Updates for Version 2.8
This commit is contained in:
parent
4bb89514cb
commit
644c441fbe
|
@ -2,15 +2,20 @@
|
|||
|
||||
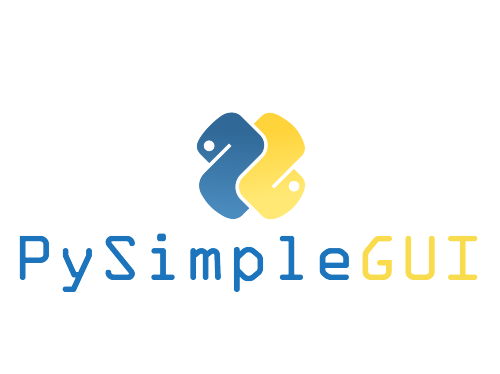
|
||||
|
||||
[](http://pepy.tech/project/pysimplegui) since Jul 11, 2018
|
||||
[](http://pepy.tech/project/pysimplegui) since Jul 11, 2018
|
||||
|
||||

|
||||
|
||||
|
||||
# PySimpleGUI
|
||||
(Ver 2.7)
|
||||
(Ver 2.8)
|
||||
[Formatted ReadTheDocs Version of this Readme](http://pysimplegui.readthedocs.io/)
|
||||
|
||||
Super-simple GUI to grasp... Powerfully customizable.
|
||||
|
||||
Note - *Python3* is required to run PySimpleGUI. It takes advantage of some Python3 features that do not translate well into Python2.
|
||||
Note - ***Python3*** is required to run PySimpleGUI. It takes advantage of some Python3 features that do not translate well into Python2.
|
||||
|
||||
Looking to take your Python code from the world of command lines and into the convenience of a GUI? Have a Raspberry Pi with a touchscreen that's going to waste because you don't have the time to learn a GUI SDK? Look no further, you've found your GUI package.
|
||||
Looking to take your Python code from the world of command lines and into the convenience of a GUI? Have a Raspberry **Pi** with a touchscreen that's going to waste because you don't have the time to learn a GUI SDK? Into Machine Learning and are sick of the command line? Look no further, **you've found your GUI package**.
|
||||
|
||||
import PySimpleGUI as sg
|
||||
|
||||
|
@ -21,9 +26,11 @@ Looking to take your Python code from the world of command lines and into the co
|
|||
|
||||
Build beautiful customized forms that fit your specific problem. Let PySimpleGUI solve your GUI problem while you solve the real problems. Do you really want to plod through the mountains of code required to program tkinter?
|
||||
|
||||
PySimpleGUI wraps tkinter so that you get all the same widgets as you would tkinter, but you interact with them in a **much** more friendly way.
|
||||
|
||||
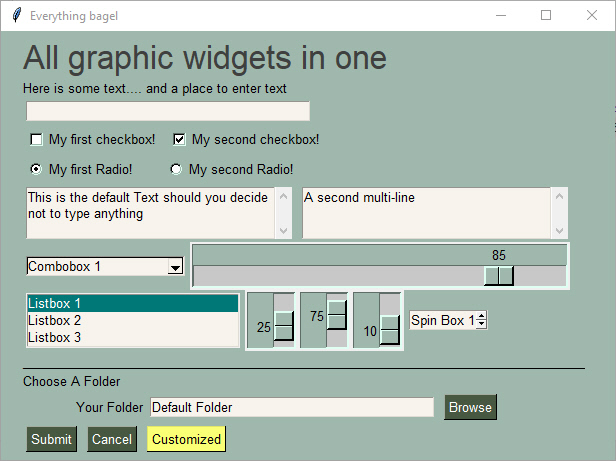
|
||||
|
||||
Perhaps you're looking for a way to interact with your Raspberry Pi in a more friendly way. The is the same form as above, except shown on a Pi.
|
||||
Perhaps you're looking for a way to interact with your **Raspberry Pi** in a more friendly way. The is the same form as above, except shown on a Pi.
