commit
bc2567130e
15
Demo_Tabs.py
15
Demo_Tabs.py
|
@ -1,17 +1,12 @@
|
|||
import PySimpleGUI as sg
|
||||
|
||||
tab1_layout = [
|
||||
[sg.T('This is inside tab 1')],
|
||||
]
|
||||
tab1_layout = [[sg.T('This is inside tab 1')]]
|
||||
|
||||
tab2_layout = [
|
||||
[sg.T('This is inside tab 2'), sg.In(key='in')],
|
||||
]
|
||||
tab2_layout = [[sg.T('This is inside tab 2')],
|
||||
[sg.In(key='in')]]
|
||||
|
||||
layout = [
|
||||
[sg.TabGroup([[sg.Tab('Tab 1', tab1_layout), sg.Tab('Tab 2', tab2_layout)]])],
|
||||
[sg.RButton('Read')]
|
||||
]
|
||||
layout = [[sg.TabGroup([[sg.Tab('Tab 1', tab1_layout, tooltip='tip'), sg.Tab('Tab 2', tab2_layout)]], tooltip='TIP2')],
|
||||
[sg.RButton('Read')]]
|
||||
|
||||
window = sg.Window('My window with tabs', default_element_size=(12,1)).Layout(layout)
|
||||
|
||||
|
|
|
@ -1,29 +1,35 @@
|
|||
import PySimpleGUI as sg
|
||||
|
||||
tab1_layout = [[sg.T('This is inside tab 1')],
|
||||
sg.ChangeLookAndFeel('GreenTan')
|
||||
tab2_layout = [[sg.T('This is inside tab 2')],
|
||||
[sg.T('Tabs can be anywhere now!')]
|
||||
]
|
||||
|
||||
tab2_layout = [[sg.T('This is inside tab 2'), sg.In(key='in')]]
|
||||
tab1_layout = [[sg.T('Type something here and click button'), sg.In(key='in')]]
|
||||
|
||||
tab3_layout = [[sg.T('This is inside tab 3')]]
|
||||
tab4_layout = [[sg.T('This is inside tab 4')]]
|
||||
|
||||
tab5_layout = [[sg.T('This is inside tab 5')]]
|
||||
tab_layout = [[sg.T('This is inside of a tab')]]
|
||||
tab_group = sg.TabGroup([[sg.Tab('Tab 7', tab_layout), sg.Tab('Tab 8', tab_layout)]])
|
||||
|
||||
tab5_layout = [[sg.T('Watch this window')],
|
||||
[sg.Output(size=(40,5))]]
|
||||
tab6_layout = [[sg.T('This is inside tab 6')],
|
||||
[sg.T('How about a second row of stuff in tab 6?')]]
|
||||
[sg.T('How about a second row of stuff in tab 6?'), tab_group]]
|
||||
|
||||
layout = [[sg.T('My Window!')], [sg.Frame('A Frame', layout=
|
||||
[[sg.TabGroup([[sg.Tab('Tab 1', tab1_layout), sg.Tab('Tab 2', tab2_layout)]]), sg.TabGroup([[sg.Tab('Inside of a frame?', tab3_layout), sg.Tab('Tab 4', tab4_layout)]])]])],
|
||||
[[sg.TabGroup([[sg.Tab('Tab 1', tab1_layout), sg.Tab('Tab 2', tab2_layout)]]), sg.TabGroup([[sg.Tab('Tab3', tab3_layout), sg.Tab('Tab 4', tab4_layout)]])]])],
|
||||
[sg.T('This text is on a row with a column'),sg.Column(layout=[[sg.T('In a column')],
|
||||
[sg.TabGroup([[sg.Tab('Tab 5', tab5_layout), sg.Tab('Tab 6', tab6_layout)]])],
|
||||
[sg.RButton('Read')]])],
|
||||
[sg.RButton('Click me')]])],
|
||||
]
|
||||
|
||||
window = sg.Window('My window with tabs', default_element_size=(12,1)).Layout(layout)
|
||||
window = sg.Window('My window with tabs', default_element_size=(12,1)).Layout(layout).Finalize()
|
||||
|
||||
print('Are there enough tabs for you?')
