Hacked up doc to make smaller (TEST ONLY!)
This commit is contained in:
parent
9814de9928
commit
c15cd4e39c
818
docs/index.md
818
docs/index.md
|
@ -2696,824 +2696,6 @@ layout = [[sg.T('Source Folder')],
|
|||
|
||||
See how much easier the key method is?
|
||||
|
||||
**Save & Open Buttons**
|
||||
|
||||
There are 4 different types of File/Folder open dialog box available. If you are looking for a file to open, the `FileBrowse` is what you want. If you want to save a file, `SaveAs` is the button. If you want to get a folder name, then `FolderBrowse` is the button to use. To open several files at once, use the `FilesBrowse` button. It will create a list of files that are separated by ';'
|
||||
|
||||
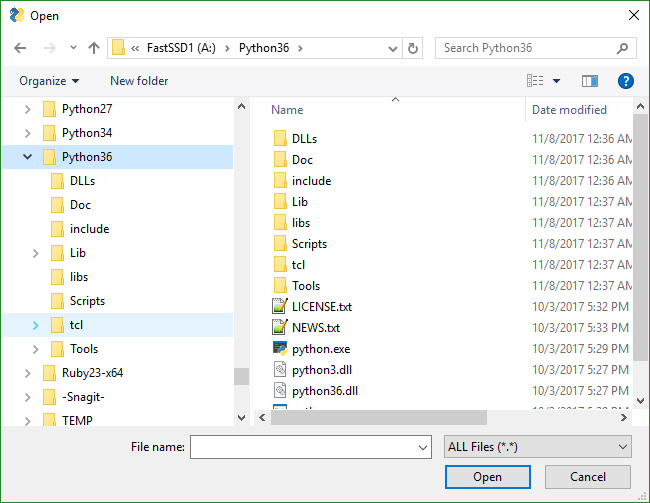
|
||||
|
||||
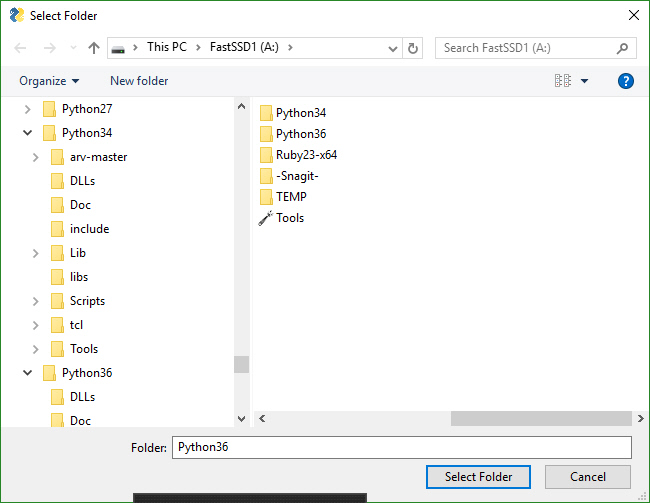
|
||||
|
||||
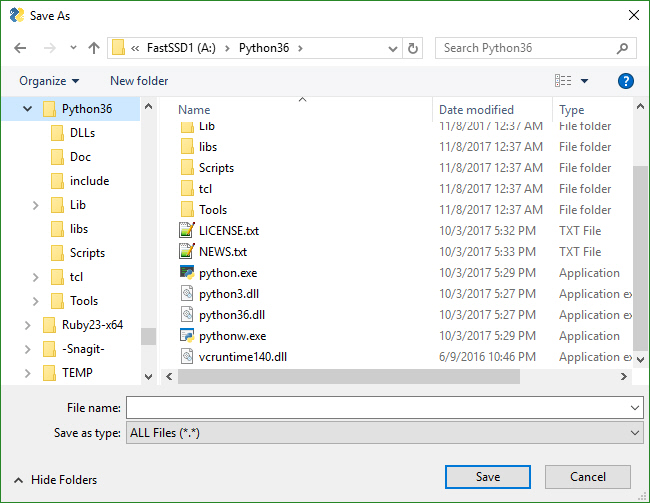
|
||||
|
||||
**Calendar Buttons**
|
||||
|
||||
These buttons pop up a calendar chooser window. The chosen date is returned as a string.
|
||||
|
||||
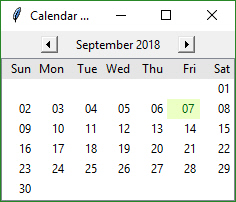
|
||||
|
||||
**Color Chooser Buttons**
|
||||
|
||||
These buttons pop up a standard color chooser window. The result is returned as a tuple. One of the returned values is an RGB hex representation.
|
||||
|
||||
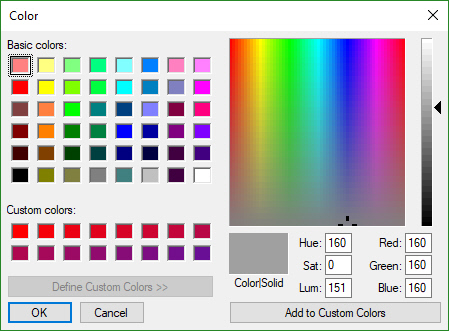
|
||||
|
||||
**Custom Buttons**
|
||||
Not all buttons are created equal. A button that closes a window is different that a button that returns from the window without closing it. If you want to define your own button, you will generally do this with the Button Element `Button`, which closes the window when clicked.
