commit
cd866a2c2e
4 changed files with 6483 additions and 89 deletions
|
@ -1,54 +0,0 @@
|
|||
#!/usr/bin/env python
|
||||
import sys
|
||||
if sys.version_info[0] >= 3:
|
||||
import PySimpleGUI as sg
|
||||
else:
|
||||
import PySimpleGUI27 as sg
|
||||
|
||||
sg.ChangeLookAndFeel('GreenTan')
|
||||
|
||||
# ------ Menu Definition ------ #
|
||||
menu_def = [['&File', ['&Open', '&Save', 'E&xit', 'Properties']],
|
||||
['&Edit', ['Paste', ['Special', 'Normal', ], 'Undo'], ],
|
||||
['&Help', '&About...'], ]
|
||||
|
||||
# ------ Column Definition ------ #
|
||||
column1 = [[sg.Text('Column 1', background_color='lightblue', justification='center', size=(10, 1))],
|
||||
[sg.Spin(values=('Spin Box 1', '2', '3'), initial_value='Spin Box 1')],
|
||||
[sg.Spin(values=('Spin Box 1', '2', '3'), initial_value='Spin Box 2')],
|
||||
[sg.Spin(values=('Spin Box 1', '2', '3'), initial_value='Spin Box 3')]]
|
||||
|
||||
layout = [
|
||||
[sg.Menu(menu_def, tearoff=True)],
|
||||
[sg.Text('(Almost) All widgets in one Window!', size=(30, 1), justification='center', font=("Helvetica", 25), relief=sg.RELIEF_RIDGE)],
|
||||
[sg.Text('Here is some text.... and a place to enter text')],
|
||||
[sg.InputText('This is my text')],
|
||||
[sg.Frame(layout=[
|
||||
[sg.Checkbox('Checkbox', size=(10,1)), sg.Checkbox('My second checkbox!', default=True)],
|
||||
[sg.Radio('My first Radio! ', "RADIO1", default=True, size=(10,1)), sg.Radio('My second Radio!', "RADIO1")]], title='Options',title_color='red', relief=sg.RELIEF_SUNKEN, tooltip='Use these to set flags')],
|
||||
[sg.Multiline(default_text='This is the default Text should you decide not to type anything', size=(35, 3)),
|
||||
sg.Multiline(default_text='A second multi-line', size=(35, 3))],
|
||||
[sg.InputCombo(('Combobox 1', 'Combobox 2'), size=(20, 1)),
|
||||
sg.Slider(range=(1, 100), orientation='h', size=(34, 20), default_value=85)],
|
||||
[sg.InputOptionMenu(('Menu Option 1', 'Menu Option 2', 'Menu Option 3'))],
|
||||
[sg.Listbox(values=('Listbox 1', 'Listbox 2', 'Listbox 3'), size=(30, 3)),
|
||||
sg.Frame('Labelled Group',[[
|
||||
sg.Slider(range=(1, 100), orientation='v', size=(5, 20), default_value=25, tick_interval=25),
|
||||
sg.Slider(range=(1, 100), orientation='v', size=(5, 20), default_value=75),
|
||||
sg.Slider(range=(1, 100), orientation='v', size=(5, 20), default_value=10),
|
||||
sg.Column(column1, background_color='lightblue')]])],
|
||||
[sg.Text('_' * 80)],
|
||||
[sg.Text('Choose A Folder', size=(35, 1))],
|
||||
[sg.Text('Your Folder', size=(15, 1), auto_size_text=False, justification='right'),
|
||||
sg.InputText('Default Folder'), sg.FolderBrowse()],
|
||||
[sg.Submit(tooltip='Click to submit this form'), sg.Cancel()]]
|
||||
|
||||
window = sg.Window('Everything bagel', default_element_size=(40, 1), grab_anywhere=False).Layout(layout)
|
||||
event, values = window.Read()
|
||||
|
||||
sg.Popup('Title',
|
||||
'The results of the window.',
|
||||
'The button clicked was "{}"'.format(event),
|
||||
'The values are', values)
|
||||
|
||||
|
6415
PySimpleGUIWeb/PySimpleGUIWeb.py
Normal file
6415
PySimpleGUIWeb/PySimpleGUIWeb.py
Normal file
File diff suppressed because it is too large
Load diff
65
PySimpleGUIWeb/readme.md
Normal file
65
PySimpleGUIWeb/readme.md
Normal file
|
@ -0,0 +1,65 @@
|
|||
|
||||
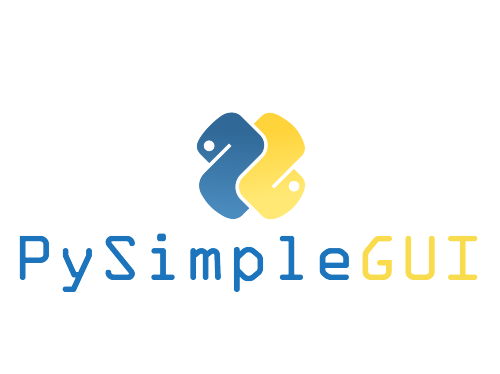
|
||||
|
||||
]
|
||||
|
||||
|
||||

