More 2.7 support
This commit is contained in:
parent
820862a4e7
commit
294d033ddf
4 changed files with 95 additions and 23 deletions
|
@ -6,6 +6,17 @@
|
|||
|
||||
You'll find that starting with a Recipe will give you a big jump-start on creating your custom GUI. Copy and paste one of these Recipes and modify it to match your requirements. Study them to get an idea of what design patterns to follow.
|
||||
|
||||
The Recipes in this Cookbook all assume you're running on a Python3 machine. If you are running Python 2.7 then your code will differ by 2 character. Replace the import statement:
|
||||
|
||||
import PySimpleGUI as sg
|
||||
|
||||
with
|
||||
|
||||
import PySimpleGUI27 as sg
|
||||
|
||||
There is a short section in the Readme with instruction on installing PySimpleGUI
|
||||
|
||||
|
||||
## Simple Data Entry - Return Values As List
|
||||
Same GUI screen except the return values are in a list instead of a dictionary and doesn't have initial values.
|
||||
|
||||
|
@ -302,7 +313,7 @@ This recipe shows just how easy it is to add a progress meter to your code.
|
|||
import PySimpleGUI as sg
|
||||
|
||||
for i in range(1000):
|
||||
sg.OneLineProgressMeter('One Line Meter Example', i+1, 1000, 'mymeter')
|
||||
sg.OneLineProgressMeter('One Line Meter Example', i+1, 1000, 'key')
|
||||
|
||||
|
||||
-----
|
||||
|
@ -530,9 +541,10 @@ you can write this line of code for the exact same result (OK, two lines with th
|
|||
|
||||
--------------------
|
||||
## Multiple Columns
|
||||
Starting in version 2.9 you can use the Column Element. A Column is required when you have a tall element to the left of smaller elements.
|
||||
|
||||
This example uses a Column. There is a Listbox on the left that is 3 rows high. To the right of it are 3 single rows of text and input. These 3 rows are in a Column Element.
|
||||
A Column is required when you have a tall element to the left of smaller elements.
|
||||
|
||||
In this example, there is a Listbox on the left that is 3 rows high. To the right of it are 3 single rows of text and input. These 3 rows are in a Column Element.
|
||||
|
||||
To make it easier to see the Column in the window, the Column background has been shaded blue. The code is wordier than normal due to the blue shading. Each element in the column needs to have the color set to match blue background.
|
||||
|
||||
|
@ -1222,6 +1234,37 @@ In this example we're defining our graph to be from -100, -100 to +100,+100. Th
|
|||
|
||||
button, values = window.Read()
|
||||
|
||||
## Tabs
|
||||
|
||||
Tabs bring not only an extra level of sophistication to your window layout, they give you extra room to add more elements. Tabs are one of the 3 container Elements, Elements that hold or contain other Elements. The other two are the Column and Frame Elements.
|
||||
|
||||
|
||||
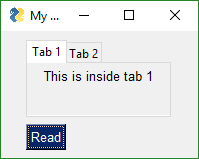
|
||||
|
||||
|
||||
|
||||
```
|
||||
import PySimpleGUI as sg
|
||||
|
||||
tab1_layout = [[sg.T('This is inside tab 1')]]
|
||||
|
||||
tab2_layout = [[sg.T('This is inside tab 2')],
|
||||
[sg.In(key='in')]]
|
||||
|
||||
layout = [[sg.TabGroup([[sg.Tab('Tab 1', tab1_layout, tooltip='tip'), sg.Tab('Tab 2', tab2_layout)]], tooltip='TIP2')],
|
||||
[sg.RButton('Read')]]
|
||||
|
||||
window = sg.Window('My window with tabs', default_element_size=(12,1)).Layout(layout)
|
||||
|
||||
while True:
|
||||
button, v = window.Read()
|
||||
print(button,values)
|
||||
if button is None: # always, always give a way out!
|
||||
break
|
||||
```
|
||||
|
||||
|
||||
|
||||
|
||||
## Creating a Windows .EXE File
|
||||
|
||||
|
|
|
@ -4,12 +4,21 @@
|
|||
|
||||
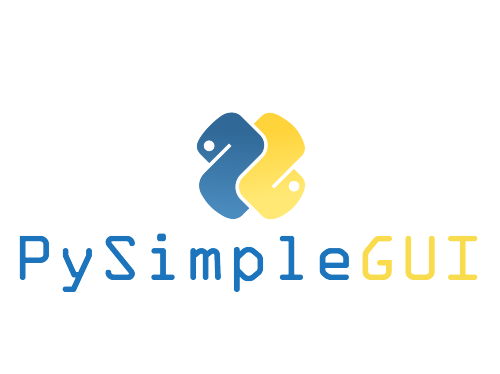
|
||||
|
||||
[](http://pepy.tech/project/pysimplegui)  [](https://www.python.org/downloads/)
|
||||
[](http://pepy.tech/project/pysimplegui) 
|
||||

