll_angle
lr_angle
man
middlebutton
mouse
pencil
pirate
plus
question_arrow
right_ptr
right_side
right_tee
rightbutton
rtl_logo
sailboat
sb_down_arrow
sb_h_double_arrow
sb_left_arrow
sb_right_arrow
sb_up_arrow
sb_v_double_arrow
shuttle
sizing
spider
spraycan
star
target
tcross
top_left_arrow
top_left_corner
top_right_corner
top_side
top_tee
trek
ul_angle
umbrella
ur_angle
watch
xterm
```
## No Cursor
If you want your mouse cursor to be invisible, then use the **string** `"none"` and your element or window will not show any cursor.
## Windows OS Specific
One windows, these cursors map to native Windows cursors:
```
arrow
center_ptr
crosshair
fleur
ibeam
icon
sb_h_double_arrow
sb_v_double_arrow
watch
xterm
```
These are also available:
```
no
starting
size
size_ne_sw
size_ns
size_nw_se
size_we
uparrow
wait
```
## Mac OS Specific
```
arrow
cross
crosshair
ibeam
plus
watch
xterm
```
These additional native cursors are available for the Mac
```
copyarrow
aliasarrow
contextualmenuarrow
text
cross-hair
closedhand
openhand
pointinghand
resizeleft
resizeright
resizeleftright
resizeup
resizedown
resizeupdown
none
notallowed
poof
countinguphand
countingdownhand
countingupanddownhand
spinning
```
# Keyboard & Mouse Capture
NOTE - keyboard capture is currently formatted uniquely among the ports. For basic letters and numbers there is no great differences, but when you start adding Shift and Control or special keyus, they all behave slightly differently. Your best bet is to simply print what is being returned to you to determine what the format for the particular port is.
Beginning in version 2.10 you can capture keyboard key presses and mouse scroll-wheel events. Keyboard keys can be used, for example, to detect the page-up and page-down keys for a PDF viewer. To use this feature, there's a boolean setting in the Window call `return_keyboard_events` that is set to True in order to get keys returned along with buttons.
Keys and scroll-wheel events are returned in exactly the same way as buttons.
For scroll-wheel events, if the mouse is scrolled up, then the `button` text will be `MouseWheel:Up`. For downward scrolling, the text returned is `MouseWheel:Down`
Keyboard keys return 2 types of key events. For "normal" keys (a,b,c, etc.), a single character is returned that represents that key. Modifier and special keys are returned as a string with 2 parts:
Key Sym:Key Code
Key Sym is a string such as 'Control_L'. The Key Code is a numeric representation of that key. The left control key, when pressed will return the value 'Control_L:17'
```python
import PySimpleGUI as sg
# Recipe for getting keys, one at a time as they are released
# If want to use the space bar, then be sure and disable the "default focus"
text_elem = sg.Text(size=(18, 1))
layout = [[sg.Text("Press a key or scroll mouse")],
[text_elem],
[sg.Button("OK")]]
window = sg.Window("Keyboard Test", layout, return_keyboard_events=True, use_default_focus=False)
# ---===--- Loop taking in user input --- #
while True:
event, value = window.read()
if event == "OK" or event == sg.WIN_CLOSED:
print(event, "exiting")
break
text_elem.update(event)
```
You want to turn off the default focus so that there no buttons that will be selected should you press the spacebar.
# Menus
## MenuBar
Beginning in version 3.01 you can add a MenuBar to your window. You specify the menus in much the same way as you do window layouts, with lists. Menu selections are returned as events and as of 3.17, also as in the values dictionary. The value returned will be the entire menu entry, including the key if you specified one.
```python
menu_def = [['File', ['Open', 'Save', 'Exit',]],
['Edit', ['Paste', ['Special', 'Normal',], 'Undo'],],
['Help', 'About...'],]
```
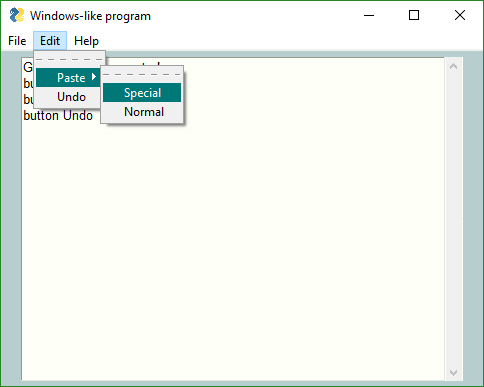
Note the placement of ',' and of []. It's tricky to get the nested menus correct that implement cascading menus. See how paste has Special and Normal as a list after it. This means that Paste has a cascading menu with items Special and Normal.
## Methods
---
To add a menu to a Window place the `Menu` or `MenuBar` element into your layout.
layout = [[sg.Menu(menu_def)]]
It doesn't really matter where you place the Menu Element in your layout as it will always be located at the top of the window.
When the user selects an item, it's returns as the event (along with the menu item's key if one was specified in the menu definition)
## ButtonMenus
Button menus were introduced in version 3.21, having been previously released in PySimpleGUIQt. They work exactly the same and are source code compatible between PySimpleGUI and PySimpleGUIQt. These types of menus take a single menu entry where a Menu Bar takes a list of menu entries.
**Return values for ButtonMenus are different than Menu Bars.**
You will get back the ButtonMenu's KEY as the event. To get the actual item selected, you will look it up in the values dictionary. This can be done with the expression `values[event]`
## Right Click Menus
Right Click Menus were introduced in version 3.21. Almost every element has a right_click_menu parameter and there is a window-level setting for rich click menu that will attach a right click menu to all elements in the window.
The menu definition is the same as the button menu definition, a single menu entry.
```python
right_click_menu = ['&Right', ['Right', '!&Click', '&Menu', 'E&xit', 'Properties']]
```
The first string in a right click menu and a button menu is ***ignored***. It is not used. Normally you would put the string that is shown on the menu bar in that location.
**Return values for right click menus are the same as MenuBars.** The value chosen is returned as the event.
## Menu Shortcut keys
You have used ALT-key in other Windows programs to navigate menus. For example Alt-F+X exits the program. The Alt-F pulls down the File menu. The X selects the entry marked Exit.
The good news is that PySimpleGUI allows you to create the same kind of menus! Your program can play with the big-boys. And, it's trivial to do.
All that's required is for your to add an "&" in front of the letter you want to appear with an underscore. When you hold the Alt key down you will see the menu with underlines that you marked.
One other little bit of polish you can add are separators in your list. To add a line in your list of menu choices, create a menu entry that looks like this: ` '---'`
This is an example Menu with underlines and a separator.
```
# ------ Menu Definition ------ #
menu_def = [['&File', ['&Open', '&Save', '---', 'Properties', 'E&xit' ]],
['&Edit', ['Paste', ['Special', 'Normal',], 'Undo'],],
['&Help', '&About...'],]
```
And this is the spiffy menu it produced:
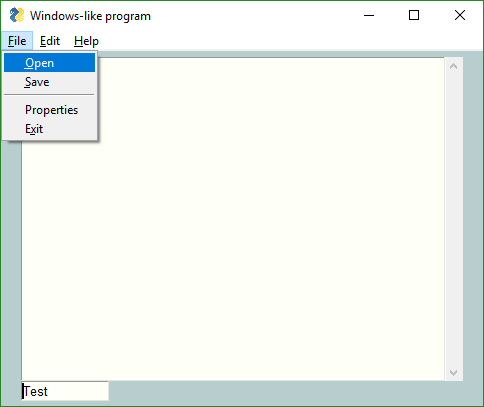
## Disabled Menu Entries
If you want one of your menu items to be disabled, then place a '!' in front of the menu entry. To disable the Paste menu entry in the previous examples, the entry would be:
`['!&Edit', ['Paste', ['Special', 'Normal',], 'Undo'],]`
If your want to change the disabled menu item flag / character from '!' to something else, change the variable `MENU_DISABLED_CHARACTER`
## Keys for Menus
Beginning in version 3.17 you can add a `key` to your menu entries. The `key` value will be removed prior to be inserted into the menu. When you receive Menu events, the entire menu entry, including the `key` is returned. A key is indicated by adding `::` after a menu entry, followed by the key.
To add the `key` `_MY_KEY_` to the Special menu entry, the code would be:
`['&Edit', ['Paste', ['Special::_MY_KEY_', 'Normal',], 'Undo'],]`
If you want to change the characters that indicate a key follows from '::' to something else, change the variable `MENU_KEY_SEPARATOR`
## The Menu Definitions
Having read through the Menu section, you may have noticed that the right click menu and the button menu have a format that is a little odd as there is a part of it that is not utilized (the first very string). Perhaps the words "Not Used" should be in the examples.... But, there's a reason to retain words there that make sense.
The reason for this is an architectural one, but it also has a convienence for the user. You can put the individual menu items (button and right click) into a list and you'll have a menu bar definition.
This would work to make a menu bar from a series of these individual menu defintions:
```python
menu_bar = [right_click_menu_1, right_click_menu_2, button_menu_def ]
```
And, of course, the direction works the opposite too. You can take a Menu Bar definition and pull out an individual menu item to create a right click or button menu.
# TTK & TTK Scrollbars
In version 4.60.0 all of the scrollbars in PySimpleGUI were converted into TTK scrollbars. This change enables all parts of a PySimpleGUI to match the theme. Additionally, scrollbars can be ***further*** themed by changing the TTK Theme.
TTK Themes have been available for use in PySimpleGUI for years. The `Window` object has a parameter to indicate which theme should be used for the window. `set_options` has also been a way to set the theme for your application.
## TTK in Global Settings
A new third way to set the TTK theme was added in the 4.60.0 release, the PySimpleGUI Global Settings. There is a tab dedicated to TTK in the global settings window.
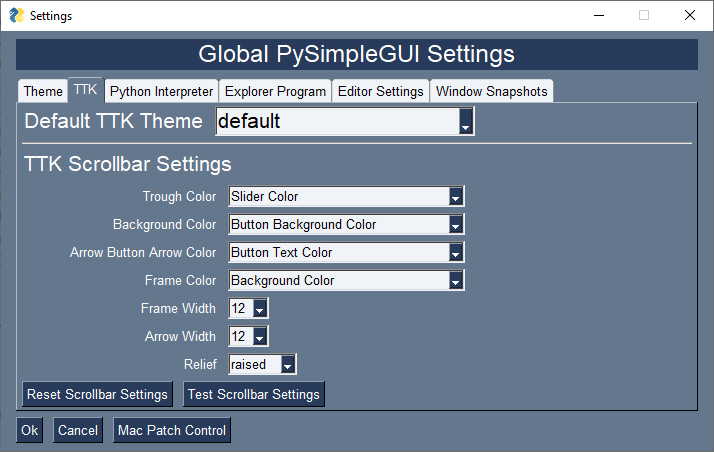
## TTK Scrollbars
TTK Scrollbars are quite flexible in how they can be styled. PySimpleGUI provides many options for these scrollbars. Like much of PySimpleGUI, TTK Scrollbars have been simplified for you so that you are not required to set each and every option. There is a trade-off, a payment, for the trivialization of features. The simplification removes a few settings that are instead done on your behalf.
All scrollbars in all elements of PySimpleGUI have been gray for 4 years and now you get not only that sweet sweet dull system-default-gray, but 1,000s of other colors ***and*** they match your PySimpleGUI theme's colors automagically.
### "Test Scrollbar Settings"
In the Global PySimpleGUI Settings window, in the TTK tab, you'll find a button that will enable you to test/preview your settings. When you click it, you will see the debug window open and some numbers printed so that the scrollbar changes over time. If all PySimpleGUI defaults are used (including the PySimpleGUI theme), it will look something like this:
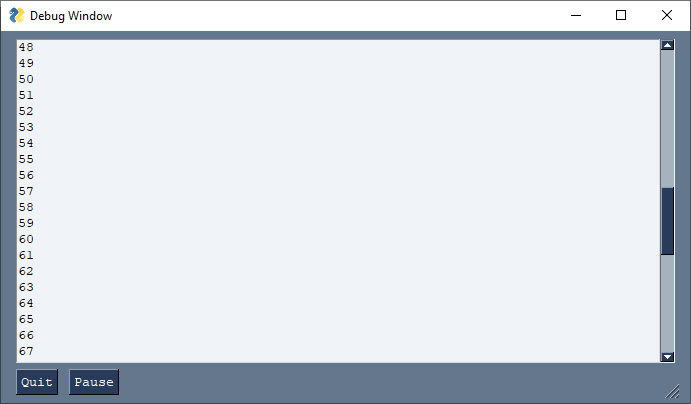
### Values of Scrollbar Settings
In the Global Settings window, you'll find this list of options for each of the TTK Scrollbar parts:
- Button Text Color
- Button Background Color
- Background Color
- Input Element Background Color
- Input Element Text Color
- Text Color
- Slider Color
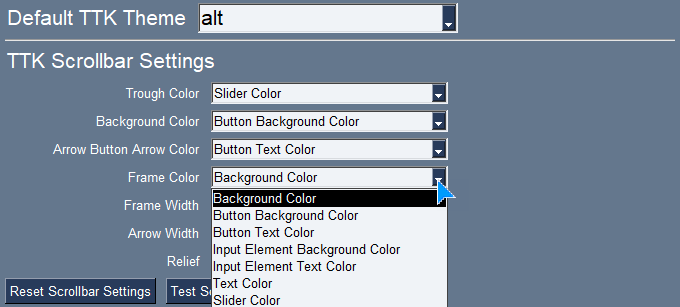
These colors are from the **PySimpleGUI Theme colors**. The term `Slider Color` means to use the color defined by the PySimpleGUI theme in use for the slider. If the slider color is defined as "blue" in the theme, then selecting `Slider Color` will result in that part of the scrollbar being blue.
In addition to the items in the drop-down, you can type color values directly into the combo-box. If you want your slider's trough color to be red, then you can type `red` into the setting in the settings window. You can also use hex RGB colors, like `#FF0000` for pure red.
### Anatomy of a Scrollbar
This diagram shows the parts of a scrollbar that are discussed in the documentation and are in docstrings for parameters. The only item not shown in the diagram is the "Frame Color". It's left vague as it depends on which TTK Theme is chosen and it's a mash-up of multiple tkinter TTK Scrollbar parameters. It's part of the magic-simplification mentioned above. Sticking with the default values almost always has great results.
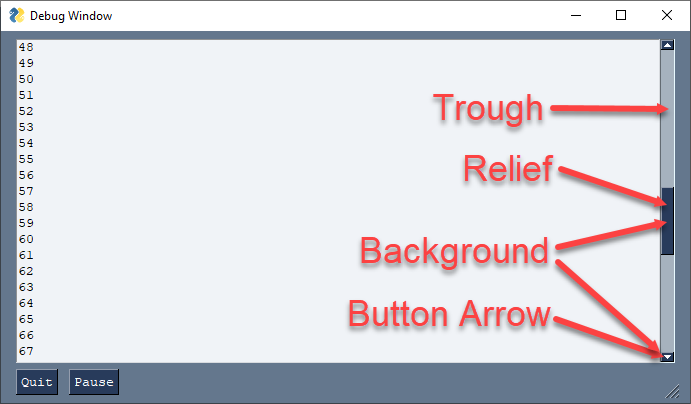
#### The "Thumb"
That bar in the middle that you grab in order to manually scroll quickly is sometimes called the "thumb".... so that's what I'll call it.
The question I'm sure many want to know is "how do I set just the color of the Thumb?" I wanted to know that too! The answer is... you can't. It will be the same color as the background color of the buttons with the arrows.
#### Background
The reason you see 2 lines coming out of the word "Background" in the diagram is that this color is used in 2 places. That background setting is used to set both the thumb and the arrow background.
#### Trough
The trough is the "ditch" of the scrollbar. The Thumb slides in the Trough.
#### Button Arrow
The arrow color is the color of the arrow on the buttons located at the ends of the scrollbar.
#### Relief
The relief setting applies to the trough and the thumb, best I can tell. It's most noticeable when you mouse over parts of the scrollbar.
#### Frame Width & Arrow Width
The Frame Width you can think of as the trough's width. The Arrow Width is the width of the triangle. It's possible to make some truly weird looking scrollbars by setting these 2 widths to be dramatically different. This odd scrollbar has a Frame Width of 16 and an Arrow Width of 8.

### Mouse-over Effects
The TTK Scrollbars in PySimpleGUI have a consistent mouse-over behavior. When the mouse moves over the buttons or the thumb, the colors "swap".
"Swap" in this context means switching foreground and background colors. The "Arrow Button Arrow Color" is the "foreground" and the "Background Color" is the "background".
These 2 examples show the effect
The first example shows the mouse is over the top button of the scrollbar. This button's colors have swapped the arrow color for the background color and vice versa. See how the button at the top of the scrollbar is the "opposite" of the bottom button?

In this second example, the mouse is over the thumb. Normally the color of the thumb is the "background" color (i.e. the color of the arrow button's background). Thus, when the mouse is moved over it, the color will become the color of the ***arrow***.

## Hierarchy of TTK Scrollbar Settings
Scrollbars have numerous places that they can be specified. The order of priorities is determined based on whether or not a level has been set. The order the settings are checked and thus the priority order is:
* Element
* Window
* set_options
* Global Settings
For example, if a `Multiline` element has the trough color for it's scrollbar defined in the layout, then that color will be used for that Multiline's scrollbar. If no scrollbar settings are set for the element, then the settings for the Window the element is contained in. Next the settings changed by a user's program calling the `set_options` function is used. And finally, if none of those are set, then the Global Settings are used.
## Scrollbar Parameter Names
All functions and objects that have a scrollbar setting use the same names for the paramters:
* sbar_trough_color
* sbar_background_color
* sbar_arrow_color
* sbar_width
* sbar_arrow_width
* sbar_frame_color
* sbar_relief
# Running Multiple Windows
This is where PySimpleGUI continues to be simple, but the problem space just went into the realm of "Complex".
If you wish to run multiple windows in your event loop, then there are 2 methods for doing this.
1. First window does not remain active while second window is visible
2. First window remains active while second window is visible
You will find the 2 design matters in 2 demo programs in the Demo Program area of the GitHub (http://www.PySimpleGUI.com)
***Critically important***
When creating a new window you must use a "fresh" layout every time. You cannot reuse a layout from a previous window. As a result you will see the layout for window 2 being defined inside of the larger event loop.
If you have a window layout that you used with a window and you've closed the window, you cannot use the specific elements that were in that window. You must RE-CREATE your `layout` variable every time you create a new window. Read that phrase again.... You must RE-CREATE your `layout` variable every time you create a new window. That means you should have a statemenat that begins with `layout = `. Sorry to be stuck on this point, but so many people seem to have trouble following this simple instruction.
## THE GOLDEN RULE OF WINDOW LAYOUTS
***Thou shalt not re-use a windows's layout.... ever!***
Or more explicitly put....
> If you are calling `Window` then you should define your window layout in the statement just prior to the `Window` call.
## Demo Programs For Multiple Windows
There are several "Demo Programs" that will help you run multiple windows. Please download these programs and FOLLOW the example they have created for you.
Here is ***some*** of the code patterns you'll find when looking through the demo programs.
## Multi-Window Design Pattern 1 - both windows active
```python
import PySimpleGUI as sg
# Design pattern 2 - First window remains active
layout = [[ sg.Text('Window 1'),],
[sg.Input(do_not_clear=True)],
[sg.Text(size=(15,1), key='-OUTPUT-')],
[sg.Button('Launch 2'), sg.Button('Exit')]]
win1 = sg.Window('Window 1', layout)
win2_active = False
while True:
ev1, vals1 = win1.read(timeout=100)
win1['-OUTPUT-'].update(vals1[0])
if ev1 == sg.WIN_CLOSED or ev1 == 'Exit':
break
if not win2_active and ev1 == 'Launch 2':
win2_active = True
layout2 = [[sg.Text('Window 2')],
[sg.Button('Exit')]]
win2 = sg.Window('Window 2', layout2)
if win2_active:
ev2, vals2 = win2.read(timeout=100)
if ev2 == sg.WIN_CLOSED or ev2 == 'Exit':
win2_active = False
win2.close()
```
## Multi-Window Design Pattern 2 - only 1 active window
```python
import PySimpleGUIQt as sg
# Design pattern 1 - First window does not remain active
layout = [[ sg.Text('Window 1'),],
[sg.Input(do_not_clear=True)],
[sg.Text(size=(15,1), key='-OUTPUT-')],
[sg.Button('Launch 2')]]
win1 = sg.Window('Window 1', layout)
win2_active=False
while True:
ev1, vals1 = win1.read(timeout=100)
if ev1 == sg.WIN_CLOSED:
break
win1.FindElement('-OUTPUT-').update(vals1[0])
if ev1 == 'Launch 2' and not win2_active:
win2_active = True
win1.Hide()
layout2 = [[sg.Text('Window 2')], # note must create a layout from scratch every time. No reuse
[sg.Button('Exit')]]
win2 = sg.Window('Window 2', layout2)
while True:
ev2, vals2 = win2.read()
if ev2 == sg.WIN_CLOSED or ev2 == 'Exit':
win2.close()
win2_active = False
win1.UnHide()
break
```
---
# The PySimpleGUI Debugger
Listen up if you are
* advanced programmers debugging some really hairy stuff
* programmers from another era that like to debug this way
* those that want to have "x-ray vision" into their code
* asked to use debugger to gather information
* running on a platform that lacks ANY debugger
* debugging a problem that happens only outside of a debugger environment
* finding yourself saying "but it works when running PyCharm"
Starting on June 1, 2019, a built-in version of the debugger `imwatchingyou` has been shipping in every copy of PySimpleGUI. It's been largely downplayed to gauge whether or not the added code and the added feature and the use of a couple of keys, would mess up any users. Over 30,000 users have installed PySimpleGUI since then and there's not be a single Issue filed nor comment/complaint made, so seems safe enough to normal users... so far....
So far no one has reported anything at all about the debugger. The assumption is that it is quietly lying dormant, waiting for you to press the `BREAK` or `CONTROL` + `BREAK` keys. It's odd no one has accidently done this and freaked out, logging an Issue.
The plain PySimpleGUI module has a debugger builtin. For the other ports, please use the package `imwatchingyou`.
## What is it? Why use it? What the heck? I already have an IDE.
This debugger provides you with something unique to most typical Python developers, the ability to "see" and interact with your code, **while it is running**. You can change variable values while your code continues to run.
Print statements are cool, but perhaps you're tired of seeing a printout of `event` and `values`:
```
Push Me {0: 'Input here'}
Push Me {0: 'Input here'}
Push Me {0: 'Input here'}
```
And would prefer to see this window updating continuously in the upper right corner of your display:

Notice how easy it is, using this window alone, to get the location that your PySimpleGUI package is coming from ***for sure***, no guessing. Expect this window to be in your debugging future as it'll get asked for from time to time.
## Preparing To Run the Debugger
If your program is running with blocking `Read` calls, then you will want to add a timeout to your reads. This is because the debugger gets it's cycles by stealing a little bit of time from these async calls... but only when you have one of these debugger windows open so no bitching about wasted CPU time as there is none.
Your event loop will be modified from this blocking:
```python
while True:
event, values = window.read()
```
To this non-blocking:
```python
while True:
event, values = window.read(timeout=200)
if event == sg.TIMEOUT_KEY:
continue
```
These 3 lines will in no way change how your application looks and performs. You can do this to any PySimpleGUI app that uses a blocking read and you'll not notice a difference. The reason this is a NOP (No-operation) is that when a timeout happens, the envent will be set to `sg.TIMEOUT_KEY`. If a timeout is returned as the event, the code simply ignores it and restarts the loop by executing a `continue` statement.
This timeout value of 200 means that your debugger GUI will be updated 5 times a second if nothing is happening. If this adds too much "drag" to your application, you can make the timeout larger. Try using 500 or 1000 instead of 100.
### What happens if you don't add a timeout
Let's say you're in a situation where a very intermettent bug has just happened and the debugger would really help you, but you don't have a timeout on your `windows.read()` call. It's OK. Recall that the way the debugger gets its "cycles" is to borrow from your `Read` calls. What you need to do is alternate between using the debugger and then generating another pass through your event loop.
Maybe it's an OK button that will cause your loop to execute again (without exiting). If so, you can use it to help move the debugger along.
Yes, this is a major pain in the ass, but it's not THAT bad and compared to nothing in a time of crisis and this is potentially your "savior tool" that's going to save your ass, pressing that OK button a few times is going to look like nothing to you. You just want to dump out the value of a variable that holds an instance of your class!
## A Sample Program For Us To Use
Now that you understand how to add the debugger to your program, let's make a simple little program that you can use to follow these examples:
```python
import PySimpleGUI as sg
window = sg.Window('Testing the Debugger', [[sg.Text('Debugger Tester'), sg.In('Input here'), sg.B('Push Me')]])
while True:
event, values = window.read(timeout=500)
if event == sg.TIMEOUT_KEY:
continue
if event == sg.WIN_CLOSED:
break
print(event, values)
window.close()
```
## Debugger Windows
### "Popout Debugger Window"
There are 2 debugger windows. One is called the "Popout" debugger window. The Popout window displays as many currently in-scope local variables as possible. This window is not interactive. It is meant to be a frequently updated "dashboard" or "snapshot" of your variables.
One "variable" shown in the popout window that is an often asked for piece of information when debugging Issues and that variable is `sg` (or whatever you named the PySimpleGUI pacakge when you did your import). The assumption is that your import is `import PySimpleGUI as sg`. If your import is different, then you'll see a different variable. The point is that it's shown here.
Exiting this window is done via the little red X, **or using the rickt-click menu** which is also used as one way to launch the Main Debugger Window
#### Ways of Launching the Popout Window
There are 3 ways of opening the Popout window.
1. Press the `BREAK` key on your keyboard.
2. Call the function `show_debugger_popout_window(location=(x,y))`
3. Add `Debug()` button to your layout - adds a little purple and yellow PySimpleGUI logo to your window
#### When you are asked for the "Location of your PySimpleGUI package or PySimpleGUI.py file" do this
If you wish to use the debugger to find the location of THIS running program's PySimpleGUI package / the PySimpleGUI.py file, then all you need to do is:
* Press the `BREAK` key on your keyboard.
* This is sometimes labelled as the `Cancel` key
* May also have `Pause` printed on key
* On some US keyboards, it is located next to `Scroll Lock` and/or above `PageUp` key
* This will open a window located in the upper right corner of your screen that looks something like this:

* The information you are seeking is shown next to the `sg` in the window
You don't need to modify your program to get this info using this technique.