|
||||
|
||||
layout = [[sg.Button('My Button')]]
|
||||
|
||||
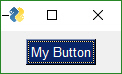
|
||||
|
||||
All buttons can have their text changed by changing the `button_text` variable in the button call. It is this text that is returned when a window is read. This text will be what tells you which button is called so make it unique. Most of the convenience buttons (Submit, Cancel, Yes, etc) are all Buttons. Some that are not are `FileBrowse` , `FolderBrowse`, `FileSaveAs`. They clearly do not close the window. Instead they bring up a file or folder browser dialog box.
|
||||
|
||||
**Button Images**
|
||||
Now this is an exciting feature not found in many simplified packages.... images on buttons! You can make a pretty spiffy user interface with the help of a few button images.
|
||||
|
||||
Your button images need to be in PNG or GIF format. When you make a button with an image, set the button background to the same color as the background. There's a button color TRANSPARENT_BUTTON that you can set your button color to in order for it to blend into the background. Note that this value is currently the same as the color as the default system background on Windows. If you want to set the button background color to the current system default, use the value COLOR_SYSTEM_DEFAULT as the background color.
|
||||
|
||||
This example comes from the `Demo Media Player.py` example program. Because it's a non-blocking button, it's defined as `RButton`. You also put images on blocking buttons by using `Button`.
|
||||
|
||||
sg.RButton('Restart Song', button_color=sg.TRANSPARENT_BUTTON,
|
||||
image_filename=image_restart, image_size=(50, 50), image_subsample=2, border_width=0)
|
||||
|
||||
Three parameters are used for button images.
|
||||
|
||||
image_filename - Filename. Can be a relative path
|
||||
image_size - Size of image file in pixels
|
||||
image_subsample - Amount to divide the size by. 2 means your image will be 1/2 the size. 3 means 1/3
|
||||
|
||||
Here's an example window made with button images.
|
||||
|
||||
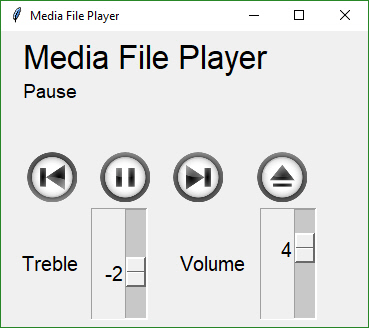
|
||||
|
||||
You'll find the source code in the file Demo Media Player. Here is what the button calls look like to create media player window
|
||||
```python
|
||||
sg.RButton('Pause', button_color=sg.TRANSPARENT_BUTTON,
|
||||
image_filename=image_pause,
|
||||
image_size=(50, 50),
|
||||
image_subsample=2,
|
||||
border_width=0)
|
||||
```
|
||||
This is one you'll have to experiment with at this point. Not up for an exhaustive explanation.
|
||||
|
||||
**Realtime Buttons**
|
||||
|
||||
Normally buttons are considered "clicked" when the mouse button is let UP after a downward click on the button. What about times when you need to read the raw up/down button values. A classic example for this is a robotic remote control. Building a remote control using a GUI is easy enough. One button for each of the directions is a start. Perhaps something like this:
|
||||
|
||||
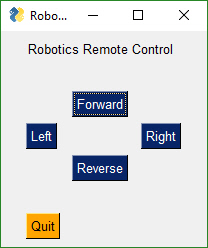
|
||||
|
||||
This window has 2 button types. There's the normal "Read Button" (Quit) and 4 "Realtime Buttons".
|
||||
|
||||
Here is the code to make, show and get results from this window:
|
||||
|
||||
```python
|
||||
import PySimpleGUI as sg
|
||||
|
||||
gui_rows = [[sg.Text('Robotics Remote Control')],
|
||||
[sg.T(' ' * 10), sg.RealtimeButton('Forward')],
|
||||
[sg.RealtimeButton('Left'), sg.T(' ' * 15), sg.RealtimeButton('Right')],
|
||||
[sg.T(' ' * 10), sg.RealtimeButton('Reverse')],
|
||||
[sg.T('')],
|
||||
[sg.Quit(button_color=('black', 'orange'))]
|
||||
]
|
||||
|
||||
window = sg.Window('Robotics Remote Control', auto_size_text=True).Layout(gui_rows)
|
||||
|
||||
#
|
||||
# Some place later in your code...
|
||||
# You need to perform a Read or Refresh call on your window every now and then or
|
||||
# else it will apprear as if the program has locked up.
|
||||
#
|
||||
# your program's main loop
|
||||
while (True):
|
||||
# This is the code that reads and updates your window
|
||||
event, values = window.Read(timeout=0)
|
||||
if event is not None:
|
||||
print(event)
|
||||
if event == 'Quit' or values is None:
|
||||
break
|
||||
|
||||
window.Close() # Don't forget to close your window!
|
||||
```
|
||||
|
||||
This loop will read button values and print them. When one of the Realtime buttons is clicked, the call to `window.Read` will return a button name matching the name on the button that was depressed or the key if there was a key assigned to the button. It will continue to return values as long as the button remains depressed. Once released, the Read will return timeout events until a button is again clicked.
