Readme update... note number of changes looks huge for some reason. Could be PyCharm plugin
This commit is contained in:
parent
0645b49624
commit
5c6aad4271
|
@ -189,6 +189,8 @@ While simple to use, PySimpleGUI has significant depth to be explored by more ad
|
||||||
Menus with ALT-hotkey
|
Menus with ALT-hotkey
|
||||||
Tooltips
|
Tooltips
|
||||||
Clickable links
|
Clickable links
|
||||||
|
Transparent windows
|
||||||
|
Movable windows
|
||||||
No async programming required (no callbacks to worry about)
|
No async programming required (no callbacks to worry about)
|
||||||
|
|
||||||
|
|
||||||
|
@ -1316,6 +1318,9 @@ Read the Window's input values and button clicks but without blocking. It will
|
||||||
#### Refresh()
|
#### Refresh()
|
||||||
Cause changes to the window to be displayed on the screen. Normally not needed unless the changes are immediately required or if it's going to be a while before another call to Read.
|
Cause changes to the window to be displayed on the screen. Normally not needed unless the changes are immediately required or if it's going to be a while before another call to Read.
|
||||||
|
|
||||||
|
#### SetIcon(icon)
|
||||||
|
Sets the window's icon that will be shown on the titlebar.
|
||||||
|
|
||||||
#### Fill(values_dict)
|
#### Fill(values_dict)
|
||||||
Populates the windows fields with the values shown in the dictionary.
|
Populates the windows fields with the values shown in the dictionary.
|
||||||
|
|
||||||
|
@ -1335,6 +1340,12 @@ Fills in a window's fields based on previously saved file
|
||||||
|
|
||||||
Returns the size (w,h) of the screen in pixels
|
Returns the size (w,h) of the screen in pixels
|
||||||
|
|
||||||
|
#### Move(x, y)
|
||||||
|
Move window to (x,y) position on the screen
|
||||||
|
|
||||||
|
#### Minimize()
|
||||||
|
Sends the window to the taskbar
|
||||||
|
|
||||||
#### CloseNonBlocking()
|
#### CloseNonBlocking()
|
||||||
|
|
||||||
Closes a non-blocking window
|
Closes a non-blocking window
|
||||||
|
@ -1363,6 +1374,10 @@ Makes a window disappear while leaving the icon on the taskbar
|
||||||
|
|
||||||
Makes a window reappear that was previously made to disappear using Disappear()
|
Makes a window reappear that was previously made to disappear using Disappear()
|
||||||
|
|
||||||
|
#### SetAlpha(alpha)
|
||||||
|
|
||||||
|
Sets the window's transparency. 0 is completely transparent. 1 is fully visible, normal . Can also use the property Window.AlphaChannel instead of method function call
|
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
@ -1377,7 +1392,7 @@ Makes a window reappear that was previously made to disappear using Disappear()
|
||||||
Calendar picker
|
Calendar picker
|
||||||
Date Chooser
|
Date Chooser
|
||||||
Read window
|
Read window
|
||||||
Close window
|
Close window ("Button" & all shortcut buttons)
|
||||||
Realtime
|
Realtime
|
||||||
Checkboxes
|
Checkboxes
|
||||||
Radio Buttons
|
Radio Buttons
|
||||||
|
@ -1395,11 +1410,7 @@ Makes a window reappear that was previously made to disappear using Disappear()
|
||||||
Table
|
Table
|
||||||
Tree
|
Tree
|
||||||
Tab, TabGroup
|
Tab, TabGroup
|
||||||
Async/Non-Blocking Windows
|
|
||||||
Tabbed windows
|
|
||||||
Persistent Windows
|
|
||||||
Redirect Python Output/Errors to scrolling Window
|
|
||||||
"Higher level" APIs (e.g. MessageBox, YesNobox, ...)
|
|
||||||
|
|
||||||
## Common Element Parameters
|
## Common Element Parameters
|
||||||
Some parameters that you will see on almost all Elements are:
|
Some parameters that you will see on almost all Elements are:
|
||||||
|
@ -1438,13 +1449,15 @@ Specifies the font family, size, and style. Font families on Windows include:
|
||||||
* Verdana
|
* Verdana
|
||||||
* Helvetica (the default I think)
|
* Helvetica (the default I think)
|
||||||
|
|
||||||
If you wish to leave the font family set to the default, you can put anything not matching one of the above names. The examples use the family 'Any'. You could use "default" if that's more clear.
