commit
1eb3c26878
136
PySimpleGUI.py
136
PySimpleGUI.py
|
@ -23,6 +23,18 @@ import pickle
|
|||
import calendar
|
||||
from random import randint
|
||||
|
||||
|
||||
|
||||
# 888888ba .d88888b oo dP .88888. dP dP dP
|
||||
# 88 `8b 88. "' 88 d8' `88 88 88 88
|
||||
# a88aaaa8P' dP dP `Y88888b. dP 88d8b.d8b. 88d888b. 88 .d8888b. 88 88 88 88
|
||||
# 88 88 88 `8b 88 88'`88'`88 88' `88 88 88ooood8 88 YP88 88 88 88
|
||||
# 88 88. .88 d8' .8P 88 88 88 88 88. .88 88 88. ... Y8. .88 Y8. .8P 88
|
||||
# dP `8888P88 Y88888P dP dP dP dP 88Y888P' dP `88888P' `88888' `Y88888P' dP
|
||||
# .88 88
|
||||
# d8888P dP
|
||||
|
||||
|
||||
g_time_start = 0
|
||||
g_time_end = 0
|
||||
g_time_delta = 0
|
||||
|
@ -1922,7 +1934,10 @@ class Image(Element):
|
|||
else:
|
||||
self.CurrentFrameNumber = self.CurrentFrameNumber + 1 if self.CurrentFrameNumber+1< self.TotalAnimatedFrames else 0
|
||||
image = self.AnimatedFrames[self.CurrentFrameNumber]
|
||||
self.tktext_label.configure(image=image, width=image.width(), heigh=image.height())
|
||||
try: # needed in case the window was closed with an "X"
|
||||
self.tktext_label.configure(image=image, width=image.width(), heigh=image.height())
|
||||
except:
|
||||
pass
|
||||
|
||||
|
||||
|
||||
|
@ -2032,7 +2047,12 @@ class Graph(Element):
|
|||
print('*** WARNING - The Graph element has not been finalized and cannot be drawn upon ***')
|
||||
print('Call Window.Finalize() prior to this operation')
|
||||
return None
|
||||
return self._TKCanvas2.create_line(converted_point_from, converted_point_to, width=width, fill=color)
|
||||
try: # in case window was closed with an X
|
||||
id = self._TKCanvas2.create_line(converted_point_from, converted_point_to, width=width, fill=color)
|
||||
except:
|
||||
id = None
|
||||
return id
|
||||
|
||||
|
||||
def DrawPoint(self, point, size=2, color='black'):
|
||||
if point == (None, None):
|
||||
|
@ -2042,9 +2062,14 @@ class Graph(Element):
|
|||
print('*** WARNING - The Graph element has not been finalized and cannot be drawn upon ***')
|
||||
print('Call Window.Finalize() prior to this operation')
|
||||
return None
|
||||
return self._TKCanvas2.create_oval(converted_point[0] - size, converted_point[1] - size,
|
||||
try: # needed in case window was closed with an X
|
||||
id = self._TKCanvas2.create_oval(converted_point[0] - size, converted_point[1] - size,
|
||||
converted_point[0] + size, converted_point[1] + size, fill=color,
|
||||
outline=color)
|
||||
except:
|
||||
id = None
|
||||
return
|
||||
|
||||
|
||||
def DrawCircle(self, center_location, radius, fill_color=None, line_color='black'):
|
||||
if center_location == (None, None):
|
||||
|
@ -2054,9 +2079,13 @@ class Graph(Element):
|
|||
print('*** WARNING - The Graph element has not been finalized and cannot be drawn upon ***')
|
||||
print('Call Window.Finalize() prior to this operation')
|
||||
return None
|
||||
return self._TKCanvas2.create_oval(converted_point[0] - radius, converted_point[1] - radius,
|
||||
try: # needed in case the window was closed with an X
|
||||
id = self._TKCanvas2.create_oval(converted_point[0] - radius, converted_point[1] - radius,
|
||||
converted_point[0] + radius, converted_point[1] + radius, fill=fill_color,
|
||||
outline=line_color)
|
||||
except:
|
||||
id = None
|
||||
return id
|
||||
|
||||
def DrawOval(self, top_left, bottom_right, fill_color=None, line_color=None):
|
||||
converted_top_left = self._convert_xy_to_canvas_xy(top_left[0], top_left[1])
|
||||
|
@ -2065,8 +2094,13 @@ class Graph(Element):
|
|||
print('*** WARNING - The Graph element has not been finalized and cannot be drawn upon ***')
|
||||
print('Call Window.Finalize() prior to this operation')
|
||||
return None
|
||||
return self._TKCanvas2.create_oval(converted_top_left[0], converted_top_left[1], converted_bottom_right[0],
|
||||
try: # in case windows close with X
|
||||
id = self._TKCanvas2.create_oval(converted_top_left[0], converted_top_left[1], converted_bottom_right[0],
|
||||
converted_bottom_right[1], fill=fill_color, outline=line_color)
|
||||
