Readme update
Big update
This commit is contained in:
parent
f35fa97dfe
commit
4aa9d6299c
1 changed files with 232 additions and 105 deletions
337
readme.md
337
readme.md
|
@ -1,130 +1,257 @@
|
|||
# PySimpleGUI
|
||||
|
||||
... is a simple GUI, but also powerfully customizable. It's simple from the programmer's view point. The idea is to make adding a GUI to a Python program be a simple and trivial task.
|
||||
|
||||
I was frustrated by having to deal with the dos prompt when I had a powerful Windows machine right in front of me. Why is it SO difficult to do even the simplest of input/output to a window in Python??
|
||||
|
||||
Python itself doesn't have a SIMPLE solution... nor did the *many* GUI packages I tried. Most tried to do TOO MUCH, making it impossible for users to get started quickly. Others were just plain broken, requiring multiple files or other packages.
|
||||
|
||||
The PySimpleGUI solution is focused on the developer. How can the desired result be achieved in as little and as simple code as possible? This was the mantra used to create PySimpleGUI.
|
||||
|
||||
You can add a GUI to your command line with a single line of code. With 3 or 4 lines of code you can add a fully custom designed GUI.
|
||||
|
||||
The customization power comes from the form/dialog box builder that enables users to experience all of the normal GUI widgets without having to write a lot of code.
|
||||
|
||||
Features include:
|
||||
Text
|
||||
Single Line Input
|
||||
Buttons including these types:
|
||||
File Browse
|
||||
Folder Browse
|
||||
Non-closing return
|
||||
Close form
|
||||
Checkboxes
|
||||
Radio Buttons
|
||||
Icons
|
||||
Multi-line Text Input
|
||||
Scrollable Output
|
||||
Progress Bar
|
||||
Async/Non-Blocking Windows
|
||||
Persistent Windows
|
||||
Redirect Python Output/Errors to scrolling Window
|
||||
'Higher level' APIs (e.g. MessageBox, YesNobox, ...)
|
||||
|
||||
|
||||
## Getting Started with PySimpleGUI
|
||||
|
||||
To use `import PySimpleGUI as SG`
|
||||
|
||||
For examples download
|
||||
|
||||
DisplayHash.py - Shows you how to use the most basic functionality
|
||||
ColorDemo.py - COLORS are a big part of the fun of a GUI, right?
|
||||
|
||||
HowDoI.py - More advanced 'Chat-style' windows that don't close with button clicks
|
||||
|
||||
|
||||
### Prerequisites
|
||||
|
||||
Python 3
|
||||
tkinter
|
||||
|
||||
Should run on all Python platforms that have tkinter running on them. Has been thoroughly tested on Windows. While not tested elsewhere, should work on Linux, Mac, Pi, etc.
|
||||
|
||||
### Installing
|
||||
|
||||
pip install PySimpleGUI
|
||||
or
|
||||
Simply download the file - PySimpleGUI.py and import it into your code
|
||||
|
||||
## Running the tests
|
||||
|
||||
|
||||
## Deployment
|
||||
|
||||
PySimpleGUI can be broken down into 2 types of API's:
|
||||
* High Level single call functions
|
||||
* Custom form functions
|
||||
|
||||
|
||||
**High Level API Calls**
|
||||
|
||||
The classic "input a value, print result" example.
|
||||
Often command line programs simply take some value as input on the command line, do something with it and then display the results. Moving from the command line to a GUI is very simple.
|
||||
This code prompts user to input a line of text and then displays that text in a messages box:
|
||||
|
||||
import PySimpleGUI_local as SG
|
||||
|
||||
rc = SG.GetTextBox('Title', 'Please input something')
|
||||
SG.MsgBox('Results', 'The value returned from GetTextBox', rc)
|
||||
# PySimpleGUI
|
||||
|
||||
This really is a simple GUI, but also powerfully customizable.