|
||||
|
||||
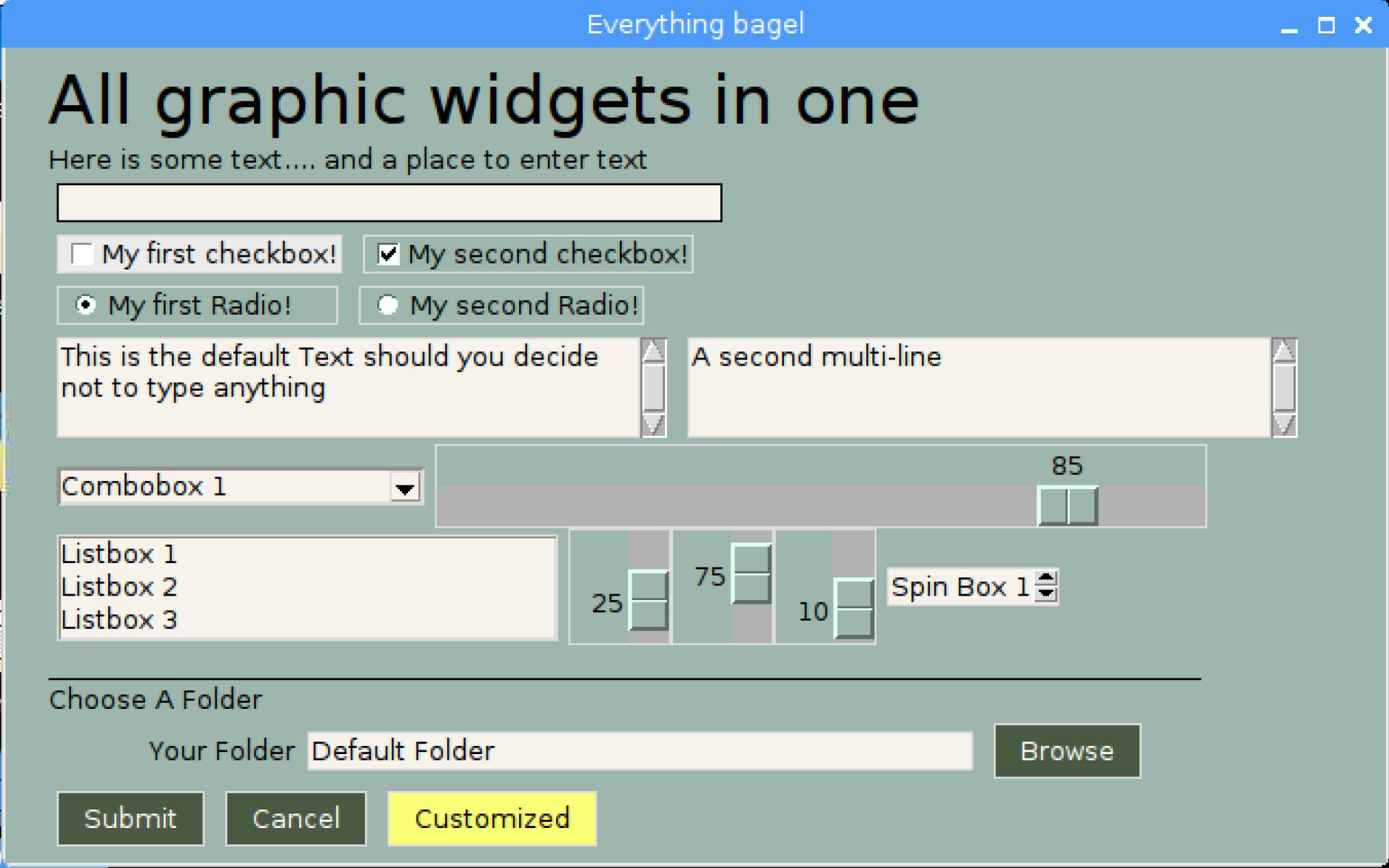
|
||||
|
||||
|
@ -40,7 +47,7 @@ You can build an async media player GUI with custom buttons in 30 lines of code.