except:
|
||||
id = None
|
||||
|
||||
return id
|
||||
|
||||
def DrawArc(self, top_left, bottom_right, extent, start_angle, style=None, arc_color='black'):
|
||||
converted_top_left = self._convert_xy_to_canvas_xy(top_left[0], top_left[1])
|
||||
|
@ -2076,9 +2110,13 @@ class Graph(Element):
|
|||
print('*** WARNING - The Graph element has not been finalized and cannot be drawn upon ***')
|
||||
print('Call Window.Finalize() prior to this operation')
|
||||
return None
|
||||
return self._TKCanvas2.create_arc(converted_top_left[0], converted_top_left[1], converted_bottom_right[0],
|
||||
try: # in case closed with X
|
||||
id = self._TKCanvas2.create_arc(converted_top_left[0], converted_top_left[1], converted_bottom_right[0],
|
||||
converted_bottom_right[1], extent=extent, start=start_angle, style=tkstyle,
|
||||
outline=arc_color)
|
||||
except:
|
||||
id = None
|
||||
return id
|
||||
|
||||
def DrawRectangle(self, top_left, bottom_right, fill_color=None, line_color=None):
|
||||
converted_top_left = self._convert_xy_to_canvas_xy(top_left[0], top_left[1])
|
||||
|
@ -2087,8 +2125,13 @@ class Graph(Element):
|
|||
print('*** WARNING - The Graph element has not been finalized and cannot be drawn upon ***')
|
||||
print('Call Window.Finalize() prior to this operation')
|
||||
return None
|
||||
return self._TKCanvas2.create_rectangle(converted_top_left[0], converted_top_left[1], converted_bottom_right[0],
|
||||
try: # in case closed with X
|
||||
id = self._TKCanvas2.create_rectangle(converted_top_left[0], converted_top_left[1], converted_bottom_right[0],
|
||||
converted_bottom_right[1], fill=fill_color, outline=line_color)
|
||||
except:
|
||||
id = None
|
||||
return id
|
||||
|
||||
|
||||
def DrawText(self, text, location, color='black', font=None, angle=0):
|
||||
if location == (None, None):
|
||||
|
@ -2098,9 +2141,12 @@ class Graph(Element):
|
|||
print('*** WARNING - The Graph element has not been finalized and cannot be drawn upon ***')
|
||||
print('Call Window.Finalize() prior to this operation')
|
||||
return None
|
||||
text_id = self._TKCanvas2.create_text(converted_point[0], converted_point[1], text=text, font=font, fill=color,
|
||||
try: # in case closed with X
|
||||
id = self._TKCanvas2.create_text(converted_point[0], converted_point[1], text=text, font=font, fill=color,
|
||||
angle=angle)
|
||||
return text_id
|
||||
except:
|
||||
id = None
|
||||
return id
|
||||
|
||||
|
||||
def DrawImage(self, filename=None, data=None, location=(None, None), color='black', font=None, angle=0):
|
||||
|
@ -2120,8 +2166,11 @@ class Graph(Element):
|
|||
print('Call Window.Finalize() prior to this operation')
|
||||
return None
|
||||
self.Images.append(image)
|
||||
text_id = self._TKCanvas2.create_image(converted_point, image=image, anchor=tk.NW)
|
||||
return text_id
|
||||
try: # in case closed with X
|
||||
id = self._TKCanvas2.create_image(converted_point, image=image, anchor=tk.NW)
|
||||
except:
|
||||
id = None
|
||||
return id
|
||||
|
||||
|
||||
|
||||
|
@ -2130,7 +2179,10 @@ class Graph(Element):
|
|||
print('*** WARNING - The Graph element has not been finalized and cannot be drawn upon ***')
|
||||
print('Call Window.Finalize() prior to this operation')
|
||||
return None
|
||||
self._TKCanvas2.delete('all')
|
||||
try: # in case window was closed with X
|
||||
self._TKCanvas2.delete('all')
|
||||
except:
|
||||
pass
|
||||
|
||||
|
||||
def DeleteFigure(self, id):
|
||||
|
@ -4715,13 +4767,34 @@ else:
|
|||
i += 1
|
||||
|
||||
|
||||
# ------------------------------------------------------------------------------------------------------------------ #
|
||||
# ------------------------------------------------------------------------------------------------------------------ #
|
||||
# ===================================== TK CODE STARTS HERE ====================================================== #
|
||||
# ------------------------------------------------------------------------------------------------------------------ #
|
||||