If your variable's value is too long and doesn't fit, then you'lll need to collect this information using the "Main Debugger Window"
#### What's NOT Listed In The Popout Debugger Window
The Popup window is a "Snapshot" of your local variables at the time the window was opened. This means **any variables that did not exist at the time the Popout was created will not be shown**. This window does **NOT** expand in size by adding new variables. Maybe in the future.
### The "Main Debugger Window"
Now we're talking serious Python debugging!
Ever wish you had a `repl>>>` prompt that you could run while your program is running. Well, that's pretty much what you're getting with the PySimpleGUI debugger Main Window! Cool, huh? If you're not impressed, go get a cup of coffee and walk off that distraction in your head before carring on because we're in to some seriously cool shit here....
You'll find that this window has 2 tabs, one is labelled `Variables` and the other is labelled `REPL & Watches`
#### Ways of Opening the Main Debugger Window
There are 3 ways to open the Main Debugger Window
1. Press `Control` + `Break` on your PC keyboard
2. From the Popout Debug Window, right click and choose `Debugger` from the right click menu
3. From your code call `show_debugger_window(location=(x,y))`
#### The "Variables" Tab of Main Debugger Window
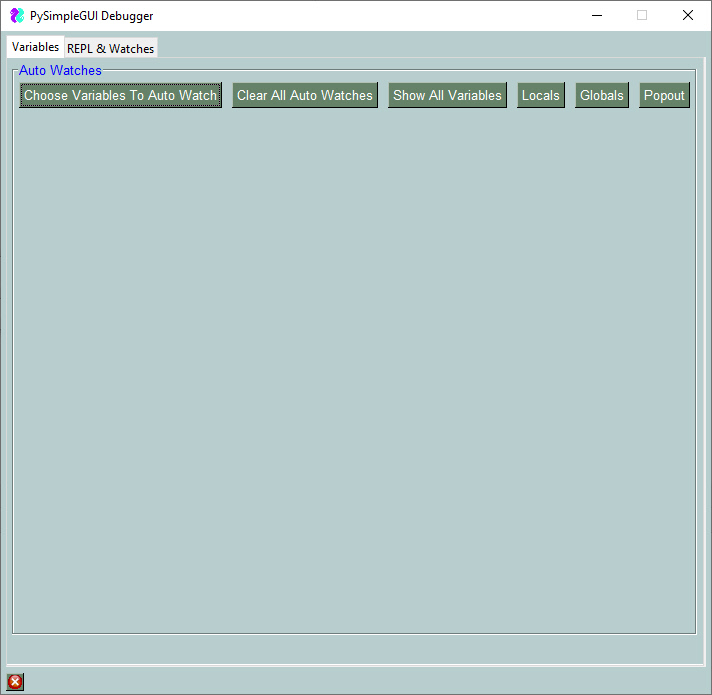
Notice the the "frame" surrounding this window is labelled "Auto Watches" in blue. Like the Popup window, this debugger window also "Watches" variables, which means continuously updates them as often as you call `Window.read`.
The maximum number of "watches" you can have any any one time is 9.
##### Choosing variables to watch
You can simply click "Show All Variable" button and the list of watched variables will be automatically populard by the first 9 variables it finds. Or you can click the "Choose Variables to Auto Watch" button where you can individually choose what variables, **and expressions** you wish to display.
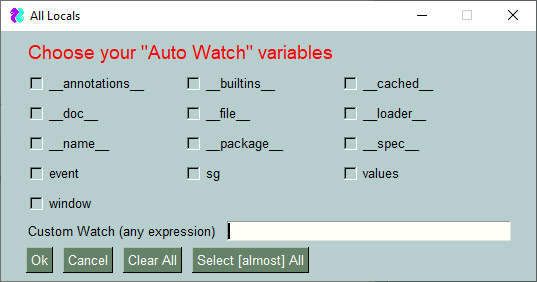
In this window we're checking checkboxes to display these variables:
`event`, `sg`, `values`, `window`, `__file__`
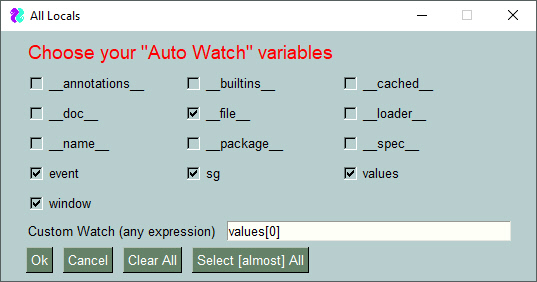
Additionally, you can see at the bottom of the window a "Custom Watch" has been defined. This can be any experession you want. Let's say you have a window with a LOT of values. Rather than looking through the `values` variable and finding the entry with the key you are looking for, the values variable's entry for a specific key is displayed.
In this example the Custom Watch entered was `values[0]`. After clicking on the "OK" button, indicating the variables are chosen that we wish to watch, this is the Main window that is shown:
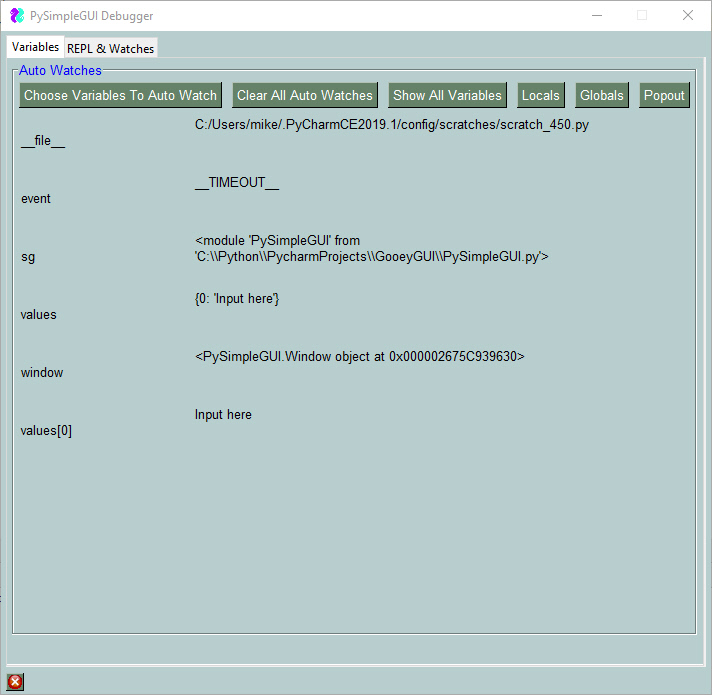
We can see the variables we checked as well as the defined expression `values[0]`. If you leave this window open, these values with continuously be updated, on the fly, every time we call the line in our example code `window.read(timeout=500)`. This means that the Main Debugger Window and these variables we defined will be updated every 500 milliseconds.
#### The REPL & Watches Tab
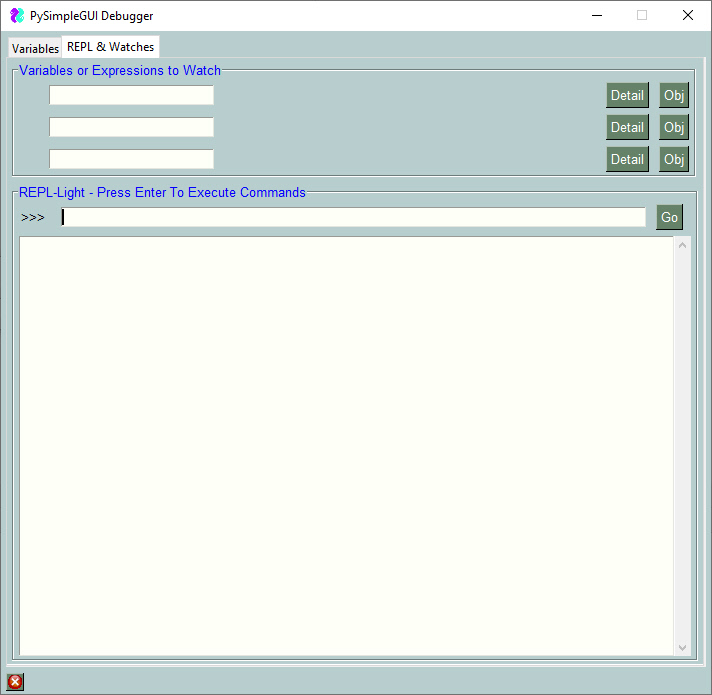
This tab is provided to you as a way to interact with your running program on a real-time basis.
If you want to quickly look at the values of variables, nearly ANY variables, then type the information into one of the 3 spaces provided to "Watch" either variables or experessions. In this example, the variable window was typed into the first slow.
***Immediately*** after typing the character 'w', the information to the right was displayed. No button needs to be clicked. You merely neeed to type in a valid experession and it will be displayed to you.... and it will be displayed on an on-going, constantly-refreshing-basis.
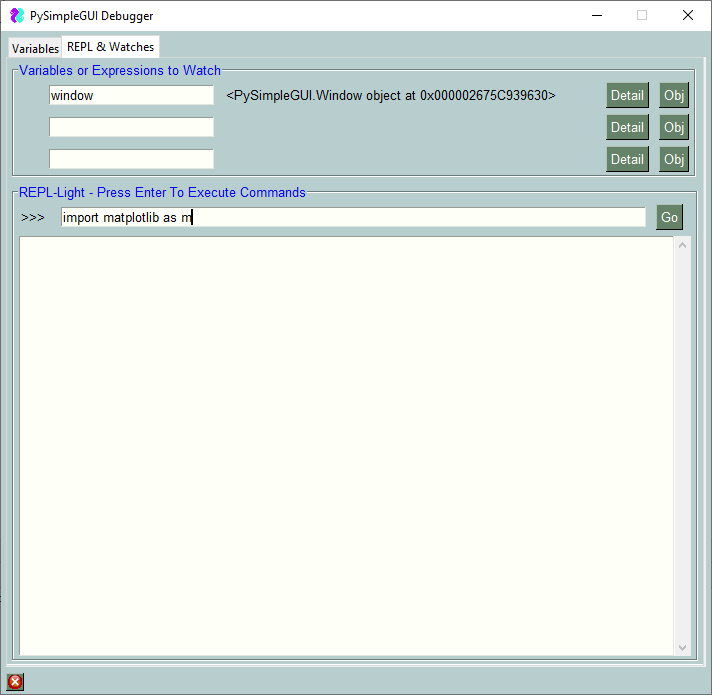
If the area to the right of the input field is too small, then you can click on the "Detail" button and you will be shown a popup, scrolled window with all of the information displayed as if it were printed.
I'm sure you've had the lovely experience of printing an object. When clicking the "Detail" button next to the `window` variable being shown, this window is shown:
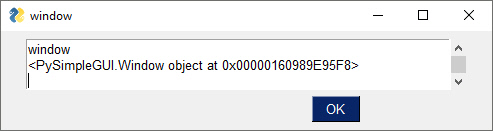
Oh, Python, -sigh-. I just want to see my `window` object printed.
#### `Obj` Button to the Rescue!
PySimpleGUI has a fun and very useful function that is discussed in the docs named `ObjToString` which takes an object and converts it's **contents** it into a nicely formatted string. This function is used to create the text output when you click the `Obj` button. The result is this instead of the tiny window shown previously:
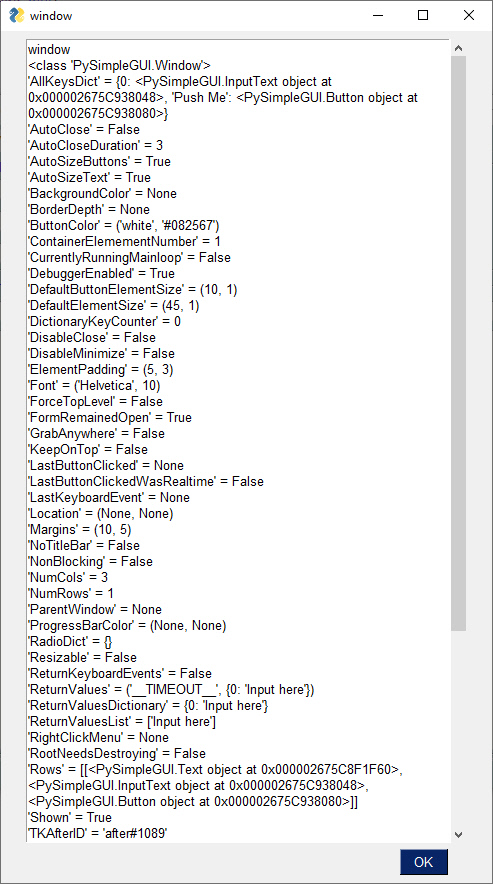
## The REPL Prompt
While not **really** a Python REPL prompt, this window's `REPL >>>` prompt is meant to act as much like one as possible. Here you can enter experessions and code too.
The uses for this prompt are so numerous and diverse that listing them all won't be attempted.
### Your "XRay" and "Endoscope" into Your Program
Think of this prompt as a way to get specific diagnostics information about your ***running*** program. It cannot be stressed enough that the power and the usefullness of this tool is in its ability to diagnose a running program, after you've already started it running.
### Execute Code
In addition to displaying information, getting paths to packages, finding version information, you can execute code from the PySimpleGUI Debugger's `REPL >>>` prompt. You can type in any expression as well as any **executable statement**.
For example, want to see what `PopupError` looks like while you're running your program. From the REPL prompt, type:
`sg.PopupError('This is an error popup')`
The result is that you are shown a popup window with the text you supplied.
### KNOW Answers to Questions About Your Program
Using this runtime tool, you can be confident in the data you collect. Right?
***There's no better way to find what version of a package that your program is using than to ask your program.*** This is so true. Think about it. Rather than go into PyCharm, look at your project's "Virtual Environment", follow some path to get to a window that lists packages installed for that project, get the verstion and your're done, right? Well, maybe. But are you CERTAIN your program is using THAT version of the package in question?
SO MUCH time has been wasted in the past when people KNEW, for sure, what version they were running. Or, they had NO CLUE what version, or no clue to find out. There's nothing wrong with not knowing how to do something. We ALL start there. Geeez..
A real world example.....
## How To Use the Debugger to Find The Version Number of a Package
Let's pull together everything we've learned to now and use the debugger to solve a problem that happens often and sometimes it's not at all obvious how to find the answer.
We're using ***Matplotlib*** and want to find the "Version".
For this example, the little 12-line program in the section "A Sample Program For Us To Use" is being used.
That program does not import `matplotlib`. We have a couple of choices, we can change the code, we can can import the package from the debugger. Let's use the debgger.
Pull up the Main Debugger Window by pressing `CONTROL+BREAK` keys. Then click the "REPL * Watches" tab. At the `>>>` prompt we'll first import the package by typing:
`import matplotlib as m`
The result returned from Python calls that don't return anything is the value None. You will see the command you entered in the output area followed by "None", indicating success.
finally, type:
`m.__version__`
The entire set of operations is shown in this window:
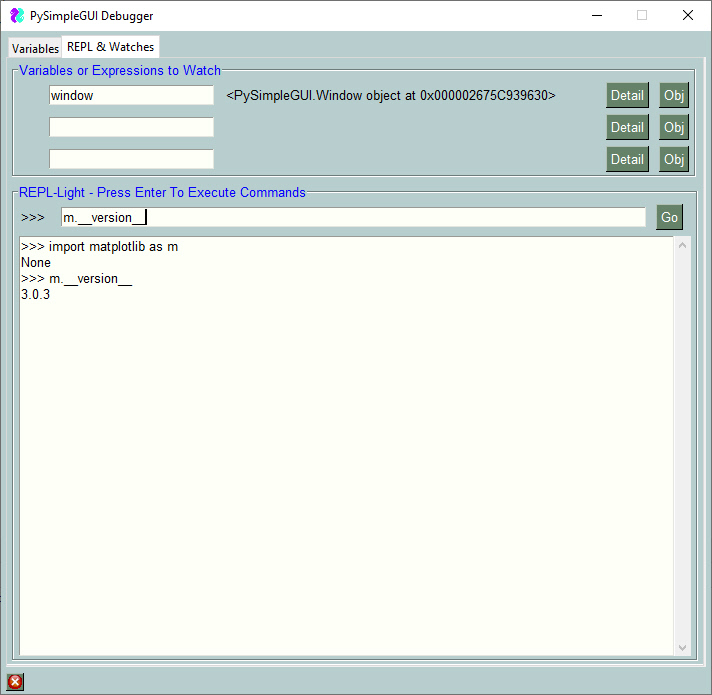
By convention you'll find many modules have a variable `__version__` that has the package's version number. PySimpleGUI has one. As you can see matplotlib has one. The `requests` module has this variable.
For maximum compatibility, PySimpleGUI not only uses `__version__`, but also has the version contained in another variable `version` which has the version number because in some situations the `__version__` is not available but the `version` variable is avaiable.
**It is recommended that you use the variable `version` to get the PySimpleGUI version** as it's so far been the most successful method.
tkinter, however does NOT.... of course.... follow this convention. No, to get the tkinter version, you need to look at the variable:
`TkVersion`
Here's the output from the REPL in the debugger showing the tkinter version:
```
>>> import tkinter as t
None
>>> t.TkVersion
8.6
>>> t.__version__
Exception module 'tkinter' has no attribute '__version__'
```
---
# User Settings API
In release 4.30.0 there is a new set of API calls available to help with "user settings". Think of user settings as a dictionary that is automatically written to your hard drive. That's basically what it is.
In release 4.50.0 support for INI files was added in addition to the existing JSON file format.
While using JSON or config files to save and load a settings dictionary isn't very difficult, it is still code you'll need to write if you want to save settings as part of your GUI. Since having "settings" for a GUI based program isn't uncommon, it made sense to build this capability into PySimpleGUI. Clearly you can still use your own method for saving settings, but if you're looking for a simple and easy way to do it, these calls are likely about as easy as it gets.
There have already been some demo programs written that use JSON files to store settings. You can expect that this capability will begin to show up in more demos in the future since it's now part of PySimpleGUI.
User settings are stored in a Python dictionary which is saved to / loaded from disk. Individual settings are thus keys into a dictionary. **You do not need to explicitly read nor write the file**. Changing any entry will cause the file to be saved. Reading any entry will cause the file to be read if it hasn't already been read.
## Two Interfaces
There are 2 ways to access User Settings
1. User Settings function calls
2. The `UserSettings` class
They both offer the same basic operations. The class interface has an added benefit of being able to access the individual settings using the same syntax as Python dictionary.
If you want to use INI files, then you'll need to use the object interface.
## List of Calls for Function Interface
|Function|Description|
| --- | --- |
|user_settings|Returns settings as a dictionary|
|user_settings_delete_entry|Deletes a setting|
|user_settings_delete_filename|Deletes the settings file|
|user_settings_file_exists|Returns True if settings file specified exists|
|user_settings_filename|Returns full path and filename of current settings file|
|user_settings_get_entry|Returns value for a setting. If no setting found, then specified default value is returned|
|user_settings_load|Loads dictionary from the settings file. This is not normally needed||
|user_settings_save|Saves settings to current or newly specified file. Not normally needed|
|user_settings_set_entry|Sets an entry to a particular value
|user_settings_write_new_dictionary|Writes a specified dictionary to settings file|
## Operations
There are 2 categories that the calls can be divided into.
1. File operations
2. Settings operations
File operations involve working with the JSON file itself. They include:
* Setting the path and/or filename
* Load/save the file (these are somewhat optional as the saving loading/saving is done automatically)
* Deleting the settings file
* Checking if settings file exists
Generally speaking, a setting is specified with a key which is generally a string. Settings operations are for working with the individual settings and include:
* Get the value of a setting (returns a default value if not found)
* Set the value of a setting (also saves the settings to disk)
Any setting operation may cause the file to be written. This is because a "get" operation can include returning a default value if the setting isn't found. This means a new entry is made in your settings dictionary is one didn't exist before. Since a new entry is made, that means it needs to be also be written to disk.
## Filenames
The settings filename defaults the filename of your Python file making the call with the extension ".json" added. If your Python program is called `test.py` then your default settings filename will be `test.json`.
In addition to the filename having a default value, the path to the file also has a default value. The default depends on your operating system.
|Operating System|Default Path|
| --- | --- |
| Windows | \user\user_name\AppData\Local\PySimpleGUI\settings |
| Linux | ~/.config/PySimpleGUI/settings |
| Mac | ~/Library/Application Support/PySimpleGUI/settings |
When calling the User Settings APIs, if a parameter is named `filename`, you can specify a full path or just the filename. This will save you the trouble of having to split up your path and filename in your code. If you specify only the path, the the filename will be added to that path and named as defined earlier.
Like the rest of PySimpleGUI, the idea is for you to write as little code as possible. The default values for the filename and path should be fine for you to use. They will be stored in a location on your system that is meant to store user settings.
### Setting Filename
If you want to see what the current filename is for your settings, then you can call `user_settings_filename()` with no parameters and you'll get back an absolute path and filename.
To make the code for specifying the folder and filename as simple as possible, the 2 parts are separated in the call specifying the name of the settings file. However, it is possible to supply a full and complete folder + filename as well.
The default filename for your settings file is the name of the file that makes the call to the User Settings API's with the `.py` extension changed to a `.json` extension. If your source file is called `demo.py`, then your settings filename will be `demo.json`.
#### Setting only the filename
If you want to control the name of the file and/or the path to the settings file, then you will use the `user_settings_filename` call. This function takes 2 parameters.
```python
user_settings_filename(filename=None, path=None)
```
If you set only the path, then the filename will default to the value already described. If you set only the filename, then the path will be the default path is dependent on your operating system. See the table above for the locations for each OS.
```python
import PySimpleGUI as sg
sg.user_settings_filename(filename='my_settings.json')
print(sg.user_settings_filename())
```
If you are running on Windows, then the result of running this code will be this printed on the console:
```
C:\Users\your_use_name\AppData\Local\PySimpleGUI\settings\my_settings.json
```
You are not restricted to naming your settings file to an extension of .json. That is simply the default extension that's used by PySimpleGUI. You can use any extension you would like, including no extension.
#### Setting only the path
Maybe you don't care about the settings filename itself, but you do care about where the settings are stored. Let's say you want the settings to be stored in the same folder as your Python source file. Specifying `path='.'` will achieve this.
#### Setting a fully qualified filename
If you want to specify the full absolute path and filename of the settings file, you can do it by using the filename parameter. Instead of passing the filename only, pass in a fully qualified path and filename. If you want to name your settings file `a:\temp\my_settings`, then your call will look like this:
```python
sg.user_settings_filename(filename=r'a:\temp\my_settings')
```
You are not required to break your file down into 2 parameters. You could if you wanted to however. The equivalent to the above call using 2 parameters would be:
```python
sg.user_settings_filename(filename='my_settings' , path=r'a:\temp')
```
### Getting the current filename
Calling `user_settings_filename` with no parameters will return the full path and filename of your settings file as a single string.
### File Loading / Saving
Generally speaking you will not need to load or save your settings file. It is automatically saved after every change.
Note that reading a setting can also cause the file to be written. If you read a setting and the setting did not exist, then your call to `user_settings_get_entry` will return the default value you specified. As a result, the dictionary is updated with this default value and in return the file is written with this value as well.
One of the situations where you may want to explicitly read/load the settings file is if you're expecting it to be modified by another program.
Like so much of PySimpleGUI, as much as possible is automatically done on your behalf. This includes the requirement of saving and loading your settings file. Even naming your settings file is optional.
## The `UserSettings` Class Interface
The `UserSettings` class makes working with settings look like a Python dictionary. The familiar [ ] syntax is used to read, write and delete entries.
### Creating a `UserSettings` Object
The first step is to create your setting object. The parameters are the same as calling the `user_settings_filename` function. If you want to use the default values, then leave the parameters unchanged.
```python
settings = sg.UserSettings()
```
This is the same as calling `sg.user_settings_filename()`
### Reading, Writing, and Deleting an Individual Settings Using [ ] Syntax
The first operation will be to create the User Settings object.
```python
settings = sg.UserSettings()
```
To read a setting the dictionary-style [ ] syntax is used. If the item's name is `'-item-'`, then reading the value is achieved by writing
```python
item_value = settings['-item-']
```
Writing the setting is the same syntax except the expression is reversed.
```python
settings['-item-'] = new_value
```
To delete an item, again the dictionary style syntax is used.
```python
del settings['-item-']
```
You can also call the delete_entry method to delete the entry.
```python
settings.delete_entry('-item-')
```
### `UserSettings` Methods
You'll find all of the `UserSettings` methods available to you detailed in the Call Reference documentation.
One operation in particular that is not achievable using the [ ] notation is a "get" operation with a default value. For dictionaries, this method is `get` and for the `UserSettings` class the method is also called `get`. They both have an optional second parameter that represents a "default value" should the key not be found in the dictionary.
If you would like a setting with key `'-item-'` to return an empty string `''` instead of `None` if they key isn't found, then you can use this code to achieve that:
```python
value = settings.get('-item-', '')
```
It's the same kind of syntax that you're used to using with dictionaries.
### Default Value
Normally the default value will be `None` if a key is not found and you get the value of the entry using the bracket format:
```python
item_value = settings['-item-']
```
You can change the default value by calling `settings.set_default_value(new_default)`. This will set the default value to return in the case when no key is found. Note that an exception is not raised when there is a key error (see next section on error handling). Instead, the default value is returned with a warning displayed.
## Displaying the Settings Dictionary
The class interface makes it easy to dump out the dictionary. If you print the UserSettings object you'll get a printout of the dictionary.
Note that you'll need to "load" the settings from disk if you haven't performed any operations on the settings.
```python
settings = sg.UserSettings()
settings.load()
print(settings)
```
If you were to print the dictionary after creating the object, then the `load` is not needed
```python
settings = sg.UserSettings()
print(settings['-item-'])
print(settings)
```
To print the dictionary using the function call interface:
```python
print(sg.user_settings())
```
## Error Handling for User Settings
From a GUI perspective, user settings are not critical to the GUI operations itself. There is nothing about settings that will cause your window to not function. As a result, errors that occur in the User Settings are "soft errors". An error message is displayed along with information about how you called the function, when possible, and then execution continues.