|
|||
|
||||
I was frustrated by having to deal with the dos prompt when I had a powerful Windows machine right in front of me. Why is it SO difficult to do even the simplest of input/output to a window in Python??
|
||||
|
||||
There are a number of 'easy to use' Python GUIs, but they're **very** limiting. PySimpleGUI takes the best of packages like `EasyGUI`and `WxSimpleGUI` , both really handy but limited. The primary difference between these and PySimpleGUI is that in addition to getting the simple Message Boxes you also get the ability to make your own forms that are highly customizeable. Don't like the standard Message Box? Then make your own!
|
||||
There are a number of 'easy to use' Python GUIs, but they're **very** limiting. PySimpleGUI takes the best of packages like `EasyGUI`and `WxSimpleGUI` , both really handy but limited. The primary difference between these and `PySimpleGUI` is that in addition to getting the simple Message Boxes you also get the ability to **make your own forms** that are highly customizeable. Don't like the standard Message Box? Then make your own!
|
||||
|
||||
Every call has optional parameters so that you can change the look and feel. Don't like the button color? It's easy to change by adding a button_color parameter to your widget.
|
||||
|
||||
|
@ -73,9 +80,12 @@ The `PySimpleGUI` package is focused on the ***developer***. How can the desire
|
|||
Persistent Windows
|
||||
Redirect Python Output/Errors to scrolling window
|
||||
'Higher level' APIs (e.g. MessageBox, YesNobox, ...)
|
||||
Single-Line-Of-Coide Proress Bar & Debug Print
|
||||
Single-Line-Of-Code Proress Bar & Debug Print
|
||||
Complete control of colors, look and feel
|
||||
Button images
|
||||
Return values as dictionary
|
||||
Set focus
|
||||
Bind return key to buttons
|
||||
|
||||
|
||||
An example of many widgets used on a single form. A little further down you'll find the TWENTY lines of code required to create this complex form. Try it if you don't believe it. Start Python, copy and paste the code below into the >>> prompt and hit enter. This will pop up...
|
||||
|
@ -480,6 +490,10 @@ This is the code that **displays** the form, collects the information and return
|
|||
|
||||
## Return values
|
||||
|
||||
As of version 2.8 there are 2 forms of return values, list and dictionary.
|
||||
### Return values as a list
|
||||
By default return values are a list of values, one entry for each input field.
|
||||
|
||||
Return information from FlexForm, SG's primary form builder interface, is in this format:
|
||||
|
||||
button, (value1, value2, ...)
|
||||
|
@ -498,14 +512,41 @@ If you have a SINGLE value being returned, it is written this way:
|
|||
button, (value1,) = form.LayoutAndRead(form_rows)
|
||||
|
||||
|
||||
Another way of parsing the return values is to store the list of values into a variable representing the list of values.
|
||||
Another way of parsing the return values is to store the list of values into a variable representing the list of values and then index each individual value. This is not the preferred way of doing it.
|
||||
|
||||
button, value_list = form.LayoutAndRead(form_rows)
|
||||
value1 = value_list[0]
|
||||
value2 = value_list[1]
|
||||
...
|
||||
|
||||
### Return values as a dictionary
|
||||
|
||||
If you wish to receive the return values as a dictionary rather than a simple list, then you'll have to one thing...
|
||||
* Mark each input element you wish to be in the dictionary with the keyword `key`.
|
||||
|
||||
If **any** element in the form has a `key`, then **all** of the return values are returned via a dictionary. If some elements do not have a key, then they are numbered starting at zero.