# ------------------------------------------------------------------------------------------------------------------ #
|
||||
# 888 888 d8b 888
|
||||
# 888 888 Y8P 888
|
||||
# 888 888 888
|
||||
# 888888 888 888 888 88888b. 888888 .d88b. 888d888
|
||||
# 888 888 .88P 888 888 "88b 888 d8P Y8b 888P"
|
||||
# 888 888888K 888 888 888 888 88888888 888
|
||||
# Y88b. 888 "88b 888 888 888 Y88b. Y8b. 888
|
||||
# "Y888 888 888 888 888 888 "Y888 "Y8888 888
|
||||
|
||||
def PackFormIntoFrame(form, containing_frame, toplevel_form:Window):
|
||||
# My crappy tkinter code starts here
|
||||
|
||||
# ░░░░░░░░░░░█▀▀░░█░░░░░░
|
||||
# ░░░░░░▄▀▀▀▀░░░░░█▄▄░░░░
|
||||
# ░░░░░░█░█░░░░░░░░░░▐░░░
|
||||
# ░░░░░░▐▐░░░░░░░░░▄░▐░░░
|
||||
# ░░░░░░█░░░░░░░░▄▀▀░▐░░░
|
||||
# ░░░░▄▀░░░░░░░░▐░▄▄▀░░░░
|
||||
# ░░▄▀░░░▐░░░░░█▄▀░▐░░░░░
|
||||
# ░░█░░░▐░░░░░░░░▄░█░░░░░
|
||||
# ░░░█▄░░▀▄░░░░▄▀▐░█░░░░░
|
||||
# ░░░█▐▀▀▀░▀▀▀▀░░▐░█░░░░░
|
||||
# ░░▐█▐▄░░▀░░░░░░▐░█▄▄░░░
|
||||
# ░░░▀▀▄░░░░░░░░▄▐▄▄▄▀░░░
|
||||
# ░░░░░░░░░░░░░░░░░░░░░░░
|
||||
|
||||
# ======================== TK CODE STARTS HERE ========================================= #
|
||||
|
||||
def PackFormIntoFrame(form, containing_frame, toplevel_form):
|
||||
def CharWidthInPixels():
|
||||
return tkinter.font.Font().measure('A') # single character width
|
||||
|
||||
|
@ -5193,7 +5266,7 @@ def PackFormIntoFrame(form, containing_frame, toplevel_form:Window):
|
|||
listbox_frame = tk.Frame(tk_row_frame)
|
||||
element.TKStringVar = tk.StringVar()
|
||||
element.TKListbox = tk.Listbox(listbox_frame, height=element_size[1], width=width,
|
||||
selectmode=element.SelectMode, font=font)
|
||||
selectmode=element.SelectMode, font=font, exportselection=False)
|
||||
for index, item in enumerate(element.Values):
|
||||
element.TKListbox.insert(tk.END, item)
|
||||
if element.DefaultValues is not None and item in element.DefaultValues:
|
||||
|
@ -5898,7 +5971,7 @@ def PackFormIntoFrame(form, containing_frame, toplevel_form:Window):
|
|||
return
|
||||
|
||||
|
||||
def ConvertFlexToTK(MyFlexForm:Window):
|
||||
def ConvertFlexToTK(MyFlexForm):
|
||||
master = MyFlexForm.TKroot
|
||||
master.title(MyFlexForm.Title)
|
||||
InitializeResults(MyFlexForm)
|
||||
|
@ -6831,11 +6904,18 @@ def ObjToString(obj, extra=' '):
|
|||
for item in sorted(obj.__dict__)))
|
||||
|
||||
|
||||
######
|
||||
# # #### ##### # # ##### ####
|
||||
# # # # # # # # # # #
|
||||
###### # # # # # # # # ####
|
||||
# # # ##### # # ##### #
|
||||
# # # # # # # # #
|
||||
# #### # #### # ####
|
||||
|
||||
|
||||
# ------------------------------------------------------------------------------------------------------------------ #
|
||||
# ===================================== Upper PySimpleGUI ======================================================== #
|
||||
# Pre-built dialog boxes for all your needs These are the "high level API calls #
|
||||
# ------------------------------------------------------------------------------------------------------------------ #
|
||||
|
||||
# ----------------------------------- The mighty Popup! ------------------------------------------------------------ #
|
||||
|
||||
def Popup(*args, title=None, button_color=None, background_color=None, text_color=None, button_type=POPUP_BUTTONS_OK,
|
||||
|
@ -7462,7 +7542,17 @@ def PopupAnimated(image_source, message=None, background_color=None, text_color=
|
|||
|
||||
window.Refresh() # call refresh instead of Read to save significant CPU time
|
||||
|
||||
|
||||
"""
|
||||
d8b
|
||||
Y8P
|
||||
|
||||
88888b.d88b. 8888b. 888 88888b.
|
||||
888 "888 "88b "88b 888 888 "88b
|
||||
888 888 888 .d888888 888 888 888
|
||||
888 888 888 888 888 888 888 888
|
||||
888 888 888 "Y888888 888 888 888
|
||||
|
||||
"""
|
||||
|
||||
def main():
|
||||
from random import randint
|
||||
|
|
|
@ -8,8 +8,6 @@ import calendar
|
|||
import threading
|
||||
from collections import deque
|
||||
from queue import Queue
|
||||
# import remi.gui as gui
|
||||
# from remi import start, App
|
||||
import remi
|
||||
import logging
|
||||
import traceback
|
||||
|
|
|
@ -8,7 +8,7 @@
|
|||
|
||||