|
||||
|
||||
**File Types**
|
||||
The `FileBrowse` & `SaveAs` buttons have an additional setting named `file_types`. This variable is used to filter the files shown in the file dialog box. The default value for this setting is
|
||||
|
||||
FileTypes=(("ALL Files", "*.*"),)
|
||||
|
||||
This code produces a window where the Browse button only shows files of type .TXT
|
||||
|
||||
layout = [[sg.In() ,sg.FileBrowse(file_types=(("Text Files", "*.txt"),))]]
|
||||
|
||||
NOTE - Mac users will not be able to use the file_types parameter. tkinter has a bug on Macs that will crash the program is a file_type is attempted so that feature had to be removed. Sorry about that!
|
||||
|
||||
***The ENTER key***
|
||||
The ENTER key is an important part of data entry for windows. There's a long tradition of the enter key being used to quickly submit windows. PySimpleGUI implements this by tying the ENTER key to the first button that closes or reads a window.
|
||||
|
||||
The Enter Key can be "bound" to a particular button so that when the key is pressed, it causes the window to return as if the button was clicked. This is done using the `bind_return_key` parameter in the button calls.
|
||||
If there are more than 1 button on a window, the FIRST button that is of type Close window or Read window is used. First is determined by scanning the window, top to bottom and left to right.
|
||||
|
||||
### Methods
|
||||
|
||||
```python
|
||||
Update(text=None,
|
||||
button_color=(None, None),
|
||||
disabled=None,
|
||||
image_data=None,
|
||||
image_filename=None,
|
||||
visible=None,
|
||||
image_subsample=None,
|
||||
image_size=None)
|
||||
```
|
||||
Parameters explained:
|
||||
|
||||
|Name|Meaning|
|
||||
|---|---|
|
||||
|text|(Default value = None)|
|
||||
|button_color|(Default value = (None)|
|
||||
|disabled|disable or enable state of the element (Default value = None)|
|
||||
|image_data|(Default value = None)|
|
||||
|image_filename|(Default value = None)|
|
||||
|visible|change visibility of element (Default value = None)|
|
||||
|image_subsample|(Default value = None)|
|
||||
|image_size|(Default value = None)|
|
||||
|||
|
||||
|
||||
GetText - Returns the current text shown on a button
|
||||
|
||||
GetText() - method
|
||||
|
||||
|
||||
|
||||
## ButtonMenu Element
|
||||
|
||||
The ButtonMenu element produces a unique kind of effect. It's a button, that when clicked, shows you a menu. It's like clicking one of the top-level menu items on a MenuBar. As a result, the menu definition take the format of a single menu entry from a normal menu definition. A normal menu definition is a list of lists. This definition is one of those lists.
|
||||
|
||||
Here is a sample definition:
|
||||
```python
|
||||
['Menu', ['&Pause Graph', 'Menu item::optional_key']]
|
||||
```
|
||||
The very first string normally specifies what is shown on the menu bar. In this case, the value is **not used**. You set the text for the button using a different parameter, the `button_text` parm.
|
||||
|
||||
|
||||
|
||||
```python
|
||||
ButtonMenu(button_text,
|
||||
menu_def,
|
||||
tooltip=None,
|
||||
disabled=False,
|
||||
image_filename=None,
|
||||
image_data=None,
|
||||
image_size=(None, None),
|
||||
image_subsample=None,
|
||||
border_width=None,
|
||||
size=(None, None),
|
||||
auto_size_button=None,
|
||||
button_color=None,
|
||||
font=None,
|
||||
pad=None,
|
||||
key=None,
|
||||
tearoff=False,
|
||||
visible=True)
|
||||
```
|
||||
Parameters explained:
|
||||
|
||||
|Name|Meaning|
|
||||
|---|---|
|
||||
|button_text||
|
||||
|menu_def||
|
||||
|tooltip|text, that will appear the you hover on (Default value = None)|
|
||||
|disabled|set disable state for element (Default value = False)|
|
||||
|image_filename|(Default value = None)|
|
||||
|image_data|(Default value = None)|
|
||||
|image_size|(Default value = (None, None))|
|
||||
|image_subsample|(Default value = None)|
|
||||
|border_width|(Default value = None)|
|
||||
|size|(common_key) (w,h) w=characters-wide, h=rows-high (Default value = (None)|
|
||||
|auto_size_button|(Default value = None)|
|
||||
|button_color|(Default value = None)|
|
||||
|font|(common_key) specifies the font family, size, etc (Default value = None)|
|
||||
|pad|(common_key) Amount of padding to put around element (Default value = None)|
|
||||
|key|(common_key) Used with window.FindElement and with return values (Default value = None)|
|
||||
|tearoff|(Default value = False)|
|
||||
|visible|set visibility state of the element (Default value = True)|
|
||||
|||
|
||||
|
||||
One use of this element is to make a "fake menu bar" that has a colored background. Normal menu bars cannot have their background color changed. Not so with ButtonMenus.
|
||||
|
||||
This is the effect:
|
||||
|
||||
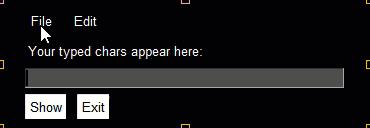
|
||||
|
||||
Return values for ButtonMenus are sent via the return values dictionary. If a selection is made, then an event is generated that will equal the ButtonMenu's key value. Use that key value to look up the value selected by the user. This is the same mechanism as the Menu Bar Element, but differs from the pop-up (right click) menu.
|
||||
|
||||
|
||||
|
||||
## VerticalSeparator Element
|
||||
This element has limited usefulness and is being included more for completeness than anything else. It will draw a line between elements.
