Release 4.20.0
This commit is contained in:
parent
08e77912d9
commit
fdbd8d700a
|
@ -1,6 +1,6 @@
|
|||
#!/usr/bin/python3
|
||||
|
||||
version = __version__ = "4.19.0.12 Unreleased \n - Window.set_title added, removed resetting stdout when flush happens, fixed MenuBar tearoff not working, fixed get folder for Macs, fixed multiline color problem, option to set tooltip font, make typing module import optional, docstring, combobox drop-down portion font change, ability to have multiple progress bar themes at one time, setting radio button to False will clear entire group, added changing title to Tab update, ButtonMenu - font for menu set to same as button, fix for Menu.update losing font setting, display detailed tkinter version, fix table/tree padding"
|
||||
version = __version__ = "4.20.0 Released 6-Jun-2020"
|
||||
|
||||
port = 'PySimpleGUI'
|
||||
|
||||
|
@ -13516,7 +13516,6 @@ def Popup(*args, title=None, button_color=None, background_color=None, text_colo
|
|||
message = str(message)
|
||||
if message.count('\n'):
|
||||
message_wrapped = message
|
||||
# message_wrapped = textwrap.fill(message, local_line_width)
|
||||
else:
|
||||
message_wrapped = textwrap.fill(message, local_line_width)
|
||||
message_wrapped_lines = message_wrapped.count('\n') + 1
|
||||
|
@ -15622,10 +15621,7 @@ def main():
|
|||
# theme('dark brown 2')
|
||||
# theme('dark red')
|
||||
# theme('Light Green 6')
|
||||
try:
|
||||
ver = version[:version.index('\n')]
|
||||
except:
|
||||
ver = version
|
||||
ver = version.split('\n')[0]
|
||||
|
||||
tkversion = tkinter.TkVersion
|
||||
tclversion = tkinter.TclVersion
|
||||
|
|
File diff suppressed because it is too large
Load Diff
|
@ -7,10 +7,10 @@
|
|||
It's been a little while getting back around to the Cookbook. As a result, some of the information was using older design patterns and some better examples need to be included. There have been about 1/3 of the changes made so far that need to get made, so be patient.
|
||||
# The PySimpleGUI Cookbook
|
||||
|
||||
Welcome to the PySimpleGUI Cookbook! It's provided as but one component of a larger documentation effort for the PySimpleGUI package. It's purpose is to give you a jump start.
|
||||
Welcome to the PySimpleGUI Cookbook! It's provided as but one component of a larger documentation effort for the PySimpleGUI package. Its purpose is to give you a jump start.
|
||||
You'll find that starting with a Recipe will give you a big jump-start on creating your custom GUI. Copy and paste one of these Recipes and modify it to match your requirements. Study them to get an idea of some design patterns to follow.
|
||||
|
||||
This document is not a replacement for the main documentation at http://www.PySimpleGUI.org. If you're looking for answers, they're most likely there in the detailed explanations and call defintions. That document is updated much more frequently than this one.
|
||||
This document is not a replacement for the main documentation at http://www.PySimpleGUI.org. If you're looking for answers, they're most likely there in the detailed explanations and call definitions. That document is updated much more frequently than this one.
|
||||
|
||||
See the main doc on installation. Typically it's `pip install pysimplegui` to install.
|
||||
|
||||
|
@ -97,7 +97,7 @@ This will be the most common pattern you'll follow if you are not using an "even
|
|||
|
||||
When you "read" a window, you are returned a tuple consisting of an `event` and a dictionary of `values`.
|
||||
|
||||
The`event` is what caused the read to return. It could be a button press, some text clicked, a list item chosen, etc, or `None` if the user closes the window using the X.
|
||||
The `event` is what caused the read to return. It could be a button press, some text clicked, a list item chosen, etc, or `None` if the user closes the window using the X.
|
||||
|
||||
The `values` is a dictionary of values of all the input-style elements. Dictionaries use keys to define entries. If your elements do not specificy a key, one is provided for you. These auto-numbered keys are ints starting at zero.
