Merge pull request #1483 from PySimpleGUI/Dev-latest
Rename of PySimpleGUIdebugger to imwatchingyou
This commit is contained in:
commit
aff84bfafb
|
@ -1,5 +1,5 @@
|
||||||
import PySimpleGUI as sg
|
import PySimpleGUI as sg
|
||||||
import PySimpleGUIdebugger # STEP 1
|
import imwatchingyou # STEP 1
|
||||||
|
|
||||||
"""
|
"""
|
||||||
Demo program that shows you how to integrate the PySimpleGUI Debugger
|
Demo program that shows you how to integrate the PySimpleGUI Debugger
|
||||||
|
@ -7,11 +7,11 @@ import PySimpleGUIdebugger # STEP 1
|
||||||
In this example, the debugger is not started initiallly. You click the "Debug" button to launch it
|
In this example, the debugger is not started initiallly. You click the "Debug" button to launch it
|
||||||
There are THREE steps, and they are copy and pastes.
|
There are THREE steps, and they are copy and pastes.
|
||||||
1. At the top of your app to debug add
|
1. At the top of your app to debug add
|
||||||
import PySimpleGUIdebugger
|
import imwatchingyou
|
||||||
2. Initialize the debugger at the start of your program by calling:
|
2. Initialize the debugger at the start of your program by calling:
|
||||||
PySimpleGUIdebugger.initialize()
|
imwatchingyou.initialize()
|
||||||
3. At the top of your app's Event Loop add:
|
3. At the top of your app's Event Loop add:
|
||||||
PySimpleGUIdebugger.refresh(locals(), globals())
|
imwatchingyou.refresh(locals(), globals())
|
||||||
"""
|
"""
|
||||||
|
|
||||||
layout = [
|
layout = [
|
||||||
|
@ -32,14 +32,14 @@ debug_started = False
|
||||||
|
|
||||||
while True: # Your Event Loop
|
while True: # Your Event Loop
|
||||||
if debug_started:
|
if debug_started:
|
||||||
debug_started = PySimpleGUIdebugger.refresh(locals(), globals()) # STEP 3 - refresh debugger
|
debug_started = imwatchingyou.refresh(locals(), globals()) # STEP 3 - refresh debugger
|
||||||
event, values = window.Read(timeout=timeout)
|
event, values = window.Read(timeout=timeout)
|
||||||
if event in (None, 'Exit'):
|
if event in (None, 'Exit'):
|
||||||
break
|
break
|
||||||
elif event == 'Ok':
|
elif event == 'Ok':
|
||||||
print('You clicked Ok.... this is where print output goes')
|
print('You clicked Ok.... this is where print output goes')
|
||||||
elif event == 'Debug' and not debug_started:
|
elif event == 'Debug' and not debug_started:
|
||||||
PySimpleGUIdebugger.initialize() # STEP 2
|
imwatchingyou.initialize() # STEP 2
|
||||||
debug_started = True
|
debug_started = True
|
||||||
counter += 1
|
counter += 1
|
||||||
window.Element('_OUT_').Update(values['_IN_'])
|
window.Element('_OUT_').Update(values['_IN_'])
|
|
@ -1,6 +1,5 @@
|
||||||
import subprocess
|
|
||||||
import sys
|
|
||||||
import PySimpleGUI as sg
|
import PySimpleGUI as sg
|
||||||
|
import textwrap
|
||||||
|
|
||||||
|
|
||||||
"""
|
"""
|
||||||
|
@ -14,19 +13,21 @@ COLOR_SCHEME = 'LightGreen'
|
||||||
WIDTH_VARIABLES = 12
|
WIDTH_VARIABLES = 12
|
||||||
WIDTH_RESULTS = 36
|
WIDTH_RESULTS = 36
|
||||||
|
|
||||||
|
WIDTH_LOCALS = 80
|
||||||
|
|
||||||
# done purely for testing / show
|
# done purely for testing / show
|
||||||
def func(x=''):