|
||||
|
||||

|
||||

|
||||
|
||||
|
||||
|
||||
|
@ -31,7 +31,7 @@ This Readme is for information ***specific to*** the Web port of PySimpleGUI.
|
|||
PySimpleGUIWeb enables you to run your PySimpleGUI programs in your web browser. It utilizes a package called Remi to achieve this amazing package.
|
||||
|
||||
|
||||
## Engineering Pre-Release Version 0.7.0
|
||||
## Engineering Pre-Release Version 0.9.0
|
||||
|
||||
[Announcements of Latest Developments](https://github.com/MikeTheWatchGuy/PySimpleGUI/issues/142)
|
||||
|
||||
|
@ -83,6 +83,9 @@ PySimpleGUIWeb runs only on Python 3. Legacy Python (2.7) is not supported.
|
|||
* Element padding
|
||||
* Read with timeout
|
||||
* Read with timeout = 0
|
||||
* Popup Windows
|
||||
* Multiple windows
|
||||
|
||||
|
||||
|
||||
# Running online using repl.it
|
||||
|
@ -179,6 +182,10 @@ New features
|
|||
* Popup support!
|
||||
* Support for multiple windows
|
||||
|
||||
## 0.9.0 PySimpleGUIWeb 14-Feb-2019
|
||||
|
||||
* Support for Window.Hide, Window.UnHide (better multi-window support)
|
||||
|
||||
|
||||
# Design
|
||||
# Author
|
||||
|
|
164
docs/index.md
164
docs/index.md
|
@ -17,7 +17,10 @@
|
|||

|
||||

|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
# PySimpleGUI
|
||||
|
||||
|
@ -30,15 +33,15 @@
|
|||
|
||||
|
||||
|
||||

|
||||

|
||||
|
||||

|
||||

|
||||
|
||||

|
||||

|
||||
|
||||

|
||||
|
||||

|
||||

|
||||
|
||||
[Announcements of Latest Developments](https://github.com/MikeTheWatchGuy/PySimpleGUI/issues/142)
|
||||
|
||||
|
@ -67,6 +70,7 @@ As of 9/25/2018 **both Python 3 and Python 2.7 are supported**! The Python 3 v
|
|||
|
||||
# Qt Version
|
||||
|
||||
### Github
|
||||
|
||||
Check out the new PySimpleGUI port to the Qt GUI Framework. You can learn more on the [PySimpleGUIQt GitHub site](https://github.com/MikeTheWatchGuy/PySimpleGUI/tree/master/PySimpleGUIQt). **There is a separate Readme file for the Qt version** that you'll find there.
|
||||
|
||||
|
@ -114,11 +118,12 @@ Educators in particular should be interested. Students can not only post their
|
|||
|
||||
Depending on how you're viewing this document, you may or may not see an embedded browser window below that is running PySimpleGUI code.
|
||||
|
||||
<iframe height="1000px" width="100%" src="https://repl.it/@PySimpleGUI/PySimpleGUIWeb-Demos?lite=true" scrolling="no" frameborder="no" allowtransparency="true" allowfullscreen="true" sandbox="allow-forms allow-pointer-lock allow-popups allow-same-origin allow-scripts allow-modals"></iframe>
|
||||
<iframe height="10400px" width="100%" src="https://repl.it/@PySimpleGUI/PySimpleGUIWeb-Demos?lite=true" scrolling="no" frameborder="no" allowtransparency="true" allowfullscreen="true" sandbox="allow-forms allow-pointer-lock allow-popups allow-same-origin allow-scripts allow-modals"></iframe>
|
||||
|
||||
# Support
|
||||
|
||||
PySimpleGUI is an active project. Bugs are fixed, features are added, often. Should you run into trouble, open an issue on the GitHub site and you'll receive help by someone in the community.
|
||||
PySimpleGUI is an active project. Bugs are fixed, features are added, often. Should you run into trouble, open an issue on the GitHub site and you'll receive help by someone in the community. It's very early in the port however with MANY more Elements left to implement. 3 down, 20-something to go.
|
||||
|
||||
|
||||
# Platforms
|
||||
|
||||
|
@ -191,7 +196,9 @@ In addition to a primary GUI, you can add a Progress Meter to your code with ONE
|
|||
|
||||
It's simple to show animated GIFs.
|
||||
|
||||
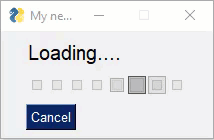
|
||||