|
||||
|
||||
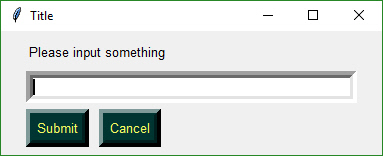
|
||||
|
||||
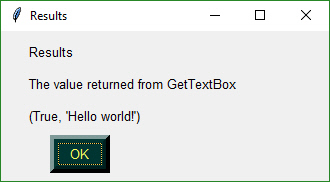
|
||||
I was frustrated by having to deal with the dos prompt when I had a powerful Windows machine right in front of me. Why is it SO difficult to do even the simplest of input/output to a window in Python??
|
||||
|
||||
Python itself doesn't have a simple solution... nor did the *many* GUI packages I tried. Most tried to do TOO MUCH, making it impossible for users to get started quickly. Others were just plain broken, requiring multiple files or other packages that were missing.
|
||||
|
||||
The PySimpleGUI solution is focused on the ***developer***. How can the desired result be achieved in as little and as simple code as possible? This was the mantra used to create PySimpleGUI.
|
||||
|
||||
You can add a GUI to your command line with a single line of code. With 3 or 4 lines of code you can add a fully customized GUI.
|
||||
|
||||
The customization power comes from the form/dialog box builder that enables users to experience all of the normal GUI widgets without having to write a lot of code.
|
||||
|
||||
Features of PySimpleGUI include:
|
||||
Text
|
||||
Single Line Input
|
||||
Buttons including these types:
|
||||
File Browse
|
||||
Folder Browse
|
||||
Non-closing return
|
||||
Close form
|
||||
Checkboxes
|
||||
Radio Buttons
|
||||
Icons
|
||||
Multi-line Text Input
|
||||
Scroll-able Output
|
||||
Progress Bar
|
||||
Async/Non-Blocking Windows
|
||||
Tabbed forms
|
||||
Persistent Windows
|
||||
Redirect Python Output/Errors to scrolling Window
|
||||
'Higher level' APIs (e.g. MessageBox, YesNobox, ...)
|
||||
|
||||
|
||||
## Getting Started with PySimpleGUI
|
||||
|
||||
### Installing
|
||||
|
||||
pip install PySimpleGUI
|
||||
or
|
||||
Simply download the file - PySimpleGUI.py and import it into your code
|
||||
|
||||
|
||||
### Prerequisites
|
||||
|
||||
Python 3
|
||||
tkinter
|
||||
|
||||
Should run on all Python platforms that have tkinter running on them. Has been thoroughly tested on Windows. While not tested elsewhere, should work on Linux, Mac, Pi, etc.
|
||||
|
||||
### Using
|
||||
|
||||
To us in your code, simply import....
|
||||
`import PySimpleGUI as SG`
|
||||
|
||||
Then use either "high level" API calls or build your own forms.
|
||||
|
||||
SG.MsgBox('This is my first message box')
|
||||
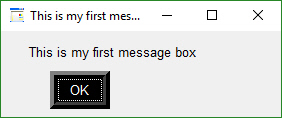
|
||||
|
||||
Yes, it's just that easy to have a window appear on the screen using Python.
|
||||
|
||||
## APIs
|
||||
|
||||
PySimpleGUI can be broken down into 2 types of API's:
|
||||
* High Level single call functions
|
||||
* Custom form functions
|
||||
|
||||
|
||||
### Python Language Features
|
||||
|
||||
There are a couple of Python language features that PySimpleGUI utilizes heavily that should be understood first...
|
||||
* Variable number of arguments to a function call
|
||||
* Optional parameters to a function call
|
||||
|
||||
#### Variable Number of Arguments
|
||||
|
||||
The "High Level" API calls that *output* values take a variable number of arguments so that they match a "print" statement as much as possible. The idea is to make it simple for the programmer to output as many items as desired and in any format. The user need not convert the variables to be output into the strings. The PySimpleGUI functions do that for the user.
|
||||
|
||||
SG.MsgBox('Variable number of parameters example', my_variable, second_variable, "etc")
|
||||
|
||||
Each new item begins on a new line in the Message Box
|
||||
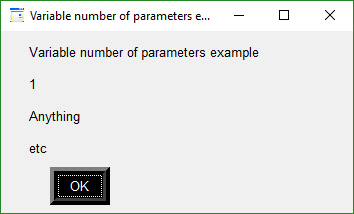
|
||||
|
||||
#### Optional Parameters to a Function Call
|
||||
|
||||
This feature of the Python language is utilized ***heavily*** as a method of customizing forms and part of forms. Rather than requiring the programmer to specify every possible option for a widget, instead only the options the caller wants to override are specified.