|
||||
|
||||
|
@ -240,7 +240,7 @@ window = sg.Window('Pattern 2B', layout)
|
|||
while True: # Event Loop
|
||||
event, values = window.read()
|
||||
print(event, values)
|
||||
if event in (None, 'Exit'):
|
||||
if event == sg.WIN_CLOSED or event == 'Exit':
|
||||
break
|
||||
if event == 'Show':
|
||||
# Update the "output" text element to be the value of "input" element
|
||||
|
@ -257,9 +257,9 @@ The way we're achieving output here is by changing a Text Element with this stat
|
|||
window['-OUTPUT-'].update(values['-IN-'])
|
||||
```
|
||||
|
||||
`window['-OUTPUT-']` returns the element that has the key `'-OUTPUT-'`. Then the `update` method for that element is called so that the value of the Text Element is modified.
|
||||
`window['-OUTPUT-']` returns the element that has the key `'-OUTPUT-'`. Then the `update` method for that element is called so that the value of the Text Element is modified. Be sure you have supplied a `size` that is large enough to display your output. If the size is too small, the output will be truncated.
|
||||
|
||||
Note - if you need to interact with elements prior to calling `window.read()` you will need to "finalize" your window first.
|
||||
If you need to interact with elements prior to calling `window.read()` you will need to "finalize" your window first using the `finalize` parameter when you create your `Window`. "Interacting" means calling that element's methods such as `update`, `draw_line`, etc.
|
||||
|
||||
------------
|
||||
|
||||
|
|
|
@ -16,6 +16,7 @@
|
|||
 
|
||||
[](../../commits/master)
|
||||
[](../../commits/master)
|
||||

|
||||
|
||||
# PySimpleGUI User's Manual
|
||||
|
||||
|
@ -52,7 +53,7 @@ window = sg.Window('Window Title', layout)
|
|||
# Event Loop to process "events" and get the "values" of the inputs
|
||||
while True:
|
||||
event, values = window.read()
|
||||
if event in (None, 'Cancel'): # if user closes window or clicks cancel
|
||||
if event == sg.WIN_CLOSED or event == 'Cancel': # if user closes window or clicks cancel
|
||||
break
|
||||
print('You entered ', values[0])
|
||||
|
||||
|
@ -107,8 +108,6 @@ and returns the value input as well as the button clicked.
|
|||
* 170+ Demo Programs teach you how to integrate with many popular packages like OpenCV, Matplotlib, PyGame, etc.
|
||||
* 200 pages of documentation, a Cookbook, built-in help using docstrings, in short it's heavily documented
|
||||
|
||||
#### July-2019 Note - This readme is being generated from the PySimpleGUI.py file located on GitHub. As a result, some of the calls or parameters may not match the PySimpleGUI that you pip installed.