|
||||
|
||||
while True:
|
||||
b, v = window.Read()
|
||||
print(b,v)
|
||||
if b is None: # always, always give a way out!
|
||||
button, values = window.Read()
|
||||
print(button,values)
|
||||
if button is None: # always, always give a way out!
|
||||
break
|
|
@ -195,7 +195,7 @@ ELEM_TYPE_CANVAS = 40
|
|||
ELEM_TYPE_FRAME = 41
|
||||
ELEM_TYPE_GRAPH = 42
|
||||
ELEM_TYPE_TAB = 50
|
||||
ELEM_TYPE_MULTI_TAB = 51
|
||||
ELEM_TYPE_TAB_GROUP = 51
|
||||
ELEM_TYPE_INPUT_SLIDER = 10
|
||||
ELEM_TYPE_INPUT_LISTBOX = 11
|
||||
ELEM_TYPE_OUTPUT = 300
|
||||
|
@ -318,7 +318,7 @@ class Element():
|
|||
rc = self.FindReturnKeyBoundButton(element)
|
||||
if rc is not None:
|
||||
return rc
|
||||
if element.Type == ELEM_TYPE_MULTI_TAB:
|
||||
if element.Type == ELEM_TYPE_TAB_GROUP:
|
||||
rc = self.FindReturnKeyBoundButton(element)
|
||||
if rc is not None:
|
||||
return rc
|
||||
|
@ -1393,7 +1393,7 @@ class Frame(Element):
|
|||
# Tab #
|
||||
# ---------------------------------------------------------------------- #
|
||||
class Tab(Element):
|
||||
def __init__(self, title, layout, title_color=None, background_color=None, size=(None, None), font=None, pad=None, border_width=None, key=None, tooltip=None):
|
||||
def __init__(self, title, layout, title_color=None, background_color=None, font=None, pad=None, border_width=None, key=None, tooltip=None):
|
||||
|
||||
self.UseDictionary = False
|
||||
self.ReturnValues = None
|
||||
|
@ -1409,7 +1409,7 @@ class Tab(Element):
|
|||
|
||||
self.Layout(layout)
|
||||
|
||||
super().__init__(ELEM_TYPE_TAB, background_color=background_color, text_color=title_color, size=size, font=font, pad=pad, key=key, tooltip=tooltip)
|
||||
super().__init__(ELEM_TYPE_TAB, background_color=background_color, text_color=title_color, font=font, pad=pad, key=key, tooltip=tooltip)
|
||||
return
|
||||
|
||||
def AddRow(self, *args):
|
||||
|
@ -1450,7 +1450,7 @@ class Tab(Element):
|
|||
# TabGroup #
|
||||
# ---------------------------------------------------------------------- #
|
||||
class TabGroup(Element):
|
||||
def __init__(self, layout, title_color=None, background_color=None, size=(None, None), font=None, pad=None, border_width=None, key=None, tooltip=None):
|
||||
def __init__(self, layout, title_color=None, background_color=None, font=None, pad=None, border_width=None, key=None, tooltip=None):
|
||||
|
||||
self.UseDictionary = False
|
||||
self.ReturnValues = None
|
||||
|
@ -1465,7 +1465,7 @@ class TabGroup(Element):
|
|||
|
||||
self.Layout(layout)
|
||||
|
||||
super().__init__(ELEM_TYPE_MULTI_TAB, background_color=background_color, text_color=title_color, size=size, font=font, pad=pad, key=key, tooltip=tooltip)
|
||||
super().__init__(ELEM_TYPE_TAB_GROUP, background_color=background_color, text_color=title_color, font=font, pad=pad, key=key, tooltip=tooltip)
|
||||
return
|
||||
|
||||
def AddRow(self, *args):
|
||||
|
@ -2491,7 +2491,7 @@ def BuildResultsForSubform(form, initialize_only, top_level_form):
|
|||
if element.ReturnValues[0] is not None: # if a button was clicked
|
||||
button_pressed_text = element.ReturnValues[0]
|
||||
|
||||
if element.Type == ELEM_TYPE_MULTI_TAB:
|
||||
if element.Type == ELEM_TYPE_TAB_GROUP:
|
||||
element.DictionaryKeyCounter = top_level_form.DictionaryKeyCounter
|
||||
element.ReturnValuesList = []
|
||||
element.ReturnValuesDictionary = {}
|
||||
|