|
||||
|
||||
Here is the function definition for the MsgBox function. The details aren't important. What is important is seeing that there is a long list of potential tweaks that a caller can make. However, they don't have to be specified on each and every call.
|
||||
|
||||
def MsgBox(*args,
|
||||
ButtonColor=None,
|
||||
ButtonType=MSG_BOX_OK,
|
||||
AutoClose=False,
|
||||
AutoCloseDuration=None,
|
||||
Icon=DEFAULT_WINDOW_ICON,
|
||||
LineWidth=MESSAGE_BOX_LINE_WIDTH,
|
||||
Font=None):
|
||||
|
||||
If the caller wanted to change the button color to be black on yellow, the call would look something like this:
|
||||
|
||||
SG.MsgBox('This box has a custom button color',
|
||||
ButtonColor=('black', 'yellow'))
|
||||
|
||||
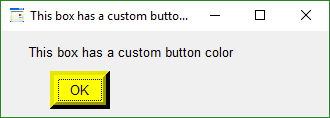
|
||||
|
||||
### High Level API Calls
|
||||
|
||||
The classic "input a value, print result" example.
|
||||
Often command line programs simply take some value as input on the command line, do something with it and then display the results. Moving from the command line to a GUI is very simple.
|
||||
This code prompts user to input a line of text and then displays that text in a messages box:
|
||||
|
||||
import PySimpleGUI_local as SG
|
||||
|
||||
rc = SG.GetTextBox('Title', 'Please input something')
|
||||
SG.MsgBox('Results', 'The value returned from GetTextBox', rc)
|
||||
|
||||
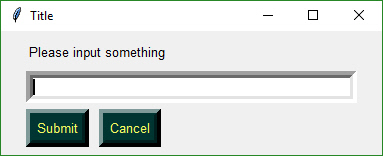
|
||||
|
||||
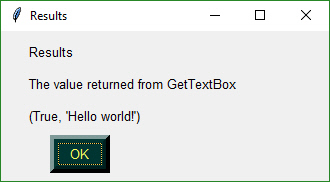
|
||||
|
||||
#### Message Boxes
|
||||
In addition to MsgBox, you'll find a several API calls that are shortcuts to common messages boxes. You can achieve similar results by calling MsgBox with the correct parameters.
|
||||
|
||||
The differences tend to be the number and types of buttons. Here are the calls and the windows that are created.
|
||||
|
||||
import PySimpleGUI as SG
|
||||
|
||||
`SG.MsgBoxOK('This is an OK MsgBox')`
|
||||
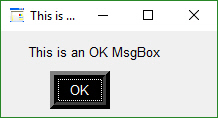
|
||||
|
||||
SG.MsgBoxOKCancel('This is an OK Cancel MsgBox')
|
||||
|
||||
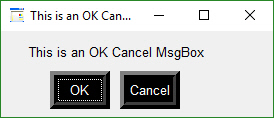
|
||||
|
||||
SG.MsgBoxCancel('This is a Cancel MsgBox')
|
||||
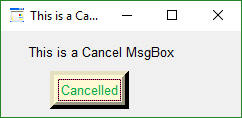
|
||||
|
||||
SG.MsgBoxYesNo('This is a Yes No MsgBox')
|
||||
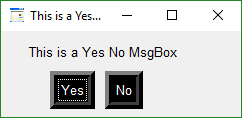
|
||||
|
||||
SG.MsgBoxError('This is an error MsgBox')
|
||||
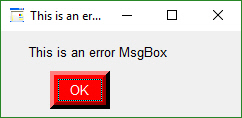
|
||||
|
||||
SG.MsgBoxAutoClose('This is an autoclose MsgBox')
|
||||
|
||||
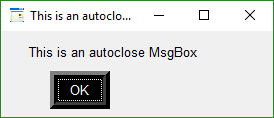
|
||||
|
||||
SG.ScrolledTextBox(my_text, Height=10)
|
||||
|
||||
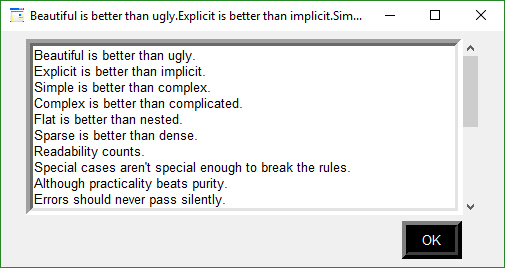
|
||||
|
||||
Take a moment to look at that last one. It's such a simple API call and yet the result is awesome. Rather than seeing text scrolling past on your display, you can capture that text and present it in a scrolled interface.
