diff --git a/PySimpleGUI.py b/PySimpleGUI.py
index 5ae791de..1c6aace3 100644
--- a/PySimpleGUI.py
+++ b/PySimpleGUI.py
@@ -1,5 +1,5 @@
#!/usr/bin/python3
-version = __version__ = "4.1.0.3 Unreleased"
+version = __version__ = "4.1.0.5 Unreleased"
import sys
@@ -18,15 +18,14 @@ else:
import tkFont
import ScrolledText
-import types
import datetime
import time
import pickle
import calendar
-from random import randint
import textwrap
-import operator
import inspect
+from typing import List, Any, Union
+from random import randint
# 888888ba .d88888b oo dP .88888. dP dP dP
# 88 `8b 88. "' 88 d8' `88 88 88 88
@@ -38,6 +37,7 @@ import inspect
# d8888P dP
+
g_time_start = 0
g_time_end = 0
g_time_delta = 0
@@ -229,9 +229,9 @@ MENU_KEY_SEPARATOR = '::'
def RGB(red, green, blue):
"""
Given integer values of Red, Green, Blue, return a color string "#RRGGBB"
- :param (int) red: int: Red portion from 0 to 255
- :param (int) green: int: Green portion from 0 to 255
- :param (int) blue: int: Blue portion from 0 to 255
+ :param red: (int) Red portion from 0 to 255
+ :param green: (int) Green portion from 0 to 255
+ :param blue: (int) Blue portion from 0 to 255
:return: (str) A single RGB String in the format "#RRGGBB" where each pair is a hex number.
"""
return '#%02x%02x%02x' % (red, green, blue)
@@ -405,17 +405,17 @@ class Element():
def __init__(self, type, size=(None, None), auto_size_text=None, font=None, background_color=None, text_color=None, key=None, pad=None, tooltip=None, visible=True):
"""
Element base class. Only used internally. User will not create an Element object by itself
+
:param type: (int - could be enum) The type of element. These constants all start with "ELEM_TYPE_"
:param size: (int,int) (width ,height ) w=characters-wide, h=rows-high
:param auto_size_text: (bool) True if the Widget should be shrunk to exactly fit the number of chars to show
:param font: (str or tuple) specifies the font family, size, bold, .... See docs on format of this field
:param background_color: (str) color of background. Can be in #RRGGBB format or a color name "black"
:param text_color: (str) element's text color. Can be in #RRGGBB format or a color name "black"
- :param key: (any type) Identifies an Element. Should be UNIQUE to this window.
+ :param key: (Any) Identifies an Element. Should be UNIQUE to this window.
:param pad: (int, int) or ((int,int),(int,int)) Amount of padding to put around element in pixels (left/right, top/bottom)
:param tooltip: (str) text, that will appear when mouse hovers over the element
:param visible: (bool) set visibility state of the element (Default = True)
-
"""
self.Size = size
self.Type = type
@@ -445,6 +445,7 @@ class Element():
def _RightClickMenuCallback(self, event):
"""
Callback function that's called when a right click happens. Shows right click menu as result
+
:param event: information provided by tkinter about the event including x,y location of click
"""
@@ -454,7 +455,8 @@ class Element():
def _MenuItemChosenCallback(self, item_chosen): # TEXT Menu item callback
"""
Callback function called when user chooses a menu item from menubar, Button Menu or right click menu
- :param item_chosen: str. String holding the value chosen.
+
+ :param item_chosen: (str) String holding the value chosen.
"""
# print('IN MENU ITEM CALLBACK', item_chosen)
@@ -468,8 +470,9 @@ class Element():
"""
Searches for which Button has the flag Button.BindReturnKey set. It is called recursively when a
"Container Element" is encountered. Func has to walk entire window including these "sub-forms"
+
:param form: the Window object to search
- :return Button Object if a button is found, else None if no button found
+ :return: union[Button, None] Button Object if a button is found, else None if no button found
"""
for row in form.Rows:
for element in row:
@@ -502,7 +505,8 @@ class Element():
"""
Callback that's called when a text element is clicked on with events enabled on the Text Element.
Result is that control is returned back to user (quits mainloop).
- :param event:
+
+ :param event:
"""
if self.Key is not None:
@@ -515,8 +519,9 @@ class Element():
def _ReturnKeyHandler(self, event):
"""
+ Internal callback for the ENTER / RETURN key. Results in calling the ButtonCallBack for element that has the return key bound to it, just as if button was clicked.
- :param event:
+ :param event:
"""
MyForm = self.ParentForm
@@ -526,7 +531,8 @@ class Element():
def _ListboxSelectHandler(self, event):
"""
- Callback function for when a listbox item is selected
+ Internal callback function for when a listbox item is selected
+
:param event: Information from tkinter about the callback
"""
@@ -542,8 +548,8 @@ class Element():
def _ComboboxSelectHandler(self, event):
"""
-
- :param event:
+ Internal callback function for when an entry is selected in a Combobox.
+ :param event: Event data from tkinter (not used)
"""
# first, get the results table built
@@ -557,7 +563,9 @@ class Element():
self.ParentForm.TKroot.quit() # kick the users out of the mainloop
def _RadioHandler(self):
- """ """
+ """
+ Internal callback for when a radio button is selected and enable events was set for radio
+ """
if self.Key is not None:
self.ParentForm.LastButtonClicked = self.Key
else:
@@ -567,7 +575,9 @@ class Element():
self.ParentForm.TKroot.quit()
def _CheckboxHandler(self):
- """ """
+ """
+ Internal callback for when a checkbnox is selected and enable events was set for checkbox
+ """
if self.Key is not None:
self.ParentForm.LastButtonClicked = self.Key
else:
@@ -578,9 +588,9 @@ class Element():
def _TabGroupSelectHandler(self, event):
"""
+ Internal callback for when a Tab is selected and enable events was set for TabGroup
- :param event:
-
+ :param event: Event data passed in by tkinter (not used)
"""
if self.Key is not None:
self.ParentForm.LastButtonClicked = self.Key
@@ -592,9 +602,9 @@ class Element():
def _KeyboardHandler(self, event):
"""
+ Internal callback for when a key is pressed andd return keyboard events was set for window
- :param event:
-
+ :param event: Event data passed in by tkinter (not used)
"""
if self.Key is not None:
self.ParentForm.LastButtonClicked = self.Key
@@ -606,9 +616,9 @@ class Element():
def _ClickHandler(self, event):
"""
+ Internal callback for when a mouse was clicked... I think.
- :param event:
-
+ :param event: Event data passed in by tkinter (not used)
"""
if self.Key is not None:
self.ParentForm.LastButtonClicked = self.Key
@@ -620,9 +630,9 @@ class Element():
def SetTooltip(self, tooltip_text):
"""
+ Called by application to change the tooltip text for an Element. Normally invoked using the Element Object such as: window.Element('key').SetToolTip('New tip').
- :param tooltip_text:
-
+ :param tooltip_text: (str) the text to show in tooltip.
"""
self.TooltipObject = ToolTip(self.Widget, text=tooltip_text, timeout=DEFAULT_TOOLTIP_TIME)
@@ -651,7 +661,7 @@ class Element():
# ---------------------------------------------------------------------- #
class InputText(Element):
"""
- Display a single text input field. Based on the tkinter Widget Entry
+ Display a single text input field. Based on the tkinter Widget `Entry`
"""
def __init__(self, default_text='', size=(None, None), disabled=False, password_char='',
justification=None, background_color=None, text_color=None, font=None, tooltip=None,
@@ -667,14 +677,14 @@ class InputText(Element):
:param background_color: (str) color of background in one of the color formats
:param text_color: (str) color of the text
:param font: (str / tuple) specifies the font family, size, etc
- :param tooltip: (str) Text shown when cursor hovers over the element
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
:param change_submits: (bool) * DEPRICATED DO NOT USE! Same as enable_events
:param enable_events: (bool) If True then changes to this element are immediately reported as an event. Use this instead of change_submits (Default = False)
:param do_not_clear: (bool) If False then the field will be set to blank after ANY event (button, any event) (Default = True)
:param key: (any) Value that uniquely identifies this element from all other elements. Used when Finding an element or in return values. Must be unique to the window
:param focus: (bool) Determines if initial focus should go to this element.
:param pad: (int, int) or ((int, int), (int, int)) Tuple(s). Amount of padding to put around element. Normally (horizontal pixels, vertical pixels) but can be split apart further into ((horizontal left, horizontal right), (vertical above, vertical below))
- :param right_click_menu: [[]]. A list of lists of Menu items to show when this element is right clicked. See docs for format.
+ :param right_click_menu: (list(list)) [ [ ] ] A list of lists of Menu items to show when this element is right clicked. See docs for format.
:param visible: (bool) set visibility state of the element (Default = True)
"""
self.DefaultText = default_text
@@ -693,14 +703,14 @@ class InputText(Element):
def Update(self, value=None, disabled=None, select=None, visible=None):
"""
- Changes some of the settings for the Input Element
+ Changes some of the settings for the Input Element. Must call `Window.Read` or `Window.Finalize` prior
+
:param value: (str) new text to display as default text in Input field
:param disabled: (bool) disable or enable state of the element (sets Entry Widget to readonly or normal)
:param select: (bool) if True, then the text will be selected
:param visible: (bool) change visibility of element
-
"""
- #NOTE - Read or Finalize must be called on Window prior to Update call
+
if disabled is True:
self.TKEntry['state'] = 'readonly'
elif disabled is False:
@@ -720,7 +730,8 @@ class InputText(Element):
def Get(self):
"""
- Read and return the current value of the input element
+ Read and return the current value of the input element. Must call `Window.Read` or `Window.Finalize` prior
+
:return: (str) current value of Input field or '' if error encountered
"""
try:
@@ -731,8 +742,9 @@ class InputText(Element):
def SetFocus(self, force=False):
"""
- Sets focus to this element using focus_set. Will use focus_force if force flag set
- :param force: (bool) if True then focus_force will be called instead of focus_set
+ Sets focus to this element using focus_set. Will use focus_force if force flag set. Must call `Window.Read` or `Window.Finalize` prior
+
+ :param force: (bool) if True then tkinter's `Entry.focus_force` will be called instead of `Entry.focus_set`
"""
try:
if force:
@@ -757,34 +769,34 @@ I = InputText
# Combo #
# ---------------------------------------------------------------------- #
class Combo(Element):
- """ComboBox Element"""
+ """
+ ComboBox Element - A combination of a single-line input and a drop-down menu. User can type in their own value or choose from list.
+ """
def __init__(self, values, default_value=None, size=(None, None), auto_size_text=None, background_color=None,
text_color=None, change_submits=False, enable_events=False, disabled=False, key=None, pad=None,
tooltip=None, readonly=False, font=None, visible=True):
- """Combo Element - Combo Box, Drop Down Menu, ...
-
- :param values: values to choice
- :param default_value: Choices to be displayed. List of strings
- :param size: (w,h) w=characters-wide, h=rows-high
- :param auto_size_text: True if size should fit the text length
- :param background_color: color of background
- :param text_color: color of the text
- :param change_submits: If True, pressing Enter key submits window (Default = False)
- :param enable_events: Turns on the element specific events.(Default = False)
- :param disabled: set disable state for element (Default = False)
- :param key: Used with window.FindElement and with return values
- :param pad: Amount of padding to put around element
- :param tooltip: text, that will appear the you hover on
- :param readonly: make element readonly (Default = False)
- :param font: specifies the font family, size, etc
- :param visible: set visibility state of the element (Default = True)
+ """
+ :param values: List[Any] values to choose. While displayed as text, the items returned are what the caller supplied, not text
+ :param default_value: (Any) Choice to be displayed as initial value. Must match one of values variable contents
+ :param size: (int, int) (width, height) width = characters-wide, height = rows-high
+ :param auto_size_text: (bool) True if element should be the same size as the contents
+ :param background_color: (str) color of background
+ :param text_color: (str) color of the text
+ :param change_submits: (bool) DEPRICATED DO NOT USE. Use `enable_events` instead
+ :param enable_events: (bool) Turns on the element specific events. Combo event is when a choice is made
+ :param disabled: (bool) set disable state for element
+ :param key: (Any) Used with window.FindElement and with return values to uniquely identify this element
+ :param pad: (int, int) or ((int, int),(int,int)) Amount of padding to put around element (left/right, top/bottom) or ((left, right), (top, bottom))
+ :param tooltip: (str) text that will appear when mouse hovers over this element
+ :param readonly: (bool) make element readonly (user can't change). True means user cannot change
+ :param font: Union[str, tuple] specifies the font family, size, etc
+ :param visible: (bool) set visibility state of the element
"""
self.Values = values
self.DefaultValue = default_value
self.ChangeSubmits = change_submits or enable_events
self.Widget = self.TKCombo = None # type: ttk.Combobox
- # self.InitializeAsDisabled = disabled
self.Disabled = disabled
self.Readonly = readonly
bg = background_color if background_color else DEFAULT_INPUT_ELEMENTS_COLOR
@@ -795,14 +807,15 @@ class Combo(Element):
def Update(self, value=None, values=None, set_to_index=None, disabled=None, readonly=None, font=None, visible=None):
"""
- :param value: change which value is current selected
- :param values: change list of choices
- :param set_to_index: change selection to a particular choice
- :param disabled: disable or enable state of the element
- :param readonly: make element readonly
- :param font: specifies the font family, size, etc
- :param visible: change visibility of element
+ Changes some of the settings for the Combo Element. Must call `Window.Read` or `Window.Finalize` prior
+ :param value: (Any) change which value is current selected hased on new list of previous list of choices
+ :param values: List[Any] change list of choices
+ :param set_to_index: (int) change selection to a particular choice starting with index = 0
+ :param disabled: (bool) disable or enable state of the element
+ :param readonly: (bool) if True make element readonly (user cannot change any choices)
+ :param font: Union[str, tuple] specifies the font family, size, using one of the two font formats
+ :param visible: (bool) control visibility of element
"""
if values is not None:
try:
@@ -841,10 +854,12 @@ class Combo(Element):
elif visible is True:
self.TKCombo.pack()
+
def GetSelectedItemsIndexes(self):
"""
Get the list of chosen items and return them as a list of indexes (offsets within the list)
- :return: [int,] List of indexes of currently selected items
+
+ :return: List[int] List of indexes of currently selected items
"""
if not self.TKStringVar:
return []
@@ -870,29 +885,27 @@ Drop = InputCombo
# Option Menu #
# ---------------------------------------------------------------------- #
class OptionMenu(Element):
- """Option Menu is an Element available ONLY on the tkinter port of PySimpleGUI. It's is a widget that is unique
+ """
+ Option Menu is an Element available ONLY on the tkinter port of PySimpleGUI. It's is a widget that is unique
to tkinter. However, it looks much like a ComboBox. Instead of an arrow to click to pull down the list of
choices, another little graphic is shown on the widget to indicate where you click. After clicking to activate,
it looks like a Combo Box that you scroll to select a choice.
-
-
"""
def __init__(self, values, default_value=None, size=(None, None), disabled=False, auto_size_text=None,
background_color=None, text_color=None, key=None, pad=None, tooltip=None, visible=True):
- """Option Menu Element
-
- :param values: ??????????????????
- :param default_value: ?????????????????????????//
- :param size: (w,h) w=characters-wide, h=rows-high
- :param disabled: set disable state for element (Default = False)
- :param auto_size_text: True if size should fit the text length
- :param background_color: color of background
- :param text_color: color of the text
- :param key: Used with window.FindElement and with return values
- :param pad: Amount of padding to put around element
- :param tooltip: text, that will appear the you hover on
- :param visible: set visibility state of the element (Default = True)
+ """
+ :param values: List[Any] Values to be displayed
+ :param default_value: (Any) the value to choose by default
+ :param size: Tuple[int, int] (width, height) size in characters (wide) and rows (high)
+ :param disabled: (bool) control enabled / disabled
+ :param auto_size_text: (bool) True if size of Element should match the contents of the items
+ :param background_color: (str) color of background
+ :param text_color: (str) color of the text
+ :param key: (Any) Used with window.FindElement and with return values to uniquely identify this element
+ :param pad: (int, int) or ((int, int),(int,int)) Amount of padding to put around element (left/right, top/bottom) or ((left, right), (top, bottom))
+ :param tooltip: (str) text that will appear when mouse hovers over this element
+ :param visible: (bool) set visibility state of the element
"""
self.Values = values
self.DefaultValue = default_value
@@ -905,13 +918,13 @@ class OptionMenu(Element):
text_color=fg, key=key, pad=pad, tooltip=tooltip, visible=visible)
def Update(self, value=None, values=None, disabled=None, visible=None):
- """OptionMenu Element Update
-
- :param value: ??????????????????
- :param values: ??????????????????
- :param disabled: disable or enable state of the element
- :param visible: change visibility of element
+ """
+ Changes some of the settings for the OptionMenu Element. Must call `Window.Read` or `Window.Finalize` prior
+ :param value: (Any) the value to choose by default
+ :param values: List[Any] Values to be displayed
+ :param disabled: (bool) disable or enable state of the element
+ :param visible: (bool) control visibility of element
"""
if values is not None:
self.Values = values
@@ -965,26 +978,25 @@ class Listbox(Element):
bind_return_key=False, size=(None, None), disabled=False, auto_size_text=None, font=None,
background_color=None, text_color=None, key=None, pad=None, tooltip=None, right_click_menu=None,
visible=True):
- """Listbox Element
-
- :param values: list of values to display. Can be any type including mixed types as long as they have __str__ method
- :param default_values: list of objects for listbox
- :param select_mode: can be a string or a constant value defined as a variable. Generally speaking strings are used for these kinds of options
- :param change_submits: If True, pressing Enter key submits window (Default = False)
- :param enable_events: Turns on the element specific events.(Default = False)
- :param bind_return_key: (Default = False)
- :param size: (w,h) w=characters-wide, h=rows-high
- :param disabled: set disable state for element (Default = False)
- :param auto_size_text: True if size should fit the text length
- :param font: specifies the font family, size, etc
- :param background_color: color of background
- :param text_color: color of the text
- :param key: Used with window.FindElement and with return values
- :param pad: Amount of padding to put around element
- :param tooltip: text, that will appear the you hover on
- :param right_click_menu: see "Right Click Menus"
- :param visible: set visibility state of the element (Default = True)
+ """
+ :param values: List[Any] list of values to display. Can be any type including mixed types as long as they have __str__ method
+ :param default_values: List[Any] which values should be initially selected
+ :param select_mode: [str] can be a string or a constant value defined as a variable. Generally speaking strings are used for these kinds of options. Valid choices begin with "LISTBOX_SELECT_MODE_"
+ :param change_submits: (bool) DO NOT USE. Only listed for backwards compat - Use enable_events instead
+ :param enable_events: (bool) Turns on the element specific events. Listbox generates events when an item is clicked
+ :param bind_return_key: (bool) If True, then the return key will cause a the Listbox to generate an event
+ :param size: Tuple(int, int) (width, height) width = characters-wide, height = rows-high
+ :param disabled: (bool) set disable state for element
+ :param auto_size_text: (bool) True if element should be the same size as the contents
+ :param font: Union[str, tuple] specifies the font family, size. Uses one of two font specifications formats
+ :param background_color: (str) color of background
+ :param text_color: (str) color of the text
+ :param key: (Any) Used with window.FindElement and with return values to uniquely identify this element
+ :param pad: (int, int) or ((int, int),(int,int)) Amount of padding to put around element (left/right, top/bottom) or ((left, right), (top, bottom))
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
+ :param right_click_menu: List[List[str]] see "Right Click Menus" for format
+ :param visible: (bool) set visibility state of the element
"""
self.Values = values
self.DefaultValues = default_values
@@ -1013,15 +1025,15 @@ class Listbox(Element):
def Update(self, values=None, disabled=None, set_to_index=None, scroll_to_index=None, visible=None):
"""
- Update some of the element's settings
- :param values: new list of choices to be shown to user
- :param disabled: disable or enable state of the element
- :param set_to_index: highlights the item at this index as if user clicked
- :param scroll_to_index: scroll the listbox so that this index is the first shown
- :param visible: change visibility of element
+ Changes some of the settings for the Listbox Element. Must call `Window.Read` or `Window.Finalize` prior
+ :param values: List[Any] new list of choices to be shown to user
+ :param disabled: (bool) disable or enable state of the element
+ :param set_to_index: (int) highlights the item at this index as if user clicked
+ :param scroll_to_index: (int) scroll the listbox so that this index is the first shown
+ :param visible: (bool) control visibility of element
"""
-
+
if disabled == True:
self.TKListbox.configure(state='disabled')
elif disabled == False:
@@ -1050,8 +1062,9 @@ class Listbox(Element):
def SetValue(self, values):
"""
+ Set listbox highlighted choices
- :param values: new values to choice
+ :param values: List[Any] new values to choose based on previously set values
"""
for index, item in enumerate(self.Values):
@@ -1066,19 +1079,20 @@ class Listbox(Element):
def GetListValues(self):
- # type: (Listbox) -> []
+ # type: (Listbox) -> List[Any]
"""
Returns list of Values provided by the user in the user's format
- :return: List. List of values. Can be any / mixed types -> []
+
+ :return: List[Any]. List of values. Can be any / mixed types -> []
"""
return self.Values
def SetFocus(self, force=False):
"""
- Moves the focus to this element
- :param force: Bool. If True, will call focus_force instead of focus_set
- :return:
+ Moves the focus to this Listbox
+
+ :param force: (bool). If True, will call focus_force instead of focus_set
"""
try:
if force:
@@ -1102,34 +1116,32 @@ class Listbox(Element):
# Radio #
# ---------------------------------------------------------------------- #
class Radio(Element):
- """Radio Button Element - Used in a group of other Radio Elements to provide user with ability to select only
+ """
+ Radio Button Element - Used in a group of other Radio Elements to provide user with ability to select only
1 choice in a list of choices.
-
-
"""
def __init__(self, text, group_id, default=False, disabled=False, size=(None, None), auto_size_text=None,
background_color=None, text_color=None, font=None, key=None, pad=None, tooltip=None,
change_submits=False, enable_events=False, visible=True):
"""
- :param text: Text to display next to button
- :param group_id: Groups together multiple Radio Buttons. Can be any value
- :param default: Bool. Initial state (Default = False)
- :param disabled: set disable state for element (Default = False)
- :param size: (w,h) w=characters-wide, h=rows-high
- :param auto_size_text: True if size should fit the text length
- :param background_color: color of background
- :param text_color: color of the text
- :param font: specifies the font family, size, etc
- :param key: Used with window.FindElement and with return values
- :param pad: Amount of padding to put around element
- :param tooltip: text, that will appear the you hover on
- :param change_submits: If True, pressing Enter key submits window (Default = False)
- :param enable_events: Turns on the element specific events.(Default = False)
- :param visible: set visibility state of the element (Default = True)
-
+ :param text: (str) Text to display next to button
+ :param group_id: (Any) Groups together multiple Radio Buttons. Any type works
+ :param default: (bool). Set to True for the one element of the group you want initially selected
+ :param disabled: (bool) set disable state
+ :param size: (int, int) (width, height) width = characters-wide, height = rows-high
+ :param auto_size_text: (bool) if True will size the element to match the length of the text
+ :param background_color: (str) color of background
+ :param text_color: (str) color of the text
+ :param font: Union[str, tuple] specifies the font family, size, etc
+ :param key: (Any) Used with window.FindElement and with return values to uniquely identify this element
+ :param pad: (int, int) or ((int, int),(int,int)) Amount of padding to put around element (left/right, top/bottom) or ((left, right), (top, bottom))
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
+ :param change_submits: (bool) DO NOT USE. Only listed for backwards compat - Use enable_events instead
+ :param enable_events: (bool) Turns on the element specific events. Radio Button events happen when an item is selected
+ :param visible: (bool) set visibility state of the element
"""
-
+
self.InitialState = default
self.Text = text
self.TKRadio = None
@@ -1145,13 +1157,13 @@ class Radio(Element):
def Update(self, value=None, disabled=None, visible=None):
"""
+ Changes some of the settings for the Radio Button Element. Must call `Window.Read` or `Window.Finalize` prior
- :param value: Bool. if True change to selected
- :param disabled: disable or enable state of the element
- :param visible: change visibility of element
-
+ :param value: (bool) if True change to selected and set others in group to unselected
+ :param disabled: (bool) disable or enable state of the element
+ :param visible: (bool) control visibility of element
"""
-
+
if value is not None:
try:
self.TKIntVar.set(self.EncodedRadioValue)
@@ -1168,13 +1180,19 @@ class Radio(Element):
self.TKRadio.pack()
def ResetGroup(self):
- """ """
+ """
+ Sets all Radio Buttons in the group to not selected
+
+ :return:
+ """
self.TKIntVar.set(0)
def Get(self):
+ # type: (Radio) -> bool
"""
- A snapshot of the value of Radio Button (True/False)
- :return: Bool - True is radio button is chosen
+ A snapshot of the value of Radio Button -> (bool)
+
+ :return: (bool) True if this radio button is selected
"""
return self.TKIntVar.get() == self.EncodedRadioValue
@@ -1191,39 +1209,35 @@ class Radio(Element):
# ---------------------------------------------------------------------- #
# Checkbox #
# ---------------------------------------------------------------------- #
-##########################################################################
-# June 15, 2019 - This is the last element that has been converted to use the new
-# Doc strings
-# Note - The renaming of the member function to have _ if internal only has NOT yet been done!
class Checkbox(Element):
- """ """
-
+ """
+ Checkbox Element - Displays a checkbox and text next to it
+ """
def __init__(self, text, default=False, size=(None, None), auto_size_text=None, font=None, background_color=None,
text_color=None, change_submits=False, enable_events=False, disabled=False, key=None, pad=None,
tooltip=None, visible=True):
"""
- :param text: Text to display next to checkbox
- :param default: Bool + None. Initial state. True = Checked, False = unchecked, None = Not available (grayed out) (Default = False)
- :param size: (w,h) w=characters-wide, h=rows-high
- :param auto_size_text: True if size should fit the text length
- :param font: specifies the font family, size, etc
- :param background_color: color of background
- :param text_color: color of the text
- :param change_submits: If True, pressing Enter key submits window (Default = False)
- :param enable_events: Turns on the element specific events.(Default = False)
- :param disabled: set disable state for element (Default = False)
- :param key: Used with window.FindElement and with return values
- :param pad: Amount of padding to put around element
- :param tooltip: text, that will appear the you hover on
- :param visible: set visibility state of the element (Default = True)
-
+ :param text: (str) Text to display next to checkbox
+ :param default: (bool). Set to True if you want this checkbox initially checked
+ :param size: (int, int) (width, height) width = characters-wide, height = rows-high
+ :param auto_size_text: (bool) if True will size the element to match the length of the text
+ :param font: Union[str, tuple] specifies the font family, size, etc
+ :param background_color: (str) color of background
+ :param text_color: (str) color of the text
+ :param change_submits: (bool) DO NOT USE. Only listed for backwards compat - Use enable_events instead
+ :param enable_events: (bool) Turns on the element specific events. Checkbox events happen when an item changes
+ :param disabled: (bool) set disable state
+ :param key: (Any) Used with window.FindElement and with return values to uniquely identify this element
+ :param pad: (int, int) or ((int, int),(int,int)) Amount of padding to put around element (left/right, top/bottom) or ((left, right), (top, bottom))
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
+ :param visible: (bool) set visibility state of the element
"""
-
+
self.Text = text
self.InitialState = default
self.Value = None
- self.TKCheckbutton = None
+ self.TKCheckbutton = self.Widget = None # type: tk.Checkbutton
self.Disabled = disabled
self.TextColor = text_color if text_color else DEFAULT_TEXT_COLOR
self.ChangeSubmits = change_submits or enable_events
@@ -1233,18 +1247,24 @@ class Checkbox(Element):
tooltip=tooltip, visible=visible)
def Get(self):
- """ """
+ # type: (Checkbox) -> bool
+ """
+ Return the current state of this checkbox
+
+ :return: (bool) Current state of checkbox
+ """
return self.TKIntVar.get()
def Update(self, value=None, disabled=None, visible=None):
"""
+ Changes some of the settings for the Checkbox Element. Must call `Window.Read` or `Window.Finalize` prior.
+ Note that changing visibility may cause element to change locations when made visible after invisible
- :param value: Bool if True checks the checkbox
- :param disabled: disable or enable state of the element
- :param visible: change visibility of element
-
+ :param value: (bool) if True checks the checkbox, False clears it
+ :param disabled: (bool) disable or enable element
+ :param visible: (bool) control visibility of element
"""
-
+
if value is not None:
try:
self.TKIntVar.set(value)
@@ -1276,34 +1296,35 @@ Check = Checkbox
# ---------------------------------------------------------------------- #
class Spin(Element):
- """ """
-
+ """
+ A spinner with up/down buttons and a single line of text. Choose 1 values from list
+ """
+
def __init__(self, values, initial_value=None, disabled=False, change_submits=False, enable_events=False,
size=(None, None), auto_size_text=None, font=None, background_color=None, text_color=None, key=None,
pad=None, tooltip=None, visible=True):
"""
- :param values: List of valid values
- :param initial_value: String with initial value
- :param disabled: set disable state for element (Default = False)
- :param change_submits: If True, pressing Enter key submits window (Default = False)
- :param enable_events: Turns on the element specific events.(Default = False)
- :param size: (w,h) w=characters-wide, h=rows-high
- :param auto_size_text: True if size should fit the text length
- :param font: specifies the font family, size, etc
- :param background_color: color of background
- :param text_color: color of the text
- :param key: Used with window.FindElement and with return values
- :param pad: Amount of padding to put around element
- :param tooltip: text, that will appear the you hover on
- :param visible: set visibility state of the element (Default = True)
-
+ :param values: List[Any] List of valid values
+ :param initial_value: [Any] Initial item to show in window. Choose from list of values supplied
+ :param disabled: (bool) set disable state
+ :param change_submits: (bool) DO NOT USE. Only listed for backwards compat - Use enable_events instead
+ :param enable_events: (bool) Turns on the element specific events. Spin events happen when an item changes
+ :param size: (int, int) (width, height) width = characters-wide, height = rows-high
+ :param auto_size_text: (bool) if True will size the element to match the length of the text
+ :param font: Union[str, tuple] specifies the font family, size, etc
+ :param background_color: (str) color of background
+ :param text_color: (str) color of the text
+ :param key: (Any) Used with window.FindElement and with return values to uniquely identify this element
+ :param pad: (int, int) or ((int, int),(int,int)) Amount of padding to put around element (left/right, top/bottom) or ((left, right), (top, bottom))
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
+ :param visible: (bool) set visibility state of the element
"""
-
+
self.Values = values
self.DefaultValue = initial_value
self.ChangeSubmits = change_submits or enable_events
- self.TKSpinBox = None
+ self.TKSpinBox = self.Widget = None # type: tk.Spinbox
self.Disabled = disabled
bg = background_color if background_color else DEFAULT_INPUT_ELEMENTS_COLOR
fg = text_color if text_color is not None else DEFAULT_INPUT_TEXT_COLOR
@@ -1314,14 +1335,14 @@ class Spin(Element):
def Update(self, value=None, values=None, disabled=None, visible=None):
"""
+ Changes some of the settings for the Spin Element. Must call `Window.Read` or `Window.Finalize` prior
- :param value: set the current value
- :param values: set available choices
- :param disabled: disable or enable state of the element
- :param visible: change visibility of element
-
+ :param value: (Any) set the current value from list of choices
+ :param values: List[Any] set available choices
+ :param disabled: (bool) disable or enable state of the element
+ :param visible: (bool) control visibility of element
"""
-
+
if values != None:
old_value = self.TKStringVar.get()
self.Values = values
@@ -1342,14 +1363,13 @@ class Spin(Element):
elif visible is True:
self.TKSpinBox.pack()
- def SpinChangedHandler(self, event):
+ def _SpinChangedHandler(self, event):
"""
+ Callback function. Used internally only. Called by tkinter when Spinbox Widget changes. Results in Window.Read() call returning
- :param event:
-
+ :param event: passed in from tkinter
"""
# first, get the results table built
- # modify the Results table in the parent FlexForm object
if self.Key is not None:
self.ParentForm.LastButtonClicked = self.Key
else:
@@ -1359,7 +1379,13 @@ class Spin(Element):
self.ParentForm.TKroot.quit() # kick the users out of the mainloop
def Get(self):
- """ """
+ """
+ Return the current chosen value showing in spinbox.
+ This value will be the same as what was provided as list of choices. If list items are ints, then the
+ item returned will be an int (not a string)
+
+ :return: (Any) The currently visible entry
+ """
return self.TKStringVar.get()
def __del__(self):
@@ -1375,36 +1401,36 @@ class Spin(Element):
# Multiline #
# ---------------------------------------------------------------------- #
class Multiline(Element):
- """ """
-
+ """
+ Multiline Element - Display and read multiple lines of text. This is both an input and output element.
+ """
+
def __init__(self, default_text='', enter_submits=False, disabled=False, autoscroll=False, border_width=None,
size=(None, None), auto_size_text=None, background_color=None, text_color=None, change_submits=False,
enable_events=False, do_not_clear=True, key=None, focus=False, font=None, pad=None, tooltip=None,
right_click_menu=None, visible=True):
"""
- :param default_text: text to display in multiline (Default value = '')
- :param enter_submits: ???????????? (Default = False)
- :param disabled: set disable state for element (Default = False)
- :param autoscroll: ?????????? (Default = False)
- :param border_width: width of border around element
- :param size: (w,h) w=characters-wide, h=rows-high
- :param auto_size_text: True if size should fit the text length
- :param background_color: color of background
- :param text_color: color of the text
- :param change_submits: If True, pressing Enter key submits window (Default = False)
- :param enable_events: Turns on the element specific events.(Default = False)
- :param do_not_clear: see docx (Default = True)
- :param key: Used with window.FindElement and with return values
- :param focus: if focus should be set to this
- :param font: specifies the font family, size, etc
- :param pad: Amount of padding to put around element
- :param tooltip: text, that will appear the you hover on
- :param right_click_menu: see "Right Click Menus"
- :param visible: set visibility state of the element (Default = True)
-
+ :param default_text: (str) Initial text to show
+ :param enter_submits: (bool) if True, the Window.Read call will return is enter key is pressed in this element
+ :param disabled: (bool) set disable state
+ :param autoscroll: (bool) If True the contents of the element will automatically scroll as more data added to the end
+ :param border_width: (int) width of border around element in pixels
+ :param size: (int, int) (width, height) width = characters-wide, height = rows-high
+ :param auto_size_text: (bool) if True will size the element to match the length of the text
+ :param background_color: (str) color of background
+ :param text_color: (str) color of the text
+ :param change_submits: (bool) DO NOT USE. Only listed for backwards compat - Use enable_events instead
+ :param enable_events: (bool) Turns on the element specific events. Spin events happen when an item changes
+ :param do_not_clear: if False the element will be cleared any time the Window.Read call returns
+ :param key: (Any) Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element
+ :param focus: (bool) if True initial focus will go to this element
+ :param font: Union[str, tuple] specifies the font family, size, etc
+ :param pad: (int, int) or ((int, int),(int,int)) Amount of padding to put around element (left/right, top/bottom) or ((left, right), (top, bottom))
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
+ :param right_click_menu: List[List[str]] see "Right Click Menus"
+ :param visible: (bool) set visibility state of the element
"""
-
self.DefaultText = default_text
self.EnterSubmits = enter_submits
@@ -1417,6 +1443,7 @@ class Multiline(Element):
self.ChangeSubmits = change_submits or enable_events
self.RightClickMenu = right_click_menu
self.BorderWidth = border_width if border_width is not None else DEFAULT_BORDER_WIDTH
+ self.TKText = self.Widget = None # type: tk.scrolledtext.ScrolledText
super().__init__(ELEM_TYPE_INPUT_MULTILINE, size=size, auto_size_text=auto_size_text, background_color=bg,
text_color=fg, key=key, pad=pad, tooltip=tooltip, font=font or DEFAULT_FONT, visible=visible)
@@ -1425,18 +1452,18 @@ class Multiline(Element):
def Update(self, value=None, disabled=None, append=False, font=None, text_color=None, background_color=None,
visible=None, autoscroll=None):
"""
+ Changes some of the settings for the Multiline Element. Must call `Window.Read` or `Window.Finalize` prior
- :param value: new text to display
- :param disabled: disable or enable state of the element
- :param append: (Default = False)
- :param font: specifies the font family, size, etc
- :param text_color: color of the text
- :param background_color: color of background
- :param visible: change visibility of element
- :param autoscroll: ??????????????????
-
+ :param value: (str) new text to display
+ :param disabled: (bool) disable or enable state of the element
+ :param append: (bool) if True then new value will be added onto the end of the current value. if False then contents will be replaced.
+ :param font: Union[str, tuple] specifies the font family, size, etc
+ :param text_color: (str) color of the text
+ :param background_color: (str) color of background
+ :param visible: (bool) set visibility state of the element
+ :param autoscroll: (bool) if True then contents of element are scrolled down when new text is added to the end
"""
-
+
if autoscroll is not None:
self.Autoscroll = autoscroll
if value is not None:
@@ -1469,11 +1496,20 @@ class Multiline(Element):
self.TKText.pack()
def Get(self):
- """ """
+ """
+ Return current contents of the Multiline Element
+
+ :return: (str) current contents of the Multiline Input element
+ """
+
return self.TKText.get(1.0, tk.END)
def SetFocus(self, force=False):
- """ """
+ """
+ Moves the focus to this Multiline
+
+ :param force: (bool). If True, will call focus_force instead of focus_set
+ """
try:
if force:
self.TKText.focus_force()
@@ -1482,7 +1518,6 @@ class Multiline(Element):
except:
pass
-
def __del__(self):
""" """
super().__del__()
@@ -1493,32 +1528,32 @@ class Multiline(Element):
# ---------------------------------------------------------------------- #
class Text(Element):
"""
- Text - Display some text in the window. Can be single or multiple lines but no scrolling if multiple lines.
+ Text - Display some text in the window. Usually this means a single line of text. However, the text can also
+ be multiple lines. If multi-lined there are no scroll bars.
"""
-
+
def __init__(self, text, size=(None, None), auto_size_text=None, click_submits=False, enable_events=False,
relief=None, font=None, text_color=None, background_color=None, justification=None, pad=None, key=None,
right_click_menu=None, tooltip=None, visible=True):
"""
- :param text: The text to display (required)
- :param size: (w,h) w=characters-wide, h=rows-high
- :param auto_size_text: True if size should fit the text length
- :param click_submits: ????????????????????(Default = False)
- :param enable_events: Turns on the element specific events.(Default = False)
- :param relief: relief style. Values are same as progress meter relief values. Can be a constant or a string: `RELIEF_RAISED RELIEF_SUNKEN RELIEF_FLAT RELIEF_RIDGE RELIEF_GROOVE RELIEF_SOLID`
- :param font: specifies the font family, size, etc
- :param text_color: color of the text
- :param background_color: color of background
- :param justification: justification for data display
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
- :param right_click_menu: see "Right Click Menus"
- :param tooltip: text, that will appear the you hover on
- :param visible: set visibility state of the element (Default = True)
-
+ :param text: (str) The text to display. Can include /n to achieve multiple lines
+ :param size: (int, int) (width, height) width = characters-wide, height = rows-high
+ :param auto_size_text: (bool) if True size of the Text Element will be sized to fit the string provided in 'text' parm
+ :param click_submits: (bool) DO NOT USE. Only listed for backwards compat - Use enable_events instead
+ :param enable_events: (bool) Turns on the element specific events. Text events happen when the text is clicked
+ :param relief: (str/enum) relief style around the text. Values are same as progress meter relief values. Should be a constant that is defined at starting with "RELIEF_" - `RELIEF_RAISED, RELIEF_SUNKEN, RELIEF_FLAT, RELIEF_RIDGE, RELIEF_GROOVE, RELIEF_SOLID`
+ :param font: Union[str, tuple] specifies the font family, size, etc
+ :param text_color: (str) color of the text
+ :param background_color: (str) color of background
+ :param justification: (str) how string should be aligned within space provided by size. Valid choices = `left`, `right`, `center`
+ :param pad: (int, int) or ((int, int),(int,int)) Amount of padding to put around element (left/right, top/bottom) or ((left, right), (top, bottom))
+ :param key: (Any) Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element
+ :param right_click_menu: List[List[str]] see "Right Click Menus"
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
+ :param visible: (bool) set visibility state of the element
"""
-
+
self.DisplayText = str(text)
self.TextColor = text_color if text_color else DEFAULT_TEXT_COLOR
self.Justification = justification
@@ -1537,13 +1572,13 @@ class Text(Element):
def Update(self, value=None, background_color=None, text_color=None, font=None, visible=None):
"""
+ Changes some of the settings for the Text Element. Must call `Window.Read` or `Window.Finalize` prior
- :param value: new value to show
- :param background_color: color of background
- :param text_color: color of the text
- :param font: specifies the font family, size, etc
- :param visible: change visibility of element
-
+ :param value: (str) new text to show
+ :param background_color: (str) color of background
+ :param text_color: (str) color of the text
+ :param font: Union[str, tuple] specifies the font family, size, etc
+ :param visible: (bool) set visibility state of the element
"""
if value is not None:
@@ -1576,29 +1611,29 @@ T = Text
# ---------------------------------------------------------------------- #
class StatusBar(Element):
""" """
-
+
def __init__(self, text, size=(None, None), auto_size_text=None, click_submits=None, enable_events=False,
relief=RELIEF_SUNKEN, font=None, text_color=None, background_color=None, justification=None, pad=None,
key=None, tooltip=None, visible=True):
"""
:param text: (required) text that is to be displayed in the widget
- :param size: (w,h) w=characters-wide, h=rows-high
- :param auto_size_text: True if size should fit the text length
+ :param size: (w,h) w=characters-wide, h=rows-high
+ :param auto_size_text: True if size should fit the text length
:param click_submits: ????????????????????
:param enable_events: Turns on the element specific events.(Default = False)
:param relief: relief style. Values are same as progress meter relief values. Can be a constant or a string: `RELIEF_RAISED RELIEF_SUNKEN RELIEF_FLAT RELIEF_RIDGE RELIEF_GROOVE RELIEF_SOLID` (Default value = RELIEF_SUNKEN)
- :param font: specifies the font family, size, etc
- :param text_color: color of the text
- :param background_color: color of background
- :param justification: justification for data display
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
- :param tooltip: text, that will appear the you hover on
+ :param font: specifies the font family, size, etc
+ :param text_color: color of the text
+ :param background_color: color of background
+ :param justification: justification for data display
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
:param visible: set visibility state of the element (Default = True)
"""
-
+
self.DisplayText = text
self.TextColor = text_color if text_color else DEFAULT_TEXT_COLOR
self.Justification = justification
@@ -1608,6 +1643,7 @@ class StatusBar(Element):
bg = DEFAULT_TEXT_ELEMENT_BACKGROUND_COLOR
else:
bg = background_color
+ self.TKText = self.Widget = None # type: tk.Label
super().__init__(ELEM_TYPE_STATUSBAR, size=size, auto_size_text=auto_size_text, background_color=bg,
font=font or DEFAULT_FONT, text_color=self.TextColor, pad=pad, key=key, tooltip=tooltip,
visible=visible)
@@ -1616,15 +1652,15 @@ class StatusBar(Element):
def Update(self, value=None, background_color=None, text_color=None, font=None, visible=None):
"""
+ Changes some of the settings for the Status Bar Element. Must call `Window.Read` or `Window.Finalize` prior
:param value: ??????????????????????????
- :param background_color: color of background
- :param text_color: color of the text
- :param font: specifies the font family, size, etc
- :param visible: change visibility of element
-
+ :param background_color: color of background
+ :param text_color: color of the text
+ :param font: specifies the font family, size, etc
+ :param visible: (bool) control visibility of element
"""
-
+
if value is not None:
self.DisplayText = value
stringvar = self.TKStringVar
@@ -1667,7 +1703,7 @@ class TKProgressBar():
:param border_width: (Default value = DEFAULT_PROGRESS_BAR_BORDER_WIDTH)
:param orientation: 'horizontal' or 'vertical' ('h' or 'v' work) (Default value = 'vertical')(Default value = 'horizontal')
:param BarColor: ????????????????????????