|
def func(x=''):
|
||||||
return 'return value from func()={}'.format(x)
|
return 'return value from func()={}'.format(x)
|
||||||
|
|
||||||
|
|
||||||
def non_user_init():
|
def _non_user_init():
|
||||||
global watcher_window
|
global watcher_window
|
||||||
sg.ChangeLookAndFeel(COLOR_SCHEME)
|
sg.ChangeLookAndFeel(COLOR_SCHEME)
|
||||||
def InVar(key1, key2):
|
def InVar(key1, key2):
|
||||||
row1 = [sg.T(' '),
|
row1 = [sg.T(' '),
|
||||||
sg.I(key=key1, size=(WIDTH_VARIABLES,1)),
|
sg.I(key=key1, size=(WIDTH_VARIABLES,1)),
|
||||||
sg.T('',key=key1+'CHANGED_', size=(WIDTH_RESULTS,1)),sg.B('Detail', key=key1+'DETAIL_'),sg.B('Obj', key=key1+'OBJ_'), sg.T(' '),
|
sg.T('',key=key1+'CHANGED_', size=(WIDTH_RESULTS,1)),sg.B('Detail', key=key1+'DETAIL_'),sg.B('Obj', key=key1+'OBJ_'), sg.T(' '),
|
||||||
sg.T(' '), sg.I(key=key2, size=(WIDTH_VARIABLES, 1)), sg.T('',key=key2 + 'CHANGED_', size=(WIDTH_RESULTS, 1)), sg.B('Detail', key=key2+'DETAIL_'),sg.B('Obj', key=key1+'OBJ_')]
|
sg.T(' '), sg.I(key=key2, size=(WIDTH_VARIABLES, 1)), sg.T('',key=key2 + 'CHANGED_', size=(WIDTH_RESULTS, 1)), sg.B('Detail', key=key2+'DETAIL_'),sg.B('Obj', key=key2+'OBJ_')]
|
||||||
return row1
|
return row1
|
||||||
|
|
||||||
variables_frame = [ InVar('_VAR1_', '_VAR2_'),
|
variables_frame = [ InVar('_VAR1_', '_VAR2_'),
|
||||||
|
@ -38,7 +39,7 @@ def non_user_init():
|
||||||
|
|
||||||
layout = [ [sg.Frame('Variables or Expressions to Watch', variables_frame, )],
|
layout = [ [sg.Frame('Variables or Expressions to Watch', variables_frame, )],
|
||||||
[sg.Frame('REPL-Light', interactive_frame,)],
|
[sg.Frame('REPL-Light', interactive_frame,)],
|
||||||
[sg.Button('Exit')]]
|
[sg.Button('All Local Variables',key='_LOCALS_'), sg.Button('Exit')]]
|
||||||
|
|
||||||
window = sg.Window('PySimpleGUI Debugger', layout, icon=PSGDebugLogo).Finalize()
|
window = sg.Window('PySimpleGUI Debugger', layout, icon=PSGDebugLogo).Finalize()
|
||||||
window.Element('_VAR1_').SetFocus()
|
window.Element('_VAR1_').SetFocus()
|
||||||
|
@ -47,95 +48,124 @@ def non_user_init():
|
||||||
return window
|
return window
|
||||||
|
|
||||||
def _event_once(mylocals, myglobals):
|
def _event_once(mylocals, myglobals):
|
||||||
global myrc, watcher_window
|
global myrc, watcher_window, locals_window
|
||||||
if not watcher_window:
|
if not watcher_window:
|
||||||
return False
|
return False
|
||||||
_window = watcher_window
|
if locals_window:
|
||||||
_event, _values = _window.Read(timeout=1)
|
_locals_window_event_loop(mylocals)
|
||||||
if _event in (None, 'Exit'):
|
# _window = watcher_window
|
||||||
_window.Close()
|
event, values = watcher_window.Read(timeout=1)
|
||||||
|
if event in (None, 'Exit'):
|
||||||
|
watcher_window.Close()
|
||||||
watcher_window = None
|
watcher_window = None
|
||||||
return False
|
return False
|
||||||
cmd = _values['_INTERACTIVE_']
|
cmd = values['_INTERACTIVE_']
|
||||||
if _event == 'Run':
|
|
||||||
_runCommand(cmd=cmd, window=_window)
|
if event == 'Go':
|
||||||
elif _event == 'Go':
|
watcher_window.Element('_INTERACTIVE_').Update('')
|
||||||
_window.Element('_INTERACTIVE_').Update('')