|
||||
|
||||
This sample program demonstrates these 2 steps as well as how to address the return values (e.g. `values['name']`)
|
||||
|
||||
|
||||
import PySimpleGUI as sg
|
||||
form = sg.FlexForm('Simple data entry form')
|
||||
layout = [
|
||||
[sg.Text('Please enter your Name, Address, Phone')],
|
||||
[sg.Text('Name', size=(15, 1)), sg.InputText('1')],
|
||||
[sg.Text('Address', size=(15, 1)), sg.InputText('2', key='address')],
|
||||
[sg.Text('Phone', size=(15, 1)), sg.InputText('3', key='phone')],
|
||||
[sg.Submit(), sg.Cancel()]
|
||||
]
|
||||
|
||||
button, values = form.LayoutAndRead(layout)
|
||||
|
||||
sg.MsgBox(button, values, values[0], values['address'], values['phone'])
|
||||
|
||||
|
||||
---
|
||||
|
||||
|
||||
## All Widgets / Elements
|
||||
This code utilizes as many of the elements in one form as possible.
|
||||
|
||||
|
@ -588,7 +629,7 @@ Parameter Descriptions. You will find these same parameters specified for each
|
|||
auto_size_text - Bool. True if elements should size themselves according to contents
|
||||
auto_size_buttons - Bool. True if button elements should size themselves according to their text label
|
||||
scale - Set size of element to be a multiple of the Element size
|
||||
location - Location to place window in pixels
|
||||
location - (x,y) Location to place window in pixels
|
||||
button_color - Default color for buttons (foreground, background). Can be text or hex
|
||||
progress_bar_color - Foreground and background colors for progress bars
|
||||
is_tabbed_form - Bool. If True then form is a tabbed form
|
||||
|
@ -598,6 +639,9 @@ Parameter Descriptions. You will find these same parameters specified for each
|
|||
icon - .ICO file that will appear on the Task Bar and end of Title Bar
|
||||
|
||||
|
||||
#### Window Location
|
||||
PySimpleGUI computes the exact center of your window and centers the window on the screen. If you want to locate your window elsewhere, such as the system default of (0,0), if you have 2 ways of doing this. The first is when the form is created. Use the `location` parameter to set where the window. The second way of doing this is to use the `SetOptions` call which will set the default window location for all windows in the future.
|
||||
|
||||
#### Sizes
|
||||
Note several variables that deal with "size". Element sizes are measured in characters. A Text Element with a size of 20,1 has a size of 20 characters wide by 1 character tall.
|
||||
|
||||
|
@ -892,7 +936,7 @@ Checkbox elements are like Radio Button elements. They return a bool indicating
|
|||
.
|
||||
|
||||
text - Text to display next to checkbox
|
||||
default- Bool. Initial state
|
||||
default- Bool + None. Initial state. True = Checked, False = unchecked, None = Not available (grayed out)
|
||||
scale - Amount to scale size of element
|
||||
size - (width, height) size of element in characters
|
||||
auto_size_text- Bool. True if should size width to fit text
|
||||
|
@ -1158,11 +1202,13 @@ Recall that values is a list as well. Multiple tabs in the form would return li
|
|||
Starting in version 2.5 you can change the background colors for the window and the Elements.
|
||||
|
||||
Your forms can go from this:
|
||||
|
||||
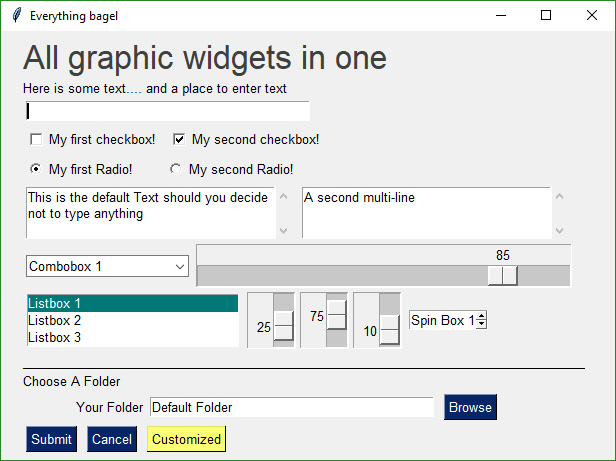
|
||||
|
||||
|
||||
to this... with one function call...