- :param key: Used with window.FindElement and with return values
+ :param key: Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element
"""
@@ -1744,14 +1780,14 @@ class TKOutput(tk.Frame):
def __init__(self, parent, width, height, bd, background_color=None, text_color=None, font=None, pad=None):
"""
- :param parent:
- :param width:
- :param height:
- :param bd:
- :param background_color: color of background
- :param text_color: color of the text
- :param font: specifies the font family, size, etc
- :param pad: Amount of padding to put around element
+ :param parent:
+ :param width:
+ :param height:
+ :param bd:
+ :param background_color: color of background
+ :param text_color: color of the text
+ :param font: specifies the font family, size, etc
+ :param pad: Amount of padding to put around element
"""
self.frame = tk.Frame(parent)
@@ -1777,7 +1813,7 @@ class TKOutput(tk.Frame):
def write(self, txt):
"""
- :param txt:
+ :param txt:
"""
try:
@@ -1808,23 +1844,23 @@ class TKOutput(tk.Frame):
# ---------------------------------------------------------------------- #
class Output(Element):
""" """
-
+
def __init__(self, size=(None, None), background_color=None, text_color=None, pad=None, font=None, tooltip=None,
key=None, right_click_menu=None, visible=True):
"""
- :param size: (w,h) w=characters-wide, h=rows-high
- :param background_color: color of background
- :param text_color: color of the text
- :param pad: Amount of padding to put around element
- :param font: specifies the font family, size, etc
- :param tooltip: text, that will appear the you hover on
- :param key: Used with window.FindElement and with return values
- :param right_click_menu: see "Right Click Menus"
+ :param size: (w,h) w=characters-wide, h=rows-high
+ :param background_color: color of background
+ :param text_color: color of the text
+ :param pad: Amount of padding to put around element
+ :param font: specifies the font family, size, etc
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element
+ :param right_click_menu: List[List[str]] see "Right Click Menus" for format
:param visible: set visibility state of the element (Default = True)
"""
-
+
self._TKOut = None
bg = background_color if background_color else DEFAULT_INPUT_ELEMENTS_COLOR
fg = text_color if text_color is not None else DEFAULT_INPUT_TEXT_COLOR
@@ -1843,12 +1879,12 @@ class Output(Element):
def Update(self, value=None, visible=None):
"""
+ Changes some of the settings for the Output Element. Must call `Window.Read` or `Window.Finalize` prior
- :param value:
- :param visible: change visibility of element
-
+ :param value:
+ :param visible: (bool) control visibility of element
"""
-
+
if value is not None:
self._TKOut.output.delete('1.0', tk.END)
self._TKOut.output.insert(tk.END, value)
@@ -1871,7 +1907,7 @@ class Output(Element):
# ---------------------------------------------------------------------- #
class Button(Element):
""" """
-
+
def __init__(self, button_text='', button_type=BUTTON_TYPE_READ_FORM, target=(None, None), tooltip=None,
file_types=(("ALL Files", "*.*"),), initial_folder=None, disabled=False, change_submits=False,
enable_events=False, image_filename=None, image_data=None, image_size=(None, None),
@@ -1882,29 +1918,29 @@ class Button(Element):
:param button_text: Text to be displayed on the button (Default value = '')
:param button_type: You should NOT be setting this directly (Default value = BUTTON_TYPE_READ_FORM)
:param target: key or (row,col) target for the button
- :param tooltip: text, that will appear the you hover on
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
:param file_types: the filetypes that will be used to match files (Default value = (("ALL Files", "*.*"),))
:param initial_folder: starting path for folders and files
:param disabled: set disable state for element (Default = False)
:param change_submits: If True, pressing Enter key submits window (Default = False)
:param enable_events: Turns on the element specific events.(Default = False)
:param image_filename: image filename if there is a button image
- :param image_data: in-RAM image to be displayed on button
+ :param image_data: in-RAM image to be displayed on button
:param image_size: size of button image in pixels (Default = (None))
- :param image_subsample:amount to reduce the size of the image
+ :param image_subsample:amount to reduce the size of the image
:param border_width: width of border around button in pixels
:param size: (w,h) w=characters-wide, h=rows-high (Default = (None))
:param auto_size_button: True if button size is determined by button text
:param button_color: (text color, backound color)
- :param font: specifies the font family, size, etc
+ :param font: specifies the font family, size, etc
:param bind_return_key: If True the return key will cause this button to fire (Default = False)
- :param focus: if focus should be set to this
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
+ :param focus: if focus should be set to this
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element
:param visible: set visibility state of the element (Default = True)
"""
-
+
self.AutoSizeButton = auto_size_button
self.BType = button_type
self.FileTypes = file_types
@@ -1936,7 +1972,7 @@ class Button(Element):
def ButtonReleaseCallBack(self, parm):
"""
- :param parm:
+ :param parm:
"""
self.LastButtonClickedWasRealtime = False
@@ -1946,7 +1982,7 @@ class Button(Element):
def ButtonPressCallBack(self, parm):
"""
- :param parm:
+ :param parm:
"""
self.ParentForm.LastButtonClickedWasRealtime = True
@@ -2100,18 +2136,18 @@ class Button(Element):
def Update(self, text=None, button_color=(None, None), disabled=None, image_data=None, image_filename=None,
visible=None, image_subsample=None, image_size=None):
"""
+ Changes some of the settings for the Button Element. Must call `Window.Read` or `Window.Finalize` prior
:param text: sets button text
:param button_color: (text, background) (Default = (None))
- :param disabled: disable or enable state of the element
- :param image_data: in-RAM image to be displayed on button
+ :param disabled: (bool) disable or enable state of the element
+ :param image_data: in-RAM image to be displayed on button
:param image_filename: image filename if there is a button image
- :param visible: change visibility of element
- :param image_subsample:amount to reduce the size of the image
- :param image_size:
-
+ :param visible: (bool) control visibility of element
+ :param image_subsample:amount to reduce the size of the image
+ :param image_size:
"""
-
+
try:
if text is not None:
self.TKButton.configure(text=text)
@@ -2201,7 +2237,7 @@ Butt = Button
# ---------------------------------------------------------------------- #
class ButtonMenu(Element):
""" """
-
+
def __init__(self, button_text, menu_def, tooltip=None, disabled=False,
image_filename=None, image_data=None, image_size=(None, None), image_subsample=None, border_width=None,
size=(None, None), auto_size_button=None, button_color=None, font=None, pad=None, key=None,
@@ -2210,24 +2246,24 @@ class ButtonMenu(Element):
:param button_text: Text to be displayed on the button (Default value = '')
:param menu_def: ??????????????????
- :param tooltip: text, that will appear the you hover on
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
:param disabled: set disable state for element (Default = False)
:param image_filename: image filename if there is a button image
- :param image_data: in-RAM image to be displayed on button
+ :param image_data: in-RAM image to be displayed on button
:param image_size: size of button image in pixels (Default = (None))
- :param image_subsample:amount to reduce the size of the image
+ :param image_subsample:amount to reduce the size of the image
:param border_width: width of border around button in pixels
:param size: (w,h) w=characters-wide, h=rows-high (Default = (None))
:param auto_size_button: True if button size is determined by button text
:param button_color: (text color, backound color)
- :param font: specifies the font family, size, etc
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
+ :param font: specifies the font family, size, etc
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element
:param tearoff: ?????????????????? (Default = False)
:param visible: set visibility state of the element (Default = True)
"""
-
+
self.MenuDefinition = menu_def
self.AutoSizeButton = auto_size_button
self.ButtonText = button_text
@@ -2254,7 +2290,7 @@ class ButtonMenu(Element):
def _MenuItemChosenCallback(self, item_chosen): # ButtonMenu Menu Item Chosen Callback
"""
- :param item_chosen:
+ :param item_chosen:
"""
# print('IN MENU ITEM CALLBACK', item_chosen)
@@ -2266,12 +2302,12 @@ class ButtonMenu(Element):
def Update(self, menu_definition, visible=None):
"""
+ Changes some of the settings for the ButtonMenu Element. Must call `Window.Read` or `Window.Finalize` prior
- :param menu_definition: ??????????????????
- :param visible: change visibility of element
-
+ :param menu_definition: (List[List]) New menu definition (in menu definition format)
+ :param visible: (bool) control visibility of element
"""
-
+
self.MenuDefinition = menu_definition
if menu_definition is not None:
self.TKMenu = tk.Menu(self.TKButtonMenu, tearoff=self.Tearoff) # create the menubar
@@ -2302,18 +2338,18 @@ class ProgressBar(Element):
:param max_value: max value of progressbar
:param orientation: 'horizontal' or 'vertical' ('h' or 'v' work) (Default value = 'vertical')
- :param size: (w,h) w=characters-wide, h=rows-high
- :param auto_size_text: True if size should fit the text length
+ :param size: (w,h) w=characters-wide, h=rows-high
+ :param auto_size_text: True if size should fit the text length
:param bar_color: (Default = (None))
:param style: ????????????????????????????
- :param border_width: width of border around button
+ :param border_width: width of border around button
:param relief: ????????????????????????????
- :param key: Used with window.FindElement and with return values
- :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element
+ :param pad: Amount of padding to put around element
:param visible: set visibility state of the element (Default = True)
"""
-
+
self.MaxValue = max_value
self.TKProgressBar = None
self.Cancelled = False
@@ -2335,7 +2371,7 @@ class ProgressBar(Element):
:param max: changes the max value
"""
-
+
if self.ParentForm.TKrootDestroyed:
return False
self.TKProgressBar.Update(current_count, max=max)
@@ -2349,11 +2385,11 @@ class ProgressBar(Element):
def Update(self, visible=None):
"""
+ Changes some of the settings for the ProgressBar Element. Must call `Window.Read` or `Window.Finalize` prior
- :param visible: change visibility of element
-
+ :param visible: (bool) control visibility of element
"""
-
+
if visible is False:
self.TKProgressBar.TKProgressBarForReal.pack_forget()
elif visible is True:
@@ -2373,24 +2409,24 @@ class ProgressBar(Element):
# ---------------------------------------------------------------------- #
class Image(Element):
""" """
-
+
def __init__(self, filename=None, data=None, background_color=None, size=(None, None), pad=None, key=None,
tooltip=None, right_click_menu=None, visible=True, enable_events=False):
"""
:param filename: file name if the image is in a file
:param data: if image is in RAM (PIL format?)
- :param background_color: color of background
- :param size: (w,h) w=characters-wide, h=rows-high
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
- :param tooltip: text, that will appear the you hover on
- :param right_click_menu: see "Right Click Menus"
+ :param background_color: color of background
+ :param size: (w,h) w=characters-wide, h=rows-high
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
+ :param right_click_menu: List[List[str]] see "Right Click Menus" for format
:param visible: set visibility state of the element (Default = True)
:param enable_events: Turns on the element specific events.(Default = False)
"""
-
+
self.Filename = filename
self.Data = data
self.tktext_label = None
@@ -2411,14 +2447,14 @@ class Image(Element):
def Update(self, filename=None, data=None, size=(None, None), visible=None):
"""
+ Changes some of the settings for the Image Element. Must call `Window.Read` or `Window.Finalize` prior
- :param filename:
- :param data:
- :param size: (w,h) w=characters-wide, h=rows-high
- :param visible: change visibility of element
-
+ :param filename:
+ :param data:
+ :param size: (w,h) w=characters-wide, h=rows-high
+ :param visible: (bool) control visibility of element
"""
-
+
if filename is not None:
image = tk.PhotoImage(file=filename)
elif data is not None:
@@ -2443,11 +2479,11 @@ class Image(Element):
def UpdateAnimation(self, source, time_between_frames=0):
"""
- :param source:
+ :param source:
:param time_between_frames: (Default value = 0)
"""
-
+
if self.Source != source:
self.AnimatedFrames = None
self.Source = source
@@ -2497,22 +2533,22 @@ class Image(Element):
# ---------------------------------------------------------------------- #
class Canvas(Element):
""" """
-
+
def __init__(self, canvas=None, background_color=None, size=(None, None), pad=None, key=None, tooltip=None,
right_click_menu=None, visible=True):
"""
:param canvas: ????????????????????????
- :param background_color: color of background
- :param size: (w,h) w=characters-wide, h=rows-high
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
- :param tooltip: text, that will appear the you hover on
- :param right_click_menu: see "Right Click Menus"
+ :param background_color: color of background
+ :param size: (w,h) w=characters-wide, h=rows-high
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
+ :param right_click_menu: List[List[str]] see "Right Click Menus" for format
:param visible: set visibility state of the element (Default = True)
"""
-
+
self.BackgroundColor = background_color if background_color is not None else DEFAULT_BACKGROUND_COLOR
self._TKCanvas = canvas
self.RightClickMenu = right_click_menu
@@ -2539,7 +2575,7 @@ class Canvas(Element):
# ---------------------------------------------------------------------- #
class Graph(Element):
""" """
-
+
def __init__(self, canvas_size, graph_bottom_left, graph_top_right, background_color=None, pad=None,
change_submits=False, drag_submits=False, enable_events=False, key=None, tooltip=None,
right_click_menu=None, visible=True, float_values=False):
@@ -2548,19 +2584,19 @@ class Graph(Element):
:param canvas_size: ????????????????????????
:param graph_bottom_left: ????????????????????????
:param graph_top_right: ????????????????????????
- :param background_color: color of background
- :param pad: Amount of padding to put around element
+ :param background_color: color of background
+ :param pad: Amount of padding to put around element
:param change_submits: If True, pressing Enter key submits window (Default = False)
:param drag_submits: ???????????????????????? (Default = False)
:param enable_events: Turns on the element specific events.(Default = False)
- :param key: Used with window.FindElement and with return values
- :param tooltip: text, that will appear the you hover on
- :param right_click_menu: see "Right Click Menus"
+ :param key: Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
+ :param right_click_menu: List[List[str]] see "Right Click Menus" for format
:param visible: set visibility state of the element (Default = True)
:param float_values: bool: If True x,y coordinates are returned as floats, not ints
"""
-
+
self.CanvasSize = canvas_size
self.BottomLeft = graph_bottom_left
self.TopRight = graph_top_right
@@ -2581,8 +2617,8 @@ class Graph(Element):
def _convert_xy_to_canvas_xy(self, x_in, y_in):
"""
- :param x_in:
- :param y_in:
+ :param x_in:
+ :param y_in:
"""
if None in (x_in, y_in):
@@ -2596,8 +2632,8 @@ class Graph(Element):
def _convert_canvas_xy_to_xy(self, x_in, y_in):
"""
- :param x_in:
- :param y_in:
+ :param x_in:
+ :param y_in:
"""
if None in (x_in, y_in):
@@ -2615,8 +2651,8 @@ class Graph(Element):
def DrawLine(self, point_from, point_to, color='black', width=1):
"""
- :param point_from:
- :param point_to:
+ :param point_from:
+ :param point_to:
:param color: (Default value = 'black')
:param width: (Default value = 1)
@@ -2638,7 +2674,7 @@ class Graph(Element):
def DrawPoint(self, point, size=2, color='black'):
"""
- :param point:
+ :param point:
:param size: (w,h) w=characters-wide, h=rows-high (Default value = 2)
:param color: (Default value = 'black')
@@ -2661,9 +2697,9 @@ class Graph(Element):
def DrawCircle(self, center_location, radius, fill_color=None, line_color='black'):
"""
- :param center_location:
- :param radius:
- :param fill_color:
+ :param center_location:
+ :param radius:
+ :param fill_color:
:param line_color: (Default value = 'black')
"""
@@ -2685,10 +2721,10 @@ class Graph(Element):
def DrawOval(self, top_left, bottom_right, fill_color=None, line_color=None):
"""
- :param top_left:
- :param bottom_right:
- :param fill_color:
- :param line_color:
+ :param top_left:
+ :param bottom_right:
+ :param fill_color:
+ :param line_color:
"""
converted_top_left = self._convert_xy_to_canvas_xy(top_left[0], top_left[1])
@@ -2708,11 +2744,11 @@ class Graph(Element):
def DrawArc(self, top_left, bottom_right, extent, start_angle, style=None, arc_color='black'):
"""
- :param top_left:
- :param bottom_right:
- :param extent:
- :param start_angle:
- :param style:
+ :param top_left:
+ :param bottom_right:
+ :param extent:
+ :param start_angle:
+ :param style:
:param arc_color: (Default value = 'black')
"""
@@ -2734,10 +2770,10 @@ class Graph(Element):
def DrawRectangle(self, top_left, bottom_right, fill_color=None, line_color=None):
"""
- :param top_left:
- :param bottom_right:
- :param fill_color:
- :param line_color:
+ :param top_left:
+ :param bottom_right:
+ :param fill_color:
+ :param line_color:
"""
converted_top_left = self._convert_xy_to_canvas_xy(top_left[0], top_left[1])
@@ -2757,10 +2793,10 @@ class Graph(Element):
def DrawText(self, text, location, color='black', font=None, angle=0):
"""
- :param text:
- :param location:
+ :param text:
+ :param location:
:param color: (Default value = 'black')
- :param font: specifies the font family, size, etc
+ :param font: specifies the font family, size, etc
:param angle: (Default value = 0)
"""
@@ -2781,11 +2817,11 @@ class Graph(Element):
def DrawImage(self, filename=None, data=None, location=(None, None), color='black', font=None, angle=0):
"""
- :param filename:
- :param data:
- :param location:
+ :param filename:
+ :param data:
+ :param location:
:param color: (Default value = 'black')
- :param font: specifies the font family, size, etc
+ :param font: specifies the font family, size, etc
:param angle: (Default value = 0)
"""
@@ -2826,7 +2862,7 @@ class Graph(Element):
def DeleteFigure(self, id):
"""
- :param id:
+ :param id:
"""
try:
@@ -2839,10 +2875,10 @@ class Graph(Element):
def Update(self, background_color, visible=None):
"""
+ Changes some of the settings for the Graph Element. Must call `Window.Read` or `Window.Finalize` prior
:param background_color: color of background
- :param visible: change visibility of element
-
+ :param visible: (bool) control visibility of element
"""
if self._TKCanvas2 is None:
print('*** WARNING - The Graph element has not been finalized and cannot be drawn upon ***')
@@ -2857,8 +2893,8 @@ class Graph(Element):
def Move(self, x_direction, y_direction):
"""
- :param x_direction:
- :param y_direction:
+ :param x_direction:
+ :param y_direction:
"""
zero_converted = self._convert_xy_to_canvas_xy(0, 0)
@@ -2873,9 +2909,9 @@ class Graph(Element):
def MoveFigure(self, figure, x_direction, y_direction):
"""
- :param figure:
- :param x_direction:
- :param y_direction:
+ :param figure:
+ :param x_direction:
+ :param y_direction:
"""
zero_converted = self._convert_xy_to_canvas_xy(0, 0)
@@ -2890,9 +2926,9 @@ class Graph(Element):
def RelocateFigure(self, figure, x, y):
"""
- :param figure:
- :param x:
- :param y:
+ :param figure:
+ :param x:
+ :param y:
"""
zero_converted = self._convert_xy_to_canvas_xy(0, 0)
@@ -2917,7 +2953,7 @@ class Graph(Element):
def ButtonReleaseCallBack(self, event):
"""
- :param event:
+ :param event:
"""
self.ClickPosition = (None, None)
@@ -2936,7 +2972,7 @@ class Graph(Element):
def ButtonPressCallBack(self, event):
"""
- :param event:
+ :param event:
"""
self.ClickPosition = self._convert_canvas_xy_to_xy(event.x, event.y)
@@ -2953,7 +2989,7 @@ class Graph(Element):
def MotionCallBack(self, event):
"""
- :param event:
+ :param event:
"""
if not self.MouseButtonDown:
@@ -2988,7 +3024,7 @@ class Graph(Element):
# ---------------------------------------------------------------------- #
class Frame(Element):
""" """
-
+
def __init__(self, title, layout, title_color=None, background_color=None, title_location=None,
relief=DEFAULT_FRAME_RELIEF, size=(None, None), font=None, pad=None, border_width=None, key=None,
tooltip=None, right_click_menu=None, visible=True):
@@ -3000,17 +3036,17 @@ class Frame(Element):
:param background_color: color of background
:param title_location: ????????????????????????
:param relief: relief style. Values are same as progress meter relief values. Can be a constant or a string: `RELIEF_RAISED RELIEF_SUNKEN RELIEF_FLAT RELIEF_RIDGE RELIEF_GROOVE RELIEF_SOLID` (Default value = DEFAULT_FRAME_RELIEF)
- :param size: (w,h) w=characters-wide, h=rows-high
- :param font: specifies the font family, size, etc
- :param pad: Amount of padding to put around element
- :param border_width: width of border around element
- :param key: Used with window.FindElement and with return values
- :param tooltip: text, that will appear the you hover on
- :param right_click_menu: see "Right Click Menus"
+ :param size: (w,h) w=characters-wide, h=rows-high
+ :param font: specifies the font family, size, etc
+ :param pad: Amount of padding to put around element
+ :param border_width: width of border around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
+ :param right_click_menu: List[List[str]] see "Right Click Menus" for format
:param visible: set visibility state of the element (Default = True)
"""
-
+
self.UseDictionary = False
self.ReturnValues = None
self.ReturnValuesList = []
@@ -3037,7 +3073,7 @@ class Frame(Element):
def AddRow(self, *args):
"""Parms are a variable number of Elements
- :param *args:
+ :param *args:
"""
NumRows = len(self.Rows) # number of existing rows is our row number
@@ -3056,7 +3092,7 @@ class Frame(Element):
def Layout(self, rows):
"""
- :param rows:
+ :param rows:
"""
for row in rows:
@@ -3065,7 +3101,7 @@ class Frame(Element):
def _GetElementAtLocation(self, location):
"""
- :param location:
+ :param location:
"""
(row_num, col_num) = location
@@ -3075,11 +3111,11 @@ class Frame(Element):
def Update(self, visible=None):
"""
+ Changes some of the settings for the Frame Element. Must call `Window.Read` or `Window.Finalize` prior
- :param visible: change visibility of element
-
+ :param visible: (bool) control visibility of element
"""
-
+
if visible is False:
self.TKFrame.pack_forget()
elif visible is True:
@@ -3099,14 +3135,14 @@ class Frame(Element):
# ---------------------------------------------------------------------- #
class VerticalSeparator(Element):
""" """
-
+
def __init__(self, pad=None):
"""
- :param pad: Amount of padding to put around element
+ :param pad: Amount of padding to put around element
"""
-
+
self.Orientation = 'vertical' # for now only vertical works
super().__init__(ELEM_TYPE_SEPARATOR, pad=pad)
@@ -3125,26 +3161,26 @@ VSep = VerticalSeparator
# ---------------------------------------------------------------------- #
class Tab(Element):
""" """
-
+
def __init__(self, title, layout, title_color=None, background_color=None, font=None, pad=None, disabled=False,
border_width=None, key=None, tooltip=None, right_click_menu=None, visible=True):
"""
- :param title:
+ :param title:
:param layout: ?????????????????????
- :param title_color:
- :param background_color: color of background
- :param font: specifies the font family, size, etc
- :param pad: Amount of padding to put around element
+ :param title_color:
+ :param background_color: color of background
+ :param font: specifies the font family, size, etc
+ :param pad: Amount of padding to put around element
:param disabled: set disable state for element (Default = False)
:param border_width: width of border around element
- :param key: Used with window.FindElement and with return values
- :param tooltip: text, that will appear the you hover on
- :param right_click_menu: see "Right Click Menus"
+ :param key: Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
+ :param right_click_menu: List[List[str]] see "Right Click Menus" for format
:param visible: set visibility state of the element (Default = True)
"""
-
+
self.UseDictionary = False
self.ReturnValues = None
self.ReturnValuesList = []
@@ -3171,7 +3207,7 @@ class Tab(Element):
def AddRow(self, *args):
"""Parms are a variable number of Elements
- :param *args:
+ :param *args:
"""
NumRows = len(self.Rows) # number of existing rows is our row number
@@ -3190,7 +3226,7 @@ class Tab(Element):
def Layout(self, rows):
"""
- :param rows:
+ :param rows:
"""
for row in rows:
@@ -3199,12 +3235,12 @@ class Tab(Element):
def Update(self, disabled=None, visible=None): # TODO Disable / enable of tabs is not complete
"""
+ Changes some of the settings for the Tab Element. Must call `Window.Read` or `Window.Finalize` prior
- :param disabled: disable or enable state of the element
- :param visible: change visibility of element
-
+ :param disabled: (bool) disable or enable state of the element
+ :param visible: (bool) control visibility of element
"""
-
+
if disabled is None:
return
self.Disabled = disabled
@@ -3219,7 +3255,7 @@ class Tab(Element):
def _GetElementAtLocation(self, location):
"""
- :param location:
+ :param location:
"""
(row_num, col_num) = location
@@ -3240,29 +3276,29 @@ class Tab(Element):
# ---------------------------------------------------------------------- #
class TabGroup(Element):
""" """
-
+
def __init__(self, layout, tab_location=None, title_color=None, selected_title_color=None, background_color=None,
font=None, change_submits=False, enable_events=False, pad=None, border_width=None, theme=None,
key=None, tooltip=None, visible=True):
"""
- :param layout:
- :param tab_location:
- :param title_color:
- :param selected_title_color:
- :param background_color: color of background
- :param font: specifies the font family, size, etc
+ :param layout:
+ :param tab_location:
+ :param title_color:
+ :param selected_title_color:
+ :param background_color: color of background
+ :param font: specifies the font family, size, etc
:param change_submits: If True, pressing Enter key submits window (Default = False)
:param enable_events: Turns on the element specific events.(Default = False)
- :param pad: Amount of padding to put around element
+ :param pad: Amount of padding to put around element
:param border_width: width of border around element
- :param theme:
- :param key: Used with window.FindElement and with return values
- :param tooltip: text, that will appear the you hover on
+ :param theme:
+ :param key: Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
:param visible: set visibility state of the element (Default = True)
"""
-
+
self.UseDictionary = False
self.ReturnValues = None
self.ReturnValuesList = []
@@ -3289,7 +3325,7 @@ class TabGroup(Element):
def AddRow(self, *args):
"""Parms are a variable number of Elements
- :param *args:
+ :param *args:
"""
NumRows = len(self.Rows) # number of existing rows is our row number
@@ -3308,7 +3344,7 @@ class TabGroup(Element):
def Layout(self, rows):
"""
- :param rows:
+ :param rows:
"""
for row in rows:
@@ -3317,7 +3353,7 @@ class TabGroup(Element):
def _GetElementAtLocation(self, location):
"""
- :param location:
+ :param location:
"""
(row_num, col_num) = location
@@ -3328,7 +3364,7 @@ class TabGroup(Element):
def FindKeyFromTabName(self, tab_name):
"""
- :param tab_name:
+ :param tab_name:
"""
for row in self.Rows:
@@ -3340,7 +3376,7 @@ class TabGroup(Element):
def SelectTab(self, index):
"""
- :param index:
+ :param index:
"""
try:
@@ -3361,17 +3397,17 @@ class TabGroup(Element):
# ---------------------------------------------------------------------- #
class Slider(Element):
""" """
-
+
def __init__(self, range=(None, None), default_value=None, resolution=None, tick_interval=None, orientation=None,
disable_number_display=False, border_width=None, relief=None, change_submits=False,
enable_events=False, disabled=False, size=(None, None), font=None, background_color=None,
text_color=None, key=None, pad=None, tooltip=None, visible=True):
"""
- :param range: (min, max) slider's range
- :param default_value: default setting (within range)
+ :param range: (min, max) slider's range
+ :param default_value: default setting (within range)
:param resolution: how much each 'tick' should represent. Default = 1
- :param tick_interval:
+ :param tick_interval:
:param orientation: 'horizontal' or 'vertical' ('h' or 'v' work)
:param disable_number_display: (Default = False)
:param border_width: width of border around element
@@ -3380,16 +3416,16 @@ class Slider(Element):
:param enable_events: Turns on the element specific events.(Default = False)
:param disabled: set disable state for element (Default = False)
:param size: (w,h) w=characters-wide, h=rows-high (Default = (None))
- :param font: specifies the font family, size, etc
- :param background_color: color of background
- :param text_color: color of the text
- :param key: Used with window.FindElement and with return values
- :param pad: Amount of padding to put around element
- :param tooltip: text, that will appear the you hover on
+ :param font: specifies the font family, size, etc
+ :param background_color: color of background
+ :param text_color: color of the text
+ :param key: Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element
+ :param pad: Amount of padding to put around element
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
:param visible: set visibility state of the element (Default = True)
"""
-
+
self.TKScale = None # type: tk.Scale
self.Range = (1, 10) if range == (None, None) else range
self.DefaultValue = self.Range[0] if default_value is None else default_value
@@ -3411,14 +3447,15 @@ class Slider(Element):
def Update(self, value=None, range=(None, None), disabled=None, visible=None):
"""
+ Changes some of the settings for the Slider Element. Must call `Window.Read` or `Window.Finalize` prior
:param value: set current selection to value new value in slider
:param range: new range in slider
- :param disabled: disable or enable state of the element
- :param visible: change visibility of element
+ :param disabled: (bool) disable or enable state of the element
+ :param visible: (bool) control visibility of element
"""
-
+
if value is not None:
try:
self.TKIntVar.set(value)
@@ -3439,7 +3476,7 @@ class Slider(Element):
def _SliderChangedHandler(self, event):
"""
- :param event:
+ :param event:
"""
# first, get the results table built
@@ -3465,8 +3502,8 @@ class TkFixedFrame(tk.Frame):
def __init__(self, master, **kwargs):
"""
- :param master:
- :param **kwargs:
+ :param master:
+ :param **kwargs:
"""
tk.Frame.__init__(self, master, **kwargs)
@@ -3497,7 +3534,7 @@ class TkScrollableFrame(tk.Frame):
:param master: ????????????????????????
:param vertical_only: ????????????????????????
- :param **kwargs:
+ :param **kwargs:
"""
tk.Frame.__init__(self, master, **kwargs)
@@ -3546,7 +3583,7 @@ class TkScrollableFrame(tk.Frame):
def resize_frame(self, e):
"""
- :param e:
+ :param e:
"""
self.canvas.itemconfig(self.frame_id, height=e.height, width=e.width)
@@ -3554,7 +3591,7 @@ class TkScrollableFrame(tk.Frame):
def yscroll(self, event):
"""
- :param event:
+ :param event:
"""
if event.num == 5 or event.delta < 0:
@@ -3565,7 +3602,7 @@ class TkScrollableFrame(tk.Frame):
def xscroll(self, event):
"""
- :param event:
+ :param event:
"""
if event.num == 5 or event.delta < 0:
@@ -3576,8 +3613,8 @@ class TkScrollableFrame(tk.Frame):
def bind_mouse_scroll(self, parent, mode):
"""
- :param parent:
- :param mode:
+ :param parent:
+ :param mode:
"""
# ~~ Windows only
@@ -3589,7 +3626,7 @@ class TkScrollableFrame(tk.Frame):
def set_scrollregion(self, event=None):
"""Set the scroll region on the canvas
- :param event:
+ :param event:
"""
self.canvas.configure(scrollregion=self.canvas.bbox('all'))
@@ -3600,23 +3637,23 @@ class TkScrollableFrame(tk.Frame):
# ---------------------------------------------------------------------- #
class Column(Element):
""" """
-
+
def __init__(self, layout, background_color=None, size=(None, None), pad=None, scrollable=False,
vertical_scroll_only=False, right_click_menu=None, key=None, visible=True):
"""
:param layout: ????????????????????????
- :param background_color: color of background
- :param size: (w,h) w=characters-wide, h=rows-high
- :param pad: Amount of padding to put around element
+ :param background_color: color of background
+ :param size: (w,h) w=characters-wide, h=rows-high
+ :param pad: Amount of padding to put around element
:param scrollable: ???????????????????????? (Default = False)
:param vertical_scroll_only: ???????????????????????? (Default = False)
- :param right_click_menu: see "Right Click Menus"
- :param key: Used with window.FindElement and with return values
+ :param right_click_menu: List[List[str]] see "Right Click Menus" for format
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
:param visible: set visibility state of the element (Default = True)
"""
-
+
self.UseDictionary = False
self.ReturnValues = None
self.ReturnValuesList = []
@@ -3641,7 +3678,7 @@ class Column(Element):
def AddRow(self, *args):
"""Parms are a variable number of Elements
- :param *args:
+ :param *args:
"""
NumRows = len(self.Rows) # number of existing rows is our row number
@@ -3660,7 +3697,7 @@ class Column(Element):
def Layout(self, rows):
"""
- :param rows:
+ :param rows:
"""
for row in rows:
@@ -3669,7 +3706,7 @@ class Column(Element):
def _GetElementAtLocation(self, location):
"""
- :param location:
+ :param location:
"""
(row_num, col_num) = location
@@ -3679,11 +3716,11 @@ class Column(Element):
def Update(self, visible=None):
"""
+ Changes some of the settings for the Column Element. Must call `Window.Read` or `Window.Finalize` prior
- :param visible: change visibility of element
-
+ :param visible: (bool) control visibility of element
"""
-
+
if visible is False:
if self.TKColFrame:
self.TKColFrame.pack_forget()
@@ -3712,25 +3749,25 @@ class Column(Element):
# ---------------------------------------------------------------------- #
class Pane(Element):
""" """
-
+
def __init__(self, pane_list, background_color=None, size=(None, None), pad=None, orientation='vertical',
show_handle=True, relief=RELIEF_RAISED, handle_size=None, border_width=None, key=None, visible=True):
"""
- :param pane_list:
- :param background_color: color of background
- :param size: (w,h) w=characters-wide, h=rows-high
- :param pad: Amount of padding to put around element
+ :param pane_list:
+ :param background_color: color of background
+ :param size: (w,h) w=characters-wide, h=rows-high
+ :param pad: Amount of padding to put around element
:param orientation: 'horizontal' or 'vertical' ('h' or 'v' work) (Default value = 'vertical')
:param show_handle: (Default = True)
:param relief: relief style. Values are same as progress meter relief values. Can be a constant or a string: `RELIEF_RAISED RELIEF_SUNKEN RELIEF_FLAT RELIEF_RIDGE RELIEF_GROOVE RELIEF_SOLID`
- :param handle_size:
+ :param handle_size:
:param border_width: width of border around element
- :param key: Used with window.FindElement and with return values
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
:param visible: set visibility state of the element (Default = True)
"""
-
+
self.UseDictionary = False
self.ReturnValues = None
self.ReturnValuesList = []
@@ -3755,11 +3792,11 @@ class Pane(Element):
def Update(self, visible=None):
"""
+ Changes some of the settings for the Pane Element. Must call `Window.Read` or `Window.Finalize` prior
- :param visible: change visibility of element
-
+ :param visible: (bool) control visibility of element
"""
-
+
if visible is False:
self.PanedWindow.pack_forget()
elif visible is True:
@@ -3778,16 +3815,16 @@ class TKCalendar(ttk.Frame):
def __init__(self, master=None, target_element=None, close_when_chosen=True, default_date=(None, None, None), **kw):
"""WIDGET-SPECIFIC OPTIONS
-
+
locale, firstweekday, year, month, selectbackground,
selectforeground
- :param master:
- :param target_element:
+ :param master:
+ :param target_element:
:param close_when_chosen: (Default = True)
:param default_date: (Default = (None))
:param None:, None))
- :param **kw:
+ :param **kw:
"""
self._TargetElement = target_element
@@ -3830,8 +3867,8 @@ class TKCalendar(ttk.Frame):
def __setitem__(self, item, value):
"""
- :param item:
- :param value:
+ :param item:
+ :param value:
"""
if item in ('year', 'month'):
@@ -3846,7 +3883,7 @@ class TKCalendar(ttk.Frame):
def __getitem__(self, item):
"""
- :param item:
+ :param item:
"""
if item in ('year', 'month'):
@@ -3902,8 +3939,8 @@ class TKCalendar(ttk.Frame):
def __setup_selection(self, sel_bg, sel_fg):
"""
- :param sel_bg:
- :param sel_fg:
+ :param sel_bg:
+ :param sel_fg:
"""
self._font = tkinter.font.Font()
@@ -3918,7 +3955,7 @@ class TKCalendar(ttk.Frame):
def __minsize(self, evt):
"""
- :param evt:
+ :param evt:
"""
width, height = self._calendar.master.geometry().split('x')
@@ -3943,8 +3980,8 @@ class TKCalendar(ttk.Frame):
def _show_selection(self, text, bbox):
"""Configure canvas for a new selection.
- :param text:
- :param bbox:
+ :param text:
+ :param bbox:
"""
x, y, width, height = bbox
@@ -3962,7 +3999,7 @@ class TKCalendar(ttk.Frame):
def _pressed(self, evt):
"""Clicked somewhere in the calendar.