One reason for treating these as soft errors and thus not raising an exception is that raising an exception will crash your GUI. If you have redirected your output, which many GUIs do, then you will see no error information and your window will simply disappear. If you double clicked a .py file to launch your GUI, both the GUI and the console window will instantly disappear if the GUI crashes, leaving you no information to help you debug the problem.
The only time errors can occur are during file operations. Typically these errors happen because you've specified a bad path or you don't have write permission for the path you specified.
Example error message. If you executed this code:
```python
def main():
sg.user_settings_filename(path='...')
sg.user_settings_set_entry('-test-',123)
```
Then you'll get an error when trying to set the '-test-' entry because `'...'` is not a valid path.
```
*** Error saving settings to file:***
...\scratch_1065.json [Errno 2] No such file or directory: '...\\scratch_1065.json'
The PySimpleGUI internal reporting function is save
The error originated from:
File "C:/Users/mike/.PyCharmCE2019.1/config/scratches/scratch_1065.py"
line 8
in main
sg.user_settings_set_entry('-test-',123)
```
You should be able to easily figure out these errors as they are file operations and the error messages are clear in detailing what's happened and where the call originated.
### Silencing the Errors
If you're the type that doesn't want to see any error messages printed out on your console, then you can silence the error output.
When using the class interface, there is a parameter `silent_on_error` that you can set to `True`.
For the function interface, call the function `user_settings_silent_on_error()` and set the parameter to `True`
## Config INI File Support
Using INI files has some advantages over JSON, particularly when humans are going to be modifying the settings files directly.
To specify use of INI files instead of JSON, set the parameter `use_config_file=True` when creating your `UserSetting` object.
```python
settings = sg.UserSettings('my_config.ini', use_config_file=True, convert_bools_and_none=True)
```
Note the 2 parameters that are specific for .ini files:
* `use_config_file` - Set to `True` to indicate you're using an INI file
* `convert_bools_and_none` - Defaults to `True`. Normally all settings from INI files are strings. This parameter will convert 'True', 'False', and 'None' to Python values `True`, `False`, `None`
There is also an additional method added `delete_section` which will delete an entire section from your INI file.
### Example File
Let's use this as our example INI file:
```
[My Section]
filename = test1234
filename2 = number 2
filename3 = number 3
[Section 2]
var = 123
[Empty]
[last section]
bool = True
```
### Getting / Setting Entries
Just like the JSON files, you can access the individual settings using the UserSettings class by using the `[ ]` notation or by calling `get` and `set` methods.
The big difference with the INI file support is the addition of an extra lookup / parameter, the section name.
To access the entry `var` in section `Section 2`, you can use wither of these:
```python
settings['Section 2']['var']
settings['Section 2'].get('var', 'Default Value')
```
The advantage of using the `get` method is that if the entry is not present, a default value will be returned instead.
To set an entry, you also have 2 choices:
```python
settings['Section 2']['var'] = 'New Value'
settings['Section 2'].set('var', 'New Value')
```
### Accessing INI File Sections
Once you have created your `UserSettings` object, then you'll be accessing entries using 2 keys instead of 1 like JSON uses.
To access an entire section, you'll write:
`settings['section']`
To get all of 'My Section' it will be:
`settings['My Section']` which returns a section object that behaves much like a dictionary.
To access a value within a section, add on one more lookup. To get the value of the `filename` setting in the `My Section` section, it's done with this code:
`settings['My Section']['filename']`
### Deleting Entries
To delete an individual entry, you can use several different techniques. One is to use `del`
```python
del settings['My Section1']['test']
```
This deletes the setting `test` in the section `My Section1`
You can also do this by calling the `delete_entry` method
```python
settings.delete_entry(section='My Section1', key='test')
```
### Deleting Sections
If you want to delete an entire section, you have 2 methods for doing this. One is to call the method `UserSettings.delete_section` and pass in the name of the section to be deleted.
```python
settings.delete_section(section='My Section1')
```
The other is to lookup the section and then call `delete_section` on that section.
```python
settings['My Section1'].delete_section()
```
### Printing Settings for INI Files
If you print (or cast to a string) a section or a UserSettings object for an INI file, then you will get a nicely formated output that shows the sections and what settings are in each section.
Going back to the example INI file from earlier. Printing the UserSettings object for this file produces this output:
```
My Section:
filename : test1234
filename2 : number 2
filename3 : number 3
Section 2:
var : 123
Empty:
last section:
bool : True
```
### INI File Comments (WARNING)
If you have created an INI file using a test editor or it was created output of Python, then when the file is written, your comments will be stripped out. Code is being added to PySimpleGUI to merge back your comments, but the code isn't done yet. You'll either live with this limitation for now or write your own merge code.
Yea, I know, it's a bummer, but the plan is to overcome this Python limitation.
## Coding Convention for User Settings Keys
The User Settings prompted a new coding convention that's been added to PySimpleGUI examples. As you're likely aware, keys in layouts have the format `'-KEY-`'. For UserSettings, a similar format is used, but instead of the string being in all upper case, the characters are lower case. In the example below, the user setting for "filename" has a User Setting key of `'-filename-'`. Coding conventions are a good thing to have in your projects. You don't have to follow this one of course, but you're urged to create your own for places in your code that it makes sense. You could say that PEP8 is one giant coding convention for the Python language as a whole. You don't have to follow it, but most Python programmers do. We follow it "by convention".
The reason this is done in PySimpleGUI is so that the keys are immediately recognizable. Perhaps your application has dictionaries that you use. If you follow the PySimpleGUI coding convention of Element keys have the format `'-KEY-'` and User Settings keys have the format of `'-key-'`, then you'll immediately understand what a specific key is used for. Your company may have its own coding conventions so follow those if appropriate instead of what you see in the PySimpleGUI examples.
## Example User Settings Usage
One of the primary places settings are likely to be used is for filenames / folder names. How many times have you run the same program and needed to enter the same filename? Even if the name of the file is on your clipboard, it's still a pain in the ass to paste it into the input field every time you run the code. Wouldn't it be so much simpler if your program remembered the last value you entered? Well, that's exactly why this set of APIs was developed.... again it was from laziness that this capability gained life.
If you want your `Input` elements to default to an entry from your settings, then you simply set the first parameter (`default_text`) to the value of a setting from your settings file.
Let's say your layout had this typical file input row:
```python
[sg.Input(key='-IN-'), sg.FileBrowse()]
```
To automatically fill in the `Input` to be the last value entered, use this layout row:
```python
[sg.Input(sg.user_settings_get_entry('-filename-', ''), key='-IN-'), sg.FileBrowse()]
```
When your user clicks OK or closes the window in a way that is in a positive way (instead of cancelling), then add this statement to save the value.
```python
sg.user_settings_set_entry('-filename-', values['-IN-'])
```
Here's an entire program demonstrating this way of using user settings
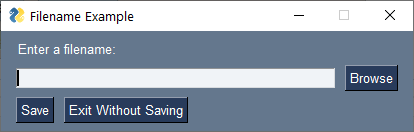
```python
import PySimpleGUI as sg
layout = [[sg.Text('Enter a filename:')],
[sg.Input(sg.user_settings_get_entry('-filename-', ''), key='-IN-'), sg.FileBrowse()],
[sg.B('Save'), sg.B('Exit Without Saving', key='Exit')]]
window = sg.Window('Filename Example', layout)
while True:
event, values = window.read()
if event in (sg.WINDOW_CLOSED, 'Exit'):
break
elif event == 'Save':
sg.user_settings_set_entry('-filename-', values['-IN-'])
window.close()
```
In 2 lines of code you've just made life for your user so much easier. And, by not specifying a location and name for your file, the settings are stored out of sight / out of mind. If you wanted to have the settings be stored with your program file so that it's more visible, then add this statement before your layout:
```python
sg.user_settings_filename(path='.')
```
## Example Using UserSettings Class with [ ] Syntax
The same example can be written using the `UserSettings` class and the [ ] lookup syntax.
Here's the same program as above.
```python
import PySimpleGUI as sg
settings = sg.UserSettings()
layout = [[sg.Text('Enter a filename:')],
[sg.Input(settings.get('-filename-', ''), key='-IN-'), sg.FileBrowse()],
[sg.B('Save'), sg.B('Exit Without Saving', key='Exit')]]
window = sg.Window('Filename Example', layout)
while True:
event, values = window.read()
if event in (sg.WINDOW_CLOSED, 'Exit'):
break
elif event == 'Save':
settings['-filename-'] = values['-IN-']
window.close()
```
If you were to place these 2 examples in the same file so that one ran after the other, you will find that the same settings file is used and thus the value saved in the first example will be read by the second one.
There was one additional line of code added:
```python
settings.set_default_value('') # Set the default not-found value to ''
```
Strictly speaking, this line isn't needed because the Input Element now takes `None` to be the same as a value of `''`, but to produce identical results I added this line of code.
## Demo Programs
There are a number of demo programs that show how to use UserSettings to create a richer experience for your users by remember the last value input into input elements or by adding a Combobox with a history of previously entered values. These upgrades make for a much easier to use GUI, especially when you find yourself typing in the same values or using the same files/folders.
## Brief Caution - User Settings Stick Around
If you're using the default path, remember that previous runs of your file may have old settings that are still in your settings file. It can get confusing when you've forgotten that you previously wrote a setting. Not seeing the filename can have drawbacks like this.
Also, because the settings automatically save after every update, it can be easy to accidently overwrite a previously saved setting. If you want to avoid this, then perhaps it's best that you work with a dictionary within your code and then explicitly save your dictionary when you're ready to commit it to disk.
To save your Python dictionary to a settings file, simply call `user_settings_write_new_dictionary(dict)`, passing in your dictionary as the parameter.
-------------------------
# Extending PySimpleGUI
PySimpleGUI doesn't and can't provide every single setting available in the underlying GUI framework. Not all tkinter options are available for a `Text` Element. Same with PySimpleGUIQt and the other ports.
There are a few of reasons for this.
1. Time & resource limits - The size of the PySimpleGUI development team is extremely small
2. PySimpleGUI provides a "Unified API". This means the code is, in theory, portable across all of the PySimpleGUI ports without chaning the user's code (except for the import)
3. PySimpleGUI is meant, by design, to be simple and cover 80% of the GUI problems.
However, PySimpleGUI programs are ***not*** dead ends!! Writing PySimpleGUI code and then getting to a point where you really really feel like you need to extend the Listbox to include the ability to change the "Selected" color. Maybe that's super-critical to your project. And maybe you find out late that the base PySimpleGUI code doesn't expose that tkinter capability. Fear not! The road does continue!!
## Widget Access
Most of the user extensions / enhancements are at the "Element" level. You want some Element to do a trick that you cannot do using the existing PySimpleGUI APIs. It's just not possible. What to do?
What you need is access to the underlying GUI framework's "Widget". The good news is that you HAVE that access ready and waiting for you, for all of the ports of PySimpleGUI, not just the tkinter one.
### `Element.Widget` is The GUI Widget
The class variable `Widget` contains the tkinter, Qt, WxPython, or Remi widget. With that variable you can modify that widget directly.
***You must first `Read` or `Finalize` the window before accessing the `Widget` class variable***
The reason for the Finalize requirement is that until a Window is Read or is Finalized it is not actually created and populated with GUI Widgets. The GUI Widgets are created when you do these 2 operations.
Side note - You can stop using the `.Finalize()` call added onto your window creation and instead use the `finalize` parameter in the `Window` call.
OLD WAY:
```python
window = sg.Window('Window Title', layout).Finalize()
```
THE NEW WAY:
```python
window = sg.Window('Window Title', layout, finalize=True)
```
It's cleaner and less confusing for beginners who aren't necessarily trained in how chaining calls work. Py**Simple**GUI.
### Example Use of `Element.Widget`
So far there have been 2 uses of this capability. One already mentioned is adding a new capability. The other way it's been used has been to fix a bug or make a workaround for a quirky behavior.
A recent Issue posted was that focus was always being set on a button in a tab when you switch tabs in tkinter. The user didn't want this to happen as it was putting an ugly black line around their nicely made graphical button.
There is no current way in PySimpleGUI to "disable focus" on an Element. That's essentially what was needed, the ability to tell tkinter that this widget should never get focus.
There is a way to tell tkinter that a widget should not get focus. The downside is that if you use your tab key to navigate, that element will never get focus. So, it's not only blocking focus for this automatic problem, but blocking it for all uses. Of course you can still click on the button.
The way through for this user was to modify the tkinter widget directly and tell it not to get focus. This was done in a single line of code:
```python
window[button_key].Widget.config(takefocus=0)
```
The absolute beauty to this solution is that tkinter does NOT need to be imported into the user's program for this statement to run. Python already know what kind of object `.Widget` is and can thus show you the various methods and class variables for that object. Most all tkinter options are strings so you don't need to import tkinter to get any enums.
### Finding Your Element's Widget Type
Of course, in order to call the methods or access the object's class variables, you need to know the type of the underlying Widget being used. This document could list them all, but the downside is the widget could change types (not a good thing for people using the .Widget already!). It also saves space and time in getting this documentation published and available to you.
So, here's the way to get your element's widget's type:
```python
print(type(window[your_element_key].Widget))
```
In the case of the button example above, what is printed is:
``
I don't think that could be any clearer. Your job at this point is to look at the tkinter documentation to see what the methods are for the tkinter `Button` widget.
## Window Level Access
For this one you'll need some specific variables for the time being as there is no `Window` class variable that holds the window's representation in the GUI library being used.
For tkinter, at the moment, the window's root object is this:
```python
sg.Window.TKroot
```
The type will vary in PySimpleGUI. It will either be:
`tkinter.Tk()`
`tkinter.Toplevel()`
Either way you'll access it using the same `Window` variable `sg.Window.TKroot`
Watch this space in the future for the more standardized variable name for this object. It may be something like `Window.Widget` as the Elements use or something like `Window.GUIWindow`.
## Binding tkiner "events"
If you wish to receive events directly from tkinter, but do it in a PySimpleGUI way, then you can do that and get those events returned to you via your standard `Window.read()` call.
Both the Elements and Window objects have a method called `bind`. You specify 2 parameters to this function. One is the string that is used to tell tkinter what events to bind. The other is a "key modifier" for Elements and a "key" for Windows.
The `key_modifier` in the `Element.bind` call is something that is added to your key. If your key is a string, then this modifier will be appended to your key and the event will be a single string.
If your element's key is not a string, then a tuple will be returned as the event (your_key, key_modifier)
This will enable you to continue to use your weird, non-string keys. Just be aware that you'll be getting back a tuple instead of your key in these situations.
The best example of when this can happen is in a Minesweeper game where each button is already a tuple of the (x,y) position of the button. Normal left clicks will return (x,y). A right click that was generated as a result of bind call will be ((x,y), key_modifier).
It'll be tricky for the user to parse these events, but it's assumed you're an advanced user if you're using this capability and are also using non-string keys.
An Element member variable `user_bind_event` will contain information that tkinter passed back along with the event. It's not required for most operations and none of the demos currently use this variable, but it's there just in case. The contents of the variable are tkinter specific and set by tkinter so you'll be digging into the tkinter docs if you're using an obscure binding of some kind.
tkinter events must be in between angle brackets
```python
window['-KEY-'].bind('', 'STRING TO APPEND')
```
Events can also be binded to the window
```python
window.bind('', 'STRING TO APPEND')
```
List of tkinter events:
| Event | Description |
| :------------------------------- | ------------------------------------------------------------ |
| Button-1 / ButtonPress-1 / 1 | Left button is pressed over an element. 1 corresponds to the left button, 2 to the middle button, 3 to the right button. Buttons can go up to 5 |
| ButtonRelease-1 | Left button is released over an element. |
| Double-Button-1 | An element was double clicked. The 'Double' modifier was used. See below for more modifiers. |
| B1-Motion | Left button is held and moved around over an element. |
| Motion | Mouse pointer is moved over an element |
| Enter | Mouse pointer entered the element |
| Leave | Mouse pointer left the element |
| Key / KeyPress Keypress-a / a | A key was pressed. [Keysyms](https://www.tcl.tk/man/tcl8.6/TkCmd/keysyms.htm) can be used to bind specific key/s. When using keysyms, 'Key' or 'KeyPress' can be omitted. |
| KeyReleased | A key was released. |
| FocusIn | Keyboard has focused on element. |
| FocusOut | Keyboard switched focus from element. |
| Visibility | Some part of the element is seen on screen |
Modifier keys can be put in front of events.
| Windows | MacOS |
| ------- | ------- |
| Control | Command |
| Alt | Option |
| Shift |<==|
| Double | <== |
| Triple | <== |
| Quadruple | <== |
The following will bind Ctrl+z to the window:
```python
window.bind('', 'STRING TO APPEND')
```
To unbind an event from an element, use the `unbind` method.
```python
window['-KEY-'].unbind('TKINTER EVENT')
```
Here is sample code that shows these bindings in action.
Four main things are occurring.
1. Any button clicks in the window will return an event "Window Click" from window.read()
2. Right clicking the "Go" buttons will return an event "Go +RIGHT CLICK+" from window.read()
3. When the second Input Element receives focus, an event "-IN2- +FOCUS+" will be returned from window.read()
4. If the "Unbind " button is pressed, the right click binding of the "Go" button will be unbinded.
```python
import PySimpleGUI as sg
sg.theme('Dark Green 2')
layout = [ [sg.Text('My Window')],
[sg.Input(key='-IN1-')],
[sg.Input(key='-IN2-')],
[sg.Button('Go'), sg.Button('Unbind'),sg.Button('Exit')]
]
window = sg.Window('Window Title', layout, finalize=True)
window.bind("", 'Window Click')
window['Go'].bind("", ' +RIGHT CLICK+')
window['-IN2-'].bind("", ' +FOCUS+')
while True: # Event Loop
event, values = window.read()
print(event, values)
if event in (sg.WIN_CLOSED, 'Exit'):
break
if event == 'Unbind':
window['Go'].unbind('')
window.close()
```
[Tkinter bindings documentation](https://tcl.tk/man/tcl8.6/TkCmd/bind.htm#M18)
------------------
# Troubleshooting
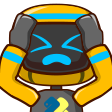
This section was added in early 2022, so it's a bit late in arriving, but it's a start. This section meant to be found when searching for common errors.
Some errors are too broad to cover here like module not found. That one has a huge number of possible root causes.
Instead, we're covering errors that are likely problems in your source code and they cannot be found by the PySimpleGUI code.
If it's any consolation, I run into these same errors frequently! We're programmers and mistakes happen.
## TypeError: list indices must be integers or slices, not ...
You'll get this error when you miss adding a comma at the end of a row in your layout.
Having extra commas isn't a problem and sometimes can be helpful to prevent this error. By leaving an extra comma on the last row, then you'll be able to add more rows without the fear of this error.
This is a **good** layout:
```python
import PySimpleGUI as sg
layout = [[sg.Text('Row 1')],
[sg.Text('Row 2')],]
event, values = sg.Window('Window Title', layout).read(close=True)
```
This one is **not good**
If the commas are removed from the layout, then we'll get this TypeError...
```python
import PySimpleGUI as sg
layout = [[sg.Text('Row 1')]
[sg.Text('Row 2')]]
event, values = sg.Window('Window Title', layout).read(close=True)
```
Generates the TypeErrpr:
```
Traceback (most recent call last):
File "scratch_356.py", line 4, in
[sg.Text('Row 2')]]
TypeError: list indices must be integers or slices, not Text
Process finished with exit code 1
```
---
# Debug Output
Be sure and check out the EasyPrint (Print) function described in the high-level API section. Leave your code the way it is, route your stdout and stderror to a scrolling window.
For a fun time, add these lines to the top of your script
```python
import PySimpleGUI as sg
print = sg.Print
```
This will turn all of your print statements into prints that display in a window on your screen rather than to the terminal.
# "Demo Programs" Applications
There are too many to list!!
There are over 170 sample programs to give you a jump start.
These programs are an integral part of the overall PySimpleGUI documentation and learning system. They will give you a headstart in a way you can learn from and understand. They also show you integration techiques to other packages that have been figured out for you.
You will find Demo Programs located in a subfolder named "Demo Programs" under the top level and each of the PySimpleGUI ports on GitHub.
Demo programs for plain PySimpleGUI (tkinter)
https://github.com/PySimpleGUI/PySimpleGUI/tree/master/DemoPrograms
Demo programs for PySimpleGUIQt:
https://github.com/PySimpleGUI/PySimpleGUI/tree/master/PySimpleGUIQt/Demo%20Programs
Demo programs for PySimpleGUIWx:
https://github.com/PySimpleGUI/PySimpleGUI/tree/master/PySimpleGUIWx/Demo%20Programs
Demo programs for PySimpleGUIWeb:
https://github.com/PySimpleGUI/PySimpleGUI/tree/master/PySimpleGUIWeb/Demo%20Programs
There are not many programs under each of the port's folders because the main Demo Programs should run on all of the other platforms with minimal changes (often only the import statement changes).
You will also find a lot of demos running on Trinket
http://Trinket.PySimpleGUI.org
## Packages Used In Demos
While the core PySimpleGUI code does not utilize any 3rd party packages, some of the demos do. They add a GUI to a few popular packages. These packages include:
* [Chatterbot](https://github.com/gunthercox/ChatterBot)
* [Mido](https://github.com/olemb/mido)
* [Matplotlib](https://matplotlib.org/)
* [PyMuPDF](https://github.com/rk700/PyMuPDF)
* OpenCV
* pymunk
* psutil
* pygame
* Forecastio
# Creating a Windows .EXE File
It's possible to create a single .EXE file that can be distributed to Windows users. There is no requirement to install the Python interpreter on the PC you wish to run it on. Everything it needs is in the one EXE file, assuming you're running a somewhat up to date version of Windows.
Installation of the packages, you'll need to install PySimpleGUI and PyInstaller (you need to install only once)
```bash
pip install PySimpleGUI
pip install PyInstaller
```
To create your EXE file from your program that uses PySimpleGUI, `my_program.py`, enter this command in your Windows command prompt:
```bash
pyinstaller -wF my_program.py
```
You will be left with a single file, `my_program.exe`, located in a folder named `dist` under the folder where you executed the `pyinstaller` command.
That's all... Run your `my_program.exe` file on the Windows machine of your choosing.
> "It's just that easy."
(famous last words that screw up just about anything being referenced)
Your EXE file should run without creating a "shell window". Only the GUI window should show up on your taskbar.
If you get a crash with something like:
```python
ValueError: script '.......\src\tkinter' not found
```
Then try adding **`--hidden-import tkinter`** to your command
# Creating a Mac App File
There are reports that PyInstaller can be used to create App files. It's not been officially tested.
Run this command on your Mac
> pyinstaller --onefile --add-binary='/System/Library/Frameworks/Tk.framework/Tk':'tk' --add-binary='/System/Library/Frameworks/Tcl.framework/Tcl':'tcl' your_program.py
Another also mentioned it may be helpful to add the "windowed" option so that a console is not opened. That should make the command:
> pyinstaller --onefile --add-binary='/System/Library/Frameworks/Tk.framework/Tk':'tk' --windowed --add-binary='/System/Library/Frameworks/Tcl.framework/Tcl':'tcl' your_program.py
This info was located on Reddit with the source traced back to:
https://github.com/pyinstaller/pyinstaller/issues/1350
---
# Known Issues
Well, there are a few quirks, and problems of course. Check the [GitHub Issues database](https://github.com/PySimpleGUI/PySimpleGUI/issues) for a list of them.
As previously mentioned **this is where you should post all problems and enhancements.**
Random crashes have been rare. The code is stable and hasn't been "quirky" nor have there been many "emergency" releases.
## MACS & tkinter
Macs and PySimpleGUI did not play well together up until Nov 2019 and the release of ttk buttons. Prior to that buttons had to be white. Now the Mac can use any color for buttons and they work great. Images on buttons work as well.
The problems were the normal tk.Button was not working correctly on the Mac. You couldn't set the button color. If you tried it appeared as if the text was missing.
Users have recently reported the ability to install Python 3.7 from the Python.org website and not use the Homebrew version. This resolved all of the button color problems.
Regardless of where you get your Python / tkinter, Macs can now enjoy using all of the look and feel color themes that Windows and Linux users are able to achieve.
Many PySimpleGUI users have switched from PySimpleGUI to PySimpleGUIQt due to the button problems. IF you're one of them, ***you should consider switching back***. One reason to return to PySimpleGUI is that features tend to get implemented on PySimpleGUI (tkinter version) and then later on the other ports. There are a number of other reasons to give tkinter another try.
## Multiple threads
Consider this is a ***stern warning***
### **Do not attempt** to call `PySimpleGUI` from multiple threads! At least the `tkinter` based port because tkinter is not threadsafe and has known issues with multiple threads
Tkinter also wants to be the MAIN thread in your code. So, if you have to run multiple threads, make sure the GUI is the main thread.
There is ONE (and only one?) safe call you can make into PySimpleGUI from a thread - `window.write_event_value`
There are several examples of using threads with PySimpleGUI. Use the Demo Programs! Use the Cookbook! They will give you an immediate and valuable jump start.
Be sure and **delete** your windows after you close them if you are running with multiple threads. There is a chance another thread's garbage collect will attempt to delete the window when not in the mainthread which will cause tkinter to crash.
### The dreaded "Tcl_AsyncDelete: async handler deleted by the wrong thread" error
This crash has plagued and mystified tkinter users for some time now. It happens when the user is running multiple threads in their application. Even if the user doesn't make any calls that are into tkinter, this problem can still cause your program to crash.
I'm thrilled to say there's a solution and it's easy to implement. If you're getting this error, then here is what is causing it.
When you close a window and delete the layout, the tkinter widgets that were in use in the window are no longer needed. Python marks them to be handled by the "Garbage Collector". They're deleted but not quite gone from memory. Then, later, while your thread is running, the Python Garbage Collect algorithm decides it's time to run garbage collect. When it tells tkinter to free up the memory, the tkinter code looks to see what context it is running under. It sees that it's a thread, not the main thread, and generates this exception.
The way around this is actually quite easy.
When you are finished with a window, be sure to:
* Close the Window
* Set the `layout` variable to None
* Set the `window` variable to None
* Trigger Python's Garbage Collect to run immediately
The sequence looks like this in code:
```python
import gc
# Do all your windows stuff... make a layout... show your window... then when time to exit
window.close()
layout = None
window = None
gc.collect()
```
This will ensure that the tkinter widgets are all deleted in the context of the main-thread and another thread won't accidentally run the Garbage Collect
# Contributing to PySimpleGUI
### Open Source License, but Private Development
PySimpleGUI is different than most projects on GitHub. It is licensed using the "Open Source License" LGPL3. However, the coding and development of the project is not "open source".