|
||||
|
||||
#### High Level User Input
|
||||
|
||||
There are 3 very basic user input high-level function calls. It's expected that for most applications, a custom input form will be created.
|
||||
- GetTextBox
|
||||
- GetFileBox
|
||||
- GetFolderBox
|
||||
|
||||
`submit_clicked, value = SG.GetTextBox('Title', 'Please enter anything')`
|
||||
|
||||
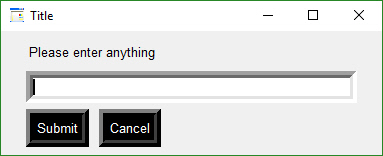
|
||||
|
||||
submit_clicked, value = SG.GetFileBox('Title', 'Choose a file')
|
||||
|
||||
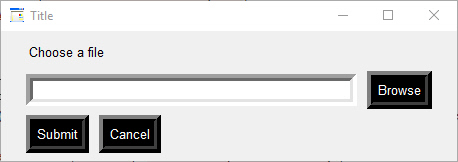
|
||||
|
||||
submit_clicked, value = SG.GetPathBox('Title', 'Choose a folder')
|
||||
|
||||
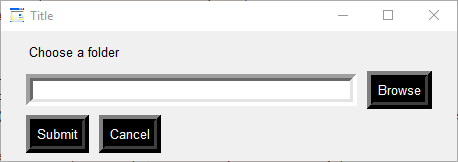
|
||||
|
||||
|
||||
**Custom Form API Calls**
|
||||
Here is a complete form - design, display, return information straight into caller's variables
|
||||
|
||||
# ------- Form design ------- #
|
||||
layout = [[Text('The SHA-1 Hash for the file')],
|
||||
[InputText(), FileBrowse()],
|
||||
[Submit(), Cancel()]]
|
||||
# ------- Form show ------- #
|
||||
(button, (source_filename,)) = FlexForm('Display A Hash in GooeyGUI', AutoSizeText=True).LayoutAndShow(layout)
|
||||
### Custom Form API Calls
|
||||
This is the FUN part of the programming of this GUI. In order to really get the most out of the API, you should be using an IDE that supports auto complete or will show you the definition of the function. This will make customizing go smoother.
|
||||
|
||||
Important initial concepts
|
||||
Return information from FlexForm, SG's primary form builder interface, is in this format:
|
||||
|
||||
(button, (value1, value2, ...))
|
||||
It's both not enjoyable nor helpful to immediately jump into tweaking each and every little thing available to you. Let's start with a basic Browse for a file and do something with it.
|
||||
|
||||
Don't forget all those ()'s of your values won't be coreectly assigned.
|
||||
# COPY THIS DESIGN PATTERN!
|
||||
|
||||
If you have a SINGLE value being returned, it is written this way:
|
||||
with SG.FlexForm('SHA-1 & 256 Hash', AutoSizeText=True) as form:
|
||||
form_rows = [[SG.Text('SHA-1 and SHA-256 Hashes for the file')],
|
||||
[SG.InputText(), SG.FileBrowse()],
|
||||
[SG.Submit(), SG.Cancel()]]
|
||||
(button, (source_filename, )) = form.LayoutAndShow(form_rows)
|
||||
|
||||
(button, (value1,))
|
||||
This context manager contains all of the code needed to specify, show and retrieve results for this form:
|
||||
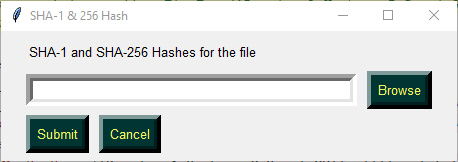
|
||||
|
||||
Forgetting the comma will mess you up but good
|
||||
It's important to use the "with" context manager. PySimpleGUI uses `tkinter`. `tkinter` is very picky about who releases objects and when. The `with` takes care of disposing of everything properly for you.