|
||||
|
||||
|
||||
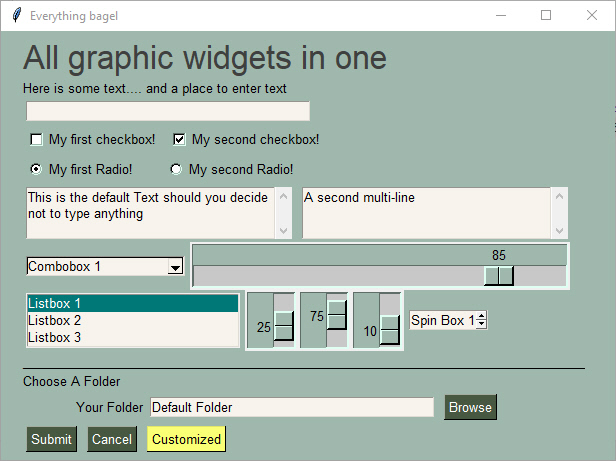
|
||||
|
||||
|
||||
|
@ -1418,6 +1464,7 @@ A MikeTheWatchGuy production... entirely responsible for this code.... unless it
|
|||
| 2.5.0 | July 26, 2018 - Colors. Listbox scrollbar. tkinter Progress Bar instead of homegrown.
|
||||
| 2.6.0 | July 27, 2018 - auto_size_button setting. License changed to LGPL 3+
|
||||
| 2.7.0 | July 30, 2018 - realtime buttons, window_location default setting
|
||||
| 2.8.0 | Aug 9, 2018 - New None default option for Checkbox element, text color option for all elements, return values as a dictionary, setting focus, binding return key
|
||||
|
||||
|
||||
### Release Notes
|
||||
|
@ -1432,6 +1479,7 @@ New debug printing capability. `sg.Print`
|
|||
Listboxes are still without scrollwheels. The mouse can drag to see more items. The mouse scrollwheel will also scroll the list and will `page up` and `page down` keys.
|
||||
|
||||
2.7 Is the "feature complete" release. Pretty much all features are done and in the code
|
||||
2.8 More text color controls. The caller has more control over things like the focus and what buttons should be clicked when enter key is pressed. Return values as a dictionary! (NICE addition)
|
||||
|
||||
|
||||
### Upcoming
|
||||
|
@ -1497,7 +1545,7 @@ Here are the steps to run that application
|
|||
|
||||
The pip command is all there is to the setup.
|
||||
|
||||
The way HowDoI works is that it uses your search term to look through stack overflow posts. It finds the best answer, gets the code from the answer, and presents it as a response. It gives you the correct answer OFTEN. It's a miracle that it work SO well.
|
||||
The way HowDoI works is that it uses your search term to look through stack overflow posts. It finds the best answer, gets the code from the answer, and presents it as a response. It gives you the correct answer OFTEN. It's a miracle that it work SO well.
|
||||
For Python questions, I simply start my query with 'Python'. Let's say you forgot how to reverse a list in Python. When you run HowDoI and ask this question, this is what you'll see.