|
||||
|
||||
|
||||
How about embedding a game inside of a GUI? This game of Pong is written in tkinter and then dropped into the PySimpleGUI window creating a game that has an accompanying GUI.
|
||||
|
@ -462,6 +469,12 @@ PySimpleGUI Runs on all Python3 platforms that have tkinter running on them. It
|
|||
If you wish to create an EXE from your PySimpleGUI application, you will need to install `PyInstaller`. There are instructions on how to create an EXE at the bottom of this ReadMe
|
||||
|
||||
|
||||
## Qt Version
|
||||
|
||||
Please see the Qt specific documentation. The readme that contains all you need to know about Qt specific matters is here:
|
||||
https://pysimplegui.readthedocs.io/readmeqt/
|
||||
|
||||
|
||||
## Using - Python 3
|
||||
|
||||
To use in your code, simply import....
|
||||
|
@ -809,7 +822,9 @@ time_between_frames - amount of time in milliseconds to use between frames
|
|||
|
||||
***To close animated popups***, call PopupAnimated with `image_source=None`. This will close all of the currently open PopupAnimated windows.
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
# Progress Meters!
|
||||
We all have loops in our code. 'Isn't it joyful waiting, watching a counter scrolling past in a text window? How about one line of code to get a progress meter, that contains statistics about your code?
|
||||
|
||||
|
@ -1622,7 +1637,7 @@ Populates the windows fields with the values shown in the dictionary.
|
|||
#### Element(key, silent_on_error=False) (shorthand version)
|
||||
#### FindElement(key, silent_on_error=False)
|
||||
|
||||
Returns the Element that has a matching key. If the key is not found, an Error Element is returned so that the program will not crash should the user try to perform an "update". A Popup message will be shown
|
||||
Rerturns the Element that has a matching key. If the key is not found, an Error Element is returned so that the program will not crash should the user try to perform an "update". A Popup message will be shown
|
||||
|
||||
#### FindElementWithFocus()
|
||||
|
||||
|
@ -2408,9 +2423,9 @@ Parameter definitions
|
|||
visible - if False will create image as hidden
|
||||
size_px - size of image in pixels
|
||||
|
||||
### `Update` Method
|
||||
### `Update`Image Methods
|
||||
|
||||
Like other Elements, the Image Element has an `Update` method. Call Update if you want to change the image.
|
||||
Like other Elements, the Image Element has an `Uupdate` method. Call Update if you want to change the image.
|
||||
|
||||
def Update(self, filename=None, data=None, visible=None):
|
||||
|
||||
|
@ -2435,7 +2450,7 @@ UpdateAnimation(source,
|
|||
You can call the method without setting the `time_between_frames` value and it will show a frame and immediately move on to the next frame. This enables you to do the inter-frame timing.
|
||||
|
||||
|
||||
|
||||
|
||||
|
||||
## Button Element
|
||||
|
||||
|
@ -3285,7 +3300,7 @@ Unlike Tables there is no standard format for trees. Thus the data structure pa
|
|||
def TreeData()
|
||||
def Insert(self, parent, key, text, values, icon=None)
|
||||
```
|
||||
|
||||
|
||||
To "insert" data into the tree the TreeData method Insert is called.
|
||||
|
||||
`Insert(parent_key, key, display_text, values)`
|
||||
|
@ -4271,6 +4286,55 @@ There are too many to list!!
|
|||
|
||||
There are over 130 sample programs to give you a jump start.
|
||||
|
||||
Use the example programs as a starting basis for your GUI. Copy, paste, modify and run! The demo files are:
|
||||
|
||||
| Source File| Description |
|
||||
|--|--|
|
||||
|**Demo_All_Widgets.py**| Nearly all of the Elements shown in a single window
|
||||
|**Demo_Borderless_Window.py**| Create clean looking windows with no border
|
||||
|**Demo_Button_States.py**| One way of implementing disabling of buttons
|
||||
|**Demo_Calendar.py** | Demo of the Calendar Chooser button
|
||||
|**Demo_Canvas.py** | window with a Canvas Element that is updated outside of the window
|
||||
|**Demo_Chat.py** | A chat window with scrollable history
|
||||
|**Demo_Chatterbot.py** | Front-end to Chatterbot Machine Learning project
|
||||
|**Demo_Color.py** | How to interact with color using RGB hex values and named colors
|
||||
|**Demo_Columns.py** | Using the Column Element to create more complex windows