|
The fonts will vary from system to system, however, Tk 8.0 automatically maps Courier, Helvetica and Times to their corresponding native family names on all platforms. Also, font families cannot cause a font specification to fail on Tk 8.0 and greater.
|
||||||
|
|
||||||
There are 2 formats that can be used... a string, and a tuple
|
If you wish to leave the font family set to the default, you can put anything not a font name as the family. The PySimpleGUI Demo programs and documentation use the family 'Any' to demonstrate this fact.. You could use "default" if that's more clear to you.
|
||||||
|
|
||||||
|
There are 2 formats that can be used to specify a font... a string, and a tuple
|
||||||
Tuple - (family, size, styles)
|
Tuple - (family, size, styles)
|
||||||
String - "Family Size Styles"
|
String - "Family Size Styles"
|
||||||
|
|
||||||
To specify an underlined, Helvetica font with a size of 15 the values would be
|
To specify an underlined, Helvetica font with a size of 15 the values:
|
||||||
('Helvetica', 15, 'underline italics')
|
('Helvetica', 15, 'underline italics')
|
||||||
'Helvetica 15 underline italics'
|
'Helvetica 15 underline italics'
|
||||||
|
|
||||||
|
@ -2204,10 +2217,13 @@ Here's an example window made with button images.
|
||||||
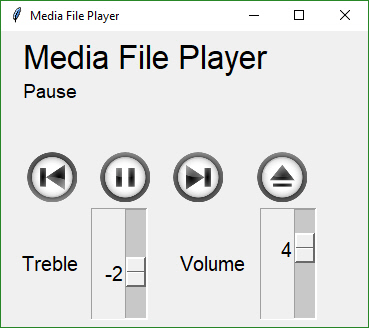
|
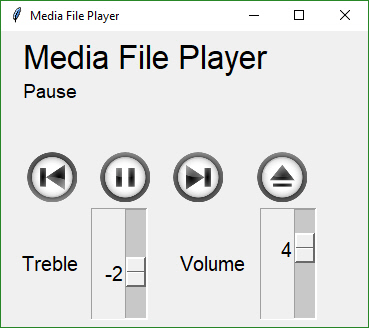
|
||||||
|
|
||||||
You'll find the source code in the file Demo Media Player. Here is what the button calls look like to create media player window
|
You'll find the source code in the file Demo Media Player. Here is what the button calls look like to create media player window
|
||||||
|
```python
|
||||||
sg.RButton('Pause', button_color=sg.TRANSPARENT_BUTTON,
|
sg.RButton('Pause', button_color=sg.TRANSPARENT_BUTTON,
|
||||||
image_filename=image_pause, image_size=(50, 50), image_subsample=2, border_width=0)
|
image_filename=image_pause,
|
||||||
|
image_size=(50, 50),
|
||||||
|
image_subsample=2,
|
||||||
|
border_width=0)
|
||||||
|
```
|
||||||
This is one you'll have to experiment with at this point. Not up for an exhaustive explanation.
|
This is one you'll have to experiment with at this point. Not up for an exhaustive explanation.
|
||||||
|
|
||||||
**Realtime Buttons**
|
**Realtime Buttons**
|
||||||
|
@ -2221,30 +2237,37 @@ This window has 2 button types. There's the normal "Simple Button" (Quit) and 4
|
||||||
|
|
||||||
Here is the code to make, show and get results from this window:
|
Here is the code to make, show and get results from this window:
|
||||||
|
|
||||||
window = sg.Window('Robotics Remote Control', auto_size_text=True)
|
```python
|
||||||
|
import PySimpleGUI as sg
|
||||||
|
|
||||||
window_rows = [[sg.Text('Robotics Remote Control')],
|
gui_rows = [[sg.Text('Robotics Remote Control')],
|
||||||
[sg.T(' '*10), sg.RealtimeButton('Forward')],
|
[sg.T(' ' * 10), sg.RealtimeButton('Forward')],
|
||||||
[ sg.RealtimeButton('Left'), sg.T(' '*15), sg.RealtimeButton('Right')],
|
[sg.RealtimeButton('Left'), sg.T(' ' * 15), sg.RealtimeButton('Right')],
|
||||||
[sg.T(' '*10), sg.RealtimeButton('Reverse')],
|
[sg.T(' ' * 10), sg.RealtimeButton('Reverse')],
|
||||||
[sg.T('')],
|
[sg.T('')],
|
||||||
[sg.Quit(button_color=('black', 'orange'))]
|
[sg.Quit(button_color=('black', 'orange'))]
|
||||||
]
|
]
|
||||||
|
|
||||||
window.Layout(window_rows, non_blocking=True).Read()
|
window = sg.Window('Robotics Remote Control', auto_size_text=True).Layout(gui_rows)
|
||||||
|
|
||||||
Somewhere later in your code will be your main event loop. This is where you do your polling of devices, do input/output, etc. It's here that you will read your window's buttons.