|
||||
|
||||
## GUI Development does not have to be difficult nor painful. It can be (and is) FUN
|
||||
|
||||
#### What users are saying about PySimpleGUI
|
||||
|
@ -296,7 +295,7 @@ window = sg.Window('Window Title', layout)
|
|||
# Event Loop to process "events"
|
||||
while True:
|
||||
event, values = window.read()
|
||||
if event in (None, 'Cancel'):
|
||||
if event in (sg.WIN_CLOSED, 'Cancel'):
|
||||
break
|
||||
|
||||
window.close()
|
||||
|
@ -431,7 +430,7 @@ import tkinter
|
|||
import cv2, PySimpleGUI as sg
|
||||
USE_CAMERA = 0 # change to 1 for front facing camera
|
||||
window, cap = sg.Window('Demo Application - OpenCV Integration', [[sg.Image(filename='', key='image')], ], location=(0, 0), grab_anywhere=True), cv2.VideoCapture(USE_CAMERA)
|
||||
while window(timeout=20)[0] is not None:
|
||||
while window(timeout=20)[0] != sg.WIN_CLOSED:
|
||||
window['image'](data=cv2.imencode('.png', cap.read()[1])[1].tobytes())
|
||||
```
|
||||
|
||||
|
@ -1340,7 +1339,7 @@ You can, and will be able to for some time, use both names. However, at some po
|
|||
|
||||
The help system will work with both names as will your IDE's docstring viewing. However, the result found will show the CamelCase names. For example `help(sg.Window.read)` will show the CamelCase name of the method/function. This is what will be returned:
|
||||
|
||||
`Read(self, timeout=None, timeout_key='__TIMEOUT__')`
|
||||
`Read(self, timeout=None, timeout_key='__TIMEOUT__', close=False)`
|
||||
|
||||
## The Renaming Convention
|
||||
|
||||
|
@ -1844,6 +1843,7 @@ Parameter Descriptions:
|
|||
| str | transparent_color | This color will be completely see-through in your window. Can even click through |
|
||||
| str | title | Title that will be shown on the window |
|
||||
| str | icon | Same as Window icon parameter. Can be either a filename or Base64 value. For Windows if filename, it MUST be ICO format. For Linux, must NOT be ICO |
|
||||
| None | **RETURN** | No return value
|
||||
|
||||
***To close animated popups***, call PopupAnimated with `image_source=None`. This will close all of the currently open PopupAnimated windows.
|
||||
|
||||
|
@ -2328,7 +2328,7 @@ The button value from a Read call will be one of 2 values:
|
|||
|
||||
If a button has a key set when it was created, then that key will be returned, regardless of what text is shown on the button. If no key is set, then the button text is returned. If no button was clicked, but the window returned anyway, the event value is the key that caused the event to be generated. For example, if `enable_events` is set on an `Input` Element and someone types a character into that `Input` box, then the event will be the key of the input box.
|
||||
|
||||
### **None is returned when the user clicks the X to close a window.**
|
||||
### **WIN_CLOSED (None) is returned when the user clicks the X to close a window.**
|
||||
|
||||
If your window has an event loop where it is read over and over, remember to give your user an "out". You should ***always check for a None value*** and it's a good practice to provide an Exit button of some kind. Thus design patterns often resemble this Event Loop:
|
||||
|
||||
|
@ -2551,7 +2551,7 @@ Clicking the Submit button caused the window call to return. The call to Popup
|
|||
|
||||
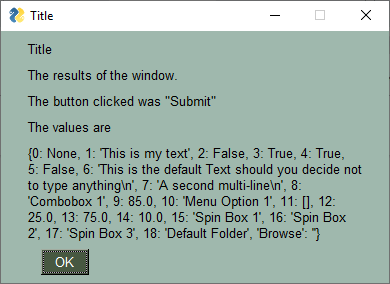
|
||||
|
||||
**`Note, event values can be None`**. The value for `event` will be the text that is displayed on the button element when it was created or the key for the button. If the user closed the window using the "X" in the upper right corner of the window, then `event` will be `None`. It is ***vitally*** ***important*** that your code contain the proper checks for None.
|
||||
**`Note, event values can be None`**. The value for `event` will be the text that is displayed on the button element when it was created or the key for the button. If the user closed the window using the "X" in the upper right corner of the window, then `event` will be `sg.WIN_CLOSED` which is equal to `None`. It is ***vitally*** ***important*** that your code contain the proper checks for `sg.WIN_CLOSED`.
|
||||
|
||||
For "persistent windows", **always give your users a way out of the window**. Otherwise you'll end up with windows that never properly close. It's literally 2 lines of code that you'll find in every Demo Program. While you're at it, make sure a `window.close()` call is after your event loop so that your window closes for sure.
|
||||
|
||||
|
@ -2851,7 +2851,7 @@ Call to force a window to go through the final stages of initialization. This w
|
|||
|
||||
If you want to call an element's `Update` method or call a `Graph` element's drawing primitives, you ***must*** either call `Read` or `Finalize` prior to making those calls.