|
||||
|**Demo_Compare_Files.py** | Using a simple GUI front-end to create a compare 2-files utility
|
||||
|**Demo_Cookbook_Browser.py** | Source code browser for all Recipes in Cookbook
|
||||
|**Demo_Dictionary.py** | Specifying and using return values in dictionary format
|
||||
**Demo_DOC_Viewer_PIL.py** | Display a PDF, HTML, ebook file, etc in your window
|
||||
|**Demo_DisplayHash1and256.py** | Using high level API and custom window to implement a simple display hash code utility
|
||||
|**Demo_DuplicateFileFinder.py** | High level API used to get a folder that is used by utility that finds duplicate files. Uses progress meter to show progress. 2 lines of code required to add GUI and meter
|
||||
|**Demo_Fill_Form.py** | How to perform a bulk-fill for a window. Saving and loading a window from disk
|
||||
|**Demo Font Sizer.py** | Demonstrates Elements updating other Elements
|
||||
|**Demo_Func_Callback_Simulator.py** | For the Raspberry Pi crowd. Event loop that simulates traditional GUI callback functions should you already have an architecture that uses them
|
||||
|**Demo_GoodColors.py** | Using some of the pre-defined PySimpleGUI individual colors
|
||||
|**Demo_HowDoI.py** | This is a utility to be experienced! It will change how you code
|
||||
|**Demo_Img_Viewer.py** | Display jpg, png,tiff, bmp files
|
||||
|**Demo_Keyboard.py** | Using blocking keyboard events
|
||||
|**Demo_Keyboard_Realtime.py** | Using non-blocking / realtime keyboard events
|
||||
|**Demo_Machine_Learning.py** | A sample Machine Learning front end
|
||||
|**Demo_Matplotlib.py** | Integrating with Matplotlib to create a single graph
|
||||
|**Demo_Matplotlib_Animated.py** | Animated Matplotlib line graph
|
||||
|**Demo_Matplotlib_Animated_Scatter.py** | Animated Matplotlib scatter graph
|
||||
|**Demo_Matplotlib_Browser.py** | Browse Matplotlib gallery
|
||||
|**Demo_Media_Player.py** | Non-blocking window with a media player layout. Demonstrates button graphics, Update method
|
||||
|**Demo_MIDI_Player.py** | GUI wrapper for Mido MIDI package. Functional MIDI player that controls attached MIDI devices
|
||||
|**Demo_NonBlocking_Form.py** | a basic async window
|
||||
|**Demo_OpenCV.py** | Integrated with OpenCV
|
||||
|**Demo_Password_Login** | Password protection using SHA1
|
||||
|**Demo_PDF_Viewer.py** | Submitted by a user! Previews PDF documents. Uses keyboard input & mouse scrollwheel to navigate
|
||||
|**Demo_Pi_LEDs.py** | Control GPIO using buttons
|
||||
|**Demo_Pi_Robotics.py** | Simulated robot control using realtime buttons
|
||||
|**Demo_PNG_Vierwer.py** | Uses Image Element to display PNG files
|
||||
| **Demo_Progress_Meters.py** | Demonstrates using 2 progress meters simultaneously
|
||||
|**Demo_Recipes.py** | A collection of various Recipes. Note these are not the same as the Recipes in the Recipe Cookbook
|
||||
|**Demo_Script_Launcher.py** | Demonstrates one way of adding a front-end onto several command line scripts
|
||||
|**Demo_Script_Parameters.py** | Add a 1-line GUI to the front of your previously command-line only scripts
|
||||
|**Demo_Tabbed_Form.py** | Using the Tab feature
|
||||
|**Demo_Table_Simulation.py** | Use input fields to display and edit tables
|
||||
|**Demo_Timer.py** | Simple non-blocking window
|
||||
|
||||
## Packages Used In Demos
|
||||
|
||||
|
||||
|
@ -4476,7 +4540,7 @@ Listboxes are still without scrollwheels. The mouse can drag to see more items.
|
|||
|
||||
3.0 We've come a long way baby! Time for a major revision bump. One reason is that the numbers started to confuse people the latest release was 2.30, but some people read it as 2.3 and thought it went backwards. I kinda messed up the 2.x series of numbers, so why not start with a clean slate. A lot has happened anyway so it's well earned.
|
||||
|
||||
One change that will set PySimpleGUI apart is the parlor trick of being able to move the window by clicking on it anywhere. This is turned on by default. It's not a common way to interact with windows. Normally you have to move using the titlebar. Not so with PySimpleGUI. Now you can drag using any part of the window. You will want to turn off for windows with sliders. This feature is enabled in the Window call.
|
||||