@ -2575,7 +2575,7 @@ def BuildResultsForSubform(form, initialize_only, top_level_form):
|
|||
# if an input type element, update the results
|
||||
if element.Type != ELEM_TYPE_BUTTON and element.Type != ELEM_TYPE_TEXT and element.Type != ELEM_TYPE_IMAGE and\
|
||||
element.Type != ELEM_TYPE_OUTPUT and element.Type != ELEM_TYPE_PROGRESS_BAR and \
|
||||
element.Type!= ELEM_TYPE_COLUMN and element.Type != ELEM_TYPE_FRAME and element.Type != ELEM_TYPE_MULTI_TAB \
|
||||
element.Type!= ELEM_TYPE_COLUMN and element.Type != ELEM_TYPE_FRAME and element.Type != ELEM_TYPE_TAB_GROUP \
|
||||
and element.Type != ELEM_TYPE_TAB:
|
||||
AddToReturnList(form, value)
|
||||
AddToReturnDictionary(top_level_form, element, value)
|
||||
|
@ -2614,7 +2614,7 @@ def FillSubformWithValues(form, values_dict):
|
|||
FillSubformWithValues(element, values_dict)
|
||||
if element.Type == ELEM_TYPE_FRAME:
|
||||
FillSubformWithValues(element, values_dict)
|
||||
if element.Type == ELEM_TYPE_MULTI_TAB:
|
||||
if element.Type == ELEM_TYPE_TAB_GROUP:
|
||||
FillSubformWithValues(element, values_dict)
|
||||
if element.Type == ELEM_TYPE_TAB:
|
||||
FillSubformWithValues(element, values_dict)
|
||||
|
@ -2654,7 +2654,7 @@ def _FindElementFromKeyInSubForm(form, key):
|
|||
matching_elem = _FindElementFromKeyInSubForm(element, key)
|
||||
if matching_elem is not None:
|
||||
return matching_elem
|
||||
if element.Type == ELEM_TYPE_MULTI_TAB:
|
||||
if element.Type == ELEM_TYPE_TAB_GROUP:
|
||||
matching_elem = _FindElementFromKeyInSubForm(element, key)
|
||||
if matching_elem is not None:
|
||||
return matching_elem
|
||||
|
@ -3178,25 +3178,25 @@ def PackFormIntoFrame(form, containing_frame, toplevel_form):
|
|||
element.TKFrame.configure(background=element.BackgroundColor,
|
||||
highlightbackground=element.BackgroundColor,
|
||||
highlightcolor=element.BackgroundColor)
|
||||
if element.TextColor != COLOR_SYSTEM_DEFAULT and element.TextColor is not None:
|
||||
element.TKFrame.configure(foreground=element.TextColor)
|
||||
# if element.TextColor != COLOR_SYSTEM_DEFAULT and element.TextColor is not None:
|
||||
# element.TKFrame.configure(foreground=element.TextColor)
|
||||
if element.BorderWidth is not None:
|
||||
element.TKFrame.configure(borderwidth=element.BorderWidth)
|
||||
if element.Tooltip is not None:
|
||||
element.TooltipObject = ToolTip(element.TKFrame, text=element.Tooltip,
|
||||
timeout=DEFAULT_TOOLTIP_TIME)
|
||||
# ------------------------- MultiTab element ------------------------- #
|
||||
elif element_type == ELEM_TYPE_MULTI_TAB:
|
||||
# ------------------------- TabGroup element ------------------------- #
|
||||
elif element_type == ELEM_TYPE_TAB_GROUP:
|
||||
element.TKNotebook = ttk.Notebook(tk_row_frame)
|
||||
PackFormIntoFrame(element, toplevel_form.TKroot, toplevel_form)
|
||||
|
||||
# element.TKNotebook.pack(side=tk.LEFT)
|
||||
if element.BackgroundColor != COLOR_SYSTEM_DEFAULT and element.BackgroundColor is not None:
|
||||
element.TKNotebook.configure(background=element.BackgroundColor,
|
||||
highlightbackground=element.BackgroundColor,
|
||||
highlightcolor=element.BackgroundColor)
|
||||
if element.TextColor != COLOR_SYSTEM_DEFAULT and element.TextColor is not None:
|
||||
element.TKNotebook.configure(foreground=element.TextColor)
|
||||
# if element.BackgroundColor != COLOR_SYSTEM_DEFAULT and element.BackgroundColor is not None:
|
||||