This project does not accept user submitted code.
#### Write Applications, Use PySimpleGUI, Write Tutorials, Teach Others
These are a few of the ways you can directly contribute to PySimpleGUI. Using the package to make cool stuff and helping others learn how to use it to make cool stuff and a big help to PySimpleGUI. **Everyone** learns from seeing other people's implementations. It's through user's creating applications that new problems and needs are discovered. These have had a profound and positive impact on the project in the past.
#### Pull Requests
Pull requests are *not being accepted* for the project. This includes sending code changes via other means than "pull requests". Plainly put, core code you send will not be used.
#### Bug Fixes
If you file an Issue for a bug, have located the bug, and found a fix in 10 lines of code or less.... and you wish to share your fix with the community, then feel free to include it with the filed Issue. If it's longer than 10 lines and wish to discuss it, then send an email to help@PySimpleGUI.org.
## Thank You
The support from the user community has been amazing. Your passion for creating PySimpleGUI applications is infectious. Every "thank you" is noticed and appreciated! Your passion for wanting to see PySimpleGUI improve is neither ignored nor unappreciated.
It's understood that this way of development of a Python package is unorthodox. You may find it frustrating and slow, but hope you can respect the decision for it to operate in this manner and be supportive.
## GitHub Repos
If you've created a GitHub for your project that uses PySimpleGUI then please post screenshots in in the "User's Screenshots" Issue on the PySimpleGUI GitHub. Say a little something about it and I'll also add it to the announcements. People *love* success stories and showing your GUI's screen visually communicates your success.
## Versions
|Version | Description |
|--|--|
| 1.0.9 | July 10, 2018 - Initial Release |
| 1.0.21 | July 13, 2018 - Readme updates |
| 2.0.0 | July 16, 2018 - ALL optional parameters renamed from CamelCase to all_lower_case
| 2.1.1 | July 18, 2018 - Global settings exposed, fixes
| 2.2.0| July 20, 2018 - Image Elements, Print output
| 2.3.0 | July 23, 2018 - Changed form.Read return codes, Slider Elements, Listbox element. Renamed some methods but left legacy calls in place for now.
| 2.4.0 | July 24, 2018 - Button images. Fixes so can run on Raspberry Pi
| 2.5.0 | July 26, 2018 - Colors. Listbox scrollbar. tkinter Progress Bar instead of homegrown.
| 2.6.0 | July 27, 2018 - auto_size_button setting. License changed to LGPL 3+
| 2.7.0 | July 30, 2018 - realtime buttons, window_location default setting
| 2.8.0 | Aug 9, 2018 - New None default option for Checkbox element, text color option for all elements, return values as a dictionary, setting focus, binding return key
| 2.9.0 | Aug 16,2018 - Screen flash fix, `do_not_clear` input field option, `autosize_text` defaults to `True` now, return values as ordered dict, removed text target from progress bar, rework of return values and initial return values, removed legacy Form.Refresh() method (replaced by Form.ReadNonBlockingForm()), COLUMN elements!!, colored text defaults
| 2.10.0 | Aug 25, 2018 - Keyboard & Mouse features (Return individual keys as if buttons, return mouse scroll-wheel as button, bind return-key to button, control over keyboard focus), SaveAs Button, Update & Get methods for InputText, Update for Listbox, Update & Get for Checkbox, Get for Multiline, Color options for Text Element Update, Progress bar Update can change max value, Update for Button to change text & colors, Update for Image Element, Update for Slider, Form level text justification, Turn off default focus, scroll bar for Listboxes, Images can be from filename or from in-RAM, Update for Image). Fixes - text wrapping in buttons, msg box, removed slider borders entirely and others
| 2.11.0 | Aug 29, 2018 - Lots of little changes that are needed for the demo programs to work. Buttons have their own default element size, fix for Mac default button color, padding support for all elements, option to immediately return if list box gets selected, FilesBrowse button, Canvas Element, Frame Element, Slider resolution option, Form.Refresh method, better text wrapping, 'SystemDefault' look and feel setting
| 2.20.0 | Sept 4, 2018 - Some sizable features this time around of interest to advanced users. Renaming of the MsgBox functions to Popup. Renaming GetFile, etc, to PopupGetFile. High-level windowing capabilities start with Popup, PopupNoWait/PopupNonblocking, PopupNoButtons, default icon, change_submits option for Listbox/Combobox/Slider/Spin/, New OptionMenu element, updating elements after shown, system default color option for progress bars, new button type (Dummy Button) that only closes a window, SCROLLABLE Columns!! (yea, playing in the Big League now), LayoutAndShow function removed, form.Fill - bulk updates to forms, FindElement - find element based on key value (ALL elements have keys now), no longer use grid packing for row elements (a potentially huge change), scrolled text box sizing changed, new look and feel themes (Dark, Dark2, Black, Tan, TanBlue, DarkTanBlue, DarkAmber, DarkBlue, Reds, Green)
| 2.30.0 | Sept 6, 2018 - Calendar Chooser (button), borderless windows, load/save form to disk
| 3.0.0 | Sept 7, 2018 - The "fix for poor choice of 2.x numbers" release. Color Chooser (button), "grab anywhere" windows are on by default, disable combo boxes, Input Element text justification (last part needed for 'tables'), Image Element changes to support OpenCV?, PopupGetFile and PopupGetFolder have better no_window option
| 3.01.01 | Sept 10, 2018 - Menus! (sort of a big deal)
| 3.01.02 | Step 11, 2018 - All Element.Update functions have a `disabled` parameter so they can be disabled. Renamed some parameters in Update function (sorry if I broke your code), fix for bug in Image.Update. Wasn't setting size correctly, changed grab_anywhere logic again,added grab anywhere option to PupupGetText (assumes disabled)
| 3.02.00 | Sept 14, 2018 - New Table Element (Beta release), MsgBox removed entirely, font setting for InputText Element, **packing change** risky change that allows some Elements to be resized,removed command parameter from Menu Element, new function names for ReadNonBlocking (Finalize, PreRead), change to text element autosizing and wrapping (yet again), lots of parameter additions to Popup functions (colors, etc).
| 3.03.00 | New feature - One Line Progress Meters, new display_row_numbers for Table Element, fixed bug in EasyProgresssMeters (function will soon go away), OneLine and Easy progress meters set to grab anywhere but can be turned off.
| 03,04.00 | Sept 18, 2018 - New features - Graph Element, Frame Element, more settings exposed to Popup calls. See notes below for more.
| 03.04.01 | Sept 18, 2018 - See release notes
| 03.05.00 | Sept 20, 2018 - See release notes
| 03.05.01 | Sept 22, 2018 - See release notes
| 03.05.02 | Sept 23, 2018 - See release notes
| 03.06.00 | Sept 23, 2018 - Goodbye FlexForm, hello Window
| 03.08.00 | Sept 25, 2018 - Tab and TabGroup Elements\
| 01.00.00 for 2.7 | Sept 25, 2018 - First release for 2.7
| 03.08.04 | Sept 30, 2018 - See release notes
| 03.09.00 | Oct 1, 2018 |
| 2.7 01.01.00 | Oct 1, 2018
| 2.7 01.01.02 | Oct 8, 2018
| 03.09.01 | Oct 8, 2018
| 3.9.3 & 1.1.3 | Oct 11, 2018
| 3.9.4 & 1.1.4 | Oct 16, 2018
| 3.10.1 & 1.2.1 | Oct 20, 2018
| 3.10.3 & 1.2.3 | Oct 23, 2018
| 3.11.0 & 1.11.0 | Oct 28, 2018
| 3.12.0 & 1.12.0 | Oct 28, 2018
| 3.13.0 & 1.13.0 | Oct 29, 2018
| 3.14.0 & 1.14.0 | Nov 2, 2018
| 3.15.0 & 1.15.0 | Nov 20, 2018
| 3.16.0 & 1.16.0 | Nov 26, 2018
| 3.17.0 & 1.17.0 | Dec 1, 2018
## Release Notes
2.3 - Sliders, Listbox's and Image elements (oh my!)
If using Progress Meters, avoid cancelling them when you have another window open. It could lead to future windows being blank. It's being worked on.
New debug printing capability. `sg.Print`
2.5 Discovered issue with scroll bar on `Output` elements. The bar will match size of ROW not the size of the element. Normally you never notice this due to where on a form the `Output` element goes.
Listboxes are still without scrollwheels. The mouse can drag to see more items. The mouse scrollwheel will also scroll the list and will `page up` and `page down` keys.
2.7 Is the "feature complete" release. Pretty much all features are done and in the code
2.8 More text color controls. The caller has more control over things like the focus and what buttons should be clicked when enter key is pressed. Return values as a dictionary! (NICE addition)
2.9 COLUMNS! This is the biggest feature and had the biggest impact on the code base. It was a difficult feature to add, but it was worth it. Can now make even more layouts. Almost any layout is possible with this addition.
.................. insert releases 2.9 to 2.30 .................
3.0 We've come a long way baby! Time for a major revision bump. One reason is that the numbers started to confuse people the latest release was 2.30, but some people read it as 2.3 and thought it went backwards. I kinda messed up the 2.x series of numbers, so why not start with a clean slate. A lot has happened anyway so it's well earned.
One change that will set PySimpleGUI apart is the parlor trick of being able to move the window by clicking on it anywhere. This is turned on by default. It's not a common way to interact with windows. Normally you have to move using the titlebar. Not so with PySimpleGUI. Now you can drag using any part of the window. You will want to turn off for windows with sliders. This feature is enabled in the Window call.
Related to the Grab Anywhere feature is the no_titlebar option, again found in the call to Window. Your window will be a spiffy, borderless window. It's a really interesting effect. Slight problem is that you do not have an icon on the taskbar with these types of windows, so if you don't supply a button to close the window, there's no way to close it other than task manager.
3.0.2 Still making changes to Update methods with many more ahead in the future. Continue to mess with grab anywhere option. Needed to disable in more places such as the PopupGetText function. Any time these is text input on a form, you generally want to turn off the grab anywhere feature.
#### 3.2.0
Biggest change was the addition of the Table Element. Trying to make changes so that form resizing is a possibility but unknown if will work in the long run. Removed all MsgBox, Get* functions and replaced with Popup functions. Popups had multiple new parameters added to change the look and feel of a popup.
#### 3.3.0
OneLineProgressMeter function added which gives you not only a one-line solution to progress meters, but it also gives you the ability to have more than 1 running at the same time, something not possible with the EasyProgressMeterCall
#### 3.4.0
* Frame - New Element - a labelled frame for grouping elements. Similar
to Column
* Graph (like a Canvas element except uses the caller's
coordinate system rather than tkinter's).
* initial_folder - sets starting folder for browsing type buttons (browse for file/folder).
* Buttons return key value rather than button text **If** a `key` is specified,
*
OneLineProgressMeter! Replaced EasyProgressMeter (sorry folks that's
the way progress works sometimes)
* Popup - changed ALL of the Popup calls to provide many more customization settings
* Popup
* PopupGetFolder
* PopupGetFile
* PopupGetText
* Popup
* PopupNoButtons
* PopupNonBlocking
* PopupNoTitlebar
* PopupAutoClose
* PopupCancel
* PopupOK
* PopupOKCancel
* PopupYesNo
#### 3.4.1
* Button.GetText - Button class method. Returns the current text being shown on a button.
* Menu - Tearoff option. Determines if menus should allow them to be torn off
* Help - Shorcut button. Like Submit, cancel, etc
* ReadButton - shortcut for ReadFormButton
#### 3.5.0
* Tool Tips for all elements
* Clickable text
* Text Element relief setting
* Keys as targets for buttons
* New names for buttons:
* Button = SimpleButton
* RButton = ReadButton = ReadFormButton
* Double clickable list entries
* Auto sizing table widths works now
* Feature DELETED - Scaling. Removed from all elements
#### 3.5.1
* Bug fix for broken PySimpleGUI if Python version < 3.6 (sorry!)
* LOTS of Readme changes
#### 3.5.2
* Made `Finalize()` in a way that it can be chained
* Fixed bug in return values from Frame Element contents
#### 3.6.0
* Renamed FlexForm to Window
* Removed LookAndFeel capability from Mac platform.
#### 3.8.0
* Tab and TabGroup Elements - awesome new capabilities
#### 1.0.0 Python 2.7
It's official. There is a 2.7 version of PySimpleGUI!
#### 3.8.2
* Exposed `TKOut` in Output Element
* `DrawText` added to Graph Elements
* Removed `Window.UpdateElements`
* `Window.grab_anywere` defaults to False
#### 3.8.3
* Listbox, Slider, Combobox, Checkbox, Spin, Tab Group - if change_submits is set, will return the Element's key rather than ''
* Added change_submits capability to Checkbox, Tab Group
* Combobox - Can set value to an Index into the Values table rather than the Value itself
* Warnings added to Drawing routines for Graph element (rather than crashing)
* Window - can "force top level" window to be used rather than a normal window. Means that instead of calling Tk to get a window, will call TopLevel to get the window
* Window Disable / Enable - Disables events (button clicks, etc) for a Window. Use this when you open a second window and want to disable the first window from doing anything. This will simulate a 'dialog box'
* Tab Group returns a value with Window is Read. Return value is the string of the selected tab
* Turned off grab_anywhere for Popups
* New parameter, default_extension, for PopupGetFile
* Keyboard shortcuts for menu items. Can hold ALT key to select items in men
* Removed old-style Tabs - Risky change because it hit fundamental window packing and creation. Will also break any old code using this style tab (sorry folks this is how progress happens)
#### 3.8.6
* Fix for Menus.
* Fixed table colors. Now they work
* Fixed returning keys for tabs
* Window Hide / UnHide methods
* Changed all Popups to remove context manager
* Error checking for Graphing objects and for Element Updates
### 3.9.0 & 1.1.0
* The FIRST UNIFIED version of the code!
* Python 2.7 got a TON of features . Look back to 1.0 release for the list
* Tab locations - Can place Tabs on top, bottom, left, right now instead of only the top
### 3.9.1 & 1.1.2
* Tab features
* Themes
* Enable / Disable
* Tab text colors
* Selected tab color
* New GetListValues method for Listbox
* Can now have multiple progress bars in 1 window
* Fix for closing debug-output window with other windows open
* Topanga Look and Feel setting
* User can create new look and feel settings / can access the look and feel table
* New PopupQuick call. Shows a non-blocking popup window with auto-close
* Tree Element partially done (don't use despite it showing up)
### 3.9.3 & 1.1.3
* Disabled setting when creating element for:
* Input
* Combo
* Option Menu
* Listbox
* Radio
* Checkbox
* Spinner
* Multiline
* Buttons
* Slider
* Doc strings on all Elements updated
* Buttons can take image data as well as image files
* Button Update can change images
* Images can have background color
* Table element new num_rows parameter
* Table Element new alternating_row_color parameter
* Tree Element
* Window Disappear / Reappear methods
* Popup buttons resized to same size
* Exposed look and feel table
### 3.9.4 & 1.1.4
* Parameter order change for Button.Update so that new button ext is at front
* New Graph.DrawArc method
* Slider tick interval parameter for labeling sliders
* Menu tearoff now disabled by default
* Tree Data printing simplified and made prettier
* Window resizable parameter. Defaults to not resizable
* Button images can have text over them now
* BUG fix in listbox double-click. First bug fix in months
* New Look And Feel capability. List predefined settings using ListOfLookAndFeelValues
### 3.10.1 & 1.2.1
* Combobox new readonly parameter in init and Update
* Better default sizes for Slider
* Read of Tables now returns which rows are selected (big damned deal feature)
* PARTIAL support of Table.Update with new values (use at your own peril)
* Alpha channel setting for Windows
* Timeout setting for Window.Read (big damned deal feature)
* Icon can be base64 image now in SetIcon call
* Window.FindElementWithFocus call
* Window.Move allows moving window anywhere on screen
* Window.Minimize will minimize to taskbar
* Button background color can be set to system default (i.e. not changed)
### 3.10.2 & 1.2.2
Emergency patch release... going out same day as previous release
* The timeout timer for the new Read with timer wasn't being properly shut down
* The Image.Update method appears to not have been written correctly. It didn't handle base64 images like the other elements that deal with images (buttons)
### 3.10.3 & 1.2.3
* New element - Vertical Separator
* New parameter for InputText - change_submits. If True will cause Read to return when a button fills in the InputText element
* Read with timeout = 0 is same as read non blocking and is the new preferred method
* Will return event == None if window closed
* New Close method will close all window types
* Scrollbars for Tables automatically added (no need for a Column Element)
* Table Update method complete
* Turned off expand when packing row frame... was accidentally turned on (primary reason for this release)
* Try added to Image Update so won't crash if bad image passed in
### 3.11.0 & 1.11.0
* Syncing up the second digit of the releases so that they stay in sync better. the 2.7 release is built literally from the 3.x code so they really are the same
* Reworked Read call... significantly.
* Realtime buttons work with timeouts or blocking read
* Removed default value parm on Buttons and Button Updates
* New Tree Element parm show_expanded. Causes Tree to be shown as fully expanded
* Tree Element now returns which rows are selected when Read
* New Window method BringToFront
* Shortcut buttons no longer close windows!
* Added CloseButton, CButton that closes the windows
### 3.12.0 & 1.12.0
* Changed Button to be the same as ReadButton which means it will no longer close the window
* All shortcut buttons no longer close the window
* Updating a table clears selected rows information in return values
* Progress meter uses new CloseButton
* Popups use new CloseButton
### 3.13.0 & 1.13.0
* Improved multiple window handling of Popups when the X is used to close
* Change submits added for:
* Multiline
* Input Text
* Table
* Tree
* Option to close calendar chooser when date selected
* Update for Tree Element
* Scroll bars for Trees
### 3.14.0 & 1.14.0
* More windowing changes...
* using a hidden root windowing (Tk())
* all children are Toplevel() windows
* Read only setting for:
* Input Text
* Multiline
* Font setting for InputCombo, Multiline
* change_submits setting for Radio Element
* SetFocus for multiline, input elements
* Default mon, day, year for calendar chooser button
* Tree element update, added ability to change a single key
* Message parm removed from ReadNonBlocking
* Fix for closing windows using X
* CurrentLocation method for Windows
* Debug Window options
* location
* font
* no_button
* no_titlebar
* grab_anywhere
* keep_on_top
* New Print / EasyPrint options
* location
* font
* no_button
* no_titlebar
* grab_anywhere
* keep_on_top
* New popup, PopupQuickMessage
* PopupGetFolder, PopupGetFile new initial_folder parm
### 3.15.0 & 1.15.0
* Error checking for InputText.Get method
* Text color, background color added to multiline element.Update
* Update method for Output Element - gives ability to clear the output
* Graph Element - Read returns values if new flages set
* Change submits, drag submits
* Returns x,y coordinates
* Column element new parm vertical_scroll_only
* Table element new parm - bind return key - returns if return or double click
* New Window parms - size, disable_close
* "Better" multiwindow capabilities
* Window.Size property
* Popups - new title parm, custom_text
* title sets the window title
* custom_text - single string or tuple string sets text on button(s)
### 3.16.0 & 1.16.0
* Bug fix in PopupScrolled
* New `Element` shortcut function for `FindElement`
* Dummy Stretch Element made for backwards compatibility with Qt
* Timer function prints in milliseconds now, was seconds
### 3.17.0 &1.17.0 2-Dec-2018
3.17.0 2-Dec-2017
* Tooltip offset now programmable. Set variable DEFAULT_TOOLTIP_OFFSET. Defaults to (20,-20)
* Tooltips are always on top now
* Disable menu items
* Menu items can have keys
* StatusBar Element (preparing for a real status bar in Qt)
* enable_events parameter added to ALL Elements capable of generating events
* InputText.Update select parameter will select the input text
* Listbox.Update - set_to_index parameter will select a single items
* Menus can be updated!
* Menus have an entry in the return values
* LayoutAndRead depricated
* Multi-window support continues (X detection)
* PopupScrolled now has a location parameter
* row_height parameter to Table Element
* Stretch Element (DUMMY) so that can be source code compatible with Qt
* ButtonMenu Element (DUMMY) so can be source code compat with Qt. Will implement eventually
## 3.18.0 11-Dec-2018
NOTE - **Menus are broken** on version 2.7. Don't know how long they've been this way. Please get off legacy Python if that's what you're running.
* Default progress bar length changed to shorter
* Master window and tracking of num open windows moved from global to Window class variable
* Element visibility setting (when created and when Updating element)
* Input text visiblity
* Combo visiblity
* Combo replaces InputCombo as the primary class name
* Option menu visibility
* Listbox visiblity
* Listbox new SetFocus method
* Radio visibility
* Checkbox visibility
* Spin visiblity
* Spin new Get method returns current value
* Multiline visiblity
* Text visibility
* StatusBar visiblity
* Output visibility
* Button visibility
* Button SetFocus
* ProgressBar - New Update method (used only for visibility)
* Image - clickable images! enable_events parameter
* Image visibility
* Canvas visibility
* Graph visibility
* Graph - new DrawImage capability (finally)
* Frame visibility
* Tab visibility (may not be fully functional)
* TabGroup visibility
* Slider visibility
* Slider - new disable_number_display parameter
* Column visibilty
* Menu visibility - Not functional
* Table visibility
* Table - new num_rows parm for Update - changes number of visible rows
* Tree visiblity
* Window - New element_padding parameter will get padding for entire window
* OneLineProgressMeter - Completely REPLACED the implementation
* OneLineProgressMeter - can get reason for the cancellation (cancel button versus X)
* EasyProgressMeter - completely removed. Use OneLineProgressMeter instead
* Debug window, EasyPrint, Print - debug window will re-open if printed to after being closed
* SetOptions - can change the error button color
* Much bigger window created when running PySimpleGUI.py by itself. Meant to help with regression testing
## 3.19.2 13-Dec-2018
* Warning for Mac's when trying to change button color
* New parms for Button.Update - image_size and image_subsample
* Buttons - remove highlight when border depth == 0
* OneLineProgressMeter - better layout implementation
## 3.20.0 & 1.20.0 18-Dec-2018
* New Pane Element
* Graph.DeleteFigure method
* disable_minimize - New parameter for Window
* Fix for 2.7 menus
* Debug Window no longer re-routes stdout by default
* Can re-route by specifying in Print / EasyPrint call
* New non-blocking for PopupScrolled
* Can set title for PopupScrolled window
## 3.21.0 & 1.21.0 28-Dec-2018
* ButtonMenu Element
* Embedded base64 default icon
* Input Text Right click menu
* Disabled Input Text are now 'readonly' instead of disabled
* Listbox right click menu
* Multiline right click menu
* Text right click menu
* Output right click menu
* Image right click menu
* Canvas right click menu
* Graph right click menu
* Frame right click menu
* Tab, tabgroup right click menu (unsure if works correctly)
* Column right click menu
* Table right click menu
* Tree right click menu
* Window level right click menu
* Window icon can be filename or bytes (Base64 string)
* Window.Maximize method
* Attempted to use Styles better with Combobox
* Fixed bug blocking setting bar colors in OneLineProgressMeter
# 3.22.0 PySimpleGUI / 1.22.0 PySimpleGUI27
* Added type hints to some portions of the code
* Output element can be made invisible
* Image sizing and subsample for Button images
* Invisibility for ButtonMenusup
* Attempt at specifying size of Column elements (limited success)
* Table Element
* New row_colors parameter
* New vertical_scroll_only parameter - NOTE - will have to disable to get horizontal scrollbars
* Tree Element
* New row_height parameter
* New feature - Icons for tree entries using filename or Base64 images
* Fix for bug sending back continuous mouse events
* New parameter silence_on_error for FindElement / Element calls
* Slider returns float now
* Fix for Menus when using Python 2.7
* Combobox Styling (again)
# 3.2.0 PySimpleGUI / 1.23.0 PySimpleGUI27 16-Jan-2019
* Animated GIFs!
* Calendar Chooser stays on top of other windows
* Fixed bug of no column headings for Tables
* Tables now use the font parameter
# 3.24.0 1.24.0 16-Jan-2019
* PopupAnimated - A popup call for showing "loading" type of windows
# 3.25 & 1.25 20-Feb-2019
* Comments :-)
* Convert Text to string right away
* Caught exceptions when main program shut down with X
* Caught exceptions in all of the graphics primitives
* Added parameter exportselection=False to Listbox so can use multiple listboxes
* OneLineProgressMeter - Can now change the text on every call if desired
## 3.27.0 PySimpleGUI 31-Mar-2019
Mixup.... 3.26 changes don't appear to have been correctly released so releasing in 3.27 now
* do_not_clear now defaults to TRUE!!!
* Input Element
* Multiline Element
* Enable Radio Buttons to be in different containers
* Ability to modify Autoscroll setting in Multiline.Update call
* PopupGetFolder, PopupGetFile, PopupGetText - title defaults to message if none provided
* PopupAnimated - image_source can be a filename or bytes (base64)
* Option Menu can now have values updated
## 3.28.0 11-Apr-2019 PySimpleGUI
* NEW Window Parameter - layout - second parameter. Can pass in layout directly now!
* New shortcuts
* I = InputText
* B = Btn = Butt = Button
* Convert button text to string when creating buttons
* Buttons are returned now as well as input fields when searching for element with focus
## 3.29 22-Apr-2019
* New method for `Graph` - `RelocateFigure`
* Output Element no longer accepts focus
## 3.32.0 PySimpleGUI 24-May-2019
* Rework of ALLL Tooltips. Was always displaying at uttuper left part of element. Not displays closer to where mouse entered or edited
* New Element.Widget base class variable. Brings tkinter into the newer architecture of user accessibility to underlying GUI Frameworks' widgets
* New SetTooltip Element method. Means all Elements gain this method. Can set the tooltip on the fly now for all elements
* Include scroll bar when making visible / invisible Listbox Elements
* New Radio Element method - `Radio.ResetGroup()` sets all elements in the Radio Group to False* Added borderwidth to Multiline Element
* `Button.Click()` - new method - Generates a button click even as if a user clicked a button (at the tkinter level)
* Made a Graph.Images dictionary to keep track of images being used in a graph. When graph is deleted, all of the accociated images should be deleted too.'