|
watcher_window.Element('_OUTPUT_').Update(">>> {}\n".format(cmd), append=True, autoscroll=True)
|
||||||
_window.Element('_OUTPUT_').Update(">>> {}\n".format(cmd), append=True, autoscroll=True)
|
|
||||||
expression = """
|
expression = """
|
||||||
global myrc
|
global myrc
|
||||||
PySimpleGUIdebugger.PySimpleGUIdebugger.myrc = {} """.format(cmd)
|
imwatchingyou.imwatchingyou.myrc = {} """.format(cmd)
|
||||||
try:
|
try:
|
||||||
exec(expression, myglobals, mylocals)
|
exec(expression, myglobals, mylocals)
|
||||||
_window.Element('_OUTPUT_').Update('{}\n'.format(myrc),append=True, autoscroll=True)
|
watcher_window.Element('_OUTPUT_').Update('{}\n'.format(myrc),append=True, autoscroll=True)
|
||||||
|
|
||||||
except Exception as e:
|
except Exception as e:
|
||||||
_window.Element('_OUTPUT_').Update('Exception {}\n'.format(e),append=True, autoscroll=True)
|
watcher_window.Element('_OUTPUT_').Update('Exception {}\n'.format(e),append=True, autoscroll=True)
|
||||||
|
|
||||||
elif _event.endswith('_DETAIL_'):
|
elif event.endswith('_DETAIL_'):
|
||||||
expression = """
|
expression = """
|
||||||
global myrc
|
global myrc
|
||||||
PySimpleGUIdebugger.PySimpleGUIdebugger.myrc = {} """.format(_values['_VAR{}_'.format(_event[4])])
|
imwatchingyou.imwatchingyou.myrc = {} """.format(values['_VAR{}_'.format(event[4])])
|
||||||
try:
|
try:
|
||||||
exec(expression, myglobals, mylocals)
|
exec(expression, myglobals, mylocals)
|
||||||
sg.PopupScrolled(str(_values['_VAR{}_'.format(_event[4])]) + '\n' + str(myrc))
|
sg.PopupScrolled(str(values['_VAR{}_'.format(event[4])]) + '\n' + str(myrc))
|
||||||
except:
|
except:
|
||||||
print('Detail failed')
|
print('Detail failed')
|
||||||
elif _event.endswith('_OBJ_'):
|
elif event.endswith('_OBJ_'):
|
||||||
var = _values['_VAR{}_'.format(_event[4])]
|
var = values['_VAR{}_'.format(event[4])]
|
||||||
expression = """
|
expression = """
|
||||||
global myrc
|
global myrc
|
||||||
PySimpleGUIdebugger.PySimpleGUIdebugger.myrc = {} """.format(var)
|
imwatchingyou.imwatchingyou.myrc = {} """.format(var)
|
||||||
try:
|
try:
|
||||||
exec(expression, myglobals, mylocals)
|
exec(expression, myglobals, mylocals)
|
||||||
sg.PopupScrolled(sg.ObjToStringSingleObj(myrc))
|
sg.PopupScrolled(sg.ObjToStringSingleObj(myrc))
|
||||||
except:
|
except:
|
||||||
print('Detail failed')
|
print('Detail failed')
|
||||||
|
elif event == '_LOCALS_' and locals_window is None:
|
||||||
|
locals_window = _locals_window_initialize(mylocals)
|
||||||
|
|
||||||
# -------------------- Process the "watch list" ------------------
|
# -------------------- Process the "watch list" ------------------
|
||||||
for i in range(1, 7):
|
for i in range(1, 7):
|
||||||
key = '_VAR{}_'.format(i)
|
key = '_VAR{}_'.format(i)
|
||||||
out_key = '_VAR{}_CHANGED_'.format(i)
|
out_key = '_VAR{}_CHANGED_'.format(i)
|
||||||
myrc =''
|
myrc =''
|
||||||
if _window.Element(key):
|
if watcher_window.Element(key):
|
||||||
if _values[key]:
|
if values[key]:
|
||||||
expression = """
|
expression = """
|
||||||
global myrc
|
global myrc
|
||||||
PySimpleGUIdebugger.PySimpleGUIdebugger.myrc = {} """.format(_values[key])