|
||||
|
||||

|
||||
|
||||

|
||||
|
||||
|
||||
|
||||
# PySimpleGUIWeb
|
||||
|
||||
PySimpleGUI running in your web browser
|
||||
|
||||
## Primary PySimpleGUI Documentation
|
||||
|
||||
To get instructions on how use PySimpleGUI's APIs, please reference the [main documentation](http://www.PySimpleGUI.org).
|
||||
This Readme is for information ***specific to*** the Web port of PySimpleGUI.
|
||||
|
||||
|
||||
## What is PySimpleGUIWeb?
|
||||
|
||||
PySimpleGUIWeb enables you to run your PySimpleGUI programs in your web browser. It utilizes a package called Remi to achieve this amazing package.
|
||||
|
||||
At the moment (22-Jan-2019) the port has barely begun but it's far enough along to see that it's going to work. The Text, Input Text and Button elements are "functional". You can run simple PySimpleGUI programs and they actually WORK correctly.
|
||||
|
||||
## Engineering Pre-Release Version 0.1.0
|
||||
|
||||
[Announcements of Latest Developments](https://github.com/MikeTheWatchGuy/PySimpleGUI/issues/142)
|
||||
|
||||
Having trouble? Visit the [GitHub site ](http://www.PySimpleGUI.com) and log an Issue.
|
||||
|
||||
|
||||
## What Works
|
||||
|
||||
Text Element
|
||||
Input Text Element
|
||||
Button Element
|
||||
|
||||
Things like colors, fonts, etc, are not yet completed. This is SO early in the process, but it's exciting to see at the same time.
|
||||
|
||||
# Release Notes:
|
||||
|
||||
### 0.1.0 - 22-Jan-2019
|
||||
|
||||
* Initial release
|
||||
* Text Element
|
||||
* Input Text Element
|
||||
* Button Element
|
||||
* Window class
|
||||
|
||||
|
||||
# Design
|
||||
# Author
|
||||
Mike B.
|
||||
|
||||
|
||||
# License
|
||||
GNU Lesser General Public License (LGPL 3) +
|
||||
|
||||
# Acknowledgments
|
|
@ -1,7 +1,7 @@
|
|||
|
||||
|
||||
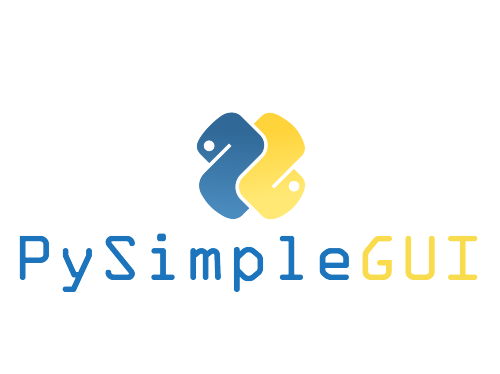
|
||||
|
||||
[](http://pepy.tech/project/pysimplegui)
|
||||
](http://pepy.tech/project/pysimplegui)
|
||||
|
||||
|
||||

|
||||
|
@ -12,38 +12,6 @@
|
|||
|
||||
|
||||
|
||||
# PySimpleGUIWx
|
||||
|
||||
The WxPython port of PySimpleGUI
|
||||
|
||||
## Why?
|
||||
|
||||
Why use PySimpleGUIWx?
|
||||
|
||||
There are a couple of easy reasons to use PySimpleGUIWx over PySimpleGUIQt. One is footprint. PyInstaller EXE for PySimpleGUIWx is 9 MB, on Qt it's 240 MB. Another is cool widgets. WxPython has some nice advanced widgets that will hopefully be offered thought PySimpleGUIWx.
|
||||
|
||||
Why use PySimpleGUI?
|
||||
1. It's simple and easy to program GUIs
|
||||
2. You can move between the GUI frameworks tkinter, Qt and WxPython by chaning a single line of code, the import statemlkent.
|
||||
3. It's fun
|
||||
|
||||
|
||||
|
||||
|
||||
## The Engineering Pre-
|
||||
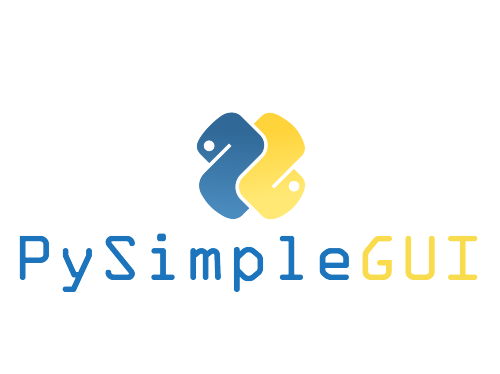
|
||||
|
||||
[](http://pepy.tech/project/pysimplegui)
|
||||
|
||||
|
||||

|
||||
|
||||

|
||||
|
||||

|
||||
|
||||
|
||||
|
||||
# PySimpleGUIWx
|
||||
|
||||
The WxPython port of PySimpleGUI
|
||||
|
@ -380,5 +348,5 @@ Starting with release 0.4.0, most of the Popup functions work. This means you c
|
|||
|
||||
# Acknowledgments
|
||||
<!--stackedit_data:
|
||||
eyJoaXN0b3J5IjpbNjg3MTU5NTA0XX0=
|
||||
eyJoaXN0b3J5IjpbODg2MzA1Mjk2XX0=
|
||||
-->
|
Loading…
Add table
Add a link
Reference in a new issue