* Added `Graph.SetFocus()` to give a Graph Element the focus just as you can input elements
* Table new parameter - `hide_vertical_scroll` if True will hide the table's vertical bars
* Window - new parameter - `transparent_color`. Causes a single color to become completely transparent such that you see through the window, you can click through the window. Its like tineows never was there.
* The new `Window.AllKeysDict = {}` has been adopted by all PySimpleGUI ports. It's a new method of automatically creating missing keys, storing and retrieving keys in general for a window.
* Changed how `window.Maximize` is implemented previously used the '-fullscreen' attribute. Now uses the 'zoomed' state
* Window gets a new `Normal()` method to return from Maximize state. Sets root.state('normal')
* Window.Close() now closes the special `Window.hidden_master_root` window when the "last" window is closed
* `Window.SetTransparentColor` method added. Same effect as if window was created with parameter set
* An Element's Widget stored in `.Widget` attribute
* Making ComboBox's ID unique by using it's Key
* Changed Multiline to be sunken and have a border depth setting now
* Removed a second canvas that was being used for Graph element.
* Changed how no titlebar is implemented running on Linux versus Windows. -type splash now used for Linux
* PopupScrolled - Added back using CloseButton to close the window
* Fixed PopupGetFolder to use correct PySimpleGUI program constructs (keys)
* PopupGetText populated values carrectly using the value variable, used keys
* PopupAnimated finally gets a completely transparent background
## 3.33.0 and 1.33 PySimpleGUI 25-May-2019
* Emergency fix due to debugger. Old bug was that Image Element was not testing for COLOR_SYSTEM_DEFAULT correctly.
## 3.34.0 PySimpleGUI & 1.34.0 PySimpleGUI27 25-May-2019
pip rhw w cenf
* Fixed Window.Maximize and Window.Normal - needed special code for Linux
* Check for DEFAULT_SCROLLBAR_COLOR not being the COLOR_SYSTEM_DEFAULT (crashed)
## 3.35 PySimpleGUI & 1.35 PySimpleGUI27 27-May-2019
* Bug fix - when setting default for Checkbox it was also disabling the element!
## 3.36 PySimpleGUI & 1.36 PySimpleGUI27 29-May-2019
A combination of user requests, and needs of new `imwatchingyou` debugger
* New Debugger Icon for future built-in debugger
* Fixed bug in FindBoundReturnKey - needed to also check Panes
* NEW Window functions to turn on/off the Grab Anywhere feature
* `Window.GrabAnyWhereOn()`
* `Window.GrabAnyWhereOff()`
* New "Debugger" button that's built-in like other buttons. It's a TINY button with a logo. For future use when a debugger is built into PySimpleGUI itself (SOON!)
* Change Text Element Wrap Length calculation. Went fromn +40 pixels to +10 pixels in formula
* PopupGetFile has new parameter - `multiple_files`. If True then allows selection of multiple files
## 3.37 PySimpleGUI & 1.37 PySimpleGUI27 1-June-2019
* The built-in debugger is HERE - might not WORK exactly yet, but a lot of code went into te PySimpleGUI.py file for this. At the moment, the `imwatchingyou` package is THE way to use a PySimpleGUI debugger. But soon enough you won't need that project in order to debug your program.
* Some strange code reformatting snuck in. There are 351 differences between this and previous release. I'm not sure what happened but am looking at every change by hand.
* New Calendar Button features
* locale, format - new parameters to TKCalendar call
* Use custom icon for window if one has been set
* New parameters to CalendarButton - `locale`, `format`
* The bulk of the built-in PySimpleGUI debugger has been added but is not yet "officially supported". Try pressing "break" or "ctrl+break" on your keyboard.
* New bindings for break / pause button and debugger
* New Debug button will launch debugger.
* New parameter `debugger_enabled` added to Window call. Default is __enabled__.
* Your progam's call to Read is all that's needed to refresh debugger
* New `Window` methods to control debugger access
* `EnableDebugger` - turns on HOTKEYS to debugger
* `DisableDebugger` - turns off HOTKEYS to debugger
* Restored wrap len for Text elements back from +10 to +40 pixels
* `PopupGetFolder`, `PopupGetFile` - fixed so that the "hidden" master window stays hidden (a Linux problem)
* Added support for Multiple Files to `PopupGetFiles` when no_window option has been set.
## 3.38 PySimpleGUI, 1.38 PySimpleGUI27
* Multiline - now has a "read only" state if created as "Disabled"
* Multiline - If window is created as resizable, then Multiline Elements will now expand when the window is enlarged, a feature long asked for.
* Output Element expands in the Y Direction
* "Expandable Rows" option added to PackFormIntoFrame allowing future elements to also expand
* Error Element - silence_on_error option
* Text Element wrapping - FINALLY got it right? No more "Fudge factor" added
* PopupScrolled - Windows are now resizable
* Option to "launch built-in debugger" from the test harness
* Rememeber that the Debugger is still in this code! It may or may not be operational as it's one version back from the latest release of the `imwatchingyou` debugger code. This code needs to be integrated back in
## 3.39 PySimpleGUI & 1.39 PySimpleGUI27 13-June-2019
* Ported the imwatchingyou debugger code into PySimpleGUI code
* Replaced old debugger built-in code with the newer imwatchingyou version
* Required removing all of the 'sg.' before PySimpleGUI calls since not importing
* Dynamically create the debugger object when first call to `refresh` or `show` is made
* Started the procecss of renaming Class Methods that are private to start with _
* Needed for the automatic documentation generation that's being worked on
* Fixed crash when clicking the Debug button
* Fixed bug in DeleteFigure. Needed to delete image separately
* Added more type hints
* New `TabGroup` method `SelectTab(index)` selects a `Tab` within a `TabGroup`
* New `Table.Update` parameter - `select_rows`. List of rows to select (0 is first)
* Error checking in `Window.Layout` provides error "hints" to the user
* Looks for badly placed ']'
* Looks for functions missing '()'
* Pops up a window warning user instead of crashing
* May have to revisit if the popups start getting in the way
* New implementations of `Window.Disable()` and `Window.Enable()`
* Previously did not work correctly at all
* Now using the "-disabled" attribute
* Allow Comboboxes to have empty starting values
* Was crashing
* Enables application to fill these in later
# 4.0.0 PySimpleGUI & 2.0.0 PySimpleGUI27 19-June-2019
* DOC STRINGS DOCS STRINGS DOC STRINGS!
* Your IDE is about to become very happy
* All Elements have actual documentation in the call signature
* The Readme and ReadTheDocs will be generated going forward using the CODE
* HUGE Thanks for @nngogol for both copying & adding all those strings, but also for making an entire document creation system.
* New __version__ string for PySimpleGUI.py
* New parameter to ALL `SetFocus` calls.
* def SetFocus(self, force=False)
* If force is True, then a call to `focus_force` is made instead of `focus_set`
* Get - New Radio Button Method. Returns True is the Radio Button is set
* Rename of Debugger class to _Debugger so IDEs don't get confused
* User read access to last Button Color set now available via property `Button.ButtonColor`
* Rename of a number of callback handlers to start with _
* Fix for memory leak in Read call. Every call to read lost a little memory due to root.protocol calls
* Listbox.Update - New parameter - scroll_to_index - scroll view so that index is shown at the top
* First PyPI release to use new documentation!
## PySimpleGUI 4.1 Anniversary Release! 4-Aug-2019
NEVER has there been this long of a lag, sorry to all users!
Long time coming. Docstrings continue to be a focus.
* Version can be found using PySimpleGUI.version
* New bit of licensing info at the top of the file
* Types used in the doc strings. Also type hints in some comments. Because also running on 2.7 can't use full typing
* Added using of Warnings. Just getting started using this mechanism. May be great, maybe not. We'll see with this change
* Added TOOLTIP_BACKGROUND_COLOR which can be changed (it's tkinter only setting however so undertand this!)
* Graph.DrawText. Ability to set `text_location` when drawing text onto a Graph Element. Determines what part of the text will be located at the point you provide when you draw the text. Choices are:
* TEXT_LOCATION_TOP
* TEXT_LOCATION_BOTTOM
* TEXT_LOCATION_LEFT
* TEXT_LOCATION_RIGHT
* TEXT_LOCATION_TOP_LEFT
* TEXT_LOCATION_TOP_RIGHT
* TEXT_LOCATION_BOTTOM_LEFT
* TEXT_LOCATION_BOTTOM_RIGT
* TEXT_LOCATION_CENTER
* Flag ENABLE_TK_WINDOWS = False. If True, all windows will be made using only tk.Tk()
* SetFocus available for all elements now due to it being added to base class. May NOT work on all elements however
* Added Combo.GetSElectedItemsIndexes() - returns a list of all currently selected items
* Fixed Listbox.Update - set_to_index changed to be an int, list or tuple
* Added parent parameter to call to tkinter's askopenfilename, directory, filenames. Not sure why the root wasn't passed in before
* Button.Update - also sets the activebackground to the button's background color
* Graph - New parameter when creating. `float_values`. If True, then you're indicating that your coordinate system is float not int based
* Graph.Update - made background color optional parm so that visible only can be set
* Frame.Layout returns self now for chaining
* TabGroup.Layout returns self now for chaining
* Column.Layout returns self now for chaining
* Menu.Update menu_definition is now optional to allow for changing visibility only
* Added inivisiblity support for menu bars
* Table.Update supports setting alternating row color and row_colors (list of rows and the color to set)
* Set window.TimeoutKey to TIMEOUT_KEY initially
* Window - check for types for title (should be string) and layout (should be list) and warns user if not correct
* Window - renamed some methods by adding _ in front (like Show) as they are NOT user callable
* Another shortcut! Elem = Element = FindElement
* SaveToDisk - will not write buttons to file. Fixed problems due to buttons having keys
* Remapped Windowl.CloseNonBlockingForm, Window.CloseNonBlocking to be Window.CloseNonBlocking
* Fix for returning values from a combo list. Wasn't handling current value not in list of provided values
* Spin - Returns an actual value from list provided when Spin was created or updated
* Chaneged FillFormWithValues to use the new internal AllKeysDict dictionary
* Added try when creating combo. Problem happens when window is created twice. Prior window had already created the style
* Added list of table (tree) ids to the Table element
* Enabled autoclose to use fractions of a second
* Added a try around one of the destroys because it could fail if user aborted
* Popup - Icon is no longer set to default by default
* Fix for debugger trying to execute a REPL comand. The exec is only avilable in Python 3
* main() will display the version number in big letters when program is running
### 4.2 PySimpleGUI 2.2 for PySimpleGUI27 18 - Aug 2019
The cool lookup release! No more need for FindElement. You can continue to use FindElement.
However, your code will look weird and ancient. ;-) (i.e. readable)
MORE Docstring and main doc updates!
* Finally 2.7 gets an upgrade and with it doc strings. It however doesn't get a full-version bump like main PySimpleGUI as this may be its last release.
* New `window[key] == window.FindElement(key)`
* New Update calling method. Can directly call an Element and it will call its Update method
* `window[key](value=new_value) == window.FindElement(key).Update(value=new_value)`
* Made Tearoff part of element so anything can be a menu in theory
* Removed a bunch of `__del__` calls. Hoping it doesn't bite me in memory leaks
* Combo.Get method added
* Combo.GetSelectedItemsIndexes removed
* New Graph methods SendFigureToBack, BringFigureToFront
* Butten release changed for better Graph Dragging
* Now returns key+"Up" for the event
* Also returns the x,y coords in the values
* Tab.Select method added
* TabGroup.Get method added - returns key of currently selected Tab
* Window finalize parameter added - Will call finalize if a layout is also included. No more need for Finalize!!
* Quiet, steady change to PEP8 user interface started
* Now available are Window methods - read, layout, finalize, find_element, element, close
* Should provide 100% PEP with these alone for most PySimpleGUI programs
* Added finding focus across ALL elements by using the .Widget member variable
* Fixed sizing Columns! NOW they will finally be the size specified
* Fixed not using the initialdir paramter in PopupGetFile if the no_window option is set
## 4.3 PySimpleGUI Release 22-Aug-2019
PEP8 PEP8 PEP8
Layout controls! Can finally center stuff
Some rather impactful changes this time
Let's hope it doesn't all blow up in our faces!
* PEP8 interfaces added for Class methods & functions
* Finally a PEP8 compliant interface for PySimpleGUI!!
* The "old CamelCase" are still in place and will be for quite some time
* Can mix and match at will if you want, but suggest picking one and sticking with it
* All docs and demo programs will need to be changed
* Internally saving parent row frame for layout checks
* Warnings on all Update calls - checks if Window.Read or Window.Finalize has been called
* Warning if a layout is attempted to be used twice
* Shows an "Error Popup" to get the user's attention for sure
* Removed all element-specific SetFocus methods and made it available to ALL elements
* Listbox - no_scrollbar parameter added. If True then no scrollbar will be shown
* NEW finalize bool parameter added to Window. Removes need to "chain" .Finalize() call.
* NEW element_justification parameter for Column, Frame, Tab Elements and Window
* Valid values are 'left', 'right', 'center'. Only first letter checked so can use 'l', 'c','r'
* Default = 'left'
* Result is that all Elements INSIDE of this container will be justified as specified
* Works well with new Sizer Elements
* NEW justification parameter for Column elements.
* Justifies Column AND the row it's on to this setting (left, right, center)
* Enables individual rows to be justified in addition to the entire window
* NEW Sizer Element
* Has width and height parameters. Can set one or both
* Causes the element it is contained within to expand according to width and height of Sizer Element
* Helps greatly with centering. Frames will shrink to fit the contents for example. Use Sizer to pad out to right size
* Added Window.visibility_changed to match the PySimpleGUIQt call
* Fixed Debugger so that popout window shows any newly added locals
## 4.4 PySimpleGUI Release 5-Sep-2019
* window() - "Calling" your Window object will perform a Read call
* InputText - move cursor to end following Update
* Shortcuts - trying to get a manageable and stable set of Normal, Short, Super-short
* DD - DropDown (Combo)
* LB, LBox - Listbox
* R, Rad - Radio
* ML, MLine - Multiline
* BMenu - ButtonMenu
* PBar, Prog - ProgressBar
* Col - Column
* Listbox - new method GetIndexes returns currently selected items as a list of indexes
* Output - new method Get returns the contents of the output element
* Button - For Macs don't don't allow setting button color. Previously only warned
* ButtonMenu - new Click method will click the button just like a normal Button's Click method
* Column scrolling finally works correctly with mousewheel. Shift+Mouse Scroll will scroll horizontally
* Table - Get method is a dummy version a Get because Qt port got a real Get method
* Table - Will add numerical column headers if Column Headsing is set to None when creating Table Element
* Table - FIXED the columns crazily resizing themselves bug!!
* Table - Can resize individual columns now
* Tree - was not returning Keys but instead the string representation of the key
* SetIcon will set to default base64 icon if there's an error loading icon
* Fix for duplicate key error. Was attempting to add a "unique key counter" onto end of keys if duplicate, but needed to turn into string first
* Columns
* No longer expand nor fill
* Sizing works for both scrolled and normal
* Setting focus - fixed bug when have tabs, columns, frames that have elements that can get the focus. Setting focus on top-level window
* InputText elements will now cause rows to expand due to X direction expansion
* Frame - Trying to set the size but doesn't seem to be setting it correctly
* Tabs will now expand & fill now (I hope this is OK!!!)
## 4.5 PySimpleGUI Release 04-Nov-2019
* Metadata!
* All elements have a NEW metadata parameter that you can set to anything and access with Element.metadata
* Windows can have metadata too
* Window.finalize() - changed internally to do a fully window.read with timeout=1 so that it will complete all initializations correctly
* Removed typing import
* ButtonReboundCallback - Used with tkinter's Widget.bind method. Use this as a "target" for your bind and you'll get the event back via window.read()
* NEW Element methods that will work on a variety of elements:
* set_size - sets width, height. Can set one or both
* get_size - returns width, heigh of Element (underlying Widget), usually in PIXELS
* hide_row - hides the entire row that an element occupies
* unhide_row - makes visible the entire row that an element occupies
* expand - causes element to expand to fill available space in X or Y or both directions
* InputText Element - Update got new parameters: text_color=None, background_color=None, move_cursor_to='end'
* RadioButton - fix in Update. Was causing problems with loading a window from disk
* Text Element - new border width parameter that is used when there's a relief set for the text element
* Output Element - special expand method like the one for all other elements
* Frame element - Can change the text for the frame using Update method
* Slider element - can change range. Previously had to change value to change the range
* Scrollable frame / column - change to how mousewheel scrolls. Was causing all things to scroll when scrolling a single column
* NOTE - may have a bad side effect for scrolling tables with a mouse wheel
* Fix for icon setting when creating window. Wasn't defaulting to correct icon
* Window.get_screen_size() returns the screen width and height. Does not have to be a window that's created already as this is a class method
* Window.GetScreenDimensions - will return size even if the window has been destroyed by using get_screen_size
* Now deleting window read timers every time done with them
* Combo no longer defaults to first entry
* New Material1 and Material2 look and feel color schemes
* change_look_and_feel has new "force" parameter. Set to True to force colors when using a Mac
* Fix in popup_get_files when 0 length of filename
* Fix in Window.SetIcon - properly sets icon using file with Linux now. Was always defaulting
## 4.6 PySimpleGUI 16-Nov-2019
* Themes!!!
* Added a LOT of Look and Feel themes. Total < 100 now
* Doctring comments for some missing functions
* PEP8 bindings for button_rebound_collback, set_tooltip, set_focus
* Spin Element Update - shortened code
* Allow tk.PhotoImage objeft to be passed into Image.update as the data
* DrawRectangle - added line_width parameter. Defaults to 1
* Fix for Slider - was only setting the trough color if the background color was being set_focus
* Added a deiconify call to Window.Normal so it can be used to restore a window that has been minimized. Not working on Linux
* Combo - Fix for not allowing a "0" to be specified as the default
* Table - Saving the Frame that contains a table in the member variable table_frame. This will enable the frame to be changed to expandable in the future so that the table can be resized as a window expands.
* LOTS AND LOTS of Look and Feel themes!!!!
* Added SystemDefaultForReal to look and feel that will prodce 100% not styled windows
* Changed the "gray" strings in look and feel table into RGB strtings (e.g. gray25 = #404040). No all graphics subsystems
* Removed Mac restriction from Look and Feel setting changes. All color settings are changed EXCEPT for the button color now on a Mac
* "Fuzzy Logic" Look and Feel Theme Selection - No longer have to memorize every character and get the case right. Now can get "close enough" and it'll working
* New function - preview_all_look_and_feel_themes. Causes a window to be shown that shows all of the currently available look and feel themes
* Removed use of CloseButton in popup get file, folder, text. Was causing problems where input fields stopped working. See bug on GitHub
## 4.7.0 PySimpleGUI 26-Nov-2019
TTK WIDGETS! Welcome back Mac Users!
* Significant progress on using ttk widgets properly
* Added ttk buttons - MACS can use colored buttons again!! (Big damned deal)
* The existing ttk based Elements are now correctly being colored and styled
* Ability to set the ttk theme for individual windows or system-wide, but no longer on a single Element basis
* Ability to use ttk buttons on a selective basis for non-Mac systems
* port variable == 'PySimpleGUI' so that your code can determine which PySimpleGUI is running
* InputText new parameter - use_readonly_for_dsiable defaults to True enables user to switch between a true disable and readonly setting when disabling
* Rework of progress bar's ttk style name
* Button - new parameter use_ttk_buttons - True = force use, False = force not used, None = let PySimpleGUI determine use
* Macs are forced to use ttk buttons EXCEPT when an image is added to the button
* TabGroup - can no longer set ttk theme directly
* Window new parameters
* ttk_theme - sets the theme for the entire window
* use_ttk_buttons - sets ttk button use policy for the entire window
* More Window layout error checking - checks that rows are iterables (a list). If not, an error popup is shown to help user find error
* Fixed progessbars not getting a key auto assigned to theme
* New Window method - send_to_back (SendToBack) - sends the window to the bottom of stack of all windows
* Fixed normal tk button text - was left justifying instead of centering
* Fixed table colors - wasn't setting correctly due to bad ttk styling
* Fixed tree ccolors - wasn't setting correctly due to bad ttk styling
* TabGroups now function correction with colors including currently selected tab color and background color of non-tab area (next to the tabs)
* New set_options parameters
* use_ttk_buttons - sets system-wide policy for using ttk buttons. Needed for things like popups to work with ttk buttons
* ttk_theme - sets system-wide tth theme
* progress_meter_style parameter no longer used and generates a warning
* list_of_look_and_feel_values now sorts the list prior to returning
* Removed Mac restriction on Button colors from look and feel calls. Now can set button colors to anything!
* popup_scrolled new parameters - all popups need more parameters but these are for sure needed for the scrolled popup
* background_color
* text_color
* no_titlebar
* grab_anywhere
* keep_on_top
* font
* Test harness changes to help test new ttk stuff (want to shrink this window in the future so will fit on Trinket, Pi, etc
## 4.8.0 PySimpleGUI 4-Dec-2019
Multicolored multiline text! Often asked for feature going way back
ttk Buttons can have images
Print in color!
* Multiline Element got 2 new parameters to the update method
* text_color_for_value - color for the newly added text
* background_color_for_value - background color of the newly added text
* New Print/EasyPrint parameters and capability
* text_color, background_color - control the text's color and background color when printing to "Debug Window"
* Must be done only when used in mode where stdout is not re-routed (the default)
* Wouldn't it be really nice if normal print calls had this parameter?
* Print(event, text_color='green', background_color='white', end='')
* ttk Buttons
* can have images. No longer forces Buttons with images to be the old tk Butons. Now you can choose either
* can update the button color
* can update the button image
* Set warning filter so that warnings are repeated
* New global variables:
* CURRENT_LOOK_AND_FEEL - The current look and feel setting in use. Starts out as "Default"
* BROWSE_FILES_DELIMITER - Defaults to ";" It is the string placed between entries returned from a FilesBrowse button
* TRANSPARENT_BUTTON - Depricated - was being used incorrectly as it was a relic from the early days. It's value was a color of gray
* Window - gentle reminder if you don't choose a look and feel for your window. It's easy to stop them. Add a change_look_and_feel line
* Test harness uses a debug window so don't be shocked when 2 windows appear when running PySimpleGUI by itself
* Prints the "Event" in Green on White text
* Prints the "values" normally
## 4.9.0 PySimpleGUI 7-Dec-2019
The "Finally Nailed Tabs" release
* Colors for Tabs!
* When creating TabGroup can now specify
* Text & Background color of all tabs
* Text & Background color of selected tab
* If nothing is specified then the Look and Feel theme will be used (which turned out GREAT)
* Tab visibility - Can finally control individual tab's visibility using update and when creating
* More "Look and Feel" Themes! There's no excuse to be grey again. There are now 126 themes to choose from. Here are the 32 new themes"
DefaultNoMoreNagging
DarkBlack1
DarkBlue12
DarkBlue13
DarkBlue14
DarkBlue15
DarkBlue16
DarkBlue17
DarkBrown5
DarkBrown6
DarkGreen2
DarkGreen3
DarkGreen4
DarkGreen5
DarkGreen6
DarkGrey4
DarkGrey5
DarkGrey6
DarkGrey7
DarkPurple6
DarkRed2
DarkTeal10
DarkTeal11
DarkTeal12
DarkTeal9
LightBlue6
LightBlue7
LightBrown12
LightBrown13
LightGray1
LightGreen10
LightGreen9
LightGrey6
* preview_all_look_and_feel_themes now has a columns parameter to control number of entries per rows
* also made each theme display smaller due to large number of themes
## 4.10.0 PySimpleGUI 9-Dec-2019
"Oh crap the debugger is broken!" + "Pretty Progress Bars" release
* Fix for built-in debugger not working
* Important due to upcoming educational usage
* Has been broken since 4.5.0 when a change to Finalize was made
* ProgessBar element colors set using Look and Feel colors
* Combination of button color, input element, and input element text are used
## 4.11.0 PySimpleGUI 10-Dec-2019
The Element & Window bindings release
* Element.bind - New method of all Elements
* Enables tkinter bindings to be added to any element
* Will get an event returned from window.read() if the tkinter event happens
* Window.bind - New method for Windows, just like Elements
* Enables tkinter bindings to be added to Windows
* Will get an event returned from window.read() if the tkinter event happens
* TabGroup fonts - can now set the font and font size for Tab text
## 4.12.0 PySimpleGUI 14-Dec-2019
Finally no more outlines around TK Elements on Linux
* Fixed a long-term problem of the mysterious white border around (almost) all TK Elements on Linux
* Ability to set the disabled button colors
* New Button and Button.update parameter - disabled_button_color
* Specified as (Text Color, Background Color) just like button colors
* For Normal / TK Buttons - can set button text color only
* For TTK Buttons - can set both a disabled button and text color
* Either parameter can be None to use current setting
* Removed use of CloseButton from Popups (still have a bug in the CloseButton code but not in popups now)
* Combobox - removed requirement of setting disabled if want to set to readonly using update method
* Fix for cancelling out of file/folder browse on Linux caused target to be cleared instead of just cancelling
* Removed try block around setting button colors - if user sets a bad color, well don't do that
* Now deleting windows after closing them for popup
## 4.13.0 PySimpleGUI 18-Dec-2019
Table and Tree header colors, expanded Graph methods
* Element.expand new parameter - expand_row. If true then row will expand along with the widgets. Provides better resizing control
* Using math.floor now instead of an int cast in Graph Element's unit conversions
* Graph.draw_point - now using caller's graph units for specifying point size
* Graph.draw_circle - converted radius size from user's graph units.
* Graph.draw_circle - added line_width parameter
* Graph.draw_oval - added line_width parameter
* Graph.get_figures_at_location - new method for getting a list of figures at a particular point
* Graph.get_bounding_box - returns bounding box for a previously drawn figure
* Table and Tree Elements
* 3 new element creation parameters
* header_text_color - color of the text for the column headings
* header_background_color - color of the background of column headings
* header_font - font family, style , size for the column headings
* Defaults to using the current look and feel setting
* Uses similar algorithm as Tabs - Input Text background and text colors are used
* Spin element - fixed bug that showed "None" if default value is "None"
* Test Harness sets incorrect look and feel on purpose so a random one is chosen
## 4.14.0 PySimpleGUI 23-Dec-2019
THEMES!