- :param evt:
+ :param evt:
"""
x, y, widget = evt.x, evt.y, evt.widget
@@ -4039,24 +4076,24 @@ class TKCalendar(ttk.Frame):
# ---------------------------------------------------------------------- #
class Menu(Element):
""" """
-
+
def __init__(self, menu_definition, background_color=None, size=(None, None), tearoff=False, pad=None, key=None,
visible=True):
"""
- :param menu_definition:
- :param background_color: color of background
- :param size: (w,h) w=characters-wide, h=rows-high
+ :param menu_definition:
+ :param background_color: color of background
+ :param size: (w,h) w=characters-wide, h=rows-high
:param tearoff: (Default = False)
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
:param visible: set visibility state of the element (Default = True)
"""
-
+
self.BackgroundColor = background_color if background_color is not None else DEFAULT_BACKGROUND_COLOR
self.MenuDefinition = menu_definition
- self.TKMenu = None # type: tk.Menu
+ self.Widget = self.TKMenu = None # type: tk.Menu
self.Tearoff = tearoff
self.MenuItemChosen = None
@@ -4067,7 +4104,7 @@ class Menu(Element):
def _MenuItemChosenCallback(self, item_chosen): # Menu Menu Item Chosen Callback
"""
- :param item_chosen:
+ :param item_chosen:
"""
# print('IN MENU ITEM CALLBACK', item_chosen)
@@ -4077,35 +4114,39 @@ class Menu(Element):
if self.ParentForm.CurrentlyRunningMainloop:
self.ParentForm.TKroot.quit() # kick the users out of the mainloop
- def Update(self, menu_definition, visible=None):
+ def Update(self, menu_definition=None, visible=None):
+ """
+ Update a menubar - can change the menu definition and visibility
+
+ :param menu_definition: List[[str]] New menu defintion
+ :param visible: (bool) control visibility of element
"""
- :param menu_definition:
- :param visible: change visibility of element
+ if menu_definition is not None:
+ self.MenuDefinition = menu_definition
+ self.TKMenu = tk.Menu(self.ParentForm.TKroot, tearoff=self.Tearoff) # create the menubar
+ menubar = self.TKMenu
+ for menu_entry in menu_definition:
+ # print(f'Adding a Menubar ENTRY {menu_entry}')
+ baritem = tk.Menu(menubar, tearoff=self.Tearoff)
+ pos = menu_entry[0].find('&')
+ # print(pos)
+ if pos != -1:
+ if pos == 0 or menu_entry[0][pos - 1] != "\\":
+ menu_entry[0] = menu_entry[0][:pos] + menu_entry[0][pos + 1:]
+ if menu_entry[0][0] == MENU_DISABLED_CHARACTER:
+ menubar.add_cascade(label=menu_entry[0][len(MENU_DISABLED_CHARACTER):], menu=baritem, underline=pos)
+ menubar.entryconfig(menu_entry[0][len(MENU_DISABLED_CHARACTER):], state='disabled')
+ else:
+ menubar.add_cascade(label=menu_entry[0], menu=baritem, underline=pos)
- """
-
- self.MenuDefinition = menu_definition
- self.TKMenu = tk.Menu(self.ParentForm.TKroot, tearoff=self.Tearoff) # create the menubar
- menubar = self.TKMenu
- for menu_entry in menu_definition:
- # print(f'Adding a Menubar ENTRY {menu_entry}')
- baritem = tk.Menu(menubar, tearoff=self.Tearoff)
- pos = menu_entry[0].find('&')
- # print(pos)
- if pos != -1:
- if pos == 0 or menu_entry[0][pos - 1] != "\\":
- menu_entry[0] = menu_entry[0][:pos] + menu_entry[0][pos + 1:]
- if menu_entry[0][0] == MENU_DISABLED_CHARACTER:
- menubar.add_cascade(label=menu_entry[0][len(MENU_DISABLED_CHARACTER):], menu=baritem, underline=pos)
- menubar.entryconfig(menu_entry[0][len(MENU_DISABLED_CHARACTER):], state='disabled')
- else:
- menubar.add_cascade(label=menu_entry[0], menu=baritem, underline=pos)
+ if len(menu_entry) > 1:
+ AddMenuItem(baritem, menu_entry[1], self)
- if len(menu_entry) > 1:
- AddMenuItem(baritem, menu_entry[1], self)
- self.ParentForm.TKroot.configure(menu=self.TKMenu)
- # TODO add visible code for menus
+ if visible == False:
+ self.ParentForm.TKroot.configure(menu=[]) # this will cause the menubar to disappear
+ elif self.TKMenu is not None:
+ self.ParentForm.TKroot.configure(menu=self.TKMenu)
def __del__(self):
""" """
@@ -4120,7 +4161,7 @@ MenuBar = Menu # another name for Menu to make it clear it's the Menu Bar
# ---------------------------------------------------------------------- #
class Table(Element):
""" """
-
+
def __init__(self, values, headings=None, visible_column_map=None, col_widths=None, def_col_width=10,
auto_size_columns=True, max_col_width=20, select_mode=None, display_row_numbers=False, num_rows=None,
row_height=None, font=None, justification='right', text_color=None, background_color=None,
@@ -4129,37 +4170,37 @@ class Table(Element):
key=None, tooltip=None, right_click_menu=None, visible=True):
"""
- :param values:
- :param headings:
- :param visible_column_map:
- :param col_widths:
+ :param values:
+ :param headings:
+ :param visible_column_map:
+ :param col_widths:
:param def_col_width: (Default value = 10)
:param auto_size_columns: (Default = True)
:param max_col_width: (Default value = 20)
- :param select_mode:
+ :param select_mode:
:param display_row_numbers: (Default = False)
- :param num_rows:
- :param row_height:
- :param font: specifies the font family, size, etc
+ :param num_rows:
+ :param row_height:
+ :param font: specifies the font family, size, etc
:param justification: (Default value = 'right')
- :param text_color: color of the text
- :param background_color: color of background
- :param alternating_row_color:
- :param row_colors:
+ :param text_color: color of the text
+ :param background_color: color of background
+ :param alternating_row_color:
+ :param row_colors:
:param vertical_scroll_only: (Default = True)
:param hide_vertical_scroll: (Default = False)
- :param size: (w,h) w=characters-wide, h=rows-high
+ :param size: (w,h) w=characters-wide, h=rows-high
:param change_submits: If True, pressing Enter key submits window (Default = False)
:param enable_events: Turns on the element specific events.(Default = False)
:param bind_return_key: (Default = False)
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
- :param tooltip: text, that will appear the you hover on
- :param right_click_menu: see "Right Click Menus"
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
+ :param right_click_menu: List[List[str]] see "Right Click Menus" for format
:param visible: set visibility state of the element (Default = True)
"""
-
+
self.Values = values
self.ColumnHeadings = headings
self.ColumnsToDisplay = visible_column_map
@@ -4193,14 +4234,14 @@ class Table(Element):
def Update(self, values=None, num_rows=None, visible=None, select_rows=None):
"""
+ Changes some of the settings for the Table Element. Must call `Window.Read` or `Window.Finalize` prior
- :param values:
- :param num_rows:
- :param visible: change visibility of element
- :param select_rows:
-
+ :param values:
+ :param num_rows:
+ :param visible: (bool) control visibility of element
+ :param select_rows:
"""
-
+
if values is not None:
children = self.TKTreeview.get_children()
for i in children:
@@ -4229,7 +4270,7 @@ class Table(Element):
def treeview_selected(self, event):
"""
- :param event:
+ :param event:
"""
selections = self.TKTreeview.selection()
@@ -4247,7 +4288,7 @@ class Table(Element):
def treeview_double_click(self, event):
"""
- :param event:
+ :param event:
"""
selections = self.TKTreeview.selection()
@@ -4272,7 +4313,7 @@ class Table(Element):
# ---------------------------------------------------------------------- #
class Tree(Element):
""" """
-
+
def __init__(self, data=None, headings=None, visible_column_map=None, col_widths=None, col0_width=10,
def_col_width=10, auto_size_columns=True, max_col_width=20, select_mode=None, show_expanded=False,
change_submits=False, enable_events=False, font=None, justification='right', text_color=None,
@@ -4280,32 +4321,32 @@ class Tree(Element):
right_click_menu=None, visible=True):
"""
- :param data:
- :param headings:
- :param visible_column_map:
- :param col_widths:
+ :param data:
+ :param headings:
+ :param visible_column_map:
+ :param col_widths:
:param col0_width: (Default value = 10)
:param def_col_width: (Default value = 10)
:param auto_size_columns: (Default = True)
:param max_col_width: (Default value = 20)
- :param select_mode:
+ :param select_mode:
:param show_expanded: (Default = False)
:param change_submits: If True, pressing Enter key submits window (Default = False)
:param enable_events: Turns on the element specific events.(Default = False)
- :param font: specifies the font family, size, etc
+ :param font: specifies the font family, size, etc
:param justification: (Default value = 'right')
- :param text_color: color of the text
- :param background_color: color of background
- :param num_rows:
- :param row_height:
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
- :param tooltip: text, that will appear the you hover on
- :param right_click_menu: see "Right Click Menus"
+ :param text_color: color of the text
+ :param background_color: color of background
+ :param num_rows:
+ :param row_height:
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
+ :param right_click_menu: List[List[str]] see "Right Click Menus" for format
:param visible: set visibility state of the element (Default = True)
"""
-
+
self.TreeData = data
self.ColumnHeadings = headings
self.ColumnsToDisplay = visible_column_map
@@ -4335,7 +4376,7 @@ class Tree(Element):
def treeview_selected(self, event):
"""
- :param event:
+ :param event:
"""
selections = self.TKTreeview.selection()
@@ -4353,7 +4394,7 @@ class Tree(Element):
def add_treeview_data(self, node):
"""
- :param node:
+ :param node:
"""
# print(f'Inserting {node.key} under parent {node.parent}')
@@ -4378,16 +4419,16 @@ class Tree(Element):
def Update(self, values=None, key=None, value=None, text=None, icon=None, visible=None):
"""
+ Changes some of the settings for the Tree Element. Must call `Window.Read` or `Window.Finalize` prior
- :param values:
- :param key: Used with window.FindElement and with return values
- :param value:
- :param text:
- :param icon:
- :param visible: change visibility of element
-
+ :param values:
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
+ :param value:
+ :param text:
+ :param icon:
+ :param visible: (bool) control visibility of element
"""
-
+
if values is not None:
children = self.TKTreeview.get_children()
for i in children:
@@ -4432,11 +4473,11 @@ class TreeData(object):
def __init__(self, parent, key, text, values, icon=None):
"""
- :param parent:
- :param key: Used with window.FindElement and with return values
- :param text:
- :param values:
- :param icon:
+ :param parent:
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
+ :param text:
+ :param values:
+ :param icon:
"""
self.parent = parent
@@ -4449,7 +4490,7 @@ class TreeData(object):
def _Add(self, node):
"""
- :param node:
+ :param node:
"""
self.children.append(node)
@@ -4463,8 +4504,8 @@ class TreeData(object):
def _AddNode(self, key, node):
"""
- :param key: Used with window.FindElement and with return values
- :param node:
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
+ :param node:
"""
self.tree_dict[key] = node
@@ -4472,11 +4513,11 @@ class TreeData(object):
def Insert(self, parent, key, text, values, icon=None):
"""
- :param parent:
- :param key: Used with window.FindElement and with return values
- :param text:
- :param values:
- :param icon:
+ :param parent:
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
+ :param text:
+ :param values:
+ :param icon:
"""
node = self.Node(parent, key, text, values, icon)
@@ -4491,8 +4532,8 @@ class TreeData(object):
def _NodeStr(self, node, level):
"""
- :param node:
- :param level:
+ :param node:
+ :param level:
"""
return '\n'.join(
@@ -4508,7 +4549,7 @@ class ErrorElement(Element):
def __init__(self, key=None):
"""Error Element
- :param key: Used with window.FindElement and with return values
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
"""
self.Key = key
@@ -4518,10 +4559,11 @@ class ErrorElement(Element):
def Update(self, silent_on_error=True, *args, **kwargs):
"""
+ Update method for the Error Element, an element that should not be directly used by developer
- :param silent_on_error: (Default = True)
- :param *args:
- :param **kwargs:
+ :param silent_on_error: (bool) if False, then a Popup window will be shown
+ :param *args: (Any) meant to "soak up" any normal paramters passed in
+ :param **kwargs: (Any) meant to "soak up" any keyword parameters that were passed in
"""
if not silent_on_error:
@@ -4552,8 +4594,10 @@ Stretch = ErrorElement
# Window CLASS #
# ------------------------------------------------------------------------- #
class Window:
- """ """
-
+ """
+ Represents a single Window
+ """
+
NumOpenWindows = 0
user_defined_icon = None
hidden_master_root = None
@@ -4566,47 +4610,47 @@ class Window:
auto_size_text=None, auto_size_buttons=None, location=(None, None), size=(None, None),
element_padding=None, margins=(None, None), button_color=None, font=None,
progress_bar_color=(None, None), background_color=None, border_depth=None, auto_close=False,
- auto_close_duration=DEFAULT_AUTOCLOSE_TIME, icon=DEFAULT_WINDOW_ICON, force_toplevel=False,
+ auto_close_duration=DEFAULT_AUTOCLOSE_TIME, icon=None, force_toplevel=False,
alpha_channel=1, return_keyboard_events=False, use_default_focus=True, text_justification=None,
no_titlebar=False, grab_anywhere=False, keep_on_top=False, resizable=False, disable_close=False,
disable_minimize=False, right_click_menu=None, transparent_color=None, debugger_enabled=True):
"""
- :param title:
- :param layout:
+ :param title:
+ :param layout:
:param default_element_size: (Default value = DEFAULT_ELEMENT_SIZE)
- :param default_button_element_size:
- :param auto_size_text: True if size should fit the text length
- :param auto_size_buttons:
+ :param default_button_element_size:
+ :param auto_size_text: True if size should fit the text length
+ :param auto_size_buttons:
:param location: (Default = (None))
:param size: (w,h) w=characters-wide, h=rows-high (Default = (None))
- :param element_padding:
+ :param element_padding:
:param margins: (Default = (None))
- :param button_color: button color (foreground, background)
- :param font: specifies the font family, size, etc
+ :param button_color: button color (foreground, background)
+ :param font: specifies the font family, size, etc
:param progress_bar_color: (Default = (None))
- :param background_color: color of background
- :param border_depth:
+ :param background_color: color of background
+ :param border_depth:
:param auto_close: (Default = False)
:param auto_close_duration: (Default value = DEFAULT_AUTOCLOSE_TIME)
- :param icon: Icon to display (Default value = DEFAULT_WINDOW_ICON)
+ :param icon: Icon to display. Filled in with default icon in init (Default value = None)
:param force_toplevel: (Default = False)
:param alpha_channel: (Default value = 1)
:param return_keyboard_events: (Default = False)
:param use_default_focus: (Default = True)
- :param text_justification:
+ :param text_justification:
:param no_titlebar: (Default = False)
:param grab_anywhere: If True can grab anywhere to move the window (Default = False)
- :param location: Location on screen to display
+ :param location: Location on screen to display
:param resizable: (Default = False)
:param disable_close: (Default = False)
:param disable_minimize: (Default = False)
- :param right_click_menu: see "Right Click Menus"
- :param transparent_color:
+ :param right_click_menu: List[List[str]] see "Right Click Menus" for format
+ :param transparent_color:
:param debugger_enabled: (Default = True)
"""
-
+
self.AutoSizeText = auto_size_text if auto_size_text is not None else DEFAULT_AUTOSIZE_TEXT
self.AutoSizeButtons = auto_size_buttons if auto_size_buttons is not None else DEFAULT_AUTOSIZE_BUTTONS
self.Title = title
@@ -4691,7 +4735,7 @@ class Window:
def AddRow(self, *args):
"""Parms are a variable number of Elements
- :param *args:
+ :param *args:
"""
NumRows = len(self.Rows) # number of existing rows is our row number
@@ -4728,7 +4772,7 @@ class Window:
def AddRows(self, rows):
"""
- :param rows:
+ :param rows:
"""
for row in rows:
@@ -4737,7 +4781,7 @@ class Window:
def Layout(self, rows):
"""
- :param rows:
+ :param rows:
"""
self.AddRows(rows)
@@ -4747,7 +4791,7 @@ class Window:
def LayoutAndRead(self, rows, non_blocking=False):
"""
- :param rows:
+ :param rows:
:param non_blocking: (Default = False)
"""
@@ -4760,7 +4804,7 @@ class Window:
def LayoutAndShow(self, rows):
"""
- :param rows:
+ :param rows:
"""
raise DeprecationWarning('LayoutAndShow is no longer supported... ')
@@ -4809,8 +4853,8 @@ class Window:
def SetIcon(self, icon=None, pngbase64=None):
"""
- :param icon:
- :param pngbase64:
+ :param icon:
+ :param pngbase64:
"""
if type(icon) is bytes:
@@ -4838,7 +4882,7 @@ class Window:
def _GetElementAtLocation(self, location):
"""
- :param location:
+ :param location:
"""
(row_num, col_num) = location
@@ -4885,7 +4929,7 @@ class Window:
"""
# ensure called only 1 time through a single read cycle
if not Window.read_call_from_debugger:
- refresh_debugger()
+ _refresh_debugger()
timeout = int(timeout) if timeout is not None else None
if timeout == 0: # timeout of zero runs the old readnonblocking
event, values = self.ReadNonBlocking()
@@ -5054,7 +5098,7 @@ class Window:
Find element object associated with the provided key
:returns Found element object, an Error Element, or None
- :param key: Used with window.FindElement and with return values
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
:param silent_on_error: (Default = False)
"""
@@ -5089,9 +5133,9 @@ class Window:
def _BuildKeyDictForWindow(self, top_window, window, key_dict):
"""
- :param top_window:
- :param window:
- :param key_dict:
+ :param top_window:
+ :param window:
+ :param key_dict:
"""
for row_num, row in enumerate(window.Rows):
@@ -5195,7 +5239,7 @@ class Window:
def StartMove(self, event):
"""
- :param event:
+ :param event:
"""
try:
@@ -5208,7 +5252,7 @@ class Window:
def StopMove(self, event):
"""
- :param event:
+ :param event:
"""
try:
@@ -5221,7 +5265,7 @@ class Window:
def OnMotion(self, event):
"""
- :param event:
+ :param event:
"""
try:
@@ -5237,7 +5281,7 @@ class Window:
def _KeyboardCallback(self, event):
"""
- :param event:
+ :param event:
"""
self.LastButtonClicked = None
@@ -5254,7 +5298,7 @@ class Window:
def _MouseWheelCallback(self, event):
"""
- :param event:
+ :param event:
"""
self.LastButtonClicked = None
@@ -5352,7 +5396,7 @@ class Window:
def SetAlpha(self, alpha):
"""
- :param alpha:
+ :param alpha:
"""
# Change the window's transparency
@@ -5369,7 +5413,7 @@ class Window:
def AlphaChannel(self, alpha):
"""
- :param alpha:
+ :param alpha:
"""
self._AlphaChannel = alpha
@@ -5386,6 +5430,7 @@ class Window:
""" """
return int(self.TKroot.winfo_x()), int(self.TKroot.winfo_y())
+
@property
def Size(self):
""" """
@@ -5397,7 +5442,7 @@ class Window:
def Size(self, size):
"""
- :param size:
+ :param size:
"""
try:
@@ -5414,7 +5459,7 @@ class Window:
def SetTransparentColor(self, color):
"""
- :param color:
+ :param color:
"""
try:
@@ -5437,7 +5482,7 @@ class Window:
def _callback_main_debugger_window_create_keystroke(self, event):
"""
- :param event:
+ :param event:
"""
_Debugger.debugger._build_main_debugger_window()
@@ -5445,7 +5490,7 @@ class Window:
def _callback_popout_window_create_keystroke(self, event):
"""
- :param event:
+ :param event:
"""
_Debugger.debugger._build_floating_window()
@@ -5470,7 +5515,7 @@ class Window:
def __exit__(self, *a):
"""
- :param *a:
+ :param *a:
"""
self.__del__()
@@ -5508,19 +5553,19 @@ def FolderBrowse(button_text='Browse', target=(ThisRow, -1), initial_folder=None
:param button_text: text in the button (Default value = 'Browse')
:param target: key or (row,col) target for the button (Default value = (ThisRow, -1))
:param initial_folder: starting path for folders and files
- :param tooltip: text, that will appear the you hover on
- :param size: (w,h) w=characters-wide, h=rows-high
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
+ :param size: (w,h) w=characters-wide, h=rows-high
:param auto_size_button: True if button size is determined by button text
:param button_color: button color (foreground, background)
:param disabled: set disable state for element (Default = False)
:param change_submits: If True, pressing Enter key submits window (Default = False)
:param enable_events: Turns on the element specific events.(Default = False)
- :param font: specifies the font family, size, etc
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
+ :param font: specifies the font family, size, etc
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
"""
-
+
return Button(button_text=button_text, button_type=BUTTON_TYPE_BROWSE_FOLDER, target=target,
initial_folder=initial_folder, tooltip=tooltip, size=size, auto_size_button=auto_size_button,
disabled=disabled, button_color=button_color, change_submits=change_submits,
@@ -5538,16 +5583,16 @@ def FileBrowse(button_text='Browse', target=(ThisRow, -1), file_types=(("ALL Fil
:param target: key or (row,col) target for the button (Default value = (ThisRow, -1))
:param file_types: (Default value = (("ALL Files", "*.*")))
:param initial_folder: starting path for folders and files
- :param tooltip: text, that will appear the you hover on
- :param size: (w,h) w=characters-wide, h=rows-high
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
+ :param size: (w,h) w=characters-wide, h=rows-high
:param auto_size_button: True if button size is determined by button text
:param button_color: button color (foreground, background)
:param change_submits: If True, pressing Enter key submits window (Default = False)
:param enable_events: Turns on the element specific events.(Default = False)
- :param font: specifies the font family, size, etc
+ :param font: specifies the font family, size, etc
:param disabled: set disable state for element (Default = False)
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
"""
return Button(button_text=button_text, button_type=BUTTON_TYPE_BROWSE_FILE, target=target, file_types=file_types,
@@ -5568,15 +5613,15 @@ def FilesBrowse(button_text='Browse', target=(ThisRow, -1), file_types=(("ALL Fi
:param file_types: (Default value = (("ALL Files", "*.*")))
:param disabled: set disable state for element (Default = False)
:param initial_folder: starting path for folders and files
- :param tooltip: text, that will appear the you hover on
- :param size: (w,h) w=characters-wide, h=rows-high
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
+ :param size: (w,h) w=characters-wide, h=rows-high
:param auto_size_button: True if button size is determined by button text
:param button_color: button color (foreground, background)
:param change_submits: If True, pressing Enter key submits window (Default = False)
:param enable_events: Turns on the element specific events.(Default = False)
- :param font: specifies the font family, size, etc
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
+ :param font: specifies the font family, size, etc
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
"""
return Button(button_text=button_text, button_type=BUTTON_TYPE_BROWSE_FILES, target=target, file_types=file_types,
@@ -5597,15 +5642,15 @@ def FileSaveAs(button_text='Save As...', target=(ThisRow, -1), file_types=(("ALL
:param file_types: (Default value = (("ALL Files", "*.*")))
:param initial_folder: starting path for folders and files
:param disabled: set disable state for element (Default = False)
- :param tooltip: text, that will appear the you hover on
- :param size: (w,h) w=characters-wide, h=rows-high
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
+ :param size: (w,h) w=characters-wide, h=rows-high
:param auto_size_button: True if button size is determined by button text
:param button_color: button color (foreground, background)
:param change_submits: If True, pressing Enter key submits window (Default = False)
:param enable_events: Turns on the element specific events.(Default = False)
- :param font: specifies the font family, size, etc
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
+ :param font: specifies the font family, size, etc
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
"""
return Button(button_text=button_text, button_type=BUTTON_TYPE_SAVEAS_FILE, target=target, file_types=file_types,
@@ -5626,15 +5671,15 @@ def SaveAs(button_text='Save As...', target=(ThisRow, -1), file_types=(("ALL Fil
:param file_types: (Default value = (("ALL Files", "*.*")))
:param initial_folder: starting path for folders and files
:param disabled: set disable state for element (Default = False)
- :param tooltip: text, that will appear the you hover on
- :param size: (w,h) w=characters-wide, h=rows-high
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
+ :param size: (w,h) w=characters-wide, h=rows-high
:param auto_size_button: True if button size is determined by button text
:param button_color: button color (foreground, background)
:param change_submits: If True, pressing Enter key submits window (Default = False)
:param enable_events: Turns on the element specific events.(Default = False)
- :param font: specifies the font family, size, etc
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
+ :param font: specifies the font family, size, etc
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
"""
return Button(button_text=button_text, button_type=BUTTON_TYPE_SAVEAS_FILE, target=target, file_types=file_types,
@@ -5649,16 +5694,16 @@ def Save(button_text='Save', size=(None, None), auto_size_button=None, button_co
"""
:param button_text: text in the button (Default value = 'Save')
- :param size: (w,h) w=characters-wide, h=rows-high
+ :param size: (w,h) w=characters-wide, h=rows-high
:param auto_size_button: True if button size is determined by button text
:param button_color: button color (foreground, background)
:param bind_return_key: (Default = True)
:param disabled: set disable state for element (Default = False)
- :param tooltip: text, that will appear the you hover on
- :param font: specifies the font family, size, etc
- :param focus: if focus should be set to this
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
+ :param font: specifies the font family, size, etc
+ :param focus: if focus should be set to this
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
"""
return Button(button_text=button_text, button_type=BUTTON_TYPE_READ_FORM, tooltip=tooltip, size=size,
@@ -5672,16 +5717,16 @@ def Submit(button_text='Submit', size=(None, None), auto_size_button=None, butto
"""
:param button_text: text in the button (Default value = 'Submit')
- :param size: (w,h) w=characters-wide, h=rows-high
+ :param size: (w,h) w=characters-wide, h=rows-high
:param auto_size_button: True if button size is determined by button text
:param button_color: button color (foreground, background)
:param disabled: set disable state for element (Default = False)
:param bind_return_key: (Default = True)
- :param tooltip: text, that will appear the you hover on
- :param font: specifies the font family, size, etc
- :param focus: if focus should be set to this
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
+ :param font: specifies the font family, size, etc
+ :param focus: if focus should be set to this
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
"""
return Button(button_text=button_text, button_type=BUTTON_TYPE_READ_FORM, tooltip=tooltip, size=size,
@@ -5696,16 +5741,16 @@ def Open(button_text='Open', size=(None, None), auto_size_button=None, button_co
"""
:param button_text: text in the button (Default value = 'Open')
- :param size: (w,h) w=characters-wide, h=rows-high
+ :param size: (w,h) w=characters-wide, h=rows-high
:param auto_size_button: True if button size is determined by button text
:param button_color: button color (foreground, background)
:param disabled: set disable state for element (Default = False)
:param bind_return_key: (Default = True)
- :param tooltip: text, that will appear the you hover on
- :param font: specifies the font family, size, etc
- :param focus: if focus should be set to this
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
+ :param font: specifies the font family, size, etc
+ :param focus: if focus should be set to this
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
"""
return Button(button_text=button_text, button_type=BUTTON_TYPE_READ_FORM, tooltip=tooltip, size=size,
@@ -5719,16 +5764,16 @@ def OK(button_text='OK', size=(None, None), auto_size_button=None, button_color=
"""
:param button_text: text in the button (Default value = 'OK')
- :param size: (w,h) w=characters-wide, h=rows-high
+ :param size: (w,h) w=characters-wide, h=rows-high
:param auto_size_button: True if button size is determined by button text
:param button_color: button color (foreground, background)
:param disabled: set disable state for element (Default = False)
:param bind_return_key: (Default = True)
- :param tooltip: text, that will appear the you hover on
- :param font: specifies the font family, size, etc
- :param focus: if focus should be set to this
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
+ :param font: specifies the font family, size, etc
+ :param focus: if focus should be set to this
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
"""
return Button(button_text=button_text, button_type=BUTTON_TYPE_READ_FORM, tooltip=tooltip, size=size,
@@ -5742,16 +5787,16 @@ def Ok(button_text='Ok', size=(None, None), auto_size_button=None, button_color=
"""
:param button_text: text in the button (Default value = 'Ok')
- :param size: (w,h) w=characters-wide, h=rows-high
+ :param size: (w,h) w=characters-wide, h=rows-high
:param auto_size_button: True if button size is determined by button text
:param button_color: button color (foreground, background)
:param disabled: set disable state for element (Default = False)
:param bind_return_key: (Default = True)
- :param tooltip: text, that will appear the you hover on
- :param font: specifies the font family, size, etc
- :param focus: if focus should be set to this
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
+ :param font: specifies the font family, size, etc
+ :param focus: if focus should be set to this
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
"""
return Button(button_text=button_text, button_type=BUTTON_TYPE_READ_FORM, tooltip=tooltip, size=size,
@@ -5765,16 +5810,16 @@ def Cancel(button_text='Cancel', size=(None, None), auto_size_button=None, butto
"""
:param button_text: text in the button (Default value = 'Cancel')
- :param size: (w,h) w=characters-wide, h=rows-high
+ :param size: (w,h) w=characters-wide, h=rows-high
:param auto_size_button: True if button size is determined by button text
:param button_color: button color (foreground, background)
:param disabled: set disable state for element (Default = False)
- :param tooltip: text, that will appear the you hover on
- :param font: specifies the font family, size, etc
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
+ :param font: specifies the font family, size, etc
:param bind_return_key: (Default = False)
- :param focus: if focus should be set to this
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
+ :param focus: if focus should be set to this
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
"""
return Button(button_text=button_text, button_type=BUTTON_TYPE_READ_FORM, tooltip=tooltip, size=size,
@@ -5788,16 +5833,16 @@ def Quit(button_text='Quit', size=(None, None), auto_size_button=None, button_co
"""
:param button_text: text in the button (Default value = 'Quit')
- :param size: (w,h) w=characters-wide, h=rows-high
+ :param size: (w,h) w=characters-wide, h=rows-high
:param auto_size_button: True if button size is determined by button text
:param button_color: button color (foreground, background)
:param disabled: set disable state for element (Default = False)
- :param tooltip: text, that will appear the you hover on
- :param font: specifies the font family, size, etc
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
+ :param font: specifies the font family, size, etc
:param bind_return_key: (Default = False)
- :param focus: if focus should be set to this
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
+ :param focus: if focus should be set to this
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
"""
return Button(button_text=button_text, button_type=BUTTON_TYPE_READ_FORM, tooltip=tooltip, size=size,
@@ -5811,16 +5856,16 @@ def Exit(button_text='Exit', size=(None, None), auto_size_button=None, button_co
"""
:param button_text: text in the button (Default value = 'Exit')
- :param size: (w,h) w=characters-wide, h=rows-high
+ :param size: (w,h) w=characters-wide, h=rows-high
:param auto_size_button: True if button size is determined by button text
:param button_color: button color (foreground, background)
:param disabled: set disable state for element (Default = False)
- :param tooltip: text, that will appear the you hover on
- :param font: specifies the font family, size, etc
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
+ :param font: specifies the font family, size, etc
:param bind_return_key: (Default = False)
- :param focus: if focus should be set to this
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
+ :param focus: if focus should be set to this
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
"""
return Button(button_text=button_text, button_type=BUTTON_TYPE_READ_FORM, tooltip=tooltip, size=size,
@@ -5834,16 +5879,16 @@ def Yes(button_text='Yes', size=(None, None), auto_size_button=None, button_colo
"""
:param button_text: text in the button (Default value = 'Yes')
- :param size: (w,h) w=characters-wide, h=rows-high
+ :param size: (w,h) w=characters-wide, h=rows-high
:param auto_size_button: True if button size is determined by button text
:param button_color: button color (foreground, background)
:param disabled: set disable state for element (Default = False)
- :param tooltip: text, that will appear the you hover on
- :param font: specifies the font family, size, etc
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
+ :param font: specifies the font family, size, etc
:param bind_return_key: (Default = True)
- :param focus: if focus should be set to this
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
+ :param focus: if focus should be set to this
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
"""
return Button(button_text=button_text, button_type=BUTTON_TYPE_READ_FORM, tooltip=tooltip, size=size,
@@ -5857,16 +5902,16 @@ def No(button_text='No', size=(None, None), auto_size_button=None, button_color=
"""
:param button_text: text in the button (Default value = 'No')
- :param size: (w,h) w=characters-wide, h=rows-high
+ :param size: (w,h) w=characters-wide, h=rows-high
:param auto_size_button: True if button size is determined by button text
:param button_color: button color (foreground, background)
:param disabled: set disable state for element (Default = False)
- :param tooltip: text, that will appear the you hover on
- :param font: specifies the font family, size, etc
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
+ :param font: specifies the font family, size, etc
:param bind_return_key: (Default = False)
- :param focus: if focus should be set to this
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
+ :param focus: if focus should be set to this
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
"""
return Button(button_text=button_text, button_type=BUTTON_TYPE_READ_FORM, tooltip=tooltip, size=size,
@@ -5880,16 +5925,16 @@ def Help(button_text='Help', size=(None, None), auto_size_button=None, button_co
"""
:param button_text: text in the button (Default value = 'Help')
- :param size: (w,h) w=characters-wide, h=rows-high
+ :param size: (w,h) w=characters-wide, h=rows-high
:param auto_size_button: True if button size is determined by button text
:param button_color: button color (foreground, background)
:param disabled: set disable state for element (Default = False)
- :param font: specifies the font family, size, etc
- :param tooltip: text, that will appear the you hover on
+ :param font: specifies the font family, size, etc
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
:param bind_return_key: (Default = False)
- :param focus: if focus should be set to this
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
+ :param focus: if focus should be set to this
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
"""
return Button(button_text=button_text, button_type=BUTTON_TYPE_READ_FORM, tooltip=tooltip, size=size,
@@ -5903,16 +5948,16 @@ def Debug(button_text='', size=(None, None), auto_size_button=None, button_color
"""
:param button_text: text in the button (Default value = '')
- :param size: (w,h) w=characters-wide, h=rows-high
+ :param size: (w,h) w=characters-wide, h=rows-high
:param auto_size_button: True if button size is determined by button text
:param button_color: button color (foreground, background)
:param disabled: set disable state for element (Default = False)
- :param font: specifies the font family, size, etc
- :param tooltip: text, that will appear the you hover on
+ :param font: specifies the font family, size, etc
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
:param bind_return_key: (Default = False)
- :param focus: if focus should be set to this
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
+ :param focus: if focus should be set to this
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
"""
return Button(button_text=button_text, button_type=BUTTON_TYPE_SHOW_DEBUGGER, tooltip=tooltip, size=size,
@@ -5929,20 +5974,20 @@ def SimpleButton(button_text, image_filename=None, image_data=None, image_size=(
:param button_text: text in the button
:param image_filename: image filename if there is a button image
- :param image_data: in-RAM image to be displayed on button
+ :param image_data: in-RAM image to be displayed on button
:param image_size: size of button image in pixels
- :param image_subsample:amount to reduce the size of the image
+ :param image_subsample:amount to reduce the size of the image
:param border_width: width of border around element
- :param tooltip: text, that will appear the you hover on
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
:param size: (w,h) w=characters-wide, h=rows-high (Default = (None))
:param auto_size_button: True if button size is determined by button text
:param button_color: button color (foreground, background)
- :param font: specifies the font family, size, etc
+ :param font: specifies the font family, size, etc
:param bind_return_key: (Default = False)
:param disabled: set disable state for element (Default = False)
- :param focus: if focus should be set to this
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
+ :param focus: if focus should be set to this
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
"""
return Button(button_text=button_text, button_type=BUTTON_TYPE_CLOSES_WIN, image_filename=image_filename,
@@ -5960,20 +6005,20 @@ def CloseButton(button_text, image_filename=None, image_data=None, image_size=(N
:param button_text: text in the button
:param image_filename: image filename if there is a button image
- :param image_data: in-RAM image to be displayed on button
+ :param image_data: in-RAM image to be displayed on button
:param image_size: size of button image in pixels
- :param image_subsample:amount to reduce the size of the image
+ :param image_subsample:amount to reduce the size of the image
:param border_width: width of border around element
- :param tooltip: text, that will appear the you hover on
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
:param size: (w,h) w=characters-wide, h=rows-high (Default = (None))
:param auto_size_button: True if button size is determined by button text
:param button_color: button color (foreground, background)
- :param font: specifies the font family, size, etc
+ :param font: specifies the font family, size, etc
:param bind_return_key: (Default = False)
:param disabled: set disable state for element (Default = False)
- :param focus: if focus should be set to this
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
+ :param focus: if focus should be set to this
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
"""
return Button(button_text=button_text, button_type=BUTTON_TYPE_CLOSES_WIN, image_filename=image_filename,
@@ -5994,20 +6039,20 @@ def ReadButton(button_text, image_filename=None, image_data=None, image_size=(No
:param button_text: text in the button
:param image_filename: image filename if there is a button image
- :param image_data: in-RAM image to be displayed on button
+ :param image_data: in-RAM image to be displayed on button
:param image_size: size of button image in pixels
- :param image_subsample:amount to reduce the size of the image
+ :param image_subsample:amount to reduce the size of the image
:param border_width: width of border around element
- :param tooltip: text, that will appear the you hover on
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
:param size: (w,h) w=characters-wide, h=rows-high (Default = (None))
:param auto_size_button: True if button size is determined by button text
:param button_color: button color (foreground, background)
- :param font: specifies the font family, size, etc
+ :param font: specifies the font family, size, etc
:param bind_return_key: (Default = False)
:param disabled: set disable state for element (Default = False)
- :param focus: if focus should be set to this
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
+ :param focus: if focus should be set to this
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
"""
return Button(button_text=button_text, button_type=BUTTON_TYPE_READ_FORM, image_filename=image_filename,
@@ -6029,20 +6074,20 @@ def RealtimeButton(button_text, image_filename=None, image_data=None, image_size
:param button_text: text in the button
:param image_filename: image filename if there is a button image
- :param image_data: in-RAM image to be displayed on button
+ :param image_data: in-RAM image to be displayed on button
:param image_size: size of button image in pixels
- :param image_subsample:amount to reduce the size of the image
+ :param image_subsample:amount to reduce the size of the image
:param border_width: width of border around element
- :param tooltip: text, that will appear the you hover on
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
:param size: (w,h) w=characters-wide, h=rows-high (Default = (None))
:param auto_size_button: True if button size is determined by button text
:param button_color: button color (foreground, background)
- :param font: specifies the font family, size, etc
+ :param font: specifies the font family, size, etc
:param disabled: set disable state for element (Default = False)
:param bind_return_key: (Default = False)
- :param focus: if focus should be set to this
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
+ :param focus: if focus should be set to this
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
"""
return Button(button_text=button_text, button_type=BUTTON_TYPE_REALTIME, image_filename=image_filename,
@@ -6060,20 +6105,20 @@ def DummyButton(button_text, image_filename=None, image_data=None, image_size=(N
:param button_text: text in the button
:param image_filename: image filename if there is a button image
- :param image_data: in-RAM image to be displayed on button
+ :param image_data: in-RAM image to be displayed on button
:param image_size: size of button image in pixels
- :param image_subsample:amount to reduce the size of the image
+ :param image_subsample:amount to reduce the size of the image
:param border_width: width of border around element
- :param tooltip: text, that will appear the you hover on
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
:param size: (w,h) w=characters-wide, h=rows-high (Default = (None))
:param auto_size_button: True if button size is determined by button text
:param button_color: button color (foreground, background)
- :param font: specifies the font family, size, etc
+ :param font: specifies the font family, size, etc
:param disabled: set disable state for element (Default = False)
:param bind_return_key: (Default = False)
- :param focus: if focus should be set to this
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
+ :param focus: if focus should be set to this
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
"""
return Button(button_text=button_text, button_type=BUTTON_TYPE_CLOSES_WIN_ONLY, image_filename=image_filename,
@@ -6092,27 +6137,27 @@ def CalendarButton(button_text, target=(None, None), close_when_date_chosen=True
"""
:param button_text: text in the button
- :param target:
+ :param target:
:param close_when_date_chosen: (Default = True)
:param default_date_m_d_y: (Default = (None))
- :param None:
+ :param None:
:param image_filename: image filename if there is a button image
- :param image_data: in-RAM image to be displayed on button
+ :param image_data: in-RAM image to be displayed on button
:param image_size: (Default = (None))
- :param image_subsample:amount to reduce the size of the image
- :param tooltip: text, that will appear the you hover on
+ :param image_subsample:amount to reduce the size of the image
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
:param border_width: width of border around element
:param size: (w,h) w=characters-wide, h=rows-high (Default = (None))
:param auto_size_button: True if button size is determined by button text
:param button_color: button color (foreground, background)
:param disabled: set disable state for element (Default = False)
- :param font: specifies the font family, size, etc
+ :param font: specifies the font family, size, etc
:param bind_return_key: (Default = False)
- :param focus: if focus should be set to this
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
- :param locale:
- :param format:
+ :param focus: if focus should be set to this
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
+ :param locale:
+ :param format:
"""
button = Button(button_text=button_text, button_type=BUTTON_TYPE_CALENDAR_CHOOSER, target=target,
@@ -6135,22 +6180,22 @@ def ColorChooserButton(button_text, target=(None, None), image_filename=None, im
"""
:param button_text: text in the button
- :param target:
+ :param target:
:param image_filename: image filename if there is a button image
- :param image_data: in-RAM image to be displayed on button
+ :param image_data: in-RAM image to be displayed on button
:param image_size: (Default = (None))
- :param image_subsample:amount to reduce the size of the image
- :param tooltip: text, that will appear the you hover on
+ :param image_subsample:amount to reduce the size of the image
+ :param tooltip: (str) text, that will appear when mouse hovers over the element
:param border_width: width of border around element
:param size: (w,h) w=characters-wide, h=rows-high (Default = (None))
:param auto_size_button: True if button size is determined by button text
:param button_color: button color (foreground, background)
:param disabled: set disable state for element (Default = False)
- :param font: specifies the font family, size, etc
+ :param font: specifies the font family, size, etc
:param bind_return_key: (Default = False)
- :param focus: if focus should be set to this
- :param pad: Amount of padding to put around element
- :param key: Used with window.FindElement and with return values
+ :param focus: if focus should be set to this
+ :param pad: Amount of padding to put around element
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
"""
return Button(button_text=button_text, button_type=BUTTON_TYPE_COLOR_CHOOSER, target=target,
@@ -6165,9 +6210,9 @@ def ColorChooserButton(button_text, target=(None, None), image_filename=None, im
def AddToReturnDictionary(form, element, value):
"""
- :param form:
- :param element:
- :param value:
+ :param form:
+ :param element:
+ :param value:
"""
form.ReturnValuesDictionary[element.Key] = value
@@ -6183,8 +6228,8 @@ def AddToReturnDictionary(form, element, value):
def AddToReturnList(form, value):
"""
- :param form:
- :param value:
+ :param form:
+ :param value:
"""
form.ReturnValuesList.append(value)
@@ -6195,7 +6240,7 @@ def AddToReturnList(form, value):
def InitializeResults(form):
"""
- :param form:
+ :param form:
"""
BuildResults(form, True, form)
@@ -6207,7 +6252,7 @@ def InitializeResults(form):
def DecodeRadioRowCol(RadValue):
"""
- :param RadValue:
+ :param RadValue:
"""
container = RadValue // 100000
@@ -6219,9 +6264,9 @@ def DecodeRadioRowCol(RadValue):
def EncodeRadioRowCol(container, row, col):
"""
- :param container:
- :param row:
- :param col:
+ :param container:
+ :param row:
+ :param col:
"""
RadValue = container * 100000 + row * 1000 + col
@@ -6234,9 +6279,9 @@ def EncodeRadioRowCol(container, row, col):
def BuildResults(form, initialize_only, top_level_form):
"""
- :param form:
- :param initialize_only:
- :param top_level_form:
+ :param form:
+ :param initialize_only:
+ :param top_level_form:
"""
# Results for elements are:
@@ -6257,9 +6302,9 @@ def BuildResults(form, initialize_only, top_level_form):
def BuildResultsForSubform(form, initialize_only, top_level_form):
"""
- :param form:
- :param initialize_only:
- :param top_level_form:
+ :param form:
+ :param initialize_only:
+ :param top_level_form:
"""
button_pressed_text = top_level_form.LastButtonClicked
@@ -6476,8 +6521,8 @@ def BuildResultsForSubform(form, initialize_only, top_level_form):
def FillFormWithValues(form, values_dict):
"""
- :param form:
- :param values_dict:
+ :param form:
+ :param values_dict:
"""
FillSubformWithValues(form, values_dict)
@@ -6486,8 +6531,8 @@ def FillFormWithValues(form, values_dict):
def FillSubformWithValues(form, values_dict):
"""
- :param form:
- :param values_dict:
+ :param form:
+ :param values_dict:
"""
for row_num, row in enumerate(form.Rows):
@@ -6530,8 +6575,8 @@ def FillSubformWithValues(form, values_dict):
def _FindElementFromKeyInSubForm(form, key):
"""
- :param form:
- :param key: Used with window.FindElement and with return values
+ :param form:
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
"""
for row_num, row in enumerate(form.Rows):
@@ -6603,9 +6648,9 @@ if sys.version_info[0] >= 3:
def AddMenuItem(top_menu, sub_menu_info, element, is_sub_menu=False, skip=False):
"""
- :param top_menu:
- :param sub_menu_info:
- :param element:
+ :param top_menu:
+ :param sub_menu_info:
+ :param element:
:param is_sub_menu: (Default = False)
:param skip: (Default = False)
@@ -6662,9 +6707,9 @@ else:
def AddMenuItem(top_menu, sub_menu_info, element, is_sub_menu=False, skip=False):
"""
- :param top_menu:
- :param sub_menu_info:
- :param element:
+ :param top_menu:
+ :param sub_menu_info:
+ :param element:
:param is_sub_menu: (Default = False)
:param skip: (Default = False)
@@ -6742,9 +6787,9 @@ else:
def PackFormIntoFrame(form, containing_frame, toplevel_form):
"""
- :param form:
- :param containing_frame:
- :param toplevel_form:
+ :param form:
+ :param containing_frame:
+ :param toplevel_form:
"""
def CharWidthInPixels():
@@ -7428,7 +7473,7 @@ def PackFormIntoFrame(form, containing_frame, toplevel_form):
if text_color is not None and text_color != COLOR_SYSTEM_DEFAULT:
element.TKSpinBox.configure(fg=text_color)
if element.ChangeSubmits:
- element.TKSpinBox.bind('', element.SpinChangedHandler)
+ element.TKSpinBox.bind('', element._SpinChangedHandler)
if element.Disabled == True:
element.TKSpinBox['state'] = 'disabled'
if element.Tooltip is not None:
@@ -7881,7 +7926,7 @@ def PackFormIntoFrame(form, containing_frame, toplevel_form):
def add_treeview_data(node):
"""
- :param node:
+ :param node:
"""
# print(f'Inserting {node.key} under parent {node.parent}')
@@ -8005,7 +8050,7 @@ def PackFormIntoFrame(form, containing_frame, toplevel_form):
def ConvertFlexToTK(MyFlexForm):
"""
- :param MyFlexForm:
+ :param MyFlexForm:
"""
MyFlexForm # type: Window
@@ -8053,7 +8098,7 @@ def ConvertFlexToTK(MyFlexForm):
def StartupTK(my_flex_form: Window):
"""
- :param my_flex_form: Window:
+ :param my_flex_form: Window:
"""
# global _my_windows
@@ -8159,8 +8204,8 @@ def StartupTK(my_flex_form: Window):
def _GetNumLinesNeeded(text, max_line_width):
"""
- :param text:
- :param max_line_width:
+ :param text:
+ :param max_line_width:
"""
if max_line_width == 0:
@@ -8180,7 +8225,7 @@ def _GetNumLinesNeeded(text, max_line_width):
def ConvertArgsToSingleString(*args):
"""
- :param *args:
+ :param *args:
"""
max_line_total, width_used, total_lines, = 0, 0, 0
@@ -8218,7 +8263,7 @@ class QuickMeter(object):
:param title: text to display in eleemnt
:param current_value: current value
:param max_value: max value of QuickMeter
- :param key: Used with window.FindElement and with return values
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
:param *args: stuff to output
:param orientation: 'horizontal' or 'vertical' ('h' or 'v' work) (Default value = 'vertical')(Default value = 'v')
:param bar_color: ???????????????????????????????????
@@ -8245,7 +8290,7 @@ class QuickMeter(object):
def BuildWindow(self, *args):
"""
- :param *args:
+ :param *args:
"""
layout = []
@@ -8275,9 +8320,9 @@ class QuickMeter(object):
def UpdateMeter(self, current_value, max_value, *args): ### support for *args when updating
"""
- :param current_value:
- :param max_value:
- :param *args:
+ :param current_value:
+ :param max_value:
+ :param *args:
"""
self.current_value = current_value
@@ -8332,10 +8377,10 @@ def OneLineProgressMeter(title, current_value, max_value, key, *args, orientatio
:param title: text to display
:param current_value: current progressbar value
:param max_value: max value of progressbar
- :param key: Used with window.FindElement and with return values
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
:param *args: stuff to output.
:param orientation: 'horizontal' or 'vertical' ('h' or 'v' work) (Default value = 'vertical')(Default value = 'v')
- :param bar_color:
+ :param bar_color:
:param button_color: button color (foreground, background)
:param size: (w,h) w=characters-wide, h=rows-high (Default value = DEFAULT_PROGRESS_BAR_SIZE)
:param border_width: width of border around element
@@ -8357,7 +8402,7 @@ def OneLineProgressMeter(title, current_value, max_value, key, *args, orientatio
def OneLineProgressMeterCancel(key):
"""
- :param key: Used with window.FindElement and with return values
+ :param key: Used with window.FindElement and with return values to uniquely identify this element
"""
try:
@@ -8374,7 +8419,7 @@ def OneLineProgressMeterCancel(key):
def GetComplimentaryHex(color):
"""
- :param color:
+ :param color:
"""
# strip the # from the beginning
@@ -8399,13 +8444,13 @@ class DebugWin():
grab_anywhere=False, keep_on_top=False, do_not_reroute_stdout=True):
"""
- :param size: (w,h) w=characters-wide, h=rows-high
+ :param size: (w,h) w=characters-wide, h=rows-high
:param location: (Default = (None))
- :param font: specifies the font family, size, etc
+ :param font: specifies the font family, size, etc
:param no_titlebar: (Default = False)
:param no_button: (Default = False)
:param grab_anywhere: If True can grab anywhere to move the window (Default = False)
- :param location: Location on screen to display
+ :param location: Location on screen to display
:param do_not_reroute_stdout: (Default = True)
"""
@@ -8439,9 +8484,9 @@ class DebugWin():
def Print(self, *args, end=None, sep=None):
"""
- :param *args:
- :param end:
- :param sep:
+ :param *args:
+ :param end:
+ :param sep:
"""
sepchar = sep if sep is not None else ' '
@@ -8482,16 +8527,16 @@ def EasyPrint(*args, size=(None, None), end=None, sep=None, location=(None, None
no_button=False, grab_anywhere=False, keep_on_top=False, do_not_reroute_stdout=True):
"""
- :param *args:
- :param size: (w,h) w=characters-wide, h=rows-high
- :param end:
- :param sep:
+ :param *args:
+ :param size: (w,h) w=characters-wide, h=rows-high
+ :param end:
+ :param sep:
:param location: (Default = (None))
- :param font: specifies the font family, size, etc
+ :param font: specifies the font family, size, etc
:param no_titlebar: (Default = False)
:param no_button: (Default = False)
:param grab_anywhere: If True can grab anywhere to move the window (Default = False)
- :param location: Location on screen to display
+ :param location: Location on screen to display
:param do_not_reroute_stdout: (Default = True)
"""
@@ -8513,73 +8558,13 @@ def EasyPrintClose():
DebugWin.debug_window = None
-# ======================== Scrolled Text Box =====#
-# ===================================================#
-def PopupScrolled(*args, button_color=None, yes_no=False, auto_close=False, auto_close_duration=None, size=(None, None),
- location=(None, None), title=None, non_blocking=False):
- """
-
- :param *args:
- :param button_color: button color (foreground, background)
- :param yes_no: (Default = False)
- :param auto_close: (Default = False)
- :param auto_close_duration:
- :param size: (w,h) w=characters-wide, h=rows-high
- :param location: (Default = (None))
- :param title:
- :param non_blocking: (Default = False)
-
- """
- if not args: return
- width, height = size
- width = width if width else MESSAGE_BOX_LINE_WIDTH
- window = Window(title=title or args[0], auto_size_text=True, button_color=button_color, auto_close=auto_close,
- auto_close_duration=auto_close_duration, location=location, resizable=True)
- max_line_total, max_line_width, total_lines, height_computed = 0, 0, 0, 0
- complete_output = ''
- for message in args:
- # fancy code to check if string and convert if not is not need. Just always convert to string :-)
- # if not isinstance(message, str): message = str(message)
- message = str(message)
- longest_line_len = max([len(l) for l in message.split('\n')])
- width_used = min(longest_line_len, width)
- max_line_total = max(max_line_total, width_used)
- max_line_width = width
- lines_needed = _GetNumLinesNeeded(message, width_used)
- height_computed += lines_needed
- complete_output += message + '\n'
- total_lines += lines_needed
- height_computed = MAX_SCROLLED_TEXT_BOX_HEIGHT if height_computed > MAX_SCROLLED_TEXT_BOX_HEIGHT else height_computed
- if height:
- height_computed = height
- window.AddRow(Multiline(complete_output, size=(max_line_width, height_computed)))
- pad = max_line_total - 15 if max_line_total > 15 else 1
- # show either an OK or Yes/No depending on paramater
- button = DummyButton if non_blocking else CloseButton
- if yes_no:
- window.AddRow(Text('', size=(pad, 1), auto_size_text=False), button('Yes'), button('No'))
- else:
- window.AddRow(Text('', size=(pad, 1), auto_size_text=False),
- button('OK', size=(5, 1), button_color=button_color))
-
- if non_blocking:
- button, values = window.Read(timeout=0)
- else:
- button, values = window.Read()
- # window.Close()
- return button
-
-
-ScrolledTextBox = PopupScrolled
-
-
# ============================== SetGlobalIcon ======#
# Sets the icon to be used by default #
# ===================================================#
def SetGlobalIcon(icon):
"""
- :param icon:
+ :param icon:
"""
# global _my_windows
@@ -8616,29 +8601,29 @@ def SetOptions(icon=None, button_color=None, element_size=(None, None), button_e
:param button_element_size: (Default = (None))
:param margins: tkinter margins around outsize (Default = (None))
:param element_padding: (Default = (None))
- :param auto_size_text: True if size should fit the text length
- :param auto_size_buttons:
- :param font: specifies the font family, size, etc
+ :param auto_size_text: True if size should fit the text length
+ :param auto_size_buttons:
+ :param font: specifies the font family, size, etc
:param border_width: width of border around element
- :param slider_border_width:
- :param slider_relief:
- :param slider_orientation:
- :param autoclose_time:
- :param message_box_line_width:
- :param progress_meter_border_depth:
- :param progress_meter_style:
- :param progress_meter_relief:
- :param progress_meter_color:
- :param progress_meter_size:
- :param text_justification:
- :param background_color: color of background
- :param element_background_color:
- :param text_element_background_color:
- :param input_elements_background_color:
- :param input_text_color:
- :param scrollbar_color:
- :param text_color: color of the text
- :param element_text_color:
+ :param slider_border_width:
+ :param slider_relief:
+ :param slider_orientation:
+ :param autoclose_time:
+ :param message_box_line_width:
+ :param progress_meter_border_depth:
+ :param progress_meter_style:
+ :param progress_meter_relief:
+ :param progress_meter_color:
+ :param progress_meter_size:
+ :param text_justification:
+ :param background_color: color of background
+ :param element_background_color:
+ :param text_element_background_color:
+ :param input_elements_background_color:
+ :param input_text_color:
+ :param scrollbar_color:
+ :param text_color: color of the text
+ :param element_text_color:
:param debug_win_size: (Default = (None))
:param window_location: (Default = (None))
:param error_button_color: (Default = (None))
@@ -9073,7 +9058,7 @@ def ListOfLookAndFeelValues():
def ChangeLookAndFeel(index):
"""
- :param index:
+ :param index:
"""
# global LOOK_AND_FEEL_TABLE
@@ -9104,19 +9089,13 @@ def ChangeLookAndFeel(index):
print('** Warning - Look and Feel value not valid. Change your ChangeLookAndFeel call. **')
-# ============================== sprint ======#
-# Is identical to the Scrolled Text Box #
-# Provides a crude 'print' mechanism but in a #
-# GUI environment #
-# ============================================#
-sprint = ScrolledTextBox
# Converts an object's contents into a nice printable string. Great for dumping debug data
def ObjToStringSingleObj(obj):
"""
- :param obj:
+ :param obj:
"""
if obj is None:
@@ -9128,7 +9107,7 @@ def ObjToStringSingleObj(obj):
def ObjToString(obj, extra=' '):
"""
- :param obj:
+ :param obj:
:param extra: (Default value = ' ')
"""
@@ -9141,6 +9120,16 @@ def ObjToString(obj, extra=' '):
for item in sorted(obj.__dict__)))
+def test_func(parm):
+ """
+
+ :param parm:
+ :return:
+ """
+ return 'my return'
+
+
+
######
# # #### ##### # # ##### ####
# # # # # # # # # # #
@@ -9157,28 +9146,29 @@ def ObjToString(obj, extra=' '):
def Popup(*args, title=None, button_color=None, background_color=None, text_color=None, button_type=POPUP_BUTTONS_OK,
auto_close=False, auto_close_duration=None, custom_text=(None, None), non_blocking=False,
- icon=DEFAULT_WINDOW_ICON, line_width=None,
+ icon=None, line_width=None,
font=None, no_titlebar=False, grab_anywhere=False, keep_on_top=False, location=(None, None)):
- """Popup - Display a popup box with as many parms as you wish to include
- :return:
+ """
+ Popup - Display a popup Window with as many parms as you wish to include. This is the GUI equivalent of the
+ "print" statement. It's also great for "pausing" your program's flow until the user can read some error messages
- :param *args:
- :param title:
- :param button_color: button color (foreground, background)
- :param background_color: color of background
- :param text_color: color of the text
- :param button_type: (Default value = POPUP_BUTTONS_OK)
- :param auto_close: (Default = False)
- :param auto_close_duration:
- :param custom_text:
- :param non_blocking: (Default = False)
- :param icon: Icon to display (Default value = DEFAULT_WINDOW_ICON)
- :param line_width: Width of lines in characters
- :param font: specifies the font family, size, etc
- :param no_titlebar: (Default = False)
- :param grab_anywhere: If True can grab anywhere to move the window (Default = False)
- :param location: Location on screen to display
- :param location: (Default = (None))
+ :param *args: Variable number of your arguments. Load up the call with stuff to see!