One change that will set PySimpleGUI apart is the parlor trick of being able to move the window by clicking on it anywhere. This is turned on by default. It's not a common way to interact with windows. Normally you have to move using the titlebar. Not so with PySimpleGUI. Now you can drag using any part of the window. You will want to turn this off for windows with sliders. This feature is enabled in the Window call.
|
||||
|
||||
Related to the Grab Anywhere feature is the no_titlebar option, again found in the call to Window. Your window will be a spiffy, borderless window. It's a really interesting effect. Slight problem is that you do not have an icon on the taskbar with these types of windows, so if you don't supply a button to close the window, there's no way to close it other than task manager.
|
||||
|
||||
|
@ -4826,7 +4890,7 @@ Emergency patch release... going out same day as previous release
|
|||
## 3.20.0 & 1.20.0 18-Dec-2018
|
||||
|
||||
* New Pane Element
|
||||
* Graph.DeleteFigure method
|
||||
* Graphh.DeleteFigure method
|
||||
* disable_minimize - New parameter for Window
|
||||
* Fix for 2.7 menus
|
||||
* Debug Window no longer re-routes stdout by default
|
||||
|
@ -4932,67 +4996,11 @@ You can also look up elements using their keys. This is an excellent way to upd
|
|||
This is a language feature that is featured **heavily** in all of the API calls, both functions and classes. Elements are configured, in-place, by setting one or more optional parameters. For example, a Text element's color is chosen by setting the optional `text_color` parameter.
|
||||
|
||||
**tkinter**
|
||||
tkinter is the "official" GUI that Python supports. It runs on Windows, Linux, and Mac. It was chosen as the first target GUI framework due to its ***ubiquity***. Nearly all Python installations, with the exception of Ubuntu Linux, come pre-loaded with tkinter. It is the "simplest" of the GUI frameworks to get up an running (among Qt, WxPython, Kivy, etc).
|
||||
|
||||
From the start of the PSG project, tkinter was not meant to be the only underlying GUI framework for PySimpleGUI. It is merely a starting point. All journeys begin with one step forward and choosing tkinter was the first of many steps for PySimpleGUI.
|
||||
|
||||
|
||||
|
||||
## Author
|
||||
MikeB
|
||||
|
||||
## Demo Code Contributors
|
||||
|
||||
[JorjMcKie](https://github.com/JorjMcKie) - PDF and image viewers (plus a number of code suggestions)
|
||||
[Otherion](https://github.com/Otherion) - Table Demos Panda & CSV. Loads of suggestions to the core APIs
|
||||
|
||||
## License
|
||||
|
||||
GNU Lesser General Public License (LGPL 3) +
|
||||
|
||||
## Acknowledgments
|
||||
|
||||
* [JorjMcKie](https://github.com/JorjMcKie) was the motivator behind the entire project. His wxsimpleGUI concepts sparked PySimpleGUI into existence
|
||||
* [Fredrik Lundh](https://wiki.python.org/moin/FredrikLundh) for his work on `tkinter`
|
||||
* [Ruud van der Ham](https://forum.pythonistacafe.com/u/Ruud) for all the help he's provided as a Python-mentor. Quite a few tricky bits of logic was supplied by Ruud. The dual-purpose return values scheme is Ruud's for example
|
||||
* **Numerous** users who provided feature suggestions! Many of the cool features were suggested by others. If you were one of them and are willing to take more credit, I'll list you here if you give me permission. Most are too modest
|
||||
* [moshekaplan](https://github.com/moshekaplan)/**[tkinter_components](https://github.com/moshekaplan/tkinter_components)** wrote the code for the Calendar Chooser Element. It was lifted straight from GitHub
|
||||
* [Bryan Oakley](https://stackoverflow.com/users/7432/bryan-oakley) for the code that enables the `grab_anywhere` feature.
|
||||
* [Otherion](https://github.com/Otherion) for help with Tables, being a sounding board for new features, naming functions, ..., all around great help
|
||||
* [agjunyent](https://github.com/agjunyent) figured out how to properly make tabs and wrote prototype code that demonstrated how to do it
|
||||
* [jfongattw](https://github.com/jfongattw) huge suggestion... dictionaries. turned out to be
|
||||
* one of the most critical constructs in PySimpleGUI
|
||||
