Readme updates. Button color
Still working on completing the Readme. Changed the global button colors to white on black, the new signature for PySimpleGUI.
This commit is contained in:
parent
fe2a5b1dba
commit
a430c86ad2
|
@ -0,0 +1,10 @@
|
|||
import PySimpleGUI as sg
|
||||
|
||||
rc, number = sg.GetTextBox('Title goes here', 'Enter a number')
|
||||
if not rc:
|
||||
sg.MsgBoxError('You have cancelled')
|
||||
exit(0)
|
||||
|
||||
msg = '\n'.join([f'{i}' for i in range(0,int(number))])
|
||||
|
||||
sg.ScrolledTextBox(msg, Height=10)
|
|
@ -31,7 +31,8 @@ NICE_BUTTON_COLORS = ((GREENS[3], TANS[0]), ('#000000','#FFFFFF'),('#FFFFFF', '#
|
|||
(YELLOWS[0], GREENS[3]), (YELLOWS[0], BLUES[2]))
|
||||
# DEFAULT_BUTTON_COLOR = (YELLOWS[0], PURPLES[0]) # (Text, Background) or (Color "on", Color) as a way to remember
|
||||
# DEFAULT_BUTTON_COLOR = (GREENS[3], TANS[0]) # Foreground, Background (None, None) == System Default
|
||||
DEFAULT_BUTTON_COLOR = (YELLOWS[0], GREENS[4]) # Foreground, Background (None, None) == System Default
|
||||
# DEFAULT_BUTTON_COLOR = (YELLOWS[0], GREENS[4]) # Foreground, Background (None, None) == System Default
|
||||
DEFAULT_BUTTON_COLOR = ('white', 'black') # Foreground, Background (None, None) == System Default
|
||||
# DEFAULT_BUTTON_COLOR = (YELLOWS[0], PURPLES[2]) # Foreground, Background (None, None) == System Default
|
||||
DEFAULT_ERROR_BUTTON_COLOR =("#FFFFFF", "#FF0000")
|
||||
DEFAULT_CANCEL_BUTTON_COLOR = (GREENS[3], TANS[0])
|
||||
|
@ -1221,7 +1222,7 @@ def _GetNumLinesNeeded(text, max_line_width):
|
|||
# ===================================================#
|
||||
def MsgBox(*args, ButtonColor=None, ButtonType=MSG_BOX_OK, AutoClose=False, AutoCloseDuration=None, Icon=DEFAULT_WINDOW_ICON, LineWidth=MESSAGE_BOX_LINE_WIDTH, Font=None):
|
||||
'''
|
||||
|
||||
Show message box. Displays one line per user supplied argument. Takes any Type of variable to display.
|
||||
:param args:
|
||||
:param ButtonColor:
|
||||
:param ButtonType:
|
||||
|
@ -1232,10 +1233,13 @@ def MsgBox(*args, ButtonColor=None, ButtonType=MSG_BOX_OK, AutoClose=False, Aut
|
|||
:param Font:
|
||||
:return:
|
||||
'''
|
||||
if not args: return
|
||||
with FlexForm(args[0], AutoSizeText=True, ButtonColor=ButtonColor, AutoClose=AutoClose, AutoCloseDuration=AutoCloseDuration, Icon=Icon, Font=Font) as form:
|
||||
if not args:
|
||||
args_to_print = ['']
|
||||
else:
|
||||
args_to_print = args
|
||||
with FlexForm(args_to_print[0], AutoSizeText=True, ButtonColor=ButtonColor, AutoClose=AutoClose, AutoCloseDuration=AutoCloseDuration, Icon=Icon, Font=Font) as form:
|
||||
max_line_total, total_lines = 0,0
|
||||
for message in args:
|
||||
for message in args_to_print:
|
||||
# fancy code to check if string and convert if not is not need. Just always convert to string :-)
|
||||
# if not isinstance(message, str): message = str(message)
|
||||
message = str(message)
|
||||
|
@ -1273,6 +1277,15 @@ def MsgBox(*args, ButtonColor=None, ButtonType=MSG_BOX_OK, AutoClose=False, Aut
|
|||
# Lazy function. Same as calling MsgBox with parms #
|
||||
# ===================================================#
|
||||
def MsgBoxAutoClose(*args, ButtonColor=None,AutoClose=True, AutoCloseDuration=DEFAULT_AUTOCLOSE_TIME, Font=None):
|
||||
'''
|
||||
Display a standard MsgBox that will automatically close after a specified amount of time
|
||||
:param args:
|
||||
:param ButtonColor:
|
||||
:param AutoClose:
|
||||
:param AutoCloseDuration:
|
||||
:param Font:
|
||||
:return:
|
||||
'''
|
||||
MsgBox(*args, ButtonColor=ButtonColor, AutoClose=AutoClose, AutoCloseDuration=AutoCloseDuration, Font=Font)
|
||||
return
|
||||
|
||||
|
@ -1281,13 +1294,31 @@ def MsgBoxAutoClose(*args, ButtonColor=None,AutoClose=True, AutoCloseDuration=DE