|
||||
|
||||
It works best when placed between columns or elements that span multiple rows. If on a "normal" row with elements that are only 1 row high, then it will only span that one row.
|
||||
|
||||
|
||||
|
||||
```python
|
||||
VerticalSeparator(pad=None)
|
||||
```
|
||||
|
||||
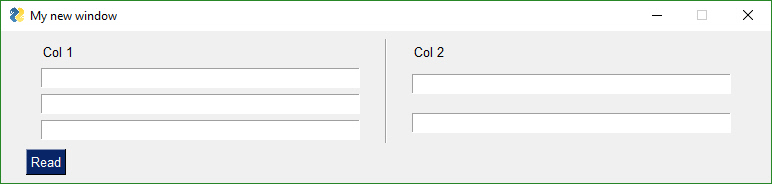
|
||||
|
||||
```python
|
||||
VerticalSeparator(pad=None)
|
||||
```
|
||||
Parameters explained:
|
||||
|
||||
|Name|Meaning|
|
||||
|---|---|
|
||||
|pad|(common_key) Amount of padding to put around element (Default value = None)|
|
||||
|||
|
||||
|
||||
## ProgressBar Element
|
||||
The `ProgressBar` element is used to build custom Progress Bar windows. It is HIGHLY recommended that you use OneLineProgressMeter that provides a complete progress meter solution for you. Progress Meters are not easy to work with because the windows have to be non-blocking and they are tricky to debug.
|
||||
|
||||
The **easiest** way to get progress meters into your code is to use the `OneLineProgressMeter` API. This consists of a pair of functions, `OneLineProgressMeter` and `OneLineProgressMeterCancel`. You can easily cancel any progress meter by calling it with the current value = max value. This will mark the meter as expired and close the window.
|
||||
You've already seen OneLineProgressMeter calls presented earlier in this readme.
|
||||
|
||||
```python
|
||||
sg.OneLineProgressMeter('My Meter', i+1, 1000, 'key', 'Optional message')
|
||||
```
|
||||
|
||||
The return value for `OneLineProgressMeter` is:
|
||||
`True` if meter updated correctly
|
||||
`False` if user clicked the Cancel button, closed the window, or vale reached the max value.
|
||||
|
||||
#### Progress Mater in Your window
|
||||
Another way of using a Progress Meter with PySimpleGUI is to build a custom window with a `ProgressBar` Element in the window. You will need to run your window as a non-blocking window. When you are ready to update your progress bar, you call the `UpdateBar` method for the `ProgressBar` element itself.
|
||||
|
||||
|
||||
|
||||
```python
|
||||
import PySimpleGUI as sg
|
||||
|
||||
# layout the window
|
||||
layout = [[sg.Text('A custom progress meter')],
|
||||
[sg.ProgressBar(10000, orientation='h', size=(20, 20), key='progressbar')],
|
||||
[sg.Cancel()]]
|
||||
|
||||
# create the window`
|
||||
window = sg.Window('Custom Progress Meter').Layout(layout)
|
||||
progress_bar = window.FindElement('progressbar')
|
||||
# loop that would normally do something useful
|
||||
for i in range(10000):
|
||||
# check to see if the cancel button was clicked and exit loop if clicked
|
||||
event, values = window.Read(timeout=0)
|
||||
if event == 'Cancel' or event is None:
|
||||
break
|
||||
# update bar with loop value +1 so that bar eventually reaches the maximum
|
||||
progress_bar.UpdateBar(i + 1)
|
||||
# done with loop... need to destroy the window as it's still open
|
||||
window.Close())
|
||||
```
|
||||
|
||||
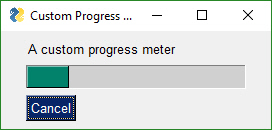
|
||||
|
||||
```python
|
||||
ProgressBar(max_value,
|
||||
orientation=None,
|
||||
size=(None, None),
|
||||
auto_size_text=None,
|
||||
bar_color=(None, None),
|
||||
style=None,
|
||||
border_width=None,
|
||||
relief=None,
|
||||
key=None,
|
||||
pad=None,
|
||||
visible=True)
|
||||
```
|
||||
Parameters explained:
|
||||
|
||||
|Name|Meaning|
|
||||
|---|---|
|
||||
|max_value||
|
||||
|orientation|(Default value = None)|
|
||||
|size|(common_key) (w,h) w=characters-wide, h=rows-high (Default value = (None, None))|
|
||||
|auto_size_text|True if size should fit the text length (Default value = None)|
|
||||
|bar_color|(Default value = (None)|
|
||||
|style|(Default value = None)|
|
||||
|border_width|(Default value = None)|
|
||||
|relief|(Default value = None)|
|
||||
|key|(common_key) Used with window.FindElement and with return values (Default value = None)|
|
||||
|pad|(common_key) Amount of padding to put around element (Default value = None)|
|
||||
|visible|set visibility state of the element (Default value = True)|
|
||||
|||
|
||||
|
||||
|
||||
|
||||
|
||||
## Output Elements
|
||||
The Output Element is a re-direction of Stdout. Anything "printed" will be displayed in this element.
|
||||
|
||||
Note that you will NOT see what you `print` until you call either window.Read or window.Refresh. If you want to immediately see what was printed, call window.Refresh() immediately after your print statement.
