Columns, one-line GUI
This commit is contained in:
parent
aa09dbdafb
commit
b039372347
1 changed files with 57 additions and 0 deletions
|
@ -535,4 +535,61 @@ Perhaps you don't want all the statistics that the EasyProgressMeter provides an
|
|||
|
||||
----
|
||||
|
||||
## The One-Line GUI
|
||||
|
||||
For those of you into super-compact code, a complete customized GUI can be specified, shown, and received the results using a single line of Python code. The way this is done is to combine the call to `FlexForm` and the call to `LayoutAndRead`. `FlexForm` returns a `FlexForm` object which has the `LayoutAndRead` method.
|
||||
|
||||
|
||||
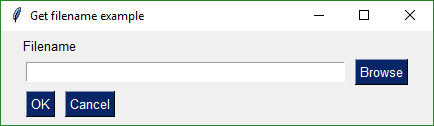
|
||||
|
||||
|
||||
Instead of
|
||||
|
||||
import PySimpleGUI as sg
|
||||
|
||||
layout = [[sg.Text('Filename')],
|
||||
[sg.Input(), sg.FileBrowse()],
|
||||
[sg.OK(), sg.Cancel()] ]
|
||||
|
||||
button, (number,) = sg.FlexForm('Get filename example').LayoutAndRead(layout)
|
||||
|
||||
you can write this line of code for the exact same result (OK, two lines with the import):
|
||||
|
||||
import PySimpleGUI as sg
|
||||
|
||||
button, (filename,) = sg.FlexForm('Get filename example'). LayoutAndRead([[sg.Text('Filename')], [sg.Input(), sg.FileBrowse()], [sg.OK(), sg.Cancel()] ])
|
||||
--------------------
|
||||
## Multiple Columns
|
||||
Starting in version 2.9 (not yet released but you can get from current GitHub) you can use the Column Element. A Column is required when you have a tall element to the left of smaller elements.
|
||||
|
||||
This example uses a Column. There is a Listbox on the left that is 3 rows high. To the right of it are 3 single rows of text and input. These 3 rows are in a Column Element.
|
||||
|
||||
To make it easier to see the Column in the window, the Column background has been shaded blue. The code is wordier than normal due to the blue shading. Each element in the column needs to have the color set to match blue background.
|
||||
|
||||
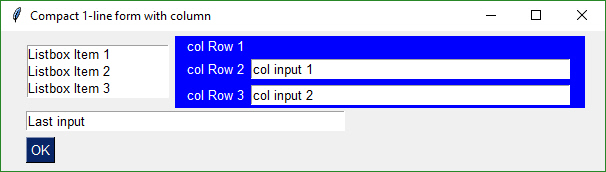
|
||||
|
||||
|
||||
import PySimpleGUI as sg
|
||||
|
||||
# Demo of how columns work
|
||||
# Form has on row 1 a vertical slider followed by a COLUMN with 7 rows
|
||||
# Prior to the Column element, this layout was not possible
|
||||
# Columns layouts look identical to form layouts, they are a list of lists of elements.
|
||||
|
||||
# sg.ChangeLookAndFeel('BlueMono')
|
||||
|
||||
# Column layout
|
||||
col = [[sg.Text('col Row 1', text_color='white', background_color='blue')],
|
||||
[sg.Text('col Row 2', text_color='white', background_color='blue'), sg.Input('col input 1')],
|
||||
[sg.Text('col Row 3', text_color='white', background_color='blue'), sg.Input('col input 2')]]
|
||||
|
||||
layout = [[sg.Listbox(values=('Listbox Item 1', 'Listbox Item 2', 'Listbox Item 3'), select_mode=sg.LISTBOX_SELECT_MODE_MULTIPLE, size=(20,3)), sg.Column(col, background_color='blue')],
|
||||
[sg.Input('Last input')],
|
||||
[sg.OK()]]
|
||||
|
||||
# Display the form and get values
|
||||
# If you're willing to not use the "context manager" design pattern, then it's possible
|
||||
# to collapse the form display and read down to a single line of code.
|
||||
button, values = sg.FlexForm('Compact 1-line form with column').LayoutAndRead(layout)
|
||||
|
||||
sg.MsgBox(button, values, line_width=200)
|
||||
|
|
Loading…
Add table
Add a link
Reference in a new issue