# element.TKNotebook.configure(background=element.BackgroundColor,
|
||||
# highlightbackground=element.BackgroundColor,
|
||||
# highlightcolor=element.BackgroundColor)
|
||||
# if element.TextColor != COLOR_SYSTEM_DEFAULT and element.TextColor is not None:
|
||||
# element.TKNotebook.configure(foreground=element.TextColor)
|
||||
if element.BorderWidth is not None:
|
||||
element.TKNotebook.configure(borderwidth=element.BorderWidth)
|
||||
if element.Tooltip is not None:
|
||||
|
|
|
@ -9,7 +9,7 @@
|
|||
|
||||
# PySimpleGUI
|
||||
|
||||

|
||||

|
||||
|
||||
|
||||
[Announcements of Latest Developments](https://github.com/MikeTheWatchGuy/PySimpleGUI/issues/142)
|
||||
|
@ -1971,22 +1971,80 @@ Let me say up front that the Table Element has Beta status. The reason is that s
|
|||
|
||||
|
||||
|
||||
## Tabbed windows
|
||||
Tabbed windows are shown using the `ShowTabbedForm` call. The call has the format
|
||||
## Tab and Tab Group Elements
|
||||
|
||||
results = ShowTabbedForm('Title for the form',
|
||||
(form,layout,'Tab 1 label'),
|
||||
(form2,layout2, 'Tab 2 label'), ...)
|
||||
Tabs have been a part of PySimpleGUI since the initial release. However, the initial implementation applied tabs at the top level only. The entire window had to be tabbed. There with other limitations that came along with that implementation. That all changed in version 3.8.0 with the new elements - Tab and TabGroup. The old implementation of Tabs was removed in version 3.8.0 as well.
|
||||
|
||||
Each of the tabs of the form is in fact a window. The same steps are taken to create the form as before. A `Window` is created, then rows are filled with Elements, and finally the form is shown. When calling `ShowTabbedForm`, each form is passed in as a tuple. The tuple has the format: `(the form, the rows, a string shown on the tab)`
|
||||
Tabs are another "Container Element". The other Container Elements include:
|
||||
* Frame
|
||||
* Column
|
||||
|
||||
Results are returned as a list of lists. For each form you'll get a list that's in the same format as a normal window. A single tab's values would be:
|
||||
You layout a Frame in exactly the same way as a Frame or Column elements, by passing in a list of elements.
|
||||
|
||||
(button, (values))
|
||||
How you place a Tab into a Window is different than Graph or Frame elements. You cannot place a tab directly into a Window's layout. It much first be placed into a TabGroup. The TabGroup can then be placed into the Window.
|
||||
|
||||
Recall that values is a list as well. Multiple tabs in the form would return like this:
|
||||
Let's look at this Window as an example:
|
||||
|
||||
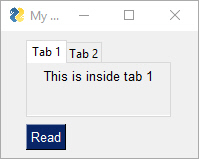
|
||||
|
||||
View of second tab:
|
||||
|
||||
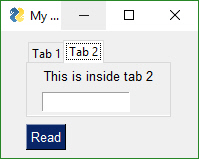
|
||||
|
||||
|
||||
First we have the Tab layout definitions. They mirror what you see in the screen shots. Tab 1 has 1 Text Element in it. Tab 2 has a Text and an Input Element.
|
||||
|
||||
|
||||
tab1_layout = [[sg.T('This is inside tab 1')]]
|
||||
|
||||
tab2_layout = [[sg.T('This is inside tab 2')],
|
||||
[sg.In(key='in')]]
|
||||
|
||||
The layout for the entire window looks like this:
|
||||
|
||||
layout = [[sg.TabGroup([[sg.Tab('Tab 1', tab1_layout), sg.Tab('Tab 2', tab2_layout)]])],
|
||||
[sg.RButton('Read')]]
|
||||
|
||||
The Window layout has the TabGroup and within the tab Group are the two Tab elements.