|
imwatchingyou.imwatchingyou.myrc = {} """.format(values[key])
|
||||||
try:
|
try:
|
||||||
exec(expression, myglobals, mylocals)
|
exec(expression, myglobals, mylocals)
|
||||||
except Exception as e:
|
except Exception as e:
|
||||||
pass
|
pass
|
||||||
_window.Element(out_key).Update(myrc)
|
watcher_window.Element(out_key).Update(myrc)
|
||||||
else:
|
else:
|
||||||
_window.Element(out_key).Update('')
|
watcher_window.Element(out_key).Update('')
|
||||||
return True
|
return True
|
||||||
|
|
||||||
|
|
||||||
def _runCommand(cmd, timeout=None, window=None):
|
def _locals_window_event_loop(my_locals):
|
||||||
""" run shell command
|
global locals_window
|
||||||
@param cmd: command to execute
|
|
||||||
@param timeout: timeout for command execution
|
|
||||||
@param window: the PySimpleGUI window that the output is going to (needed to do refresh on)
|
|
||||||
@return: (return code from command, command output)
|
|
||||||
"""
|
|
||||||
p = subprocess.Popen(cmd, shell=True, stdout=subprocess.PIPE, stderr=subprocess.STDOUT)
|
|
||||||
output = ''
|
|
||||||
for line in p.stdout:
|
|
||||||
line = line.decode(errors='replace' if (sys.version_info) < (3, 5) else 'backslashreplace').rstrip()
|
|
||||||
output += line
|
|
||||||
print(line)
|
|
||||||
window.Refresh() if window else None # yes, a 1-line if, so shoot me
|
|
||||||
|
|
||||||
retval = p.wait(timeout)
|
output_text = ''
|
||||||
return (retval, output)
|
for key in my_locals:
|
||||||
|
value = "{} : {}".format(key, str(my_locals[key]))
|
||||||
|
wrapped = '\n'.join(textwrap.wrap(value,WIDTH_LOCALS))
|
||||||
|
output_text += '\n' + wrapped
|
||||||
|
|
||||||
|
locals_window.Element('_MULTI_').Update(output_text)
|
||||||
|
|
||||||
|
event, values = locals_window.Read(timeout=1)
|
||||||
|
|
||||||
|
if event in (None, 'Exit'):
|
||||||
|
locals_window.Close()
|
||||||
|
locals_window = None
|
||||||
|
|
||||||
|
|
||||||
|
def _locals_window_initialize(my_locals):
|
||||||
|
sg.ChangeLookAndFeel(COLOR_SCHEME)
|
||||||
|
|
||||||
|
output_text = ''
|
||||||
|
num_lines = 1
|
||||||
|
for key in my_locals:
|
||||||
|
value = "{} : {}".format(key, str(my_locals[key]))
|
||||||
|
num_lines += len(textwrap.wrap(value, WIDTH_LOCALS))
|
||||||
|
wrapped = '\n'.join(textwrap.wrap(value,WIDTH_LOCALS))
|
||||||
|
output_text += wrapped
|
||||||
|
|
||||||
|
layout = [[sg.Multiline(output_text, key='_MULTI_', size=(WIDTH_LOCALS, num_lines))],
|
||||||
|
[sg.Exit()]]
|
||||||
|
|
||||||
|
window = sg.Window('All Locals', layout, icon=PSGDebugLogo).Finalize()
|
||||||
|
sg.ChangeLookAndFeel('SystemDefault')
|
||||||
|
|
||||||
|
return window
|
||||||
|
|
||||||
def refresh(locals, globals):
|
def refresh(locals, globals):
|
||||||
return _event_once(locals, globals)
|
return _event_once(locals, globals)
|
||||||
|
|
||||||
def initialize():
|
def initialize():
|
||||||
global watcher_window
|
global watcher_window
|
||||||
watcher_window = non_user_init()
|
watcher_window = _non_user_init()
|
||||||
|
|
||||||
myrc = ''
|
myrc = ''
|
||||||
watcher_window = None
|
locals_window = watcher_window = None