* theme is replacing change_look_and_feel. The old calls will still be usable however
* LOTS of new theme functions. Search for "theme_" to find them in this documentation. There's a section discussing themes too
* "Dark Blue 3" is the default theme now. All windows will be colored using this theme unless the user sets another one
* Removed the code that forced Macs to use gray
* New element.set_cursor - can now set a cursor for any of the elements. Pass in a cursor string and cursor will appear when mouse over widget
* Combo - enable setting to any value, not just those in the list
* Combo - changed how initial value is set
* Can change the font on tabs by setting font parameter in TabGroup
* Table heading font now defaults correctly
* Tree heading font now defaults correctly
* Renamed DebugWin to _DebugWin to discourage use
## 4.15.0 PySimpleGUI 08-Jan-2020
Dynamic Window Layouts! Extend your layouts with `Window.extend_layout`
Lots of fixes
* Window.extend_layout
* Graph.change_coordinates - realtime change of coordinate systems for the Graph element
* theme_text_element_background_color - new function to expose this setting
* Radio & Checkbox colors changed to be ligher/darker than background
* Progress bar - allow updates of value > max value
* Output element does deletes now so that cleanup works. Can use in multiple windows as a result
* DrawArc (draw_arc) - New width / line width parameter
* RGB does more error checking, converts types
* More descriptive errors for find element
* popup_error used interally now sets keep on top
* Element Re-use wording changed so that it's clear the element is the problem not the layout when re-use detected
* Window.Close (Window.close) - fix for not immediately seeing the window disappear on Linux when clicking "X"
* Window.BringToFront (bring_to_front) - on Windows needed to use topmost to bring window to front insteade of lift
* Multiline Scrollbar - removed the scrollbar color. It doesn't work on Windows so keeping consistent
* Changed how Debug Windows are created. Uses finalize now instead of the read non-blocking
* Fix for Debug Window being closed by X causing main window to also close
* Changed all "black" and "white" in the Look and Feel table to #000000 and #FFFFFF
* Added new color processing functions for internal use (hsl and hsv related)
* popup - extended the automatic wrapping capabilities to messages containing \n
* Test harness uses a nicer colors for event, value print outs.
* _timeit decorator for timing functions
## 4.15.1 PySimpleGUI 09-Jan-2020
Quick patch to remove change to popup
## 4.15.2 PySimpleGUI 15-Jan-2020
Quick patch to remove f-string for 3.5 compat.
## 4.16.0 PySimpleGUI 20-Feb-2020
The "LONG time coming" release. System Tray, Read with close + loads more changes
Note - there is a known problem with the built-in debugger created when the new read with close was added
* System Tray - Simulates the System Tray feature currently in PySimpleGUIWx and PySimpleGUIQt. All calls are the same. The icon is located just above the system tray (bottom right corner)
* Window.read - NEW close parameter will close the window for you after the read completes. Can make a single line window using this
* Window.element_list - Returns a list of all elements in the window
* Element.unbind - can remove a previously created binding
* Element.set_size - retries using "length" if "height" setting fails
* Listbox.update - select_mode parameter added
* Listbox.update - no longer selects the first entry when all values are changed
* Listbox.get - new. use to get the current values. Will be the same as the read return dictionary
* Checkbox.update - added ability to change background and text colors. Uses the same combuted checkbox background color (may remove)
* Multiline.update - fix for when no value is specified
* Multiline - Check for None when creating. Ignores None rather than converting to string
* Text.update - added changing value to string. Assumed caller was passing in string previously.
* Text Element - justification can be specified with a single character. l=left, r=right, c=center. Previously had to fully spell out
* Input Element - justification can be specified with a single character. l=left, r=right, c=center. Previously had to fully spell out
* StatusBar Element - justification can be specified with a single character. l=left, r=right, c=center. Previously had to fully spell out
* Image Element - can specify no image when creating. Previously gave a warning and required filename = '' to indicate nothing set
* Table Element - justification can be specified as an "l" or "r" instead of full word left/right
* Tree Element - justification can be specified as an "l" or "r" instead of full word left/right
* Graph.draw_point - changed to using 1/2 the size rather than size. Set width = 0 so no outline will be drawn
* Graph.draw_polygon - new drawing method! Can now draw polygons that fill
* Layout error checking and reporting added for - Frame, Tab, TabGroup, Column, Window
* Menu - Ability to set the font for the menu items
* Debug window - fix for problem closing using the "Quit" button
* print_to_element - print-like call that can be used to output to a Multiline element as if it is an Output element
## 4.17.0 PySimpleGUI 24-Mar-2020
The "it's been a minute" release
Improved DocStrings and documentation!
Upgrade utility
"Printing" directly to Multiline
* New upgrade utility to upgrade your installed package using GitHub version
* Can invoke from command line. Run `python -m PySimpleGUI.PySimpleGUI upgrade`
* The test harness GUI has an upgrade button
* Multiline.print - Add multiline element to the front of any print statement. Also supports color output
* Debug informmation like path and version displayed in test harness GUI
* Added back the TRANSPARENT_BUTTON variable until can find a better way to deprecate
* All elements were losing padding when made invisible. Fixed
* Image.update - fixed crash due to not checking for type before getting size
* Image.update_animation_no_buffering - playback GIF animations of any length
* Graph element - Fixed divide by zero error in convert function
* TabGroup will now autonumber keys if none specified
* Measuring strings more accurately during layout
* Using specific font for measurement
* Used to compute TTK button height
* Used to compute Slider length
* Used to compute header widths in Tables, Trees
* Used to compute column widths in Tables, Trees
* Used to compute row heights in Tables
* Removed padx from row frames. Was using window's margins. Now padx & pady = 0. Was causing too every Column element to have extra padding
* Added no_titlebar to one line progress meter
* popup_notify - Creates a "notification window" that is like the System Tray Message window
* shell_with_animation - launch a shell command that runs while an animated GIF is shown
* Fixed problem with debugger not working after the recent close parameter addition to Window.read
## 4.18.0 PySimpleGUI 26-Mar-2020
An "Oh F**k" Release - Table column sizes were bad
* Fixed bug in Table Element's column size computation
* popup_animated has new title parameter
* Checkbox - update can change the text
## 4.18.1 PySimpleGUI 12-Apr-2020
Emergency patch - f-string managed to get into the code resulting crashes on 3.5 systems (Pi's for example)
## 4.18.2 PySimpleGUI 12-Apr-2020
The Epic Fail release.... import error on 3.5 for subprocess.
## 4.19.0 PySimpleGUI 5-May-2020
New Date Chooser
Scrollable columns with mousewheel!! (oh please work right!)
WINDOW_CLOSE & WIN_CLOSE events
Long list of stuff!
* Imported from typing again to get correct docstrings
* Print and MLine.Print fixed sep char handling
* New parameter to Muliline.print(autoscroll parameter)
* New autoscroll parameter added to _print_to_element
* popup_get_date
* Complete reworking on Calendar Chooser Button
* Has a LOT more paramteter
* Can set location!
* icon parm popup_animated
* popup button size (6,1) for all popups
* NEW CALENDAR chooser integrated
* Graph.draw_lines - new method to allow for multiline lines that may not be a full polygon
* System Tray fixed the docstrings
* color chooser set parent window (needed for icon?)
* scrollable column scrollwheel fixed
* fixed TabGroup border width (wasn't getting set properly at all)
* EXPERIMENTAL Scrollable Columns
* Fixed Debug Printing to work like a normal "print"
* Fixed _print_to_element to work like a normal "print"
* Fixed light green 1 theme definition - Text color wasn't being set
* fix for install from GitHub
* fix for Column scrolling with comboboxes
* Added Text.get
* Spin.update fix
* import typing again
* fixes for Pi
* test for valid ttk_theme names
* fix for Text.get docstring
* added tuples to some docstrings
* added code for better tag handling for Multiline elements (fixes a potential memory leak... thanks Ruud)
* WIN_CLOSE & WINDOW_CLOSED constants added. Both are None
* EVENT_TIMEOUT and TIMEOUT_EVENT constants added to both be the same as TIMEOUT_KEY
* Some changes in test harness that tested recent changes (may still need shortening for trinket or others)
* Changed the misleading TRANSPARENT_BUTTON constant with an attempt using themes calls
## 4.20.0 PySimpleGUI 6-Jun-2020
Fixes and new features... broad range
* Fix for Typing import for Pi (3.4) users. Now "tries" to import typing
* Tooltip fonts - can change the font for tooltips
* Fixed tearoff for Menus. Had stoppped working
* Radio - If element is updated to False, the entire group of radio buttons will be set to false tooltips
* Multiline - fix for colors. Only set tags for output that has specific colors
* Multiline - keeping track of disabled with Disabled mumber variable
* Progress bar
* Added class variable "uniqueness counter" so that every bar will have its own settings
* Needed in case the same key is used in another window
* Fix for stdout being reset if someone sets flush on their call to print
* Mac special case added to tkfiledialog.askdirectory just like on askopenfilename
* Tab - can "update" the title
* Menu update - was not applying font when updating the menu
* Window.set_title - allows you to change the title for a window
* Added searching through Panes when looking for element with focus
* Removed Python 2 Add Menu Item code
* Added font to buttonmenu.
* Added font to the combobox drop-down list (wow what a pain)
* Table now uses the element's padding rather than 0,0
* Tree now uses the element's padding rather than 0,0
* set_options - added ability to set the tooltip font
* Fixed a couple of docstrings
* Reworked main() test harness to display DETAILED tkinter info and use better colors
## 4.21.0 PySimpleGUI 27-Jun-2020
Horizontal Separator, cprint, docstrings
* New color printing function cprint - enables easy color printing to an element
* Tons of docstring fixups (300+ changes)
* Removed old Python2 checks
* Added Element.set_vscroll_position - scroll to a particular % of the way into a scrollable widget
* Input Text - new parameters
* border_width
* read_only (for tkinter will have to be disabled OR readonly. Cannot be both)
* disabled_readonly_background_color
* disabled_readonly_text_color
* Radio - Backed out the change that cleared all buttons in group because already have that ability using reset_group
* Graph drag mouse up events returned as either a string + "+UP" (as before) or as a tuple with "+UP" being added onto a tuple key
* Vertical separator - added key and color - color defaults to text color
* Horizontal separator! (FINALLY). Color defaults to text color
* Fix for Table and Tree elements not automatically getting a key generated if one wasn't supplied
* Made key parameter for one_line_progress_meter have a default value so don't have to specify it when you have only 1 running
* theme_add_new - adds a new theme entry given a theme name and a dictionary entry. This way you don't have to directly modify the theme dictionary
* Added initial_folder to popup_get_folder when there is no window
* Added default_path to popup_get_file when there is no window
* Fix for removing too many PySimpleGUI installs when using the GitHub upgrade tooltip
## 4.22.0 PySimpleGUI 28-Jun-2020
More cprint stuff
* Additional window and key parameter to cprint
* May seem like a small change, but the results are powerful
* Can now easily "print" to anywhere, in color!
## 4.23.0 PySimpleGUI 3-Jul-2020
Table Colors Fix - workaround for problems with tables and tree colors in Python 3.7.2 to 3.9+
Mac crash fixed - tkinter.TclError: expected boolean value but got "" (hopefully)
New shortcut "k" parameter for all elements that is the same as "key" - may be experimental / temporary if not well received
More error checks
popup extensions
* Fix for missing Table and Tree colors created in tk 8.6.9
* This is a problem in all versions of Python 3.7.2 - 3.9.0 with no target fix date published
* As a result of no fixes in sight, added a fix in PySimpleGUI if the tk version is 8.6.9
* New Element creation parameter "k" - exact same thing as "key" but shorter. Helps with complex layouts
* New error reporting on all element.update calls - checks to see if element has been fully created
* set_options - new option to supress popup_errors coming from PySimpleGUI.py
* Mac specific crash fix - if on a Mac, no longer calling wm_overrideredirect as it crashes the Mac now
* Additional error checking (shows error instead of asserting:
* Check for Widget creation before widget operations like bind, unbind, expand
* Check for window finalize / read before some window operations like maximize, hide, etc
* docstrings - more added. Fixed up a number of missing / erroneous ones
* Tree element - caches images so that will not create new ones if previously used on another Tree item
* popup - two new options
* any_key_closes - bool. If True, any key pressed will close the window
* image - can be a bytes (base64) or string (filename). Image will be shown at top of the popup
* all popups - new image parameter (base64 or string)
* a few new built-in icons
## 4.24.0 PySimpleGUI 3-Jul-2020
Selective control over tk 8.6.9 treeview color patch
* Disabled the code that patched the problem with background colors for Tree and Table elements
* Can enable the patched code by calling set_options
* To enable set parameter enable_treeview_869_patch = True (defaults to false)
## 4.25.0 PySimpleGUI 17-Jul-2020
Biggest / most impactful set of changes in a while (fingers crossed)
Modal windows
Multithreaded Window.write_event_value method
stdout re-route to any Multiline
table/tree highlights
k element parameter
* New "k" parameter for all elements.
* Same as "key"
* Created so layouts can be even more compact if desired
* New docstring for keys (basically anything except a list)
* Popups
* New text wrap behavior. Will wrap text between \n in user's string
* All popups are now "modal" unless they are non-blocking (can be turned off using new parameter)
* New button color and table/tree highlight color format
* Colors can still be tuple (text, background)
* Can also be a single string with format "text on background" (e.g. "white on red")
* Multiline
* Automatically refresh window when updating multiline or output elements
* For cprint use Multiline's autoscroll setting
* New autoscroll parameter in Multiline.print
* New parameters to make the print and cprint stuff much easier
* write_only=False (so that it's not returned when read)
* auto_refresh=False
* reroute_stdout=False
* reroute_stderr=False
* reroute_cprint=False (removes need to call the cprint cprint_set_output_destination function)
* Table / Tree Elements
* Re-enabled the tk 8.6.9 background color fix again
* selected_row_colors=(None, None) - tuple or string
* Automatically sets the selected row color based on the theme colors! (uses the button color)
* Can use 2 other constants for colors
* OLD_TABLE_TREE_SELECTED_ROW_COLORS - ('#FFFFFF', '#4A6984') the old blueish color
* ALTERNATE_TABLE_AND_TREE_SELECTED_ROW_COLORS - (SystemHighlightText, SystemHighlight)
* Tree image caching happens at the element level now
* Window
* make_modal - new method to turn a window into a modal window
* modal parameter when window is created. Default is False
* write_event_value - new method that can be called by threads! This will "queue" an event and a value for the next window.read()
* Display an error popup if read a closed window 100+ times (stops you from eating 100% of the CPU time)
* was_closed method added - returns True if a window has been closed
* Combo - don't select first entry if updated with a new set of values
* Tooltip - fix for stuck-on tooltips
* New theme_previewer with scrollbars. 3 new parameters
* cprint - now has all parameters shown in docstring versus using *args **kwargs
* New global variable __tclversion_detailed__ - string with full tkinter version (3 numbers instead of 2)
* Warning is displayed if tcl version is found to be 8.5.
## 4.26.0 PySimpleGUI 18-Jul-2020
* Multi-threaded tkvar initialization location changed so that thread doesn't intialize it now
* Removed thread key - no longer needed
* Window.write_event_values - now requires both parms
* Upgrade button typo
## 4.27.4 PySimpleGUI 3-Aug-2020
Multi-window support done right!
New capabilities for printing, Multiline
Main app additions
Theme searching
* read_all_windows - function that reads all currently open windows.
* Finally the efficient multi-window solution
* No longer need to do round-robin type scheduling
* Easily convert existing programs from single to multi-windows
* Demo programs with multi-window design patterns all updated
* Ideal for "floating palette / toolbar" window adds-ons
* Can read with timeout including timeout=0
* theme_previewer
* search option
* button in main app
* reset to previous theme following preview
* Sponsor button in main app
* Theme previewer in main app
* Progress bar
* colors can use the single string "foreground on background" color format
* update_bar combined with update for a single update interface
* Better element key error handling
* 3 options to control how lookup errors are handled
* popup now shows
* file, function, line #, actual line of code with error
* erroneous key provided
* best matching key
* will automatically try to continue with best matching key
* can assert with key error if desired (true by default)
* fix for get item
* Up/down arrow bindings for spinner if enabling events
* Multiline
* new justification parameter on creation and update
* print - justification parameter added
* cprint - justification parameter added - note tricky to set color of single word but possible
* Added mousewheel for Linux return_keyboard_events enabled
* Added get_globals function for extending easier
* Refactored callbacks
* Image element - can clear image by not setting any parameters when calling update
* Column Element's Widget member variable now being set
* Window's starting window location saved
* Early experimental "Move all windows in sync" when using grab_anywhere (coming soon)
* Fix for 3.4 (can't use f-strings)
## 4.28.0 PySimpleGUI 3-Aug-2020
Element pinning for invisibility!
* Better visible/invisible handling
* pin - new function to place an element in a layout that will hold its position
* border_width added to Canvas and Graph (so that they will default to 0)
* Combobox
* button color will match theme's button color
* background color set correctly when readonly indicated
* Spin element
* spin button color set to background color of spinner
* spin arrow color automatically set to text color
* Bad element key popup - fix for displaying correct line info in some situations
## 4.29.0 PySimpleGUI 25-Aug-2020
Custom titlebar capabilities (several new features required)
Better Alignment
Calendar button works again
* Window.visiblity_changed now refreshes the window
* Added Column.contents_changed which will update the scrollbar so corrently match the contents
* Separators expand only in 1 direction now
* Added 8 SYMBOLS:
SYMBOL_SQUARE = '█'
SYMBOL_CIRCLE = '⚫'
SYMBOL_CIRCLE_OUTLINE = '◯'
SYMBOL_UP = '▲'
SYMBOL_RIGHT = '►'
SYMBOL_LEFT = '◄'
SYMBOL_DOWN = '▼'
SYMBOL_X = '❎'
* New dark themes - dark grey 8, dark grey 9, dark green 9, dark purple 7
* When closing window no longer deletes the tkroot variable and rows but instead set to None
* Changd no-titlebar code to use try/except. Previously removed for Mac due to tk 8.6.10 errors calling wm_overrideredirect
* Fix for Column/window element justification
* New vertical_alignment parm for Column, Frame, pin
* New layout helper functions - vtop/vcenter/vbottom - Can pass an element or a row of elements
* Fixed statusbar expansion
* Added disabled button to theme previewer
* Fixed grab anywhere stop motion bug - was setting position to None and causing error changed to event.x
* Expanded main to include popup tests, theme tests, ability to hide tabs
* Grab parameter for Text Element, Column Element
* Added tclversion_detailed to get the detailed tkinter version
* All themes changed the progress bar definition that had a "DEFAULT" indicator. New constant DEFAULT_PROGRESS_BAR_COMPUTE indicates the other theme colors should be used to create the progess bar colors.
* Added expand_x and expand_y parameters to Columns
* Fix for Calendar Button. Still needs to be fixed for read_all_windows
* Force focus when no-titlebar window. Needed for Raspberry Pi
* Added Window.force_focus
* No longer closes the hidden master window. Closing it caused a memory leak within tkinter
* Disable close on one_line_progress_meter. There is a cancel button that will close the window
* Changed back toplevel to no parent - was causing problems with timeout=0 windows
## 4.30.0 PySimpleGUI 14-Oct-2020
User Settings APIs, lots more themes, theme swatch previewer, test harness additions
* Added shrink parameter to pin,
* added variable Window.maximized,
* added main_sdk_help_window function,
* New themes - DarkGrey10,DarkGrey11 DarkGrey12 DarkGrey13 DarkGrey14, Python, DarkBrown7
* Highlight Thickness for Button, Radio, Input elements
* Set to 1 now instead of 0 so that focus can be seen
* Color is automatically set for buttons, checkboxes, radio buttons
* Color can be manually set for Buttons using `highlight_colors` parameter
* Only used by Linux
* user_settings APIs
* Whole new set of API calls for handling "user settings"
* Settings are saved to json file
* For more info, see the documentation
* Radio.update - added text, background & text colors parameters
* Multiline & Output Elements:
* added parameter echo_stdout_stderr
* if True then stdout & stderr will go to the console AND to the Multiline
* "ver" is shortened version string
* modal docstring fix in some popups
* image parameter implemented in popup_scrolled
* Graph.draw_image - removed color, font, angle parameters
* fixed blank entry with main program's theme previewer
* added Window.set_min_size
* error message function for soft errors
* focus indicator for Button Checkbox Radio using highlights
* added main_sdk_help Window
* added theme_previewer_swatches function
* added "Buy Me A Coffee" button
* updated `pin` layout helper function - added `shrink` parameter
* Main debugger window set to keep on top
## 4.31.0 PySimpleGUI 13-Nov-2020
User Settings class, write_event_value fixes, Menus get colors, Mac no_titlebar patch
* InputText element - Now treating None as '' for default
* Combo - handling update calls with both disabled and readonly set
* Spin - readonly
* Added parameter added when creating
* Added parameter to update
* Spin.get() now returns value rather than string version of value
* Multiline print now autoscrolls by default
* FileSaveAs and SaveAs now has default_extension parameter like the popup_get_file has
* Button Menu - Color and font changes
* New create parameters - background color, text color, disabled text color, item font
* Fixed problem with button always being flat
* Menu (Menubar) - Color changes
* New create paramters - text color, disabled text color.
* Hooked up background color parameter that was already there but not functional
* write_event_value - fixed race conditions
* Window.read() and read_all_windows() now checks the thread queue for events before starting tkinter's mainloop in case events are queued
* Window.set_cursor added so that window's cursor can be set just like can be set for individual elements
* Icon is now set when no_window option used on popup_get_file or popup_get_folder
* Reformatted the theme definitions to save a LOT of lines of code
* UserSettings class
* Added a class interface for User Settings
* Can still use the function interface if desired
* One advantage of class is that [ ] can be used to get and set entries
* Looks and acts much like a "persistent global dictionary"
* The User Settings function interfaces now use the class
* main_get_debug_data()
* Function added that will display a popup and add to the clipboard data needed for GitHub Issues
* Added button to Test Harness to display the popup with version data
* Mac - Added parm enable_mac_notitlebar_patch to set_options to enable apply a "patch" if the Window has no_titlebar set.
## 4.32.0 PySimpleGUI 17-Nov-2020
Menu colors and font, fixes
* Menu, ButtonMenu, and right click menu now default to theme colors and Window font
* The background color for menus is the InputText background color
* The text color for menus is the InputText text color
* The font defaults to the Window font
* These theme colors have worked well in the past as they are the settings used for Table and Tree headers
* All settings can be changed
* Added ability to set the right click menu colors and font
* New parameters added to Window to control right click look
* Fixed problem with Button.update.
* Was crashing if button color changed to COLOR_SYSTEM_DEFAULT
* Fixed problem with right click menus introduced in the previous release
* Auto-close windows can now be finalized (previously could not do this)
* Window.read with timeout is faster
## 4.32.1 PySimpleGUI 17-Nov-2020
* Bug in finalize code
## 4.33.0 PySimpleGUI 2-Jan-2021 (Happy New Year!)
The let's kickoff 2021 release!
Custom Titlebars, Fix for Docstrings so PyCharm 2020 works correctly, New shortcuts, Window Close Attempted
* Custom Titlebar - new element
* Initial reason was Trinket, but great feature overall
* Allows windows to be styled to match the colors of the window
* Automatically uses theme for colors
* Automatically used when running on Trinket
* Can specify using them by using set_options, a Titlebar element, or parameters in Window creation
* Documentation is coming soonish
* Demo exists showing how to use (it's enough that you won't need a Cookbook / detailed docs to add it to your own program)
* Changes include adding a 16x16 pixel version of the PySimpleGUI icon
* popups - If custom titlebar is set using set_options (and is thus globally applied) then popups will use the custom titlebar
* MASSIVE number of changes to docstrings so that PyCharm again works correctly. All Unions had to be changed to use "|" instead
* Internal functions added to check what OS is being used rather than having os.platform checks all over thee place
* Element.Visible removed. Element.visible property returns current visibility state for element
* This is a read-only property
* Added dummy Element.update method so that PyCharm doesn't complain about a missing method
* InputElement class changed to Input. Both will work as InputElement is now an alias
* Fix in Spin.update. Was using an illegal state value of "enable" rather than "normal"
* New Shortcuts for Elements
* Sp = Spin
* SBar = StatusBar
* BM = ButtonMenu
* Progress = ProgressBar
* Im = Image
* G = Graph
* Fr = Frame
* Sl = Slider
* Button - Allow button color background to be specified as None. This will cause the theme's color to be auto chosen as background
* Image.DrawArc - fill_color parameter added
* Column - update now uses the expand information so that a column will re-pack correctly when made invisible to visible. Added fill parm to pack call
* New Window parameters:
* enable_close_attempted_event=False
* titlebar_background_color=None
* titlebar_text_color=None
* titlebar_font=None
* titlebar_icon=None
* use_custom_titlebar=None
* Removed "Faking Timeout" print because the state that triggered it can be reached using a normal auto-closing window
* Can now intercept window close attempts when the user clicks X
* If you don't want "X" to close the window, set enable_close_attempted_event to True when creating window
* When enabled a WINDOW_CLOSE_ATTEMPTED_EVENT will be returned from read instead of WIN_CLOSED
* Multiple aliases for the key: WINDOW_CLOSE_ATTEMPTED_EVENT, WIN_X_EVENT, WIN_CLOSE_ATTEMPTED_EVENT
* New Window property - key_dict
* Returns the "All keys dictionary" which has keys and elements for all elements that have keys specified
* pin helper function got 2 new parameters - expand_x=None, expand_y=None. Was needed for the Titlebar element
* no_titlebar implementation changed on Linux. Not using "splash" and instead using "dock". Was needed to get the minimize/restore to work
* New set_options parameters in support of custom Titlebar element
* use_custom_titlebar=None
* titlebar_background_color=None
* titlebar_text_color=None
* titlebar_font=None
* titlebar_icon=None
* get_globals removed - not required for normal PySimpleGUI. Was only used by patched packages which will need to fork PySimpleGUI for this feature.
* popup - support for custom titlebar!