+ :param title: Optional title for the window
+ :param button_color: Tuple(str, str) Color of the buttons shown (text color, button color)
+ :param background_color: (str) Window background color
+ :param text_color: (str) text color
+ :param button_type: (enum) determines which pre-defined buttons will be shown (Default value = POPUP_BUTTONS_OK). The user normally will NOT change this value. There are many Popup functions and they call Popup, changing this parameter to get the desired effect.
+ :param auto_close: (bool) If True the window will automatically close
+ :param auto_close_duration: (int) time in seconds to keep window open before closing it automatically
+ :param custom_text: Union[tuple(str, str), str] A string or pair of strings that contain the text to display on the buttons
+ :param non_blocking: (bool) If True then will immediately return from the function without waiting for the uder's input.
+ :param icon: Union[str, bytes] icon to display on the window. Same format was Window call
+ :param line_width: (int) Width of lines in characters to use. Defaults to MESSAGE_BOX_LINE_WIDTH
+ :param font: Union[str, tuple(font, size, modifiors) specifies the font family, size, etc
+ :param no_titlebar: (bool) If True will not show the frame around the window and the titlebar across the top
+ :param grab_anywhere: (bool) If True can grab anywhere to move the window. If no_titlebar is True, grab_anywhere should likely be enabled too
+ :param location: (int, int) Location on screen to display the top left corner of window. Defaults to centered on screen
+ :return: Union[str, None] Returns text of the button that was pressed. None will be returned if user closed window with X
"""
if not args:
@@ -9263,34 +9253,109 @@ def Popup(*args, title=None, button_color=None, background_color=None, text_colo
def MsgBox(*args):
"""
- :param *args:
+ :param *args:
"""
raise DeprecationWarning('MsgBox is no longer supported... change your call to Popup')
+
+
+
+# ======================== Scrolled Text Box =====#
+# ===================================================#
+def PopupScrolled(*args, button_color=None, yes_no=False, auto_close=False, auto_close_duration=None, size=(None, None),
+ location=(None, None), title=None, non_blocking=False):
+ """
+ Show a scrolled Popup window containing the user's text that was supplied. Use with as many items to print as you
+ want, just like a print statement.
+
+ :param *args: Variable number of items to print
+ :param button_color: button color (foreground, background)
+ :param yes_no: (Default = False)
+ :param auto_close: (Default = False)
+ :param auto_close_duration:
+ :param size: (w,h) w=characters-wide, h=rows-high
+ :param location: (Default = (None))
+ :param title:
+ :param non_blocking: (Default = False)
+ :return: Union[str, None] Returns text of the button that was pressed. None will be returned if user closed window with X
+ """
+ if not args: return
+ width, height = size
+ width = width if width else MESSAGE_BOX_LINE_WIDTH
+ window = Window(title=title or args[0], auto_size_text=True, button_color=button_color, auto_close=auto_close,
+ auto_close_duration=auto_close_duration, location=location, resizable=True)
+ max_line_total, max_line_width, total_lines, height_computed = 0, 0, 0, 0
+ complete_output = ''
+ for message in args:
+ # fancy code to check if string and convert if not is not need. Just always convert to string :-)
+ # if not isinstance(message, str): message = str(message)
+ message = str(message)
+ longest_line_len = max([len(l) for l in message.split('\n')])
+ width_used = min(longest_line_len, width)
+ max_line_total = max(max_line_total, width_used)
+ max_line_width = width
+ lines_needed = _GetNumLinesNeeded(message, width_used)
+ height_computed += lines_needed
+ complete_output += message + '\n'
+ total_lines += lines_needed
+ height_computed = MAX_SCROLLED_TEXT_BOX_HEIGHT if height_computed > MAX_SCROLLED_TEXT_BOX_HEIGHT else height_computed
+ if height:
+ height_computed = height
+ window.AddRow(Multiline(complete_output, size=(max_line_width, height_computed)))
+ pad = max_line_total - 15 if max_line_total > 15 else 1
+ # show either an OK or Yes/No depending on paramater
+ button = DummyButton if non_blocking else CloseButton
+ if yes_no:
+ window.AddRow(Text('', size=(pad, 1), auto_size_text=False), button('Yes'), button('No'))
+ else:
+ window.AddRow(Text('', size=(pad, 1), auto_size_text=False),
+ button('OK', size=(5, 1), button_color=button_color))
+
+ if non_blocking:
+ button, values = window.Read(timeout=0)
+ else:
+ button, values = window.Read()
+ # window.Close()
+ return button
+
+
+ScrolledTextBox = PopupScrolled
+
+# ============================== sprint ======#
+# Is identical to the Scrolled Text Box #
+# Provides a crude 'print' mechanism but in a #
+# GUI environment #
+# This is in addition to the Print function #
+# which routes output to a "Debug Window" #
+# ============================================#
+sprint = ScrolledTextBox
+
+
+
+
# --------------------------- PopupNoButtons ---------------------------
def PopupNoButtons(*args, title=None, button_color=None, background_color=None, text_color=None, auto_close=False,
- auto_close_duration=None, non_blocking=False, icon=DEFAULT_WINDOW_ICON, line_width=None, font=None,
+ auto_close_duration=None, non_blocking=False, icon=None, line_width=None, font=None,
no_titlebar=False, grab_anywhere=False, keep_on_top=False, location=(None, None)):
"""Show a Popup but without any buttons
- :return:
- :param *args:
- :param title:
+ :param *args:
+ :param title:
:param button_color: button color (foreground, background)
- :param background_color: color of background
- :param text_color: color of the text
+ :param background_color: color of background
+ :param text_color: color of the text
:param auto_close: (Default = False)
- :param auto_close_duration:
+ :param auto_close_duration:
:param non_blocking: (Default = False)
- :param icon: Icon to display (Default value = DEFAULT_WINDOW_ICON)
- :param line_width: Width of lines in characters
- :param font: specifies the font family, size, etc
+ :param icon: Icon to display
+ :param line_width: Width of lines in characters
+ :param font: specifies the font family, size, etc
:param no_titlebar: (Default = False)
:param grab_anywhere: If True can grab anywhere to move the window (Default = False)
- :param location: Location on screen to display
- :param location:
+ :param location: Location on screen to display
+ :param location:
"""
Popup(*args, title=title, button_color=button_color, background_color=background_color, text_color=text_color,
@@ -9303,28 +9368,28 @@ def PopupNoButtons(*args, title=None, button_color=None, background_color=None,
# --------------------------- PopupNonBlocking ---------------------------
def PopupNonBlocking(*args, title=None, button_type=POPUP_BUTTONS_OK, button_color=None, background_color=None,
text_color=None,
- auto_close=False, auto_close_duration=None, non_blocking=True, icon=DEFAULT_WINDOW_ICON,
+ auto_close=False, auto_close_duration=None, non_blocking=True, icon=None,
line_width=None, font=None, no_titlebar=False, grab_anywhere=False, keep_on_top=False,
location=(None, None)):
- """Show Popup box and immediately return (does not block)
- :return:
+ """
+ Show Popup window and immediately return (does not block)
- :param *args:
- :param title:
+ :param *args:
+ :param title:
:param button_type: (Default value = POPUP_BUTTONS_OK)
:param button_color: button color (foreground, background)
- :param background_color: color of background
- :param text_color: color of the text
+ :param background_color: color of background
+ :param text_color: color of the text
:param auto_close: (Default = False)
- :param auto_close_duration:
+ :param auto_close_duration:
:param non_blocking: (Default = True)
- :param icon: Icon to display (Default value = DEFAULT_WINDOW_ICON)
- :param line_width: Width of lines in characters
- :param font: specifies the font family, size, etc
+ :param icon: Icon to display
+ :param line_width: Width of lines in characters
+ :param font: specifies the font family, size, etc
:param no_titlebar: (Default = False)
:param grab_anywhere: If True can grab anywhere to move the window (Default = False)
- :param location: Location on screen to display
- :param location:
+ :param location: Location on screen to display
+ :param location:
"""
Popup(*args, title=title, button_color=button_color, background_color=background_color, text_color=text_color,
@@ -9340,27 +9405,27 @@ PopupNoWait = PopupNonBlocking
# --------------------------- PopupQuick - a NonBlocking, Self-closing Popup ---------------------------
def PopupQuick(*args, title=None, button_type=POPUP_BUTTONS_OK, button_color=None, background_color=None,
text_color=None,
- auto_close=True, auto_close_duration=2, non_blocking=True, icon=DEFAULT_WINDOW_ICON, line_width=None,
+ auto_close=True, auto_close_duration=2, non_blocking=True, icon=None, line_width=None,
font=None, no_titlebar=False, grab_anywhere=False, keep_on_top=False, location=(None, None)):
- """Show Popup box that doesn't block and closes itself
- :return:
+ """
+ Show Popup box that doesn't block and closes itself
- :param *args:
- :param title:
+ :param *args:
+ :param title:
:param button_type: (Default value = POPUP_BUTTONS_OK)
:param button_color: button color (foreground, background)
- :param background_color: color of background
- :param text_color: color of the text
+ :param background_color: color of background
+ :param text_color: color of the text
:param auto_close: (Default = True)
:param auto_close_duration: (Default value = 2)
:param non_blocking: (Default = True)
- :param icon: Icon to display (Default value = DEFAULT_WINDOW_ICON)
- :param line_width: Width of lines in characters
- :param font: specifies the font family, size, etc
+ :param icon: Icon to display
+ :param line_width: Width of lines in characters
+ :param font: specifies the font family, size, etc
:param no_titlebar: (Default = False)
:param grab_anywhere: If True can grab anywhere to move the window (Default = False)
- :param location: Location on screen to display
- :param location:
+ :param location: Location on screen to display
+ :param location:
"""
Popup(*args, title=title, button_color=button_color, background_color=background_color, text_color=text_color,
@@ -9373,29 +9438,28 @@ def PopupQuick(*args, title=None, button_type=POPUP_BUTTONS_OK, button_color=Non
# --------------------------- PopupQuick - a NonBlocking, Self-closing Popup with no titlebar and no buttons ---------------------------
def PopupQuickMessage(*args, title=None, button_type=POPUP_BUTTONS_NO_BUTTONS, button_color=None, background_color=None,
text_color=None,
- auto_close=True, auto_close_duration=2, non_blocking=True, icon=DEFAULT_WINDOW_ICON,
+ auto_close=True, auto_close_duration=2, non_blocking=True, icon=None,
line_width=None,
font=None, no_titlebar=True, grab_anywhere=False, keep_on_top=False, location=(None, None)):
- """Show Popup box that doesn't block and closes itself
- :return:
+ """
+ Show Popup window with no titlebar, doesn't block, and auto closes itself.
- :param *args:
- :param title:
+ :param *args:
+ :param title:
:param button_type: (Default value = POPUP_BUTTONS_NO_BUTTONS)
:param button_color: button color (foreground, background)
- :param background_color: color of background
- :param text_color: color of the text
+ :param background_color: color of background
+ :param text_color: color of the text
:param auto_close: (Default = True)
:param auto_close_duration: (Default value = 2)
:param non_blocking: (Default = True)
- :param icon: Icon to display (Default value = DEFAULT_WINDOW_ICON)
- :param line_width: Width of lines in characters
- :param font: specifies the font family, size, etc
+ :param icon: Icon to display
+ :param line_width: Width of lines in characters
+ :param font: specifies the font family, size, etc
:param no_titlebar: (Default = True)
:param grab_anywhere: If True can grab anywhere to move the window (Default = False)
- :param location: Location on screen to display
- :param location:
-
+ :param location: Location on screen to display
+ :param location:
"""
Popup(*args, title=title, button_color=button_color, background_color=background_color, text_color=text_color,
button_type=button_type,
@@ -9407,26 +9471,26 @@ def PopupQuickMessage(*args, title=None, button_type=POPUP_BUTTONS_NO_BUTTONS, b
# --------------------------- PopupNoTitlebar ---------------------------
def PopupNoTitlebar(*args, title=None, button_type=POPUP_BUTTONS_OK, button_color=None, background_color=None,
text_color=None,
- auto_close=False, auto_close_duration=None, non_blocking=False, icon=DEFAULT_WINDOW_ICON,
+ auto_close=False, auto_close_duration=None, non_blocking=False, icon=None,
line_width=None, font=None, grab_anywhere=True, keep_on_top=False, location=(None, None)):
- """Display a Popup without a titlebar. Enables grab anywhere so you can move it
- :return:
+ """
+ Display a Popup without a titlebar. Enables grab anywhere so you can move it
- :param *args:
- :param title:
+ :param *args:
+ :param title:
:param button_type: (Default value = POPUP_BUTTONS_OK)
:param button_color: button color (foreground, background)
- :param background_color: color of background
- :param text_color: color of the text
+ :param background_color: color of background
+ :param text_color: color of the text
:param auto_close: (Default = False)
- :param auto_close_duration:
+ :param auto_close_duration:
:param non_blocking: (Default = False)
- :param icon: Icon to display (Default value = DEFAULT_WINDOW_ICON)
- :param line_width: Width of lines in characters
- :param font: specifies the font family, size, etc
+ :param icon: Icon to display
+ :param line_width: Width of lines in characters
+ :param font: specifies the font family, size, etc
:param grab_anywhere: (Default = True)
- :param location: Location on screen to display
- :param location:
+ :param location: Location on screen to display
+ :param location:
"""
Popup(*args, title=title, button_color=button_color, background_color=background_color, text_color=text_color,
@@ -9444,28 +9508,28 @@ PopupAnnoying = PopupNoTitlebar
# --------------------------- PopupAutoClose ---------------------------
def PopupAutoClose(*args, title=None, button_type=POPUP_BUTTONS_OK, button_color=None, background_color=None,
text_color=None,
- auto_close=True, auto_close_duration=None, non_blocking=False, icon=DEFAULT_WINDOW_ICON,
+ auto_close=True, auto_close_duration=None, non_blocking=False, icon=None,
line_width=None, font=None, no_titlebar=False, grab_anywhere=False, keep_on_top=False,
location=(None, None)):
"""Popup that closes itself after some time period
:return:
- :param *args:
- :param title:
+ :param *args:
+ :param title:
:param button_type: (Default value = POPUP_BUTTONS_OK)
:param button_color: button color (foreground, background)
- :param background_color: color of background
- :param text_color: color of the text
+ :param background_color: color of background
+ :param text_color: color of the text
:param auto_close: (Default = True)
- :param auto_close_duration:
+ :param auto_close_duration:
:param non_blocking: (Default = False)
- :param icon: Icon to display (Default value = DEFAULT_WINDOW_ICON)
- :param line_width: Width of lines in characters
- :param font: specifies the font family, size, etc
+ :param icon: Icon to display
+ :param line_width: Width of lines in characters
+ :param font: specifies the font family, size, etc
:param no_titlebar: (Default = False)
:param grab_anywhere: If True can grab anywhere to move the window (Default = False)
- :param location: Location on screen to display
- :param location:
+ :param location: Location on screen to display
+ :param location:
"""
Popup(*args, title=title, button_color=button_color, background_color=background_color, text_color=text_color,
@@ -9480,27 +9544,26 @@ PopupTimed = PopupAutoClose
# --------------------------- PopupError ---------------------------
def PopupError(*args, title=None, button_color=(None, None), background_color=None, text_color=None, auto_close=False,
- auto_close_duration=None, non_blocking=False, icon=DEFAULT_WINDOW_ICON, line_width=None, font=None,
+ auto_close_duration=None, non_blocking=False, icon=None, line_width=None, font=None,
no_titlebar=False, grab_anywhere=False, keep_on_top=False, location=(None, None)):
- """Popup with colored button and 'Error' as button text
- :return:
+ """
+ Popup with colored button and 'Error' as button text
- :param *args:
- :param title:
+ :param *args:
+ :param title:
:param button_color: button color (foreground, background)
- :param background_color: color of background
- :param text_color: color of the text
+ :param background_color: color of background
+ :param text_color: color of the text
:param auto_close: (Default = False)
- :param auto_close_duration:
+ :param auto_close_duration:
:param non_blocking: (Default = False)
- :param icon: Icon to display (Default value = DEFAULT_WINDOW_ICON)
- :param line_width: Width of lines in characters
- :param font: specifies the font family, size, etc
+ :param icon: Icon to display
+ :param line_width: Width of lines in characters
+ :param font: specifies the font family, size, etc
:param no_titlebar: (Default = False)
:param grab_anywhere: If True can grab anywhere to move the window (Default = False)
- :param location: Location on screen to display
+ :param location: Location on screen to display
:param location: (Default = (None))
-
"""
tbutton_color = DEFAULT_ERROR_BUTTON_COLOR if button_color == (None, None) else button_color
Popup(*args, title=title, button_type=POPUP_BUTTONS_ERROR, background_color=background_color, text_color=text_color,
@@ -9512,26 +9575,26 @@ def PopupError(*args, title=None, button_color=(None, None), background_color=No
# --------------------------- PopupCancel ---------------------------
def PopupCancel(*args, title=None, button_color=None, background_color=None, text_color=None, auto_close=False,
- auto_close_duration=None, non_blocking=False, icon=DEFAULT_WINDOW_ICON, line_width=None, font=None,
+ auto_close_duration=None, non_blocking=False, icon=None, line_width=None, font=None,
no_titlebar=False, grab_anywhere=False, keep_on_top=False, location=(None, None)):
"""Display Popup with "cancelled" button text
:return:
- :param *args:
- :param title:
+ :param *args:
+ :param title:
:param button_color: button color (foreground, background)
- :param background_color: color of background
- :param text_color: color of the text
+ :param background_color: color of background
+ :param text_color: color of the text
:param auto_close: (Default = False)
- :param auto_close_duration:
+ :param auto_close_duration:
:param non_blocking: (Default = False)
- :param icon: Icon to display (Default value = DEFAULT_WINDOW_ICON)
- :param line_width: Width of lines in characters
- :param font: specifies the font family, size, etc
+ :param icon: Icon to display
+ :param line_width: Width of lines in characters
+ :param font: specifies the font family, size, etc
:param no_titlebar: (Default = False)
:param grab_anywhere: If True can grab anywhere to move the window (Default = False)
- :param location: Location on screen to display
- :param location:
+ :param location: Location on screen to display
+ :param location:
"""
Popup(*args, title=title, button_type=POPUP_BUTTONS_CANCELLED, background_color=background_color,
@@ -9543,27 +9606,26 @@ def PopupCancel(*args, title=None, button_color=None, background_color=None, tex
# --------------------------- PopupOK ---------------------------
def PopupOK(*args, title=None, button_color=None, background_color=None, text_color=None, auto_close=False,
- auto_close_duration=None, non_blocking=False, icon=DEFAULT_WINDOW_ICON, line_width=None, font=None,
+ auto_close_duration=None, non_blocking=False, icon=None, line_width=None, font=None,
no_titlebar=False, grab_anywhere=False, keep_on_top=False, location=(None, None)):
- """Display Popup with OK button only
- :return:
+ """
+ Display Popup with OK button only
- :param *args:
- :param title:
+ :param *args:
+ :param title:
:param button_color: button color (foreground, background)
- :param background_color: color of background
- :param text_color: color of the text
+ :param background_color: color of background
+ :param text_color: color of the text
:param auto_close: (Default = False)
- :param auto_close_duration:
+ :param auto_close_duration:
:param non_blocking: (Default = False)
- :param icon: Icon to display (Default value = DEFAULT_WINDOW_ICON)
- :param line_width: Width of lines in characters
- :param font: specifies the font family, size, etc
+ :param icon: Icon to display
+ :param line_width: Width of lines in characters
+ :param font: specifies the font family, size, etc
:param no_titlebar: (Default = False)
:param grab_anywhere: If True can grab anywhere to move the window (Default = False)
- :param location: Location on screen to display
- :param location:
-
+ :param location: Location on screen to display
+ :param location:
"""
Popup(*args, title=title, button_type=POPUP_BUTTONS_OK, background_color=background_color, text_color=text_color,
non_blocking=non_blocking, icon=icon, line_width=line_width, button_color=button_color, auto_close=auto_close,
@@ -9575,25 +9637,24 @@ def PopupOK(*args, title=None, button_color=None, background_color=None, text_co
def PopupOKCancel(*args, title=None, button_color=None, background_color=None, text_color=None, auto_close=False,
auto_close_duration=None, non_blocking=False, icon=DEFAULT_WINDOW_ICON, line_width=None, font=None,
no_titlebar=False, grab_anywhere=False, keep_on_top=False, location=(None, None)):
- """Display popup with OK and Cancel buttons
- :return: OK, Cancel or None
+ """
+ Display popup with OK and Cancel buttons
- :param *args:
- :param title:
+ :param *args:
+ :param title:
:param button_color: button color (foreground, background)
- :param background_color: color of background
- :param text_color: color of the text
+ :param background_color: color of background
+ :param text_color: color of the text
:param auto_close: (Default = False)
- :param auto_close_duration:
+ :param auto_close_duration:
:param non_blocking: (Default = False)
- :param icon: Icon to display (Default value = DEFAULT_WINDOW_ICON)
- :param line_width: Width of lines in characters
- :param font: specifies the font family, size, etc
+ :param icon: Icon to display
+ :param line_width: Width of lines in characters
+ :param font: specifies the font family, size, etc
:param no_titlebar: (Default = False)
:param grab_anywhere: If True can grab anywhere to move the window (Default = False)
- :param location: Location on screen to display
- :param location:
-
+ :param location: Location on screen to display
+ :return: Union["OK", "Cancel", None]
"""
return Popup(*args, title=title, button_type=POPUP_BUTTONS_OK_CANCEL, background_color=background_color,
text_color=text_color,
@@ -9604,27 +9665,27 @@ def PopupOKCancel(*args, title=None, button_color=None, background_color=None, t
# --------------------------- PopupYesNo ---------------------------
def PopupYesNo(*args, title=None, button_color=None, background_color=None, text_color=None, auto_close=False,
- auto_close_duration=None, non_blocking=False, icon=DEFAULT_WINDOW_ICON, line_width=None, font=None,
+ auto_close_duration=None, non_blocking=False, icon=None, line_width=None, font=None,
no_titlebar=False, grab_anywhere=False, keep_on_top=False, location=(None, None)):
- """Display Popup with Yes and No buttons
- :return: Yes, No or None
+ """
+ Display Popup with Yes and No buttons
- :param *args:
- :param title:
+ :param *args:
+ :param title:
:param button_color: button color (foreground, background)
- :param background_color: color of background
- :param text_color: color of the text
+ :param background_color: color of background
+ :param text_color: color of the text
:param auto_close: (Default = False)
- :param auto_close_duration:
+ :param auto_close_duration:
:param non_blocking: (Default = False)
- :param icon: Icon to display (Default value = DEFAULT_WINDOW_ICON)
- :param line_width: Width of lines in characters
- :param font: specifies the font family, size, etc
+ :param icon: Icon to display
+ :param line_width: Width of lines in characters
+ :param font: specifies the font family, size, etc
:param no_titlebar: (Default = False)
:param grab_anywhere: If True can grab anywhere to move the window (Default = False)
- :param location: Location on screen to display
- :param location:
-
+ :param location: Location on screen to display
+ :param location:
+ :return: Union["Yes", "No", None]
"""
return Popup(*args, title=title, button_type=POPUP_BUTTONS_YES_NO, background_color=background_color,
text_color=text_color,
@@ -9641,27 +9702,27 @@ def PopupYesNo(*args, title=None, button_color=None, background_color=None, text
def PopupGetFolder(message, title=None, default_path='', no_window=False, size=(None, None), button_color=None,
- background_color=None, text_color=None, icon=DEFAULT_WINDOW_ICON, font=None, no_titlebar=False,
+ background_color=None, text_color=None, icon=None, font=None, no_titlebar=False,
grab_anywhere=False, keep_on_top=False, location=(None, None), initial_folder=None):
- """Display popup with text entry field and browse button. Browse for folder
- :return: Contents of text field. None if closed using X or cancelled
+ """
+ Display popup with text entry field and browse button. Browse for folder
- :param message:
- :param title:
+ :param message:
+ :param title:
:param default_path: (Default value = '')
:param no_window: (Default = False)
- :param size: (w,h) w=characters-wide, h=rows-high
+ :param size: (w,h) w=characters-wide, h=rows-high
:param button_color: button color (foreground, background)
- :param background_color: color of background
- :param text_color: color of the text
- :param icon: Icon to display (Default value = DEFAULT_WINDOW_ICON)
- :param font: specifies the font family, size, etc
+ :param background_color: color of background
+ :param text_color: color of the text
+ :param icon: Icon to display
+ :param font: specifies the font family, size, etc
:param no_titlebar: (Default = False)
:param grab_anywhere: If True can grab anywhere to move the window (Default = False)
- :param location: Location on screen to display
+ :param location: Location on screen to display
:param location: (Default = (None))
- :param initial_folder:
-
+ :param initial_folder:
+ :return: Union[str, None] Contents of text field. None if closed using X or cancelled
"""
# global _my_windows
@@ -9716,31 +9777,31 @@ def PopupGetFolder(message, title=None, default_path='', no_window=False, size=(
def PopupGetFile(message, title=None, default_path='', default_extension='', save_as=False, multiple_files=False,
file_types=(("ALL Files", "*.*"),),
no_window=False, size=(None, None), button_color=None, background_color=None, text_color=None,
- icon=DEFAULT_WINDOW_ICON, font=None, no_titlebar=False, grab_anywhere=False, keep_on_top=False,
+ icon=None, font=None, no_titlebar=False, grab_anywhere=False, keep_on_top=False,
location=(None, None), initial_folder=None):
- """Display popup with text entry field and browse button. Browse for file
- :return: string representing the path chosen, None if cancelled or window closed with X
+ """
+ Display popup with text entry field and browse button. Browse for file
- :param message:
- :param title:
+ :param message:
+ :param title:
:param default_path: (Default value = '')
:param default_extension: (Default value = '')
:param save_as: (Default = False)
:param multiple_files: (Default = False)
:param file_types: (Default value = (("ALL Files", "*.*")))
:param no_window: (Default = False)
- :param size: (w,h) w=characters-wide, h=rows-high
+ :param size: (w,h) w=characters-wide, h=rows-high
:param button_color: button color (foreground, background)
- :param background_color: color of background
- :param text_color: color of the text
- :param icon: Icon to display (Default value = DEFAULT_WINDOW_ICON)
- :param font: specifies the font family, size, etc
+ :param background_color: color of background
+ :param text_color: color of the text
+ :param icon: Icon to display
+ :param font: specifies the font family, size, etc
:param no_titlebar: (Default = False)
:param grab_anywhere: If True can grab anywhere to move the window (Default = False)
- :param location: Location on screen to display
+ :param location: Location on screen to display
:param location: (Default = (None))
- :param initial_folder:
-
+ :param initial_folder:
+ :return: Union[str, None] string representing the path chosen, None if cancelled or window closed with X
"""
if no_window:
@@ -9808,26 +9869,26 @@ def PopupGetFile(message, title=None, default_path='', default_extension='', sav
# --------------------------- PopupGetText ---------------------------
def PopupGetText(message, title=None, default_text='', password_char='', size=(None, None), button_color=None,
- background_color=None, text_color=None, icon=DEFAULT_WINDOW_ICON, font=None, no_titlebar=False,
+ background_color=None, text_color=None, icon=None, font=None, no_titlebar=False,
grab_anywhere=False, keep_on_top=False, location=(None, None)):
- """Display Popup with text entry field
- :return: Text entered or None if window was closed
+ """
+ Display Popup with text entry field
- :param message:
- :param title:
+ :param message:
+ :param title:
:param default_text: (Default value = '')
:param password_char: Passwork character if this is a password field (Default value = '')
- :param size: (w,h) w=characters-wide, h=rows-high
+ :param size: (w,h) w=characters-wide, h=rows-high
:param button_color: button color (foreground, background)
- :param background_color: color of background
- :param text_color: color of the text
- :param icon: Icon to display (Default value = DEFAULT_WINDOW_ICON)
- :param font: specifies the font family, size, etc
+ :param background_color: color of background
+ :param text_color: color of the text
+ :param icon: Icon to display
+ :param font: specifies the font family, size, etc
:param no_titlebar: (Default = False)
:param grab_anywhere: If True can grab anywhere to move the window (Default = False)
- :param location: Location on screen to display
+ :param location: Location on screen to display
:param location: (Default = (None))
-
+ :return: Union[str, None] Text entered or None if window was closed
"""
layout = [[Text(message, auto_size_text=True, text_color=text_color, background_color=background_color, font=font)],
@@ -9854,20 +9915,21 @@ def PopupAnimated(image_source, message=None, background_color=None, text_color=
grab_anywhere=True, keep_on_top=True, location=(None, None), alpha_channel=None,
time_between_frames=0, transparent_color=None):
"""
-
- :param image_source:
- :param message:
- :param background_color: color of background
- :param text_color: color of the text
- :param font: specifies the font family, size, etc
- :param no_titlebar: (Default = True)
- :param grab_anywhere: (Default = True)
- :param keep_on_top: (Default = True)
- :param location:
- :param alpha_channel:
+ "Plays" an animated GIF file. This function has its own internal clocking meaning you can call it at any frequency
+ and the rate the frames of video is shown remains constant. Maybe your frames update every 30 ms but your
+ event loop is running every 10 ms.
+ :param image_source: Union[str, bytes] Either a filename or a base64 string.
+ :param message: An optional message to be shown with the animation
+ :param background_color: (str) color of background
+ :param text_color: (str) color of the text
+ :param font: Union[str, tuple) specifies the font family, size, etc
+ :param no_titlebar: (bool) If True then the titlebar and window frame will not be shown
+ :param grab_anywhere: (bool) If True then you can move the window just clicking anywhere on window, hold and drag
+ :param keep_on_top: (bool) If True then Window will remain on top of all other windows currently shownn
+ :param location: (int, int) (x,y) location on the screen to place the top left corner of your window. Default is to center on screen
+ :param alpha_channel:
:param time_between_frames: (Default value = 0)
- :param transparent_color:
-
+ :param transparent_color:
"""
if image_source is None:
for image in Window.animated_popup_dict:
@@ -9950,7 +10012,7 @@ class _Debugger():
def _build_main_debugger_window(self, location=(None, None)):
"""
- :param location:
+ :param location:
"""
ChangeLookAndFeel(COLOR_SCHEME)
@@ -9958,7 +10020,7 @@ class _Debugger():
def InVar(key1):
"""
- :param key1:
+ :param key1:
"""
row1 = [T(' '),
@@ -10021,8 +10083,8 @@ class _Debugger():
def _refresh_main_debugger_window(self, mylocals, myglobals):
"""
- :param mylocals:
- :param myglobals:
+ :param mylocals:
+ :param myglobals:
"""
if not self.watcher_window: # if there is no window setup, nothing to do
@@ -10160,7 +10222,7 @@ class _Debugger():
def _display_all_vars(self, dict):
"""
- :param dict:
+ :param dict:
"""
num_cols = 3
@@ -10204,7 +10266,7 @@ class _Debugger():
def _choose_auto_watches(self, my_locals):
"""
- :param my_locals:
+ :param my_locals:
"""
ChangeLookAndFeel(COLOR_SCHEME)
@@ -10281,7 +10343,7 @@ class _Debugger():
def _build_floating_window(self, location=(None, None)):
"""
- :param location:
+ :param location:
"""
if self.popout_window: # if floating window already exists, close it first
@@ -10393,8 +10455,8 @@ class _Debugger():
def show_debugger_window(location=(None, None), *args):
"""
- :param location:
- :param *args:
+ :param location:
+ :param *args:
"""
if _Debugger.debugger is None:
@@ -10417,8 +10479,8 @@ def show_debugger_window(location=(None, None), *args):
def show_debugger_popout_window(location=(None, None), *args):
"""
- :param location:
- :param *args:
+ :param location:
+ :param *args:
"""
if _Debugger.debugger is None:
@@ -10439,13 +10501,17 @@ def show_debugger_popout_window(location=(None, None), *args):
debugger._build_floating_window(location=location)
-def refresh_debugger():
- """ """
+def _refresh_debugger():
+ """
+ Refreshes the debugger windows. USERS should NOT be calling this function. Within PySimpleGUI it is called for the USER every time the Window.Read function is called.
+
+ :return: rc (bool) False if user closed the main debugger window.
+ """
if _Debugger.debugger is None:
_Debugger.debugger = _Debugger()
debugger = _Debugger.debugger
Window.read_call_from_debugger = True
- frame = inspect.currentframe()
+ # frame = inspect.currentframe()
frame = inspect.currentframe().f_back
# frame, *others = inspect.stack()[1]
try:
@@ -10461,24 +10527,23 @@ def refresh_debugger():
+# d8b
+# Y8P
+#
+# 88888b.d88b. 8888b. 888 88888b.
+# 888 "888 "88b "88b 888 888 "88b
+# 888 888 888 .d888888 888 888 888
+# 888 888 888 888 888 888 888 888
+# 888 888 888 "Y888888 888 888 888
-"""
- d8b
- Y8P
-
-88888b.d88b. 8888b. 888 88888b.
-888 "888 "88b "88b 888 888 "88b
-888 888 888 .d888888 888 888 888
-888 888 888 888 888 888 888 888
-888 888 888 "Y888888 888 888 888
-
-"""
-
-
def main():
- """ """
+ """
+ The PySimpleGUI "Test Harness". This is meant to be a super-quick test of the Elements.
+
+ :return:
+ """
from random import randint
ChangeLookAndFeel('GreenTan')
@@ -10546,7 +10611,6 @@ def main():
tab2 = Tab('Graph Number 2', [[]])
layout1 = [
- [Menu(menu_def)],
[Image(data=DEFAULT_BASE64_ICON)],
[Text('You are running the py file itself', font='ANY 15', tooltip='My tooltip', key='_TEXT1_')],
[Text('You should be importing it rather than running it', font='ANY 15')],
@@ -10564,7 +10628,7 @@ def main():
Button('Exit', tooltip='Exit button')],
]
- layout = [[Column(layout1)]]
+ layout = [[Menu(menu_def, key='_MENU_')],[Column(layout1)]]
window = Window('Window Title', layout,
font=('Helvetica', 13),
@@ -10593,10 +10657,12 @@ def main():
i += 1
if event == 'Button':
window.Element('_TEXT1_').SetTooltip('NEW TEXT')
- window.SetTransparentColor('#9FB8AD')
- window.Maximize()
+ # window.SetTransparentColor('#9FB8AD')
+ # window.Maximize()
+ window.Element('_MENU_').Update(visible=True)
elif event == 'Normal':
window.Normal()
+ window.Element('_MENU_').Update(visible=False)
elif event == 'Popout':
show_debugger_popout_window()
elif event == 'Launch Debugger':
@@ -10604,7 +10670,7 @@ def main():
# TimerStop()
window.Close()
-
+# -------------------------------- ENTRY POINT IF RUN STANDALONE -------------------------------- #
if __name__ == '__main__':
main()
exit(69)
diff --git a/docs/index.md b/docs/index.md
index 21446bdf..16e84cc3 100644
--- a/docs/index.md
+++ b/docs/index.md
@@ -1,5 +1,3 @@
-
-
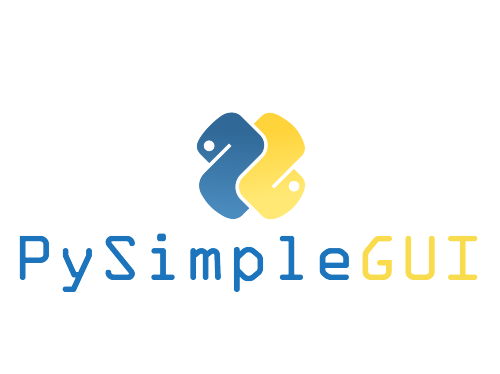
[](http://pepy.tech/project/pysimplegui) tkinter
@@ -518,6 +516,14 @@ Dictionaries are used by more advanced PySimpleGUI users. You'll know that dict
"High level calls" are those that start with "Popup". They are the most basic form of communications with the user. They are named after the type of window they create, a pop-up window. These windows are meant to be short lived while, either delivering information or collecting it, and then quickly disappearing.
+Think of Popups as your first windows, sorta like your first bicycle. It worked well, but was limited. It probably wasn't long before you wanted more features and it seemed too limiting for your newly found sense of adventure.
+
+When you've reached the point with Popups that you are thinking of filing a GitHub "Enhancement Issue" to get the Popup call extended to include a new feature that you think would be helpful.... not just to you but others is what you had in mind, right? For the good of others.
+
+It's at THIS time that you should immediately turn to the section entitled "Custom Window API Calls - Your First Window". Congratulations, you just graduated and are not an official "GUI Designer". Oh, nevermind that you only started learning Python 2 weeks ago, you're a real GUI Designer now so buck up and start acting like one.
+
+But, for now, let's stick with these 1-line window calls, the Popups.
+
## Popup Output
Think of the `Popup` call as the GUI equivalent of a `print` statement. It's your way of displaying results to a user in the windowed world. Each call to Popup will create a new Popup window.
@@ -572,24 +578,54 @@ Preview of popups:
+Popup - Display a popup Window with as many parms as you wish to include. This is the GUI equivalent of the
+"print" statement. It's also great for "pausing" your program's flow until the user can read some error messages
+
```
-Popup(*args, Variable number of arguments you want to display
- button_color=None, Color of buttons (text_color, background_color)
- background_color=None, Color of background
- text_color=None, Color of text
- button_type=POPUP_BUTTONS_OK, Type of button layout
- auto_close=False, If True window will automatically close
- auto_close_duration=None, Number of seconds for autoclose
- non_blocking=False, If True returns immediately
- icon=DEFAULT_WINDOW_ICON, Icon to use on the taskbar
- line_width=None, Width of lines in characters
- font=None, Font to use for characters
- no_titlebar=False, If True no titlebar will be shown
- grab_anywhere=False, If True can move window by grabbing anywhere
- keep_on_top=False, If True window will be on top of other windows
- location=(None,None)): (x,y) coordinates to show the window
+Popup(args,
+ title=None,
+ button_color=None,
+ background_color=None,
+ text_color=None,
+ button_type=0,
+ auto_close=False,
+ auto_close_duration=None,
+ custom_text=(None, None),
+ non_blocking=False,
+ icon=None,
+ line_width=None,
+ font=None,
+ no_titlebar=False,
+ grab_anywhere=False,
+ keep_on_top=False,
+ location=(None, None))
```
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|*args|Variable number of your arguments. Load up the call with stuff to see!|
+|title|Optional title for the window|
+|button_color|Tuple(str, str) Color of the buttons shown (text color, button color)|
+|background_color|(str) Window background color|
+|text_color|(str) text color|
+|button_type|(enum) determines which pre-defined buttons will be shown (Default value = POPUP_BUTTONS_OK). The user normally will NOT change this value. There are many Popup functions and they call Popup, changing this parameter to get the desired effect.|
+|auto_close|(bool) If True the window will automatically close|
+|auto_close_duration|(int) time in seconds to keep window open before closing it automatically|
+|custom_text|Union[tuple(str, str), str] A string or pair of strings that contain the text to display on the buttons|
+|non_blocking|(bool) If True then will immediately return from the function without waiting for the uder's input.|
+|icon|Union[str, bytes] icon to display on the window. Same format was Window call|
+|line_width|(int) Width of lines in characters to use. Defaults to MESSAGE_BOX_LINE_WIDTH|
+|font|Union[str, tuple(font, size, modifiors) specifies the font family, size, etc|
+|no_titlebar|(bool) If True will not show the frame around the window and the titlebar across the top|
+|grab_anywhere|(bool) If True can grab anywhere to move the window. If no_titlebar is True, grab_anywhere should likely be enabled too|
+|location|(int, int) Location on screen to display the top left corner of window. Defaults to centered on screen|
+|||
+| **return** | Union[str, None] Returns text of the button that was pressed. None will be returned if user closed window with X |
+
+The other output Popups are variations on parameters. Usually the button_type parameter is the primary one changed.
+
The other output Popups are variations on parameters. Usually the button_type parameter is the primary one changed.
The choices for button_type are:
@@ -601,7 +637,8 @@ POPUP_BUTTONS_OK_CANCEL
POPUP_BUTTONS_OK
POPUP_BUTTONS_NO_BUTTONS
```
-Note that you should not call Popup yourself with different button_types. Rely on the Popup function named that sets that value for you. For example PopupYesNo will set the button type to POPUP_BUTTONS_YES_NO for you.
+
+**Note that you should not call Popup yourself with different button_types.** Rely on the Popup function named that sets that value for you. For example PopupYesNo will set the button type to POPUP_BUTTONS_YES_NO for you.
#### Scrolled Output
There is a scrolled version of Popups should you have a lot of information to display.
@@ -642,22 +679,45 @@ Use these Popups instead of making a custom window to get one data value, call
### PopupGetText
Use this Popup to get a line of text from the user.
+Display Popup with text entry field
+
```
-PopupGetText(message,The message you wish to display with the input field
- default_text='', Text to initially fill into the input field
- password_char='', Passwork character if this is a password field
- size=(None,None), Size of the window
- button_color=None, Color to use for buttons (foreground, background)
- background_color=None, Background color for window
- text_color=None, Text color for window
- icon=DEFAULT_WINDOW_ICON, Icon to display on taskbar
- font=None, Font to use for text
- no_titlebar=False, If True no titlebar will be shown
- grab_anywhere=False, If True can grab anywhere to move the window
- keep_on_top=False, If True window will stay on top of other windows
- location=(None,None)) Location on screen to display window
+PopupGetText(message,
+ title=None,
+ default_text="",
+ password_char="",
+ size=(None, None),
+ button_color=None,
+ background_color=None,
+ text_color=None,
+ icon=None,
+ font=None,
+ no_titlebar=False,
+ grab_anywhere=False,
+ keep_on_top=False,
+ location=(None, None))
```
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|message||
+|title||
+|default_text|(Default value = '')|
+|password_char|Passwork character if this is a password field (Default value = '')|
+|size|(w,h) w=characters-wide, h=rows-high|
+|button_color|button color (foreground, background)|
+|background_color|color of background|
+|text_color|color of the text|
+|icon|Icon to display|
+|font|specifies the font family, size, etc|
+|no_titlebar|(Default = False)|
+|grab_anywhere|If True can grab anywhere to move the window (Default = False)|
+|location|(Default = (None))|
+|||
+| **return** | Union[str, None] Text entered or None if window was closed |
+
```python
import PySimpleGUI as sg
text = sg.PopupGetText('Title', 'Please input something')
@@ -671,25 +731,55 @@ sg.Popup('Results', 'The value returned from PopupGetText', text)
### PopupGetFile
Gets a filename from the user. There are options to configure the type of dialog box to show. Normally an "Open File" dialog box is shown.