|
||||
|
||||
|
||||
|
||||
```python
|
||||
Output(size=(None, None))
|
||||
```
|
||||
|
||||
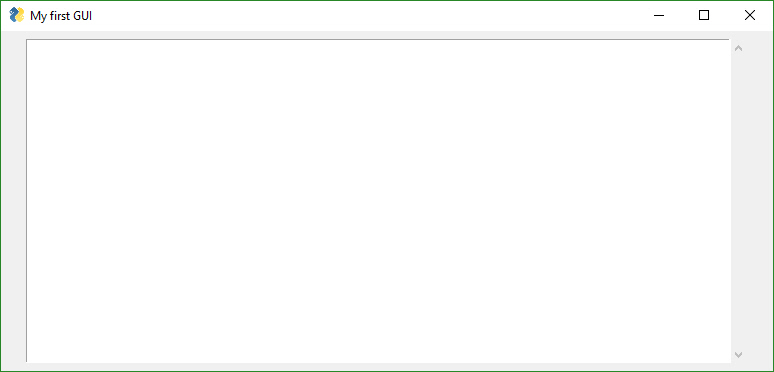
|
||||
|
||||
```python
|
||||
Output(size=(None, None),
|
||||
background_color=None,
|
||||
text_color=None,
|
||||
pad=None,
|
||||
font=None,
|
||||
tooltip=None,
|
||||
key=None,
|
||||
right_click_menu=None,
|
||||
visible=True)
|
||||
```
|
||||
Parameters explained:
|
||||
|
||||
|Name|Meaning|
|
||||
|---|---|
|
||||
|size|(common_key) (w,h) w=characters-wide, h=rows-high (Default value = (None, None))|
|
||||
|background_color|color of background (Default value = None)|
|
||||
|text_color|color of the text (Default value = None)|
|
||||
|pad|(common_key) Amount of padding to put around element (Default value = None)|
|
||||
|font|(common_key) specifies the font family, size, etc (Default value = None)|
|
||||
|tooltip|text, that will appear the you hover on (Default value = None)|
|
||||
|key|(common_key) Used with window.FindElement and with return values (Default value = None)|
|
||||
|right_click_menu|see "Right Click Menus" (Default value = None)|
|
||||
|visible|set visibility state of the element (Default value = True)|
|
||||
|||
|
||||
|
||||
### Methods
|
||||
|
||||
```python
|
||||
Update(value=None,
|
||||
visible=None)
|
||||
```
|
||||
Parameters explained:
|
||||
|
||||
|Name|Meaning|
|
||||
|---|---|
|
||||
|value|(Default value = None)|
|
||||
|visible|change visibility of element (Default value = None)|
|
||||
|||
|
||||
|
||||
----
|
||||
|
||||
UpdateBar - ?
|
||||
|
||||
----
|
||||
|
||||
Here's a complete solution for a chat-window using an Async window with an Output Element
|
||||
|
||||
```python
|
||||
import PySimpleGUI as sg
|
||||
|
||||
# Blocking window that doesn't close
|
||||
def ChatBot():
|
||||
layout = [[(sg.Text('This is where standard out is being routed', size=[40, 1]))],
|
||||
[sg.Output(size=(80, 20))],
|
||||
[sg.Multiline(size=(70, 5), enter_submits=True),
|
||||
sg.RButton('SEND', button_color=(sg.YELLOWS[0], sg.BLUES[0])),
|
||||
sg.Button('EXIT', button_color=(sg.YELLOWS[0], sg.GREENS[0]))]]
|
||||
|
||||
window = sg.Window('Chat Window', default_element_size=(30, 2)).Layout(layout)
|
||||
|
||||
# ---===--- Loop taking in user input and using it to query HowDoI web oracle --- #
|
||||
while True:
|
||||
event, value = window.Read()
|
||||
if event == 'SEND':
|
||||
print(value)
|
||||
else:
|
||||
break
|
||||
|
||||
ChatBot()
|
||||
```
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
## Column Element
|
||||
Starting in version 2.9 you'll be able to do more complex layouts by using the Column Element. Think of a Column as a window within a window. And, yes, you can have a Column within a Column if you want.
|
||||
|
||||
Columns are specified in exactly the same way as a window is, as a list of lists.
|
||||
|
||||
Columns are needed when you have an element that has a height > 1 line on the left, with single-line elements on the right. Here's an example of this kind of layout:
|
||||
|
||||
|
||||
|
||||
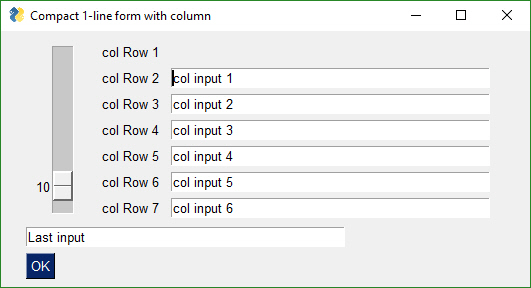
|
||||
|
||||
The Column Element has 1 required parameter and 1 optional (the layout and the background color). Setting the background color has the same effect as setting the window's background color, except it only affects the column rectangle.