* Changed from pathlib to os.path
## 4.34.0 PySimpleGUI 18-Jan-2021
Fix popup_scrolled, big swap of PEP8 names from alias to def statements
* Quick "Emergency" release since popup_scrolled crashes. BAD bad thing that has to be corrected ASAP
* Changed all of the functions and methods so that the definition is PEP8 compliant and and alias is not compliant
* Built-in SDK help
* Added a "Summary mode"
* Make window smaller to fit on more monitors
* Added aliases to end of help for each element
* metadata
* Changed into a class Property so that it shows up in the docs correctly
* The Element, Window and SystemTray classes all got this same change
* Added all elements to the docstring for window[key] style lookups to make PyCharm happier
* Moved all PEP8 function aliases to a centralized spot at the end of the code
* sdk_help alias of main_sdk_help
* Several new demos including a demo browser
## 4.35.0 PySimpleGUI 3-Mar-2021
Emojis, Global settings, Exec APIs
* Emojis! Help has arrived!
* Official PySimpleGUI emojis now usable for your applications
* Used in the error messages
* Has the PSG super-hero logo on his/her chest
* Number 1 PySimpleGUI Goal remains the same.... FUN!
* EMOJI_BASE64_LIST is the list of all emojis. These are formed from the EMOJI_BASE64_SAD_LIST and EMOJI_BASE64_HAPPY_LIST
* "Take me to error"
* It's been close to 2 years in the making, but finally it's here.
* Suppress error popups are
* Mac loses Modal windows setting
* Another Mac feature turned off. The modal setting is now ignored for the Mac. Will turn back on if fixed in tkinter.
* Built-in SDK Help
* Expanded to include init and update parms summary
* Function search capability
* Mode to filter out non-PEP8 compliant functions
* Function search
* Link to external live documentation at bottom
* Sorted list now
* Summary checkbox immediately updates window when changed
* Global Settings & Global Settings Window
* Can set defaults that all programs using PySimpleGUI package will use
* sg.main() has a button "Global Settings"
* Directly access the settings window by calling sg.main_global_pysimplegui_settings()
* Main settings include:
* Default theme
* Editor to use
* Specification of how to launch your editor to editor a specific file at a specific line #
* Python interpreter to use when calling `execute_py_file()`
* Theme (see themes section too)
* User Settings
* Option added to set the default user settings path
* user_settings_path: default path for user_settings API calls. Expanded with os.path.expanduser so can contain ~ to represent user
* pysimplegui_settings_path: default path for the global PySimpleGUI user_settings
* pysimplegui_settings_filename: default filename for the global PySimpleGUI user_settings
* The initial values can be found with constants: DEFAULT_USER_SETTINGS_
* Buttons
* Button color string more robust, less crashes due to bad user formatting
* If a single color specified, then only the button background will be set/modified
* "Disabled means ignore"
* The parameter "disabled" is a tertiary now instead of bool
* disabled True/False still works as it always has
* If disabled parameter is set to the value BUTTON_DISABLED_MEANS_IGNORE, then the button will stop returning events
* Enables you to create your own disabled button colors / behavior. Especially important with buttons with images
* There is a new toggle button demo that shows how to use this feature
* TRANSPARENT_BUTTON is being updated now when the theme changes. It's not recommended for use, but just in case, it's being updated.
* files_delimiter parameter added to BrowseFiles
* Themes
* Spaces can now be used in the theme names
* themes_global() - Gets and sets the theme globally
* Easy and dangerous all in 1 call
* Can also change this setting using the global settings window via sg.main() or main_global_pysimplegui_settings()
* Swatch previewer copies colors onto clipboard correctly now
* Exec APIs - A new set of APIs for executing subprocesses
* execute_command_subprocess
* execute_py_file
* execute_editor
* execute_file_explorer
* execute_get_results
* Debug button color fixed
* popup_get_file
* fixed files_delimiter not being passed correctly to button
* files_delimiter parameter added
* Column - auto expands entire ROW if y-expand is set to True
* popups
* fixed problem when using custom buttons
* Print - easy_print or Debug Print
* Addition of color / c parameter
* erase_all parameter added
* 100% use of Multiline element. Output Element no longer used for Print
* Auto refreshes the multiline
* Window.close needed a tkroot.update() added in order to close these windows
* Graph
* Update coordinate when user bound event happens
* Update coordinate when right click menu item elected
* Checkbox
* Box color parameter added. Normally computed. Now directly accessable to users
* Radio buttons
* Circle color parameter added. Like Checkbox, this is a computed value but is now accessable
* Slider
* Trough color is now settable on a per-element basis
* Input
* update method now includes ability to change the password character
* Listbox
* update values converts items to list
* OptionMenu
* Does not set a default if none was specified
* Use correct font and colors for the list
* Added size parm to update
* Combo
* Ausizes 1 additional character wide to account for arrow
* fixed update bug when default was previously specified
* Added size parm to update
* Element.set_cursor
* now has a color parameter to set the color of the BEAM for input and other elements like them
## 4.36.0 PySimpleGUI 14-Mar-2021
Happy Pi Day!
Exec APIs 1.1, some others fixes too
* Exec APIs
* Fixed the Popen problems found in 3.8+
* Add quotes on all platforms now, not just Windows
* Added checks for COLOR_SYSTEM_DEFAULT to a number of the element .update mehtods
* Changed GreenTan theme to use black
* Fix for button update when cubsample used
* Changed image update anumiation to start & stop at correct frame
* Added return values for popup_animated
* Themes - gray or grey can be used to select the gray themes. Spelling doesn't matter now
* New scrollbar parm for Multiline Element - will use a Text Widget now if scrollbar is False
* New Text element class methods for measuring size of characters
* Debugger theme changed and red button removed
## 4.37.0 PySimpleGUI 15-Mar-2021
Happy "Pi with significant rounding error day"!
I'll eventually figure out this subprocess thing... honest...
* Exec APIs
* More control needed over routing of STDOUT
* Additional parm added pipe_output to execute_command_subprocess
* execute_get_results has a timeout parm now
* execute_subprocess_still_running added to check if a subprocess is still running
* Exposed the "running" functions so they can be used by Demos
* Used internally to see if running on Windows, Linux, Mac, Trinket
* Makes it one less import and the code already existed. All that needed to happen is the _ removed from the front of function name
## 4.38.0 PySimpleGUI 21-Mar-2021
The "so much for no new releases for a while" release
* Changed name of the NEW parm in Multiline element from scrollbar to no_scrollbar
* This matches the other elements that also have this same parameter (Listbox)
* Wanted to get this release posted prior to users writing code that uses it (it's only been 1 week)
* This is the actual purpose for the release... so that it doesn't linger to the point it breaks being backwards compatible
* Some additional debugger stuff... nothing to see here... keep moving.... will let you know when there's more
* Added icon parameter to popup_scrolled
* New Exec API call - execute_find_callers_filename
* It basically looks backwards until PySimpleGUI isn't found
* Hopefully will help in error messages to determine who is calling PySimpleGUI
* Made a constant variable for the & char used by Menus in PySimpleGUI for shortcuts
* Also fixed a couple of places where they were being erroneously stripped from the normal menu text
* Better error reporting for duplicatea keys
* Found a problem with using print for errors - rerouted stdout/stderr can cause MORE errors
* Interestingly, popups work great for these errors as they do not have a cascading error effect
## 4.39.0 PySimpleGUI 11-Apr-2021
Window.write_event_value is solid release!
The s parm debut (alias for size... works like k does for key)
GitHub Issues GUI
* write_event_value fixed(THANK YOU daemon2021!)
* GitHub issue GUI added - access via sg.main() or sg.main_open_github_issue()
* s parm added to all elements
* Element.block_focus - blocks an element from getting focus when using keyboard
* Listbox
* Set the selected colors to be opposite of normal text/background colors
* Added highlight parms to Listbox so that they can be directly set
* The expand method now works for Listbox element
* Button on Mac can be tk Buttons
* In the past the Mac could only use ttk buttons
* Now setting use_ttk=False will cause the tk buttons to be used
* Right Click Menu
* Window get new parameter right_click_menu_tearoff to enable tearoff feature for right click menus
* Buttons and ButtonMenus can now use right click menus. Will automatically use the Window's right click menu
* New constants
* MENU_RIGHT_CLICK_EDITME_EXIT = ['_', ['Edit Me', 'Exit']] - a common menu for simple programs
* MENU_RIGHT_CLICK_DISABLED = [[]] to block an element from getting a window's right click menu
* parameter right_click_entry_selected_colors added to Window - a simple dual color string or a tuple - used for right click menus and pop_menu
* Error windows - better line wrapping
* Show an error window if a bad Image specified in the Image element
* expand_x & expand_y parms for vtop vbottom vcenter,
* Added framework_version
* RealtimeButton works again!
* New popup - popup_menu will show a torn off right click menu at the indicated location
* new comment figlets
* More permissive layouts - embedded layouts for PSG+ features
* Added more symbols for buttons - double L/R & arrowheads,
* Moved theme tests into a tab" in sg.main
## 4.40.0 PySimpleGUI 25-Apr-2021
The "A4 Release" (440 Hz)
Buttons get a boost
Fix for Graph Dragging caused by last release
Gray Gray Gray should you wish to go colorless
* Right-click Menu constant - MENU_RIGHT_CLICK_EXIT - Has only "Exit"
* Constant = ['', ['Exit']]
* Checkbox.get() now returns a bool instead of int
* Button colors
* mouseover_colors - new parm to Button. Sets the color when mouse moves over the button
* When a TK Button this is Only visible on Linux systems when mouse is over button
* On Windows, these colors appear when the button is clicked
* Default is to swap the button's normal colors (text = background, background = text)
* Close Window Only button type changed to work on all windows, not just non-blocking ones
* ColorChooserButton - target now matches other choosers (target=(ThisRow, -1))
* TabGroup - new size parm that will set size in either or both directions
* Fix for TCL error when scrolling a column
* Themes
* Fix for COLOR_SYSTEM_DEFAULT error when choosing "default1" Theme
* New Theme - "Gray Gray Gray" - sets no colors. The window will likely be some shades of gray
* Changed error messages to use "theme" instead of the old "change_look_and_feel"
*
* Debug window - problems was if set the erase_all parm and Debug window is then closed
* timer_start, timer_stop - stop now returns the milliseconds elapsed instead of printing them
* popup_menu - now uses the passed in title if provided
* GitHub Issues GUI
* Made necessary changes to be 3.4 compatible. You can post Issues directly from your Pi running 3.4
* Changed layout so that the window is smaller
* New Help window uses tabs to make smaller
* Fix for extend_layout when adding to a scrollable column
* Added back functions accidently lost during a PEP8 rework
* added back popup_annoying, popup_no_border, popup_no_frame, popup_no_wait, popup_timed, sgprint, sgprint_close
## 4.41.0 PySimpleGUI 12-May-2021
New Readme & Other PyPI info
Fixed Syntax error in Text.update
* 2 more menu definition constants (simply a shortcut way to add right click menu)
* MENU_RIGHT_CLICK_EDITME_VER_EXIT = ['', ['Edit Me', 'Version', 'Exit']]
* MENU_RIGHT_CLICK_EDITME_VER_SETTINGS_EXIT = ['', ['Edit Me', 'Settings', 'Version', 'Exit']]
* 3 new SYMBOL constants. Use code completion with SYMBOL_ to find some handy symbols
* SYMBOL_CHECK = '✅'
* SYMBOL_BALLOT_X ='☒'
* SYMBOL_BALLOT_CHECK = '☑'
* Syntax error, and thus crash, if the check for widget was created is false
* Fix for scrollable Column (was able to scroll further than should have been possible)
* More docstrings to explain Column.contents_changed is needed when layout is extended
* Docstring addition for read_all_windows to explain which windows will be read and what's returned when a window is closed
* Titlebar docstring fix - return was misplaced. rtype changed to Column
* Added execute_py_get_interpreter to return the current global setting
* get_versions() function added to aid in dubugging. print(sg.get_versions())
* Returns a human readable string with labels next to each version.
* Python version x.x.x
* Port (tkinter)
* tkinter version
* PySimpleGUI version
* PySimpleGUI filename with full path
## 4.42.0 PySimpleGUI 23-May-2021
New Sizegrip Element
New MenubarCustom pseudo-Element
Grab Anywhere feature improved
* New Sizegrip element
* Needed in order to resize windows that doesn't have a titlebar
* Place as the last element on the last row of your layout
* New MenubarCustom Element
* Needed when using a custom Titlebar
* Provides the ability to have a window that is entirely themed
* Without it, was not possible to have a custom Titlebar with a menubar
* Works like the traditional Menu Element (the item chosen is returned as the event)
* Added new elements to the SDK Reference built into PySimpleGUI and in the call reference documentation online
* Grab Anywhere
* Finally got the appropriate elements and widgets excluded! Yeah!
* Now Multiline, Input, Slider, Pane, Sizegrip, active scrollbars will not move the window
* Additionally, a new method Element.grab_anywhere_exclude() will exclude your element from being grabbed
* Useful for Graph elements
* Sometimes you'll have a window with graphs that you can to be able to move using Graph element
* Other times, you are using your Graph element with drag option set. In this case, you will want to exclude it.
* Improved torn-off menu placement. Now places them at the window's location
* Combo element new bind_return_key parameter - if set, when return key is pressed and element is focused, then event will be generated. Works like the Listbox's bind_return_key
* Fix for changing the title of a Tab using
## 4.43.0 PySimpleGUI 23-May-2021
Happy User Appreciate Day!
Multiline expand_x, expand_y parms
Window.ding() - because FUN is the #1 goal
* Added 2 new parms to Multiline Element
* expand_x - if True, then the element will expand in the X direction
* expand_y - if True, then the element will expand in the Y direction
* replaces the need to perform: window['-MULTILINE KEY-'].expand(True, True, True)
* Defaults to FALSE to be backward compatible
* popup_scrolled
* changed to be resizable by default and expands the multline too
* if no_titlebar is set, then a Sizegrip will be added, unless no_sizerip parm = True
* easy_print(sg.Print)
* changed to be resizable by default and exands the multiline too
* if no_titlebar is set, then a Sizegrip will be added
* Window.ding() added - get your user's attention when errors happen or just for FUN
* Added Element.grab_anywhere_include - includes an element in grab_anywhere in case you have something like a Multiline element that you can to move the window using that element
## 4.44.0 PySimpleGUI 13-Jun-2021
popup with history
clipboard functions
fonts for printing
* Added clipboard_set and clipboard_get functions
* Known tkinter problem requires application to remain running until pasted. Found a workaround for Windows.
* History feature added to popup_get_file and popup_get_folder
* Set parameter history=True
* Your users will love it! (promise)
* font parameter added for Multiline-type of outputs so font can be changed on a per char basis. Added to:
* Multiline.print
* cprint
* Debug print - Print, easy_print
* Listbox visibility fix
* Tree, Table expansion fixed
* Combo size not changed unless the size parameter changes in the update call
* Canvas removed from return values
* Versions string returned from get_versions() is clearer
* cwd automatically set for folder of application being launched when execute_py_file is called with cwd=None
* Fix for Mac for popup_get_file
* Better button error handling when bad Unicode chars are used or bad colors provided
* Open GitHub Issue GUI improved. Added collapse button for top section
* See-through mode in test harness changed to be a toggle
* Several error messages changed to error popups with traceback
* Combo added to list of elements that initially get focus when default focus is used
* Sizegrip autoexpands row so that it anchors correctly to right hand side
* MENU_SEPARATOR_LINE constant
* Button highlightthickness set to 0 if padding on the button is 0 in either x or y
* `__version__` fix for pip installed versions
* Release dedicated to Lester Moore
## 4.45.0 PySimpleGUI 21-Jun-2021
Happy 1M installs and 3 year anniversary edition!
* Fix for no titlebar windows on Raspberry Pi
* This appears to have fixed a problem on REPL.It
* And also on the Mac!
* Setting twice now - not sure if will cause a side effect
* Docstring updates for more clarity on Window.current_location
* Menu Element (recorded the Udemy lesson which generally results in finding some problems)
* fix for update modifying the caller's data!
* fix for color settings incorrectly in update
* fixed docstring
* fixed menu tearoff location after update
* Better string length handling in the error popups
* NEW popup - popup_error_with_traceback
* Provides the same error window as used internally with PySimpleGUI
* Changed Output element's docstring to explain Multiline is now recommended instead
* Fix for combo and input element readonly state not being recalled when updating disabled value
* Moved *args to end in one_line_progress_meter
## 4.46.0 PySimpleGUI 10-Aug-2021
McRelease - Lots of Mac changes including new Mac patch control panel in global settings
expand_x, expand_y in the constructors
docstrings reformatted
Text Elements really autosize now
* Multiline.print & cprint
* Added autoscroll parameter - defaults to True (backward compatible)
* will now take a single color and use as text color
* Text element - autosize with size of None, None creates an expanding Label widget with size and width of None and wraplen=0 (truely autosizing it appears!),
* ButtonMenu
* use font for button as menu font if none is supplied
* fixed mutible problem - makes a copy of menu definition when making ButtonMenu
* made menu definition optional so can change only some other settings
* Mac
* New window added to control the patches and feature disables. Access by calling main_mac_feature_control or through the global settings window from main()
* Disables grab anywhere if a titlebar is present
* Right click menu bound to Button2 which is the right button on a Mac (Button3 for all other systems)
* FINALLY found the no-titlebar problem - weird tkinter bug. Can't set alpha channel while making window if no titlebar on Mac (credit to Tanay for this find!!)
* Allowed Modal windows again
* Will not try to apply no titlebar patch if tkinter version >= 8.6.10 regardless of user settings
* Disable the alpha chan to zero if the no titlebar patch is set on the Mac. Will see the window move to center of screen for these windows.
* Added no-titlebar batch to toolore neetips
- Fixed problem with titles on some Linux systems set class_ for Toplevel windows
- Menu defintion bug fix when menu shortcut char in first pos and item is disabled !&Item
- Sizegrip fixed expansion problem
- Added kill application button to error popup
- one_line_progress_meter
- keep_on_top parameter added
- no_button parameter added so that no cancel button is shown
- Deprication warning added to FindElement as first step of moving out of non-PEP8 world,
- Added TabGroup.add_tab to add new tab to TabGroup at runtime
- execute_py_file
* set cwd='.' if dir not found
* check for file exists
- Right click menu
- added to Radio Checkbox Tabgroup Spin Slider
- Elements that don't have a right_click_menu parm now pick up the default from the Window
- Added a right click menu callback to cover portions of the window that don't have an element on them
- Changed Button binding for Mac to Button2 (the right button rather than middle on the other systems)
- Made right click menus based on button release (MUCH better)
- docstrings
- Reformatted all docstrings to line up the desriptions for better readability
- Added type and rtype to docstrings that were missing any entries
- Updated all font entires in docstrings to include list as well as string
- Added stderr to Debug print if rerouting stdout
- expand_x and expand_y now in the constructor of all elements. No longer need to call Element.expand after finalizing window if using these.
- Added Window.perform_long_operation to automatically run users functions as threads
- Fixed Text.get() was returning not the latest value when set by another element
- Set cursor color to the same as the text color for Input Combo Spin Multiline Output
- Added echo_stdout to debug print so that stdout can be captured when run as a subprocess
- Addition of autosave parameter for UserSettings
- Test harness
- Made progress meter shorter so that the test harness fit better on smaller screens (a constant battle)
- Compacted Test Harness significantly so it's 690x670
- Added Sizegrip to Debug Window
- New Grab Anywhere move code
- Move all windows at the same timed if using grab_anywhere (experimental) (set Window._move_all_windows = True)
- Table element set the headers to stretch if expand_x is True
- Element.set_size if Graph element then also set the member variable CanvasSize
- added exception details when making window with 0 alpha
- Check for no color setting when setting the cursor color for inputs (must test for gray gray gray theme in the future regression tests)
- Added exception details if have a problem with the wm_overriderediect
- Addition of "project information" to the issue your opportunity to share something about what you're making
## 4.47.0 PySimpleGUI 30-Aug-2021
Stretch & VStretch - A new era of element alignment!
Upgrade from GitHub - uses pip for real now
Image element - Simpler to use
`size` and `pad` parms can be ints in addition to tuples to speed up coding
- `rstrip` parm added to `Multiline` element
- `Combo.update` fixed bug added in 4.45.0 with disabled not working correctly when calling update
- Changed font type in all docstrings to be (str or (str, int[, str]) or None) (thank you Jason!!)
- Added code from Jason (slightly modified) for _fixed_map
- Fix for default element size was incorrectly using as the default for parm in Window.
- Needed to set it in the init code rather than using the parm to set it.
- `Window.location` gets a new parm `more_accurate` (defaults to `False`). If `True`, uses window's geometry
- Added `Window.keep_on_top_set` and `Window.keep_on_top_clear`. Makes window behave like was set when creating Window
- Image Element
- Added new constant `BLANK_BASE64` that essentially erases an Image element if assigned to it. It's 1x1 pixel and Alpha=0
- Image element New `source` parameter as the first parm.
- Can be a string or a bytestring. Backwards compatible because first was filename.
- Works for both the init and the update. No need to specify any name at all... just pass in the thing you want to change to. Greatly shortens code.
- Ths idea is to not have to specify the parameter name. `sg.Image('filename')` and `sg.Image(base64)` both work without using any parameter names.
- Fix for `Image.update` docstring
- Element sizes, when being created, can be an **int**. If `size=int`, then it represents a `size=(int, 1)`. GREATLY shortens layouts.
- Sometimes this these things only become apparent later even though it seems obvious
- padding - Another tuple / int convenience change.
- Tired of typing `pad=(0,0)`? Yea, me too. Now we can type `pad=0`.
- If an int is specified instead of a tuple, then a tuple will be created to be same as the int. `pad=0` is the same as `pad=(0,0)`
- Add NEW upgrade from GitHub code. Thank you @israel-dryer!
- Change in ttk style naming to ensure more unique style names are used
- Cast key to string when making a ttk style
- Added `"___"` between unique counter and user's key when making a unique style string for ttk widgets. Fixed problem with elements from one window interfering with another window elements
- Changed Upgrade From GitHub Code
- When upgrading, use the interpreter from the global settings for the upgrade! This could get tricky, but trying to make it logical
- Output of the pip command shown in an upgrade window using a `Multiline` element so errors can be copied from it.
- Added printing of the value of `sys.executable` to the upgrade information
- `Stretch` and `VStretch` Elements - a promising solution to element justification!
- Redefinition of the `Stretch` element. No longer returns an Error Element. It now returns a Text element that does the same kind of operation as the PySimpleGUIQt's `Stretch` element! Very nice!
- `VStretch` stretches vertically instead of horizontally
- UserSettings APIs
- Changed the repr method of the user settings object to use the pretty printer to format the dictionary information into a nicer string
## 4.48.0 PySimpleGUI 25-Sept-2021
- Highlights:
- Table clicking
- Push element
- p = pad in layouts
* Table Element - Feature expansion
* enable_click_events - New parameter and associated events
* If parm is True, then events will be generated that are tuples when a user clicks on the table and headers
* The event format is a tuple: ('-TABLE KEY-', '+CICKED+', (3, 3)) 3 items in the tuple:
1. The Table's key
2. "An additional event name" in this case I've called it "+CLICKED+"
3. The (row, col)
* The (row, col) will match the user's data unless one of these is clicked:
* A header (will return a row of -1)
* A row number (these are artificially generated numbers) and has a column of -1
* set_options - new keep_on_top option. Makes all windows you create, including popups, have keep_on_top set to True
* User Settings APIs
* user_settings_object() - returns the UserSettings object that is used by the function interface for the user_settings APIs
* Improved print by returning the pprint formattted dictionary rather than just the string version
* Docstrings
* set_clipboard takes str or bytes
* ProgressBar - better size parm description
* Fixed return type for Window.read_all_windows
* ProgressBar - new size_px parameter allows you to specify your meter directly in pixels
* pad alias! Lazy coders and those wanting ultra-compact layouts may like this one
* You can use the parameter p just like the parameter pad
* pad joins the parameters size (s) and key (k)
* Push Element
* Alias for Stretch - they are the exact same thing
* Stretch was a term used by Qt.
* Push "feels" more like what it does. It "pushes" other elements around
* Alias is P - like many other Elements, it has a 1-letter alias that can be used to write more compact code
* Removed printing of Mac warnings about global settings at the startup
* Redefined the Debug button to be a simple button with a the graphic as before
* Added a right click menu to the SDK reference so the window can be closed if moved off the screen too far
## 4.49.0 PySimpleGUI 30-Sept-2021
- Highlights
- popup_get_file bug fix (primary reason for a quick release)
- VPush
- popup_get_file fix
- VPush = VP = VStretch
- Same concept as Push element except in the Vertical direction
- `Image.update_animation_no_buffering` bug fix wasn't checking timer between frames (DOH!)
- `one_line_progress_meter` no longer returns a not OK when max reached. Makes the user if statements much easier to get only cancel as False
- Note that this is a backward compatibility problem is you are relying on a False being returned when the counter reaches the maximum
- `popup_get_file` fixed bug when `show_hidden` is set. Added to docstring
- Added `popup_get_file`, get_folder, get_data to the test harness under the popups tab
- Changed docstring for Multiline default value to Any and added a cast to string
- Added more tests and information to the `sg.main()` test harness
## 4.50.0 PySimpleGUI 17-Oct-2021
UserSettings API - support for .INI files
Listbox horizontal scrollbar
Column Element allow None for 1 size direction
* UserSettings API
* INI File Support
* Read access: `settings[section][key]`
Modify existing section and key: `settings[section][key] = new_value`
Create a new key in an existing section: `settings[section][new_key] = new_value`
Create a new section and key: `settings[new_section][new_key] = new_value`
Delete a section: `settings.delete_section(section)`
Save the INI file: `settings.save()`
* Available for UserSettings object only, not the function interface
* Demo Program released specific to .ini features
* Option to convert strings to Python values for True, False, None
* Added checks for running on Trinket or Replit so path can be set to "." if on either
* Added `running_replit` function. Returns True if environment is repl.it
* New option in set_options - `warn_button_key_duplicates` will show a warning if duplicate keys found on buttons. Defaults to OFF (duplicate key attempts on Buttons are common and OK)
* Right Click Menus
* New Element method `Element.set_right_click_menu`
* Enables changing a right click menu after initial window is created
* If none specified, uses the parent's menu
* Added `Window.get_size_accurate()` to get the window's size based on the geometry string from tkinter
* Removed moving of the theme color swatch preview window and allowed to center now
* Added check for bad value returned from tkinter when table clicked event happens
* Removed print when 8.6.9 ttk treeview code is patched
* Removed a debug print accidentally left in the bind code
* Listbox - added horizontal scrollbar option
* New `pin` layout helper function implementation (hopefully better, not worse)
* Column Element - Allow `None` to be used in any part of the `size`.