+Display popup with text entry field and browse button. Browse for file
+
```
-PopupGetFile(message, Message to show in the window
- default_path='', Path browsing should start from
- default_extension='', Which filetype is the default
- save_as=False, Determines which dialog box stype to show
- file_types=(("ALL Files", "*.*"),), Which filetypes are displayed
- no_window=False, if True no window is displayed except the dialog box
- size=(None,None), Size of window
- button_color=None, Color of buttons
- background_color=None, Color of window background
- text_color=None, Color of text in window
- icon=DEFAULT_WINDOW_ICON, Icon to show on taskbar
- font=None, Font to use
- no_titlebar=False, If True does not display a titlebar
- grab_anywhere=False, if True can grab window anywhere to move it
- keep_on_top=False, if True window will be on top of others
- location=(None,None)) Location on screen to show window
+PopupGetFile(message,
+ title=None,
+ default_path="",
+ default_extension="",
+ save_as=False,
+ multiple_files=False,
+ file_types=(('ALL Files', '*.*'),),
+ no_window=False,
+ size=(None, None),
+ button_color=None,
+ background_color=None,
+ text_color=None,
+ icon=None,
+ font=None,
+ no_titlebar=False,
+ grab_anywhere=False,
+ keep_on_top=False,
+ location=(None, None),
+ initial_folder=None)
```
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|message||
+|title||
+|default_path|(Default value = '')|
+|default_extension|(Default value = '')|
+|save_as|(Default = False)|
+|multiple_files|(Default = False)|
+|file_types|(Default value = (("ALL Files", "*.*")))|
+|no_window|(Default = False)|
+|size|(w,h) w=characters-wide, h=rows-high|
+|button_color|button color (foreground, background)|
+|background_color|color of background|
+|text_color|color of the text|
+|icon|Icon to display|
+|font|specifies the font family, size, etc|
+|no_titlebar|(Default = False)|
+|grab_anywhere|If True can grab anywhere to move the window (Default = False)|
+|location|(Default = (None))|
+|initial_folder||
+|||
+| **return** | Union[str, None] string representing the path chosen, None if cancelled or window closed with X |
+
If configured as an Open File Popup then (save_as is not True) the dialog box will look like this.
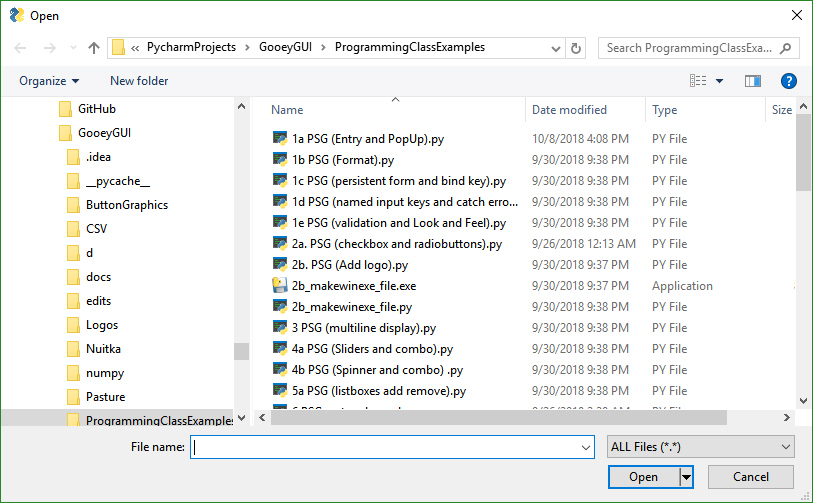
@@ -713,22 +803,47 @@ sg.Popup('Results', 'The value returned from PopupGetFile', text)
The window created to get a folder name looks the same as the get a file name. The difference is in what the browse button does. `PopupGetFile` shows an Open File dialog box while `PopupGetFolder` shows an Open Folder dialog box.
+Display popup with text entry field and browse button. Browse for folder
+
```
-PopupGetFolder(message, Message to display in window
- default_path='', Path to start browsing
- no_window=False, If True no window will be shown
- size=(None,None), Size of window
- button_color=None, Color of buttons
- background_color=None, Background color of window
- text_color=None, Color of window text
- icon=DEFAULT_WINDOW_ICON, Icon to show on taskbar
- font=None, Font to use for window
- no_titlebar=False, If True no titlebar will be shown
- grab_anywhere=False, If True can grab anywhere on window to move
- keep_on_top=False, If True window will be on top
- location=(None, None)) Location on screen to create window
+PopupGetFolder(message,
+ title=None,
+ default_path="",
+ no_window=False,
+ size=(None, None),
+ button_color=None,
+ background_color=None,
+ text_color=None,
+ icon=None,
+ font=None,
+ no_titlebar=False,
+ grab_anywhere=False,
+ keep_on_top=False,
+ location=(None, None),
+ initial_folder=None)
```
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|message||
+|title||
+|default_path|(Default value = '')|
+|no_window|(Default = False)|
+|size|(w,h) w=characters-wide, h=rows-high|
+|button_color|button color (foreground, background)|
+|background_color|color of background|
+|text_color|color of the text|
+|icon|Icon to display|
+|font|specifies the font family, size, etc|
+|no_titlebar|(Default = False)|
+|grab_anywhere|If True can grab anywhere to move the window (Default = False)|
+|location|(Default = (None))|
+|initial_folder||
+|||
+| **return** | Union[str, None] Contents of text field. None if closed using X or cancelled |
+
This is a typpical call
```python
@@ -744,34 +859,38 @@ This is a typpical call
The animated Popup enables you to easily display a "loading" style animation specified through a GIF file that is either stored in a file or a base64 variable.
-```python
+"Plays" an animated GIF file. This function has its own internal clocking meaning you can call it at any frequency
+ and the rate the frames of video is shown remains constant. Maybe your frames update every 30 ms but your
+ event loop is running every 10 ms.
+
+```
PopupAnimated(image_source,
- message=None,
- background_color=None,
- text_color=None,
- font=None,
- no_titlebar=True,
- grab_anywhere=True,
- keep_on_top=True,
- location=(None, None),
- alpha_channel=None,
- time_between_frames=0,
- transparent_color=None)
+ message=None,
+ background_color=None,
+ text_color=None,
+ font=None,
+ no_titlebar=True,
+ grab_anywhere=True,
+ keep_on_top=True,
+ location=(None, None),
+ alpha_channel=None,
+ time_between_frames=0,
+ transparent_color=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|image_source||
-|message||
-|background_color|color of background|
-|text_color|color of the text|
-|font|specifies the font family, size, etc|
-|no_titlebar|(Default = True)|
-|grab_anywhere|(Default = True)|
-|keep_on_top|(Default = True)|
-|location||
+|image_source|Union[str, bytes] Either a filename or a base64 string.|
+|message|An optional message to be shown with the animation|
+|background_color|(str) color of background|
+|text_color|(str) color of the text|
+|font|Union[str, tuple) specifies the font family, size, etc|
+|no_titlebar|(bool) If True then the titlebar and window frame will not be shown|
+|grab_anywhere|(bool) If True then you can move the window just clicking anywhere on window, hold and drag|
+|keep_on_top|(bool) If True then Window will remain on top of all other windows currently shownn|
+|location|(int, int) (x,y) location on the screen to place the top left corner of your window. Default is to center on screen|
|alpha_channel||
|time_between_frames|(Default value = 0)|
|transparent_color||
@@ -1411,70 +1530,335 @@ You can learn more about these async / non-blocking windows toward the end of th
# Window Object - Beginning a window
The first step is to create the window object using the desired window customization.
-This is the definition of the Window object:
+ Represents a single Window
-```python
-Window( title,
- default_element_size=DEFAULT_ELEMENT_SIZE,
- default_button_element_size=(None,None),
- auto_size_text=None,
- auto_size_buttons=None,
- location=(None,None),
- size=(None,None),
- element_padding=None,
- button_color=None,
- font=None,
- progress_bar_color=(None,None),
- background_color=None,
- border_depth=None,
- auto_close=False,
- auto_close_duration=DEFAULT_AUTOCLOSE_TIME,
- icon=DEFAULT_WINDOW_ICON,
- force_toplevel=False,
- alpha_channel=1,
- return_keyboard_events=False,
- use_default_focus=True,
- text_justification=None,
- no_titlebar=False,
- grab_anywhere=False,
- keep_on_top=False,
- resizable=False,
- disable_close=False,
- disable_minimize=False,
- right_click_menu=None):
+```
+Window(title,
+ layout=None,
+ default_element_size=(45, 1),
+ default_button_element_size=(None, None),
+ auto_size_text=None,
+ auto_size_buttons=None,
+ location=(None, None),
+ size=(None, None),
+ element_padding=None,
+ margins=(None, None),
+ button_color=None,
+ font=None,
+ progress_bar_color=(None, None),
+ background_color=None,
+ border_depth=None,
+ auto_close=False,
+ auto_close_duration=3,
+ icon=None,
+ force_toplevel=False,
+ alpha_channel=1,
+ return_keyboard_events=False,
+ use_default_focus=True,
+ text_justification=None,
+ no_titlebar=False,
+ grab_anywhere=False,
+ keep_on_top=False,
+ resizable=False,
+ disable_close=False,
+ disable_minimize=False,
+ right_click_menu=None,
+ transparent_color=None,
+ debugger_enabled=True)
```
-Parameter Descriptions. You will find these same parameters specified for each `Element` and some of them in `Row` specifications. The `Element` specified value will take precedence over the `Row` and `window` values.
+Parameter Descriptions:
|Name|Meaning|
-| ----- | ---- |
-| default_element_size | Size of elements in window in characters (width, height) |
-| default_button_element_size | Size of buttons on this window |
-| auto_size_text | Bool. True if elements should size themselves according to contents. Defaults to True |
-| auto_size_buttons | Bool. True if button elements should size themselves according to their text label |
-| location | (x,y) Location to place window in pixels |
-| size | (w,h) forces a window to be a paricular size|
-| element_padding | (w,h) default padding amount for elements|
-| font | Font name and size for elements of the window |
-| button_color | Default color for buttons (foreground, background). Can be text or hex |
-| progress_bar_color | Foreground and background colors for progress bars |
-| background_color | Color of the window background |
-| border_depth | Amount of 'bezel' to put on input boxes, buttons, etc. |
-| auto_close | Bool. If True window will autoclose |
-| auto_close_duration | Duration in seconds before window closes |
-| icon | .ICO file that will appear on the Task Bar and end of Title Bar |
-| force_top_level | Bool. If set causes a tk.Tk window to be used as primary window rather than tk.TopLevel. Used to get around Matplotlib problem |
-| alpha_channel | Float 0 to 1. 0 is invisible, 1 is fully visible, Anything between will be semi-transparent |
-| return_keyboard_events | if True key presses are returned as buttons |
-| use_default_focus | if True and no focus set, then automatically set a focus |
-| text_justification | Justification to use for Text Elements in this window |
-| no_titlebar | Create window without a titlebar |
-| grab_anywhere | Grab any location on the window to move the window |
-| keep_on_top | if True then window will always stop on top of other windows on the screen. Great for floating toolbars. |
-| resizable | if True | user can manually changge the wize of the window. Defaults to False|
-| disable_close | if True user will not be able to close using the X. |
-| disable_minimize | if True user will not be able to minimize the window|
-| right_click_menu | menu definition that will be used on wall elements that support right click. If a definition is specified on an element then it will be used instead.|
+|---|---|
+|title||
+|layout||
+|default_element_size|(Default value = DEFAULT_ELEMENT_SIZE)|
+|default_button_element_size||
+|auto_size_text|True if size should fit the text length|
+|auto_size_buttons||
+|location|Location on screen to display|
+|size|(w,h) w=characters-wide, h=rows-high (Default = (None))|
+|element_padding||
+|margins|(Default = (None))|
+|button_color|button color (foreground, background)|
+|font|specifies the font family, size, etc|
+|progress_bar_color|(Default = (None))|
+|background_color|color of background|
+|border_depth||
+|auto_close|(Default = False)|
+|auto_close_duration|(Default value = DEFAULT_AUTOCLOSE_TIME)|
+|icon|Icon to display. Filled in with default icon in init (Default value = None)|
+|force_toplevel|(Default = False)|
+|alpha_channel|(Default value = 1)|
+|return_keyboard_events|(Default = False)|
+|use_default_focus|(Default = True)|
+|text_justification||
+|no_titlebar|(Default = False)|
+|grab_anywhere|If True can grab anywhere to move the window (Default = False)|
+|resizable|(Default = False)|
+|disable_close|(Default = False)|
+|disable_minimize|(Default = False)|
+|right_click_menu|List[List[str]] see "Right Click Menus" for format|
+|transparent_color||
+|debugger_enabled|(Default = True)|
+
+## Methods
+
+### AddRow
+
+Parms are a variable number of Elements
+
+```
+AddRow(args)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|*args||
+
+### AddRows
+
+```
+AddRows(rows)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|rows||
+
+### BringToFront
+
+```python
+BringToFront()
+```
+
+### Close
+
+```python
+Close()
+```
+
+```python
+CurrentLocation()
+```
+
+```python
+Disable()
+```
+
+```python
+DisableDebugger()
+```
+
+```python
+Disappear()
+```
+
+Find element object associated with the provided key
+:returns Found element object, an Error Element, or None
+
+```
+Element(key,
+ silent_on_error=False)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|key|Used with window.FindElement and with return values to uniquely identify this element|
+|silent_on_error|(Default = False)|
+
+```python
+Enable()
+```
+
+```python
+EnableDebugger()
+```
+
+```
+Fill(values_dict)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|values_dict|?????????????????|
+
+```python
+Finalize()
+```
+
+Find element object associated with the provided key
+:returns Found element object, an Error Element, or None
+
+```
+Find(key,
+ silent_on_error=False)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|key|Used with window.FindElement and with return values to uniquely identify this element|
+|silent_on_error|(Default = False)|
+
+Find element object associated with the provided key
+:returns Found element object, an Error Element, or None
+
+```
+FindElement(key,
+ silent_on_error=False)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|key|Used with window.FindElement and with return values to uniquely identify this element|
+|silent_on_error|(Default = False)|
+
+```python
+FindElementWithFocus()
+```
+
+```python
+GetScreenDimensions()
+```
+
+```python
+GrabAnyWhereOff()
+```
+
+```python
+GrabAnyWhereOn()
+```
+
+```python
+Hide()
+```
+
+```
+Layout(rows)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|rows||
+
+```
+LoadFromDisk(filename)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|filename|?????????????????|
+
+```python
+Maximize()
+```
+
+```python
+Minimize()
+```
+
+```
+Move(x,
+ y)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|x|x coordinate|
+|y|y coordinate|
+
+```python
+Normal()
+```
+
+```
+Read(timeout=None,
+ timeout_key="__TIMEOUT__")
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|timeout|timeout to wait, till Read will execute itself|
+|timeout_key|(Default value = TIMEOUT_KEY)|
+
+```python
+Reappear()
+```
+
+```python
+Refresh()
+```
+
+```
+SaveToDisk(filename)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|filename|?????????????????|
+
+```
+SetAlpha(alpha)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|alpha||
+
+```
+SetIcon(icon=None,
+ pngbase64=None)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|icon||
+|pngbase64||
+
+```
+SetTransparentColor(color)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|color||
+
+```python
+UnHide()
+```
+
+```python
+VisibilityChanged()
+```
### Window Location
PySimpleGUI computes the exact center of your window and centers the window on the screen. If you want to locate your window elsewhere, such as the system default of (0,0), if you have 2 ways of doing this. The first is when the window is created. Use the `location` parameter to set where the window. The second way of doing this is to use the `SetOptions` call which will set the default window location for all windows in the future.
@@ -1528,33 +1912,6 @@ To keep a window on top of all other windows on the screen, set keep_on_top = Tr
PySimpleGUI will set a default focus location for you. This generally means the first input field. You can set the focus to a particular element. If you are going to set the focus yourself, then you should turn off the automatic focus by setting `use_default_focus=False` in your Window call.
-### Window Methods (things you can do with a Window object)
-
-There are a few methods (functions) that you will see in this document that act on Windows. The ones you will primarily be calling are:
-
- window.Layout(layout) - Recommend moving away from this nethod and using layout parameter instead. Turns your definition of the Window into Window
- window.Finalize() - creates the tkinter objects for the Window. Normally you do not call this
- window.Read() - Read the Windows values and get the button / key that caused the Read to return. Can have an optional timeout
- window.ReadNonBlocking() - NO LONGER USED!
- window.Refresh() - Use if updating elements and want to show the updates prior to the nex Read
- window.Fill(values_dict) - Fill each Element with entry from the dictionary passed in
- window.SaveToDisk(filename) - Save the Window's values to disk
- window.LoadFromDisk(filename) - Load the Window's values from disk
- window.Close() - To close your window, if a button hasn't already closed it
- window.Disable() - Use to disable the window inputwhen opening another window on top of the primnary Window
- window.Enable() - Re-enable a Disabled window
- window.FindElement(key, silent_on_error=False) - Returns the element that has a matching key value
- window.Element(key, silent_on_error=False) - EXACTLY the same as calling FindElement
- window.Move(x,y) - Moves window to location x,y on screen'
- window.SetAlpha(alpha) - Changes window transparency
- window.BringToFront() - Brings the window to the top of other windows on the screen
- window.Disappear(), Reappear() - Uses alpha channel to make window disappear
- window.Hide(), UnHide() - Hides a window
- window.CurrentLocation() - Returns current window location
- window.Size = w,h - Forces a window to be a particular size. Note this is a property not a method
- window.Size - Tuple (w,h)The size of the current window. Note this is a property
- window.Minimize() - Minimizes window to taskbar
-
## Window Methods
There are a number of operations you can do on a window after you've created the window. You call these after creating your Windows object.
@@ -1585,33 +1942,7 @@ If you set the timeout = 0, then the Read will immediately return rather than wa
While this call will technically still work, it is being removed. If you want to get the same result, call Read with timeout = 0.
-Read the Window's input values and button clicks but without blocking. It will immediately return. **Consider using Read with non-zero timeout instead!**
-
-Will consume 100% of your CPU if you do not have other blocking calls in your event loop.
-
-|Name|Meaning|
-|-----|-----|
-| `Refresh() ` | Cause changes to the window to be displayed on the screen. Normally not needed unless the changes are immediately required or if it's going to be a while before another call to Read. |
-| `SetIcon(icon, pngbase64) ` | Sets the window's icon that will be shown on the titlebar. Can either be a filename or a base64 string.|
-| `Fill(values_dict) ` | Populates the windows fields with the values shown in the dictionary.|
-| `FindElementWithFocus() ` | Returns the Element that currently has the focus. Returns None if no Elements were found.|
-| `SaveToDisk(filename) ` | Saves the window's values to disk |
-| `LoadFromDisk(filename) ` | Fills in a window's fields based on previously saved file |
-| `GetScreenDimensions() ` | Returns the size (w,h) of the screen in pixels |
-| `CurrentLocation() ` | Returns current screen position (x,y) |
-| `Move(x, y) ` | Move window to (x,y) position on the screen |
-| `Minimize() ` | Sends the window to the taskbar |
-| `Close() ` | Closes a window, blocking or non-blocking|
-| `Disable() ` | Stops a window from responding until Enable is called|
-| `Enable() ` | Re-enables a previously disabled window|
-| `Hide() ` | Completely hides a window, including removing from the taskbar|
-| `UnHide() ` | Restores a window hidden using Hide|
-| `Disappear() ` | Makes a window disappear while leaving the icon on the taskbar|
-| `Reappear() ` | Makes a window reappear that was previously made to disappear using Disappear()|
-| `SetAlpha(alpha)` | Sets the window's transparency. 0 is completely transparent. 1 is fully visible, normal . Can also use the property Window.AlphaChannel instead of method function call|
-| CloseNonBlocking()
(NO LONGER USED.
use Close instead) | Closes a non-blocking window |
-| FindElement(key, silent_on_error=False) (shorthand version
Element) | Returns the Element that has a matching key. If the key is not found, an Error Element is returned so that the program will not crash should the user try to perform an "update". A Popup message will be shown |
-|||
+The only time you will see this call is in OLD CODE. Please file an Issue if you see this call in any code or in any documentation. It must go away never to be seen again.
# Elements
"Elements" are the building blocks used to create windows. Some GUI APIs use the term "Widget" to describe these graphic elements.
@@ -1733,8 +2064,8 @@ Many of the main method calls and Element names have shortcuts. This enables yo
## Text Element | `T == Txt == Text`
Basic Element. It displays text. That's it.
- Text - Display some text in the window. Can be single or multiple lines but no scrolling if multiple lines.
-
+ Text - Display some text in the window. Usually this means a single line of text. However, the text can also
+ be multiple lines. If multi-lined there are no scroll bars.
```python
layout = [
@@ -1744,63 +2075,81 @@ layout = [
```
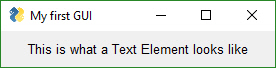
-```python
+```
Text(text,
- size=(None, None),
- auto_size_text=None,
- click_submits=False,
- enable_events=False,
- relief=None,
- font=None,
- text_color=None,
- background_color=None,
- justification=None,
- pad=None,
- key=None,
- right_click_menu=None,
- tooltip=None,
- visible=True)
+ size=(None, None),
+ auto_size_text=None,
+ click_submits=False,
+ enable_events=False,
+ relief=None,
+ font=None,
+ text_color=None,
+ background_color=None,
+ justification=None,
+ pad=None,
+ key=None,
+ right_click_menu=None,
+ tooltip=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|text|The text to display (required)|
-|size|(w,h) w=characters-wide, h=rows-high|
-|auto_size_text|True if size should fit the text length|
-|click_submits|????????????????????(Default = False)|
-|enable_events|Turns on the element specific events.(Default = False)|
-|relief|relief style. Values are same as progress meter relief values. Can be a constant or a string: `RELIEF_RAISED RELIEF_SUNKEN RELIEF_FLAT RELIEF_RIDGE RELIEF_GROOVE RELIEF_SOLID`|
-|font|specifies the font family, size, etc|
-|text_color|color of the text|
-|background_color|color of background|
-|justification|justification for data display|
-|pad|Amount of padding to put around element|
-|key|Used with window.FindElement and with return values|
-|right_click_menu|see "Right Click Menus"|
-|tooltip|text, that will appear the you hover on|
-|visible|set visibility state of the element (Default = True)|
+|text|(str) The text to display. Can include /n to achieve multiple lines|
+|size|(int, int) (width, height) width = characters-wide, height = rows-high|
+|auto_size_text|(bool) if True size of the Text Element will be sized to fit the string provided in 'text' parm|
+|click_submits|(bool) DO NOT USE. Only listed for backwards compat - Use enable_events instead|
+|enable_events|(bool) Turns on the element specific events. Text events happen when the text is clicked|
+|relief|(str/enum) relief style around the text. Values are same as progress meter relief values. Should be a constant that is defined at starting with "RELIEF_" - `RELIEF_RAISED, RELIEF_SUNKEN, RELIEF_FLAT, RELIEF_RIDGE, RELIEF_GROOVE, RELIEF_SOLID`|
+|font|Union[str, tuple] specifies the font family, size, etc|
+|text_color|(str) color of the text|
+|background_color|(str) color of background|
+|justification|(str) how string should be aligned within space provided by size. Valid choices = `left`, `right`, `center`|
+|pad|(int, int) or ((int, int),(int,int)) Amount of padding to put around element (left/right, top/bottom) or ((left, right), (top, bottom))|
+|key|(Any) Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element|
+|right_click_menu|List[List[str]] see "Right Click Menus"|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
+|visible|(bool) set visibility state of the element|
### Methods
-```python
+#### Update
+
+Changes some of the settings for the Text Element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
Update(value=None,
- background_color=None,
- text_color=None,
- font=None,
- visible=None)
+ background_color=None,
+ text_color=None,
+ font=None,
+ visible=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|value|new value to show|
-|background_color|color of background|
-|text_color|color of the text|
-|font|specifies the font family, size, etc|
-|visible|change visibility of element|
+|value|(str) new text to show|
+|background_color|(str) color of background|
+|text_color|(str) color of the text|
+|font|Union[str, tuple] specifies the font family, size, etc|
+|visible|(bool) set visibility state of the element|
+
+#### SetToolTip
+
+Called by application to change the tooltip text for an Element. Normally invoked using the Element Object such as: window.Element('key').SetToolTip('New tip').
+
+```
+SetTooltip(tooltip_text)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|tooltip_text|(str) the text to show in tooltip.|
---
@@ -1827,7 +2176,7 @@ If you set the parameter `enable_events` or `click_submits` then you will get an
## Multiline Element
This Element doubles as both an input and output Element.
-
+ Multiline Element - Display and read multiple lines of text. This is both an input and output element.
```python
layout = [[sg.Multiline('This is what a Multi-line Text Element looks like', size=(45,5))]]
@@ -1835,109 +2184,149 @@ layout = [[sg.Multiline('This is what a Multi-line Text Element looks like', siz
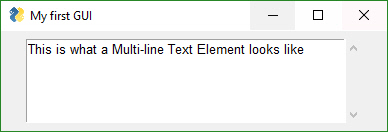
-```python
+```
Multiline(default_text="",
- enter_submits=False,
- disabled=False,
- autoscroll=False,
- border_width=None,
- size=(None, None),
- auto_size_text=None,
- background_color=None,
- text_color=None,
- change_submits=False,
- enable_events=False,
- do_not_clear=True,
- key=None,
- focus=False,
- font=None,
- pad=None,
- tooltip=None,
- right_click_menu=None,
- visible=True)
+ enter_submits=False,
+ disabled=False,
+ autoscroll=False,
+ border_width=None,
+ size=(None, None),
+ auto_size_text=None,
+ background_color=None,
+ text_color=None,
+ change_submits=False,
+ enable_events=False,
+ do_not_clear=True,
+ key=None,
+ focus=False,
+ font=None,
+ pad=None,
+ tooltip=None,
+ right_click_menu=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|default_text|text to display in multiline (Default value = '')|
-|enter_submits|???????????? (Default = False)|
-|disabled|set disable state for element (Default = False)|
-|autoscroll|?????????? (Default = False)|
-|border_width|width of border around element|
-|size|(w,h) w=characters-wide, h=rows-high|
-|auto_size_text|True if size should fit the text length|
-|background_color|color of background|
-|text_color|color of the text|
-|change_submits|If True, pressing Enter key submits window (Default = False)|
-|enable_events|Turns on the element specific events.(Default = False)|
-|do_not_clear|see docx (Default = True)|
-|key|Used with window.FindElement and with return values|
-|focus|if focus should be set to this|
-|font|specifies the font family, size, etc|
-|pad|Amount of padding to put around element|
-|tooltip|text, that will appear the you hover on|
-|right_click_menu|see "Right Click Menus"|
-|visible|set visibility state of the element (Default = True)|
+|default_text|(str) Initial text to show|
+|enter_submits|(bool) if True, the Window.Read call will return is enter key is pressed in this element|
+|disabled|(bool) set disable state|
+|autoscroll|(bool) If True the contents of the element will automatically scroll as more data added to the end|
+|border_width|(int) width of border around element in pixels|
+|size|(int, int) (width, height) width = characters-wide, height = rows-high|
+|auto_size_text|(bool) if True will size the element to match the length of the text|
+|background_color|(str) color of background|
+|text_color|(str) color of the text|
+|change_submits|(bool) DO NOT USE. Only listed for backwards compat - Use enable_events instead|
+|enable_events|(bool) Turns on the element specific events. Spin events happen when an item changes|
+|do_not_clear|if False the element will be cleared any time the Window.Read call returns|
+|key|(Any) Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element|
+|focus|(bool) if True initial focus will go to this element|
+|font|Union[str, tuple] specifies the font family, size, etc|
+|pad|(int, int) or ((int, int),(int,int)) Amount of padding to put around element (left/right, top/bottom) or ((left, right), (top, bottom))|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
+|right_click_menu|List[List[str]] see "Right Click Menus"|
+|visible|(bool) set visibility state of the element|
### Methods
-```python
+#### Update
+
+Changes some of the settings for the Multiline Element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
Update(value=None,
- disabled=None,
- append=False,
- font=None,
- text_color=None,
- background_color=None,
- visible=None,
- autoscroll=None)
+ disabled=None,
+ append=False,
+ font=None,
+ text_color=None,
+ background_color=None,
+ visible=None,
+ autoscroll=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|value|new text to display|
-|disabled|disable or enable state of the element|
-|append|(Default = False)|
-|font|specifies the font family, size, etc|
-|text_color|color of the text|
-|background_color|color of background|
-|visible|change visibility of element|
-|autoscroll|??????????????????|
+|value|(str) new text to display|
+|disabled|(bool) disable or enable state of the element|
+|append|(bool) if True then new value will be added onto the end of the current value. if False then contents will be replaced.|
+|font|Union[str, tuple] specifies the font family, size, etc|
+|text_color|(str) color of the text|
+|background_color|(str) color of background|
+|visible|(bool) set visibility state of the element|
+|autoscroll|(bool) if True then contents of element are scrolled down when new text is added to the end|
+
+#### Get
+
+Return current contents of the Multiline Element
+
+```
+Get() -> (str) current contents of the Multiline Input element
+```
+
+#### SetFocus
+
+Moves the focus to this Multiline
+
+```
+SetFocus(force=False)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|force|(bool). If True, will call focus_force instead of focus_set|
+
+#### SetTooltip
+
+Called by application to change the tooltip text for an Element. Normally invoked using the Element Object such as: window.Element('key').SetToolTip('New tip').
+
+```
+SetTooltip(tooltip_text)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|tooltip_text|(str) the text to show in tooltip.|
## Text Input Element | `InputText == Input == In`
Display a single text input field. Based on the tkinter Widget `Entry`
-
+
```python
layout = [[sg.InputText('Default text')]]
```

-```python
+```
InputText(default_text="",
- size=(None, None),
- disabled=False,
- password_char="",
- justification=None,
- background_color=None,
- text_color=None,
- font=None,
- tooltip=None,
- change_submits=False,
- enable_events=False,
- do_not_clear=True,
- key=None,
- focus=False,
- pad=None,
- right_click_menu=None,
- visible=True)
+ size=(None, None),
+ disabled=False,
+ password_char="",
+ justification=None,
+ background_color=None,
+ text_color=None,
+ font=None,
+ tooltip=None,
+ change_submits=False,
+ enable_events=False,
+ do_not_clear=True,
+ key=None,
+ focus=False,
+ pad=None,
+ right_click_menu=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -1949,7 +2338,7 @@ Parameters explained:
|background_color|(str) color of background in one of the color formats|
|text_color|(str) color of the text|
|font|(str / tuple) specifies the font family, size, etc|
-|tooltip|(str) Text shown when cursor hovers over the element|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
|change_submits|(bool) * DEPRICATED DO NOT USE! Same as enable_events|
|enable_events|(bool) If True then changes to this element are immediately reported as an event. Use this instead of change_submits (Default = False)|
|do_not_clear|(bool) If False then the field will be set to blank after ANY event (button, any event) (Default = True)|
@@ -1961,14 +2350,18 @@ Parameters explained:
### Methods
-```python
+#### Update
+
+Changes some of the settings for the Input Element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
Update(value=None,
- disabled=None,
- select=None,
- visible=None)
+ disabled=None,
+ select=None,
+ visible=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -1977,21 +2370,52 @@ Parameters explained:
|select|(bool) if True, then the text will be selected|
|visible|(bool) change visibility of element|
-Get() - Read and return the current value of the input element. Must call `Window.Read` or `Window.Finalize` prior
+#### Get
- :return: (str) current value of Input field or '' if error encountered
+Read and return the current value of the input element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
+Get() -> (str) current value of Input field or '' if error encountered
+```
+
+#### SetFocus
+
+Sets focus to this element using focus_set. Will use focus_force if force flag set. Must call `Window.Read` or `Window.Finalize` prior
+
+```
+SetFocus(force=False)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|force|(bool) if True then tkinter's `Entry.focus_force` will be called instead of `Entry.focus_set`|
+
+#### SetToolTip
+
+Called by application to change the tooltip text for an Element. Normally invoked using the Element Object such as: window.Element('key').SetToolTip('New tip').
+
+```
+SetTooltip(tooltip_text)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|tooltip_text|(str) the text to show in tooltip.|
---
-#### do_not_clear Parameter
+#### Note about the `do_not_clear` parameter
-**Important** - This trips a lot of people up. If you do not set the `do_not_clear` parameter then the input field will clear when an event takes place. The behavior is a "forms" style window development. The assumption is that you want the field to clear. If you are writing a chat program then you're thankful. The rest of you, I'm sorry.
+This used to really trip people up, but don't think so anymore. The `do_not_clear` parameter is initialized when creating the InputText Element. If set to False, then the input field's contents will be erased after every `Window.Read()` call. Use this setting for when your window is an "Input Form" type of window where you want all of the fields to be erased and start over again every time.
## Combo Element | `Combo == InputCombo == DropDown == Drop`
Also known as a drop-down list. Only required parameter is the list of choices. The return value is a string matching what's visible on the GUI.
ComboBox Element - A combination of a single-line input and a drop-down menu. User can type in their own value or choose from list.
-
```python
layout = [[sg.Combo(['choice 1', 'choice 2'])]]
@@ -1999,74 +2423,99 @@ layout = [[sg.Combo(['choice 1', 'choice 2'])]]
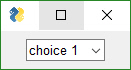
-```python
+```
Combo(values,
- default_value=None,
- size=(None, None),
- auto_size_text=None,
- background_color=None,
- text_color=None,
- change_submits=False,
- enable_events=False,
- disabled=False,
- key=None,
- pad=None,
- tooltip=None,
- readonly=False,
- font=None,
- visible=True)
+ default_value=None,
+ size=(None, None),
+ auto_size_text=None,
+ background_color=None,
+ text_color=None,
+ change_submits=False,
+ enable_events=False,
+ disabled=False,
+ key=None,
+ pad=None,
+ tooltip=None,
+ readonly=False,
+ font=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|values|values to choice|
-|default_value|Choices to be displayed. List of strings|
-|size|(w,h) w=characters-wide, h=rows-high|
-|auto_size_text|True if size should fit the text length|
-|background_color|color of background|
-|text_color|color of the text|
-|change_submits|(bool) DEPRICATED DO NOT USE. Use `enable_events` instead (Default = False)|
-|enable_events|(bool) Turns on the element specific events.(Default = False)|
-|disabled|(bool) set disable state for element (Default = False)|
-|key|(any) Used with window.FindElement and with return values|
+|values|List[Any] values to choose. While displayed as text, the items returned are what the caller supplied, not text|
+|default_value|(Any) Choice to be displayed as initial value. Must match one of values variable contents|
+|size|(int, int) (width, height) width = characters-wide, height = rows-high|
+|auto_size_text|(bool) True if element should be the same size as the contents|
+|background_color|(str) color of background|
+|text_color|(str) color of the text|
+|change_submits|(bool) DEPRICATED DO NOT USE. Use `enable_events` instead|
+|enable_events|(bool) Turns on the element specific events. Combo event is when a choice is made|
+|disabled|(bool) set disable state for element|
+|key|(Any) Used with window.FindElement and with return values to uniquely identify this element|
|pad|(int, int) or ((int, int),(int,int)) Amount of padding to put around element (left/right, top/bottom) or ((left, right), (top, bottom))|
|tooltip|(str) text that will appear when mouse hovers over this element|
-|readonly|(combo) make element readonly (user can't change). True means user cannot change (Default = False)|
-|font|(str, tuple) specifies the font family, size, etc|
-|visible|(bool) set visibility state of the element (Default = True)|
+|readonly|(bool) make element readonly (user can't change). True means user cannot change|
+|font|Union[str, tuple] specifies the font family, size, etc|
+|visible|(bool) set visibility state of the element|
### Methods
-```python
+#### Update
+
+Changes some of the settings for the Combo Element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
Update(value=None,
- values=None,
- set_to_index=None,
- disabled=None,
- readonly=None,
- font=None,
- visible=None)
+ values=None,
+ set_to_index=None,
+ disabled=None,
+ readonly=None,
+ font=None,
+ visible=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|value|change which value is current selected|
-|values|change list of choices|
-|set_to_index|change selection to a particular choice|
-|disabled|disable or enable state of the element|
-|readonly|make element readonly|
-|font|specifies the font family, size, etc|
-|visible|change visibility of element|
+|value|(Any) change which value is current selected hased on new list of previous list of choices|
+|values|List[Any] change list of choices|
+|set_to_index|(int) change selection to a particular choice starting with index = 0|
+|disabled|(bool) disable or enable state of the element|
+|readonly|(bool) if True make element readonly (user cannot change any choices)|
+|font|Union[str, tuple] specifies the font family, size, using one of the two font formats|
+|visible|(bool) control visibility of element|
+
+#### GetSelectedItemsIndexes
+
+Get the list of chosen items and return them as a list of indexes (offsets within the list)
+
+```
+GetSelectedItemsIndexes() -> List[int] List of indexes of currently selected items
+```
+
+#### SetToolTip
+
+Called by application to change the tooltip text for an Element. Normally invoked using the Element Object such as: window.Element('key').SetToolTip('New tip').
+
+```
+SetTooltip(tooltip_text)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|tooltip_text|(str) the text to show in tooltip.|
## Listbox Element
The standard listbox like you'll find in most GUIs. Note that the return values from this element will be a ***list of results, not a single result***. This is because the user can select more than 1 item from the list (if you set the right mode).
A List Box. Provide a list of values for the user to choose one or more of. Returns a list of selected rows
when a window.Read() is executed.
-
```python
layout = [[sg.Listbox(values=['Listbox 1', 'Listbox 2', 'Listbox 3'], size=(30, 6))]]
@@ -2074,80 +2523,121 @@ layout = [[sg.Listbox(values=['Listbox 1', 'Listbox 2', 'Listbox 3'], size=(30,
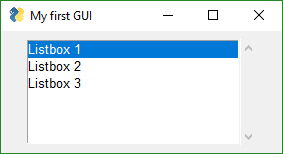
-```python
+```
Listbox(values,
- default_values=None,
- select_mode=None,
- change_submits=False,
- enable_events=False,
- bind_return_key=False,
- size=(None, None),
- disabled=False,
- auto_size_text=None,
- font=None,
- background_color=None,
- text_color=None,
- key=None,
- pad=None,
- tooltip=None,
- right_click_menu=None,
- visible=True)
+ default_values=None,
+ select_mode=None,
+ change_submits=False,
+ enable_events=False,
+ bind_return_key=False,
+ size=(None, None),
+ disabled=False,
+ auto_size_text=None,
+ font=None,
+ background_color=None,
+ text_color=None,
+ key=None,
+ pad=None,
+ tooltip=None,
+ right_click_menu=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|values|list of values to display. Can be any type including mixed types as long as they have __str__ method|
-|default_values|list of objects for listbox|
-|select_mode|can be a string or a constant value defined as a variable. Generally speaking strings are used for these kinds of options|
-|change_submits|If True, pressing Enter key submits window (Default = False)|
-|enable_events|Turns on the element specific events.(Default = False)|
-|bind_return_key|(Default = False)|
-|size|(w,h) w=characters-wide, h=rows-high|
-|disabled|set disable state for element (Default = False)|
-|auto_size_text|True if size should fit the text length|
-|font|specifies the font family, size, etc|
-|background_color|color of background|
-|text_color|color of the text|
-|key|Used with window.FindElement and with return values|
-|pad|Amount of padding to put around element|
-|tooltip|text, that will appear the you hover on|
-|right_click_menu|see "Right Click Menus"|
-|visible|set visibility state of the element (Default = True)|
+|values|List[Any] list of values to display. Can be any type including mixed types as long as they have __str__ method|
+|default_values|List[Any] which values should be initially selected|
+|select_mode|[str] can be a string or a constant value defined as a variable. Generally speaking strings are used for these kinds of options. Valid choices begin with "LISTBOX_SELECT_MODE_"|
+|change_submits|(bool) DO NOT USE. Only listed for backwards compat - Use enable_events instead|
+|enable_events|(bool) Turns on the element specific events. Listbox generates events when an item is clicked|
+|bind_return_key|(bool) If True, then the return key will cause a the Listbox to generate an event|
+|size|Tuple(int, int) (width, height) width = characters-wide, height = rows-high|
+|disabled|(bool) set disable state for element|
+|auto_size_text|(bool) True if element should be the same size as the contents|
+|font|Union[str, tuple] specifies the font family, size. Uses one of two font specifications formats|
+|background_color|(str) color of background|
+|text_color|(str) color of the text|
+|key|(Any) Used with window.FindElement and with return values to uniquely identify this element|
+|pad|(int, int) or ((int, int),(int,int)) Amount of padding to put around element (left/right, top/bottom) or ((left, right), (top, bottom))|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
+|right_click_menu|List[List[str]] see "Right Click Menus" for format|
+|visible|(bool) set visibility state of the element|
### Methods
-```python
+#### Update
+
+Changes some of the settings for the Listbox Element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
Update(values=None,
- disabled=None,
- set_to_index=None,
- scroll_to_index=None,
- visible=None)
+ disabled=None,
+ set_to_index=None,
+ scroll_to_index=None,
+ visible=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|values|new list of choices to be shown to user|
-|disabled|disable or enable state of the element|
-|set_to_index|highlights the item at this index as if user clicked|
-|scroll_to_index|scroll the listbox so that this index is the first shown|
-|visible|change visibility of element|
+|values|List[Any] new list of choices to be shown to user|
+|disabled|(bool) disable or enable state of the element|
+|set_to_index|(int) highlights the item at this index as if user clicked|
+|scroll_to_index|(int) scroll the listbox so that this index is the first shown|
+|visible|(bool) control visibility of element|
-```python
+#### SetValue
+
+Set listbox highlighted choices
+
+```
SetValue(values)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|values|new values to choice|
+|values|List[Any] new values to choose based on previously set values|
-GetListValues() - Returns list of Values provided by the user in the user's format
- :return: List. List of values. Can be any / mixed types -> []
+#### GetListValues
+
+Returns list of Values provided by the user in the user's format
+
+```
+GetListValues() -> List[Any]. List of values. Can be any / mixed types -> []
+```
+
+#### SetFocus
+
+Moves the focus to this Listbox
+
+```
+SetFocus(force=False)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|force|(bool). If True, will call focus_force instead of focus_set|
+
+#### SetToolTip
+
+Called by application to change the tooltip text for an Element. Normally invoked using the Element Object such as: window.Element('key').SetToolTip('New tip').
+
+```
+SetTooltip(tooltip_text)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|tooltip_text|(str) the text to show in tooltip.|
---
@@ -2159,8 +2649,6 @@ Another way ListBoxes can cause Reads to return is if the flag bind_return_key i
Sliders have a couple of slider-specific settings as well as appearance settings. Examples include the `orientation` and `range` settings.
-
-
```python
layout = [[sg.Slider(range=(1,500),
default_value=222,
@@ -2171,29 +2659,29 @@ layout = [[sg.Slider(range=(1,500),
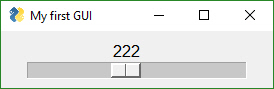
-```python
+```
Slider(range=(None, None),
- default_value=None,
- resolution=None,
- tick_interval=None,
- orientation=None,
- disable_number_display=False,
- border_width=None,
- relief=None,
- change_submits=False,
- enable_events=False,
- disabled=False,
- size=(None, None),
- font=None,
- background_color=None,
- text_color=None,
- key=None,
- pad=None,
- tooltip=None,
- visible=True)
+ default_value=None,
+ resolution=None,
+ tick_interval=None,
+ orientation=None,
+ disable_number_display=False,
+ border_width=None,
+ relief=None,
+ change_submits=False,
+ enable_events=False,
+ disabled=False,
+ size=(None, None),
+ font=None,
+ background_color=None,
+ text_color=None,
+ key=None,
+ pad=None,
+ tooltip=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -2212,9 +2700,9 @@ Parameters explained:
|font|specifies the font family, size, etc|
|background_color|color of background|
|text_color|color of the text|
-|key|Used with window.FindElement and with return values|
+|key|Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element|
|pad|Amount of padding to put around element|
-|tooltip|text, that will appear the you hover on|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
|visible|set visibility state of the element (Default = True)|
### Qt Sliders
@@ -2223,28 +2711,30 @@ There is an important difference between Qt and tkinter sliders. On Qt, the sli
### Slider Methods
-```python
+Changes some of the settings for the Slider Element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
Update(value=None,
- range=(None, None),
- disabled=None,
- visible=None)
+ range=(None, None),
+ disabled=None,
+ visible=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
|value|set current selection to value new value in slider|
|range|new range in slider|
-|disabled|disable or enable state of the element|
-|visible|change visibility of element|
+|disabled|(bool) disable or enable state of the element|
+|visible|(bool) control visibility of element|
## Radio Element
-Creates one radio button that is assigned to a group of radio buttons. Only 1 of the buttons in the group can be selected at any one time.