|
||||
 
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
# PySimpleGUI
|
||||
|
||||

|
||||
|
||||
## Now supports both Python 2.7 & 3
|
||||
|
||||

|
||||
|
||||

|
||||
|
||||
|
@ -21,8 +30,6 @@
|
|||
|
||||
[Brief Tutorial](https://pysimplegui.readthedocs.io/en/latest/tutorial/)
|
||||
|
||||
[OpenSource Article](https://opensource.com/article/18/8/pysimplegui)
|
||||
|
||||
[Latest Demos and Master Branch on GitHub](https://github.com/MikeTheWatchGuy/PySimpleGUI)
|
||||
|
||||
Super-simple GUI to use... Powerfully customizable.
|
||||
|
@ -30,16 +37,17 @@ Super-simple GUI to use... Powerfully customizable.
|
|||
Home of the 1-line custom GUI and 1-line progress meter
|
||||
|
||||
#### Note regarding Python versions
|
||||
As of 9/25/2018 **both Python 3 and Python 2.7 are supported**! The Python 3 version is called PySimpleGUI. The Python 2.7 version is called PySimpleGUI27. They are installed separately and the imports are different. See instructions in Installation section for more info.
|
||||
As of 9/25/2018 **both Python 3 and Python 2.7 are supported**! The Python 3 version is named PySimpleGUI. The Python 2.7 version is named PySimpleGUI27. They are installed separately and the imports are different. See instructions in Installation section for more info.
|
||||
|
||||
|
||||
---------------------------------
|
||||
------------------------------------------------------------------------
|
||||
|
||||
Looking for a GUI package to help with
|
||||
|
||||
Looking for a GUI package?
|
||||
* Taking your Python code from the world of command lines and into the convenience of a GUI? *
|
||||
* Have a Raspberry **Pi** with a touchscreen that's going to waste because you don't have the time to learn a GUI SDK?
|
||||
* Into Machine Learning and are sick of the command line?
|
||||
* How about distributing your Python code to Windows users as a single .EXE file that launches straight into a GUI, much like a WinForms app?
|
||||
* Would like to distribute your Python code to Windows users as a single .EXE file that launches straight into a GUI, much like a WinForms app?
|
||||
|
||||
Look no further, **you've found your GUI package**.
|
||||
|
||||
|
|
|
@ -2,7 +2,7 @@
|
|||
|
||||
# Add GUIs to your programs and scripts easily with PySimpleGUI
|
||||
|
||||
NOTE -- PySimpleGUI requires Python3. If you're running Python2, don't waste your time reading.
|
||||
PySimpleGUI now supports BOTH Python 2.7 and Python 3
|
||||
|
||||
## Introduction
|
||||
Few people run Python programs by double clicking the .py file as if it were a .exe file. When a typical user (non-programmer types) double clicks an exe file, they expect it to pop open with a window they can interact with. While GUIs, using tkinter, are possible using standard Python installations, it's unlikely many programs do this.
|
||||
|
@ -46,7 +46,7 @@ There are more complex GUIs such as those that don't close after a button is cli
|
|||
When is PySimpleGUI useful? ***Immediately***, anytime you've got a GUI need. It will take under 5 minutes for you to create and try your GUI. With those kinds of times, what do you have to lose trying it?
|
||||
|
||||
The best way to go about making your GUI in under 5 minutes is to copy one of the GUIs from the [PySimpleGUI Cookbook](https://pysimplegui.readthedocs.io/en/latest/cookbook/). Follow these steps:
|
||||
* Install PySimpleGUI
|
||||
* Install PySimpleGUI (see short section in readme on installation)
|
||||
* Find a GUI that looks similar to what you want to create
|
||||
* Copy code from Cookbook
|
||||
* Paste into your IDE and run
|
||||
|
@ -78,6 +78,19 @@ It's a reasonably sized window.
|
|||
|
||||
If you only need to collect a few values and they're all basically strings, then you would copy this recipe and modify it to suit your needs.
|
||||
|
||||
### Python 2.7 Differences
|
||||
|
||||
The only noticeable difference between PySimpleGUI code running under Python 2.7 and one running on Python 3 is the import statement.
|
||||
|
||||
Python 3.x:
|
||||
|
||||
import PySimpleGUI as sg
|
||||
|
||||
Python 2.7:
|
||||
|
||||
import PySimpleGUI27 as sg
|
||||
|
||||
|
||||
## The 5-line GUI
|
||||
|
||||
Not all GUIs take 5 minutes. Some take 5 lines of code. This is a GUI with a custom layout contained in 5 lines of code.
|
||||
|
|
24
readme.md
24
readme.md
|
@ -4,12 +4,21 @@
|
|||
|
||||
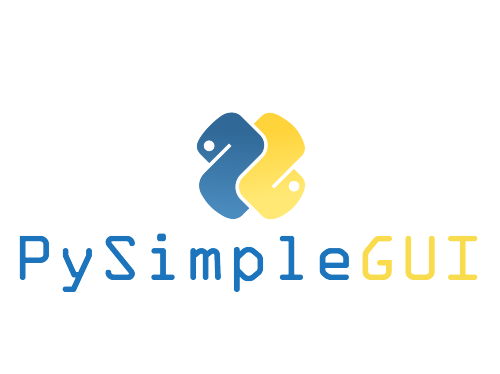
|
||||
|
||||
[](http://pepy.tech/project/pysimplegui)  [](https://www.python.org/downloads/)
|
||||
[](http://pepy.tech/project/pysimplegui) 
|
||||