* If None used on width, then Column will default to width required by contents.
* If None used on height, then Column will default to width required by contents divided by 2
* These are same values as `(None, None)` today but can invidually control now.
* Made `Window.LayoutAndRead` deprication more user friendly with a popup
* Added * to the `file_types` default so that files without an extension are shown (only a problem on non-Windows systems). Default is now `(("ALL Files", "*.* *"),)`
* Changed `popup_get_file`, the Browse buttons, etc
* `FILE_TYPES_ALL_FILES` is a new constant with this value
* `popup_scrolled` added 1 line per argument to fit the contents better in some cases
## 4.51.0 PySimpleGUI 18-Oct-2021
`relative_location` parameter for `Window`
* New parameter for `Window` - `relative_location`
* Locates the window at an **offset** from the normal location
* Very useful for multi-window applications
* Also works when you've set a default window location using the `set_options` call.
## 4.51.2 - 4.51.7
A series of dot releases to make the psg commands operational for upgrading, etc. Was a bit of a mess for a week
## 4.52.0 - A deleted release that instead fell back to 4.51.* dot releases
## 4.53.0 PySimpleGUI 24-Oct-2021
The "Mike's really excited about this release!" release
psg commands!
psgmain
psgupgrade
psghelp
psgver
psgsettings
Control Click window movement
Frame Elements with `size` parameter
I really like this release. It pulls together a ***lot*** of work over the past week. It fixes some things that have bothered me for a long time and adds support for some things that have bothered users for a long time.... so here we go....
* Added Commands that you can type or make shortcuts to
* psgmain - Runs the sg.main() test harness. Your gateway to settings, version info, etc
* psgupgrade - Upgrades PySimpleGUI to the latest version on ***GitHub***
* psghelp - view the SDK help window
* psgver - view the version numbers
* psgsettings - access the settings window (usually done via the main window)
* Don't forget to use `sudo` if you're upgrading on Linux!
* Control Key Dragging - move ***any*** PySimpleGUI window by holding down control key while holding the left mouse button down. Ignores the usual Grab Anywhere restrictions and allows dragging over Multiline elements for example
* A new, shorter, version of the 1x1 pixel BLANK_BASE64 image
* Image Element
* New logic for the `Image.update()` (with no parms). This will delete an image and now will also shrink down to 1 pixel
* Set border width to 0 so that takes up even less space when empty
* Frame Element
* Can now use the `size` parameter to create a fixed size Frame
* `element_justification` behaves properly - consider using a `Frame` with border width=0 and no text instead of a `Column` element if you need both a hard coded size and to justify the elements inside
* `set_options`
* Added `dpi_awareness` setting to turn on DPI Awareness (currently only on Windows)
* Added `scaling` parameter for system-wide Window scaling
* `Window`
* Added `scaling` parameter - will scale the contents of the window. Takes a float value
* If need scaling for **all windows** then set using the `set_options` call
* Better 3.4 compatibility
* Previously has issues with subprocesses
* Upgrade to GitHub version now works
* The new psgcommands to work too so all you 3.4, 3.5 users out there aren't left behind!
* The PySimpleGUI Tent was built to be big and has plans on staying that way
* Exec APIs - improved ability to modify interpreter to use in other programs so that your program will then pick up the latest changes
* Testing more thoroughly 3.4, 3.6, 3.7, 3.8, 3.9, 3.10 and tkinter 8.6.2 through 8.6.10
* Doc updates to the Call Reference doc - Added `Sizer` element and reorganizing a bit.
* Special thanks to Jason for providing amazing support to the PySimpleGUI users. If you think PySimpleGUI is great... if you really want to see something impressive, try logging an issue on the GitHub and watch Jason do his thing.
## 4.54.0 PySimpleGUI 6-Nov-2021
Tabs - Are even better now
Right click menu better for Tabs, Frame, Columns
relative_location proliferation
* Tab & TabGroup
* Added image_source parameter, enabling file-based and Base64 images to be added to your tabs
* image_subample parm added so images and be reduced in size
* TabGroup.add_tab also got the image support
* tab_border_width parm added to TabGroup to control the border around the tab labels
* Added constants for Tab Location for easier code completion. All begin with TAB_LOCATION_
* focus_color added to TabGroup
* Significant change to right-click menus for Tabs. Now the Tab determines the right click menu shown when right clicking a tab title. Enables a right-click to close feature.
* Frame Element
* Better right click support in blank areas
* Added grab parameter
* Btter grab support in blank areas
* VerticalSeperator - Improvement in expansion
* VPush and Push - background_color parameter added
* grab_any_where_on - unreported bug fixed
* relative_location - a recent parameter to Window has been added to all popups and to Print
* New Base64 images
* Hearts (TWO types), green checkmark, red X
* HEART_3D_BASE64
* HEART_FLAT_BASE64
* GREEN_CHECK_BASE64
* RED_X_BASE64
* Each are 90 x 90 pixels
* Use image_subsample to reduce size to 45, 30, etc
* bar_color added to ProgressMeter.update
* visible parm added to all pre-defined buttons (FileBrowse, FolderBrowse, Ok, Cancel, etc)
* Exec APIs stderr merge with stdout
* merge_stderr_with_stdout added to execute_command_subprocess and execute_py_file
* Default it TRUE
* Stderr will be merged with stdout in 1 stream
* Right click menus propagate down the container elements (Column, Frame, Tab) to the elements inside
* Window.mouse_location() - returns tuple with mouse (x,y) location
* SDK Help window now resizble
* MENU_RIGHT_CLICK_DISABLED changed to match format of normal right click menus
* psgmain and psgupgrade - changed version of Python used to relaunch to be the same as the one calling the function to invoke PySimpleGUI. Also changed the upgrade from GitHub logic to use Python interpreter for pip as used to invoke.
## 4.55.0 PySimpleGUI 7-Nov-2021
Exec APIs - Use sys.executable as default
FIXED the install from GitHub problem with psgmain/psgupgrade!
* Exec APIs Changes
* If no interpreter is set in the global settings, then the interpreter running currently (sys.executable) will be used as the default rather than the system-wide default.
* Use python NOT pythonw (if returned from sys.executable) for all upgrades from github. The pip command was running pythonw and that caused future psgmain, psgupgrade, etc, commands to fail
## 4.55.1 PySimpleGUI 7-Nov-2021
* Exec API Fix
* Fix for bug created in 4.55.0 that caused the Global Setting for Python interpreter to never be used
* The sys.executable interpreter will be used for GitHub upgrades and if no interpreter is specified in the PySimpleGUI settings
## 4.56.0 PySimpleGUI 5-Jan-2022
The "It's been a minute" & "Welcome to 2022!" release
* Addition of stdin parm to execute_command_subprocess. This is to fix problem when pyinstaller is used to make an EXE from a psg program that calls this function
* Changed getargspec call in the SDK Reference window to getfullargspec. In 3.11 getargspec is no longer supported and thus crashes
* Added try to SDK Reference event loop to catch any additional problems that may pop up in 3.11
* Added Window.move_to_center moves a window to the center of the screen. Good for when your window changes size or you want to recenter it
* Disable debugger when installing from github
* Better error reporting when a problem with the layout detected
* Removed import of site and now get the information from os.path.dirname(sys.executable). I like simpler!
* Combo added parameters to control the colors on the button used to display the items. Parms are button_background_color and button_arrow_color
* Default values continue to be the same the theme's button color if nothing is set.
* Fixed missing docstring item for Table value so that the new documentation will be accurate
* (Maybe temporarily) added print to the Text element. Was an easy addition, but is limited in how colors are controlled, scrolling, etc. May be very short-lived addition.
* New Table Element parameter right_click_selects. Default is False. If True, then will select a row using the right mouse button, but only if
* zero or one rows are selected. If multiple rows are already selected, then the right click will not change the selection. This feature enables
* a right-click-menu to be combined with table selection for features such as "delete row" using a right click menu.
* Fixed bug in Column element was incorrectly checking background color for None or COLOR_SYSTEM_DEFAULT
* Changed docstring for Table.get_last_clicked_postition to indicate what's returned now. Was not useful for tkinter port until recently when cell clicks added.
* Better auto-sizing of Columns for Tables.
* Better sizing of the row number column using the font for the header in the calculation
* Use the column heading font to help determine if the header will be what determines the width instead of the data in the column
* Don't print the error message about wm_overrideredirect while hiding the master root if running on a Mac.
* Fix for Tree Element not setting the row height if none is specified. Needed to set to value based on the font used.
* Tree Element
* Always left justify the first column. This is how it's always worked. tkinter 8.6.12 changed the behavior of the first col. This changes it back
* Better auto-size column. Uses the data as well as the Column header to determine size of column
* Table Element fix case when tables have too many headers, thus not matching the data columns
* Tree element addition of a heading for the Column 0 (the main column shown in the Tree). Default is '' which is what's shown today.
* Graph Element Experimental addition of parm motion_events If True then mouse motion over the Graph returns event of key '+MOVE' or (key, '+MOVE')
* ButtonMenu Element
* New init parm image_source Use instead of the filename and data parms. This parm is a unified one and is how several other elements work now too.
* New update parms image_source, image_size, image_subsample enables the initial image to be changed to a new one
* Fix in sdk_help crashed if asked for summary view of Titlebar or MenubarCustom because they're not classes
* Fix in open github issue the python experience and overall experience values were swapped.
* UserSettings delete_entry will show popup error now with traceback like almost all PySimpleGUI errors (can be silenced)
* TTK Button wraplen fix, height padding fix? (thank you Jason for another fix!)
* Button fix for wraplen on non-TTK buttons.
* Layout reuse error message
* Fix for set_options checking for "not None" instead of "True" for the dpi_awareness setting. Note that once turned on, there is no option to turn off.
* Docstring changes for all Element.update methods to indicate that the change will not be visible until Window.refresh or Window.read is called
* Enabled the Text class methods that measure strings and characters to be called prior to any windows being created. Method list:
* string_width_in_pixels, char_height_in_pixels, char_width_in_pixels
* Replaced the error messages that were being printed with a poper error popup
* Removed destruction of hidden master root from popup_get_file and popup_get_folder
## 4.57.0 PySimpleGUI 13-Feb 2022
A little of this, a little of that
New Emojis for 2022... collect them all!
- set_options added disable_modal_windows option to provide a single call to disable the modal feature globally (including popups)
- Added OptionMenu to the list of tkinter widgets that are ignored when the grab anywhere feature is used
- Slider update the range FIRST and then the value in the update method (thank you Jason for the fix)
- Updated docstrings for all Element.update methods to indicate that the helper function "pin" need to be used to keep an element in place if visibility changes
- Replaced sponsor tab with a tab about the udemy course as well as the buy me a coffee link
- Fixed event generation for Spin element. Also changed to use the "command" parameter to get the event. NOTE still need to handle manual entry
- New Emojis for 2022!
- New base64 image PYTHON_COLORED_HEARTS_BASE64 (yes, more hearts... apologies to the heart-haters)
- Changed +CICKEDto +CLICKED(typo) in the table header
- Added constant TABLE_CLICKED_INDICATOR that is the value '+CLICKED+' so that it can be referenced instead of user's hard cording a string
- Added class method Text.fonts_installed_list returns list of fonts as reported by tkinter
- Horizontal scrollbar for Multiline element (long awaited). New parameter added to control just this one scrollbar. The no_scrollbar existing parm refers to the vertical scrollbar
- Fix for font parameter only being applied to Text portion of popup_get_text. Should have been to the entire window.
- Updated the internal keys to use the -KEY- coding convention. Was using the really old _KEY_ coding convention.
- Added check for bad Image filename in Image.update. Will show an error popup now like the initial Image element creation error popup
- Addition of parameter paste (bool) to Input.update. Inserts as if the value was pasted rather than replacing entirely
- Fix for Listbox scrollbar not behaving correctly when making element invisible / visible
- Docstring update for Window.perform_long_operation warns users that Thread are used and thus no PySimpleGUI calls are allowed. Also added description of exactly what happens when the user's function completes.
## 4.58.0 PySimpleGUI 3-Apr-2022
A little of this and that release
More focus on focus
`bind` methods improved with `propagate` parm
Visibility losing settings fix
- `execute_get_results` Added checking for timeout error to instead of showing an error popup as it's not truly an error in this case
- `Checkbox` Added cast to bool of default parm in case user passes in an incorrect type
- `ButtonMenu.update` addition of button_text parameter. Enables changing text displayed on the ButtonMenu. Should have been an original feature.
- Open GitHub Issue GUI Tabs use 2 lines now. Added tab asking where found PSG.
- New symbols `SYMBOL_CHECKMARK_SMALL` & `SYMBOL_X_SMALL`
- `ButtonMenu.Click` - Added click PEP8 alias `ButtonMenu.click`
- Automatically add timeouts to user reads if a debugger window is opened. Debugger for multi-window applications still needs to be added
- `Window.start_thread` a simple alias for `Window.perform_long_operation`. It's clearer what's happening with this alias.
- `bind_return_key` parm added to Spin element. If element has focus and the return key is pressed, then an event is generated.
- Event generation for disabled elements
- If an element is disabled, then don't generate events (fixed specifically for Input element). However, if a Browse button fills in a disabled element, then an event should still be generated
- Don't generate events if no files / folders selected using File or Folder Browse buttons. If cancel is clicked then no longer generates an event.
- Fix docstring for image in the Titlebar element. Incorrectly said an ICO file can be used. Must be PNG or GIF
- Windows-specific code that enables the PySimpleGUI supplied icon to be shown rather than the python.exe logo
- Removed all temporary Tk() window creation calls
- Instead create the hidden master root.
- These were required for operations like getting the list of fonts from tkinter, the screensize, character width and height. This way one and only one Tk window will ever be creeated
- The reason for the change is that the Mac crashes if multiple Tk() objects are created, even if only 1 at a time is active.
- `image_source` parm added to `Button`
- It can be either a filename or a base64 string.
- This is like the Image elements parms
- Graph element doc string improvement. Describes the mouse up event.
- Improved support for focus
- `Element.get_next_focus` added. Returns the element that should get the focus after the indicated element
- `Element.get_previous_focus` added. Returns the element that should get the focus after the indicated element
- Better exception error reporting for the Element focus methods. Uses popups with tracebacks now instead of prints
- `Window.widget_to_element` returns the element that a tkinter widget is used to implement (it's a reverse lookup)
- `Element.widget` added. It's a PEP8 compliant property that returns `Element.Widget`
- `Element.key` added. It's a PEP8 compliant property that returns `Element.Key`
- Simplified Radio, Checkbox, Slider creation. Moved the command parm outside the creation and instead made a config call.
- Visibility fix
- Expand and other settings were being lost when element is made invisible and visible again.
- `propagate` parameter to the bind methods. Used to tell tkinter whether or not to propagate the event to the element / or window
- `Canvas.update` method added so that a `Canvas` can be made visible/invisible
- Removed the need for `tk.scrolledtext.ScrolledText` by adding a vertical scrollbar to a Text widget. Getting ready for addition of ttk scrollbars!
- `tooltip_offset` parm added to `set_options` as a way to set tooltip location (a hack to get around an 8.6.12 bug)
- `Table` and `Tree` elements new parameters
- `border_width` - the border width for the element
- `header_border_width` - the width of the border for the header
- `header_relief` - the type of header relief to use
- `Table` and `Tree` elements are now excluded from grab-anywhere so that headers can be resized without moving the window
## 4.59.0 PySimpleGUI 4-Apr-2022
An oh sh*t release due to yesterday's bug
New force modal Windows option
Test harness forces all windows to be modal and is no longer keep-on-top
- Removed ttk theme from the test harness. Forgot that I had changed it for testing.
- Fixed bug where disabled state was not correctly saved in update methods, causing events to not be generated (Thank you Jason, again!)
- Changed numerous elements, not just the `Input` element that demonstrated the problem
- New `force_modal_windows` parm added to `set_options`
- Forces all windows to be modal
- Overrides the `disable_modal_windows` option
- Used in the `main()` test harness to ensure all windows are modal so no window is accidentally lost
- Test Harness changes
- Set `keep_on_top=True` for all popups and windows created by test harness
- Set the main window `keep_on_top=False`. Ensures that all windows created by it should never be hidden. This is a somewhat experimental change. Let's hope for the best!
- Forced all windows except for 1 non-modal popup to be modal. This also should ensure no windows are "lost" behind the main window
## 4.60.0 PySimpleGUI 8-May-2022
TTK Scrollbars... the carpet now matches the drapes
Debug Window improvements
Built-in Screen-capture Initial Release
Test Harness and Settings Windows fit on small screens better
* Debug Window
* Added the `wait` and `blocking` parameters (they are identical)
* Setting to `True` will make the `Print` call wait for a user to press a `Click To Continue...` button
* Example use - if you want to print right before exiting your program
* Added `Pause` button
* If clicked, the `Print` will not return until user presses `Resume`
* Good for programs that are outputting information quickly and you want to pause execution so you can read the output
* TTK
* TTK Theme added to the PySimpleGUI Global Settings Window
* Theme list is retrieved from tkinter now rather than hard coded
* TTK Scrollbars
* All Scrollbars have been replaced with TTK Scrollbars
* Scrollbars now match the PySimpleGUI theme colors
* Can indicate default settings in the PySimpleGUI Global Settings Window
* Can preview the settings in the Global Settings Window
* Scrollbars settings are defined in this priority order:
* The Element's creation in your layout
* The Window's creation
* Calling `set_options`
* The defaults in the PySimpleGUI Global Settings
* The TTK Theme can change the appearance of scrollbars as well
* Impacted Elements:
* `Multiline`
* `Listbox`
* `Table`
* `Tree`
* `Output`
* Tree Element gets horizontal scrollbar setting
* `sg.main()` Test Harness
* Restructured the `main()` Test Harness to be more compact. Now fits Pi screens better.
* Made not modal so can interact with the Debug Window
* Turned off Grab Anywhere (note you can always use Control+Drag to move any PySimpleGUI window)
* Freed up graph lines as they scroll off the screen for better memory management
* Made the upgrade from GitHub status window smaller to fit small screens better
* Global Settings
* Restructured the Global Settings window to be tabbed
* Added ability to control Custom Titlebar. Set here and all of your applications will use this setting for the Titlebar and Menubar
* TTK Theme can be specified in the global settings (already mentioned above)
* New section for the Screenshot feature
* Exception handling added to `bind` methods
* Screenshots
* First / early release of a built-in screenshot feature
* Requires that PIL be installed on your system
* New `Window` method `save_window_screenshot_to_disk`
* Global Settings allows definition of a hotkey that triggers a save
* Popup is shown when hotkeys are used
* **To be clear** - PIL does not need to be installed in order to use PySimpleGUI. ONLY when a capture is attempted does PySimpleGUI try to import PIL
* It's the first step of building the larger "Gallery" feature
* The alignment is not perfect and the whole thing needs more work
* The auto-numbering freature is not yet implemented. Only 1 file is used and is overwritten if exists
* `user_settings_delete_filename` got a new parm `report_error` (off by default). The `UserSettings` object also got this parm to help control error reporting
* Themes (PySimpleGUI Themes)
* `theme_global` - added error checking and reporting should non-strandard theme names be attempted with this call
* New theme `Dark Grey 15`. Give it a try!
* New theme `Python Plus` - a more saturated blue and yellow colors. Give it a try!
* New function - `theme_button_color_background` - read-only call that returns the button background color. Previously only available as a tuple using `theme_button_color`.
* New function - `theme_button_color_text` - read-only call that returns the button text color. Previously only available as a tuple using `theme_button_color`.
* New function - `theme_use_custom_titlebar` returns `True` if Global Settings indicate custom titlebars should will be used
* `Output` Element - implementation changed to use the Multiline Element. No one should be impacted unless you were using some internal object details that was not published. I still suggest using the `Multiline` element instead so that you can access much more functionality.
* Tab errors now use the popup errors with traceback
* `Column` Element
* Fixed scrollwheel not working correctly when expand paramters used. Scrolls the canvas now not the frame.
* New `size_subsample_width` & `size_subsample_height` parameteres
* Gives much more control over the sizing of SCROLLABLE columns. Previously the size was set to 1/2 the required height and the full required width.
* The defaults are backward compatible (size_subsample_width=1, size_subsample_height=2)
* Setting both to 1 will make the Column fit the contents exactly. One use is when you expect your Column to grow or shrink over time. Or maybe you didn't like the 1/2 size that PySimpleGUI has always used before.
* Made Select Colors match the theme colors
* `Input`, `Multiline`, `Combo` elements now use matching colors for selections of characters (big-time thanks to Jason who also provided the magic code to make the combo drop-down match the theme)
* `popup_get_file` - Removed the file_types parameter use if on a Mac
* Missed catching this problem when added the no_window option
* Need to revisit this file types on the Mac topic in next release.
* Particularly bad problem because cannot catch the exception. Your code simply crashes. And the behavior isn't the same across all Macs.
* I'm really sorry Mac users that we keep running into these kinds of issues!
* Auto-correct file_types problems for Browse buttons. Automatically change the formatting from (str, str) to ((str, str),) and warns the user
* Docstring typo fixes for file_types parm
## 4.60.1 PySimpleGUI 22-May-2022
* A patch-release that fixes crash if `horizontal_scrollbar=True` when making a `Listbox` element
## 4.60.2 PySimpleGUI 26-Jul-2022
* Emergency Patch Release for Mac OS 12.3 and greater
* Adds a PySimpleGUI Mac Control Panel Controlled patch that sets the Alpha channel to 0.99 by default for these users
* Is a workaround for a bug that was introduced into Mac OS 12.3
## 4.60.3 PySimpleGUI 27-Jul-2022
* Emergency Patch Release for Mac OS 12.3 and greater
* Fixed bug in Mac OS version check in yesterday's 4.60.2 release
## Code Condition
Make it run
Make it right
Make it fast
It's a recipe for success if done right. PySimpleGUI has completed the "Make it run" phase. It's far from "right" in many ways. These are being worked on. The module has historically been particularly poor for PEP8 compliance. It was a learning exercise that turned into a somewhat complete GUI solution for lightweight problems.
While the internals to PySimpleGUI are a tad sketchy, the public interfaces into the SDK are more strictly defined and comply with PEP8 naming conventions. A set of "PEP8 Bindings" was released in summer of 2019 to ensure the externally facing interfaces all adhere to PEP8 names.
Please log bugs and suggestions **only on the PySimpleGUI GitHub**! It will only make the code stronger and better in the end, a good thing for us all, right? Logging them elsewhere doesn't enable the core developer and other PySimpleGUI users to help. To make matters worse, you may get bad advice from other sites because there are simply not many PySimpleGUI experts, yet.
## Design
A moment about the design-spirit of `PySimpleGUI`. From the beginning, this package was meant to take advantage of Python's capabilities with the goal of programming ease.
**Single File**
While not the best programming practice, the implementation resulted in a single file solution. Only one file is needed, PySimpleGUI.py. You can post this file, email it, and easily import it using one statement.
**Functions as objects**
In Python, functions behave just like object. When you're placing a Text Element into your form, you may be sometimes calling a function and other times declaring an object. If you use the word Text, then you're getting an object. If you're using `Txt`, then you're calling a function that returns a `Text` object.
**Lists**
It seemed quite natural to use Python's powerful list constructs when possible. The form is specified as a series of lists. Each "row" of the GUI is represented as a list of Elements.
**Dictionaries**
Want to view your form's results as a dictionary instead of a list... no problem, just use the `key` keyword on your elements. For complex forms with a lot of values that need to be changed frequently, this is by far the best way of consuming the results.
You can also look up elements using their keys. This is an excellent way to update elements in reaction to another element. Call `form.FindElement(key)` to get the Element.
**Named / Optional Parameters**
This is a language feature that is featured **heavily** in all of the API calls, both functions and classes. Elements are configured, in-place, by setting one or more optional parameters. For example, a Text element's color is chosen by setting the optional `text_color` parameter.
**tkinter**
tkinter is the "official" GUI that Python supports. It runs on Windows, Linux, and Mac. It was chosen as the first target GUI framework due to its ***ubiquity***. Nearly all Python installations, with the exception of Ubuntu Linux, come pre-loaded with tkinter. It is the "simplest" of the GUI frameworks to get up an running (among Qt, WxPython, Kivy, etc).
From the start of the PSG project, tkinter was not meant to be the only underlying GUI framework for PySimpleGUI. It is merely a starting point. All journeys begin with one step forward and choosing tkinter was the first of many steps for PySimpleGUI. Now there are 4 ports up and running - tkinter, WxPython, Qt and Remi (web support)
# Author & Owner
Written and owned by PySimpleGUI.
This documentation as well as all PySimpleGUI documentation and code is Copyright 2018, 2019, 2020, 2021, 2022 by PySimpleGUI
Send business correspondence to PySimpleGUI@PySimpleGUI.com
## License
GNU Lesser General Public License (LGPL 3) +
Please note that this license does **not** allow you to break copyright laws. You are licensing the software.
## Acknowledgments
There are a number of people that have been key contributors to this project both directly and indirectly. Paid professional help has been deployed a number of critical times in the project's history. This happens in the life of software development from time to time.
If you've helped, I sure hope that you feel like you've been properly thanked. That you have been recognized. If not, then say something.... drop an email to comments@PySimpleGUI.org.
Please see the readme file for usage of other Python packages by this project.
## Support
In response to a number of email contacts from individuals and corporations that are using PySimpleGUI that wanted to financially support the project a "Support" Button was added to the GitHub site. This support button is connected with a PayPal account. If you wish to help support this currently freely supplied software and free technical support, then follow this link: https://www.paypal.me/pythongui. You'll find all the ways you can help support PySimpleGUI in the readme.
The project is self-funded and there are ongoing costs just to offer the software (URLs, ReadTheDocs, etc). If you're a corporate user and find that PySimpleGUI is helping you financially, that's awesome. If you want to help ensure PySimpleGUI has a future, you now have that option to help. It's likely that at some point the costs will become too high for the project to continue to be free or perhaps continue at all, but until then we'll all enjoy the successes we're having.
## Legal
All documentation in this file and in the PySimpleGUI GitHub account are copyright 2018-2022 by PySimpleGUI Tech LLC. The PySimpleGUI code, the demo programs and other source code in the PySimpleGUI account also have are copyright owned by PySimpleGUI
The name "PySimpleGUI" and the PySimpleGUI logo are Trademarked
When in doubt, ask.