|
||||
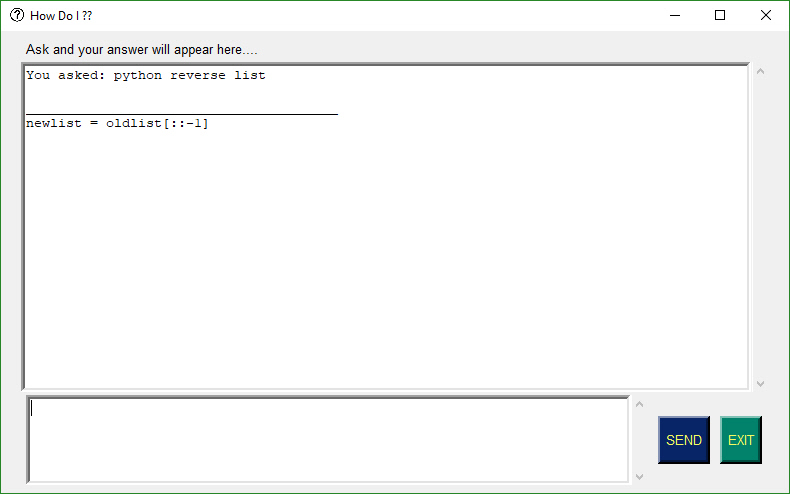
|
||||
|
||||
|
|
30
readme.md
30
readme.md
|
@ -8,13 +8,14 @@
|
|||
|
||||
|
||||
# PySimpleGUI
|
||||
(Ver 2.7)
|
||||
(Ver 2.8)
|
||||
[Formatted ReadTheDocs Version of this Readme](http://pysimplegui.readthedocs.io/)
|
||||
|
||||
Super-simple GUI to grasp... Powerfully customizable.
|
||||
|
||||
Note - ***Python3*** is required to run PySimpleGUI. It takes advantage of some Python3 features that do not translate well into Python2.
|
||||
|
||||
Looking to take your Python code from the world of command lines and into the convenience of a GUI? Have a Raspberry **Pi** with a touchscreen that's going to waste because you don't have the time to learn a GUI SDK? Look no further, **you've found your GUI package**.
|
||||
Looking to take your Python code from the world of command lines and into the convenience of a GUI? Have a Raspberry **Pi** with a touchscreen that's going to waste because you don't have the time to learn a GUI SDK? Into Machine Learning and are sick of the command line? Look no further, **you've found your GUI package**.
|
||||
|
||||
import PySimpleGUI as sg
|
||||
|
||||
|
@ -25,6 +26,8 @@ Looking to take your Python code from the world of command lines and into the co
|
|||
|
||||
Build beautiful customized forms that fit your specific problem. Let PySimpleGUI solve your GUI problem while you solve the real problems. Do you really want to plod through the mountains of code required to program tkinter?
|
||||
|
||||
PySimpleGUI wraps tkinter so that you get all the same widgets as you would tkinter, but you interact with them in a **much** more friendly way.
|
||||
|
||||
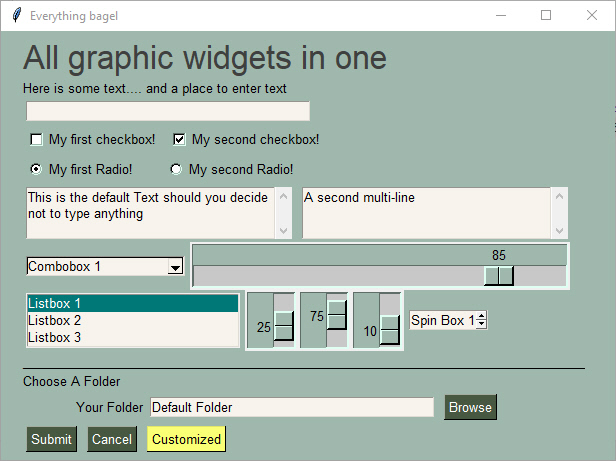
|
||||
|
||||
Perhaps you're looking for a way to interact with your **Raspberry Pi** in a more friendly way. The is the same form as above, except shown on a Pi.
|
||||
|
@ -80,6 +83,9 @@ The `PySimpleGUI` package is focused on the ***developer***. How can the desire
|
|||
Single-Line-Of-Code Proress Bar & Debug Print
|
||||
Complete control of colors, look and feel
|
||||
Button images
|
||||
Return values as dictionary
|
||||
Set focus
|
||||
Bind return key to buttons
|
||||
|
||||
|
||||
An example of many widgets used on a single form. A little further down you'll find the TWENTY lines of code required to create this complex form. Try it if you don't believe it. Start Python, copy and paste the code below into the >>> prompt and hit enter. This will pop up...