|
||||
|
||||
```python
|
||||
Column(layout,
|
||||
background_color=None,
|
||||
size=(None, None),
|
||||
pad=None,
|
||||
scrollable=False,
|
||||
vertical_scroll_only=False,
|
||||
right_click_menu=None,
|
||||
key=None,
|
||||
visible=True)
|
||||
```
|
||||
Parameters explained:
|
||||
|
||||
|Name|Meaning|
|
||||
|---|---|
|
||||
|layout||
|
||||
|background_color|color of background (Default value = None)|
|
||||
|size|(common_key) (w,h) w=characters-wide, h=rows-high (Default value = (None, None))|
|
||||
|pad|(common_key) Amount of padding to put around element (Default value = None)|
|
||||
|scrollable|(Default value = False)|
|
||||
|vertical_scroll_only|(Default value = False)|
|
||||
|right_click_menu|see "Right Click Menus" (Default value = None)|
|
||||
|key|(common_key) Used with window.FindElement and with return values (Default value = None)|
|
||||
|visible|set visibility state of the element (Default value = True)|
|
||||
|||
|
||||
|
||||
---
|
||||
|
||||
```python
|
||||
|
||||
import PySimpleGUI as sg
|
||||
|
||||
# Demo of how columns work
|
||||
# window has on row 1 a vertical slider followed by a COLUMN with 7 rows
|
||||
# Prior to the Column element, this layout was not possible
|
||||
# Columns layouts look identical to window layouts, they are a list of lists of elements.
|
||||
|
||||
window = sg.Window('Columns') # blank window
|
||||
|
||||
# Column layout
|
||||
col = [[sg.Text('col Row 1')],
|
||||
[sg.Text('col Row 2'), sg.Input('col input 1')],
|
||||
[sg.Text('col Row 3'), sg.Input('col input 2')],
|
||||
[sg.Text('col Row 4'), sg.Input('col input 3')],
|
||||
[sg.Text('col Row 5'), sg.Input('col input 4')],
|
||||
[sg.Text('col Row 6'), sg.Input('col input 5')],
|
||||
[sg.Text('col Row 7'), sg.Input('col input 6')]]
|
||||
|
||||
layout = [[sg.Slider(range=(1,100), default_value=10, orientation='v', size=(8,20)), sg.Column(col)],
|
||||
[sg.In('Last input')],
|
||||
[sg.OK()]]
|
||||
|
||||
# Display the window and get values
|
||||
# If you're willing to not use the "context manager" design pattern, then it's possible
|
||||
# to collapse the window display and read down to a single line of code.
|
||||
event, values = sg.Window('Compact 1-line window with column').Layout(layout).Read()
|
||||
|
||||
sg.Popup(event, values, line_width=200)
|
||||
```
|
||||
|
||||
----
|
||||
|
||||
## Frame Element (Labelled Frames, Frames with a title)
|
||||
|
||||
Frames work exactly the same way as Columns. You create layout that is then used to initialize the Frame.
|
||||
|
||||
|
||||
|
||||
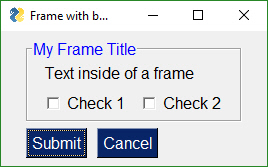
|
||||
|
||||
Notice how the Frame layout looks identical to a window layout. A window works exactly the same way as a Column and a Frame. They all are "container elements" - elements that contain other elements.
|
||||
|
||||
*These container Elements can be nested as deep as you want.* That's a pretty spiffy feature, right? Took a lot of work so be appreciative. Recursive code isn't trivial.
|
||||
|
||||
```python
|
||||
Frame(title,
|
||||
layout,
|
||||
title_color=None,
|
||||
background_color=None,
|
||||
title_location=None,
|
||||
relief="groove",
|
||||
size=(None, None),
|
||||
font=None,
|
||||
pad=None,
|
||||
border_width=None,
|
||||
key=None,
|
||||
tooltip=None,
|
||||
right_click_menu=None,
|
||||
visible=True)
|
||||
```
|
||||
Parameters explained:
|
||||
|
||||
|Name|Meaning|
|
||||
|---|---|
|
||||
|title||
|
||||
|layout||
|
||||
|title_color|(Default value = None)|
|
||||
|background_color|color of background (Default value = None)|
|
||||
|title_location|(Default value = None)|
|
||||
|relief|(Default value = DEFAULT_FRAME_RELIEF)|
|
||||
|size|(common_key) (w,h) w=characters-wide, h=rows-high (Default value = (None, None))|
|
||||
|font|(common_key) specifies the font family, size, etc (Default value = None)|
|
||||
|pad|(common_key) Amount of padding to put around element (Default value = None)|
|
||||
|border_width|(Default value = None)|
|
||||
|key|(common_key) Used with window.FindElement and with return values (Default value = None)|
|
||||
|tooltip|text, that will appear the you hover on (Default value = None)|
|
||||
|right_click_menu|see "Right Click Menus" (Default value = None)|
|
||||
|visible|set visibility state of the element (Default value = True)|
|
||||
|||
|
||||
|
||||
---
|
||||
|
||||
This code creates a window with a Frame and 2 buttons.
|
||||
|
||||
```python
|
||||
frame_layout = [
|
||||
[sg.T('Text inside of a frame')],
|
||||
[sg.CB('Check 1'), sg.CB('Check 2')],
|
||||
]
|
||||
layout = [
|
||||
[sg.Frame('My Frame Title', frame_layout, font='Any 12', title_color='blue')],
|
||||
[sg.Submit(), sg.Cancel()]
|
||||
]
|
||||
|
||||
window = sg.Window('Frame with buttons', font=("Helvetica", 12)).Layout(layout)
|
||||
```
|
||||
|
||||
|
||||
## Canvas Element
|
||||
|
||||
In my opinion, the tkinter Canvas Widget is the most powerful of the tkinter widget. While I try my best to completely isolate the user from anything that is tkinter related, the Canvas Element is the one exception. It enables integration with a number of other packages, often with spectacular results.