-Radio Button Element - Used in a group of other Radio Elements to provide user with ability to select only
- 1 choice in a list of choices.
-
+Creates one radio button that is assigned to a group of radio buttons. Only 1 of the buttons in the group can be selected at any one time.
+
+ Radio Button Element - Used in a group of other Radio Elements to provide user with ability to select only
+ 1 choice in a list of choices.
```python
layout = [
@@ -2255,128 +2745,187 @@ layout = [
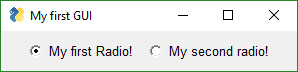
-```python
+```
Radio(text,
- group_id,
- default=False,
- disabled=False,
- size=(None, None),
- auto_size_text=None,
- background_color=None,
- text_color=None,
- font=None,
- key=None,
- pad=None,
- tooltip=None,
- change_submits=False,
- enable_events=False,
- visible=True)
+ group_id,
+ default=False,
+ disabled=False,
+ size=(None, None),
+ auto_size_text=None,
+ background_color=None,
+ text_color=None,
+ font=None,
+ key=None,
+ pad=None,
+ tooltip=None,
+ change_submits=False,
+ enable_events=False,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|text|Text to display next to button|
-|group_id|Groups together multiple Radio Buttons. Can be any value|
-|default|Bool. Initial state (Default = False)|
-|disabled|set disable state for element (Default = False)|
-|size|(w,h) w=characters-wide, h=rows-high|
-|auto_size_text|True if size should fit the text length|
-|background_color|color of background|
-|text_color|color of the text|
-|font|specifies the font family, size, etc|
-|key|Used with window.FindElement and with return values|
-|pad|Amount of padding to put around element|
-|tooltip|text, that will appear the you hover on|
-|change_submits|If True, pressing Enter key submits window (Default = False)|
-|enable_events|Turns on the element specific events.(Default = False)|
-|visible|set visibility state of the element (Default = True)|
+|text|(str) Text to display next to button|
+|group_id|(Any) Groups together multiple Radio Buttons. Any type works|
+|default|(bool). Set to True for the one element of the group you want initially selected|
+|disabled|(bool) set disable state|
+|size|(int, int) (width, height) width = characters-wide, height = rows-high|
+|auto_size_text|(bool) if True will size the element to match the length of the text|
+|background_color|(str) color of background|
+|text_color|(str) color of the text|
+|font|Union[str, tuple] specifies the font family, size, etc|
+|key|(Any) Used with window.FindElement and with return values to uniquely identify this element|
+|pad|(int, int) or ((int, int),(int,int)) Amount of padding to put around element (left/right, top/bottom) or ((left, right), (top, bottom))|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
+|change_submits|(bool) DO NOT USE. Only listed for backwards compat - Use enable_events instead|
+|enable_events|(bool) Turns on the element specific events. Radio Button events happen when an item is selected|
+|visible|(bool) set visibility state of the element|
### Methods
-```python
+#### Update
+
+Changes some of the settings for the Radio Button Element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
Update(value=None,
- disabled=None,
- visible=None)
+ disabled=None,
+ visible=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|value|Bool. if True change to selected|
-|disabled|disable or enable state of the element|
-|visible|change visibility of element|
+|value|(bool) if True change to selected and set others in group to unselected|
+|disabled|(bool) disable or enable state of the element|
+|visible|(bool) control visibility of element|
+
+#### Get
+
+A snapshot of the value of Radio Button -> (bool)
+
+```
+Get() -> (bool) True if this radio button is selected
+```
+
+#### ResetGroup
+
+Sets all Radio Buttons in the group to not selected
+
+```
+ResetGroup() ->
+```
+
+#### SetTooltip
+
+Called by application to change the tooltip text for an Element. Normally invoked using the Element Object such as: window.Element('key').SetToolTip('New tip').
+
+```
+SetTooltip(tooltip_text)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|tooltip_text|(str) the text to show in tooltip.|
## Checkbox Element | `CBox == CB == Check`
Checkbox elements are like Radio Button elements. They return a bool indicating whether or not they are checked.
-
+ Checkbox Element - Displays a checkbox and text next to it
```python
layout = [[sg.Checkbox('My first Checkbox!', default=True), sg.Checkbox('My second Checkbox!')]]
```

-```python
+```
Checkbox(text,
- default=False,
- size=(None, None),
- auto_size_text=None,
- font=None,
- background_color=None,
- text_color=None,
- change_submits=False,
- enable_events=False,
- disabled=False,
- key=None,
- pad=None,
- tooltip=None,
- visible=True)
+ default=False,
+ size=(None, None),
+ auto_size_text=None,
+ font=None,
+ background_color=None,
+ text_color=None,
+ change_submits=False,
+ enable_events=False,
+ disabled=False,
+ key=None,
+ pad=None,
+ tooltip=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|text|Text to display next to checkbox|
-|default|Bool + None. Initial state. True = Checked, False = unchecked, None = Not available (grayed out) (Default = False)|
-|size|(w,h) w=characters-wide, h=rows-high|
-|auto_size_text|True if size should fit the text length|
-|font|specifies the font family, size, etc|
-|background_color|color of background|
-|text_color|color of the text|
-|change_submits|If True, pressing Enter key submits window (Default = False)|
-|enable_events|Turns on the element specific events.(Default = False)|
-|disabled|set disable state for element (Default = False)|
-|key|Used with window.FindElement and with return values|
-|pad|Amount of padding to put around element|
-|tooltip|text, that will appear the you hover on|
-|visible|set visibility state of the element (Default = True)|
+|text|(str) Text to display next to checkbox|
+|default|(bool). Set to True if you want this checkbox initially checked|
+|size|(int, int) (width, height) width = characters-wide, height = rows-high|
+|auto_size_text|(bool) if True will size the element to match the length of the text|
+|font|Union[str, tuple] specifies the font family, size, etc|
+|background_color|(str) color of background|
+|text_color|(str) color of the text|
+|change_submits|(bool) DO NOT USE. Only listed for backwards compat - Use enable_events instead|
+|enable_events|(bool) Turns on the element specific events. Checkbox events happen when an item changes|
+|disabled|(bool) set disable state|
+|key|(Any) Used with window.FindElement and with return values to uniquely identify this element|
+|pad|(int, int) or ((int, int),(int,int)) Amount of padding to put around element (left/right, top/bottom) or ((left, right), (top, bottom))|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
+|visible|(bool) set visibility state of the element|
### Methods
-```python
+#### Update
+
+Changes some of the settings for the Checkbox Element. Must call `Window.Read` or `Window.Finalize` prior.
+Note that changing visibility may cause element to change locations when made visible after invisible
+
+```
Update(value=None,
- disabled=None,
- visible=None)
+ disabled=None,
+ visible=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|value|Bool if True checks the checkbox|
-|disabled|disable or enable state of the element|
-|visible|change visibility of element|
+|value|(bool) if True checks the checkbox, False clears it|
+|disabled|(bool) disable or enable element|
+|visible|(bool) control visibility of element|
-Get() - method
+#### Get
+
+Return the current state of this checkbox
+
+```
+Get() -> (bool) Current state of checkbox
+```
+
+#### SetTooltip
+
+Called by application to change the tooltip text for an Element. Normally invoked using the Element Object such as: window.Element('key').SetToolTip('New tip').
+
+```
+SetTooltip(tooltip_text)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|tooltip_text|(str) the text to show in tooltip.|
## Spin Element
An up/down spinner control. The valid values are passed in as a list.
-
+ A spinner with up/down buttons and a single line of text. Choose 1 values from list
```python
layout = [[sg.Spin([i for i in range(1,11)], initial_value=1), sg.Text('Volume level')]]
@@ -2384,41 +2933,41 @@ layout = [[sg.Spin([i for i in range(1,11)], initial_value=1), sg.Text('Volume
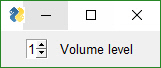
-```python
+```
Spin(values,
- initial_value=None,
- disabled=False,
- change_submits=False,
- enable_events=False,
- size=(None, None),
- auto_size_text=None,
- font=None,
- background_color=None,
- text_color=None,
- key=None,
- pad=None,
- tooltip=None,
- visible=True)
+ initial_value=None,
+ disabled=False,
+ change_submits=False,
+ enable_events=False,
+ size=(None, None),
+ auto_size_text=None,
+ font=None,
+ background_color=None,
+ text_color=None,
+ key=None,
+ pad=None,
+ tooltip=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|values|List of valid values|
-|initial_value|String with initial value|
-|disabled|set disable state for element (Default = False)|
-|change_submits|If True, pressing Enter key submits window (Default = False)|
-|enable_events|Turns on the element specific events.(Default = False)|
-|size|(w,h) w=characters-wide, h=rows-high|
-|auto_size_text|True if size should fit the text length|
-|font|specifies the font family, size, etc|
-|background_color|color of background|
-|text_color|color of the text|
-|key|Used with window.FindElement and with return values|
-|pad|Amount of padding to put around element|
-|tooltip|text, that will appear the you hover on|
-|visible|set visibility state of the element (Default = True)|
+|values|List[Any] List of valid values|
+|initial_value|[Any] Initial item to show in window. Choose from list of values supplied|
+|disabled|(bool) set disable state|
+|change_submits|(bool) DO NOT USE. Only listed for backwards compat - Use enable_events instead|
+|enable_events|(bool) Turns on the element specific events. Spin events happen when an item changes|
+|size|(int, int) (width, height) width = characters-wide, height = rows-high|
+|auto_size_text|(bool) if True will size the element to match the length of the text|
+|font|Union[str, tuple] specifies the font family, size, etc|
+|background_color|(str) color of background|
+|text_color|(str) color of the text|
+|key|(Any) Used with window.FindElement and with return values to uniquely identify this element|
+|pad|(int, int) or ((int, int),(int,int)) Amount of padding to put around element (left/right, top/bottom) or ((left, right), (top, bottom))|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
+|visible|(bool) set visibility state of the element|
### Qt Differences - `values` is a range!
@@ -2428,46 +2977,70 @@ On Qt values is a tuple representing a range. On plain PySimpleGUI this value i
### Methods
-```python
+#### Update
+
+Changes some of the settings for the Spin Element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
Update(value=None,
- values=None,
- disabled=None,
- visible=None)
+ values=None,
+ disabled=None,
+ visible=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|value|set the current value|
-|values|set available choices|
-|disabled|disable or enable state of the element|
-|visible|change visibility of element|
+|value|(Any) set the current value from list of choices|
+|values|List[Any] set available choices|
+|disabled|(bool) disable or enable state of the element|
+|visible|(bool) control visibility of element|
-Get() - method
+#### Get
+
+Return the current chosen value showing in spinbox.
+This value will be the same as what was provided as list of choices. If list items are ints, then the
+item returned will be an int (not a string)
+
+```
+Get() -> (Any) The currently visible entry
+```
+
+#### SetTooltip
+
+Called by application to change the tooltip text for an Element. Normally invoked using the Element Object such as: window.Element('key').SetToolTip('New tip').
+
+```
+SetTooltip(tooltip_text)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|tooltip_text|(str) the text to show in tooltip.|
## Image Element
Images can be placed in your window provide they are in PNG, GIF, PPM/PGM format. JPGs cannot be shown because tkinter does not naively support JPGs. You can use the Python Imaging Library (PIL) package to convert your image to PNG prior to calling PySimpleGUI if your images are in JPG format.
-
-
????????????? add image here
-```python
+```
Image(filename=None,
- data=None,
- background_color=None,
- size=(None, None),
- pad=None,
- key=None,
- tooltip=None,
- right_click_menu=None,
- visible=True,
- enable_events=False)
+ data=None,
+ background_color=None,
+ size=(None, None),
+ pad=None,
+ key=None,
+ tooltip=None,
+ right_click_menu=None,
+ visible=True,
+ enable_events=False)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -2476,29 +3049,31 @@ Parameters explained:
|background_color|color of background|
|size|(w,h) w=characters-wide, h=rows-high|
|pad|Amount of padding to put around element|
-|key|Used with window.FindElement and with return values|
-|tooltip|text, that will appear the you hover on|
-|right_click_menu|see "Right Click Menus"|
+|key|Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
+|right_click_menu|List[List[str]] see "Right Click Menus" for format|
|visible|set visibility state of the element (Default = True)|
|enable_events|Turns on the element specific events.(Default = False)|
### Methods
-```python
+Changes some of the settings for the Image Element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
Update(filename=None,
- data=None,
- size=(None, None),
- visible=None)
+ data=None,
+ size=(None, None),
+ visible=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
|filename||
|data||
|size|(w,h) w=characters-wide, h=rows-high|
-|visible|change visibility of element|
+|visible|(bool) control visibility of element|
Choose **either** a filename or in-ram data image to use to replace current image
@@ -2508,12 +3083,12 @@ You can specify an animated GIF as an image and can animate the GIF by calling `
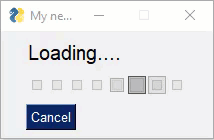
-```python
+```
UpdateAnimation(source,
- time_between_frames=0)
+ time_between_frames=0)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -2571,48 +3146,46 @@ In Oct 2018, the definition of Button changed. Previously Button would CLOSE th
The most basic Button element call to use is `Button`
-
-
```python
layout = [[sg.OK(), sg.Cancel()]]
```
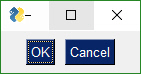
-```python
+```
Button(button_text="",
- button_type=7,
- target=(None, None),
- tooltip=None,
- file_types=(('ALL Files', '*.*'),),
- initial_folder=None,
- disabled=False,
- change_submits=False,
- enable_events=False,
- image_filename=None,
- image_data=None,
- image_size=(None, None),
- image_subsample=None,
- border_width=None,
- size=(None, None),
- auto_size_button=None,
- button_color=None,
- font=None,
- bind_return_key=False,
- focus=False,
- pad=None,
- key=None,
- visible=True)
+ button_type=7,
+ target=(None, None),
+ tooltip=None,
+ file_types=(('ALL Files', '*.*'),),
+ initial_folder=None,
+ disabled=False,
+ change_submits=False,
+ enable_events=False,
+ image_filename=None,
+ image_data=None,
+ image_size=(None, None),
+ image_subsample=None,
+ border_width=None,
+ size=(None, None),
+ auto_size_button=None,
+ button_color=None,
+ font=None,
+ bind_return_key=False,
+ focus=False,
+ pad=None,
+ key=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
|button_text|Text to be displayed on the button (Default value = '')|
|button_type|You should NOT be setting this directly (Default value = BUTTON_TYPE_READ_FORM)|
|target|key or (row,col) target for the button|
-|tooltip|text, that will appear the you hover on|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
|file_types|the filetypes that will be used to match files (Default value = (("ALL Files", "*.*"),))|
|initial_folder|starting path for folders and files|
|disabled|set disable state for element (Default = False)|
@@ -2630,7 +3203,7 @@ Parameters explained:
|bind_return_key|If True the return key will cause this button to fire (Default = False)|
|focus|if focus should be set to this|
|pad|Amount of padding to put around element|
-|key|Used with window.FindElement and with return values|
+|key|Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element|
|visible|set visibility state of the element (Default = True)|
### Shortcut, Pre-defined Buttons
@@ -2829,73 +3402,75 @@ If there are more than 1 button on a window, the FIRST button that is of type Cl
### Methods
-```python
+Changes some of the settings for the Button Element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
Update(text=None,
- button_color=(None, None),
- disabled=None,
- image_data=None,
- image_filename=None,
- visible=None,
- image_subsample=None,
- image_size=None)
+ button_color=(None, None),
+ disabled=None,
+ image_data=None,
+ image_filename=None,
+ visible=None,
+ image_subsample=None,
+ image_size=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
|text|sets button text|
|button_color|(text, background) (Default = (None))|
-|disabled|disable or enable state of the element|
+|disabled|(bool) disable or enable state of the element|
|image_data|in-RAM image to be displayed on button|
|image_filename|image filename if there is a button image|
-|visible|change visibility of element|
+|visible|(bool) control visibility of element|
|image_subsample|amount to reduce the size of the image|
|image_size||
-GetText() - Returns the current text shown on a button
+Returns the current text shown on a button
- :return: string value of button
+```
+GetText() -> string value of button
+```
## ButtonMenu Element
The ButtonMenu element produces a unique kind of effect. It's a button, that when clicked, shows you a menu. It's like clicking one of the top-level menu items on a MenuBar. As a result, the menu definition take the format of a single menu entry from a normal menu definition. A normal menu definition is a list of lists. This definition is one of those lists.
-
-
```python
['Menu', ['&Pause Graph', 'Menu item::optional_key']]
```
The very first string normally specifies what is shown on the menu bar. In this case, the value is **not used**. You set the text for the button using a different parameter, the `button_text` parm.
-```python
+```
ButtonMenu(button_text,
- menu_def,
- tooltip=None,
- disabled=False,
- image_filename=None,
- image_data=None,
- image_size=(None, None),
- image_subsample=None,
- border_width=None,
- size=(None, None),
- auto_size_button=None,
- button_color=None,
- font=None,
- pad=None,
- key=None,
- tearoff=False,
- visible=True)
+ menu_def,
+ tooltip=None,
+ disabled=False,
+ image_filename=None,
+ image_data=None,
+ image_size=(None, None),
+ image_subsample=None,
+ border_width=None,
+ size=(None, None),
+ auto_size_button=None,
+ button_color=None,
+ font=None,
+ pad=None,
+ key=None,
+ tearoff=False,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
|button_text|Text to be displayed on the button (Default value = '')|
|menu_def|??????????????????|
-|tooltip|text, that will appear the you hover on|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
|disabled|set disable state for element (Default = False)|
|image_filename|image filename if there is a button image|
|image_data|in-RAM image to be displayed on button|
@@ -2907,7 +3482,7 @@ Parameters explained:
|button_color|(text color, backound color)|
|font|specifies the font family, size, etc|
|pad|Amount of padding to put around element|
-|key|Used with window.FindElement and with return values|
+|key|Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element|
|tearoff|?????????????????? (Default = False)|
|visible|set visibility state of the element (Default = True)|
@@ -2922,19 +3497,17 @@ This element has limited usefulness and is being included more for completeness
It works best when placed between columns or elements that span multiple rows. If on a "normal" row with elements that are only 1 row high, then it will only span that one row.
-
-
```python
VerticalSeparator(pad=None)
```
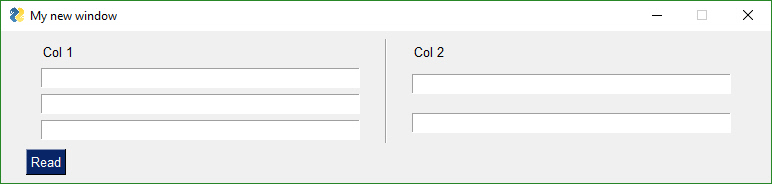
-```python
+```
VerticalSeparator(pad=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -2957,8 +3530,6 @@ The return value for `OneLineProgressMeter` is:
#### Progress Mater in Your window
Another way of using a Progress Meter with PySimpleGUI is to build a custom window with a `ProgressBar` Element in the window. You will need to run your window as a non-blocking window. When you are ready to update your progress bar, you call the `UpdateBar` method for the `ProgressBar` element itself.
-
-
```python
import PySimpleGUI as sg
@@ -2984,21 +3555,21 @@ window.Close()
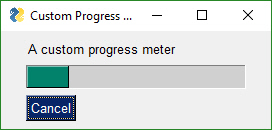
-```python
+```
ProgressBar(max_value,
- orientation=None,
- size=(None, None),
- auto_size_text=None,
- bar_color=(None, None),
- style=None,
- border_width=None,
- relief=None,
- key=None,
- pad=None,
- visible=True)
+ orientation=None,
+ size=(None, None),
+ auto_size_text=None,
+ bar_color=(None, None),
+ style=None,
+ border_width=None,
+ relief=None,
+ key=None,
+ pad=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3010,60 +3581,34 @@ Parameters explained:
|style|????????????????????????????|
|border_width|width of border around button|
|relief|????????????????????????????|
-|key|Used with window.FindElement and with return values|
+|key|Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element|
|pad|Amount of padding to put around element|
|visible|set visibility state of the element (Default = True)|
-### Methods
-
-```python
-Update(visible=None)
-```
-
-Parameters explained:
-
-|Name|Meaning|
-|---|---|
-|visible|change visibility of element|
-
-```python
-UpdateBar(current_count,
- max=None)
-```
-
-Parameters explained:
-
-|Name|Meaning|
-|---|---|
-|current_count|sets the current value|
-|max|changes the max value|
-
## Output Elements
The Output Element is a re-direction of Stdout. Anything "printed" will be displayed in this element.
Note that you will NOT see what you `print` until you call either window.Read or window.Refresh. If you want to immediately see what was printed, call window.Refresh() immediately after your print statement.
-
-
```python
Output(size=(None, None))
```
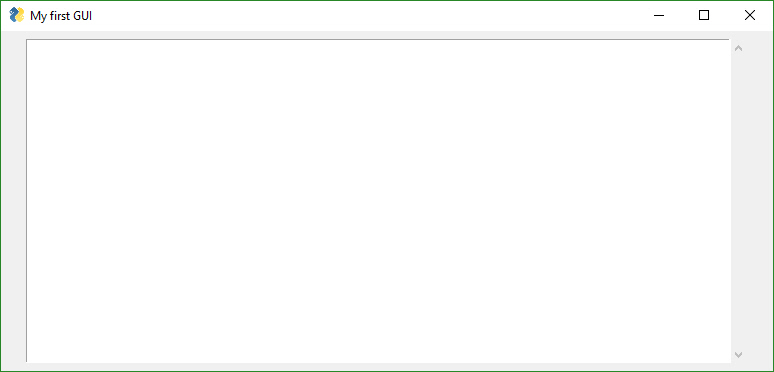
-```python
+```
Output(size=(None, None),
- background_color=None,
- text_color=None,
- pad=None,
- font=None,
- tooltip=None,
- key=None,
- right_click_menu=None,
- visible=True)
+ background_color=None,
+ text_color=None,
+ pad=None,
+ font=None,
+ tooltip=None,
+ key=None,
+ right_click_menu=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3072,24 +3617,26 @@ Parameters explained:
|text_color|color of the text|
|pad|Amount of padding to put around element|
|font|specifies the font family, size, etc|
-|tooltip|text, that will appear the you hover on|
-|key|Used with window.FindElement and with return values|
-|right_click_menu|see "Right Click Menus"|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
+|key|Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element|
+|right_click_menu|List[List[str]] see "Right Click Menus" for format|
|visible|set visibility state of the element (Default = True)|
### Methods
-```python
+Changes some of the settings for the Output Element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
Update(value=None,
- visible=None)
+ visible=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
|value||
-|visible|change visibility of element|
+|visible|(bool) control visibility of element|
----
@@ -3126,25 +3673,23 @@ Columns are specified in exactly the same way as a window is, as a list of lists
Columns are needed when you have an element that has a height > 1 line on the left, with single-line elements on the right. Here's an example of this kind of layout:
-
-
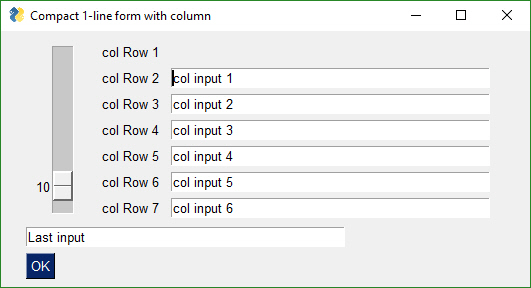
The Column Element has 1 required parameter and 1 optional (the layout and the background color). Setting the background color has the same effect as setting the window's background color, except it only affects the column rectangle.
-```python
+```
Column(layout,
- background_color=None,
- size=(None, None),
- pad=None,
- scrollable=False,
- vertical_scroll_only=False,
- right_click_menu=None,
- key=None,
- visible=True)
+ background_color=None,
+ size=(None, None),
+ pad=None,
+ scrollable=False,
+ vertical_scroll_only=False,
+ right_click_menu=None,
+ key=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3154,8 +3699,8 @@ Parameters explained:
|pad|Amount of padding to put around element|
|scrollable|???????????????????????? (Default = False)|
|vertical_scroll_only|???????????????????????? (Default = False)|
-|right_click_menu|see "Right Click Menus"|
-|key|Used with window.FindElement and with return values|
+|right_click_menu|List[List[str]] see "Right Click Menus" for format|
+|key|Used with window.FindElement and with return values to uniquely identify this element|
|visible|set visibility state of the element (Default = True)|
---
@@ -3198,32 +3743,30 @@ sg.Popup(event, values, line_width=200)
Frames work exactly the same way as Columns. You create layout that is then used to initialize the Frame.
-
-
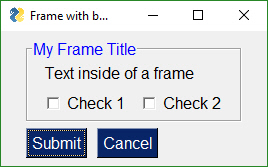
Notice how the Frame layout looks identical to a window layout. A window works exactly the same way as a Column and a Frame. They all are "container elements" - elements that contain other elements.
*These container Elements can be nested as deep as you want.* That's a pretty spiffy feature, right? Took a lot of work so be appreciative. Recursive code isn't trivial.
-```python
+```
Frame(title,
- layout,
- title_color=None,
- background_color=None,
- title_location=None,
- relief="groove",
- size=(None, None),
- font=None,
- pad=None,
- border_width=None,
- key=None,
- tooltip=None,
- right_click_menu=None,
- visible=True)
+ layout,
+ title_color=None,
+ background_color=None,
+ title_location=None,
+ relief="groove",
+ size=(None, None),
+ font=None,
+ pad=None,
+ border_width=None,
+ key=None,
+ tooltip=None,
+ right_click_menu=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3237,9 +3780,9 @@ Parameters explained:
|font|specifies the font family, size, etc|
|pad|Amount of padding to put around element|
|border_width|width of border around element|
-|key|Used with window.FindElement and with return values|
-|tooltip|text, that will appear the you hover on|
-|right_click_menu|see "Right Click Menus"|
+|key|Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
+|right_click_menu|List[List[str]] see "Right Click Menus" for format|
|visible|set visibility state of the element (Default = True)|
---
@@ -3263,20 +3806,18 @@ window = sg.Window('Frame with buttons', font=("Helvetica", 12)).Layout(layout)
In my opinion, the tkinter Canvas Widget is the most powerful of the tkinter widget. While I try my best to completely isolate the user from anything that is tkinter related, the Canvas Element is the one exception. It enables integration with a number of other packages, often with spectacular results.
-
-
-```python
+```
Canvas(canvas=None,
- background_color=None,
- size=(None, None),
- pad=None,
- key=None,
- tooltip=None,
- right_click_menu=None,
- visible=True)
+ background_color=None,
+ size=(None, None),
+ pad=None,
+ key=None,
+ tooltip=None,
+ right_click_menu=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3284,9 +3825,9 @@ Parameters explained:
|background_color|color of background|
|size|(w,h) w=characters-wide, h=rows-high|
|pad|Amount of padding to put around element|
-|key|Used with window.FindElement and with return values|
-|tooltip|text, that will appear the you hover on|
-|right_click_menu|see "Right Click Menus"|
+|key|Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
+|right_click_menu|List[List[str]] see "Right Click Menus" for format|
|visible|set visibility state of the element (Default = True)|
### Matplotlib, Pyplot Integration
@@ -3332,7 +3873,6 @@ See `Demo_Matplotlib.py` for a Recipe you can copy.
TKCanvas - not a method but a property. Returns the tkinter Canvas Widget
-
## Graph Element
All you math fans will enjoy this Element... and all you non-math fans will enjoy it too.
@@ -3360,25 +3900,23 @@ You can move your figures around on the canvas by supplying the Figure ID the x,
This Element is relatively new and may have some parameter additions or deletions. It shouldn't break your code however.
-
-
-```python
+```
Graph(canvas_size,
- graph_bottom_left,
- graph_top_right,
- background_color=None,
- pad=None,
- change_submits=False,
- drag_submits=False,
- enable_events=False,
- key=None,
- tooltip=None,
- right_click_menu=None,
- visible=True,
- float_values=False)
+ graph_bottom_left,
+ graph_top_right,
+ background_color=None,
+ pad=None,
+ change_submits=False,
+ drag_submits=False,
+ enable_events=False,
+ key=None,
+ tooltip=None,
+ right_click_menu=None,
+ visible=True,
+ float_values=False)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3390,9 +3928,9 @@ Parameters explained:
|change_submits|If True, pressing Enter key submits window (Default = False)|
|drag_submits|???????????????????????? (Default = False)|
|enable_events|Turns on the element specific events.(Default = False)|
-|key|Used with window.FindElement and with return values|
-|tooltip|text, that will appear the you hover on|
-|right_click_menu|see "Right Click Menus"|
+|key|Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
+|right_click_menu|List[List[str]] see "Right Click Menus" for format|
|visible|set visibility state of the element (Default = True)|
|float_values|bool: If True x,y coordinates are returned as floats, not ints|
@@ -3416,14 +3954,14 @@ Parameters explained:
All of the Drawing methods return a "***figure***" that can be used move and delete the figure
-```python
+```
DrawLine(point_from,
- point_to,
- color="black",
- width=1)
+ point_to,
+ color="black",
+ width=1)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3432,13 +3970,13 @@ Parameters explained:
|color|(Default value = 'black')|
|width|(Default value = 1)|
-```python
+```
DrawPoint(point,
- size=2,
- color="black")
+ size=2,
+ color="black")
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3446,14 +3984,14 @@ Parameters explained:
|size|(w,h) w=characters-wide, h=rows-high (Default value = 2)|
|color|(Default value = 'black')|
-```python
+```
DrawCircle(center_location,
- radius,
- fill_color=None,
- line_color="black")
+ radius,
+ fill_color=None,
+ line_color="black")
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3462,14 +4000,14 @@ Parameters explained:
|fill_color||
|line_color|(Default value = 'black')|
-```python
+```
DrawOval(top_left,
- bottom_right,
- fill_color=None,
- line_color=None)
+ bottom_right,
+ fill_color=None,
+ line_color=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3478,16 +4016,16 @@ Parameters explained:
|fill_color||
|line_color||
-```python
+```
DrawArc(top_left,
- bottom_right,
- extent,
- start_angle,
- style=None,
- arc_color="black")
+ bottom_right,
+ extent,
+ start_angle,
+ style=None,
+ arc_color="black")
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3498,14 +4036,14 @@ Parameters explained:
|style||
|arc_color|(Default value = 'black')|
-```python
+```
DrawRectangle(top_left,
- bottom_right,
- fill_color=None,
- line_color=None)
+ bottom_right,
+ fill_color=None,
+ line_color=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3514,15 +4052,15 @@ Parameters explained:
|fill_color||
|line_color||
-```python
+```
DrawText(text,
- location,
- color="black",
- font=None,
- angle=0)
+ location,
+ color="black",
+ font=None,
+ angle=0)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3532,49 +4070,53 @@ Parameters explained:
|font|specifies the font family, size, etc|
|angle|(Default value = 0)|
-Erase() - method
-
```python
+Erase()
+```
+
+```
DeleteFigure(id)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
|id||
-```python
+Changes some of the settings for the Graph Element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
Update(background_color,
- visible=None)
+ visible=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
|background_color|color of background|
-|visible|change visibility of element|
+|visible|(bool) control visibility of element|
-```python
+```
Move(x_direction,
- y_direction)
+ y_direction)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
|x_direction||
|y_direction||
-```python
+```
MoveFigure(figure,
- x_direction,
- y_direction)
+ x_direction,
+ y_direction)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3582,13 +4124,13 @@ Parameters explained:
|x_direction||
|y_direction||
-```python
+```
RelocateFigure(figure,
- x,
- y)
+ x,
+ y)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3596,46 +4138,42 @@ Parameters explained:
|x||
|y||
-
-
## Table Element
Out of all of the Elements, it's the Table and the Tree that are the most "problematic" in the tkinter inter and Qt implementations. They're hard is my only defense.
-
-
-```python
+```
Table(values,
- headings=None,
- visible_column_map=None,
- col_widths=None,
- def_col_width=10,
- auto_size_columns=True,
- max_col_width=20,
- select_mode=None,
- display_row_numbers=False,
- num_rows=None,
- row_height=None,
- font=None,
- justification="right",
- text_color=None,
- background_color=None,
- alternating_row_color=None,
- row_colors=None,
- vertical_scroll_only=True,
- hide_vertical_scroll=False,
- size=(None, None),
- change_submits=False,
- enable_events=False,
- bind_return_key=False,
- pad=None,
- key=None,
- tooltip=None,
- right_click_menu=None,
- visible=True)
+ headings=None,
+ visible_column_map=None,
+ col_widths=None,
+ def_col_width=10,
+ auto_size_columns=True,
+ max_col_width=20,
+ select_mode=None,
+ display_row_numbers=False,
+ num_rows=None,
+ row_height=None,
+ font=None,
+ justification="right",
+ text_color=None,
+ background_color=None,
+ alternating_row_color=None,
+ row_colors=None,
+ vertical_scroll_only=True,
+ hide_vertical_scroll=False,
+ size=(None, None),
+ change_submits=False,
+ enable_events=False,
+ bind_return_key=False,
+ pad=None,
+ key=None,
+ tooltip=None,
+ right_click_menu=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3663,9 +4201,9 @@ Parameters explained:
|enable_events|Turns on the element specific events.(Default = False)|
|bind_return_key|(Default = False)|
|pad|Amount of padding to put around element|
-|key|Used with window.FindElement and with return values|
-|tooltip|text, that will appear the you hover on|
-|right_click_menu|see "Right Click Menus"|
+|key|Used with window.FindElement and with return values to uniquely identify this element|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
+|right_click_menu|List[List[str]] see "Right Click Menus" for format|
|visible|set visibility state of the element (Default = True)|
### Read return values from Table Element
@@ -3676,20 +4214,22 @@ The values returned from a `Window.Read` call for the Tree Element are a list of
The Update method can be used to make changes to a table that's already been displayed. The call takes a single parameter, values, which is the new table to display. The entire table is replaced.
-```python
+Changes some of the settings for the Table Element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
Update(values=None,
- num_rows=None,
- visible=None,
- select_rows=None)
+ num_rows=None,
+ visible=None,
+ select_rows=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
|values||
|num_rows||
-|visible|change visibility of element|
+|visible|(bool) control visibility of element|
|select_rows||
---
@@ -3701,35 +4241,33 @@ If you click on the header, it can go into spasms for some tables. I don't under
The Tree Element and Table Element are close cousins. Many of the parameters found in the Table Element apply to Tree Elements. In particular the heading information, column widths, etc.
-
-
-```python
+```
Tree(data=None,
- headings=None,
- visible_column_map=None,
- col_widths=None,
- col0_width=10,
- def_col_width=10,
- auto_size_columns=True,
- max_col_width=20,
- select_mode=None,
- show_expanded=False,
- change_submits=False,
- enable_events=False,
- font=None,
- justification="right",
- text_color=None,
- background_color=None,
- num_rows=None,
- row_height=None,
- pad=None,
- key=None,
- tooltip=None,
- right_click_menu=None,
- visible=True)
+ headings=None,
+ visible_column_map=None,
+ col_widths=None,
+ col0_width=10,
+ def_col_width=10,
+ auto_size_columns=True,
+ max_col_width=20,
+ select_mode=None,
+ show_expanded=False,
+ change_submits=False,
+ enable_events=False,
+ font=None,
+ justification="right",
+ text_color=None,
+ background_color=None,
+ num_rows=None,
+ row_height=None,
+ pad=None,
+ key=None,
+ tooltip=None,
+ right_click_menu=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3752,9 +4290,9 @@ Parameters explained:
|num_rows||
|row_height||
|pad|Amount of padding to put around element|
-|key|Used with window.FindElement and with return values|
-|tooltip|text, that will appear the you hover on|
-|right_click_menu|see "Right Click Menus"|
+|key|Used with window.FindElement and with return values to uniquely identify this element|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
+|right_click_menu|List[List[str]] see "Right Click Menus" for format|
|visible|set visibility state of the element (Default = True)|
Unlike Tables there is no standard format for trees. Thus the data structure passed to the Tree Element must be constructed. This is done using the TreeData class. The process is as follows:
@@ -3819,10 +4357,6 @@ View of second tab:
First we have the Tab layout definitions. They mirror what you see in the screen shots. Tab 1 has 1 Text Element in it. Tab 2 has a Text and an Input Element.
-
-
-
-
```python
tab1_layout = [[sg.T('This is inside tab 1')]]
@@ -3853,24 +4387,24 @@ Check out Tabs 7 and 8. We've got a Window with a Column containing Tabs 5 and
As of Release 3.8.0, not all of *options* shown in the API definitions of the Tab and TabGroup Elements are working. They are there as placeholders.
-```python
+```
TabGroup(layout,
- tab_location=None,
- title_color=None,
- selected_title_color=None,
- background_color=None,
- font=None,
- change_submits=False,
- enable_events=False,
- pad=None,
- border_width=None,
- theme=None,
- key=None,
- tooltip=None,
- visible=True)
+ tab_location=None,
+ title_color=None,
+ selected_title_color=None,
+ background_color=None,
+ font=None,
+ change_submits=False,
+ enable_events=False,
+ pad=None,
+ border_width=None,
+ theme=None,
+ key=None,
+ tooltip=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3885,26 +4419,26 @@ Parameters explained:
|pad|Amount of padding to put around element|
|border_width|width of border around element|
|theme||
-|key|Used with window.FindElement and with return values|
-|tooltip|text, that will appear the you hover on|
+|key|Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
|visible|set visibility state of the element (Default = True)|
-```python
+```
Tab(title,
- layout,
- title_color=None,
- background_color=None,
- font=None,
- pad=None,
- disabled=False,
- border_width=None,
- key=None,
- tooltip=None,
- right_click_menu=None,
- visible=True)
+ layout,
+ title_color=None,
+ background_color=None,
+ font=None,
+ pad=None,
+ disabled=False,
+ border_width=None,
+ key=None,
+ tooltip=None,
+ right_click_menu=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3916,9 +4450,9 @@ Parameters explained:
|pad|Amount of padding to put around element|
|disabled|set disable state for element (Default = False)|
|border_width|width of border around element|
-|key|Used with window.FindElement and with return values|
-|tooltip|text, that will appear the you hover on|
-|right_click_menu|see "Right Click Menus"|
+|key|Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
+|right_click_menu|List[List[str]] see "Right Click Menus" for format|
|visible|set visibility state of the element (Default = True)|
### Reading Tab Groups
@@ -3927,49 +4461,49 @@ Tab Groups now return a value when a Read returns. They return which tab is cur
### Methods
-```python
+Changes some of the settings for the Tree Element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
Update(values=None,
- key=None,
- value=None,
- text=None,
- icon=None,
- visible=None)
+ key=None,
+ value=None,
+ text=None,
+ icon=None,
+ visible=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
|values||
-|key|Used with window.FindElement and with return values|
+|key|Used with window.FindElement and with return values to uniquely identify this element|
|value||
|text||
|icon||
-|visible|change visibility of element|
+|visible|(bool) control visibility of element|
## Pane Element
New in version 3.20 is the Pane Element, a super-cool tkinter feature. You won't find this one in PySimpleGUIQt, only PySimpleGUI. It's difficult to describe one of these things. Think of them as "Tabs without labels" that you can slide.
-
-
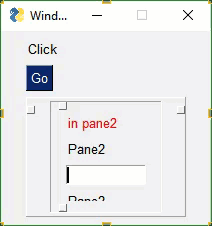
-```python
+```
Pane(pane_list,
- background_color=None,
- size=(None, None),
- pad=None,
- orientation="vertical",
- show_handle=True,
- relief="raised",
- handle_size=None,
- border_width=None,
- key=None,
- visible=True)
+ background_color=None,
+ size=(None, None),
+ pad=None,
+ orientation="vertical",
+ show_handle=True,
+ relief="raised",
+ handle_size=None,
+ border_width=None,
+ key=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3982,7 +4516,7 @@ Parameters explained:
|relief|relief style. Values are same as progress meter relief values. Can be a constant or a string: `RELIEF_RAISED RELIEF_SUNKEN RELIEF_FLAT RELIEF_RIDGE RELIEF_GROOVE RELIEF_SOLID`|
|handle_size||
|border_width|width of border around element|
-|key|Used with window.FindElement and with return values|
+|key|Used with window.FindElement and with return values to uniquely identify this element|
|visible|set visibility state of the element (Default = True)|
***Each "Pane" of a Pane Element must be a Column Element***. The parameter `pane_list` is a list of Column Elements.
@@ -4631,8 +5165,6 @@ Use realtime keyboard capture by calling
Beginning in version 3.01 you can add a MenuBar to your window. You specify the menus in much the same way as you do window layouts, with lists. Menu selections are returned as events and as of 3.17, also as in the values dictionary. The value returned will be the entire menu entry, including the key if you specified one.
-
-
```python
menu_def = [['File', ['Open', 'Save', 'Exit',]],
['Edit', ['Paste', ['Special', 'Normal',], 'Undo'],],
@@ -4645,17 +5177,19 @@ Note the placement of ',' and of []. It's tricky to get the nested menus correc
## Methods
-```python
-Update(menu_definition,
- visible=None)
+Update a menubar - can change the menu definition and visibility
+
+```
+Update(menu_definition=None,
+ visible=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|menu_definition||
-|visible|change visibility of element|
+|menu_definition|List[[str]] New menu defintion|
+|visible|(bool) control visibility of element|
---
@@ -5667,4 +6201,4 @@ GNU Lesser General Public License (LGPL 3) +
## Acknowledgments
-#### SORRY!! Will add these back. Lost due to file length limitation
+#### SORRY!! Will add these back. Lost due to file length limitation
\ No newline at end of file
diff --git a/readme.md b/readme.md
index 21446bdf..16e84cc3 100644
--- a/readme.md
+++ b/readme.md
@@ -1,5 +1,3 @@
-
-
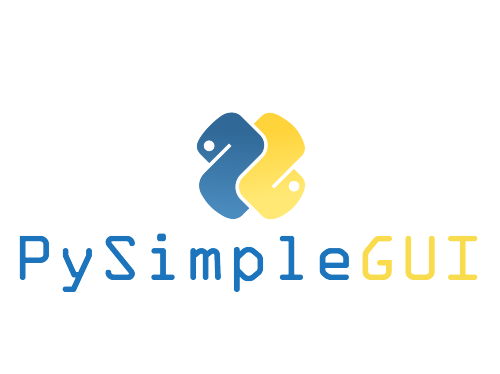
[](http://pepy.tech/project/pysimplegui) tkinter
@@ -518,6 +516,14 @@ Dictionaries are used by more advanced PySimpleGUI users. You'll know that dict
"High level calls" are those that start with "Popup". They are the most basic form of communications with the user. They are named after the type of window they create, a pop-up window. These windows are meant to be short lived while, either delivering information or collecting it, and then quickly disappearing.
+Think of Popups as your first windows, sorta like your first bicycle. It worked well, but was limited. It probably wasn't long before you wanted more features and it seemed too limiting for your newly found sense of adventure.
+
+When you've reached the point with Popups that you are thinking of filing a GitHub "Enhancement Issue" to get the Popup call extended to include a new feature that you think would be helpful.... not just to you but others is what you had in mind, right? For the good of others.
+
+It's at THIS time that you should immediately turn to the section entitled "Custom Window API Calls - Your First Window". Congratulations, you just graduated and are not an official "GUI Designer". Oh, nevermind that you only started learning Python 2 weeks ago, you're a real GUI Designer now so buck up and start acting like one.
+
+But, for now, let's stick with these 1-line window calls, the Popups.
+
## Popup Output
Think of the `Popup` call as the GUI equivalent of a `print` statement. It's your way of displaying results to a user in the windowed world. Each call to Popup will create a new Popup window.
@@ -572,24 +578,54 @@ Preview of popups:
+Popup - Display a popup Window with as many parms as you wish to include. This is the GUI equivalent of the
+"print" statement. It's also great for "pausing" your program's flow until the user can read some error messages
+
```
-Popup(*args, Variable number of arguments you want to display
- button_color=None, Color of buttons (text_color, background_color)
- background_color=None, Color of background
- text_color=None, Color of text
- button_type=POPUP_BUTTONS_OK, Type of button layout
- auto_close=False, If True window will automatically close
- auto_close_duration=None, Number of seconds for autoclose
- non_blocking=False, If True returns immediately
- icon=DEFAULT_WINDOW_ICON, Icon to use on the taskbar
- line_width=None, Width of lines in characters
- font=None, Font to use for characters
- no_titlebar=False, If True no titlebar will be shown
- grab_anywhere=False, If True can move window by grabbing anywhere
- keep_on_top=False, If True window will be on top of other windows
- location=(None,None)): (x,y) coordinates to show the window
+Popup(args,
+ title=None,
+ button_color=None,
+ background_color=None,
+ text_color=None,
+ button_type=0,
+ auto_close=False,
+ auto_close_duration=None,
+ custom_text=(None, None),
+ non_blocking=False,
+ icon=None,
+ line_width=None,
+ font=None,
+ no_titlebar=False,
+ grab_anywhere=False,
+ keep_on_top=False,
+ location=(None, None))
```
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|*args|Variable number of your arguments. Load up the call with stuff to see!|
+|title|Optional title for the window|
+|button_color|Tuple(str, str) Color of the buttons shown (text color, button color)|
+|background_color|(str) Window background color|
+|text_color|(str) text color|
+|button_type|(enum) determines which pre-defined buttons will be shown (Default value = POPUP_BUTTONS_OK). The user normally will NOT change this value. There are many Popup functions and they call Popup, changing this parameter to get the desired effect.|
+|auto_close|(bool) If True the window will automatically close|
+|auto_close_duration|(int) time in seconds to keep window open before closing it automatically|
+|custom_text|Union[tuple(str, str), str] A string or pair of strings that contain the text to display on the buttons|
+|non_blocking|(bool) If True then will immediately return from the function without waiting for the uder's input.|
+|icon|Union[str, bytes] icon to display on the window. Same format was Window call|
+|line_width|(int) Width of lines in characters to use. Defaults to MESSAGE_BOX_LINE_WIDTH|
+|font|Union[str, tuple(font, size, modifiors) specifies the font family, size, etc|
+|no_titlebar|(bool) If True will not show the frame around the window and the titlebar across the top|
+|grab_anywhere|(bool) If True can grab anywhere to move the window. If no_titlebar is True, grab_anywhere should likely be enabled too|
+|location|(int, int) Location on screen to display the top left corner of window. Defaults to centered on screen|
+|||
+| **return** | Union[str, None] Returns text of the button that was pressed. None will be returned if user closed window with X |
+
+The other output Popups are variations on parameters. Usually the button_type parameter is the primary one changed.