|
||||
|
||||
#### read(timeout=None, timeout_key=TIMEOUT_KEY)
|
||||
#### read(timeout=None, timeout_key=TIMEOUT_KEY, close=False)
|
||||
|
||||
Read the Window's input values and button clicks in a blocking-fashion
|
||||
|
||||
|
@ -3980,7 +3980,7 @@ while (True):
|
|||
# This is the code that reads and updates your window
|
||||
event, values = window.read(timeout=50)
|
||||
print(event)
|
||||
if event in ('Quit', None):
|
||||
if event in ('Quit', sg.WIN_CLOSED):
|
||||
break
|
||||
|
||||
window.close() # Don't forget to close your window!
|
||||
|
@ -5276,7 +5276,7 @@ win2_active = False
|
|||
while True:
|
||||
ev1, vals1 = win1.read(timeout=100)
|
||||
win1['-OUTPUT-'].update(vals1[0])
|
||||
if ev1 is None or ev1 == 'Exit':
|
||||
if ev1 == sg.WIN_CLOSED or ev1 == 'Exit':
|
||||
break
|
||||
|
||||
if not win2_active and ev1 == 'Launch 2':
|
||||
|
@ -5288,7 +5288,7 @@ while True:
|
|||
|
||||
if win2_active:
|
||||
ev2, vals2 = win2.read(timeout=100)
|
||||
if ev2 is None or ev2 == 'Exit':
|
||||
if ev2 == sg.WIN_CLOSED or ev2 == 'Exit':
|
||||
win2_active = False
|
||||
win2.close()
|
||||
```
|
||||
|
@ -5309,7 +5309,7 @@ win1 = sg.Window('Window 1', layout)
|
|||
win2_active=False
|
||||
while True:
|
||||
ev1, vals1 = win1.read(timeout=100)
|
||||
if ev1 is None:
|
||||
if ev1 == sg.WIN_CLOSED:
|
||||
break
|
||||
win1.FindElement('-OUTPUT-').update(vals1[0])
|
||||
|
||||
|
@ -5322,7 +5322,7 @@ while True:
|
|||
win2 = sg.Window('Window 2', layout2)
|
||||
while True:
|
||||
ev2, vals2 = win2.read()
|
||||
if ev2 is None or ev2 == 'Exit':
|
||||
if ev2 == sg.WIN_CLOSED or ev2 == 'Exit':
|
||||
win2.close()
|
||||
win2_active = False
|
||||
win1.UnHide()
|
||||
|
@ -7215,6 +7215,34 @@ Long list of stuff!
|
|||
* Some changes in test harness that tested recent changes (may still need shortening for trinket or others)
|
||||
* Changed the misleading TRANSPARENT_BUTTON constant with an attempt using themes calls
|
||||
|
||||
## 4.20.0 PySimpleGUI 6-Jun-2020
|
||||
|
||||
Fixes and new features... broad range
|
||||
|
||||
* Fix for Typing import for Pi (3.4) users. Now "tries" to import typing
|
||||
* Tooltip fonts - can change the font for tooltips
|
||||
* Fixed tearoff for Menus. Had stoppped working
|
||||
* Radio - If element is updated to False, the entire group of radio buttons will be set to false tooltips
|
||||
* Multiline - fix for colors. Only set tags for output that has specific colors
|
||||
* Multiline - keeping track of disabled with Disabled mumber variable
|
||||
* Progress bar
|
||||
* Added class variable "uniqueness counter" so that every bar will have its own settings
|
||||
* Needed in case the same key is used in another window
|
||||
* Fix for stdout being reset if someone sets flush on their call to print
|
||||
* Mac special case added to tkfiledialog.askdirectory just like on askopenfilename
|
||||
* Tab - can "update" the title
|
||||
* Menu update - was not applying font when updating the menu
|
||||
* Window.set_title - allows you to change the title for a window
|
||||
* Added searching through Panes when looking for element with focus
|
||||
* Removed Python 2 Add Menu Item code
|
||||
* Added font to buttonmenu.