|
||||
|
||||
One important thing to notice about all of these container Elements... they all take a "list of lists" at the layout. They all have a layout that starts with `[[`
|
||||
|
||||
You will want to keep this `[[ ]]` construct in your head a you're debugging your tabbed windows. It's easy to overlook one or two necessary ['s
|
||||
|
||||
As mentioned earlier, the old-style Tabs were limited to being at the Window-level only. In other words, the tabs were equal in size to the entire window. This is not the case with the "new-style" tabs. This is why you're not going to be upset when you discover your old code no longer works with the new PySimpleGUI release. It'll be worth the few moments it'll take to convert your code.
|
||||
|
||||
Check out what's possible with the NEW Tabs!
|
||||
|
||||
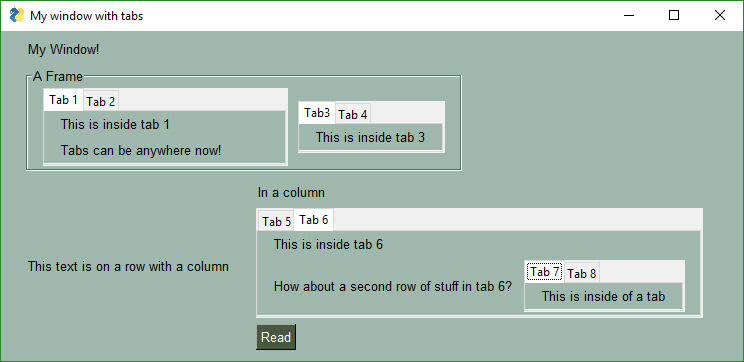
|
||||
|
||||
|
||||
Check out Tabs 7 and 8. We've got a Window with a Column containing Tabs 5 and 6. On Tab 6 are... Tabs 7 and 8.
|
||||
|
||||
As of Release 3.8.0, not all of *options* shown in the API definitions of the Tab and TabGroup Elements are working. They are there as placeholders.
|
||||
|
||||
The definition of a TabGroup is
|
||||
|
||||
TabGroup(layout,
|
||||
title_color=None
|
||||
background_color=None
|
||||
font=None
|
||||
pad=None
|
||||
border_width=None
|
||||
key=None
|
||||
tooltip=None)
|
||||
|
||||
The definition of a Tab Element is
|
||||
|
||||
Tab(title,
|
||||
layout,
|
||||
title_color=None,
|
||||
background_color=None,
|
||||
size=(None, None),font=None,
|
||||
pad=None
|
||||
border_width=None
|
||||
key=None
|
||||
tooltip=None)
|
||||
|
||||
((button1, (values1)), (button2, (values2))
|
||||
|
||||
## Colors ##
|
||||
Starting in version 2.5 you can change the background colors for the window and the Elements.
|
||||
|
@ -2592,6 +2650,8 @@ A MikeTheWatchGuy production... entirely responsible for this code.... unless it
|
|||
| 03.05.01 | Sept 22, 2018 - See release notes
|
||||
| 03.05.02 | Sept 23, 2018 - See release notes
|
||||
| 03.06.00 | Sept 23, 2018 - Goodbye FlexForm, hello Window
|
||||
| 03.08.00 | Sept 25, 2018 - Tab and TabGroup Elements
|
||||
|
||||
|
||||
### Release Notes
|
||||
2.3 - Sliders, Listbox's and Image elements (oh my!)
|
||||
|
@ -2682,6 +2742,9 @@ OneLineProgressMeter function added which gives you not only a one-line solution
|
|||
* Renamed FlexForm to Window
|
||||
* Removed LookAndFeel capability from Mac platform.
|
||||
|
||||
#### 3.8.0
|
||||
* Tab and TabGroup Elements - awesome new capabilities
|
||||
|
||||
|
||||
### Upcoming
|
||||
Make suggestions people! Future release features
|
||||
|
@ -2771,4 +2834,4 @@ For Python questions, I simply start my query with 'Python'. Let's say you forg
|
|||
|
||||
In the hands of a competent programmer, this tool is **amazing**. It's a must-try kind of program that has completely changed my programming process. I'm not afraid of asking for help! You just have to be smart about using what you find.