+
The other output Popups are variations on parameters. Usually the button_type parameter is the primary one changed.
The choices for button_type are:
@@ -601,7 +637,8 @@ POPUP_BUTTONS_OK_CANCEL
POPUP_BUTTONS_OK
POPUP_BUTTONS_NO_BUTTONS
```
-Note that you should not call Popup yourself with different button_types. Rely on the Popup function named that sets that value for you. For example PopupYesNo will set the button type to POPUP_BUTTONS_YES_NO for you.
+
+**Note that you should not call Popup yourself with different button_types.** Rely on the Popup function named that sets that value for you. For example PopupYesNo will set the button type to POPUP_BUTTONS_YES_NO for you.
#### Scrolled Output
There is a scrolled version of Popups should you have a lot of information to display.
@@ -642,22 +679,45 @@ Use these Popups instead of making a custom window to get one data value, call
### PopupGetText
Use this Popup to get a line of text from the user.
+Display Popup with text entry field
+
```
-PopupGetText(message,The message you wish to display with the input field
- default_text='', Text to initially fill into the input field
- password_char='', Passwork character if this is a password field
- size=(None,None), Size of the window
- button_color=None, Color to use for buttons (foreground, background)
- background_color=None, Background color for window
- text_color=None, Text color for window
- icon=DEFAULT_WINDOW_ICON, Icon to display on taskbar
- font=None, Font to use for text
- no_titlebar=False, If True no titlebar will be shown
- grab_anywhere=False, If True can grab anywhere to move the window
- keep_on_top=False, If True window will stay on top of other windows
- location=(None,None)) Location on screen to display window
+PopupGetText(message,
+ title=None,
+ default_text="",
+ password_char="",
+ size=(None, None),
+ button_color=None,
+ background_color=None,
+ text_color=None,
+ icon=None,
+ font=None,
+ no_titlebar=False,
+ grab_anywhere=False,
+ keep_on_top=False,
+ location=(None, None))
```
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|message||
+|title||
+|default_text|(Default value = '')|
+|password_char|Passwork character if this is a password field (Default value = '')|
+|size|(w,h) w=characters-wide, h=rows-high|
+|button_color|button color (foreground, background)|
+|background_color|color of background|
+|text_color|color of the text|
+|icon|Icon to display|
+|font|specifies the font family, size, etc|
+|no_titlebar|(Default = False)|
+|grab_anywhere|If True can grab anywhere to move the window (Default = False)|
+|location|(Default = (None))|
+|||
+| **return** | Union[str, None] Text entered or None if window was closed |
+
```python
import PySimpleGUI as sg
text = sg.PopupGetText('Title', 'Please input something')
@@ -671,25 +731,55 @@ sg.Popup('Results', 'The value returned from PopupGetText', text)
### PopupGetFile
Gets a filename from the user. There are options to configure the type of dialog box to show. Normally an "Open File" dialog box is shown.
+Display popup with text entry field and browse button. Browse for file
+
```
-PopupGetFile(message, Message to show in the window
- default_path='', Path browsing should start from
- default_extension='', Which filetype is the default
- save_as=False, Determines which dialog box stype to show
- file_types=(("ALL Files", "*.*"),), Which filetypes are displayed
- no_window=False, if True no window is displayed except the dialog box
- size=(None,None), Size of window
- button_color=None, Color of buttons
- background_color=None, Color of window background
- text_color=None, Color of text in window
- icon=DEFAULT_WINDOW_ICON, Icon to show on taskbar
- font=None, Font to use
- no_titlebar=False, If True does not display a titlebar
- grab_anywhere=False, if True can grab window anywhere to move it
- keep_on_top=False, if True window will be on top of others
- location=(None,None)) Location on screen to show window
+PopupGetFile(message,
+ title=None,
+ default_path="",
+ default_extension="",
+ save_as=False,
+ multiple_files=False,
+ file_types=(('ALL Files', '*.*'),),
+ no_window=False,
+ size=(None, None),
+ button_color=None,
+ background_color=None,
+ text_color=None,
+ icon=None,
+ font=None,
+ no_titlebar=False,
+ grab_anywhere=False,
+ keep_on_top=False,
+ location=(None, None),
+ initial_folder=None)
```
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|message||
+|title||
+|default_path|(Default value = '')|
+|default_extension|(Default value = '')|
+|save_as|(Default = False)|
+|multiple_files|(Default = False)|
+|file_types|(Default value = (("ALL Files", "*.*")))|
+|no_window|(Default = False)|
+|size|(w,h) w=characters-wide, h=rows-high|
+|button_color|button color (foreground, background)|
+|background_color|color of background|
+|text_color|color of the text|
+|icon|Icon to display|
+|font|specifies the font family, size, etc|
+|no_titlebar|(Default = False)|
+|grab_anywhere|If True can grab anywhere to move the window (Default = False)|
+|location|(Default = (None))|
+|initial_folder||
+|||
+| **return** | Union[str, None] string representing the path chosen, None if cancelled or window closed with X |
+
If configured as an Open File Popup then (save_as is not True) the dialog box will look like this.
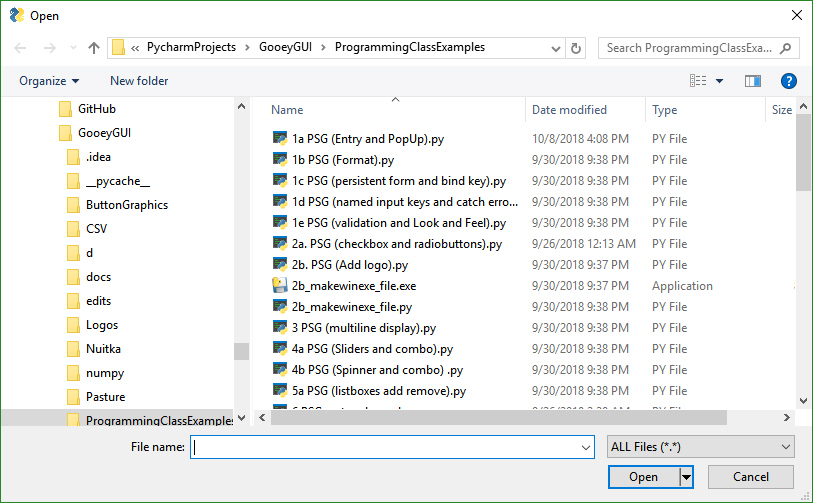
@@ -713,22 +803,47 @@ sg.Popup('Results', 'The value returned from PopupGetFile', text)
The window created to get a folder name looks the same as the get a file name. The difference is in what the browse button does. `PopupGetFile` shows an Open File dialog box while `PopupGetFolder` shows an Open Folder dialog box.
+Display popup with text entry field and browse button. Browse for folder
+
```
-PopupGetFolder(message, Message to display in window
- default_path='', Path to start browsing
- no_window=False, If True no window will be shown
- size=(None,None), Size of window
- button_color=None, Color of buttons
- background_color=None, Background color of window
- text_color=None, Color of window text
- icon=DEFAULT_WINDOW_ICON, Icon to show on taskbar
- font=None, Font to use for window
- no_titlebar=False, If True no titlebar will be shown
- grab_anywhere=False, If True can grab anywhere on window to move
- keep_on_top=False, If True window will be on top
- location=(None, None)) Location on screen to create window
+PopupGetFolder(message,
+ title=None,
+ default_path="",
+ no_window=False,
+ size=(None, None),
+ button_color=None,
+ background_color=None,
+ text_color=None,
+ icon=None,
+ font=None,
+ no_titlebar=False,
+ grab_anywhere=False,
+ keep_on_top=False,
+ location=(None, None),
+ initial_folder=None)
```
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|message||
+|title||
+|default_path|(Default value = '')|
+|no_window|(Default = False)|
+|size|(w,h) w=characters-wide, h=rows-high|
+|button_color|button color (foreground, background)|
+|background_color|color of background|
+|text_color|color of the text|
+|icon|Icon to display|
+|font|specifies the font family, size, etc|
+|no_titlebar|(Default = False)|
+|grab_anywhere|If True can grab anywhere to move the window (Default = False)|
+|location|(Default = (None))|
+|initial_folder||
+|||
+| **return** | Union[str, None] Contents of text field. None if closed using X or cancelled |
+
This is a typpical call
```python
@@ -744,34 +859,38 @@ This is a typpical call
The animated Popup enables you to easily display a "loading" style animation specified through a GIF file that is either stored in a file or a base64 variable.
-```python
+"Plays" an animated GIF file. This function has its own internal clocking meaning you can call it at any frequency
+ and the rate the frames of video is shown remains constant. Maybe your frames update every 30 ms but your
+ event loop is running every 10 ms.
+
+```
PopupAnimated(image_source,
- message=None,
- background_color=None,
- text_color=None,
- font=None,
- no_titlebar=True,
- grab_anywhere=True,
- keep_on_top=True,
- location=(None, None),
- alpha_channel=None,
- time_between_frames=0,
- transparent_color=None)
+ message=None,
+ background_color=None,
+ text_color=None,
+ font=None,
+ no_titlebar=True,
+ grab_anywhere=True,
+ keep_on_top=True,
+ location=(None, None),
+ alpha_channel=None,
+ time_between_frames=0,
+ transparent_color=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|image_source||
-|message||
-|background_color|color of background|
-|text_color|color of the text|
-|font|specifies the font family, size, etc|
-|no_titlebar|(Default = True)|
-|grab_anywhere|(Default = True)|
-|keep_on_top|(Default = True)|
-|location||
+|image_source|Union[str, bytes] Either a filename or a base64 string.|
+|message|An optional message to be shown with the animation|
+|background_color|(str) color of background|
+|text_color|(str) color of the text|
+|font|Union[str, tuple) specifies the font family, size, etc|
+|no_titlebar|(bool) If True then the titlebar and window frame will not be shown|
+|grab_anywhere|(bool) If True then you can move the window just clicking anywhere on window, hold and drag|
+|keep_on_top|(bool) If True then Window will remain on top of all other windows currently shownn|
+|location|(int, int) (x,y) location on the screen to place the top left corner of your window. Default is to center on screen|
|alpha_channel||
|time_between_frames|(Default value = 0)|
|transparent_color||
@@ -1411,70 +1530,335 @@ You can learn more about these async / non-blocking windows toward the end of th
# Window Object - Beginning a window
The first step is to create the window object using the desired window customization.
-This is the definition of the Window object:
+ Represents a single Window
-```python
-Window( title,
- default_element_size=DEFAULT_ELEMENT_SIZE,
- default_button_element_size=(None,None),
- auto_size_text=None,
- auto_size_buttons=None,
- location=(None,None),
- size=(None,None),
- element_padding=None,
- button_color=None,
- font=None,
- progress_bar_color=(None,None),
- background_color=None,
- border_depth=None,
- auto_close=False,
- auto_close_duration=DEFAULT_AUTOCLOSE_TIME,
- icon=DEFAULT_WINDOW_ICON,
- force_toplevel=False,
- alpha_channel=1,
- return_keyboard_events=False,
- use_default_focus=True,
- text_justification=None,
- no_titlebar=False,
- grab_anywhere=False,
- keep_on_top=False,
- resizable=False,
- disable_close=False,
- disable_minimize=False,
- right_click_menu=None):
+```
+Window(title,
+ layout=None,
+ default_element_size=(45, 1),
+ default_button_element_size=(None, None),
+ auto_size_text=None,
+ auto_size_buttons=None,
+ location=(None, None),
+ size=(None, None),
+ element_padding=None,
+ margins=(None, None),
+ button_color=None,
+ font=None,
+ progress_bar_color=(None, None),
+ background_color=None,
+ border_depth=None,
+ auto_close=False,
+ auto_close_duration=3,
+ icon=None,
+ force_toplevel=False,
+ alpha_channel=1,
+ return_keyboard_events=False,
+ use_default_focus=True,
+ text_justification=None,
+ no_titlebar=False,
+ grab_anywhere=False,
+ keep_on_top=False,
+ resizable=False,
+ disable_close=False,
+ disable_minimize=False,
+ right_click_menu=None,
+ transparent_color=None,
+ debugger_enabled=True)
```
-Parameter Descriptions. You will find these same parameters specified for each `Element` and some of them in `Row` specifications. The `Element` specified value will take precedence over the `Row` and `window` values.
+Parameter Descriptions:
|Name|Meaning|
-| ----- | ---- |
-| default_element_size | Size of elements in window in characters (width, height) |
-| default_button_element_size | Size of buttons on this window |
-| auto_size_text | Bool. True if elements should size themselves according to contents. Defaults to True |
-| auto_size_buttons | Bool. True if button elements should size themselves according to their text label |
-| location | (x,y) Location to place window in pixels |
-| size | (w,h) forces a window to be a paricular size|
-| element_padding | (w,h) default padding amount for elements|
-| font | Font name and size for elements of the window |
-| button_color | Default color for buttons (foreground, background). Can be text or hex |
-| progress_bar_color | Foreground and background colors for progress bars |
-| background_color | Color of the window background |
-| border_depth | Amount of 'bezel' to put on input boxes, buttons, etc. |
-| auto_close | Bool. If True window will autoclose |
-| auto_close_duration | Duration in seconds before window closes |
-| icon | .ICO file that will appear on the Task Bar and end of Title Bar |
-| force_top_level | Bool. If set causes a tk.Tk window to be used as primary window rather than tk.TopLevel. Used to get around Matplotlib problem |
-| alpha_channel | Float 0 to 1. 0 is invisible, 1 is fully visible, Anything between will be semi-transparent |
-| return_keyboard_events | if True key presses are returned as buttons |
-| use_default_focus | if True and no focus set, then automatically set a focus |
-| text_justification | Justification to use for Text Elements in this window |
-| no_titlebar | Create window without a titlebar |
-| grab_anywhere | Grab any location on the window to move the window |
-| keep_on_top | if True then window will always stop on top of other windows on the screen. Great for floating toolbars. |
-| resizable | if True | user can manually changge the wize of the window. Defaults to False|
-| disable_close | if True user will not be able to close using the X. |
-| disable_minimize | if True user will not be able to minimize the window|
-| right_click_menu | menu definition that will be used on wall elements that support right click. If a definition is specified on an element then it will be used instead.|
+|---|---|
+|title||
+|layout||
+|default_element_size|(Default value = DEFAULT_ELEMENT_SIZE)|
+|default_button_element_size||
+|auto_size_text|True if size should fit the text length|
+|auto_size_buttons||
+|location|Location on screen to display|
+|size|(w,h) w=characters-wide, h=rows-high (Default = (None))|
+|element_padding||
+|margins|(Default = (None))|
+|button_color|button color (foreground, background)|
+|font|specifies the font family, size, etc|
+|progress_bar_color|(Default = (None))|
+|background_color|color of background|
+|border_depth||
+|auto_close|(Default = False)|
+|auto_close_duration|(Default value = DEFAULT_AUTOCLOSE_TIME)|
+|icon|Icon to display. Filled in with default icon in init (Default value = None)|
+|force_toplevel|(Default = False)|
+|alpha_channel|(Default value = 1)|
+|return_keyboard_events|(Default = False)|
+|use_default_focus|(Default = True)|
+|text_justification||
+|no_titlebar|(Default = False)|
+|grab_anywhere|If True can grab anywhere to move the window (Default = False)|
+|resizable|(Default = False)|
+|disable_close|(Default = False)|
+|disable_minimize|(Default = False)|
+|right_click_menu|List[List[str]] see "Right Click Menus" for format|
+|transparent_color||
+|debugger_enabled|(Default = True)|
+
+## Methods
+
+### AddRow
+
+Parms are a variable number of Elements
+
+```
+AddRow(args)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|*args||
+
+### AddRows
+
+```
+AddRows(rows)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|rows||
+
+### BringToFront
+
+```python
+BringToFront()
+```
+
+### Close
+
+```python
+Close()
+```
+
+```python
+CurrentLocation()
+```
+
+```python
+Disable()
+```
+
+```python
+DisableDebugger()
+```
+
+```python
+Disappear()
+```
+
+Find element object associated with the provided key
+:returns Found element object, an Error Element, or None
+
+```
+Element(key,
+ silent_on_error=False)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|key|Used with window.FindElement and with return values to uniquely identify this element|
+|silent_on_error|(Default = False)|
+
+```python
+Enable()
+```
+
+```python
+EnableDebugger()
+```
+
+```
+Fill(values_dict)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|values_dict|?????????????????|
+
+```python
+Finalize()
+```
+
+Find element object associated with the provided key
+:returns Found element object, an Error Element, or None
+
+```
+Find(key,
+ silent_on_error=False)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|key|Used with window.FindElement and with return values to uniquely identify this element|
+|silent_on_error|(Default = False)|
+
+Find element object associated with the provided key
+:returns Found element object, an Error Element, or None
+
+```
+FindElement(key,
+ silent_on_error=False)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|key|Used with window.FindElement and with return values to uniquely identify this element|
+|silent_on_error|(Default = False)|
+
+```python
+FindElementWithFocus()
+```
+
+```python
+GetScreenDimensions()
+```
+
+```python
+GrabAnyWhereOff()
+```
+
+```python
+GrabAnyWhereOn()
+```
+
+```python
+Hide()
+```
+
+```
+Layout(rows)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|rows||
+
+```
+LoadFromDisk(filename)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|filename|?????????????????|
+
+```python
+Maximize()
+```
+
+```python
+Minimize()
+```
+
+```
+Move(x,
+ y)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|x|x coordinate|
+|y|y coordinate|
+
+```python
+Normal()
+```
+
+```
+Read(timeout=None,
+ timeout_key="__TIMEOUT__")
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|timeout|timeout to wait, till Read will execute itself|
+|timeout_key|(Default value = TIMEOUT_KEY)|
+
+```python
+Reappear()
+```
+
+```python
+Refresh()
+```
+
+```
+SaveToDisk(filename)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|filename|?????????????????|
+
+```
+SetAlpha(alpha)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|alpha||
+
+```
+SetIcon(icon=None,
+ pngbase64=None)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|icon||
+|pngbase64||
+
+```
+SetTransparentColor(color)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|color||
+
+```python
+UnHide()
+```
+
+```python
+VisibilityChanged()
+```
### Window Location
PySimpleGUI computes the exact center of your window and centers the window on the screen. If you want to locate your window elsewhere, such as the system default of (0,0), if you have 2 ways of doing this. The first is when the window is created. Use the `location` parameter to set where the window. The second way of doing this is to use the `SetOptions` call which will set the default window location for all windows in the future.
@@ -1528,33 +1912,6 @@ To keep a window on top of all other windows on the screen, set keep_on_top = Tr
PySimpleGUI will set a default focus location for you. This generally means the first input field. You can set the focus to a particular element. If you are going to set the focus yourself, then you should turn off the automatic focus by setting `use_default_focus=False` in your Window call.
-### Window Methods (things you can do with a Window object)
-
-There are a few methods (functions) that you will see in this document that act on Windows. The ones you will primarily be calling are:
-
- window.Layout(layout) - Recommend moving away from this nethod and using layout parameter instead. Turns your definition of the Window into Window
- window.Finalize() - creates the tkinter objects for the Window. Normally you do not call this
- window.Read() - Read the Windows values and get the button / key that caused the Read to return. Can have an optional timeout
- window.ReadNonBlocking() - NO LONGER USED!
- window.Refresh() - Use if updating elements and want to show the updates prior to the nex Read
- window.Fill(values_dict) - Fill each Element with entry from the dictionary passed in
- window.SaveToDisk(filename) - Save the Window's values to disk
- window.LoadFromDisk(filename) - Load the Window's values from disk
- window.Close() - To close your window, if a button hasn't already closed it
- window.Disable() - Use to disable the window inputwhen opening another window on top of the primnary Window
- window.Enable() - Re-enable a Disabled window
- window.FindElement(key, silent_on_error=False) - Returns the element that has a matching key value
- window.Element(key, silent_on_error=False) - EXACTLY the same as calling FindElement
- window.Move(x,y) - Moves window to location x,y on screen'
- window.SetAlpha(alpha) - Changes window transparency
- window.BringToFront() - Brings the window to the top of other windows on the screen
- window.Disappear(), Reappear() - Uses alpha channel to make window disappear
- window.Hide(), UnHide() - Hides a window
- window.CurrentLocation() - Returns current window location
- window.Size = w,h - Forces a window to be a particular size. Note this is a property not a method
- window.Size - Tuple (w,h)The size of the current window. Note this is a property
- window.Minimize() - Minimizes window to taskbar
-
## Window Methods
There are a number of operations you can do on a window after you've created the window. You call these after creating your Windows object.
@@ -1585,33 +1942,7 @@ If you set the timeout = 0, then the Read will immediately return rather than wa
While this call will technically still work, it is being removed. If you want to get the same result, call Read with timeout = 0.
-Read the Window's input values and button clicks but without blocking. It will immediately return. **Consider using Read with non-zero timeout instead!**
-
-Will consume 100% of your CPU if you do not have other blocking calls in your event loop.
-
-|Name|Meaning|
-|-----|-----|
-| `Refresh() ` | Cause changes to the window to be displayed on the screen. Normally not needed unless the changes are immediately required or if it's going to be a while before another call to Read. |
-| `SetIcon(icon, pngbase64) ` | Sets the window's icon that will be shown on the titlebar. Can either be a filename or a base64 string.|
-| `Fill(values_dict) ` | Populates the windows fields with the values shown in the dictionary.|
-| `FindElementWithFocus() ` | Returns the Element that currently has the focus. Returns None if no Elements were found.|
-| `SaveToDisk(filename) ` | Saves the window's values to disk |
-| `LoadFromDisk(filename) ` | Fills in a window's fields based on previously saved file |
-| `GetScreenDimensions() ` | Returns the size (w,h) of the screen in pixels |
-| `CurrentLocation() ` | Returns current screen position (x,y) |
-| `Move(x, y) ` | Move window to (x,y) position on the screen |
-| `Minimize() ` | Sends the window to the taskbar |
-| `Close() ` | Closes a window, blocking or non-blocking|
-| `Disable() ` | Stops a window from responding until Enable is called|
-| `Enable() ` | Re-enables a previously disabled window|
-| `Hide() ` | Completely hides a window, including removing from the taskbar|
-| `UnHide() ` | Restores a window hidden using Hide|
-| `Disappear() ` | Makes a window disappear while leaving the icon on the taskbar|
-| `Reappear() ` | Makes a window reappear that was previously made to disappear using Disappear()|
-| `SetAlpha(alpha)` | Sets the window's transparency. 0 is completely transparent. 1 is fully visible, normal . Can also use the property Window.AlphaChannel instead of method function call|
-| CloseNonBlocking()
(NO LONGER USED.
use Close instead) | Closes a non-blocking window |
-| FindElement(key, silent_on_error=False) (shorthand version
Element) | Returns the Element that has a matching key. If the key is not found, an Error Element is returned so that the program will not crash should the user try to perform an "update". A Popup message will be shown |
-|||
+The only time you will see this call is in OLD CODE. Please file an Issue if you see this call in any code or in any documentation. It must go away never to be seen again.
# Elements
"Elements" are the building blocks used to create windows. Some GUI APIs use the term "Widget" to describe these graphic elements.
@@ -1733,8 +2064,8 @@ Many of the main method calls and Element names have shortcuts. This enables yo
## Text Element | `T == Txt == Text`
Basic Element. It displays text. That's it.
- Text - Display some text in the window. Can be single or multiple lines but no scrolling if multiple lines.
-
+ Text - Display some text in the window. Usually this means a single line of text. However, the text can also
+ be multiple lines. If multi-lined there are no scroll bars.
```python
layout = [
@@ -1744,63 +2075,81 @@ layout = [
```
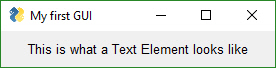
-```python
+```
Text(text,
- size=(None, None),
- auto_size_text=None,
- click_submits=False,
- enable_events=False,
- relief=None,
- font=None,
- text_color=None,
- background_color=None,
- justification=None,
- pad=None,
- key=None,
- right_click_menu=None,
- tooltip=None,
- visible=True)
+ size=(None, None),
+ auto_size_text=None,
+ click_submits=False,
+ enable_events=False,
+ relief=None,
+ font=None,
+ text_color=None,
+ background_color=None,
+ justification=None,
+ pad=None,
+ key=None,
+ right_click_menu=None,
+ tooltip=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|text|The text to display (required)|
-|size|(w,h) w=characters-wide, h=rows-high|
-|auto_size_text|True if size should fit the text length|
-|click_submits|????????????????????(Default = False)|
-|enable_events|Turns on the element specific events.(Default = False)|
-|relief|relief style. Values are same as progress meter relief values. Can be a constant or a string: `RELIEF_RAISED RELIEF_SUNKEN RELIEF_FLAT RELIEF_RIDGE RELIEF_GROOVE RELIEF_SOLID`|
-|font|specifies the font family, size, etc|
-|text_color|color of the text|
-|background_color|color of background|
-|justification|justification for data display|
-|pad|Amount of padding to put around element|
-|key|Used with window.FindElement and with return values|
-|right_click_menu|see "Right Click Menus"|
-|tooltip|text, that will appear the you hover on|
-|visible|set visibility state of the element (Default = True)|
+|text|(str) The text to display. Can include /n to achieve multiple lines|
+|size|(int, int) (width, height) width = characters-wide, height = rows-high|
+|auto_size_text|(bool) if True size of the Text Element will be sized to fit the string provided in 'text' parm|
+|click_submits|(bool) DO NOT USE. Only listed for backwards compat - Use enable_events instead|
+|enable_events|(bool) Turns on the element specific events. Text events happen when the text is clicked|
+|relief|(str/enum) relief style around the text. Values are same as progress meter relief values. Should be a constant that is defined at starting with "RELIEF_" - `RELIEF_RAISED, RELIEF_SUNKEN, RELIEF_FLAT, RELIEF_RIDGE, RELIEF_GROOVE, RELIEF_SOLID`|
+|font|Union[str, tuple] specifies the font family, size, etc|
+|text_color|(str) color of the text|
+|background_color|(str) color of background|
+|justification|(str) how string should be aligned within space provided by size. Valid choices = `left`, `right`, `center`|
+|pad|(int, int) or ((int, int),(int,int)) Amount of padding to put around element (left/right, top/bottom) or ((left, right), (top, bottom))|
+|key|(Any) Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element|
+|right_click_menu|List[List[str]] see "Right Click Menus"|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
+|visible|(bool) set visibility state of the element|
### Methods
-```python
+#### Update
+
+Changes some of the settings for the Text Element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
Update(value=None,
- background_color=None,
- text_color=None,
- font=None,
- visible=None)
+ background_color=None,
+ text_color=None,
+ font=None,
+ visible=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|value|new value to show|
-|background_color|color of background|
-|text_color|color of the text|
-|font|specifies the font family, size, etc|
-|visible|change visibility of element|
+|value|(str) new text to show|
+|background_color|(str) color of background|
+|text_color|(str) color of the text|
+|font|Union[str, tuple] specifies the font family, size, etc|
+|visible|(bool) set visibility state of the element|
+
+#### SetToolTip
+
+Called by application to change the tooltip text for an Element. Normally invoked using the Element Object such as: window.Element('key').SetToolTip('New tip').
+
+```
+SetTooltip(tooltip_text)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|tooltip_text|(str) the text to show in tooltip.|
---
@@ -1827,7 +2176,7 @@ If you set the parameter `enable_events` or `click_submits` then you will get an
## Multiline Element
This Element doubles as both an input and output Element.
-
+ Multiline Element - Display and read multiple lines of text. This is both an input and output element.
```python
layout = [[sg.Multiline('This is what a Multi-line Text Element looks like', size=(45,5))]]
@@ -1835,109 +2184,149 @@ layout = [[sg.Multiline('This is what a Multi-line Text Element looks like', siz
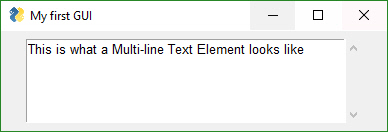
-```python
+```
Multiline(default_text="",
- enter_submits=False,
- disabled=False,
- autoscroll=False,
- border_width=None,
- size=(None, None),
- auto_size_text=None,
- background_color=None,
- text_color=None,
- change_submits=False,
- enable_events=False,
- do_not_clear=True,
- key=None,
- focus=False,
- font=None,
- pad=None,
- tooltip=None,
- right_click_menu=None,
- visible=True)
+ enter_submits=False,
+ disabled=False,
+ autoscroll=False,
+ border_width=None,
+ size=(None, None),
+ auto_size_text=None,
+ background_color=None,
+ text_color=None,
+ change_submits=False,
+ enable_events=False,
+ do_not_clear=True,
+ key=None,
+ focus=False,
+ font=None,
+ pad=None,
+ tooltip=None,
+ right_click_menu=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|default_text|text to display in multiline (Default value = '')|
-|enter_submits|???????????? (Default = False)|
-|disabled|set disable state for element (Default = False)|
-|autoscroll|?????????? (Default = False)|
-|border_width|width of border around element|
-|size|(w,h) w=characters-wide, h=rows-high|
-|auto_size_text|True if size should fit the text length|
-|background_color|color of background|
-|text_color|color of the text|
-|change_submits|If True, pressing Enter key submits window (Default = False)|
-|enable_events|Turns on the element specific events.(Default = False)|
-|do_not_clear|see docx (Default = True)|
-|key|Used with window.FindElement and with return values|
-|focus|if focus should be set to this|
-|font|specifies the font family, size, etc|
-|pad|Amount of padding to put around element|
-|tooltip|text, that will appear the you hover on|
-|right_click_menu|see "Right Click Menus"|
-|visible|set visibility state of the element (Default = True)|
+|default_text|(str) Initial text to show|
+|enter_submits|(bool) if True, the Window.Read call will return is enter key is pressed in this element|
+|disabled|(bool) set disable state|
+|autoscroll|(bool) If True the contents of the element will automatically scroll as more data added to the end|
+|border_width|(int) width of border around element in pixels|
+|size|(int, int) (width, height) width = characters-wide, height = rows-high|
+|auto_size_text|(bool) if True will size the element to match the length of the text|
+|background_color|(str) color of background|
+|text_color|(str) color of the text|
+|change_submits|(bool) DO NOT USE. Only listed for backwards compat - Use enable_events instead|
+|enable_events|(bool) Turns on the element specific events. Spin events happen when an item changes|
+|do_not_clear|if False the element will be cleared any time the Window.Read call returns|
+|key|(Any) Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element|
+|focus|(bool) if True initial focus will go to this element|
+|font|Union[str, tuple] specifies the font family, size, etc|
+|pad|(int, int) or ((int, int),(int,int)) Amount of padding to put around element (left/right, top/bottom) or ((left, right), (top, bottom))|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
+|right_click_menu|List[List[str]] see "Right Click Menus"|
+|visible|(bool) set visibility state of the element|
### Methods
-```python
+#### Update
+
+Changes some of the settings for the Multiline Element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
Update(value=None,
- disabled=None,
- append=False,
- font=None,
- text_color=None,
- background_color=None,
- visible=None,
- autoscroll=None)
+ disabled=None,
+ append=False,
+ font=None,
+ text_color=None,
+ background_color=None,
+ visible=None,
+ autoscroll=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|value|new text to display|
-|disabled|disable or enable state of the element|
-|append|(Default = False)|
-|font|specifies the font family, size, etc|
-|text_color|color of the text|
-|background_color|color of background|
-|visible|change visibility of element|
-|autoscroll|??????????????????|
+|value|(str) new text to display|
+|disabled|(bool) disable or enable state of the element|
+|append|(bool) if True then new value will be added onto the end of the current value. if False then contents will be replaced.|
+|font|Union[str, tuple] specifies the font family, size, etc|
+|text_color|(str) color of the text|
+|background_color|(str) color of background|
+|visible|(bool) set visibility state of the element|
+|autoscroll|(bool) if True then contents of element are scrolled down when new text is added to the end|
+
+#### Get
+
+Return current contents of the Multiline Element
+
+```
+Get() -> (str) current contents of the Multiline Input element
+```
+
+#### SetFocus
+
+Moves the focus to this Multiline
+
+```
+SetFocus(force=False)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|force|(bool). If True, will call focus_force instead of focus_set|
+
+#### SetTooltip
+
+Called by application to change the tooltip text for an Element. Normally invoked using the Element Object such as: window.Element('key').SetToolTip('New tip').
+
+```
+SetTooltip(tooltip_text)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|tooltip_text|(str) the text to show in tooltip.|
## Text Input Element | `InputText == Input == In`
Display a single text input field. Based on the tkinter Widget `Entry`
-
+
```python
layout = [[sg.InputText('Default text')]]
```

-```python
+```
InputText(default_text="",
- size=(None, None),
- disabled=False,
- password_char="",
- justification=None,
- background_color=None,
- text_color=None,
- font=None,
- tooltip=None,
- change_submits=False,
- enable_events=False,
- do_not_clear=True,
- key=None,
- focus=False,
- pad=None,
- right_click_menu=None,
- visible=True)
+ size=(None, None),
+ disabled=False,
+ password_char="",
+ justification=None,
+ background_color=None,
+ text_color=None,
+ font=None,
+ tooltip=None,
+ change_submits=False,
+ enable_events=False,
+ do_not_clear=True,
+ key=None,
+ focus=False,
+ pad=None,
+ right_click_menu=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -1949,7 +2338,7 @@ Parameters explained:
|background_color|(str) color of background in one of the color formats|
|text_color|(str) color of the text|
|font|(str / tuple) specifies the font family, size, etc|
-|tooltip|(str) Text shown when cursor hovers over the element|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
|change_submits|(bool) * DEPRICATED DO NOT USE! Same as enable_events|
|enable_events|(bool) If True then changes to this element are immediately reported as an event. Use this instead of change_submits (Default = False)|
|do_not_clear|(bool) If False then the field will be set to blank after ANY event (button, any event) (Default = True)|
@@ -1961,14 +2350,18 @@ Parameters explained:
### Methods
-```python
+#### Update
+
+Changes some of the settings for the Input Element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
Update(value=None,
- disabled=None,
- select=None,
- visible=None)
+ disabled=None,
+ select=None,
+ visible=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -1977,21 +2370,52 @@ Parameters explained:
|select|(bool) if True, then the text will be selected|
|visible|(bool) change visibility of element|
-Get() - Read and return the current value of the input element. Must call `Window.Read` or `Window.Finalize` prior
+#### Get
- :return: (str) current value of Input field or '' if error encountered
+Read and return the current value of the input element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
+Get() -> (str) current value of Input field or '' if error encountered
+```
+
+#### SetFocus
+
+Sets focus to this element using focus_set. Will use focus_force if force flag set. Must call `Window.Read` or `Window.Finalize` prior
+
+```
+SetFocus(force=False)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|force|(bool) if True then tkinter's `Entry.focus_force` will be called instead of `Entry.focus_set`|
+
+#### SetToolTip
+
+Called by application to change the tooltip text for an Element. Normally invoked using the Element Object such as: window.Element('key').SetToolTip('New tip').
+
+```
+SetTooltip(tooltip_text)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|tooltip_text|(str) the text to show in tooltip.|
---
-#### do_not_clear Parameter
+#### Note about the `do_not_clear` parameter
-**Important** - This trips a lot of people up. If you do not set the `do_not_clear` parameter then the input field will clear when an event takes place. The behavior is a "forms" style window development. The assumption is that you want the field to clear. If you are writing a chat program then you're thankful. The rest of you, I'm sorry.
+This used to really trip people up, but don't think so anymore. The `do_not_clear` parameter is initialized when creating the InputText Element. If set to False, then the input field's contents will be erased after every `Window.Read()` call. Use this setting for when your window is an "Input Form" type of window where you want all of the fields to be erased and start over again every time.
## Combo Element | `Combo == InputCombo == DropDown == Drop`
Also known as a drop-down list. Only required parameter is the list of choices. The return value is a string matching what's visible on the GUI.
ComboBox Element - A combination of a single-line input and a drop-down menu. User can type in their own value or choose from list.
-
```python
layout = [[sg.Combo(['choice 1', 'choice 2'])]]
@@ -1999,74 +2423,99 @@ layout = [[sg.Combo(['choice 1', 'choice 2'])]]
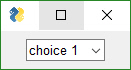
-```python
+```
Combo(values,
- default_value=None,
- size=(None, None),
- auto_size_text=None,
- background_color=None,
- text_color=None,
- change_submits=False,
- enable_events=False,
- disabled=False,
- key=None,
- pad=None,
- tooltip=None,
- readonly=False,
- font=None,
- visible=True)
+ default_value=None,
+ size=(None, None),
+ auto_size_text=None,
+ background_color=None,
+ text_color=None,
+ change_submits=False,
+ enable_events=False,
+ disabled=False,
+ key=None,
+ pad=None,
+ tooltip=None,
+ readonly=False,
+ font=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|values|values to choice|
-|default_value|Choices to be displayed. List of strings|
-|size|(w,h) w=characters-wide, h=rows-high|
-|auto_size_text|True if size should fit the text length|
-|background_color|color of background|
-|text_color|color of the text|
-|change_submits|(bool) DEPRICATED DO NOT USE. Use `enable_events` instead (Default = False)|
-|enable_events|(bool) Turns on the element specific events.(Default = False)|
-|disabled|(bool) set disable state for element (Default = False)|
-|key|(any) Used with window.FindElement and with return values|
+|values|List[Any] values to choose. While displayed as text, the items returned are what the caller supplied, not text|
+|default_value|(Any) Choice to be displayed as initial value. Must match one of values variable contents|
+|size|(int, int) (width, height) width = characters-wide, height = rows-high|
+|auto_size_text|(bool) True if element should be the same size as the contents|
+|background_color|(str) color of background|
+|text_color|(str) color of the text|
+|change_submits|(bool) DEPRICATED DO NOT USE. Use `enable_events` instead|
+|enable_events|(bool) Turns on the element specific events. Combo event is when a choice is made|
+|disabled|(bool) set disable state for element|
+|key|(Any) Used with window.FindElement and with return values to uniquely identify this element|
|pad|(int, int) or ((int, int),(int,int)) Amount of padding to put around element (left/right, top/bottom) or ((left, right), (top, bottom))|
|tooltip|(str) text that will appear when mouse hovers over this element|
-|readonly|(combo) make element readonly (user can't change). True means user cannot change (Default = False)|
-|font|(str, tuple) specifies the font family, size, etc|
-|visible|(bool) set visibility state of the element (Default = True)|
+|readonly|(bool) make element readonly (user can't change). True means user cannot change|
+|font|Union[str, tuple] specifies the font family, size, etc|
+|visible|(bool) set visibility state of the element|
### Methods
-```python
+#### Update
+
+Changes some of the settings for the Combo Element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
Update(value=None,
- values=None,
- set_to_index=None,
- disabled=None,
- readonly=None,
- font=None,
- visible=None)
+ values=None,
+ set_to_index=None,
+ disabled=None,
+ readonly=None,
+ font=None,
+ visible=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|value|change which value is current selected|
-|values|change list of choices|
-|set_to_index|change selection to a particular choice|
-|disabled|disable or enable state of the element|
-|readonly|make element readonly|
-|font|specifies the font family, size, etc|
-|visible|change visibility of element|
+|value|(Any) change which value is current selected hased on new list of previous list of choices|
+|values|List[Any] change list of choices|
+|set_to_index|(int) change selection to a particular choice starting with index = 0|
+|disabled|(bool) disable or enable state of the element|
+|readonly|(bool) if True make element readonly (user cannot change any choices)|
+|font|Union[str, tuple] specifies the font family, size, using one of the two font formats|
+|visible|(bool) control visibility of element|
+
+#### GetSelectedItemsIndexes
+
+Get the list of chosen items and return them as a list of indexes (offsets within the list)
+
+```
+GetSelectedItemsIndexes() -> List[int] List of indexes of currently selected items
+```
+
+#### SetToolTip
+
+Called by application to change the tooltip text for an Element. Normally invoked using the Element Object such as: window.Element('key').SetToolTip('New tip').
+
+```
+SetTooltip(tooltip_text)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|tooltip_text|(str) the text to show in tooltip.|
## Listbox Element
The standard listbox like you'll find in most GUIs. Note that the return values from this element will be a ***list of results, not a single result***. This is because the user can select more than 1 item from the list (if you set the right mode).
A List Box. Provide a list of values for the user to choose one or more of. Returns a list of selected rows
when a window.Read() is executed.
-
```python
layout = [[sg.Listbox(values=['Listbox 1', 'Listbox 2', 'Listbox 3'], size=(30, 6))]]
@@ -2074,80 +2523,121 @@ layout = [[sg.Listbox(values=['Listbox 1', 'Listbox 2', 'Listbox 3'], size=(30,
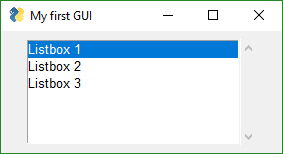
-```python
+```
Listbox(values,
- default_values=None,
- select_mode=None,
- change_submits=False,
- enable_events=False,
- bind_return_key=False,
- size=(None, None),
- disabled=False,
- auto_size_text=None,
- font=None,
- background_color=None,
- text_color=None,
- key=None,
- pad=None,
- tooltip=None,
- right_click_menu=None,
- visible=True)
+ default_values=None,
+ select_mode=None,
+ change_submits=False,
+ enable_events=False,
+ bind_return_key=False,
+ size=(None, None),
+ disabled=False,
+ auto_size_text=None,
+ font=None,
+ background_color=None,
+ text_color=None,
+ key=None,
+ pad=None,
+ tooltip=None,
+ right_click_menu=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|values|list of values to display. Can be any type including mixed types as long as they have __str__ method|
-|default_values|list of objects for listbox|
-|select_mode|can be a string or a constant value defined as a variable. Generally speaking strings are used for these kinds of options|
-|change_submits|If True, pressing Enter key submits window (Default = False)|
-|enable_events|Turns on the element specific events.(Default = False)|
-|bind_return_key|(Default = False)|
-|size|(w,h) w=characters-wide, h=rows-high|
-|disabled|set disable state for element (Default = False)|
-|auto_size_text|True if size should fit the text length|
-|font|specifies the font family, size, etc|
-|background_color|color of background|
-|text_color|color of the text|
-|key|Used with window.FindElement and with return values|
-|pad|Amount of padding to put around element|
-|tooltip|text, that will appear the you hover on|
-|right_click_menu|see "Right Click Menus"|
-|visible|set visibility state of the element (Default = True)|
+|values|List[Any] list of values to display. Can be any type including mixed types as long as they have __str__ method|
+|default_values|List[Any] which values should be initially selected|
+|select_mode|[str] can be a string or a constant value defined as a variable. Generally speaking strings are used for these kinds of options. Valid choices begin with "LISTBOX_SELECT_MODE_"|
+|change_submits|(bool) DO NOT USE. Only listed for backwards compat - Use enable_events instead|
+|enable_events|(bool) Turns on the element specific events. Listbox generates events when an item is clicked|
+|bind_return_key|(bool) If True, then the return key will cause a the Listbox to generate an event|
+|size|Tuple(int, int) (width, height) width = characters-wide, height = rows-high|
+|disabled|(bool) set disable state for element|
+|auto_size_text|(bool) True if element should be the same size as the contents|
+|font|Union[str, tuple] specifies the font family, size. Uses one of two font specifications formats|
+|background_color|(str) color of background|
+|text_color|(str) color of the text|
+|key|(Any) Used with window.FindElement and with return values to uniquely identify this element|
+|pad|(int, int) or ((int, int),(int,int)) Amount of padding to put around element (left/right, top/bottom) or ((left, right), (top, bottom))|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
+|right_click_menu|List[List[str]] see "Right Click Menus" for format|
+|visible|(bool) set visibility state of the element|
### Methods
-```python
+#### Update
+
+Changes some of the settings for the Listbox Element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
Update(values=None,
- disabled=None,
- set_to_index=None,
- scroll_to_index=None,
- visible=None)
+ disabled=None,
+ set_to_index=None,
+ scroll_to_index=None,
+ visible=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|values|new list of choices to be shown to user|
-|disabled|disable or enable state of the element|
-|set_to_index|highlights the item at this index as if user clicked|
-|scroll_to_index|scroll the listbox so that this index is the first shown|
-|visible|change visibility of element|
+|values|List[Any] new list of choices to be shown to user|
+|disabled|(bool) disable or enable state of the element|
+|set_to_index|(int) highlights the item at this index as if user clicked|
+|scroll_to_index|(int) scroll the listbox so that this index is the first shown|
+|visible|(bool) control visibility of element|
-```python
+#### SetValue
+
+Set listbox highlighted choices
+
+```
SetValue(values)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|values|new values to choice|
+|values|List[Any] new values to choose based on previously set values|
-GetListValues() - Returns list of Values provided by the user in the user's format
- :return: List. List of values. Can be any / mixed types -> []
+#### GetListValues
+
+Returns list of Values provided by the user in the user's format
+
+```
+GetListValues() -> List[Any]. List of values. Can be any / mixed types -> []
+```
+
+#### SetFocus
+
+Moves the focus to this Listbox
+
+```
+SetFocus(force=False)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|force|(bool). If True, will call focus_force instead of focus_set|
+
+#### SetToolTip
+
+Called by application to change the tooltip text for an Element. Normally invoked using the Element Object such as: window.Element('key').SetToolTip('New tip').