|
||||
* Added font to the combobox drop-down list (wow what a pain)
|
||||
* Table now uses the element's padding rather than 0,0
|
||||
* Tree now uses the element's padding rather than 0,0
|
||||
* set_options - added ability to set the tooltip font
|
||||
* Fixed a couple of docstrings
|
||||
* Reworked main() test harness to dispay DETAILED tkinter info and use bettter colors
|
||||
|
||||
### Upcoming
|
||||
|
||||
There will always be overlapping work as the ports will never actually be "complete" as there's always something new that can be built. However there's a definition for the base functionality for PySimpleGUI. This is what is being strived for with the currnt ports that are underway.
|
||||
|
|
56
readme.md
56
readme.md
|
@ -16,6 +16,7 @@
|
|||
 
|
||||
[](../../commits/master)
|
||||
[](../../commits/master)
|
||||

|
||||
|
||||
# PySimpleGUI User's Manual
|
||||
|
||||
|
@ -52,7 +53,7 @@ window = sg.Window('Window Title', layout)
|
|||
# Event Loop to process "events" and get the "values" of the inputs
|
||||
while True:
|
||||
event, values = window.read()
|
||||
if event in (None, 'Cancel'): # if user closes window or clicks cancel
|
||||
if event == sg.WIN_CLOSED or event == 'Cancel': # if user closes window or clicks cancel
|
||||
break
|
||||
print('You entered ', values[0])
|
||||
|
||||
|
@ -107,8 +108,6 @@ and returns the value input as well as the button clicked.
|
|||
* 170+ Demo Programs teach you how to integrate with many popular packages like OpenCV, Matplotlib, PyGame, etc.
|
||||
* 200 pages of documentation, a Cookbook, built-in help using docstrings, in short it's heavily documented
|
||||
|
||||
#### July-2019 Note - This readme is being generated from the PySimpleGUI.py file located on GitHub. As a result, some of the calls or parameters may not match the PySimpleGUI that you pip installed.
|
||||
|
||||
## GUI Development does not have to be difficult nor painful. It can be (and is) FUN
|
||||
|
||||
#### What users are saying about PySimpleGUI
|
||||
|
@ -296,7 +295,7 @@ window = sg.Window('Window Title', layout)
|
|||
# Event Loop to process "events"
|
||||
while True:
|
||||
event, values = window.read()
|
||||
if event in (None, 'Cancel'):
|
||||
if event in (sg.WIN_CLOSED, 'Cancel'):
|
||||
break
|
||||
|
||||
window.close()
|
||||
|
@ -431,7 +430,7 @@ import tkinter
|
|||
import cv2, PySimpleGUI as sg
|
||||
USE_CAMERA = 0 # change to 1 for front facing camera
|
||||
window, cap = sg.Window('Demo Application - OpenCV Integration', [[sg.Image(filename='', key='image')], ], location=(0, 0), grab_anywhere=True), cv2.VideoCapture(USE_CAMERA)
|
||||
while window(timeout=20)[0] is not None:
|
||||
while window(timeout=20)[0] != sg.WIN_CLOSED:
|
||||
window['image'](data=cv2.imencode('.png', cap.read()[1])[1].tobytes())
|
||||
```
|
||||
|
||||
|
@ -1340,7 +1339,7 @@ You can, and will be able to for some time, use both names. However, at some po
|
|||
|
||||
The help system will work with both names as will your IDE's docstring viewing. However, the result found will show the CamelCase names. For example `help(sg.Window.read)` will show the CamelCase name of the method/function. This is what will be returned:
|
||||
|
||||
`Read(self, timeout=None, timeout_key='__TIMEOUT__')`
|
||||
`Read(self, timeout=None, timeout_key='__TIMEOUT__', close=False)`
|
||||
|
||||
## The Renaming Convention
|
||||
|
||||
|
@ -1844,6 +1843,7 @@ Parameter Descriptions:
|
|||
| str | transparent_color | This color will be completely see-through in your window. Can even click through |
|
||||
| str | title | Title that will be shown on the window |
|
||||
| str | icon | Same as Window icon parameter. Can be either a filename or Base64 value. For Windows if filename, it MUST be ICO format. For Linux, must NOT be ICO |
|
||||
| None | **RETURN** | No return value
|
||||
|
||||
***To close animated popups***, call PopupAnimated with `image_source=None`. This will close all of the currently open PopupAnimated windows.