|
|||
# Like MsgBox but presents RED BUTTONS #
|
||||
# ===================================================#
|
||||
def MsgBoxError(*args, ButtonColor=DEFAULT_ERROR_BUTTON_COLOR,AutoClose=False, AutoCloseDuration=None, Font=None):
|
||||
'''
|
||||
Display a MsgBox with a red button
|
||||
:param args:
|
||||
:param ButtonColor:
|
||||
:param AutoClose:
|
||||
:param AutoCloseDuration:
|
||||
:param Font:
|
||||
:return:
|
||||
'''
|
||||
MsgBox(*args, ButtonColor=ButtonColor, AutoClose=AutoClose, AutoCloseDuration=AutoCloseDuration, Font=Font)
|
||||
return
|
||||
|
||||
# ============================== MsgBoxCancel =====#
|
||||
# Like MsgBox but presents RED BUTTONS #
|
||||
# #
|
||||
# ===================================================#
|
||||
def MsgBoxCancel(*args,ButtonColor=DEFAULT_CANCEL_BUTTON_COLOR,AutoClose=False, AutoCloseDuration=None, Font=None):
|
||||
'''
|
||||
Display a MsgBox with a single "Cancel" button.
|
||||
:param args:
|
||||
:param ButtonColor:
|
||||
:param AutoClose:
|
||||
:param AutoCloseDuration:
|
||||
:param Font:
|
||||
:return:
|
||||
'''
|
||||
MsgBox(*args, ButtonType=MSG_BOX_CANCELLED, ButtonColor=ButtonColor, AutoClose=AutoClose, AutoCloseDuration=AutoCloseDuration, Font=Font)
|
||||
return
|
||||
|
||||
|
@ -1295,13 +1326,31 @@ def MsgBoxCancel(*args,ButtonColor=DEFAULT_CANCEL_BUTTON_COLOR,AutoClose=False,
|
|||
# Like MsgBox but only 1 button #
|
||||
# ===================================================#
|
||||
def MsgBoxOK(*args,ButtonColor=('white', 'black'),AutoClose=False, AutoCloseDuration=None, Font=None):
|
||||
'''
|
||||
Display a MsgBox with a single buttoned labelled "OK"
|
||||
:param args:
|
||||
:param ButtonColor:
|
||||
:param AutoClose:
|
||||
:param AutoCloseDuration:
|
||||
:param Font:
|
||||
:return:
|
||||
'''
|
||||
MsgBox(*args, ButtonType=MSG_BOX_OK, ButtonColor=ButtonColor, AutoClose=AutoClose, AutoCloseDuration=AutoCloseDuration, Font=Font)
|
||||
return
|
||||
|
||||
# ============================== MsgBoxCancel =====#
|
||||
# Like MsgBox but presents RED BUTTONS #
|
||||
# ============================== MsgBoxOKCancel ====#
|
||||
# Like MsgBox but presents OK and Cancel buttons #
|
||||
# ===================================================#
|
||||
def MsgBoxOKCancel(*args,ButtonColor=None,AutoClose=False, AutoCloseDuration=None, Font=None):
|
||||
'''
|
||||
Display MsgBox with 2 buttons, "OK" and "Cancel"
|
||||
:param args:
|
||||
:param ButtonColor:
|
||||
:param AutoClose:
|
||||
:param AutoCloseDuration:
|
||||
:param Font:
|
||||
:return:
|
||||
'''
|
||||
result = MsgBox(*args, ButtonType=MSG_BOX_OK_CANCEL, ButtonColor=ButtonColor, AutoClose=AutoClose, AutoCloseDuration=AutoCloseDuration, Font=Font)
|
||||
return result
|
||||
|
||||
|
@ -1310,6 +1359,15 @@ def MsgBoxOKCancel(*args,ButtonColor=None,AutoClose=False, AutoCloseDuration=Non
|
|||
# Returns True if Yes was pressed else False #
|
||||
# ===================================================#
|
||||
def MsgBoxYesNo(*args,ButtonColor=None,AutoClose=False, AutoCloseDuration=None, Font=None):
|
||||
'''
|
||||
Display MsgBox with 2 buttons, "Yes" and "No"
|
||||
:param args:
|
||||
:param ButtonColor:
|
||||
:param AutoClose:
|
||||
:param AutoCloseDuration:
|
||||
:param Font:
|
||||
:return:
|
||||
'''
|
||||
result = MsgBox(*args,ButtonType=MSG_BOX_YES_NO, ButtonColor=ButtonColor, AutoClose=AutoClose, AutoCloseDuration=AutoCloseDuration, Font=Font)
|
||||
return result
|
||||
|
||||
|
|
53
readme.md
53
readme.md
|
@ -21,21 +21,25 @@ You can add a GUI to your command line with a single line of code. With 3 or 4
|
|||
The customization is via the form/dialog box builder that enables users to experience all of the normal GUI widgets without having to write a lot of code.