* [venim](https://github.com/venim) code to doing Alt-Selections in menus, updating Combobox using index, request to disable windows (a really good idea), checkbox and tab submits on change, returning keys for elements that have change_submits set, ...
|
||||
* [rtrrtr](https://github.com/rtrrtr) Helped get the 2.7 and 3.x code unified (big damned deal)
|
||||
* Tony Crewe (anthony.crewe@gmail.com) Generously provided his classroom materials that he has written to teach a GUI course. If you're an educator and want to trade materials with Tony, he would like to hear from you.
|
||||
* [spectre6000](https://github.com/spectre6000) - Readme updates
|
||||
* [jackyOO7](https://github.com/jackyOO7) - Demo programs. OpenCV with realtime image processing, popup keyboard, input Combo read only option.
|
||||
* [AltoRetrato](https://github.com/AltoRetrato) - Fonts for multiline and combo
|
||||
* [frakman1](https://github.com/frakman1) - Sample code error
|
||||
* [deajan](https://github.com/deajan) - Custom button text for Popups
|
||||
* [GRADESK](https://github.com/sidbmw/ICS4U) - Created by a couple of young talented programmers, this classroom management software combines SQL and a GUI to provide a much improved interface for Ottawa teachers.
|
||||
|
||||
|
||||
## How Do I
|
||||
Finally, I must thank the fine folks at How Do I.
|
||||
https://github.com/gleitz/howdoi
|
||||
Their utility has forever changed the way and pace in which I can program. I urge you to try the HowDoI.py application here on GitHub. Trust me, **it's going to be worth the effort!**
|
||||
Here are the steps to run that application
|
||||
|
||||
Install howdoi:
|
||||
pip install howdoi
|
||||
Test your install:
|
||||
python -m howdoi howdoi.py
|
||||
To run it:
|
||||
Python HowDoI.py
|
||||
|
||||
The pip command is all there is to the setup.
|
||||
|
||||
The way HowDoI works is that it uses your search term to look through stack overflow posts. It finds the best answer, gets the code from the answer, and presents it as a response. It gives you the cor
|
||||
tkinter is the "official" GUI that Python supports. It runs on W
|
||||
<!--stackedit_data:
|
||||
eyJoaXN0b3J5IjpbMjYwNTg0ODE0LDExMDIwODgzMzMsMTY3OT
|
||||
g1MDk5MiwtMTQ2MTQyODEsLTYwNjM3MTE4LC01MDkzNTkxMjMs
|
||||
LTI0ODk3NjI5LDEzMDc2OTI1OSwtMjk2NzgzNTUsLTc3NDA3ND
|
||||
IzMCwyNjYzNjQ0MTcsNDQ5NDMzMjQzLC0xMTQ4NDkwNjIzXX0=
|
||||
|
||||
eyJoaXN0b3J5IjpbLTE2MDYwMzE5MzQsMjYwNTg0ODE0LDExMD
|
||||
IwODgzMzMsMTY3OTg1MDk5MiwtMTQ2MTQyODEsLTYwNjM3MTE4
|
||||
LC01MDkzNTkxMjMsLTI0ODk3NjI5LDEzMDc2OTI1OSwtMjk2Nz
|
||||
gzNTUsLTc3NDA3NDIzMCwyNjYzNjQ0MTcsNDQ5NDMzMjQzLC0x
|
||||
MTQ4NDkwNjIzXX0=
|
||||
-->
|
27
readme.md
27
readme.md
|
@ -4972,27 +4972,10 @@ GNU Lesser General Public License (LGPL 3) +
|
|||
* [deajan](https://github.com/deajan) - Custom button text for Popups
|
||||
* [GRADESK](https://github.com/sidbmw/ICS4U) - Created by a couple of young talented programmers, this classroom management software combines SQL and a GUI to provide a much improved interface for Ottawa teachers.
|
||||
|
||||
|
||||
## How Do I
|
||||
Finally, I must thank the fine folks at How Do I.
|
||||
https://github.com/gleitz/howdoi
|
||||
Their utility has forever changed the way and pace in which I can program. I urge you to try the HowDoI.py application here on GitHub. Trust me, **it's going to be worth the effort!**
|
||||
Here are the steps to run that application
|
||||
|
||||
Install howdoi:
|
||||
pip install howdoi
|
||||
Test your install:
|
||||
python -m howdoi howdoi.py
|
||||
To run it:
|
||||
Python HowDoI.py
|
||||
|
||||
The pip command is all there is to the setup.
|
||||
|
||||
The way HowDoI works is that it uses your search term to look through stack overflow posts. It finds the best answer, gets the code from the answer, and presents it as a response. It gives you the cor
|
||||
<!--stackedit_data:
|
||||
eyJoaXN0b3J5IjpbMjYwNTg0ODE0LDExMDIwODgzMzMsMTY3OT
|
||||
g1MDk5MiwtMTQ2MTQyODEsLTYwNjM3MTE4LC01MDkzNTkxMjMs
|
||||
LTI0ODk3NjI5LDEzMDc2OTI1OSwtMjk2NzgzNTUsLTc3NDA3ND
|
||||
IzMCwyNjYzNjQ0MTcsNDQ5NDMzMjQzLC0xMTQ4NDkwNjIzXX0=
|
||||
|
||||
eyJoaXN0b3J5IjpbLTE1Nzg3NDY1ODgsMjYwNTg0ODE0LDExMD
|
||||
IwODgzMzMsMTY3OTg1MDk5MiwtMTQ2MTQyODEsLTYwNjM3MTE4
|
||||
LC01MDkzNTkxMjMsLTI0ODk3NjI5LDEzMDc2OTI1OSwtMjk2Nz
|
||||
gzNTUsLTc3NDA3NDIzMCwyNjYzNjQ0MTcsNDQ5NDMzMjQzLC0x
|
||||
MTQ4NDkwNjIzXX0=
|
||||
-->
|
Loading…
Reference in New Issue