|
||||
|
||||
## Built With
|
||||
You will use this design pattern or code template for all of your "normal" (blocking) types of input forms.
|
||||
|
||||
PySimpleGUI's goal with the API is to be easy on the programmer. An attempt was made to make the program's code visually match the window on the screen. The way this is done is that a GUI is broken up into "Rows". Then each row is broke up into "Elements" or "Widgets". Each element is specified by names such as Text, Button, Checkbox, etc.
|
||||
|
||||
## Contributing
|
||||
Some elements are shortcuts, again meant to make it easy on the programmer. Rather than writing a `Button`, with name = "Submit", etc, the caller simply writes `Submit`.
|
||||
|
||||
A MikeTheWatchGuy production... entirely responsible for this code
|
||||
Going through each line of code
|
||||
|
||||
## Versioning
|
||||
with SG.FlexForm('SHA-1 & 256 Hash', AutoSizeText=True) as form:
|
||||
This creates a new form, storing it in the variable `form`.
|
||||
|
||||
1.0.9 - July 10, 2018 - Initial Release
|
||||
|
||||
form_rows = [[SG.Text('SHA-1 and SHA-256 Hashes for the file')],
|
||||
The next few rows of code lay out the rows of elements in the window to be displayed. The variable `form_rows` holds our entire GUI window. The first row of this form has a Text element. These simply display text on the form.
|
||||
|
||||
## Authors
|
||||
[SG.InputText(), SG.FileBrowse()],
|
||||
Now we're on the second row of the form. On this row there are 2 elements. The first is an `Input` field. It's a place the user can enter `strings`. The second element is a `File Browse Button`. A file or folder browse button will always fill in the text field to it's left unless otherwise specified. In this example, the File Browse Button will interact with the `InputText` field to its left.
|
||||
|
||||
[SG.Submit(), SG.Cancel()]]
|
||||
|
||||
## License
|
||||
The last line of the `form_rows` variable assignment contains a Submit and a Cancel Button. These are buttons that will cause a form to return its valueso the caller.
|
||||
|
||||
This project is licensed under the MIT License - see the [LICENSE.md](LICENSE.md) file for details
|
||||
(button, (source_filename, )) = form.LayoutAndShow(form_rows)
|
||||
This is the code that **displays** the form, collects the information and returns the data collected. In this example we have a button return code and only 1 input field.
|
||||
|
||||
## Acknowledgments
|
||||
# Return values
|
||||
|
||||
Return information from FlexForm, SG's primary form builder interface, is in this format:
|
||||
|
||||
(button, (value1, value2, ...))
|
||||
|
||||
Don't forget all those ()'s of your values won't be coreectly assigned.
|
||||
|
||||
If you have a SINGLE value being returned, it is written this way:
|
||||
|
||||
(button, (value1,))
|
||||
|
||||
Forgetting the comma will mess you up but good
|
||||
|
||||
## Built With
|
||||
|
||||
|
||||
## Contributing
|
||||
|
||||
A MikeTheWatchGuy production... entirely responsible for this code
|
||||
|
||||
## Versioning
|
||||
|
||||
1.0.9 - July 10, 2018 - Initial Release
|
||||
|
||||
|
||||
## Authors
|
||||
|
||||
|
||||
## License
|
||||
|
||||
This project is licensed under the MIT License - see the [LICENSE.md](LICENSE.md) file for details
|
||||
|
||||
## Acknowledgments
|
||||
|
||||
* Jorj McKie was the motivator behind the entire project. His wxsimpleGUI concepts sparked PySimpleGUI into existence
|
||||
|
|
Loading…
Add table
Add a link
Reference in a new issue