|
||||
|
||||
The PySimpleGUI window that the results are shown in is an 'input' field which means you can copy and paste the results right into your code.
|
||||
The PySimpleGUI window that the results are shown in is an 'input' field which means you can copy and paste the results right into your code.
|
||||
|
|
87
readme.md
87
readme.md
|
@ -9,7 +9,7 @@
|
|||
|
||||
# PySimpleGUI
|
||||
|
||||

|
||||

|
||||
|
||||
|
||||
[Announcements of Latest Developments](https://github.com/MikeTheWatchGuy/PySimpleGUI/issues/142)
|
||||
|
@ -1971,22 +1971,80 @@ Let me say up front that the Table Element has Beta status. The reason is that s
|
|||
|
||||
|
||||
|
||||
## Tabbed windows
|
||||
Tabbed windows are shown using the `ShowTabbedForm` call. The call has the format
|
||||
## Tab and Tab Group Elements
|
||||
|
||||
results = ShowTabbedForm('Title for the form',
|
||||
(form,layout,'Tab 1 label'),
|
||||
(form2,layout2, 'Tab 2 label'), ...)
|
||||
Tabs have been a part of PySimpleGUI since the initial release. However, the initial implementation applied tabs at the top level only. The entire window had to be tabbed. There with other limitations that came along with that implementation. That all changed in version 3.8.0 with the new elements - Tab and TabGroup. The old implementation of Tabs was removed in version 3.8.0 as well.
|
||||
|
||||
Each of the tabs of the form is in fact a window. The same steps are taken to create the form as before. A `Window` is created, then rows are filled with Elements, and finally the form is shown. When calling `ShowTabbedForm`, each form is passed in as a tuple. The tuple has the format: `(the form, the rows, a string shown on the tab)`
|
||||
Tabs are another "Container Element". The other Container Elements include:
|
||||
* Frame
|
||||
* Column
|
||||
|
||||
Results are returned as a list of lists. For each form you'll get a list that's in the same format as a normal window. A single tab's values would be:
|
||||
You layout a Frame in exactly the same way as a Frame or Column elements, by passing in a list of elements.
|
||||
|
||||
(button, (values))
|
||||
How you place a Tab into a Window is different than Graph or Frame elements. You cannot place a tab directly into a Window's layout. It much first be placed into a TabGroup. The TabGroup can then be placed into the Window.
|
||||
|
||||
Recall that values is a list as well. Multiple tabs in the form would return like this:
|
||||
Let's look at this Window as an example:
|
||||
|
||||
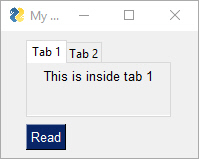
|
||||
|
||||
View of second tab:
|
||||
|
||||
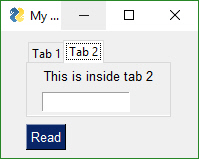
|
||||
|
||||
|
||||
First we have the Tab layout definitions. They mirror what you see in the screen shots. Tab 1 has 1 Text Element in it. Tab 2 has a Text and an Input Element.
|
||||
|
||||
|
||||
tab1_layout = [[sg.T('This is inside tab 1')]]
|
||||
|
||||
tab2_layout = [[sg.T('This is inside tab 2')],
|
||||
[sg.In(key='in')]]
|
||||
|
||||
The layout for the entire window looks like this:
|
||||
|
||||
layout = [[sg.TabGroup([[sg.Tab('Tab 1', tab1_layout), sg.Tab('Tab 2', tab2_layout)]])],
|
||||
[sg.RButton('Read')]]
|
||||
|
||||
The Window layout has the TabGroup and within the tab Group are the two Tab elements.