|
||||
|
@ -515,18 +521,19 @@ If you have a SINGLE value being returned, it is written this way:
|
|||
|
||||
### Return values as a dictionary
|
||||
|
||||
If you wish to receive the return values as a dictionary rather than a simple list, then you'll have to do 2 things:
|
||||
1. Indicate in the form creation that the return should be a dictionary by setting `use_dictionary = True`
|
||||
2. Mark each input element you wish to be in the dictionary with the keyword `key`.
|
||||
If you wish to receive the return values as a dictionary rather than a simple list, then you'll have to one thing...
|
||||
* Mark each input element you wish to be in the dictionary with the keyword `key`.
|
||||
|
||||
If **any** element in the form has a `key`, then **all** of the return values are returned via a dictionary. If some elements do not have a key, then they are numbered starting at zero.
|
||||
|
||||
This sample program demonstrates these 2 steps as well as how to address the return values (e.g. `values['name']`)
|
||||
|
||||
|
||||
import PySimpleGUI as sg
|
||||
form = sg.FlexForm('Simple data entry form', use_dictionary=True)
|
||||
form = sg.FlexForm('Simple data entry form')
|
||||
layout = [
|
||||
[sg.Text('Please enter your Name, Address, Phone')],
|
||||
[sg.Text('Name', size=(15, 1)), sg.InputText('1', key='name')],
|
||||
[sg.Text('Name', size=(15, 1)), sg.InputText('1')],
|
||||
[sg.Text('Address', size=(15, 1)), sg.InputText('2', key='address')],
|
||||
[sg.Text('Phone', size=(15, 1)), sg.InputText('3', key='phone')],
|
||||
[sg.Submit(), sg.Cancel()]
|
||||
|
@ -534,7 +541,7 @@ This sample program demonstrates these 2 steps as well as how to address the ret
|
|||
|
||||
button, values = form.LayoutAndRead(layout)
|
||||
|
||||
sg.MsgBox(button, values, values['name'], values['address'], values['phone'])
|
||||
sg.MsgBox(button, values, values[0], values['address'], values['phone'])
|
||||
|
||||
|
||||
---
|
||||
|
@ -1195,11 +1202,13 @@ Recall that values is a list as well. Multiple tabs in the form would return li
|
|||
Starting in version 2.5 you can change the background colors for the window and the Elements.
|
||||
|
||||
Your forms can go from this:
|
||||
|
||||
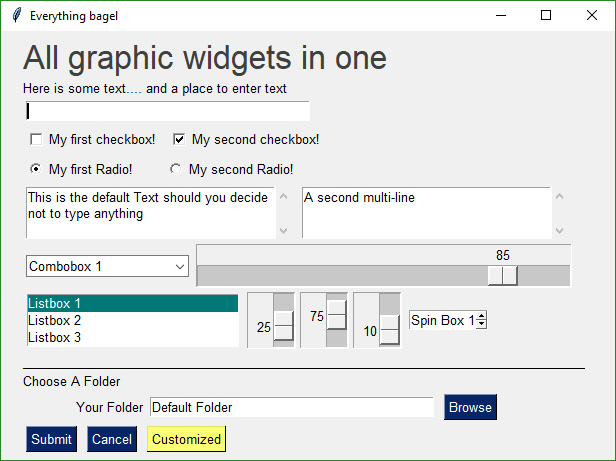
|
||||
|
||||
|
||||
to this... with one function call...