|
||||
|
||||
|
@ -2328,7 +2328,7 @@ The button value from a Read call will be one of 2 values:
|
|||
|
||||
If a button has a key set when it was created, then that key will be returned, regardless of what text is shown on the button. If no key is set, then the button text is returned. If no button was clicked, but the window returned anyway, the event value is the key that caused the event to be generated. For example, if `enable_events` is set on an `Input` Element and someone types a character into that `Input` box, then the event will be the key of the input box.
|
||||
|
||||
### **None is returned when the user clicks the X to close a window.**
|
||||
### **WIN_CLOSED (None) is returned when the user clicks the X to close a window.**
|
||||
|
||||
If your window has an event loop where it is read over and over, remember to give your user an "out". You should ***always check for a None value*** and it's a good practice to provide an Exit button of some kind. Thus design patterns often resemble this Event Loop:
|
||||
|
||||
|
@ -2551,7 +2551,7 @@ Clicking the Submit button caused the window call to return. The call to Popup
|
|||
|
||||
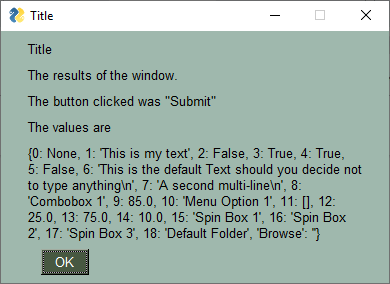
|
||||
|
||||
**`Note, event values can be None`**. The value for `event` will be the text that is displayed on the button element when it was created or the key for the button. If the user closed the window using the "X" in the upper right corner of the window, then `event` will be `None`. It is ***vitally*** ***important*** that your code contain the proper checks for None.
|
||||
**`Note, event values can be None`**. The value for `event` will be the text that is displayed on the button element when it was created or the key for the button. If the user closed the window using the "X" in the upper right corner of the window, then `event` will be `sg.WIN_CLOSED` which is equal to `None`. It is ***vitally*** ***important*** that your code contain the proper checks for `sg.WIN_CLOSED`.
|
||||
|
||||
For "persistent windows", **always give your users a way out of the window**. Otherwise you'll end up with windows that never properly close. It's literally 2 lines of code that you'll find in every Demo Program. While you're at it, make sure a `window.close()` call is after your event loop so that your window closes for sure.
|
||||
|
||||
|
@ -2851,7 +2851,7 @@ Call to force a window to go through the final stages of initialization. This w
|
|||
|
||||
If you want to call an element's `Update` method or call a `Graph` element's drawing primitives, you ***must*** either call `Read` or `Finalize` prior to making those calls.
|
||||
|
||||
#### read(timeout=None, timeout_key=TIMEOUT_KEY)
|
||||
#### read(timeout=None, timeout_key=TIMEOUT_KEY, close=False)
|
||||
|
||||
Read the Window's input values and button clicks in a blocking-fashion
|
||||
|
||||
|
@ -3980,7 +3980,7 @@ while (True):
|
|||
# This is the code that reads and updates your window
|
||||
event, values = window.read(timeout=50)
|
||||
print(event)
|
||||
if event in ('Quit', None):
|
||||
if event in ('Quit', sg.WIN_CLOSED):
|
||||
break
|
||||
|
||||
window.close() # Don't forget to close your window!