|
#
|
||||||
|
# Some place later in your code...
|
||||||
|
# You need to perform a ReadNonBlocking on your window every now and then or
|
||||||
|
# else it won't refresh.
|
||||||
|
#
|
||||||
|
# your program's main loop
|
||||||
|
while (True):
|
||||||
|
# This is the code that reads and updates your window
|
||||||
|
event, values = window.ReadNonBlocking()
|
||||||
|
if event is not None:
|
||||||
|
print(event)
|
||||||
|
if event == 'Quit' or values is None:
|
||||||
|
break
|
||||||
|
|
||||||
while (True):
|
window.CloseNonBlocking() # Don't forget to close your window!
|
||||||
# This is the code that reads and updates your window
|
```
|
||||||
event, values = window.ReadNonBlocking()
|
|
||||||
if button is not None:
|
|
||||||
sg.Print(event)
|
|
||||||
if event == 'Quit' or values is None:
|
|
||||||
break
|
|
||||||
time.sleep(.01)
|
|
||||||
|
|
||||||
This loop will read button values and print them. When one of the Realtime buttons is clicked, the call to `window.ReadNonBlocking` will return a button name matching the name on the button that was depressed. It will continue to return values as long as the button remains depressed. Once released, the ReadNonBlocking will return None for buttons until a button is again clicked.
|
This loop will read button values and print them. When one of the Realtime buttons is clicked, the call to `window.ReadNonBlocking` will return a button name matching the name on the button that was depressed or the key if there was a key assigned to the button. It will continue to return values as long as the button remains depressed. Once released, the ReadNonBlocking will return None for buttons until a button is again clicked.
|
||||||
|
|
||||||
**File Types**
|
**File Types**
|
||||||
The `FileBrowse` & `SaveAs` buttons have an additional setting named `file_types`. This variable is used to filter the files shown in the file dialog box. The default value for this setting is
|
The `FileBrowse` & `SaveAs` buttons have an additional setting named `file_types`. This variable is used to filter the files shown in the file dialog box. The default value for this setting is
|
||||||
|
@ -2844,6 +2867,9 @@ There are 2 ways to keep a window open after the user has clicked a button. One
|
||||||
|
|
||||||
The `RButton` Element creates a button that when clicked will return control to the user, but will leave the window open and visible. This button is also used in Non-Blocking windows. The difference is in which call is made to read the window. The `Read` call will block, the `ReadNonBlocking` will not block.
|
The `RButton` Element creates a button that when clicked will return control to the user, but will leave the window open and visible. This button is also used in Non-Blocking windows. The difference is in which call is made to read the window. The `Read` call will block, the `ReadNonBlocking` will not block.
|
||||||
|
|
||||||
|
Note that `InputText` and `MultiLine` Elements will be **cleared** when performing a `ReadNonBlocking`. If you do not want your input field to be cleared after a `ReadNonBlocking` then you can set the `do_not_clear` parameter to True when creating those elements. The clear is turned on and off on an element by element basis.
|
||||||
|
|
||||||
|
The reasoning behind this is that Persistent Windows are often "forms". When "submitting" a form you want to have all of the fields left blank so the next entry of data will start with a fresh window. Also, when implementing a "Chat Window" type of interface, after each read / send of the chat data, you want the input field cleared. Think of it as a Texting application. Would you want to have to clear your previous text if you want to send a second text?