|
||||
|
||||
|
||||
> Features of PySimpleGUI include:
|
||||
> Text
|
||||
> Single Line Input
|
||||
> Buttons including these types: File Browse Folder Browse Non-closing return Close form
|
||||
> Checkboxes
|
||||
> Radio Buttons
|
||||
> Icons
|
||||
> Multi-line Text Input
|
||||
> Scroll-able Output
|
||||
> Progress Bar
|
||||
> Async/Non-Blocking Windows
|
||||
> Tabbed forms
|
||||
> Persistent Windows
|
||||
> Redirect Python Output/Errors to scrolling Window
|
||||
> 'Higher level' APIs (e.g. MessageBox, YesNobox, ...)
|
||||
Features of PySimpleGUI include:
|
||||
Text
|
||||
Single Line Input
|
||||
Buttons including these types:
|
||||
File Browse
|
||||
Folder Browse
|
||||
Non-closing return
|
||||
Close form
|
||||
Checkboxes
|
||||
Radio Buttons
|
||||
Icons
|
||||
Multi-line Text Input
|
||||
Scroll-able Output
|
||||
Progress Bar
|
||||
Async/Non-Blocking Windows
|
||||
Tabbed forms
|
||||
Persistent Windows
|
||||
Redirect Python Output/Errors to scrolling Window
|
||||
'Higher level' APIs (e.g. MessageBox, YesNobox, ...)
|
||||
|
||||
|
||||
An example of many widgets used on a single form. A little further down you'll find the FIFTEEN lines of code required to create this complex form.
|
||||
|
@ -199,6 +203,19 @@ We all have loops in our code. 'Isn't it joyful waiting, watching a counter scr
|
|||
Scale=(None, None),
|
||||
BorderWidth=DEFAULT_PROGRESS_BAR_BORDER_WIDTH):
|
||||
|
||||
Here's the one-line Progress Meter in action!
|
||||
|
||||
for i in range(1,10000):
|
||||
SG.EasyProgressMeter('My Meter', i+1, 1000, 'Optional message')
|
||||
|
||||
That line of code resulted in this window popping up and updating.
|
||||
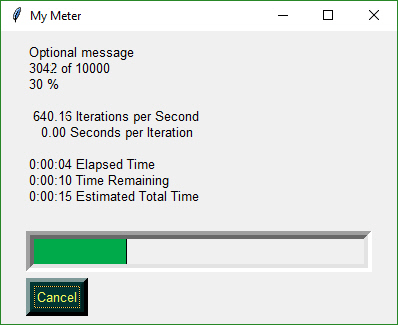
|
||||
|
||||
A meter AND fun statistics to watch while your machine grinds away, all for the price of 1 line of code.
|
||||
With a little trickery you can provide a way to break out of your loop using the Progress Meter form. The cancel button results in a `False` return value from `EasyProgressMeter`. It normally returns `True`.
|
||||
|
||||
if not SG.EasyProgressMeter('My Meter', i+1, 10000, 'Optional message'): break
|
||||
|
||||
# Custom Form API Calls
|
||||
|
||||
This is the FUN part of the programming of this GUI. In order to really get the most out of the API, you should be using an IDE that supports auto complete or will show you the definition of the function. This will make customizing go smoother.
|
||||
|
@ -479,6 +496,7 @@ A MikeTheWatchGuy production... entirely responsible for this code
|
|||
## Versioning
|
||||
|
||||
1.0.9 - July 10, 2018 - Initial Release
|
||||
1.0.21 - July 13, 2018 - Readme updates
|
||||
|
||||
## Code Condition
|
||||
> Make it run
|
||||
|
@ -497,8 +515,3 @@ This project is licensed under the MIT License - see the [LICENSE.md](LICENSE.md
|
|||
## Acknowledgments
|
||||
|
||||
* Jorj McKie was the motivator behind the entire project. His wxsimpleGUI concepts sparked PySimpleGUI into existence
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
|
Loading…
Reference in New Issue