|
||||
|
||||
|
||||
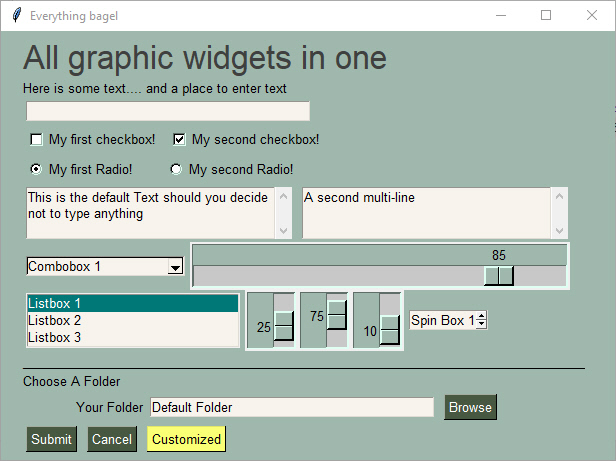
|
||||
|
||||
|
||||
|
@ -1455,7 +1464,7 @@ A MikeTheWatchGuy production... entirely responsible for this code.... unless it
|
|||
| 2.5.0 | July 26, 2018 - Colors. Listbox scrollbar. tkinter Progress Bar instead of homegrown.
|
||||
| 2.6.0 | July 27, 2018 - auto_size_button setting. License changed to LGPL 3+
|
||||
| 2.7.0 | July 30, 2018 - realtime buttons, window_location default setting
|
||||
| 2.8.0 | Aug xx, 2018 - PLANNED - New None default option for Checkbox element, text color option for all elements, return values as a dictionary
|
||||
| 2.8.0 | Aug 9, 2018 - New None default option for Checkbox element, text color option for all elements, return values as a dictionary, setting focus, binding return key
|
||||
|
||||
|
||||
### Release Notes
|
||||
|
@ -1470,6 +1479,7 @@ New debug printing capability. `sg.Print`
|
|||
Listboxes are still without scrollwheels. The mouse can drag to see more items. The mouse scrollwheel will also scroll the list and will `page up` and `page down` keys.
|
||||
|
||||
2.7 Is the "feature complete" release. Pretty much all features are done and in the code
|
||||
2.8 More text color controls. The caller has more control over things like the focus and what buttons should be clicked when enter key is pressed. Return values as a dictionary! (NICE addition)
|
||||
|
||||
|
||||
### Upcoming
|
||||
|
@ -1535,7 +1545,7 @@ Here are the steps to run that application
|
|||
|
||||
The pip command is all there is to the setup.
|
||||
|
||||
The way HowDoI works is that it uses your search term to look through stack overflow posts. It finds the best answer, gets the code from the answer, and presents it as a response. It gives you the correct answer OFTEN. It's a miracle that it work SO well.
|
||||
The way HowDoI works is that it uses your search term to look through stack overflow posts. It finds the best answer, gets the code from the answer, and presents it as a response. It gives you the correct answer OFTEN. It's a miracle that it work SO well.
|
||||
For Python questions, I simply start my query with 'Python'. Let's say you forgot how to reverse a list in Python. When you run HowDoI and ask this question, this is what you'll see.
|
||||
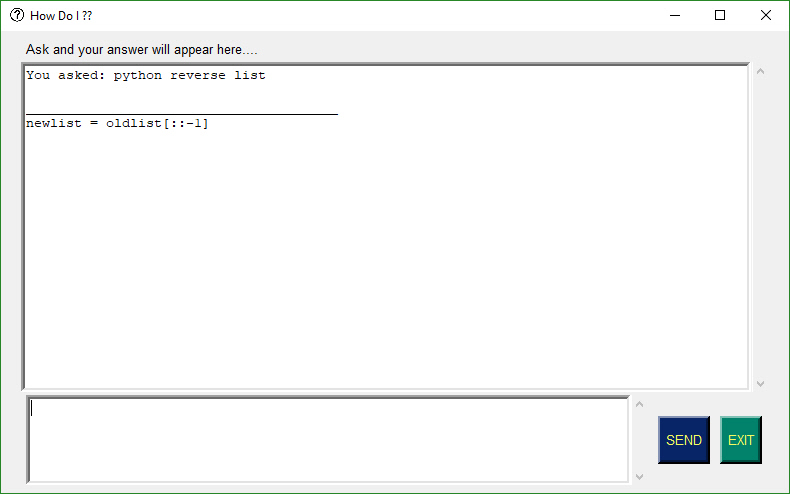
|
||||
|
||||
|
|
Loading…
Reference in New Issue