|
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
if __name__ == '__main__':
|
||||||
|
initialize()
|
||||||
|
while True:
|
||||||
|
refresh(locals(), globals())
|
|
@ -1,12 +1,11 @@
|
||||||
|
|
||||||
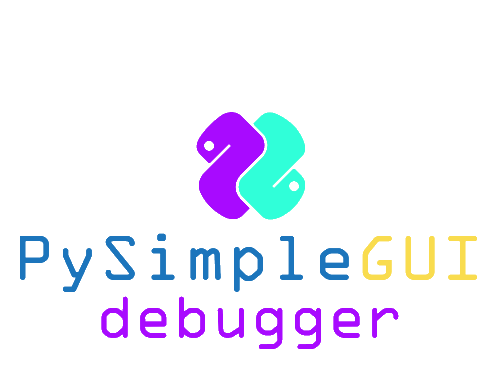
|
|
||||||
|
|
||||||
|
|
||||||

|

|
||||||
|
|
||||||
|
|
||||||
|
|
||||||
# PySimpleGUIdebugger
|
# imwatchingyou
|
||||||
|
|
||||||
A "debugger" that's based on PySimpleGUI. It was developed to help debug PySimpleGUI based programs, but it can be used to debug any program. The only requirement is that a `refresh()` function be called on a "periodic basis".
|
A "debugger" that's based on PySimpleGUI. It was developed to help debug PySimpleGUI based programs, but it can be used to debug any program. The only requirement is that a `refresh()` function be called on a "periodic basis".
|
||||||
|
|
||||||
|
@ -25,22 +24,22 @@ Check out this video as a guide. The user's window is the smaller one one top.
|
||||||
|
|
||||||
Installation is via pip:
|
Installation is via pip:
|
||||||
|
|
||||||
`pip install PySimpleGUIdebugger`
|
`pip install imwatchingyou`
|
||||||
|
|
||||||
or if you need to upgrade later:
|
or if you need to upgrade later:
|
||||||
|
|
||||||
`pip install --upgrade --no-cache-dir PySimpleGUIdebugger`
|
`pip install --upgrade --no-cache-dir imwatchingyou`
|
||||||
|
|
||||||
|
|
||||||
Note that you need to install the debugger using pip rather than downloading. There are some detailed technical reasons for this.
|
Note that you need to install the debugger using pip rather than downloading. There are some detailed technical reasons for this.
|
||||||
|
|
||||||
So, don't forget: __You must pip install PySimpleGUIdebugger in order to use it.__
|
So, don't forget: __You must pip install imwatchingyou in order to use it.__
|
||||||
|
|
||||||
|
|
||||||
|
|
||||||
## Integrating PySimpleGUIdebugger Into Your Application
|
## Integrating imwatchingyou Into Your Application
|
||||||
|
|
||||||
There are 3 lines of code to add to a PySimpleGUI program in order to make it debugger ready - The import, an initialization, once each time through the even loop.
|
There are 3 lines of code to add to a program in order to make it debugger ready - The import, an initialization, a refresh function called periodically.
|
||||||
|
|
||||||
Copy and paste these lines of code into your code just as you see them written. Don't get clever and rename anything. Don't do an "import as". Just copy the lines of code.
|
Copy and paste these lines of code into your code just as you see them written. Don't get clever and rename anything. Don't do an "import as". Just copy the lines of code.
|
||||||
|
|
||||||
|
@ -48,20 +47,20 @@ Here is an entire program including this integration code:
|
||||||
|
|
||||||
```python
|
```python
|
||||||
import PySimpleGUI as sg
|
import PySimpleGUI as sg
|
||||||
import PySimpleGUIdebugger
|
import imwatchingyou
|
||||||
"""
|
"""
|
||||||
Demo program that shows you how to integrate the PySimpleGUI Debugger
|
Demo program that shows you how to integrate the PySimpleGUI Debugger
|
||||||
into your program.