|
||||||
|
|
||||||
|
|
||||||
## Asynchronous (Non-Blocking) windows
|
## Asynchronous (Non-Blocking) windows
|
||||||
|
|
88
readme.md
88
readme.md
|
@ -189,6 +189,8 @@ While simple to use, PySimpleGUI has significant depth to be explored by more ad
|
||||||
Menus with ALT-hotkey
|
Menus with ALT-hotkey
|
||||||
Tooltips
|
Tooltips
|
||||||
Clickable links
|
Clickable links
|
||||||
|
Transparent windows
|
||||||
|
Movable windows
|
||||||
No async programming required (no callbacks to worry about)
|
No async programming required (no callbacks to worry about)
|
||||||
|
|
||||||
|
|
||||||
|
@ -1316,6 +1318,9 @@ Read the Window's input values and button clicks but without blocking. It will
|
||||||
#### Refresh()
|
#### Refresh()
|
||||||
Cause changes to the window to be displayed on the screen. Normally not needed unless the changes are immediately required or if it's going to be a while before another call to Read.
|
Cause changes to the window to be displayed on the screen. Normally not needed unless the changes are immediately required or if it's going to be a while before another call to Read.
|
||||||
|
|
||||||
|
#### SetIcon(icon)
|
||||||
|
Sets the window's icon that will be shown on the titlebar.
|
||||||
|
|
||||||
#### Fill(values_dict)
|
#### Fill(values_dict)
|
||||||
Populates the windows fields with the values shown in the dictionary.
|
Populates the windows fields with the values shown in the dictionary.
|
||||||
|
|
||||||
|
@ -1335,6 +1340,12 @@ Fills in a window's fields based on previously saved file
|
||||||
|
|
||||||
Returns the size (w,h) of the screen in pixels
|
Returns the size (w,h) of the screen in pixels
|
||||||
|
|
||||||
|
#### Move(x, y)
|
||||||
|
Move window to (x,y) position on the screen
|
||||||
|
|
||||||
|
#### Minimize()
|
||||||
|
Sends the window to the taskbar
|
||||||
|
|
||||||
#### CloseNonBlocking()
|
#### CloseNonBlocking()
|
||||||
|
|
||||||
Closes a non-blocking window
|
Closes a non-blocking window
|
||||||
|
@ -1363,6 +1374,10 @@ Makes a window disappear while leaving the icon on the taskbar
|
||||||
|
|
||||||
Makes a window reappear that was previously made to disappear using Disappear()
|
Makes a window reappear that was previously made to disappear using Disappear()
|
||||||
|
|
||||||
|
#### SetAlpha(alpha)
|
||||||
|
|
||||||
|
Sets the window's transparency. 0 is completely transparent. 1 is fully visible, normal . Can also use the property Window.AlphaChannel instead of method function call
|
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
@ -1377,7 +1392,7 @@ Makes a window reappear that was previously made to disappear using Disappear()
|
||||||
Calendar picker
|
Calendar picker
|
||||||
Date Chooser
|
Date Chooser
|
||||||
Read window
|
Read window
|
||||||
Close window
|
Close window ("Button" & all shortcut buttons)
|
||||||
Realtime
|
Realtime
|
||||||
Checkboxes
|
Checkboxes
|
||||||
Radio Buttons
|
Radio Buttons
|
||||||
|
@ -1395,11 +1410,7 @@ Makes a window reappear that was previously made to disappear using Disappear()
|
||||||
Table
|
Table
|
||||||
Tree
|
Tree
|
||||||
Tab, TabGroup
|
Tab, TabGroup
|
||||||
Async/Non-Blocking Windows
|
|
||||||
Tabbed windows
|
|
||||||
Persistent Windows
|
|
||||||
Redirect Python Output/Errors to scrolling Window
|
|
||||||
"Higher level" APIs (e.g. MessageBox, YesNobox, ...)
|
|
||||||
|
|
||||||
## Common Element Parameters
|
## Common Element Parameters
|
||||||
Some parameters that you will see on almost all Elements are:
|
Some parameters that you will see on almost all Elements are:
|
||||||
|
@ -1438,13 +1449,15 @@ Specifies the font family, size, and style. Font families on Windows include:
|
||||||
* Verdana
|
* Verdana
|
||||||
* Helvetica (the default I think)
|
* Helvetica (the default I think)
|
||||||
|
|
||||||
If you wish to leave the font family set to the default, you can put anything not matching one of the above names. The examples use the family 'Any'. You could use "default" if that's more clear.
