Merge pull request #326 from MikeTheWatchGuy/Dev-latest
More design pattern changes
This commit is contained in:
commit
b672a7dd55
|
@ -1,5 +1,6 @@
|
|||
|
||||
|
||||
|
||||
# The PySimpleGUI Cookbook
|
||||
|
||||
|
||||
|
@ -13,7 +14,6 @@ Same GUI screen except the return values are in a list instead of a dictionary a
|
|||
import PySimpleGUI as sg
|
||||
|
||||
# Very basic window. Return values as a list
|
||||
window = sg.Window('Simple data entry window')
|
||||
|
||||
layout = [
|
||||
[sg.Text('Please enter your Name, Address, Phone')],
|
||||
|
@ -23,7 +23,8 @@ Same GUI screen except the return values are in a list instead of a dictionary a
|
|||
[sg.Submit(), sg.Cancel()]
|
||||
]
|
||||
|
||||
button, values = window.LayoutAndRead(layout)
|
||||
window = sg.Window('Simple data entry window').Layout(layout)
|
||||
button, values = window.Read()
|
||||
|
||||
print(button, values[0], values[1], values[2])
|
||||
|
||||
|
@ -44,9 +45,9 @@ A simple GUI with default values. Results returned in a dictionary.
|
|||
[sg.Submit(), sg.Cancel()]
|
||||
]
|
||||
|
||||
window = sg.Window('Simple data entry GUI') # begin with a blank Window
|
||||
window = sg.Window('Simple data entry GUI').Layout(layout)
|
||||
|
||||
button, values = window.LayoutAndRead(layout)
|
||||
button, values = window.Read()
|
||||
|
||||
print(button, values['name'], values['address'], values['phone'])
|
||||
|
||||
|
@ -62,12 +63,11 @@ Browse for a filename that is populated into the input field.
|
|||
|
||||
import PySimpleGUI as sg
|
||||
|
||||
|
||||
GUI_rows = [[sg.Text('SHA-1 and SHA-256 Hashes for the file')],
|
||||
[sg.InputText(), sg.FileBrowse()],
|
||||
[sg.Submit(), sg.Cancel()]]
|
||||
|
||||
(button, (source_filename,)) = sg.Window('SHA-1 & 256 Hash').LayoutAndRead(GUI_rows)
|
||||
(button, (source_filename,)) = sg.Window('SHA-1 & 256 Hash').Layout(GUI_rows).Read()
|
||||
|
||||
print(button, source_filename)
|
||||
|
||||
|
@ -82,9 +82,9 @@ Quickly add a GUI allowing the user to browse for a filename if a filename is no
|
|||
import sys
|
||||
|
||||
if len(sys.argv) == 1:
|
||||
button, (fname,) = sg.Window('My Script').LayoutAndRead([[sg.Text('Document to open')],
|
||||
button, (fname,) = sg.Window('My Script').Layout([[sg.Text('Document to open')],
|
||||
[sg.In(), sg.FileBrowse()],
|
||||
[sg.Open(), sg.Cancel()]])
|
||||
[sg.Open(), sg.Cancel()]]).Read()
|
||||
else:
|
||||
fname = sys.argv[1]
|
||||
|
||||
|
@ -110,10 +110,9 @@ Browse to get 2 file names that can be then compared.
|
|||
[sg.Text('File 2', size=(8, 1)), sg.InputText(), sg.FileBrowse()],
|
||||
[sg.Submit(), sg.Cancel()]]
|
||||
|
||||
window = sg.Window('File Compare').Layout(gui_rows)
|
||||
|
||||
window = sg.Window('File Compare')
|
||||
|
||||
button, values = window.LayoutAndRead(gui_rows)
|
||||
button, values = window.Read()
|
||||
|
||||
print(button, values)
|
||||
|
||||
|
@ -307,8 +306,8 @@ This recipe shows just how easy it is to add a progress meter to your code.
|
|||
|
||||
|
||||
-----
|
||||
## Tabbed Form
|
||||
Tabbed forms are **easy** to make and use in PySimpleGUI. You simple may your layouts for each tab and then instead of `LayoutAndRead` you call `ShowTabbedForm`. Results are returned as a list of form results. Each tab acts like a single window.
|
||||
## Tabbed Window
|
||||
Tabbed Windows are **easy** to make and use in PySimpleGUI. You simple may your layouts for each tab and then instead of `LayoutAndRead` you call `ShowTabbedForm`. Results are returned as a list of form results. Each tab acts like a single window.
|
||||
|
||||
|
||||
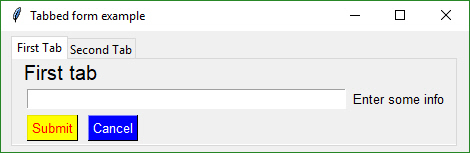
|
||||
|
@ -506,7 +505,7 @@ Perhaps you don't want all the statistics that the EasyProgressMeter provides an
|
|||
|
||||
## The One-Line GUI
|
||||
|
||||
For those of you into super-compact code, a complete customized GUI can be specified, shown, and received the results using a single line of Python code. The way this is done is to combine the call to `Window` and the call to `LayoutAndRead`. `Window` returns a `Window` object which has the `LayoutAndRead` method.
|
||||
For those of you into super-compact code, a complete customized GUI can be specified, shown, and received the results using a single line of Python code.
|
||||
|
||||
|
||||
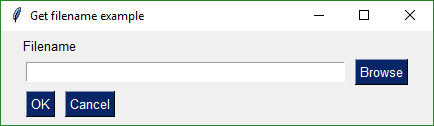
|
||||
|
@ -520,14 +519,14 @@ Instead of
|
|||
[sg.Input(), sg.FileBrowse()],
|
||||
[sg.OK(), sg.Cancel()]]
|
||||
|
||||
button, (number,) = sg.Window('Get filename example').LayoutAndRead(layout)
|
||||
button, (number,) = sg.Window('Get filename example').Layout(layout).Read()
|
||||
|
||||
you can write this line of code for the exact same result (OK, two lines with the import):
|
||||
|
||||
import PySimpleGUI as sg
|
||||
|
||||
button, (filename,) = sg.Window('Get filename example').LayoutAndRead(
|
||||
[[sg.Text('Filename')], [sg.Input(), sg.FileBrowse()], [sg.OK(), sg.Cancel()]])
|
||||
button, (filename,) = sg.Window('Get filename example').Layout(
|
||||
[[sg.Text('Filename')], [sg.Input(), sg.FileBrowse()], [sg.OK(), sg.Cancel()]]).Read()
|
||||
|
||||
--------------------
|
||||
## Multiple Columns
|
||||
|
@ -561,7 +560,7 @@ To make it easier to see the Column in the window, the Column background has bee
|
|||
|
||||
# Display the Window and get values
|
||||
|
||||
button, values = sg.Window('Compact 1-line Window with column').LayoutAndRead(layout)
|
||||
button, values = sg.Window('Compact 1-line Window with column').Layout(layout).Read()
|
||||
|
||||
sg.Popup(button, values, line_width=200)
|
||||
|
||||
|
|
Loading…
Reference in New Issue