|
||||
|
||||
One important thing to notice about all of these container Elements... they all take a "list of lists" at the layout. They all have a layout that starts with `[[`
|
||||
|
||||
You will want to keep this `[[ ]]` construct in your head a you're debugging your tabbed windows. It's easy to overlook one or two necessary ['s
|
||||
|
||||
As mentioned earlier, the old-style Tabs were limited to being at the Window-level only. In other words, the tabs were equal in size to the entire window. This is not the case with the "new-style" tabs. This is why you're not going to be upset when you discover your old code no longer works with the new PySimpleGUI release. It'll be worth the few moments it'll take to convert your code.
|
||||
|
||||
Check out what's possible with the NEW Tabs!
|
||||
|
||||
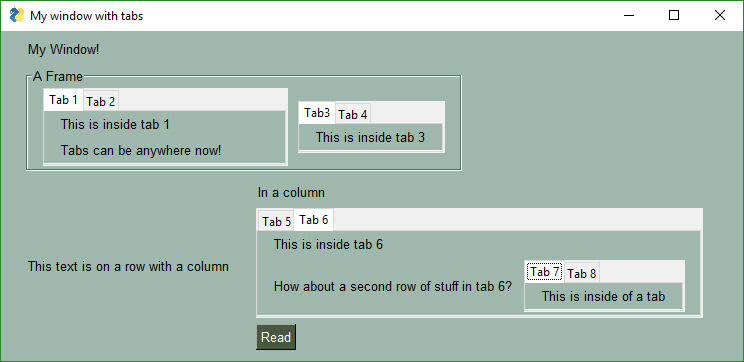
|
||||
|
||||
|
||||
Check out Tabs 7 and 8. We've got a Window with a Column containing Tabs 5 and 6. On Tab 6 are... Tabs 7 and 8.
|
||||
|
||||
As of Release 3.8.0, not all of *options* shown in the API definitions of the Tab and TabGroup Elements are working. They are there as placeholders.
|
||||
|
||||
The definition of a TabGroup is
|
||||
|
||||
TabGroup(layout,
|
||||
title_color=None
|
||||
background_color=None
|
||||
font=None
|
||||
pad=None
|
||||
border_width=None
|
||||
key=None
|
||||
tooltip=None)
|
||||
|
||||
The definition of a Tab Element is
|
||||
|
||||
Tab(title,
|
||||
layout,
|
||||
title_color=None,
|
||||
background_color=None,
|
||||
size=(None, None),font=None,
|
||||
pad=None
|
||||
border_width=None
|
||||
key=None
|
||||
tooltip=None)
|
||||
|
||||
((button1, (values1)), (button2, (values2))
|
||||
|
||||
## Colors ##
|
||||
Starting in version 2.5 you can change the background colors for the window and the Elements.
|
||||
|
@ -2592,6 +2650,8 @@ A MikeTheWatchGuy production... entirely responsible for this code.... unless it
|
|||
| 03.05.01 | Sept 22, 2018 - See release notes
|
||||
| 03.05.02 | Sept 23, 2018 - See release notes
|
||||
| 03.06.00 | Sept 23, 2018 - Goodbye FlexForm, hello Window
|
||||
| 03.08.00 | Sept 25, 2018 - Tab and TabGroup Elements
|
||||
|
||||
|
||||
### Release Notes
|
||||
2.3 - Sliders, Listbox's and Image elements (oh my!)
|
||||
|
@ -2682,6 +2742,9 @@ OneLineProgressMeter function added which gives you not only a one-line solution
|
|||
* Renamed FlexForm to Window
|
||||
* Removed LookAndFeel capability from Mac platform.
|
||||
|
||||
#### 3.8.0
|
||||
* Tab and TabGroup Elements - awesome new capabilities
|
||||
|
||||
|
||||
### Upcoming
|
||||
Make suggestions people! Future release features
|
||||
|
@ -2771,4 +2834,4 @@ For Python questions, I simply start my query with 'Python'. Let's say you forg
|
|||
|
||||
In the hands of a competent programmer, this tool is **amazing**. It's a must-try kind of program that has completely changed my programming process. I'm not afraid of asking for help! You just have to be smart about using what you find.
|
||||
|
||||
The PySimpleGUI window that the results are shown in is an 'input' field which means you can copy and paste the results right into your code.
|
||||
The PySimpleGUI window that the results are shown in is an 'input' field which means you can copy and paste the results right into your code.
|
||||
|
|
Loading…
Reference in New Issue