|
The fonts will vary from system to system, however, Tk 8.0 automatically maps Courier, Helvetica and Times to their corresponding native family names on all platforms. Also, font families cannot cause a font specification to fail on Tk 8.0 and greater.
|
||||||
|
|
||||||
There are 2 formats that can be used... a string, and a tuple
|
If you wish to leave the font family set to the default, you can put anything not a font name as the family. The PySimpleGUI Demo programs and documentation use the family 'Any' to demonstrate this fact.. You could use "default" if that's more clear to you.
|
||||||
|
|
||||||
|
There are 2 formats that can be used to specify a font... a string, and a tuple
|
||||||
Tuple - (family, size, styles)
|
Tuple - (family, size, styles)
|
||||||
String - "Family Size Styles"
|
String - "Family Size Styles"
|
||||||
|
|
||||||
To specify an underlined, Helvetica font with a size of 15 the values would be
|
To specify an underlined, Helvetica font with a size of 15 the values:
|
||||||
('Helvetica', 15, 'underline italics')
|
('Helvetica', 15, 'underline italics')
|
||||||
'Helvetica 15 underline italics'
|
'Helvetica 15 underline italics'
|
||||||
|
|
||||||
|
@ -2204,10 +2217,13 @@ Here's an example window made with button images.
|
||||||
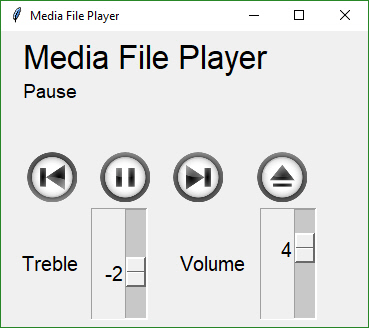
|
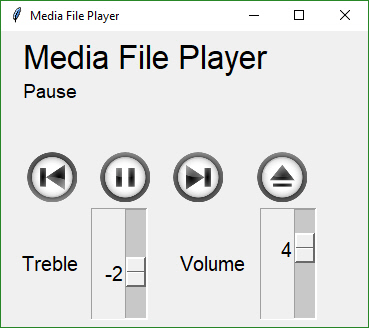
|
||||||
|
|
||||||
You'll find the source code in the file Demo Media Player. Here is what the button calls look like to create media player window
|
You'll find the source code in the file Demo Media Player. Here is what the button calls look like to create media player window
|
||||||
|
```python
|
||||||
sg.RButton('Pause', button_color=sg.TRANSPARENT_BUTTON,
|
sg.RButton('Pause', button_color=sg.TRANSPARENT_BUTTON,
|
||||||
image_filename=image_pause, image_size=(50, 50), image_subsample=2, border_width=0)
|
image_filename=image_pause,
|
||||||
|
image_size=(50, 50),
|
||||||
|
image_subsample=2,
|
||||||
|
border_width=0)
|
||||||
|
```
|
||||||
This is one you'll have to experiment with at this point. Not up for an exhaustive explanation.
|
This is one you'll have to experiment with at this point. Not up for an exhaustive explanation.
|
||||||
|
|
||||||
**Realtime Buttons**
|
**Realtime Buttons**
|
||||||
|
@ -2221,30 +2237,37 @@ This window has 2 button types. There's the normal "Simple Button" (Quit) and 4
|
||||||
|
|
||||||
Here is the code to make, show and get results from this window:
|
Here is the code to make, show and get results from this window:
|
||||||
|
|
||||||
window = sg.Window('Robotics Remote Control', auto_size_text=True)
|
```python
|
||||||
|
import PySimpleGUI as sg
|
||||||
|
|
||||||
window_rows = [[sg.Text('Robotics Remote Control')],
|
gui_rows = [[sg.Text('Robotics Remote Control')],
|
||||||
[sg.T(' '*10), sg.RealtimeButton('Forward')],
|
[sg.T(' ' * 10), sg.RealtimeButton('Forward')],
|
||||||
[ sg.RealtimeButton('Left'), sg.T(' '*15), sg.RealtimeButton('Right')],
|
[sg.RealtimeButton('Left'), sg.T(' ' * 15), sg.RealtimeButton('Right')],
|
||||||
[sg.T(' '*10), sg.RealtimeButton('Reverse')],
|
[sg.T(' ' * 10), sg.RealtimeButton('Reverse')],
|
||||||
[sg.T('')],
|
[sg.T('')],
|
||||||
[sg.Quit(button_color=('black', 'orange'))]
|
[sg.Quit(button_color=('black', 'orange'))]
|
||||||
]
|
]
|
||||||
|
|
||||||
window.Layout(window_rows, non_blocking=True).Read()
|
window = sg.Window('Robotics Remote Control', auto_size_text=True).Layout(gui_rows)
|
||||||
|
|
||||||
Somewhere later in your code will be your main event loop. This is where you do your polling of devices, do input/output, etc. It's here that you will read your window's buttons.