|
||||
|
||||
|
||||
|
||||
```python
|
||||
Canvas(canvas=None,
|
||||
background_color=None,
|
||||
size=(None, None),
|
||||
pad=None,
|
||||
key=None,
|
||||
tooltip=None,
|
||||
right_click_menu=None,
|
||||
visible=True)
|
||||
```
|
||||
Parameters explained:
|
||||
|
||||
|Name|Meaning|
|
||||
|---|---|
|
||||
|canvas|(Default value = None)|
|
||||
|background_color|color of background (Default value = None)|
|
||||
|size|(common_key) (w,h) w=characters-wide, h=rows-high (Default value = (None, None))|
|
||||
|pad|(common_key) Amount of padding to put around element (Default value = None)|
|
||||
|key|(common_key) Used with window.FindElement and with return values (Default value = None)|
|
||||
|tooltip|text, that will appear the you hover on (Default value = None)|
|
||||
|right_click_menu|see "Right Click Menus" (Default value = None)|
|
||||
|visible|set visibility state of the element (Default value = True)|
|
||||
|||
|
||||
|
||||
### Matplotlib, Pyplot Integration
|
||||
|
||||
One such integration is with Matploplib and Pyplot. There is a Demo program written that you can use as a design pattern to get an understanding of how to use the Canvas Widget once you get it.
|
||||
|
||||
def Canvas(canvas - a tkinter canvasf if you created one. Normally not set
|
||||
background_color - canvas color
|
||||
size - size in pixels
|
||||
pad - element padding for packing
|
||||
key - key used to lookup element
|
||||
tooltip - tooltip text
|
||||
|
||||
The order of operations to obtain a tkinter Canvas Widget is:
|
||||
|
||||
figure_x, figure_y, figure_w, figure_h = fig.bbox.bounds
|
||||
# define the window layout
|
||||
layout = [[sg.Text('Plot test')],
|
||||
[sg.Canvas(size=(figure_w, figure_h), key='canvas')],
|
||||
[sg.OK(pad=((figure_w / 2, 0), 3), size=(4, 2))]]
|
||||
|
||||
# create the window and show it without the plot
|
||||
window = sg.Window('Demo Application - Embedding Matplotlib In PySimpleGUI').Layout(layout).Finalize()
|
||||
|
||||
# add the plot to the window
|
||||
fig_photo = draw_figure(window.FindElement('canvas').TKCanvas, fig)
|
||||
|
||||
# show it all again and get buttons
|
||||
event, values = window.Read()
|
||||
|
||||
To get a tkinter Canvas Widget from PySimpleGUI, follow these steps:
|
||||
* Add Canvas Element to your window
|
||||
* Layout your window
|
||||
* Call `window.Finalize()` - this is a critical step you must not forget
|
||||
* Find the Canvas Element by looking up using key
|
||||
* Your Canvas Widget Object will be the found_element.TKCanvas
|
||||
* Draw on your canvas to your heart's content
|
||||
* Call `window.Read()` - Nothing will appear on your canvas until you call Read
|
||||
|
||||
See `Demo_Matplotlib.py` for a Recipe you can copy.
|
||||
|
||||
### Methods
|
||||
|
||||
TKCanvas - not a method but a property. Returns the tkinter Canvas Widget
|
||||
|
||||
|
||||
## Graph Element
|
||||
|
||||
All you math fans will enjoy this Element... and all you non-math fans will enjoy it too.
|
||||
|
||||
I've found nothing to be less fun than dealing with a graphic's coordinate system from a GUI Framework. It's always upside down from what I want. (0,0) is in the upper left hand corner. In short, it's a **pain in the ass**.
|
||||
|
||||
Graph Element to the rescue. A Graph Element creates a pixel addressable canvas using YOUR coordinate system. *You* get to define the units on the X and Y axis.
|
||||
|
||||
There are 3 values you'll need to supply the Graph Element. They are:
|
||||
* Size of the canvas in pixels
|
||||
* The lower left (x,y) coordinate of your coordinate system
|
||||
* The upper right (x,y) coordinate of your coordinate system
|
||||
|
||||
After you supply those values you can scribble all of over your graph by creating Graph Figures. Graph Figures are created, and a Figure ID is obtained by calling:
|
||||
* DrawCircle
|
||||
* DrawLine
|
||||
* DrawPoint
|
||||
* DrawRectangle
|
||||
* DrawOval
|
||||
* DrawImage
|
||||
|
||||
You can move your figures around on the canvas by supplying the Figure ID the x,y amount to move.
|
||||
|
||||
graph.MoveFigure(my_circle, 10, 10)
|
||||
|
||||
This Element is relatively new and may have some parameter additions or deletions. It shouldn't break your code however.