|
||||
 
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
# PySimpleGUI
|
||||
|
||||

|
||||
|
||||
## Now supports both Python 2.7 & 3
|
||||
|
||||

|
||||
|
||||

|
||||
|
||||
|
@ -21,8 +30,6 @@
|
|||
|
||||
[Brief Tutorial](https://pysimplegui.readthedocs.io/en/latest/tutorial/)
|
||||
|
||||
[OpenSource Article](https://opensource.com/article/18/8/pysimplegui)
|
||||
|
||||
[Latest Demos and Master Branch on GitHub](https://github.com/MikeTheWatchGuy/PySimpleGUI)
|
||||
|
||||
Super-simple GUI to use... Powerfully customizable.
|
||||
|
@ -30,16 +37,17 @@ Super-simple GUI to use... Powerfully customizable.
|
|||
Home of the 1-line custom GUI and 1-line progress meter
|
||||
|
||||
#### Note regarding Python versions
|
||||
As of 9/25/2018 **both Python 3 and Python 2.7 are supported**! The Python 3 version is called PySimpleGUI. The Python 2.7 version is called PySimpleGUI27. They are installed separately and the imports are different. See instructions in Installation section for more info.
|
||||
As of 9/25/2018 **both Python 3 and Python 2.7 are supported**! The Python 3 version is named PySimpleGUI. The Python 2.7 version is named PySimpleGUI27. They are installed separately and the imports are different. See instructions in Installation section for more info.
|
||||
|
||||
|
||||
---------------------------------
|
||||
------------------------------------------------------------------------
|
||||
|
||||
Looking for a GUI package to help with
|
||||
|
||||
Looking for a GUI package?
|
||||
* Taking your Python code from the world of command lines and into the convenience of a GUI? *
|
||||
* Have a Raspberry **Pi** with a touchscreen that's going to waste because you don't have the time to learn a GUI SDK?
|
||||
* Into Machine Learning and are sick of the command line?
|
||||
* How about distributing your Python code to Windows users as a single .EXE file that launches straight into a GUI, much like a WinForms app?
|
||||
* Would like to distribute your Python code to Windows users as a single .EXE file that launches straight into a GUI, much like a WinForms app?
|
||||
|
||||
Look no further, **you've found your GUI package**.
|
||||
|
||||
|
|
Loading…
Add table
Add a link
Reference in a new issue