|
||||
|
@ -5276,7 +5276,7 @@ win2_active = False
|
|||
while True:
|
||||
ev1, vals1 = win1.read(timeout=100)
|
||||
win1['-OUTPUT-'].update(vals1[0])
|
||||
if ev1 is None or ev1 == 'Exit':
|
||||
if ev1 == sg.WIN_CLOSED or ev1 == 'Exit':
|
||||
break
|
||||
|
||||
if not win2_active and ev1 == 'Launch 2':
|
||||
|
@ -5288,7 +5288,7 @@ while True:
|
|||
|
||||
if win2_active:
|
||||
ev2, vals2 = win2.read(timeout=100)
|
||||
if ev2 is None or ev2 == 'Exit':
|
||||
if ev2 == sg.WIN_CLOSED or ev2 == 'Exit':
|
||||
win2_active = False
|
||||
win2.close()
|
||||
```
|
||||
|
@ -5309,7 +5309,7 @@ win1 = sg.Window('Window 1', layout)
|
|||
win2_active=False
|
||||
while True:
|
||||
ev1, vals1 = win1.read(timeout=100)
|
||||
if ev1 is None:
|
||||
if ev1 == sg.WIN_CLOSED:
|
||||
break
|
||||
win1.FindElement('-OUTPUT-').update(vals1[0])
|
||||
|
||||
|
@ -5322,7 +5322,7 @@ while True:
|
|||
win2 = sg.Window('Window 2', layout2)
|
||||
while True:
|
||||
ev2, vals2 = win2.read()
|
||||
if ev2 is None or ev2 == 'Exit':
|
||||
if ev2 == sg.WIN_CLOSED or ev2 == 'Exit':
|
||||
win2.close()
|
||||
win2_active = False
|
||||
win1.UnHide()
|
||||
|
@ -7215,6 +7215,34 @@ Long list of stuff!
|
|||
* Some changes in test harness that tested recent changes (may still need shortening for trinket or others)
|
||||
* Changed the misleading TRANSPARENT_BUTTON constant with an attempt using themes calls
|
||||
|
||||
## 4.20.0 PySimpleGUI 6-Jun-2020
|
||||
|
||||
Fixes and new features... broad range
|
||||
|
||||
* Fix for Typing import for Pi (3.4) users. Now "tries" to import typing
|
||||
* Tooltip fonts - can change the font for tooltips
|
||||
* Fixed tearoff for Menus. Had stoppped working
|
||||
* Radio - If element is updated to False, the entire group of radio buttons will be set to false tooltips
|
||||
* Multiline - fix for colors. Only set tags for output that has specific colors
|
||||
* Multiline - keeping track of disabled with Disabled mumber variable
|
||||
* Progress bar
|
||||
* Added class variable "uniqueness counter" so that every bar will have its own settings
|
||||
* Needed in case the same key is used in another window
|
||||
* Fix for stdout being reset if someone sets flush on their call to print
|
||||
* Mac special case added to tkfiledialog.askdirectory just like on askopenfilename
|
||||
* Tab - can "update" the title
|
||||
* Menu update - was not applying font when updating the menu
|
||||
* Window.set_title - allows you to change the title for a window
|
||||
* Added searching through Panes when looking for element with focus
|
||||
* Removed Python 2 Add Menu Item code
|
||||
* Added font to buttonmenu.
|
||||
* Added font to the combobox drop-down list (wow what a pain)
|
||||
* Table now uses the element's padding rather than 0,0
|
||||
* Tree now uses the element's padding rather than 0,0
|
||||
* set_options - added ability to set the tooltip font
|
||||
* Fixed a couple of docstrings
|
||||
* Reworked main() test harness to dispay DETAILED tkinter info and use bettter colors
|
||||
|
||||
### Upcoming
|
||||
|
||||
There will always be overlapping work as the ports will never actually be "complete" as there's always something new that can be built. However there's a definition for the base functionality for PySimpleGUI. This is what is being strived for with the currnt ports that are underway.
|
||||
|
|
Loading…
Reference in New Issue