|
#
|
||||||
|
# Some place later in your code...
|
||||||
|
# You need to perform a ReadNonBlocking on your window every now and then or
|
||||||
|
# else it won't refresh.
|
||||||
|
#
|
||||||
|
# your program's main loop
|
||||||
|
while (True):
|
||||||
|
# This is the code that reads and updates your window
|
||||||
|
event, values = window.ReadNonBlocking()
|
||||||
|
if event is not None:
|
||||||
|
print(event)
|
||||||
|
if event == 'Quit' or values is None:
|
||||||
|
break
|
||||||
|
|
||||||
while (True):
|
window.CloseNonBlocking() # Don't forget to close your window!
|
||||||
# This is the code that reads and updates your window
|
```
|
||||||
event, values = window.ReadNonBlocking()
|
|
||||||
if button is not None:
|
|
||||||
sg.Print(event)
|
|
||||||
if event == 'Quit' or values is None:
|
|
||||||
break
|
|
||||||
time.sleep(.01)
|
|
||||||
|
|
||||||
This loop will read button values and print them. When one of the Realtime buttons is clicked, the call to `window.ReadNonBlocking` will return a button name matching the name on the button that was depressed. It will continue to return values as long as the button remains depressed. Once released, the ReadNonBlocking will return None for buttons until a button is again clicked.
|
This loop will read button values and print them. When one of the Realtime buttons is clicked, the call to `window.ReadNonBlocking` will return a button name matching the name on the button that was depressed or the key if there was a key assigned to the button. It will continue to return values as long as the button remains depressed. Once released, the ReadNonBlocking will return None for buttons until a button is again clicked.
|
||||||
|
|
||||||
**File Types**
|
**File Types**
|
||||||
The `FileBrowse` & `SaveAs` buttons have an additional setting named `file_types`. This variable is used to filter the files shown in the file dialog box. The default value for this setting is
|
The `FileBrowse` & `SaveAs` buttons have an additional setting named `file_types`. This variable is used to filter the files shown in the file dialog box. The default value for this setting is
|
||||||
|
@ -2844,6 +2867,9 @@ There are 2 ways to keep a window open after the user has clicked a button. One
|
||||||
|
|
||||||
The `RButton` Element creates a button that when clicked will return control to the user, but will leave the window open and visible. This button is also used in Non-Blocking windows. The difference is in which call is made to read the window. The `Read` call will block, the `ReadNonBlocking` will not block.
|
The `RButton` Element creates a button that when clicked will return control to the user, but will leave the window open and visible. This button is also used in Non-Blocking windows. The difference is in which call is made to read the window. The `Read` call will block, the `ReadNonBlocking` will not block.
|
||||||
|
|
||||||
|
Note that `InputText` and `MultiLine` Elements will be **cleared** when performing a `ReadNonBlocking`. If you do not want your input field to be cleared after a `ReadNonBlocking` then you can set the `do_not_clear` parameter to True when creating those elements. The clear is turned on and off on an element by element basis.
|
||||||
|
|
||||||
|
The reasoning behind this is that Persistent Windows are often "forms". When "submitting" a form you want to have all of the fields left blank so the next entry of data will start with a fresh window. Also, when implementing a "Chat Window" type of interface, after each read / send of the chat data, you want the input field cleared. Think of it as a Texting application. Would you want to have to clear your previous text if you want to send a second text?
|
||||||
|
|
||||||
|
|
||||||
## Asynchronous (Non-Blocking) windows
|
## Asynchronous (Non-Blocking) windows
|
||||||
|
|
Loading…
Reference in New Issue