+
+```
+SetTooltip(tooltip_text)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|tooltip_text|(str) the text to show in tooltip.|
---
@@ -2159,8 +2649,6 @@ Another way ListBoxes can cause Reads to return is if the flag bind_return_key i
Sliders have a couple of slider-specific settings as well as appearance settings. Examples include the `orientation` and `range` settings.
-
-
```python
layout = [[sg.Slider(range=(1,500),
default_value=222,
@@ -2171,29 +2659,29 @@ layout = [[sg.Slider(range=(1,500),
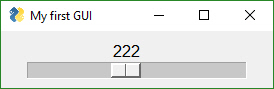
-```python
+```
Slider(range=(None, None),
- default_value=None,
- resolution=None,
- tick_interval=None,
- orientation=None,
- disable_number_display=False,
- border_width=None,
- relief=None,
- change_submits=False,
- enable_events=False,
- disabled=False,
- size=(None, None),
- font=None,
- background_color=None,
- text_color=None,
- key=None,
- pad=None,
- tooltip=None,
- visible=True)
+ default_value=None,
+ resolution=None,
+ tick_interval=None,
+ orientation=None,
+ disable_number_display=False,
+ border_width=None,
+ relief=None,
+ change_submits=False,
+ enable_events=False,
+ disabled=False,
+ size=(None, None),
+ font=None,
+ background_color=None,
+ text_color=None,
+ key=None,
+ pad=None,
+ tooltip=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -2212,9 +2700,9 @@ Parameters explained:
|font|specifies the font family, size, etc|
|background_color|color of background|
|text_color|color of the text|
-|key|Used with window.FindElement and with return values|
+|key|Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element|
|pad|Amount of padding to put around element|
-|tooltip|text, that will appear the you hover on|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
|visible|set visibility state of the element (Default = True)|
### Qt Sliders
@@ -2223,28 +2711,30 @@ There is an important difference between Qt and tkinter sliders. On Qt, the sli
### Slider Methods
-```python
+Changes some of the settings for the Slider Element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
Update(value=None,
- range=(None, None),
- disabled=None,
- visible=None)
+ range=(None, None),
+ disabled=None,
+ visible=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
|value|set current selection to value new value in slider|
|range|new range in slider|
-|disabled|disable or enable state of the element|
-|visible|change visibility of element|
+|disabled|(bool) disable or enable state of the element|
+|visible|(bool) control visibility of element|
## Radio Element
-Creates one radio button that is assigned to a group of radio buttons. Only 1 of the buttons in the group can be selected at any one time.
-Radio Button Element - Used in a group of other Radio Elements to provide user with ability to select only
- 1 choice in a list of choices.
-
+Creates one radio button that is assigned to a group of radio buttons. Only 1 of the buttons in the group can be selected at any one time.
+
+ Radio Button Element - Used in a group of other Radio Elements to provide user with ability to select only
+ 1 choice in a list of choices.
```python
layout = [
@@ -2255,128 +2745,187 @@ layout = [
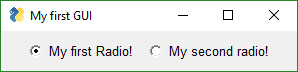
-```python
+```
Radio(text,
- group_id,
- default=False,
- disabled=False,
- size=(None, None),
- auto_size_text=None,
- background_color=None,
- text_color=None,
- font=None,
- key=None,
- pad=None,
- tooltip=None,
- change_submits=False,
- enable_events=False,
- visible=True)
+ group_id,
+ default=False,
+ disabled=False,
+ size=(None, None),
+ auto_size_text=None,
+ background_color=None,
+ text_color=None,
+ font=None,
+ key=None,
+ pad=None,
+ tooltip=None,
+ change_submits=False,
+ enable_events=False,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|text|Text to display next to button|
-|group_id|Groups together multiple Radio Buttons. Can be any value|
-|default|Bool. Initial state (Default = False)|
-|disabled|set disable state for element (Default = False)|
-|size|(w,h) w=characters-wide, h=rows-high|
-|auto_size_text|True if size should fit the text length|
-|background_color|color of background|
-|text_color|color of the text|
-|font|specifies the font family, size, etc|
-|key|Used with window.FindElement and with return values|
-|pad|Amount of padding to put around element|
-|tooltip|text, that will appear the you hover on|
-|change_submits|If True, pressing Enter key submits window (Default = False)|
-|enable_events|Turns on the element specific events.(Default = False)|
-|visible|set visibility state of the element (Default = True)|
+|text|(str) Text to display next to button|
+|group_id|(Any) Groups together multiple Radio Buttons. Any type works|
+|default|(bool). Set to True for the one element of the group you want initially selected|
+|disabled|(bool) set disable state|
+|size|(int, int) (width, height) width = characters-wide, height = rows-high|
+|auto_size_text|(bool) if True will size the element to match the length of the text|
+|background_color|(str) color of background|
+|text_color|(str) color of the text|
+|font|Union[str, tuple] specifies the font family, size, etc|
+|key|(Any) Used with window.FindElement and with return values to uniquely identify this element|
+|pad|(int, int) or ((int, int),(int,int)) Amount of padding to put around element (left/right, top/bottom) or ((left, right), (top, bottom))|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
+|change_submits|(bool) DO NOT USE. Only listed for backwards compat - Use enable_events instead|
+|enable_events|(bool) Turns on the element specific events. Radio Button events happen when an item is selected|
+|visible|(bool) set visibility state of the element|
### Methods
-```python
+#### Update
+
+Changes some of the settings for the Radio Button Element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
Update(value=None,
- disabled=None,
- visible=None)
+ disabled=None,
+ visible=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|value|Bool. if True change to selected|
-|disabled|disable or enable state of the element|
-|visible|change visibility of element|
+|value|(bool) if True change to selected and set others in group to unselected|
+|disabled|(bool) disable or enable state of the element|
+|visible|(bool) control visibility of element|
+
+#### Get
+
+A snapshot of the value of Radio Button -> (bool)
+
+```
+Get() -> (bool) True if this radio button is selected
+```
+
+#### ResetGroup
+
+Sets all Radio Buttons in the group to not selected
+
+```
+ResetGroup() ->
+```
+
+#### SetTooltip
+
+Called by application to change the tooltip text for an Element. Normally invoked using the Element Object such as: window.Element('key').SetToolTip('New tip').
+
+```
+SetTooltip(tooltip_text)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|tooltip_text|(str) the text to show in tooltip.|
## Checkbox Element | `CBox == CB == Check`
Checkbox elements are like Radio Button elements. They return a bool indicating whether or not they are checked.
-
+ Checkbox Element - Displays a checkbox and text next to it
```python
layout = [[sg.Checkbox('My first Checkbox!', default=True), sg.Checkbox('My second Checkbox!')]]
```

-```python
+```
Checkbox(text,
- default=False,
- size=(None, None),
- auto_size_text=None,
- font=None,
- background_color=None,
- text_color=None,
- change_submits=False,
- enable_events=False,
- disabled=False,
- key=None,
- pad=None,
- tooltip=None,
- visible=True)
+ default=False,
+ size=(None, None),
+ auto_size_text=None,
+ font=None,
+ background_color=None,
+ text_color=None,
+ change_submits=False,
+ enable_events=False,
+ disabled=False,
+ key=None,
+ pad=None,
+ tooltip=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|text|Text to display next to checkbox|
-|default|Bool + None. Initial state. True = Checked, False = unchecked, None = Not available (grayed out) (Default = False)|
-|size|(w,h) w=characters-wide, h=rows-high|
-|auto_size_text|True if size should fit the text length|
-|font|specifies the font family, size, etc|
-|background_color|color of background|
-|text_color|color of the text|
-|change_submits|If True, pressing Enter key submits window (Default = False)|
-|enable_events|Turns on the element specific events.(Default = False)|
-|disabled|set disable state for element (Default = False)|
-|key|Used with window.FindElement and with return values|
-|pad|Amount of padding to put around element|
-|tooltip|text, that will appear the you hover on|
-|visible|set visibility state of the element (Default = True)|
+|text|(str) Text to display next to checkbox|
+|default|(bool). Set to True if you want this checkbox initially checked|
+|size|(int, int) (width, height) width = characters-wide, height = rows-high|
+|auto_size_text|(bool) if True will size the element to match the length of the text|
+|font|Union[str, tuple] specifies the font family, size, etc|
+|background_color|(str) color of background|
+|text_color|(str) color of the text|
+|change_submits|(bool) DO NOT USE. Only listed for backwards compat - Use enable_events instead|
+|enable_events|(bool) Turns on the element specific events. Checkbox events happen when an item changes|
+|disabled|(bool) set disable state|
+|key|(Any) Used with window.FindElement and with return values to uniquely identify this element|
+|pad|(int, int) or ((int, int),(int,int)) Amount of padding to put around element (left/right, top/bottom) or ((left, right), (top, bottom))|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
+|visible|(bool) set visibility state of the element|
### Methods
-```python
+#### Update
+
+Changes some of the settings for the Checkbox Element. Must call `Window.Read` or `Window.Finalize` prior.
+Note that changing visibility may cause element to change locations when made visible after invisible
+
+```
Update(value=None,
- disabled=None,
- visible=None)
+ disabled=None,
+ visible=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|value|Bool if True checks the checkbox|
-|disabled|disable or enable state of the element|
-|visible|change visibility of element|
+|value|(bool) if True checks the checkbox, False clears it|
+|disabled|(bool) disable or enable element|
+|visible|(bool) control visibility of element|
-Get() - method
+#### Get
+
+Return the current state of this checkbox
+
+```
+Get() -> (bool) Current state of checkbox
+```
+
+#### SetTooltip
+
+Called by application to change the tooltip text for an Element. Normally invoked using the Element Object such as: window.Element('key').SetToolTip('New tip').
+
+```
+SetTooltip(tooltip_text)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|tooltip_text|(str) the text to show in tooltip.|
## Spin Element
An up/down spinner control. The valid values are passed in as a list.
-
+ A spinner with up/down buttons and a single line of text. Choose 1 values from list
```python
layout = [[sg.Spin([i for i in range(1,11)], initial_value=1), sg.Text('Volume level')]]
@@ -2384,41 +2933,41 @@ layout = [[sg.Spin([i for i in range(1,11)], initial_value=1), sg.Text('Volume
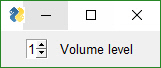
-```python
+```
Spin(values,
- initial_value=None,
- disabled=False,
- change_submits=False,
- enable_events=False,
- size=(None, None),
- auto_size_text=None,
- font=None,
- background_color=None,
- text_color=None,
- key=None,
- pad=None,
- tooltip=None,
- visible=True)
+ initial_value=None,
+ disabled=False,
+ change_submits=False,
+ enable_events=False,
+ size=(None, None),
+ auto_size_text=None,
+ font=None,
+ background_color=None,
+ text_color=None,
+ key=None,
+ pad=None,
+ tooltip=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|values|List of valid values|
-|initial_value|String with initial value|
-|disabled|set disable state for element (Default = False)|
-|change_submits|If True, pressing Enter key submits window (Default = False)|
-|enable_events|Turns on the element specific events.(Default = False)|
-|size|(w,h) w=characters-wide, h=rows-high|
-|auto_size_text|True if size should fit the text length|
-|font|specifies the font family, size, etc|
-|background_color|color of background|
-|text_color|color of the text|
-|key|Used with window.FindElement and with return values|
-|pad|Amount of padding to put around element|
-|tooltip|text, that will appear the you hover on|
-|visible|set visibility state of the element (Default = True)|
+|values|List[Any] List of valid values|
+|initial_value|[Any] Initial item to show in window. Choose from list of values supplied|
+|disabled|(bool) set disable state|
+|change_submits|(bool) DO NOT USE. Only listed for backwards compat - Use enable_events instead|
+|enable_events|(bool) Turns on the element specific events. Spin events happen when an item changes|
+|size|(int, int) (width, height) width = characters-wide, height = rows-high|
+|auto_size_text|(bool) if True will size the element to match the length of the text|
+|font|Union[str, tuple] specifies the font family, size, etc|
+|background_color|(str) color of background|
+|text_color|(str) color of the text|
+|key|(Any) Used with window.FindElement and with return values to uniquely identify this element|
+|pad|(int, int) or ((int, int),(int,int)) Amount of padding to put around element (left/right, top/bottom) or ((left, right), (top, bottom))|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
+|visible|(bool) set visibility state of the element|
### Qt Differences - `values` is a range!
@@ -2428,46 +2977,70 @@ On Qt values is a tuple representing a range. On plain PySimpleGUI this value i
### Methods
-```python
+#### Update
+
+Changes some of the settings for the Spin Element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
Update(value=None,
- values=None,
- disabled=None,
- visible=None)
+ values=None,
+ disabled=None,
+ visible=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|value|set the current value|
-|values|set available choices|
-|disabled|disable or enable state of the element|
-|visible|change visibility of element|
+|value|(Any) set the current value from list of choices|
+|values|List[Any] set available choices|
+|disabled|(bool) disable or enable state of the element|
+|visible|(bool) control visibility of element|
-Get() - method
+#### Get
+
+Return the current chosen value showing in spinbox.
+This value will be the same as what was provided as list of choices. If list items are ints, then the
+item returned will be an int (not a string)
+
+```
+Get() -> (Any) The currently visible entry
+```
+
+#### SetTooltip
+
+Called by application to change the tooltip text for an Element. Normally invoked using the Element Object such as: window.Element('key').SetToolTip('New tip').
+
+```
+SetTooltip(tooltip_text)
+```
+
+Parameter Descriptions:
+
+|Name|Meaning|
+|---|---|
+|tooltip_text|(str) the text to show in tooltip.|
## Image Element
Images can be placed in your window provide they are in PNG, GIF, PPM/PGM format. JPGs cannot be shown because tkinter does not naively support JPGs. You can use the Python Imaging Library (PIL) package to convert your image to PNG prior to calling PySimpleGUI if your images are in JPG format.
-
-
????????????? add image here
-```python
+```
Image(filename=None,
- data=None,
- background_color=None,
- size=(None, None),
- pad=None,
- key=None,
- tooltip=None,
- right_click_menu=None,
- visible=True,
- enable_events=False)
+ data=None,
+ background_color=None,
+ size=(None, None),
+ pad=None,
+ key=None,
+ tooltip=None,
+ right_click_menu=None,
+ visible=True,
+ enable_events=False)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -2476,29 +3049,31 @@ Parameters explained:
|background_color|color of background|
|size|(w,h) w=characters-wide, h=rows-high|
|pad|Amount of padding to put around element|
-|key|Used with window.FindElement and with return values|
-|tooltip|text, that will appear the you hover on|
-|right_click_menu|see "Right Click Menus"|
+|key|Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
+|right_click_menu|List[List[str]] see "Right Click Menus" for format|
|visible|set visibility state of the element (Default = True)|
|enable_events|Turns on the element specific events.(Default = False)|
### Methods
-```python
+Changes some of the settings for the Image Element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
Update(filename=None,
- data=None,
- size=(None, None),
- visible=None)
+ data=None,
+ size=(None, None),
+ visible=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
|filename||
|data||
|size|(w,h) w=characters-wide, h=rows-high|
-|visible|change visibility of element|
+|visible|(bool) control visibility of element|
Choose **either** a filename or in-ram data image to use to replace current image
@@ -2508,12 +3083,12 @@ You can specify an animated GIF as an image and can animate the GIF by calling `
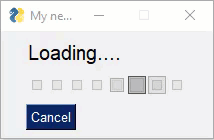
-```python
+```
UpdateAnimation(source,
- time_between_frames=0)
+ time_between_frames=0)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -2571,48 +3146,46 @@ In Oct 2018, the definition of Button changed. Previously Button would CLOSE th
The most basic Button element call to use is `Button`
-
-
```python
layout = [[sg.OK(), sg.Cancel()]]
```
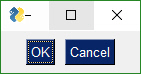
-```python
+```
Button(button_text="",
- button_type=7,
- target=(None, None),
- tooltip=None,
- file_types=(('ALL Files', '*.*'),),
- initial_folder=None,
- disabled=False,
- change_submits=False,
- enable_events=False,
- image_filename=None,
- image_data=None,
- image_size=(None, None),
- image_subsample=None,
- border_width=None,
- size=(None, None),
- auto_size_button=None,
- button_color=None,
- font=None,
- bind_return_key=False,
- focus=False,
- pad=None,
- key=None,
- visible=True)
+ button_type=7,
+ target=(None, None),
+ tooltip=None,
+ file_types=(('ALL Files', '*.*'),),
+ initial_folder=None,
+ disabled=False,
+ change_submits=False,
+ enable_events=False,
+ image_filename=None,
+ image_data=None,
+ image_size=(None, None),
+ image_subsample=None,
+ border_width=None,
+ size=(None, None),
+ auto_size_button=None,
+ button_color=None,
+ font=None,
+ bind_return_key=False,
+ focus=False,
+ pad=None,
+ key=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
|button_text|Text to be displayed on the button (Default value = '')|
|button_type|You should NOT be setting this directly (Default value = BUTTON_TYPE_READ_FORM)|
|target|key or (row,col) target for the button|
-|tooltip|text, that will appear the you hover on|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
|file_types|the filetypes that will be used to match files (Default value = (("ALL Files", "*.*"),))|
|initial_folder|starting path for folders and files|
|disabled|set disable state for element (Default = False)|
@@ -2630,7 +3203,7 @@ Parameters explained:
|bind_return_key|If True the return key will cause this button to fire (Default = False)|
|focus|if focus should be set to this|
|pad|Amount of padding to put around element|
-|key|Used with window.FindElement and with return values|
+|key|Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element|
|visible|set visibility state of the element (Default = True)|
### Shortcut, Pre-defined Buttons
@@ -2829,73 +3402,75 @@ If there are more than 1 button on a window, the FIRST button that is of type Cl
### Methods
-```python
+Changes some of the settings for the Button Element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
Update(text=None,
- button_color=(None, None),
- disabled=None,
- image_data=None,
- image_filename=None,
- visible=None,
- image_subsample=None,
- image_size=None)
+ button_color=(None, None),
+ disabled=None,
+ image_data=None,
+ image_filename=None,
+ visible=None,
+ image_subsample=None,
+ image_size=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
|text|sets button text|
|button_color|(text, background) (Default = (None))|
-|disabled|disable or enable state of the element|
+|disabled|(bool) disable or enable state of the element|
|image_data|in-RAM image to be displayed on button|
|image_filename|image filename if there is a button image|
-|visible|change visibility of element|
+|visible|(bool) control visibility of element|
|image_subsample|amount to reduce the size of the image|
|image_size||
-GetText() - Returns the current text shown on a button
+Returns the current text shown on a button
- :return: string value of button
+```
+GetText() -> string value of button
+```
## ButtonMenu Element
The ButtonMenu element produces a unique kind of effect. It's a button, that when clicked, shows you a menu. It's like clicking one of the top-level menu items on a MenuBar. As a result, the menu definition take the format of a single menu entry from a normal menu definition. A normal menu definition is a list of lists. This definition is one of those lists.
-
-
```python
['Menu', ['&Pause Graph', 'Menu item::optional_key']]
```
The very first string normally specifies what is shown on the menu bar. In this case, the value is **not used**. You set the text for the button using a different parameter, the `button_text` parm.
-```python
+```
ButtonMenu(button_text,
- menu_def,
- tooltip=None,
- disabled=False,
- image_filename=None,
- image_data=None,
- image_size=(None, None),
- image_subsample=None,
- border_width=None,
- size=(None, None),
- auto_size_button=None,
- button_color=None,
- font=None,
- pad=None,
- key=None,
- tearoff=False,
- visible=True)
+ menu_def,
+ tooltip=None,
+ disabled=False,
+ image_filename=None,
+ image_data=None,
+ image_size=(None, None),
+ image_subsample=None,
+ border_width=None,
+ size=(None, None),
+ auto_size_button=None,
+ button_color=None,
+ font=None,
+ pad=None,
+ key=None,
+ tearoff=False,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
|button_text|Text to be displayed on the button (Default value = '')|
|menu_def|??????????????????|
-|tooltip|text, that will appear the you hover on|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
|disabled|set disable state for element (Default = False)|
|image_filename|image filename if there is a button image|
|image_data|in-RAM image to be displayed on button|
@@ -2907,7 +3482,7 @@ Parameters explained:
|button_color|(text color, backound color)|
|font|specifies the font family, size, etc|
|pad|Amount of padding to put around element|
-|key|Used with window.FindElement and with return values|
+|key|Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element|
|tearoff|?????????????????? (Default = False)|
|visible|set visibility state of the element (Default = True)|
@@ -2922,19 +3497,17 @@ This element has limited usefulness and is being included more for completeness
It works best when placed between columns or elements that span multiple rows. If on a "normal" row with elements that are only 1 row high, then it will only span that one row.
-
-
```python
VerticalSeparator(pad=None)
```
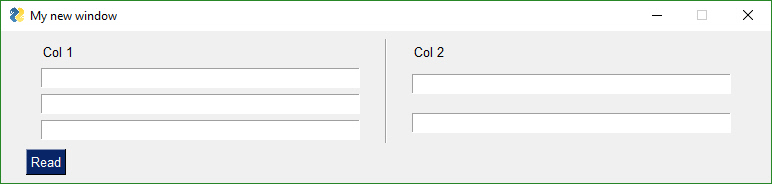
-```python
+```
VerticalSeparator(pad=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -2957,8 +3530,6 @@ The return value for `OneLineProgressMeter` is:
#### Progress Mater in Your window
Another way of using a Progress Meter with PySimpleGUI is to build a custom window with a `ProgressBar` Element in the window. You will need to run your window as a non-blocking window. When you are ready to update your progress bar, you call the `UpdateBar` method for the `ProgressBar` element itself.
-
-
```python
import PySimpleGUI as sg
@@ -2984,21 +3555,21 @@ window.Close()
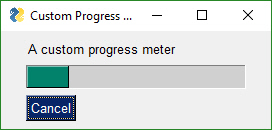
-```python
+```
ProgressBar(max_value,
- orientation=None,
- size=(None, None),
- auto_size_text=None,
- bar_color=(None, None),
- style=None,
- border_width=None,
- relief=None,
- key=None,
- pad=None,
- visible=True)
+ orientation=None,
+ size=(None, None),
+ auto_size_text=None,
+ bar_color=(None, None),
+ style=None,
+ border_width=None,
+ relief=None,
+ key=None,
+ pad=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3010,60 +3581,34 @@ Parameters explained:
|style|????????????????????????????|
|border_width|width of border around button|
|relief|????????????????????????????|
-|key|Used with window.FindElement and with return values|
+|key|Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element|
|pad|Amount of padding to put around element|
|visible|set visibility state of the element (Default = True)|
-### Methods
-
-```python
-Update(visible=None)
-```
-
-Parameters explained:
-
-|Name|Meaning|
-|---|---|
-|visible|change visibility of element|
-
-```python
-UpdateBar(current_count,
- max=None)
-```
-
-Parameters explained:
-
-|Name|Meaning|
-|---|---|
-|current_count|sets the current value|
-|max|changes the max value|
-
## Output Elements
The Output Element is a re-direction of Stdout. Anything "printed" will be displayed in this element.
Note that you will NOT see what you `print` until you call either window.Read or window.Refresh. If you want to immediately see what was printed, call window.Refresh() immediately after your print statement.
-
-
```python
Output(size=(None, None))
```
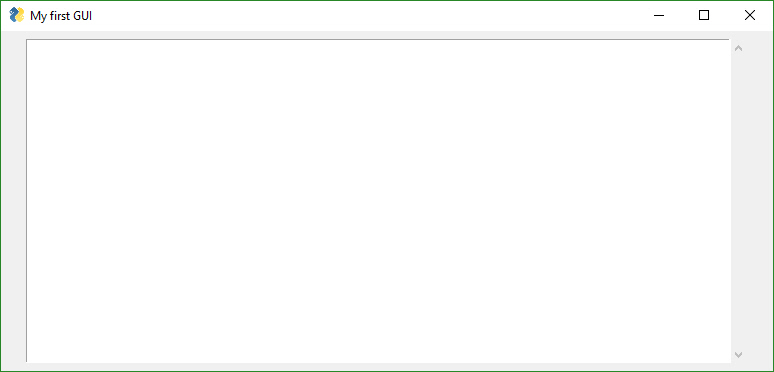
-```python
+```
Output(size=(None, None),
- background_color=None,
- text_color=None,
- pad=None,
- font=None,
- tooltip=None,
- key=None,
- right_click_menu=None,
- visible=True)
+ background_color=None,
+ text_color=None,
+ pad=None,
+ font=None,
+ tooltip=None,
+ key=None,
+ right_click_menu=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3072,24 +3617,26 @@ Parameters explained:
|text_color|color of the text|
|pad|Amount of padding to put around element|
|font|specifies the font family, size, etc|
-|tooltip|text, that will appear the you hover on|
-|key|Used with window.FindElement and with return values|
-|right_click_menu|see "Right Click Menus"|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
+|key|Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element|
+|right_click_menu|List[List[str]] see "Right Click Menus" for format|
|visible|set visibility state of the element (Default = True)|
### Methods
-```python
+Changes some of the settings for the Output Element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
Update(value=None,
- visible=None)
+ visible=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
|value||
-|visible|change visibility of element|
+|visible|(bool) control visibility of element|
----
@@ -3126,25 +3673,23 @@ Columns are specified in exactly the same way as a window is, as a list of lists
Columns are needed when you have an element that has a height > 1 line on the left, with single-line elements on the right. Here's an example of this kind of layout:
-
-
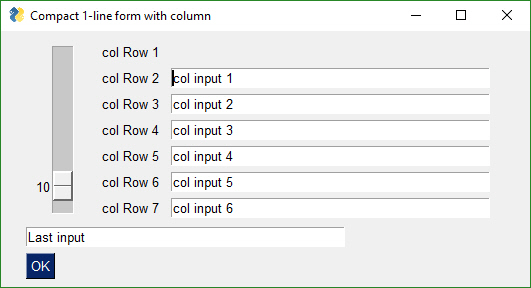
The Column Element has 1 required parameter and 1 optional (the layout and the background color). Setting the background color has the same effect as setting the window's background color, except it only affects the column rectangle.
-```python
+```
Column(layout,
- background_color=None,
- size=(None, None),
- pad=None,
- scrollable=False,
- vertical_scroll_only=False,
- right_click_menu=None,
- key=None,
- visible=True)
+ background_color=None,
+ size=(None, None),
+ pad=None,
+ scrollable=False,
+ vertical_scroll_only=False,
+ right_click_menu=None,
+ key=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3154,8 +3699,8 @@ Parameters explained:
|pad|Amount of padding to put around element|
|scrollable|???????????????????????? (Default = False)|
|vertical_scroll_only|???????????????????????? (Default = False)|
-|right_click_menu|see "Right Click Menus"|
-|key|Used with window.FindElement and with return values|
+|right_click_menu|List[List[str]] see "Right Click Menus" for format|
+|key|Used with window.FindElement and with return values to uniquely identify this element|
|visible|set visibility state of the element (Default = True)|
---
@@ -3198,32 +3743,30 @@ sg.Popup(event, values, line_width=200)
Frames work exactly the same way as Columns. You create layout that is then used to initialize the Frame.
-
-
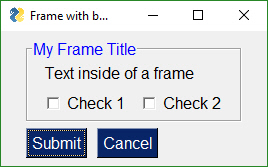
Notice how the Frame layout looks identical to a window layout. A window works exactly the same way as a Column and a Frame. They all are "container elements" - elements that contain other elements.
*These container Elements can be nested as deep as you want.* That's a pretty spiffy feature, right? Took a lot of work so be appreciative. Recursive code isn't trivial.
-```python
+```
Frame(title,
- layout,
- title_color=None,
- background_color=None,
- title_location=None,
- relief="groove",
- size=(None, None),
- font=None,
- pad=None,
- border_width=None,
- key=None,
- tooltip=None,
- right_click_menu=None,
- visible=True)
+ layout,
+ title_color=None,
+ background_color=None,
+ title_location=None,
+ relief="groove",
+ size=(None, None),
+ font=None,
+ pad=None,
+ border_width=None,
+ key=None,
+ tooltip=None,
+ right_click_menu=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3237,9 +3780,9 @@ Parameters explained:
|font|specifies the font family, size, etc|
|pad|Amount of padding to put around element|
|border_width|width of border around element|
-|key|Used with window.FindElement and with return values|
-|tooltip|text, that will appear the you hover on|
-|right_click_menu|see "Right Click Menus"|
+|key|Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
+|right_click_menu|List[List[str]] see "Right Click Menus" for format|
|visible|set visibility state of the element (Default = True)|
---
@@ -3263,20 +3806,18 @@ window = sg.Window('Frame with buttons', font=("Helvetica", 12)).Layout(layout)
In my opinion, the tkinter Canvas Widget is the most powerful of the tkinter widget. While I try my best to completely isolate the user from anything that is tkinter related, the Canvas Element is the one exception. It enables integration with a number of other packages, often with spectacular results.
-
-
-```python
+```
Canvas(canvas=None,
- background_color=None,
- size=(None, None),
- pad=None,
- key=None,
- tooltip=None,
- right_click_menu=None,
- visible=True)
+ background_color=None,
+ size=(None, None),
+ pad=None,
+ key=None,
+ tooltip=None,
+ right_click_menu=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3284,9 +3825,9 @@ Parameters explained:
|background_color|color of background|
|size|(w,h) w=characters-wide, h=rows-high|
|pad|Amount of padding to put around element|
-|key|Used with window.FindElement and with return values|
-|tooltip|text, that will appear the you hover on|
-|right_click_menu|see "Right Click Menus"|
+|key|Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
+|right_click_menu|List[List[str]] see "Right Click Menus" for format|
|visible|set visibility state of the element (Default = True)|
### Matplotlib, Pyplot Integration
@@ -3332,7 +3873,6 @@ See `Demo_Matplotlib.py` for a Recipe you can copy.
TKCanvas - not a method but a property. Returns the tkinter Canvas Widget
-
## Graph Element
All you math fans will enjoy this Element... and all you non-math fans will enjoy it too.
@@ -3360,25 +3900,23 @@ You can move your figures around on the canvas by supplying the Figure ID the x,
This Element is relatively new and may have some parameter additions or deletions. It shouldn't break your code however.
-
-
-```python
+```
Graph(canvas_size,
- graph_bottom_left,
- graph_top_right,
- background_color=None,
- pad=None,
- change_submits=False,
- drag_submits=False,
- enable_events=False,
- key=None,
- tooltip=None,
- right_click_menu=None,
- visible=True,
- float_values=False)
+ graph_bottom_left,
+ graph_top_right,
+ background_color=None,
+ pad=None,
+ change_submits=False,
+ drag_submits=False,
+ enable_events=False,
+ key=None,
+ tooltip=None,
+ right_click_menu=None,
+ visible=True,
+ float_values=False)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3390,9 +3928,9 @@ Parameters explained:
|change_submits|If True, pressing Enter key submits window (Default = False)|
|drag_submits|???????????????????????? (Default = False)|
|enable_events|Turns on the element specific events.(Default = False)|
-|key|Used with window.FindElement and with return values|
-|tooltip|text, that will appear the you hover on|
-|right_click_menu|see "Right Click Menus"|
+|key|Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
+|right_click_menu|List[List[str]] see "Right Click Menus" for format|
|visible|set visibility state of the element (Default = True)|
|float_values|bool: If True x,y coordinates are returned as floats, not ints|
@@ -3416,14 +3954,14 @@ Parameters explained:
All of the Drawing methods return a "***figure***" that can be used move and delete the figure
-```python
+```
DrawLine(point_from,
- point_to,
- color="black",
- width=1)
+ point_to,
+ color="black",
+ width=1)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3432,13 +3970,13 @@ Parameters explained:
|color|(Default value = 'black')|
|width|(Default value = 1)|
-```python
+```
DrawPoint(point,
- size=2,
- color="black")
+ size=2,
+ color="black")
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3446,14 +3984,14 @@ Parameters explained:
|size|(w,h) w=characters-wide, h=rows-high (Default value = 2)|
|color|(Default value = 'black')|
-```python
+```
DrawCircle(center_location,
- radius,
- fill_color=None,
- line_color="black")
+ radius,
+ fill_color=None,
+ line_color="black")
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3462,14 +4000,14 @@ Parameters explained:
|fill_color||
|line_color|(Default value = 'black')|
-```python
+```
DrawOval(top_left,
- bottom_right,
- fill_color=None,
- line_color=None)
+ bottom_right,
+ fill_color=None,
+ line_color=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3478,16 +4016,16 @@ Parameters explained:
|fill_color||
|line_color||
-```python
+```
DrawArc(top_left,
- bottom_right,
- extent,
- start_angle,
- style=None,
- arc_color="black")
+ bottom_right,
+ extent,
+ start_angle,
+ style=None,
+ arc_color="black")
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3498,14 +4036,14 @@ Parameters explained:
|style||
|arc_color|(Default value = 'black')|
-```python
+```
DrawRectangle(top_left,
- bottom_right,
- fill_color=None,
- line_color=None)
+ bottom_right,
+ fill_color=None,
+ line_color=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3514,15 +4052,15 @@ Parameters explained:
|fill_color||
|line_color||
-```python
+```
DrawText(text,
- location,
- color="black",
- font=None,
- angle=0)
+ location,
+ color="black",
+ font=None,
+ angle=0)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3532,49 +4070,53 @@ Parameters explained:
|font|specifies the font family, size, etc|
|angle|(Default value = 0)|
-Erase() - method
-
```python
+Erase()
+```
+
+```
DeleteFigure(id)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
|id||
-```python
+Changes some of the settings for the Graph Element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
Update(background_color,
- visible=None)
+ visible=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
|background_color|color of background|
-|visible|change visibility of element|
+|visible|(bool) control visibility of element|
-```python
+```
Move(x_direction,
- y_direction)
+ y_direction)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
|x_direction||
|y_direction||
-```python
+```
MoveFigure(figure,
- x_direction,
- y_direction)
+ x_direction,
+ y_direction)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3582,13 +4124,13 @@ Parameters explained:
|x_direction||
|y_direction||
-```python
+```
RelocateFigure(figure,
- x,
- y)
+ x,
+ y)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3596,46 +4138,42 @@ Parameters explained:
|x||
|y||
-
-
## Table Element
Out of all of the Elements, it's the Table and the Tree that are the most "problematic" in the tkinter inter and Qt implementations. They're hard is my only defense.
-
-
-```python
+```
Table(values,
- headings=None,
- visible_column_map=None,
- col_widths=None,
- def_col_width=10,
- auto_size_columns=True,
- max_col_width=20,
- select_mode=None,
- display_row_numbers=False,
- num_rows=None,
- row_height=None,
- font=None,
- justification="right",
- text_color=None,
- background_color=None,
- alternating_row_color=None,
- row_colors=None,
- vertical_scroll_only=True,
- hide_vertical_scroll=False,
- size=(None, None),
- change_submits=False,
- enable_events=False,
- bind_return_key=False,
- pad=None,
- key=None,
- tooltip=None,
- right_click_menu=None,
- visible=True)
+ headings=None,
+ visible_column_map=None,
+ col_widths=None,
+ def_col_width=10,
+ auto_size_columns=True,
+ max_col_width=20,
+ select_mode=None,
+ display_row_numbers=False,
+ num_rows=None,
+ row_height=None,
+ font=None,
+ justification="right",
+ text_color=None,
+ background_color=None,
+ alternating_row_color=None,
+ row_colors=None,
+ vertical_scroll_only=True,
+ hide_vertical_scroll=False,
+ size=(None, None),
+ change_submits=False,
+ enable_events=False,
+ bind_return_key=False,
+ pad=None,
+ key=None,
+ tooltip=None,
+ right_click_menu=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3663,9 +4201,9 @@ Parameters explained:
|enable_events|Turns on the element specific events.(Default = False)|
|bind_return_key|(Default = False)|
|pad|Amount of padding to put around element|
-|key|Used with window.FindElement and with return values|
-|tooltip|text, that will appear the you hover on|
-|right_click_menu|see "Right Click Menus"|
+|key|Used with window.FindElement and with return values to uniquely identify this element|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
+|right_click_menu|List[List[str]] see "Right Click Menus" for format|
|visible|set visibility state of the element (Default = True)|
### Read return values from Table Element
@@ -3676,20 +4214,22 @@ The values returned from a `Window.Read` call for the Tree Element are a list of
The Update method can be used to make changes to a table that's already been displayed. The call takes a single parameter, values, which is the new table to display. The entire table is replaced.
-```python
+Changes some of the settings for the Table Element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
Update(values=None,
- num_rows=None,
- visible=None,
- select_rows=None)
+ num_rows=None,
+ visible=None,
+ select_rows=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
|values||
|num_rows||
-|visible|change visibility of element|
+|visible|(bool) control visibility of element|
|select_rows||
---
@@ -3701,35 +4241,33 @@ If you click on the header, it can go into spasms for some tables. I don't under
The Tree Element and Table Element are close cousins. Many of the parameters found in the Table Element apply to Tree Elements. In particular the heading information, column widths, etc.
-
-
-```python
+```
Tree(data=None,
- headings=None,
- visible_column_map=None,
- col_widths=None,
- col0_width=10,
- def_col_width=10,
- auto_size_columns=True,
- max_col_width=20,
- select_mode=None,
- show_expanded=False,
- change_submits=False,
- enable_events=False,
- font=None,
- justification="right",
- text_color=None,
- background_color=None,
- num_rows=None,
- row_height=None,
- pad=None,
- key=None,
- tooltip=None,
- right_click_menu=None,
- visible=True)
+ headings=None,
+ visible_column_map=None,
+ col_widths=None,
+ col0_width=10,
+ def_col_width=10,
+ auto_size_columns=True,
+ max_col_width=20,
+ select_mode=None,
+ show_expanded=False,
+ change_submits=False,
+ enable_events=False,
+ font=None,
+ justification="right",
+ text_color=None,
+ background_color=None,
+ num_rows=None,
+ row_height=None,
+ pad=None,
+ key=None,
+ tooltip=None,
+ right_click_menu=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3752,9 +4290,9 @@ Parameters explained:
|num_rows||
|row_height||
|pad|Amount of padding to put around element|
-|key|Used with window.FindElement and with return values|
-|tooltip|text, that will appear the you hover on|
-|right_click_menu|see "Right Click Menus"|
+|key|Used with window.FindElement and with return values to uniquely identify this element|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
+|right_click_menu|List[List[str]] see "Right Click Menus" for format|
|visible|set visibility state of the element (Default = True)|
Unlike Tables there is no standard format for trees. Thus the data structure passed to the Tree Element must be constructed. This is done using the TreeData class. The process is as follows:
@@ -3819,10 +4357,6 @@ View of second tab:
First we have the Tab layout definitions. They mirror what you see in the screen shots. Tab 1 has 1 Text Element in it. Tab 2 has a Text and an Input Element.
-
-
-
-
```python
tab1_layout = [[sg.T('This is inside tab 1')]]
@@ -3853,24 +4387,24 @@ Check out Tabs 7 and 8. We've got a Window with a Column containing Tabs 5 and
As of Release 3.8.0, not all of *options* shown in the API definitions of the Tab and TabGroup Elements are working. They are there as placeholders.
-```python
+```
TabGroup(layout,
- tab_location=None,
- title_color=None,
- selected_title_color=None,
- background_color=None,
- font=None,
- change_submits=False,
- enable_events=False,
- pad=None,
- border_width=None,
- theme=None,
- key=None,
- tooltip=None,
- visible=True)
+ tab_location=None,
+ title_color=None,
+ selected_title_color=None,
+ background_color=None,
+ font=None,
+ change_submits=False,
+ enable_events=False,
+ pad=None,
+ border_width=None,
+ theme=None,
+ key=None,
+ tooltip=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3885,26 +4419,26 @@ Parameters explained:
|pad|Amount of padding to put around element|
|border_width|width of border around element|
|theme||
-|key|Used with window.FindElement and with return values|
-|tooltip|text, that will appear the you hover on|
+|key|Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
|visible|set visibility state of the element (Default = True)|
-```python
+```
Tab(title,
- layout,
- title_color=None,
- background_color=None,
- font=None,
- pad=None,
- disabled=False,
- border_width=None,
- key=None,
- tooltip=None,
- right_click_menu=None,
- visible=True)
+ layout,
+ title_color=None,
+ background_color=None,
+ font=None,
+ pad=None,
+ disabled=False,
+ border_width=None,
+ key=None,
+ tooltip=None,
+ right_click_menu=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3916,9 +4450,9 @@ Parameters explained:
|pad|Amount of padding to put around element|
|disabled|set disable state for element (Default = False)|
|border_width|width of border around element|
-|key|Used with window.FindElement and with return values|
-|tooltip|text, that will appear the you hover on|
-|right_click_menu|see "Right Click Menus"|
+|key|Used with window.FindElement and with return values to uniquely identify this element to uniquely identify this element|
+|tooltip|(str) text, that will appear when mouse hovers over the element|
+|right_click_menu|List[List[str]] see "Right Click Menus" for format|
|visible|set visibility state of the element (Default = True)|
### Reading Tab Groups
@@ -3927,49 +4461,49 @@ Tab Groups now return a value when a Read returns. They return which tab is cur
### Methods
-```python
+Changes some of the settings for the Tree Element. Must call `Window.Read` or `Window.Finalize` prior
+
+```
Update(values=None,
- key=None,
- value=None,
- text=None,
- icon=None,
- visible=None)
+ key=None,
+ value=None,
+ text=None,
+ icon=None,
+ visible=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
|values||
-|key|Used with window.FindElement and with return values|
+|key|Used with window.FindElement and with return values to uniquely identify this element|
|value||
|text||
|icon||
-|visible|change visibility of element|
+|visible|(bool) control visibility of element|
## Pane Element
New in version 3.20 is the Pane Element, a super-cool tkinter feature. You won't find this one in PySimpleGUIQt, only PySimpleGUI. It's difficult to describe one of these things. Think of them as "Tabs without labels" that you can slide.
-
-
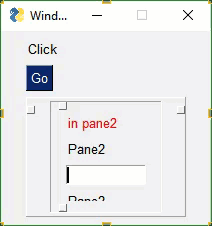
-```python
+```
Pane(pane_list,
- background_color=None,
- size=(None, None),
- pad=None,
- orientation="vertical",
- show_handle=True,
- relief="raised",
- handle_size=None,
- border_width=None,
- key=None,
- visible=True)
+ background_color=None,
+ size=(None, None),
+ pad=None,
+ orientation="vertical",
+ show_handle=True,
+ relief="raised",
+ handle_size=None,
+ border_width=None,
+ key=None,
+ visible=True)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
@@ -3982,7 +4516,7 @@ Parameters explained:
|relief|relief style. Values are same as progress meter relief values. Can be a constant or a string: `RELIEF_RAISED RELIEF_SUNKEN RELIEF_FLAT RELIEF_RIDGE RELIEF_GROOVE RELIEF_SOLID`|
|handle_size||
|border_width|width of border around element|
-|key|Used with window.FindElement and with return values|
+|key|Used with window.FindElement and with return values to uniquely identify this element|
|visible|set visibility state of the element (Default = True)|
***Each "Pane" of a Pane Element must be a Column Element***. The parameter `pane_list` is a list of Column Elements.
@@ -4631,8 +5165,6 @@ Use realtime keyboard capture by calling
Beginning in version 3.01 you can add a MenuBar to your window. You specify the menus in much the same way as you do window layouts, with lists. Menu selections are returned as events and as of 3.17, also as in the values dictionary. The value returned will be the entire menu entry, including the key if you specified one.
-
-
```python
menu_def = [['File', ['Open', 'Save', 'Exit',]],
['Edit', ['Paste', ['Special', 'Normal',], 'Undo'],],
@@ -4645,17 +5177,19 @@ Note the placement of ',' and of []. It's tricky to get the nested menus correc
## Methods
-```python
-Update(menu_definition,
- visible=None)
+Update a menubar - can change the menu definition and visibility
+
+```
+Update(menu_definition=None,
+ visible=None)
```
-Parameters explained:
+Parameter Descriptions:
|Name|Meaning|
|---|---|
-|menu_definition||
-|visible|change visibility of element|
+|menu_definition|List[[str]] New menu defintion|
+|visible|(bool) control visibility of element|
---
@@ -5667,4 +6201,4 @@ GNU Lesser General Public License (LGPL 3) +
## Acknowledgments
-#### SORRY!! Will add these back. Lost due to file length limitation
+#### SORRY!! Will add these back. Lost due to file length limitation
\ No newline at end of file