|
into your program.
|
||||||
There are THREE steps, and they are copy and pastes.
|
There are THREE steps, and they are copy and pastes.
|
||||||
1. At the top of your app to debug add
|
1. At the top of your app to debug add
|
||||||
import PySimpleGUIdebugger
|
import imwatchingyou
|
||||||
2. Initialize the debugger by calling:
|
2. Initialize the debugger by calling:
|
||||||
PySimpleGUIdebugger.initialize()
|
imwatchingyou.initialize()
|
||||||
2. At the top of your app's event loop add
|
2. At the top of your app's event loop add
|
||||||
PySimpleGUIdebugger.refresh(locals(), globals())
|
imwatchingyou.refresh(locals(), globals())
|
||||||
"""
|
"""
|
||||||
|
|
||||||
PySimpleGUIdebugger.initialize()
|
imwatchingyou.initialize()
|
||||||
|
|
||||||
layout = [
|
layout = [
|
||||||
[sg.T('A typical PSG application')],
|
[sg.T('A typical PSG application')],
|
||||||
|
@ -81,7 +80,7 @@ counter = 0
|
||||||
timeout = 100
|
timeout = 100
|
||||||
|
|
||||||
while True: # Event Loop
|
while True: # Event Loop
|
||||||
PySimpleGUIdebugger.refresh(locals(), globals()) # call the debugger to refresh the items being shown
|
imwatchingyou.refresh(locals(), globals()) # call the debugger to refresh the items being shown
|
||||||
event, values = window.Read(timeout=timeout)
|
event, values = window.Read(timeout=timeout)
|
||||||
if event in (None, 'Exit'):
|
if event in (None, 'Exit'):
|
||||||
break
|
break
|
||||||
|
@ -92,19 +91,19 @@ while True: # Event Loop
|
||||||
```
|
```
|
||||||
|
|
||||||
|
|
||||||
## Using PySimpleGUIdebugger
|
## Using imwatchingyou
|
||||||
|
|
||||||
To use the debugger in your code you will need to add TWO lines of code:
|
To use the debugger in your code you will need to add TWO lines of code:
|
||||||
The import at the top of your code:
|
The import at the top of your code:
|
||||||
`import PySimpleGUIdebugger`
|
`import imwatchingyou`
|
||||||
|
|
||||||
You need to "initialize" the PySimpleGUIdebugger package by calling near the top of your code. This is what creates the debugger window:
|
You need to "initialize" the imwatchingyou package by calling near the top of your code. This is what creates the debugger window:
|
||||||
`PySimpleGUIdebugger.initialize()`
|
`imwatchingyou.initialize()`
|
||||||
|
|
||||||
This "refresh" call that must be added to your event loop. Your `window.Read` call should have a timeout value so that it does not block. If you do not have a timeout value, the debugger will not update in realtime.
|
This "refresh" call that must be added to your event loop. Your `window.Read` call should have a timeout value so that it does not block. If you do not have a timeout value, the debugger will not update in realtime.
|
||||||
|
|
||||||
Add this line to the top of your event loop.
|
Add this line to the top of your event loop.
|
||||||
`PySimpleGUIdebugger.refresh(locals(), globals())`
|
`imwatchingyou.refresh(locals(), globals())`
|
||||||
|
|
||||||
### Using in "when needed" mode
|
### Using in "when needed" mode
|
||||||
|
|
||||||
|
@ -114,7 +113,7 @@ Here is the code, based on the code shown previously in this readme, that has a
|
||||||
|
|
||||||
```python
|
```python
|
||||||
import PySimpleGUI as sg
|
import PySimpleGUI as sg
|
||||||
import PySimpleGUIdebugger # STEP 1
|
import imwatchingyou # STEP 1
|
||||||
|
|
||||||
"""
|
"""
|
||||||
Demo program that shows you how to integrate the PySimpleGUI Debugger
|
Demo program that shows you how to integrate the PySimpleGUI Debugger
|
||||||
|
@ -122,11 +121,11 @@ import PySimpleGUIdebugger # STEP 1
|
||||||
In this example, the debugger is not started initiallly. You click the "Debug" button to launch it
|
In this example, the debugger is not started initiallly. You click the "Debug" button to launch it
|
||||||
There are THREE steps, and they are copy and pastes.