|
||||
|
||||
|
||||
|
||||
```python
|
||||
Graph(canvas_size,
|
||||
graph_bottom_left,
|
||||
graph_top_right,
|
||||
background_color=None,
|
||||
pad=None,
|
||||
change_submits=False,
|
||||
drag_submits=False,
|
||||
enable_events=False,
|
||||
key=None,
|
||||
tooltip=None,
|
||||
right_click_menu=None,
|
||||
visible=True,
|
||||
float_values=False)
|
||||
```
|
||||
Parameters explained:
|
||||
|
||||
|Name|Meaning|
|
||||
|---|---|
|
||||
|canvas_size||
|
||||
|graph_bottom_left||
|
||||
|graph_top_right||
|
||||
|background_color|color of background (Default value = None)|
|
||||
|pad|(common_key) Amount of padding to put around element (Default value = None)|
|
||||
|change_submits|If True, pressing Enter key submits window (Default value = False)|
|
||||
|drag_submits|(Default value = False)|
|
||||
|enable_events|Turns on the element specific events.(Default value = False)|
|
||||
|key|(common_key) Used with window.FindElement and with return values (Default value = None)|
|
||||
|tooltip|text, that will appear the you hover on (Default value = None)|
|
||||
|right_click_menu|see "Right Click Menus" (Default value = None)|
|
||||
|visible|set visibility state of the element (Default value = True)|
|
||||
|float_values|bool: If True x,y coordinates are returned as floats, not ints|
|
||||
|||
|
||||
|
||||
### Methods
|
||||
|
||||
`DrawLine` - draws a point, line, circle, oval, arc, rectangle, text
|
||||
|
||||
`DrawImage` - places an image onto the graph
|
||||
|
||||
`Erase` - erases entire graph
|
||||
|
||||
`Update` - changes background color
|
||||
|
||||
`Move` - moves everything an x,y direction
|
||||
|
||||
`MoveFigure` - moves an individual figure by some delta
|
||||
|
||||
`RelocateFigure` - moves figure to an absolute location
|
||||
|
||||
`DeleteFigure` - delete an individual figure
|
||||
|
||||
All of the Drawing methods return a "***figure***" that can be used move and delete the figure
|
||||
|
||||
```python
|
||||
DrawLine(point_from,
|
||||
point_to,
|
||||
color="black",
|
||||
width=1)
|
||||
```
|
||||
Parameters explained:
|
||||
|
||||
|Name|Meaning|
|
||||
|---|---|
|
||||
|point_from||
|
||||
|point_to||
|
||||
|color|(Default value = 'black')|
|
||||
|width|(Default value = 1)|
|
||||
|||
|
||||
|
||||
```python
|
||||
DrawPoint(point,
|
||||
size=2,
|
||||
color="black")
|
||||
```
|
||||
Parameters explained:
|
||||
|
||||
|Name|Meaning|
|
||||
|---|---|
|
||||
|point||
|
||||
|size|(common_key) (w,h) w=characters-wide, h=rows-high (Default value = 2)|
|
||||
|color|(Default value = 'black')|
|
||||
|||
|
||||
|
||||
```python
|
||||
DrawCircle(center_location,
|
||||
radius,
|
||||
fill_color=None,
|
||||
line_color="black")
|
||||
```
|
||||
Parameters explained:
|
||||
|
||||
|Name|Meaning|
|
||||
|---|---|
|
||||
|center_location||
|
||||
|radius||
|
||||
|fill_color|(Default value = None)|
|
||||
|line_color|(Default value = 'black')|
|
||||
|||
|
||||
|
||||
```python
|
||||
DrawOval(top_left,
|
||||
bottom_right,
|
||||
fill_color=None,
|
||||
line_color=None)
|
||||
```
|
||||
Parameters explained:
|
||||
|
||||
|Name|Meaning|
|
||||
|---|---|
|
||||
|top_left||
|
||||
|bottom_right||
|
||||
|fill_color|(Default value = None)|
|
||||
|line_color|(Default value = None)|
|
||||
|||
|
||||
|
||||
```python
|
||||
DrawArc(top_left,
|
||||
bottom_right,
|
||||
extent,
|
||||
start_angle,
|
||||
style=None,
|
||||
arc_color="black")
|
||||
```
|
||||
Parameters explained:
|
||||
|
||||
|Name|Meaning|
|
||||
|---|---|
|
||||
|top_left||
|
||||
|bottom_right||
|
||||
|extent||
|
||||
|start_angle||
|
||||
|style|(Default value = None)|
|
||||
|arc_color|(Default value = 'black')|
|
||||
|||
|
||||
|
||||
```python
|
||||
DrawRectangle(top_left,
|
||||
bottom_right,
|
||||
fill_color=None,
|
||||
line_color=None)
|
||||
```
|
||||
Parameters explained:
|
||||
|
||||
|Name|Meaning|
|
||||
|---|---|
|
||||
|top_left||
|
||||
|bottom_right||
|
||||
|fill_color|(Default value = None)|
|
||||
|line_color|(Default value = None)|
|
||||
|||
|
||||
|
||||
```python
|
||||
DrawText(text,
|
||||
location,
|
||||
color="black",
|
||||
font=None,
|
||||
angle=0)
|
||||
```
|
||||
Parameters explained:
|
||||
|
||||
|Name|Meaning|
|
||||
|---|---|
|
||||
|text||
|
||||
|location||
|
||||
|color|(Default value = 'black')|
|
||||
|font|(common_key) specifies the font family, size, etc (Default value = None)|
|
||||
|angle|(Default value = 0)|
|
||||
|||
|
||||
|
||||
Erase() - method
|
||||
|
||||
|
|
Loading…
Reference in New Issue