|
There are THREE steps, and they are copy and pastes.
|
||||||
1. At the top of your app to debug add
|
1. At the top of your app to debug add
|
||||||
import PySimpleGUIdebugger
|
import imwatchingyou
|
||||||
2. Initialize the debugger at the start of your program by calling:
|
2. Initialize the debugger at the start of your program by calling:
|
||||||
PySimpleGUIdebugger.initialize()
|
imwatchingyou.initialize()
|
||||||
3. At the top of your app's Event Loop add:
|
3. At the top of your app's Event Loop add:
|
||||||
PySimpleGUIdebugger.refresh(locals(), globals())
|
imwatchingyou.refresh(locals(), globals())
|
||||||
"""
|
"""
|
||||||
|
|
||||||
layout = [
|
layout = [
|
||||||
|
@ -147,14 +146,14 @@ debug_started = False
|
||||||
|
|
||||||
while True: # Your Event Loop
|
while True: # Your Event Loop
|
||||||
if debug_started:
|
if debug_started:
|
||||||
debug_started = PySimpleGUIdebugger.refresh(locals(), globals()) # STEP 3 - refresh debugger
|
debug_started = imwatchingyou.refresh(locals(), globals()) # STEP 3 - refresh debugger
|
||||||
event, values = window.Read(timeout=timeout)
|
event, values = window.Read(timeout=timeout)
|
||||||
if event in (None, 'Exit'):
|
if event in (None, 'Exit'):
|
||||||
break
|
break
|
||||||
elif event == 'Ok':
|
elif event == 'Ok':
|
||||||
print('You clicked Ok.... this is where print output goes')
|
print('You clicked Ok.... this is where print output goes')
|
||||||
elif event == 'Debug' and not debug_started:
|
elif event == 'Debug' and not debug_started:
|
||||||
PySimpleGUIdebugger.initialize() # STEP 2
|
imwatchingyou.initialize() # STEP 2
|
||||||
debug_started = True
|
debug_started = True
|
||||||
counter += 1
|
counter += 1
|
||||||
window.Element('_OUT_').Update(values['_IN_'])
|
window.Element('_OUT_').Update(values['_IN_'])
|
||||||
|
@ -176,13 +175,13 @@ You MUST run the debugger from the pip installed version. You cannot download t
|
||||||
|
|
||||||
## What's it good for, when should it be used??
|
## What's it good for, when should it be used??
|
||||||
|
|
||||||
Hell if I know. Maybe it's a terrible idea! Or, maybe it'll be really helpful, particularly in situations where you don't have many resources on the target system and perhaps you can't fit a debugger onto that system. PySimpleGUIdebugger provides another tool for your PySimpleGUI GUI Toolbox.
|
Hell if I know. Maybe it's a terrible idea! Or, maybe it'll be really helpful, particularly in situations where you don't have many resources on the target system and perhaps you can't fit a debugger onto that system. imwatchingyou provides another tool for your Python toolbox.
|
||||||
|
|
||||||
## The Future
|
## The Future
|
||||||
|
|
||||||
LOTS of plans for this debugger in the future. One of the immediate things I want to do is to integrate this into the PySimpleGUI.py file itself. To include the debugger with the SDK so that it doesn't have to be installed.
|
LOTS of plans for this debugger in the future. One of the immediate things I want to do is to integrate this into the PySimpleGUI.py file itself. To include the debugger with the SDK so that it doesn't have to be installed.
|
||||||
|
|
||||||
This will enable the use of a "hotkey" or other mechanism to "magically launch" your very own PySimpleGUI Debugger.
|
This will enable the use of a "hotkey" or other mechanism to "magically launch" the debugger.
|
||||||
|
|
||||||
I'll be adding a "Launch debugger" button for sure so that it's trivial for you to add this capability to your code.
|
I'll be adding a "Launch debugger" button for sure so that it's trivial for you to add this capability to your code.
|
||||||
|
|
Loading…
Reference in New Issue